Unraveling the Intricacies of the Java Visitor Design Pattern: An In-Depth Guide
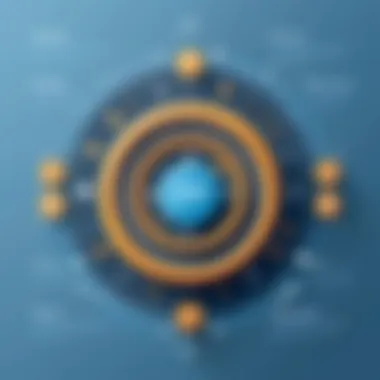
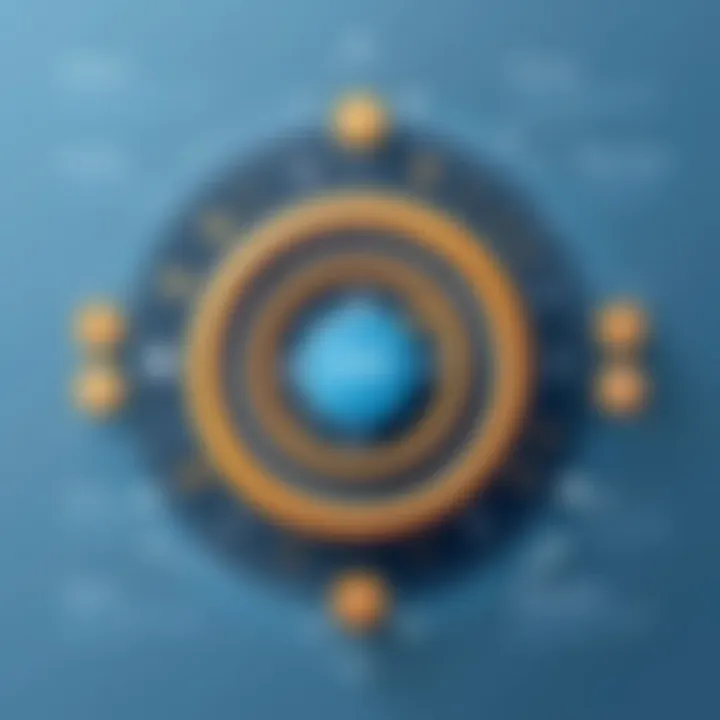
Foreword to the Java Visitor Design Pattern
The Java Visitor design pattern holds a pivotal role in object-oriented programming, acting as a catalyst for enhanced code modularity and flexibility. Understanding its nuances is crucial for Java programmers looking to optimize their code structures. This section will delve into the historical backdrop of the Visitor pattern, its evolution, and the contemporary relevance it holds in Java programming circles. By exploring the foundations of this pattern, readers will gain a comprehensive understanding of its significance.
Implementation of the Java Visitor Design Pattern
Delving into the implementation aspects of the Java Visitor design pattern unveils a world of intricate coding practices. From defining visitor interfaces to establishing concrete visitors, each step in the implementation process contributes to the pattern's robustness. This section will navigate through the key elements of implementing the Visitor pattern in Java, offering practical insights and real-world examples to elucidate complex concepts. By unraveling the implementation details, readers can grasp the mechanics behind integrating the Visitor pattern into their projects.
Benefits of the Java Visitor Design Pattern
Unveiling the benefits of the Java Visitor design pattern sheds light on the transformative potential it holds for Java developers. Enhanced code maintainability, separation of concerns, and extensibility are among the myriad advantages offered by this pattern. This section will meticulously dissect each benefit, illustrating how the Visitor pattern streamlines code architecture and fosters scalability. By highlighting the tangible perks of adopting the Visitor pattern, readers can envision the profound impact it can have on their programming endeavors.
Practical Applications of the Java Visitor Design Pattern
Examining the practical applications of the Java Visitor design pattern unveils a spectrum of use cases across diverse Java programming scenarios. From traversing complex data structures to implementing modular code transformations, the application possibilities are boundless. This section will showcase real-world examples where the Visitor pattern proves instrumental in solving programming challenges efficiently. By immersing in these practical applications, readers can glean insights into leveraging the Visitor pattern for enhancing the robustness of their Java codebases.
