Mastering Java and Selenium for Automated Testing
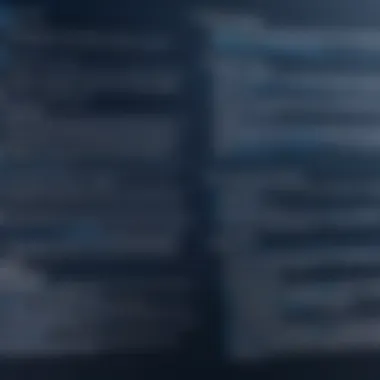
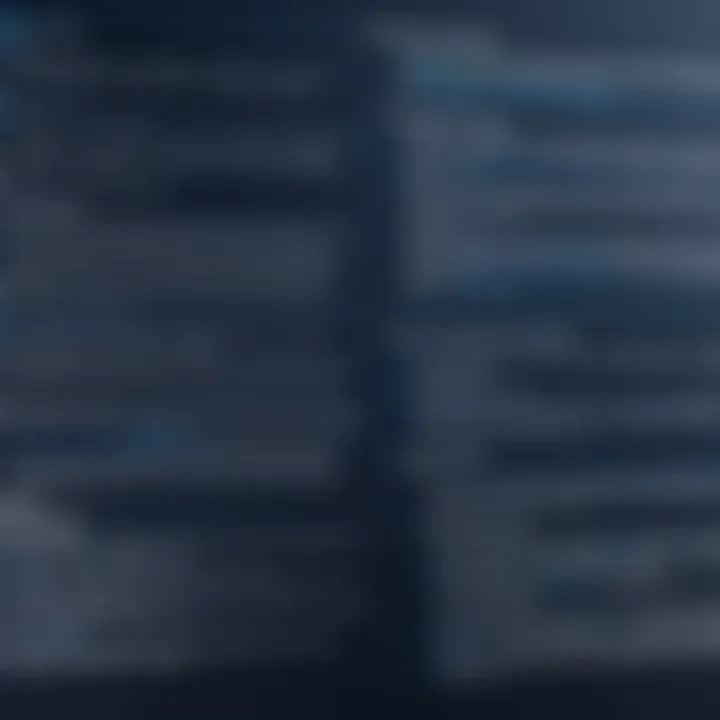
Prologue to Programming Language
Programming languages serve as the backbone of software development, acting as bridges between humans and machines. Among these, Java has stood the test of time, proving its mettle as a reliable and versatile language.
History and Background
Java made its debut in the mid-1990s, developed by Sun Microsystems, which is now part of Oracle Corporation. Initially meant for developing interactive television applications, it quickly evolved into a general-purpose programming language. The beauty of Java lies in its portability across platforms, thanks to the now-famous slogan, "Write Once, Run Anywhere." As the years progressed, Java solidified its position in the tech world, playing a pivotal role in web application development and enterprise environments.
Features and Uses
Java comes packed with features that attract both novice and experienced developers. Here are some key characteristics:
- Object-Oriented: Promotes code reusability and modularity through concepts like classes and inheritance.
- Platform-Independent: The Java Virtual Machine enables code execution on any device equipped with it.
- Multi-threaded: Java supports high-performance applications through concurrent thread execution.
- Robust and Secure: With strong memory management and a variety of built-in security features, it minimizes risks significantly.
These features make Java a preferred choice for building Android apps, web applications, and large-scale enterprise systems, among others.
Popularity and Scope
Java's popularity is evident in its extensive community and rich ecosystem of libraries and frameworks. According to various developer surveys, it's consistently ranked among the top programming languages, often leading the pack in enterprise environments. The languageâs vast reach can be attributed to its robust nature, making it suitable for everything from server-side applications to cloud computing and Internet of Things (IoT) devices.
Now, as we pivot towards Selenium, its integration with Java opens new doors for automated testing, amplifying the capabilities of Java when it comes to ensuring software quality.
The Significance of Selenium for Java Users
Selenium is a powerful framework for automating web applications, allowing developers to test their systems' functionalities across multiple browsers and platforms. By combining Selenium with Java, programmers gain a formidable toolkit that enhances testing efficiency and effectiveness. As software testing increasingly shifts towards automation, understanding this interconnection is crucial.
"The combination of Java and Selenium is like a well-oiled machine, ensuring that your applications run smoothly and perform reliably."
As you navigate through this article, expect to explore practical examples, nuanced details, and effective practices to harness the power of Java with Selenium in automated testing scenarios.
Preamble to Java and Selenium
When we step into the realm of software testing, the combination of Java and Selenium stands out as a powerful duo. This cooperation not only enhances the efficiency of automated testing but also provides a robust framework for developers and QA engineers alike. As technology helps businesses to cut costs and enhance product quality, understanding this relationship becomes imperative.
