Connect Java to SQL Server: A Detailed Guide
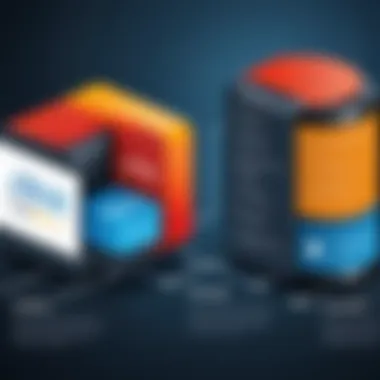
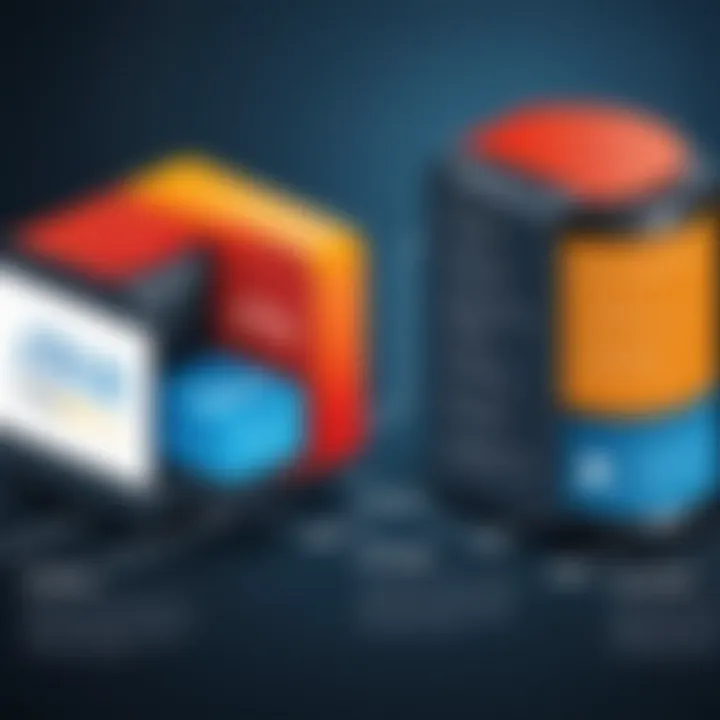
Intro
Establishing a connection between Java applications and SQL Server databases is an essential skill in the world of software development. It is not just about running queries or fetching data; this integration is the backbone of many applications that rely on robust data management. A solid connection can influence not only the performance of your application but also the overall user experience.
In this guide, we are going to navigate through the crucial steps necessary for achieving this connection. Whether you are just starting or have some experience, this article aims to cover all aspects from the basics to the nitty-gritty details.
Understanding the Basics of Java and SQL Server Connection
A few prerequisites are required before diving into the code. Here weâll touch on the importance of Java Database Connectivity (JDBC). It acts as the bridge between Java and SQL databases, simplifying the process of managing connections, executing queries, and handling results.
"Without connection, your application is like a ship in the desertâgoing nowhere."
Key Terms
- JDBC: A Java API that manages connecting to a database, sending queries, and retrieving results.
- SQL Server: A relational database management system developed by Microsoft.
- Connection String: A string containing information about a data source and the means of connecting to it.
By understanding these elements, you can start to appreciate how Java interacts with SQL Server, thus laying the groundwork for our more complex discussions later.
Setting Up Your Environment
Before coding, some groundwork is essential. Youâll need to ensure that your local environment has the necessary tools installed. Hereâs a quick checklist:
- Java Development Kit (JDK): Make sure you have the latest version that matches your operating system.
- SQL Server: You can download SQL Server Express if youâre working on a small project.
- JDBC Driver: Youâll require the JDBC driver for SQL Server, which can typically be found on Microsoftâs official site.
Once everything is installed, itâs crucial to configure your environment correctly. Set the JDBC driver in your Java projectâs classpath to allow your code to access the driver needed for establishing the connection.
Establishing the Connection
Now we arrive at the juicy partâcreating the connection. This typically involves the following steps:
- Load the JDBC driver class.
- Define the connection string that points to your SQL Server.
- Establish the connection using .
- Handle exceptions accordingly, as issues may arise when connecting.
Hereâs a basic example of how to do this in practice:
This snippet effectively establishes a connection. Notice the use of try-with-resources; it ensures that the connection is closed automatically after use, which is a best practice you should definitely adopt.