Synthesis of Information
The synthesis of information encapsulates the core takeaways from exploring the Java Visitor design pattern. By consolidating the historical evolution, implementation intricacies, benefits, and practical applications, readers will attain a holistic view of this pattern's significance. This section will weave together the threads of knowledge presented throughout the article, offering a comprehensive synthesis that reinforces the importance of incorporating the Visitor pattern in Java programming practices.
Prelims
In the realm of object-oriented programming, the exploration of design patterns stands as a pinnacle of relevance and necessity. Within this extensive guide dedicated to unraveling the intricacies of the Java Visitor design pattern, a profound insight awaits. Through meticulous examination, this article undertakes the task of elucidating not merely the surface-level implementations but delves deeper into the very essence of code structuring and optimization. By dissecting the Visitor pattern brick by brick, readers are poised to grasp the core principles essential for enhancing code modularity and flexibility.
Understanding Design Patterns
The role of design patterns in software development
Venturing into the sphere of software development, the adoption of design patterns emerges as a catalyst for streamlined coding practices and improved maintainability. The very crux of design patterns lies in encapsulating proven solutions to recurrent coding challenges, providing a roadmap for developers to navigate complex coding paradigms with ease. In this article, the magnified lens on the role of design patterns transcends mere functionality; it operates as a beacon of coding excellence, guiding programmers towards efficient and structured coding methodologies.
Benefits of implementing design patterns
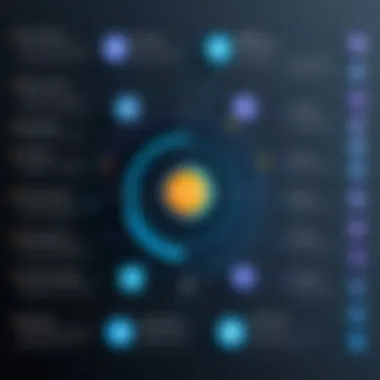
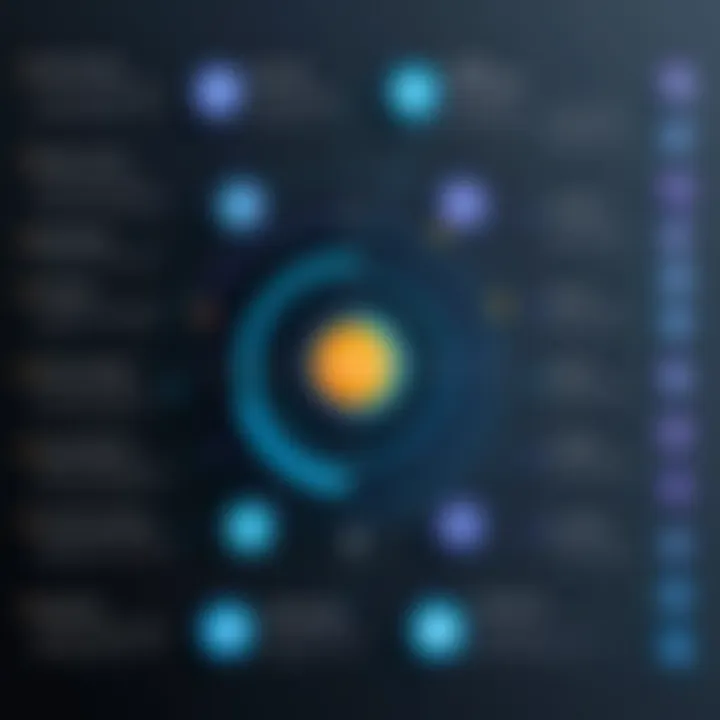
The incorporation of design patterns into the software development lifecycle yields a myriad of advantages, each contributing to the overall robustness and scalability of the codebase. From facilitating code reuse to promoting uniformity across projects, the benefits of implementing design patterns are multifaceted. As this article unfolds the layers of implementing design patterns, readers are exposed to a treasure trove of coding techniques aimed at fostering code maintainability and extensibility.
