Unveiling the Mastery of Await in JavaScript: A Deep Dive
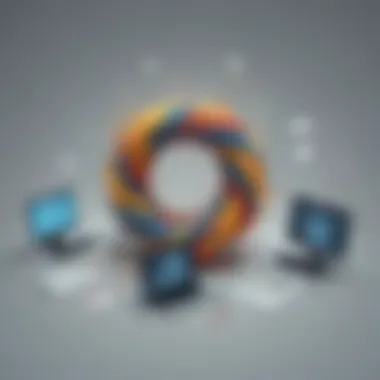
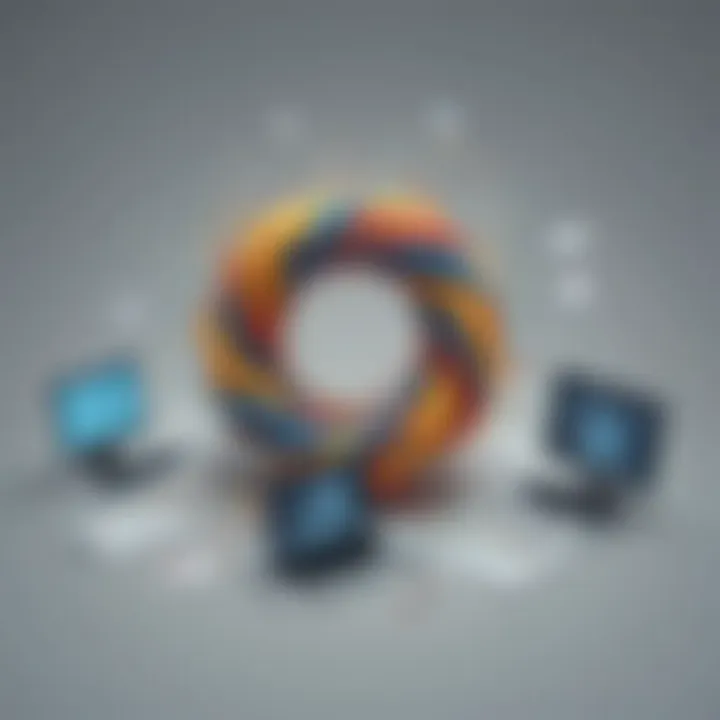
Introduction to JavaScript
JavaScript, a versatile programming language, renowned for its ability to create interactive and dynamic web pages, has become an integral part of web development. Understanding its prevalence and significance in the digital realm is crucial for any aspiring developer looking to excel in the field of programming.
History and Background
JavaScript, initially developed by Brendan Eich in 1995, has evolved significantly over the years. Originally named LiveScript, it gained prominence for its ability to bring life to static web pages and enhance user experience through dynamic content.
Features and Uses
JavaScript's flexibility lies in its ability to run on any platform, making it a cross-functional language applicable to both client-side and server-side development. From web applications to mobile app development, JavaScript's adaptability makes it a go-to language for diverse projects.
Popularity and Scope
With the rise of modern web development frameworks like React and Angular, JavaScript's popularity has skyrocketed. It is highly sought after in the job market, with opportunities ranging from front-end development to full-stack engineering roles.
Basic Syntax and Concepts
Mastering the basic syntax and concepts of JavaScript is fundamental to harnessing the power of 'await'. From understanding variables and data types to manipulating expressions and control structures, a strong foundation in these areas is indispensable.
Variables and Data Types
Variables serve as containers for storing data in JavaScript, while data types determine the nature of the values held within these variables. Understanding the nuances of data types like strings, numbers, and booleans is essential for effective programming.
Operators and Expressions
Operators in JavaScript are symbols used to perform operations on variables and values. From arithmetic operators for mathematical calculations to logical operators for decision-making, mastering the use of operators is key to writing efficient code.
Control Structures
Control structures, such as loops and conditionals, dictate the flow of a program's execution. By utilizing control structures effectively, programmers can navigate through code, perform repeated tasks, and implement logical decision-making within their scripts.