End
In summary, connecting Java with SQL Server requires attention to detailâfrom the setup of your local environment to the configuration of JDBC in your code. This guide outlines the importance of each of these steps, placing you on a solid path to leveraging Java's powerful capabilities alongside SQL Server. The intricacies can be daunting, but with a bit of practice, you should become proficient in establishing this connection, unlocking a world of opportunities in your development journey.
For further reading, you might find the following resources useful:
Preface to Java SQL Server Connection
Connecting Java applications with SQL Server databases is not just a technical necessity; it forms the backbone of data-driven applications. Understanding how to establish these connections allows developers to harness the power of SQL Server from their Java environment. What makes this an important topic is the growing reliance on relational databases in application development, especially for enterprise solutions.
When you think about it, these connections enable your application to retrieve, manipulate, and store data efficiently. Every interaction with the database, whether it's pulling user information or saving logging details, hinges on a properly established connection.
Understanding the Need for Database Connections
Database connections serve as the bridge between Java applications and SQL Server databases, facilitating seamless communication. Imagine wanting to build a Java application that fetches real-time data or updates a database based on user interactions. Without a secure and efficient connection, all these functionalities would remain just wishful thinking.
- Efficiency: A good connection reduces latency. Latency, in simple terms, is the delay before data transfer begins. While we often think of speed in computing terms, a good connection can mean the difference between a snappy application and a sluggish one.
- Data Integrity: Maintaining data integrity is crucial. A robust connection strategy helps ensure that data remains consistent and valid throughout transactions.
- Security: Poorly managed connections can open the door to security vulnerabilities. Establishing secure connections protects sensitive data and ensures that only authorized users access the database.
Understanding the nuances of this connection allows developers to choose the right strategies, prevent bottlenecks, and ultimately serve a better experience to the end-users.
Overview of Java and SQL Server
Java, a versatile and widely-used programming language, is renowned for its portability and ease of use. Combining it with SQL Server, a powerful relational database management system by Microsoft, opens a wealth of opportunities.
Java Features:
- Cross-Platform: Write once, run anywhere. Java applications can run on any system that has the Java Virtual Machine.
- Strong Community: With vast resources, forums like Reddit provide insights and support for developers.
SQL Server Features:
- Robust Performance: Designed to handle large volumes of data efficiently.
- Scalability: As your application grows, SQL Server scales effortlessly to accommodate increased load.
Together, Java and SQL Server provide a comprehensive ecosystem. This synergy maximizes data utility, ensuring developers can create applications that are not only functional but also capable of handling complex data interactions seamlessly.
A well-established Java-SQL Server connection sets the stage for optimal performance. The better the communication between your application and the database, the smoother your system will run.
Fundamentals of JDBC
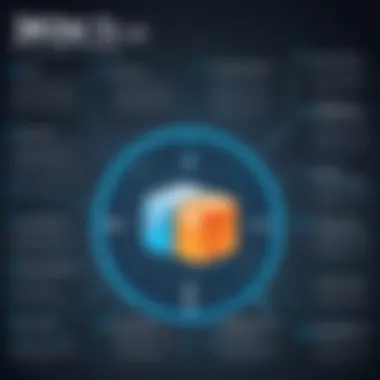
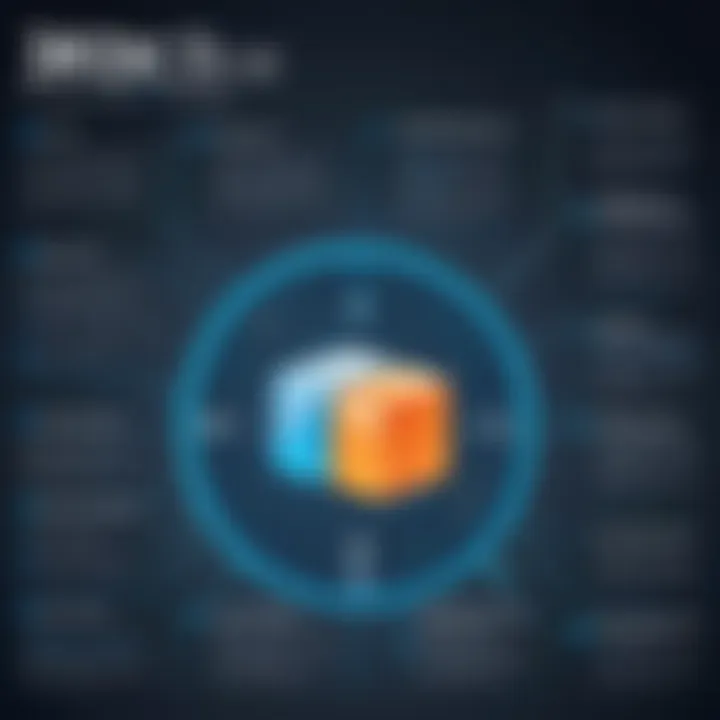
When diving into the realm of Java and SQL Server interactions, it's crucial to grasp the Fundamentals of JDBC. Java Database Connectivity, commonly called JDBC, serves as the backbone for connecting Java applications with various databases, including SQL Server. This powerful API isn't just a bridge between Java programs and databases; it encapsulates a rich set of interfaces and classes that facilitate efficient data access and manipulation. Understanding JDBC paves the way for developers to effectively manage database communications, optimize performance, and enhance application functionalities.