Java, a stalwart in programming languages, offers stability and cross-platform capabilities, making it a preferred choice for writing test scripts. On the other hand, Selenium serves as the testing framework that drives those scripts, allowing them to interact with web applications seamlessly. Together, they facilitate a smoother testing process, which is vital in todayâs fast-paced development landscape.
Prior to delving deeper, grasping the core benefits of using Java with Selenium proves beneficial.
- Efficiency: Automated testing reduces the time it takes to run tests, catching bugs early in development.
- Flexibility: Developers have the freedom to conduct extensive testing across various platforms and browsers.
- Scalability: The robust architecture of both Java and Selenium allows for scalable automated testing solutions as projects grow.
In this section, we will lay the groundwork by exploring both Java and Selenium in greater detail, setting the stage for a comprehensive examination of their integrated functionalities.
Overview of Java
Java is often regarded as the backbone of many large systems. It is an object-oriented programming language that prioritizes code reusability and maintainability. The essence of Java's appeal lies in its ability to run on any device that supports the Java Virtual Machine (JVM), hence the mantra "write once, run anywhere." This attribute makes it an ideal choice for developing automated test scripts that need to function across various environments.
Understanding the basic syntax of Java is the first step toward grasping its capabilities in automated testing. The language features a simple yet powerful set of commands which, combined with its extensive libraries, empower developers to perform complex operations with relative ease. Additionally, Java's long-standing presence means that a wealth of resources and community support exists, aiding newcomers as they learn the ropes.
Understanding Selenium
Selenium, as a web automation tool, plays a significant role in testing web applications. It acts as a bridge that allows developers to write automated tests to mimic user interactions in a browser environment. With its various components, Selenium supports testing across multiple browsers, offering flexibility that is invaluable for modern web applications.
The architecture of Selenium consists of a few key components:
- Selenium WebDriver: Facilitates the execution of test scripts in different browsers.
- Selenium IDE: A more user-friendly tool that allows for the recording and playback of tests.
- Selenium Grid: Enables parallel testing on different machines, thus saving time and maximizing testing efficiency.
Selenium operates seamlessly with Java, providing a powerful toolset for performing comprehensive testing of web applications. The synergy between these two technologies allows testers not just to run simple tests but also to target intricate scenarios that require more dynamic interactions.
Thus, this section paints a broad picture of Java and Selenium, illustrating their foundational aspects and emphasizing their relevance in the landscape of automated testing.
Setting Up Your Environment
Setting up your environment is absolutely crucial in the journey towards mastering Java and Selenium. Without a proper configuration, the efforts you put into learning and coding can end up in a tangle. Think of this as laying the foundation before constructing a house; if your base is shaky, everything built atop it is bound to crumble sooner or later. Choosing the right tools can streamline your workflow, minimize headaches down the road, and ensure a more smooth experience.
Installing Java Development Kit
To begin, acquiring the Java Development Kit (JDK) is the first step toward automating your tests with Selenium. The JDK is the heart of Java programming; it contains all the libraries, tools, and binaries needed to compile and execute Java programs. Without it, you might as well be trying to cook without any utensils.
- Downloading JDK: Head over to the official Oracle website or OpenJDK to grab the latest version. You can expect an installation size around a few hundred megabytes, which is small compared to the capabilities it brings.
- Installation Process:
Once downloaded, run the installation file. Follow the prompts in the setup wizard, but pay attention to the installation path. Setting environment variables, like your , can save you some headaches later. - Verification:
After installation, to make sure everything's running smooth, open your command line (or terminal) and type:If it returns the installed version, congrats! You're on the right track.
Configuring Your IDE for Java
Your Integrated Development Environment (IDE) is your command center; think of it as the cockpit of an airplane. Choosing the right one can make all the difference while coding. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans.
- Download and Install:
Each IDE has its unique installation steps but usually involves a simple download and executing a setup wizard. It's intuitive enough, you wonât need a walkthrough here. - Setting Up the IDE:
- Plugins and Extensions: If you want to elevate your coding experience, consider installing plugins that offer templates or code assistance, such as those that help with debugging or improve readability.
- Project Structure: Create a new project and set it to use the correct JDK.
- Defining Your Build Path: Adding necessary libraries is essential. This includes your Selenium WebDriver jar files, which you can download from the Selenium official website.
To add the jar files in IntelliJ, go to Project Structure -> Libraries -> Add.