Advanced Topics
As developers progress in their JavaScript journey, delving into advanced topics becomes imperative to unlock the language's full potential. From functions and methods to object-oriented programming and exception handling, mastering these concepts sets a programmer apart.
Functions and Methods
Functions enable developers to encapsulate code into reusable blocks, promoting modular and organized programming practices. Methods, in turn, are functions associated with objects, allowing for object manipulation and interaction.
Object-Oriented Programming
Object-oriented programming (OOP) is a paradigm that promotes the concept of objects, enabling developers to structure code around data entities. By embracing OOP principles like encapsulation, inheritance, and polymorphism, programmers can write scalable and maintainable code.
Exception Handling
Error handling is a critical aspect of programming, and understanding how to manage exceptions in JavaScript is paramount. By implementing try-catch blocks and utilizing error objects, developers can gracefully handle unexpected situations and maintain the robustness of their applications.
Hands-On Examples
Applying theoretical knowledge to practical scenarios is where true mastery of JavaScript awaits. Through simple programs, intermediate projects, and insightful code snippets, developers can cement their understanding and enhance their problem-solving skills.
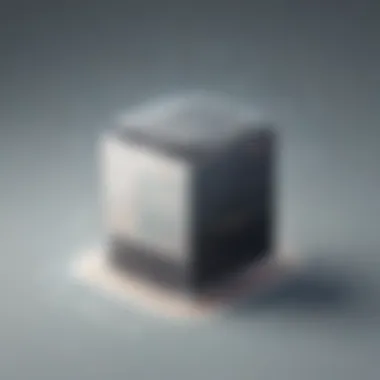
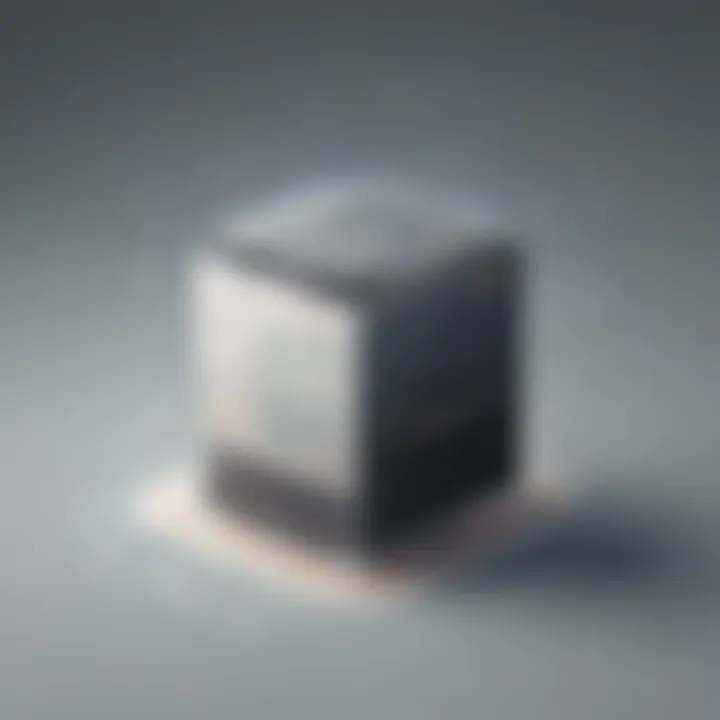
Simple Programs
By starting with small-scale programs, developers can grasp fundamental concepts and algorithmic thinking, laying a solid groundwork for more complex projects.
Intermediate Projects
Intermediate projects challenge developers to apply their skills in real-world scenarios, fostering creativity and honing their ability to design and execute functional applications.
Code Snippets
Code snippets offer succinct solutions to common programming challenges, providing valuable insights into efficient coding practices and offering inspiration for implementing logic in innovative ways.
Resources and Further Learning
Continuous learning fuels growth in programming proficiency, and JavaScript enthusiasts can benefit immensely from a plethora of resources available online.
Recommended Books and Tutorials
Books and tutorials offer in-depth explanations, code samples, and exercises to enhance one's understanding of JavaScript concepts and facilitate skill development.