What is JDBC?
JDBC is essentially a Java-based API that allows developers to connect Java applications with different databases using standard calls. Through JDBC, a programmer can send SQL statements to the database, retrieve results, and manage data with ease. Before JDBC, database interactions required complex, platform-specific code. With JDBC, that complexity is transformed into straightforward method calls. Think of JDBC as your translator when dealing with databases; it takes your requests in Java and translates them into SQL languageâthen translates the database's responses back into something Java can understand.
Key Components of JDBC
The architecture of JDBC is made up of several key components, each serving a vital purpose. Understanding these components is crucial for anyone looking to efficiently utilize JDBC in their projects.
- JDBC Driver: This is the core of any JDBC application. The driver implements the JDBC API for a specific database. It converts the Java calls into vendor-specific calls. Different types are available, like Type 1, Type 2, Type 3, and Type 4 drivers, each with its unique implementation and usage scenarios.
- Connection: This component establishes a pathway between your Java application and the database. Connection objects handle the details of establishing and maintaining connection states, and they allow transactions to be conducted reliably.
- Statement: Once you have a connection to the database, you need to create a Statement object to send SQL queries. There are various types of statements available, including Statement, PreparedStatement, and CallableStatement, suitable for different execution scenarios.
- ResultSet: After executing a query, the data that comes back from the database is encapsulated in a ResultSet object. Think of it as a table of data that makes processing the returned rows manageable and convenient.
- SQLException: Errors and exceptions are part and parcel of working with databases. SQLException handles any issues that arise during database interactions, allowing developers to troubleshoot effectively.
JDBC Driver Types
When it comes to JDBC, understanding driver types is essential, as it influences the performance and compatibility when connecting to SQL Server. Here are the various types:
- Type 1 Driver (ODBC-Bridge Driver): This driver translates JDBC calls into ODBC calls; however, itâs less preferred in todayâs applications due to overhead and limited performance.
- Type 2 Driver (Native-API Driver): This driver converts JDBC calls into database-specific calls using the native API of the database. It can provide better performance than Type 1, but it has platform dependencies.
- Type 3 Driver (Network Protocol Driver): Here, JDBC calls are translated into a specific database protocol and sent over the network to the server. This makes it quite flexible as it doesnât tie directly to a database or OS.
- Type 4 Driver (Thin Driver): This pure Java driver directly converts JDBC calls into the network protocol used by DBMS, providing the best performance and portability since it doesnât require any local database libraries.
In summary, JDBC is not just an API; it's a comprehensive solution for enabling effective communication between Java applications and databases. By mastering its fundamentals, developers set a solid foundation for creating impactful software that interacts seamlessly with SQL Server.
Setting Up the Environment
Setting up the environment is a crucial step in establishing a connection between Java applications and SQL Server databases. This phase involves preparing your development setup to ensure smooth communication between the two systems. A well-configured environment reduces the chances of encountering issues down the road and enhances the overall efficiency of your database applications. By laying down a solid foundation, you ensure that your application can interact with the SQL Server effectively, setting the stage for further development.
Installing Java Development Kit
To kick things off, the first order of business is to install the Java Development Kit (JDK). The JDK provides the necessary tools for developing Java applications, including the Java Runtime Environment (JRE) required to run your programs. Without it, you wonât be able to get off the ground.
- Download the JDK: Visit the official Oracle website or OpenJDK to find the version that suits your operating system. Choose wisely according to your system architecture, whether youâre running a 64-bit or a 32-bit OS.
- Installation Process: Once downloaded, follow the installation prompts. For most cases, the default settings will work just fine. Just keep an eye out for the option to set the JDK path in your environment variables; this is pivotal for your applications to find Java.