Overview of the Visitor Pattern
Definition of the Visitor pattern
At the core of the Visitor pattern lies a profound concept: separating the component hierarchy from the operations that can be performed upon them. This distinctive trait sets the Visitor pattern apart as a valuable tool in Java programming, emphasizing a clear segregation of concerns between object structure and algorithms. Delving deep into the definition of the Visitor pattern reveals a design elegance that transcends traditional coding practices, paving the way for enhanced modularity and code readability.
Key components and structure
As we unravel the intricate threads of the Visitor pattern, an array of key components and structural elements come to light. From the Visitor interface to ConcreteVisitor classes, each component plays a pivotal role in shaping the code architecture. The structural integrity of the Visitor pattern hinges on the seamless interaction between elements, orchestrating a symphony of visitations that imbue flexibility and extensibility into the codebase. By scrutinizing the key components and structure of the Visitor pattern, this article equips readers with the essential knowledge required to wield this powerful design pattern with finesse.
Fundamentals of the Visitor Pattern
In this pivotal section elucidating the fundamentals of the Visitor Pattern, we unearth the essence of structured programming paradigms. Understanding the core principles of the Visitor Pattern is paramount in achieving software design elegance. Through a meticulous exploration, we dissect the elements that form the bedrock of this pattern, shedding light on their intricate interplay and significance. Delving deep into the visitor pattern's core, one finds a treasure trove of modularity and extensibility that redefine the conventional programming landscape.
Visitor Pattern Structure
Elements of the Visitor pattern
Embarking on an expedition through the intricate web of the Visitor pattern's elements uncovers a rich tapestry of design ingenuity. These foundational elements serve as building blocks, orchestrating a symphony of interactions within the software architecture. The elegance lies in their versatility to encapsulate operations without disturbing the element hierarchy, fostering a dynamic and adaptable design environment. By embracing these elements, programmers open doors to a realm where flexibility and scalability reign supreme.
Relationship between elements
Navigating the labyrinthine corridors of the Visitor pattern's element relationships unravels a narrative of interconnectedness and cohesion. The symbiotic interplay between elements orchestrates a harmonious dance, facilitating seamless communication and interaction. This intricate web of relationships forms the backbone of the Visitor pattern's efficacy, enabling streamlined traversal of complex structures and operations with finesse and precision.
Visitor Pattern Participants
Visitor
Traversing the landscape of Visitor pattern participants unveils the pivotal role of the Visitor entity. Acting as a sentinel of operations, the Visitor encapsulates behavior, offering a modular approach to extend functionality without disturbing existing structures. Its minimalist design and streamlined execution make it a cornerstone of code elegance, elevating the software architecture to new heights of modularity and clarity.