Online Courses and Platforms
Online courses and platforms provide interactive learning experiences, allowing developers to engage with practical projects, collaborate with peers, and track their progress in mastering JavaScript and its intricacies.
Community Forums and Groups
Engaging with a community of like-minded individuals through forums and groups fosters collaboration, knowledge sharing, and networking opportunities. By participating in discussions and seeking guidance from experienced developers, learners can enrich their JavaScript journey and stay abreast of industry trends.
Introduction to 'await' in JavaScript
In the realm of JavaScript programming, understanding the concept of 'await' holds paramount importance. Asynchronous programming has become a cornerstone in modern software development, and 'await' plays a pivotal role in handling asynchronous operations efficiently. By delving into the intricacies of 'await', developers can streamline their code execution, improve performance, and enhance the overall user experience. This section will explore the significance of 'await' in JavaScript, shedding light on how it revolutionizes the way developers manage asynchronous tasks.
Understanding Asynchronous Programming
Introduction to Synchronous vs. Asynchronous Execution:
The distinction between synchronous and asynchronous execution lies at the heart of JavaScript development. Synchronous operations block the execution of code until a particular task is completed, whereas asynchronous operations allow the code to continue executing while waiting for tasks to finish. This fundamental difference is crucial in scenarios where time-consuming operations could hinder the responsiveness of an application. Asynchronous execution enables developers to create responsive and efficient applications by leveraging non-blocking operations.
Challenges of Callback Hell:
One of the primary challenges in asynchronous programming is the infamous 'Callback Hell.' This term refers to the nested and tangled structure of callback functions that can arise when dealing with multiple asynchronous tasks. Callback Hell not only complicates code readability but also makes error handling and debugging a daunting task. By using 'await', developers can elegantly handle this issue by writing cleaner, more maintainable code that follows a linear flow of execution, mitigating the complexities associated with nested callbacks.
Role of 'await' Keyword
Overview of 'await' in ES6:
In ES6, the introduction of the 'await' keyword revolutionized asynchronous programming in JavaScript. 'await' can only be used within functions declared with the 'async' keyword and allows developers to pause the execution of a function until a promise is settled. This simplifies the syntax for working with promises, making the code more readable and maintainable. By providing a synchronous behavior to asynchronous code, 'await' enhances the overall code structure and helps in avoiding deeply nested callbacks.
Implementing 'await' for Promises:
When implementing 'await' for promises, developers unlock a powerful mechanism to handle asynchronous operations seamlessly. By awaiting a promise, developers can retrieve the resolved value from the promise without explicitly chaining '.then()' callbacks. This not only simplifies the code but also improves error handling by utilizing 'trycatch' blocks around 'await' statements. Additionally, 'await' promotes a more intuitive and sequential coding style, allowing developers to write cleaner and more readable asynchronous code.