- Verify Installation: You can confirm successful installation by opening a terminal or command prompt and typing . If the system responds with the installed version details, you can breathe easyâyou're set!
Downloading SQL Server JDBC Driver
Next up is getting the SQL Server JDBC Driver. This driver acts as the bridge between Java applications and SQL Server, translating Java calls into SQL Server database queries. Getting this right is essential for effective data communication.
- Where to Find the Driver: You can download the JDBC Driver from the Microsoft official website. Make sure you select the correct version compatible with your version of SQL Server.
- Installation: The JDBC driver typically comes in a ZIP file. After extracting its content, identify the relevant files that will be used in your project.
- Keep It Handy: Store the JDBC driver in a dedicated folder in a location you can easily access during configuration and programming.
Configuring the Classpath
The final setup step in establishing your environment is to configure the classpath. This is the path that tells the Java Compiler and Java Runtime where to look for user-defined classes and packages, including the JDBC driver.
- What You Need to Do: Open your systemâs environment variables settings. Add the path to the JDBC driverâs file to the CLASSPATH variable. Hereâs how:
- Double Check: After setting the classpath, itâs wise to verify that the path has been correctly added. You can do this by executing in the terminal.
- For Windows, you can do this by going to Control Panel -> System and Security -> System -> Advanced System Settings -> Environment Variables.
- For Linux or macOS, you can edit your or file. Add a line like .
Important Note: Always remember that without the correct classpath, your application will not be able to locate the JDBC driver, leading to frustrating runtime errors whenever you try to connect to the database.
By completing these foundational steps in setting up the environment, you pave the way for successful interactions between your Java application and SQL Serverâallowing you to dive deeper into fetching and manipulating data with far fewer hitches.
Establishing a Connection to SQL Server
Establishing a robust connection to SQL Server is a fundamental cornerstone for any Java developer looking to interact with databases. This connection acts as a bridge between the application and the database, enabling data exchange which is essential for tasks such as querying, updating, and managing data effectively. Without this connection, all efforts to manipulate data are in vain. A well-configured connection not only ensures the smooth functioning of applications but also enhances performance by reducing latency and errors.
Furthermore, having a firm grasp on connection management allows developers to optimize their code and utilize resources wisely. For instance, understanding how connection parameters are structured can lead to better security practices and more resilient applications.
"A chain is only as strong as its weakest link," rings especially true when it comes to database connections. Mutual understanding of the technical aspects fosters a stronger, more secure link between Java and SQL Server.
Connection URL Structure
The connection URL is a critical element in establishing the connection to SQL Server. It typically follows a specific syntax that includes essential information for the driver to connect to the database successfully. This URL informs the DriverManager how to locate the database and facilitates the creation of a connection.
The general format of a SQL Server connection URL is:
This format includes the server address, the port number through which communication occurs, and the name of the database that you wish to connect to. Each parameter in this URL serves a distinct purpose, intertwining to form the backbone of the database's reachability.
Configuring Connection Parameters
Database Name
Choosing the right database name is instrumental when connecting to SQL Server. The database name signals to the application which specific dataset it should interact with, encapsulating everything from usersâ data to application-specific records. A key characteristic of the database name is that it should be precise and without spaces or special characters, which could cause confusion during the connection process.
For this article, SQL Server databases are often popular due to their robust design and tools available for development. The unique feature of having strong ACID (Atomicity, Consistency, Isolation, Durability) compliance ensures data integrity, making it a reliable choice for applications that demand accuracy.
Advantages of properly setting a database name in your connection string include increased efficiency in queries and streamlined interactions with the database, ultimately reducing the chances of runtime errors.