Element and ConcreteElement
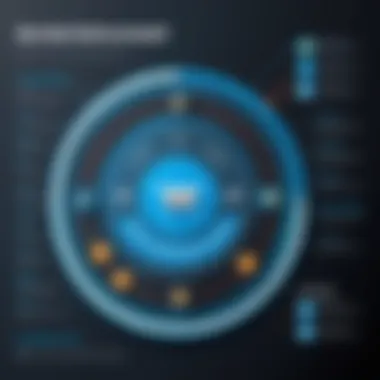
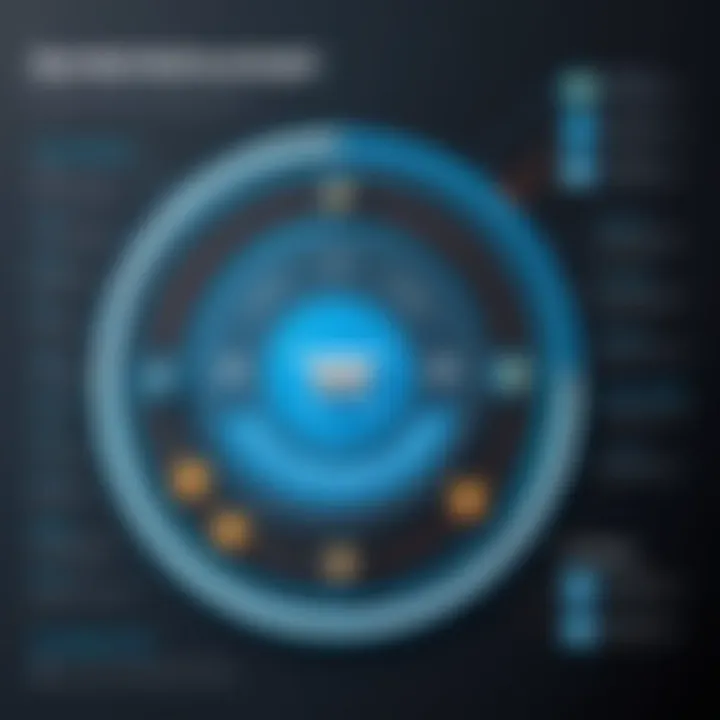
Exploring the realm of Element and ConcreteElement delves into the heart of object hierarchy and composition. These entities form the backbone of structural integrity, defining the essence of software elements and their implementations. Embracing the synergy between Element and ConcreteElement paves the way for a robust and dynamic object structure, where clarity and extensibility converge in a harmonious symphony.
ObjectStructure
Unveiling the intricate tapestry of ObjectStructure unfurls a canvas of organization and management within the software ecosystem. Serving as a repository of elements and orchestrating their traversal, ObjectStructure embodies the essence of order and efficiency. Its role in mediating relationships and interactions between elements transcends mere management, fostering a dynamic and cohesive software environment.
Implementing the Visitor Pattern in Java
The Implementation of the Visitor Pattern in Java is a crucial aspect of this comprehensive guide, delving deep into the practical application of this design pattern. By dissecting the step-by-step process of incorporating the Visitor Pattern into Java programming, readers will gain valuable insights into enhancing code structure and flexibility. Exploring the nitty-gritty details of Visitor Pattern implementation sheds light on its significance within the realm of object-oriented programming. Embracing this section means embracing a deeper understanding of software design principles and their real-world implications.
Step-by-Step Implementation
Creating Visitor and ConcreteVisitor Classes:
Generating the Visitor and ConcreteVisitor classes plays a pivotal role in implementing the Visitor Pattern efficiently. These classes form the backbone of the pattern, enabling clear separation between operations applied to elements in the object structure. The essence of Creating Visitor and ConcreteVisitor classes lies in their ability to encapsulate specific behaviors without altering the core components of the elements they interact with. While this approach promotes extensibility and maintainability, it also necessitates careful consideration of the overall design to ensure smooth integration and scalability. The unique selling point of Creating Visitor and ConcreteVisitor classes lies in their capacity to streamline complex operations and promote a modular approach to programming.
Defining Element Hierarchy:
The Element hierarchy serves as the cornerstone of the Visitor Pattern, defining the structure of elements within the object hierarchy. Establishing a clear Element hierarchy paves the way for seamless traversal and manipulation of objects, aligning with the Visitor Pattern's goal of separating concerns and facilitating new operations. Defining Element hierarchy offers a strategic advantage by promoting a logical organization of elements, enabling systematic application of visitors across various object types. However, this also introduces a level of rigidity that must be balanced with flexibility to adapt to evolving project requirements. The defining characteristic of Element hierarchy lies in its ability to establish a hierarchical relationship between elements, guiding the Visitor Pattern's traversal and interaction processes.