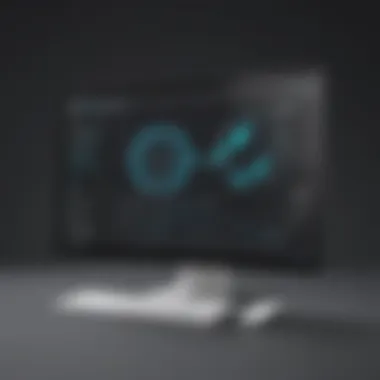
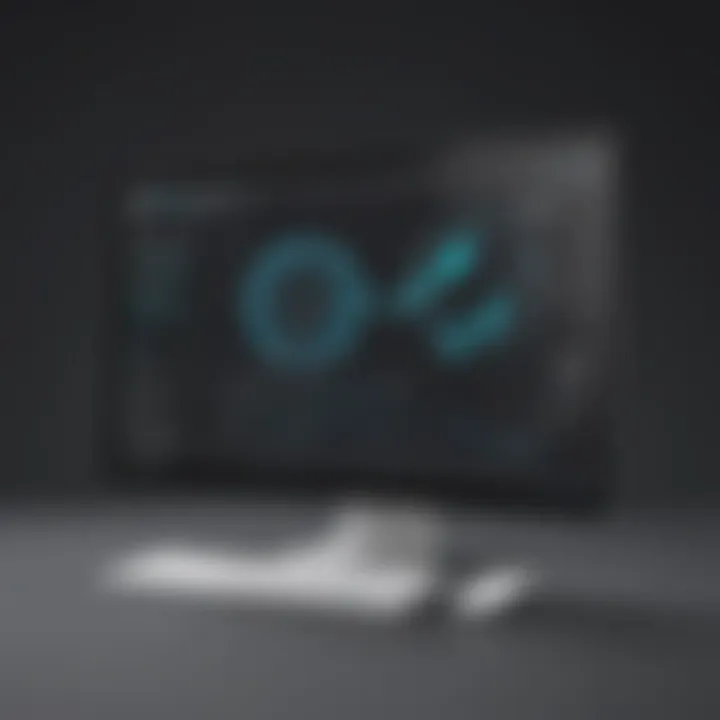
Working with Promises and 'await'
Working with Promises and 'await' is a pivotal aspect of mastering JavaScript's asynchronous programming capabilities. By delving into this topic, readers can enhance their code efficiency and functionality significantly. Promises, coupled with the 'await' keyword, offer a structured approach to handle asynchronous operations seamlessly. Understanding how to create and resolve promises lays the foundation for harnessing the full potential of 'await' in JavaScript.
Creating and Resolving Promises
Defining Promises for Asynchronous Operations
When it comes to Defining Promises for Asynchronous Operations, developers outline tasks that will be executed asynchronously. This methodology is crucial in managing tasks that require time-consuming operations without blocking the main thread. The key characteristic of Defining Promises is its ability to handle asynchronous operations in a non-blocking manner, enhancing the overall performance of the application. Developers opt for Defining Promises for its efficiency in managing complex async tasks effectively while maintaining code readability and structure.
Handling Promise Rejection
Handling Promise Rejection is an essential aspect of error management when working with promises. This functionality allows developers to handle scenarios where promises fail to fulfill, ensuring graceful error handling within the application. The key characteristic of Handling Promise Rejection is its ability to prevent unhandled promise rejections, thereby improving the robustness of the codebase. While Handling Promise Rejection adds an extra layer of error handling complexity, it serves as a crucial mechanism in ensuring the reliability of asynchronous operations.
Integrating 'await' in Promise Chains
Sequential Execution with 'await'
Sequential Execution with 'await' enables developers to execute asynchronous tasks in a synchronized manner, maintaining the order of operations within the code flow. The key characteristic of Sequential Execution with 'await' is its simplistic approach to ensure that each asynchronous task is completed before moving on to the next. This sequential nature promotes code clarity and simplifies the debugging process, enhancing the overall maintainability of the codebase.
Nesting 'await' within Promises
Nesting 'await' within Promises allows developers to handle complex asynchronous scenarios by chaining multiple asynchronous operations. This approach is beneficial in scenarios where tasks depend on the outcome of previous asynchronous actions. The unique feature of Nesting 'await' within Promises is its ability to streamline complex asynchronous logic, offering a structured approach to manage dependencies between asynchronous tasks. While nesting 'await' enhances code organization and readability, excessive nesting may lead to callback hell, requiring careful consideration during implementation.
Advanced Techniques with 'await'
In the realm of JavaScript programming, the utilization of 'await' extends beyond basic implementations to encompass advanced techniques that elevate code functionality and efficiency. Understanding and mastering advanced techniques with 'await' are crucial components in enhancing asynchronous programming paradigms. By delving into the intricacies of 'await' and its nuanced features, developers can optimize their code for better performance and reliability. Advanced techniques with 'await' not only streamline asynchronous operations but also provide avenues for error handling, timeout management, concurrency control, and parallelism.