User Credentials
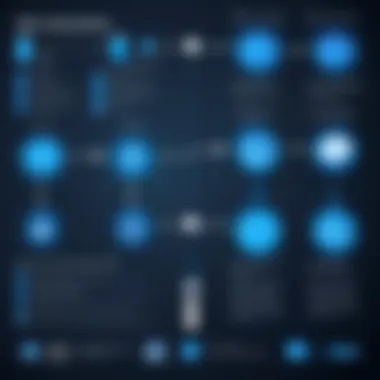
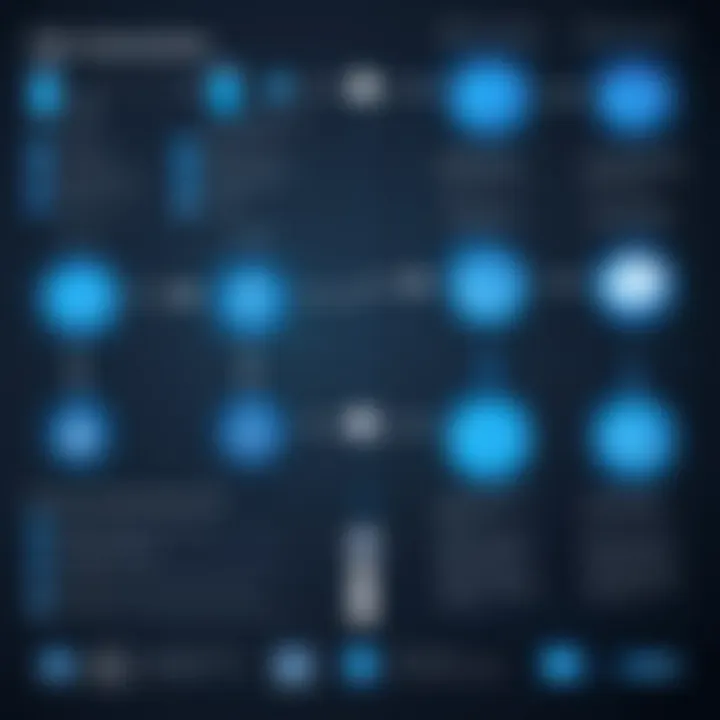
User credentials are fundamental for securing the connection between Java applications and SQL Server. Typically, these credentials are comprised of a username and a password that verify the identity of the user trying to access the database. A key feature of using user credentials is that it establishes a layer of security, preventing unauthorized access.
In this guide, emphasizing the use of unique and strong passwords is essential as it further fortifies the connection. The advantage here lies in allowing trusted users to perform necessary operations while safeguarding sensitive information against potential breaches.
Server Address
The server address indicates the location of the SQL Server instance and is a pivotal aspect of establishing a connection. This address can be an IP address or a domain name, and its configuration could directly impact the accessibility and performance of your applications. An important characteristic of the server address is ensuring its accuracy since any misrepresentation could result in an unreachable database.
In the context of SQL Server connections, the advantages of having a correctly configured server address include lower latency and faster query responses, ultimately leading to a more responsive application.
Port Number
The port number is another essential component in the connection string that allows your application to communicate with SQL Server. By default, SQL Server listens on port 1433 for incoming connections. This number must be configured correctly to ensure successful interaction with the database server. An important aspect of the port number is that it allows for multiple instances of SQL Server to run on the same machine, which can be especially useful in development or testing environments.
The unique feature of specifying a port number is that it can be reconfigured based on your network requirements; however, itâs crucial to ensure that the firewall rules permit access to the desired port. The advantage is clear: a correctly set port can lead to more stable connections and prevent potential bottlenecks during database access.
Utilizing DriverManager to Create Connection
DriverManager is a vital component of the JDBC API that is responsible for managing the database connections. It acts as a central point for establishing a connection with the SQL Server, enabling developers to obtain a connection object that they can subsequently use to execute statements and manage transactions.
To create a connection using DriverManager, the following code snippet outlines a simplified example:
In this example, the method is invoked with the connection string as an argument. The advantage of utilizing DriverManager lies in its ease of use and the ability to handle various JDBC drivers seamlessly. By managing interactions with the database driver, it abstracts much of the complexity developers would otherwise need to handle manually. This is incredibly beneficial for developers looking to focus more on application logic rather than connection details.
Executing SQL Queries
In the realm of database management, executing SQL queries stands as a pivotal element that bridges the gap between Java applications and SQL Server databases. This process enables developers to perform various operations on the data stored within the database. Whether you're fetching data, updating existing records, or managing database integrity, executing SQL queries is fundamental for effective application functionality. The ability to navigate this terrain not only enhances an applicationâs interactivity but also drives its performanceâa key consideration for any developer aiming for efficiency.
Creating a Statement Object
Before any SQL command can be executed, it's vital to create a Statement object. This object acts as a conduit through which SQL commands can be sent to the database. There are generally three types of Statement objects in JDBC: the Statement, PreparedStatement, and CallableStatement. Each serves a unique purpose and is optimized for particular use cases.