Implementing ObjectStructure:
The ObjectStructure implementation is crucial for orchestrating the interaction between elements and visitors within the Visitor Pattern. By defining how elements are organized and accessed, Implementing ObjectStructure lays the foundation for seamless visitor-element communication. The key focus of Implementing ObjectStructure is to centralize element management and visitor coordination, ensuring a cohesive approach to performing operations on the object elements. While this centralized approach enhances code readability and maintenance, it also introduces a level of dependency that must be managed effectively to prevent code entanglement. The unique feature of Implementing ObjectStructure lies in its role as the architectural glue that binds elements and visitors, facilitating efficient traversal and operation execution within the pattern.
Benefits of Using the Visitor Pattern
The importance of the Visitor pattern lies in its ability to enhance code modularity and flexibility. By structuring code in a way that separates concerns and allows for the addition of new operations with ease, the Visitor pattern brings significant advantages to software development. The pattern promotes a clean and organized codebase, making it easier to manage and maintain large-scale projects. Additionally, the Visitor pattern facilitates the implementation of complex operations on object structures without the need for extensive changes to the existing codebase.
Enhanced Modularity
Separation of concerns
When we discuss the Separation of concerns aspect in the context of the Visitor pattern, we are highlighting the practice of isolating different responsibilities within the code. This separation ensures that each component of the software system focuses on a specific task or functionality, making the codebase more organized and easier to understand. The key characteristic of Separation of concerns is its ability to improve code readability and maintenance by reducing dependencies between different parts of the system. This approach proves beneficial in scenarios where scalability and extensibility are crucial, allowing developers to make changes to one part of the system without impacting others.
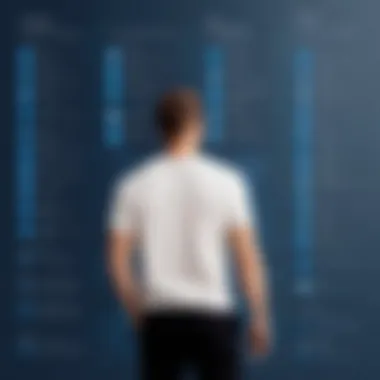
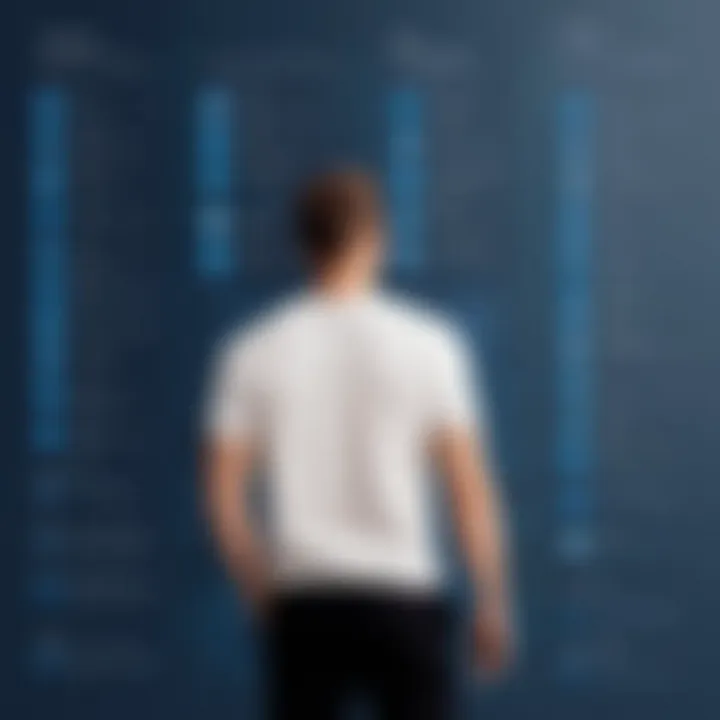
Ease of adding new operations
Another pivotal aspect of the Visitor pattern is its support for the seamless addition of new operations to existing data structures. This feature simplifies the process of extending functionalities within the system, as developers can introduce new operations without modifying the core elements. The key characteristic here is the flexibility it provides in incorporating new functionalities without risking the stability of the existing codebase. By embracing this approach, developers can adapt to changing requirements and business needs swiftly, making the codebase more adaptable and future-proof.