Error Handling and Timeout Management
Utilizing 'trycatch' for Error Handling
The incorporation of 'trycatch' blocks in error handling mechanisms plays a pivotal role in ensuring code robustness and stability. 'trycatch' facilitates the identification and management of exceptions that may arise during the execution of asynchronous operations. By encapsulating potentially error-prone code within a 'try' block, developers can catch and handle exceptions gracefully, preventing abrupt program termination. This structured approach to error handling enhances code reliability and user experience, making it a fundamental aspect of mastering asynchronous JavaScript. Despite its advantages, developers should be cautious not to overuse 'trycatch' as it can lead to obscured code logic and hinder debugging processes.
Implementing Timeout Logic with 'await'
Setting timeouts for asynchronous operations mitigates the risk of prolonged execution, ensuring that tasks do not linger indefinitely. Integrating timeout logic with 'await' enables developers to establish predefined time limits for asynchronous tasks, enhancing application responsiveness and preventing potential bottlenecks. By defining explicit timeout intervals, developers can safeguard their applications against unresponsive operations, thereby improving user satisfaction and overall performance. However, it is essential to strike a balance between timeout durations and task complexities to avoid premature timeouts or excessive delays, optimizing the execution of asynchronous code.
Concurrency Control and Parallelism
Executing Tasks Concurrently with 'await'
Concurrency control through executing tasks concurrently with 'await' empowers developers to enhance application responsiveness and efficiency by leveraging parallel processing capabilities. By orchestrating multiple asynchronous tasks simultaneously, developers can exploit system resources more effectively, reducing overall execution times and improving scalability. The seamless integration of concurrency control with 'await' offers a versatile approach to managing complex workflows, unlocking opportunities for multitasking and performance optimization. However, improper concurrency management can introduce synchronization issues and resource conflicts, underscoring the importance of meticulous task coordination.
Optimizing Performance through Parallelism
Optimizing performance through parallelism entails the strategic allocation of computational tasks across multiple processors or cores, maximizing processing capabilities and throughput. By distributing workloads in parallel, developers can harness the full potential of hardware resources, accelerating task completion and enhancing overall system performance. The integration of parallelism with 'await' in asynchronous JavaScript opens new avenues for improving code efficiency and responsiveness, particularly in demanding computational scenarios. However, effective parallelism necessitates careful consideration of task dependencies and resource allocation to prevent race conditions and maintain program integrity.
Best Practices and Optimization
In the realm of JavaScript programming, adopting best practices and optimizing code play pivotal roles in enhancing overall efficiency and functionality. Within the context of mastering JavaScript's 'await' keyword, best practices and optimization hold significant importance. By adhering to best practices, developers can streamline their codebase, improve readability, and boost performance. Optimization focuses on fine-tuning code for maximum efficiency, which is crucial in asynchronous programming where timing and resource management are critical aspects.
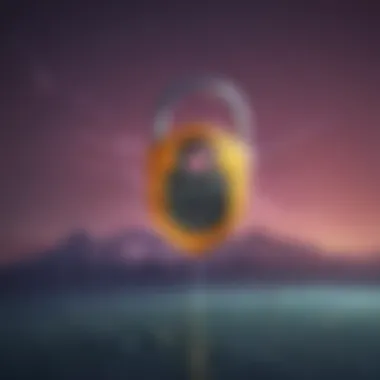
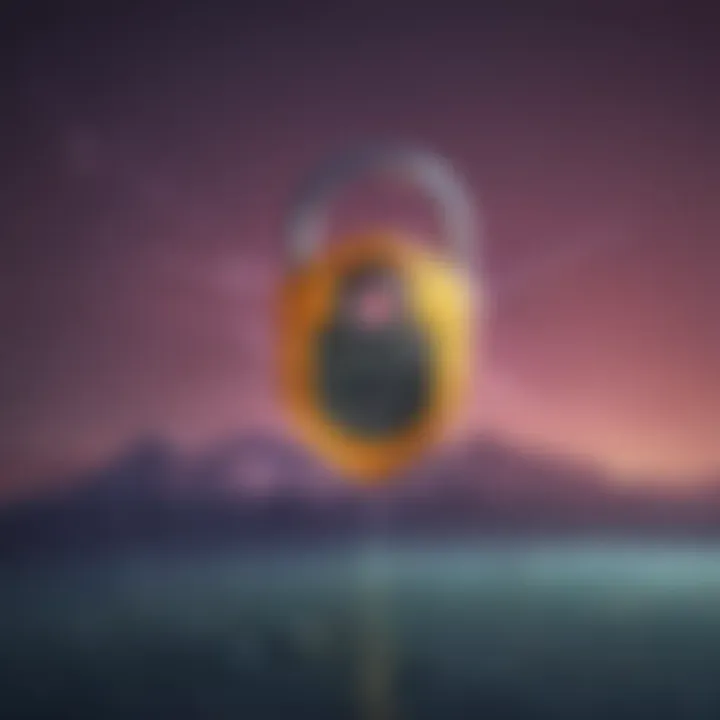
Code Refactoring Strategies
Enhancing Readability with 'await'