The basic Statement object is used for simple SQL queries without parameters, while the PreparedStatement is better suited for repeated execution of the same or similar SQL statements with varying inputs. This not only improves performance by pre-compiling the SQL but also guards against SQL injection, an ever-present threat in data handling. The CallableStatement, on the other hand, is designed for executing stored procedures, making it invaluable in complex database interactions.
Creating a Statement object is straightforward, involving a call from the object. For example:
Executing Different Types of Statements
Once a Statement object is in place, executing various kinds of SQL commands begins. Divided into several categories, the most commonly executed types of statements include SELECT, INSERT, UPDATE, and DELETE queries.
SELECT Queries
SELECT Queries are perhaps the most familiar territory for many developers working with databases. The primary role of a SELECT query is to retrieve data from the database. This command allows you to specify exactly which columns from what tables you are interested in, providing flexibility in data extraction.
One key characteristic of SELECT queries is their ability to filter results using conditions set by the WHERE clause. This allows fetching just the necessary data, thereby improving performance and usability. Additionally, SQL's inherent capability to join multiple tables expands the horizon of data retrieval, making it a popular choice for developers looking to analyze and present data meaningfully.
Yet, one must tread carefully when crafting SELECT statements, as complex queries can lead to longer execution times and increased resource usage if not optimized properly.
INSERT Queries
INSERT Queries focus on adding new records to tables in the database. The structure is rather simple: you define the table name along with the fields and corresponding values you wish to enter. This straightforward syntax highlights one of its key benefitsâthe ease of use. Beginners often find their way into database management through these queries, as they relate directly to the concept of data entry.
However, thereâs a caveat. While inserting data is crucial, poorly structured INSERT statements can introduce anomalies or violate database constraints, leading to errors that can disrupt application flow. Therefore, it's essential to be mindful when constructing and executing these queries.
UPDATE Queries
UPDATE Queries allow for existing records within a database to be modified. This capability means developers can adjust data in response to real-world changes, ensuring the accuracy of stored information. By specifying which rows to update through the WHERE clause, you maintain control over changes and minimize the risk of unexpected data loss.
A significant aspect of UPDATE Queries is their ability to operate in bulk. With a single statement, you can adjust multiple records, which can save both time and system resources. However, as with SELECT and INSERT, clarity in specifying conditions is criticalâoverly broad updates can lead to unintended data alterations.
DELETE Queries
DELETE Queries serve to remove records from tables, clearing out unnecessary or outdated data and keeping the database clean. The basic structure of a DELETE command allows developers to specify criteria under which records must be deleted, echoing the importance of precise conditions.
The critical characteristic of DELETE Queries is the potential for data loss if not executed with care. Losing crucial data in an application can lead to detrimental consequences. Therefore, implementing safeguards, such as backup processes and user confirmations, can be wise practices when performing deletions.
In summary, executing SQL queries in a Java-SQL Server environment is not just about managing data but also about ensuring that your application interacts efficiently and effectively with the database. By mastering the various types of statements available, developers enhance not just their skill set but also the overall robustness of the applications they build.
Handling Errors and Exceptions
When working with Java-SQL Server connections, the road is not always smooth sailing. Handling errors and exceptions effectively can be the difference between a minor hiccup and a major roadblock in your application. Understanding how to catch and process these issues can enhance the overall robustness of your database interactions. This section discusses the potential errors one might encounter, the specifics of various SQL exceptions, and the best practices in error handling that can keep your application ever functional even in the face of adversity.