Flexibility and Extensibility
Adding new visitors without modifying elements
One of the prominent benefits of the Visitor pattern is the capability to add new visitors without the need to alter the existing elements of the system. This flexibility allows for the seamless integration of new behaviors or functionalities without disrupting the core structure of the software. The key characteristic of this aspect is the freedom it offers in extending the system's capabilities without introducing structural changes. This approach enhances the overall flexibility of the system, enabling developers to incorporate new features independently of the existing elements.
Modifying visitor behavior
Modifying visitor behavior presents another compelling aspect of the Visitor pattern, emphasizing the ability to adjust the behavior of visitors without impacting the elements they interact with. This modifiability promotes a dynamic and adaptable system architecture, where developers can tailor visitor behaviors to specific requirements efficiently. The key characteristic in this scenario is the versatility it offers in customizing the interaction between visitors and elements, empowering developers to fine-tune the system's functionality with precision. By leveraging this capability, developers can enhance the extensibility of the codebase and respond effectively to evolving business needs.
Practical Applications of the Visitor Pattern
Use Cases in Software Development
Traversing complex data structures
Traversing complex data structures in software development plays a crucial role in efficiently navigating intricate data hierarchies. This process involves iteratively moving through interconnected data elements to perform specific operations or gather relevant information. By employing the Visitor pattern, developers can seamlessly traverse elaborate data structures, ensuring targeted actions are executed with precision. The Visitor pattern enhances the scalability and maintainability of traversal algorithms, enabling smoother interactions with diverse data sets. Despite its intricate nature, traversing complex data structures offers a robust mechanism for handling data-rich applications.
Implementing operations on object structures
Implementing operations on object structures involves applying designated functionalities to diverse object collections within a system. This facet of software development focuses on executing tailored actions on varying object types while maintaining code modularity and extensibility. With the Visitor pattern, developers can encapsulate specific operations within visitor classes, enabling seamless integration with different object structures. By adopting this approach, developers can introduce new operations without modifying existing object hierarchies, fostering enhanced code flexibility and reusability. Implementing operations on object structures through the Visitor pattern streamlines the addition of functionalities to complex systems, bolstering software adaptability and performance.
Culmination
In the realm of Java programming, grasping the intricacies and nuances of the Visitor Design Pattern holds paramount importance. This conclusive segment encapsulates the essence of the Visitor pattern's utility and the profound impact it can wield on object-oriented software development. By meticulously examining each facet discussed in this comprehensive guide, readers will glean a profound understanding of how the Visitor pattern not only streamlines code modularity but also augments flexibility and extensibility within the software architecture. Delving deeper into the Visitor pattern underscores its significance as a fundamental tool in the Java programmer's arsenal, offering a systematic approach to manage hierarchical object structures effectively.
Summary of Key Points
Significance of the Visitor pattern in Java programming
Unveiling the layers of the Visitor pattern's essence in Java programming illuminates its pivotal role in enhancing the clarity and maintainability of code. Recognized for its adeptness in facilitating the separation of concerns, the Visitor pattern stands out as a linchpin for ensuring the seamless integration of new operations without disrupting existing structures. This distinctive trait propels the Visitor pattern into the realm of indispensability for programmers seeking to uphold a modular and extensible codebase. Acknowledging the Visitor pattern's paramount importance not only accentuates its utility but also reinforces its standing as a preferred mechanism for traversing complex data structures in Java programming.
Benefits and applications in software development
Evaluating the merits of integrating the Visitor pattern in software development sheds light on its role in enabling developers to encapsulate operations within distinct visitor classes, thereby promoting code reusability and modularity. The Visitor pattern's adaptive nature empowers programmers to introduce new functionalities seamlessly, fostering a dynamic environment for catering to evolving project requirements. Furthermore, the pattern's versatility in application frameworks and compiler design scenarios underscores its adaptability across a myriad of development contexts, underscoring its prowess as a versatile and scalable solution for accommodating diverse software needs.