Getting Started with Selenium WebDriver
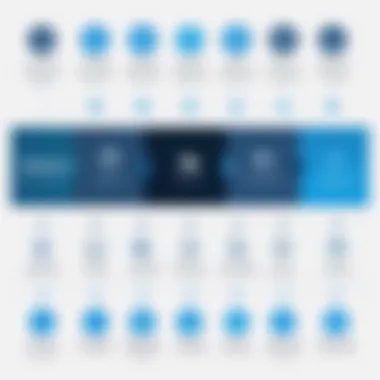
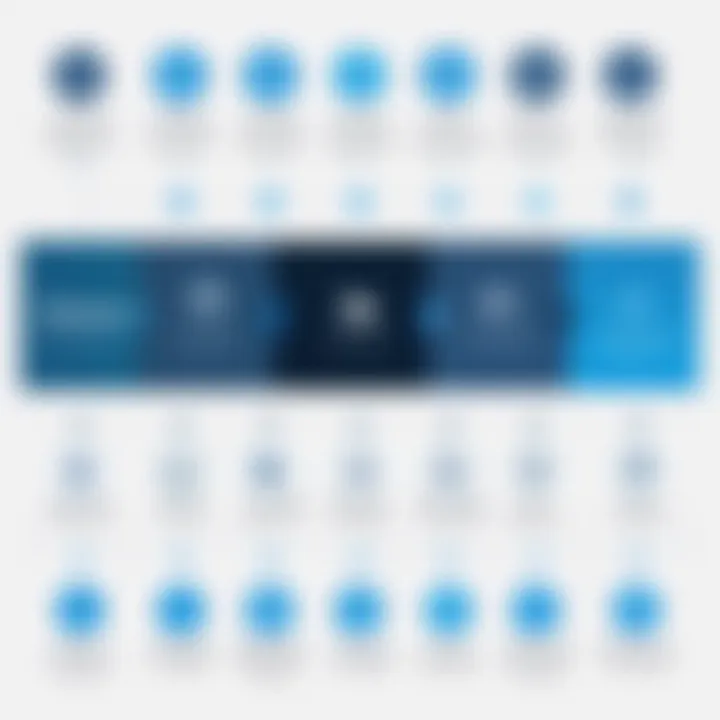
Now that your development environment shines, itâs time to kick off with Selenium WebDriver. This powerful tool enables you to automate browser actions, making it possible to write tests simulating user interactions.
- Download Selenium WebDriver: Head to the Selenium official website where you can find the WebDriver compatible with your preferred browser. Google Chrome users should look for the ChromeDriver, and Firefox users will want the GeckoDriver.
- Add WebDriver to Your Project:
Include these WebDriver binaries in your project's build path, similar to how you did with the JDK. This step ensures that the Selenium scripts can call the appropriate browser drivers when executing tests. - Writing Your First Test:
Broswing through a webpage is as simple as writing some lines of code. A basic setup may look like this:This snippet sets everything up, launches a browser, and navigates to a website. It doesn't get much easier than this!
Setting up your environment might seem daunting at first, but once everything is in place, you'll find yourself speeding through your automation tasks. So, roll up your sleeves and get ready for the ride!
Core Concepts of Java
Understanding the core concepts of Java begins with acknowledging its significance not only as a language but also as a fundamental tool for automation testing through frameworks like Selenium. These foundational principles set the stage for writing efficient code, creating robust applications, and ultimately ensuring quality in software development. When youâre diving into Java for automated testing, grasping these concepts will be crucial. They guide the structure and flow of your code, making it easier to understand and maintain over time.
Basic Syntax and Structure
Basic syntax in Java is akin to the grammar of a language; it forms the bedrock of writing any program. Knowing the syntax rules is important so that your code conveys the right meaning to the Java compiler. Java uses a series of punctuation marks, keywords, and data types to create coherent statements.
Key Features of Java Syntax:
- Case Sensitivity: Java is case sensitive. For instance, 'MyVariable' is different from 'myvariable'.
- Class-Based Structure: Every piece of code must exist within a class, which organizes your methods and variables within that class.
- Semicolons: Unlike Python, every statement in Java ends with a semicolon, marking the end of that instruction.
The focus on syntax promotes a clean structure in program writing. Consequently, as you embark upon automated testing with Selenium, familiarizing yourself with the syntax enables you to create tests without ambiguity, reducing the room for errors.
Object-Oriented Principles
Incorporating object-oriented programming (OOP) principles in Java bolsters your ability to create reusable and flexible code. This approach resonates well with automated testing in Selenium, simplifying complex test scenarios and facilitating easier maintenance.
Encapsulation
Encapsulation is the practice of wrapping data and methods into a single unit, typically known as a class. This principle guards the internal state of an object from outside interference and misuse. It offers data hiding, which is beneficial for building secure applications.
- Key Characteristics: Public and private access modifiers control visibility, enhancing security.
- Benefits in Testing: For Selenium testing scripts, encapsulating test data and functions minimizes side effects and focuses on particular test cases, leading to clearer, more manageable scripts.
- Unique Feature: Being able to define properties with getter and setter methods gives you fine control over your data while preventing unwanted changes.