Common SQL Exceptions
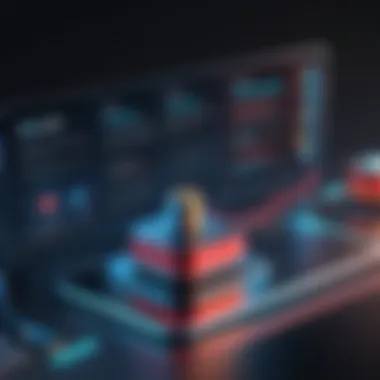
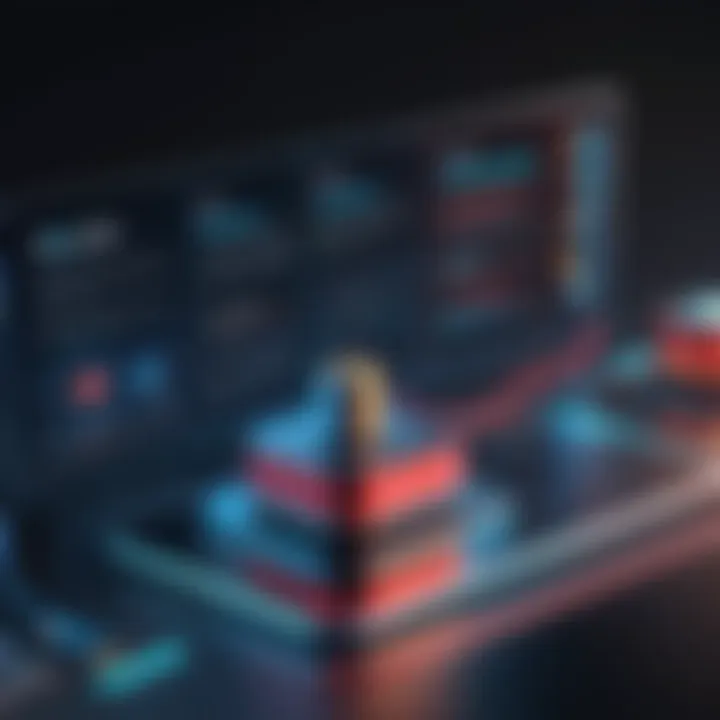
In the realm of SQL, a variety of exceptions can arise, often indicating underlying problems that need to be addressed. Here are some of the more frequent ones:
- SQLException: This is the most generic exception that signals issues with SQL operations. It might occur due to syntax errors or a failure to connect to the database.
- SQLTimeoutException: When a database operation takes longer than a predefined limit, this exception kicks in. It serves to alert developers that the connection is sluggish or unresponsive, which may impact the user experience.
- SQLIntegrityConstraintViolationException: Trying to enter duplicate data into a database that is supposed to maintain unique entries? This exception shows up to notify developers of constraint violations, such as primary or foreign key issues.
- SQLSyntaxErrorException: A typo in your SQL query or a wrong command can trigger this. It's a straightforward exception that directly correlates with errors in SQL syntax.
Best Practices for Error Handling
Dealing with errors is not merely about capturing them; itâs also about how to respond. Implementing effective error handling practices can significantly enhance the overall performance and reliability of your Java application. Here are some best practices:
- Use Specific Exceptions: Rather than catching the generic , try to catch specific types where possible. This allows for more tailored handling for each case.
- Logging: Instead of silently ignoring errors or letting them bubble up, log them! Using a consistent logging framework, such as Log4j or SLF4J, helps in tracking problems down and fixing them efficiently.
- Graceful Degradation: Make sure your application degrades gracefully when facing an error. Rather than crashing, show a friendly message to users or redirect them, ensuring a decent user experience.
- Retries: Sometimes, operations may fail due to temporary conditions. Implementing a retry mechanism for transient failures can be invaluable in maintaining user satisfaction.
- Finally Block: Always close connections in a block or utilize try-with-resources to ensure that resources are released appropriately, irrespective of whether an error occurred.
Remember: Handling exceptions wisely can turn potential disasters into mere bumps on the road. It's not just about preventing errors; it's also about how you bounce back from them, giving your application a remarkable resilience.
Closing the Connection
Establishing a connection to a SQL Server database from a Java application is only half the battle. Once the data exchange is complete, the importance of carefully closing that connection cannot be overstated. Just think of it as turning off the tap after you've filled a bucket; it keeps things tidy and ensures nothing goes to waste. The need for closing connections falls into various critical categories, each with its own implications for performance and resource management.
Importance of Connection Closure
Closing database connections minimizes the risk of resource leaks in your application. When a connection is not closed, it remains active, consuming valuable memory and other resources, which could lead to performance bottlenecks. Moreover, excessive open connections may hit the connection pool limit, resulting in failures when new connection requests arrive. This is particularly crucial in a web environment, where multiple users might be attempting to access the database simultaneously.
A best practice often discussed but sometimes ignored is that every opened connection should be closed in finally blocks or using try-with-resources statements. This ensures that the connections are closed even when exceptions occur, which helps maintain the integrity of your application while also providing a cleaner user experience.
Methods to Close Connections
There are a few ways to effectively close connections in Java. Below are some common methods:
- Using : The simplest way to close a connection is by calling the method on your Connection object. Hereâs typically how it looks in code:
- Try-with-Resources: Introduced in Java 7, this feature automatically closes resources when they are no longer needed. This is especially handy for managing connections and gives cleaner code:
Using these methods not only aids in maintaining resource integrity but also results in cleaner code, which is easier to read and maintain. Don't forget! Each connection, statement, and result set should be closed to ensure that resources donât pile up and cause issues in production environments.