Enhancing readability with the 'await' keyword involves structuring code in a coherent and logical manner that is easy to interpret and understand. The key characteristic of enhancing readability with 'await' is that it simplifies asynchronous code execution by making it more sequential and easier to follow. This strategy is a popular choice in the realm of JavaScript programming as it significantly reduces complexity and aids in maintaining codebases efficiently. The unique feature of enhancing readability with 'await' lies in its ability to eliminate callback hell and promote a more intuitive coding style, ultimately leading to improved code quality and maintainability.
Streamlining Codebase for Efficiency
Streamlining the codebase for efficiency revolves around optimizing code structure and performance. A key characteristic of this strategy is the focus on minimizing redundant or inefficient code segments, thereby enhancing the overall speed and reliability of the application. Streamlining the codebase is a beneficial choice for this article as it directly contributes to improving code efficiency and maintainability. The unique feature of streamlining the codebase lies in its ability to reduce the chances of errors, enhance developer productivity, and facilitate easier debugging processes, making it an essential aspect of mastering asynchronous JavaScript.
Performance Tuning and Benchmarking
Measuring Execution Times with 'await'
Measuring execution times with the 'await' keyword is essential for gauging the speed and performance of asynchronous operations. The key characteristic of measuring execution times with 'await' is that it provides developers with insights into the efficiency of their code, allowing for optimizations and fine-tuning where necessary. This strategy is a popular choice as it helps in identifying bottlenecks and optimizing critical code segments. The unique feature of measuring execution times with 'await' is its ability to offer precise data on code performance, enabling developers to make informed decisions for enhancing efficiency and reducing latency in their applications.
Identifying Bottlenecks for Optimization
Identifying bottlenecks for optimization involves pinpointing areas in the code that are causing slowdowns or inefficiencies in execution. The key characteristic of this strategy is its focus on improving overall performance by addressing and resolving bottlenecks that impede code efficiency. Identifying bottlenecks for optimization is a beneficial choice for this article as it facilitates the fine-tuning of asynchronous operations, leading to better responsiveness and user experience. The unique feature of this strategy lies in its capacity to enhance the scalability and robustness of applications by mitigating performance issues, making it a crucial aspect of mastering asynchronous JavaScript programming.
Real-World Applications and Examples
In the realm of JavaScript programming, exploring real-world applications and examples holds profound significance. Within the context of this in-depth guide on mastering 'await' in JavaScript, the section highlighting real-world applications serves as a bridge between theory and practical implementation. By delving into concrete examples and cases where 'await' proves instrumental, readers gain a tangible understanding of its potential in enhancing code efficiency and functionality. The practicality of real-world applications not only solidifies theoretical concepts but also provides a roadmap for incorporating 'await' into real projects.
Implementing 'await' in Web Development
Fetching Data from APIs
Delving into the nuances of fetching data from APIs stands as a crucial aspect in the landscape of web development. Within the scope of this comprehensive 'await' guide, understanding the intricacies of data retrieval from APIs sheds light on the practical utilization of asynchronous operations. The key characteristic of fetching data from APIs lies in its ability to seamlessly retrieve information from external sources, ensuring dynamic and up-to-date content for web applications. This method proves advantageous in modern web development practices by enabling developers to leverage the vast pool of data offered by external APIs, empowering them to create dynamic and interactive web experiences.