A little diligence here goes a long way.
Performance Considerations
When establishing connections between Java applications and SQL Server, performance considerations play a key role in ensuring efficient operations and responsiveness. The essence of performance in this context isnât just about speed; it's about maximizing resource utilization while minimizing latency. This section digs into two pivotal aspects: connection pooling and minimizing resource usage.
Connection Pooling
Connection pooling is a vital technique that significantly enhances performance when dealing with database connections. Imagine the time it would take to repeatedly establish and dismantle connections for each queryâyou could be looking at a bottle neck that slows down your application. By using connection pooling, you essentially create a set of established connections that can be reused, like having a handy toolbox rather than having to go out and buy new tools each time you need to fix something.
- Benefits of Connection Pooling:
- Reduced Connection Overhead: New connections can create considerable overhead both in terms of time and resources. Connection pooling mitigates this by allowing reusable connections.
- Improved Response Time: Since the connections are pre-established, your application can significantly speed up query execution.
- Resource Management: Connection pools help in managing resources better by limiting the number of connections created to what is necessary.
Implementing connection pooling in Java can be done using various methods, such as using libraries like HikariCP or Apache DBCP. Hereâs a small snippet using HikariCP:
Implementing this smartly can lead your application to scale far better even under heavy loads.
Minimizing Resource Usage
Minimizing resource usage is another cornerstone of maintaining optimal performance when connecting Java applications to SQL Server. It's not just about using fewer resources; itâs about using them wisely. Inefficient use of database resources can lead to slow performance and increased costs.
- Key Strategies for Minimizing Resource Usage:
- Efficient Queries: Ensure that your SQL queries are optimized. Avoid SELECT * when you only need specific columns. This reduces the amount of data processed and transferred.
- Using Prepared Statements: Prepared statements not only enhance performance due to their pre-compiled nature, but they also reduce the load on the database server since the same query can be reused.
- Batch Processing: When executing multiple operations, consider batch processing to reduce round trips to the SQL server. This batch technique allows you to send a group of statements in one go.
"Efficiency is doing things right; effectiveness is doing the right things."
-- Peter Drucker
In sum, performance considerations, particularly around connection pooling and resource usage, are critical to the seamless function of Java applications interacting with SQL Server. Making informed decisions about these elements can lead to robust applications that provide a smooth experience even under heavy loads.
Epilogue
When it comes to integrating Java applications with SQL Server, the conclusion is where all the threads come together. Here, we reflect on the journey of establishing a robust connection and highlight why each piece of knowledge is crucial.
First off, being able to successfully connect Java with SQL Server is not just a skill but a necessity for any serious developer. It allows for leveraging the full potential of data management and retrieval in applications. If you think about it, databases are like the backbone of modern software; they store everything from user data to transaction logs. Without a solid connection, this backbone crumbles.
Summarizing Key Takeaways
In summarizing our discussion, here are some key takeaways that stand out:
- Understanding JDBC: Familiarity with JDBC and its components is fundamental. It sets the stage for all subsequent operations, from establishing connections to executing queries.
- Connection Parameters: Knowing how to configure your connection parameters, including user credentials, server address, and port, is a must. This ensures that there are no hiccups when you try to reach the SQL Server.
- Error Handling: Implementing best practices for error handling can save a lot of headaches. Knowing how to navigate common SQL exceptions can enhance the user experience significantly.
- Closing Connections: Itâs often the little things that count, and closing your database connections properly can lead to improved resource management and performance.
- Performance Optimizations: Utilizing connection pooling and minimizing resource usage are critical in high-load environments. These strategies can differentiate a slow application from a fast, responsive one.
Future Learning Directions
Finally, as we wrap things up, there are many avenues for furthering your understanding of Java and SQL Server connections:
- Explore Advanced JDBC Features: Delve into the advanced functionalities of JDBC, such as batch processing and transaction management. This will give you a more nuanced approach when dealing with complex applications.
- Learn about ORM Frameworks: Understanding Object-Relational Mapping frameworks like Hibernate can simplify database interactions and increase productivity by reducing boilerplate code.
- Stay Updated with SQL Server Enhancements: Databases evolve, and with that, SQL Server frequently rolls out new features. Keeping abreast of these changes can offer you leveraging points for better performance and capabilities.
- Join Developer Communities: Participate in communities on platforms such as Reddit and Facebook. Real-world problems and solutions shared there can expand your understanding and expose you to best practices.