Managing Multiple Asynchronous Requests
Managing multiple asynchronous requests emerges as a pivotal component when implementing 'await' in web development. In this guide centered on mastering 'await' in JavaScript, the effective management of concurrent asynchronous operations unveils a strategic approach to optimizing performance and responsiveness in web applications. The distinctive feature of managing multiple asynchronous requests revolves around the parallel execution of multiple tasks, allowing for efficient handling of complex workflows. While this technique enhances the speed and responsiveness of web applications, it also requires meticulous planning to avoid potential bottlenecks and ensure seamless functionality.
Enhancing User Experience with 'await'
Elevating user experience through the integration of 'await' underscores the essence of user-centric design in JavaScript development. This section within the comprehensive guide elucidates how dynamic content loading plays a pivotal role in delivering seamless and engaging web experiences to visitors. By dynamically loading content based on user interactions or external events, developers can ensure a fluid and interactive user experience, enriching the overall quality of web applications.
Dynamic Content Loading
Dynamic content loading represents a cornerstone in user experience enhancement strategies, particularly in the context of JavaScript development. Understanding the crux of dynamic content loading within the scope of this guide accentuates its capability to provide users with real-time updates and information, creating a personalized and immersive browsing experience. The distinctive advantage of dynamic content loading lies in its responsiveness to user inputs and preferences, ensuring that web content remains fresh and relevant, thereby fostering user engagement and satisfaction.
Interactive Page Updates
Incorporating interactive page updates within web applications elevates the interactivity and responsiveness of digital interfaces, enriching the overall user experience. Within this guide focusing on mastering 'await' in JavaScript, the emphasis on interactive page updates underscores the role of real-time data refresh and interface modifications in enhancing user engagement. By facilitating dynamic updates to webpage elements without requiring a full reload, interactive page updates optimize the user experience by providing seamless transitions and ensuring uninterrupted interaction with the application. The strategic inclusion of interactive page updates showcases the commitment to user-centric design and fosters positive user interactions within web environments.
Conclusion and Future Trends
In the conclusion and future trends section of this comprehensive guide on mastering JavaScript Await, it is imperative to reflect on the essence of discussions. Asynchronous programming is rapidly evolving, and a firm grasp on the Conclusion and Future Trends is crucial. Understanding the upcoming trends helps in preparing developers for innovative solutions and challenges. Deciphering the landscape of Await in JavaScript paves the way for better anticipation and adaptation. By exploring the ever-changing nature of asynchronous programming, developers can streamline their code and enhance scalability. The Conclusion and Future Trends segment serves as a compass, guiding developers towards optimized coding practices and efficiency.
Mastering Asynchronous JavaScript
Summary of Key Takeaways
Delving into the Summary of Key Takeaways, it becomes apparent that it encapsulates the essence of the entire discussion on Await in JavaScript. This segment distills the complexities of asynchronous programming into digestible insights. The key takeaways offer a condensed version of crucial concepts and practical applications, aiding developers in grasping the core principles efficiently. Understanding the Summary of Key Takeaways enhances code readability and maintainability. Its concise nature allows for a quick reference to essential points, fostering a deeper comprehension of Await usage in JavaScript. The Summary of Key Takeaways acts as a roadmap for optimizing asynchronous code execution, ensuring seamless functionality and performance.
Exploring Evolving Practices in Async Programming
Exploring the Evolving Practices in Async Programming sheds light on the dynamic nature of asynchronous JavaScript. Embracing evolving practices is paramount for staying ahead in the digital realm. The segment emphasizes adaptability and foresight in utilizing Await effectively. By incorporating the latest practices, developers can elevate their coding standards and cater to modern development requirements. The Evolving Practices in Async Programming section offers insights into cutting-edge techniques and methodologies, fostering innovation and efficiency. It enables developers to future-proof their codebase and adapt to industry trends seamlessly, resulting in robust and scalable applications.