Inheritance
Inheritance allows one class to inherit the properties and methods of another. This is particularly useful for avoiding redundancy in scripting. It enables testers to create a base test class with common functionality so that all derived classes can access these features.
- Key Characteristics: Supports a parent-child relationship between classes, allowing reuse of code.
- Benefits in Testing: This simplifies code maintenance. If a base test class needs an update, you only change it once, and all derived classes automatically get the new functionality.
- Unique Feature: Polymorphism functions alongside inheritance, enabling methods to perform differently based on the object calling them, increasing flexibility in test designs.
Polymorphism
Polymorphism refers to the ability of an object to take on many forms. This is particularly valuable in testing frameworks where method behaviors may vary depending on the object invoked.
- Key Characteristics: Achieved through method overloading and method overriding.
- Benefits in Testing: In automated tests, you can design one interface and let various test cases implement the same functionality differently. This promotes adaptability in handling changes in test requirements.
- Unique Feature: It enhances code readability and reduces the complexity involved in maintaining multiple methods for various classes.
Collections Framework
The Collections Framework in Java provides a set of classes and interfaces that help manage groups of objects effectively. This becomes essential when dealing with test data in Selenium.
- Types of Collections: Lists for ordered elements, sets for unique values, and maps for key-value pairs.
- Benefits: It allows testers to manipulate data stored in collections easilyâfor instance, easily iterating through test cases or configurations without writing cumbersome loops.
The Collections Framework streamlines data manipulation in your testing scripts, so understanding it well will greatly assist you in automating tests efficiently. Every time you iterate through test results or modify input data for your Selenium script, youâll be grateful for this structured approach.
By diving deeper into Javaâs core concepts, you establish a solid foundation that will empower your testing abilities using Selenium. This foundational knowledge is critical for writing effective and reliable tests that stand the test of time.
Selenium Architecture
Understanding the architecture of Selenium is like getting the lay of the land before embarking on a trek through the wilderness of automated testing. Selenium isn't just a tool; it's a robust framework driven by multiple components that work in harmony to facilitate smooth interactions between tests and web applications. In this section, weâll navigate through the various components that constitute Selenium's architecture and examine their roles, benefits, and relevance in automated testing.
Understanding Selenium Components
Selenium WebDriver
Selenium WebDriver stands out as a primary driver behind automated web testing. It acts as an interface that allows users to communicate with web browsers in order to manipulate them programmatically. What makes WebDriver particularly notable is its capability to replicate user actions, making it a favorite among testers. With a distinct focus on real user behavior, it allows for interaction with JavaScript-heavy applications, which are becoming the norm in todayâs web landscape.
A key characteristic of Selenium WebDriver is its direct communication with the browser. Unlike previous iterations, such as Selenium RC, WebDriver uses the browserâs native support to automate tasks. This not only enhances performance but also increases reliability, ultimately leading to more robust test results. A unique advantage is its ability to execute tests in parallel across different browsers and platforms, which is a massive time-saver.
However, there are some disadvantages. For instance, setting up the testing environment can be somewhat tricky for beginners as it involves proper configuration of drivers specific to each browser. The nuance of exception handling and synchronization can be steep learning curves for new users.
Selenium IDE
Selenium Integrated Development Environment (IDE) is a valuable tool within the Selenium suite, designed primarily for those who are at the beginning of their automated testing journey. Its key characteristic is that it allows testers to record and playback tests without the need for any coding knowledge. This makes it an excellent choice for non-technical users wanting to dip their toes into automation.
A unique feature of Selenium IDE is its straightforward user interface, which allows users to easily create test scripts by simply clicking through a web application. This ease of use can be quite advantageous, especially when quick feedback is needed for UI testing. However, itâs important to note that while Selenium IDE is great for quick tests, it doesn't offer the flexibility or power that WebDriver provides for more complex scenarios.
Some might say itâs a double-edged sword; while it allows for rapid test development, the lack of detailed control means itâs not suited for advanced testing strategies or handling dynamic elements well.
Selenium Grid
Selenium Grid is the horsepower behind executing tests across multiple environments simultaneously. It's particularly useful for teams looking to ensure their applications work consistently across different browsers and devices. This capability addresses a key concern in todayâs development landscape â cross-browser compatibility.
One of the standout features of Selenium Grid is its ability to facilitate parallel execution. Test cases can be distributed across several machines, significantly speeding up the testing process. This characteristic makes it a beneficial choice for large projects with extensive test suites.
Yet, as with any tool, it's not without its challenges. Setting up a Selenium Grid requires a good grasp of network configurations and potential issues such as resource management. Teams might find it daunting at first, especially if they boast a diverse range of browsers and operating systems.
How Selenium WebDriver Works with Java
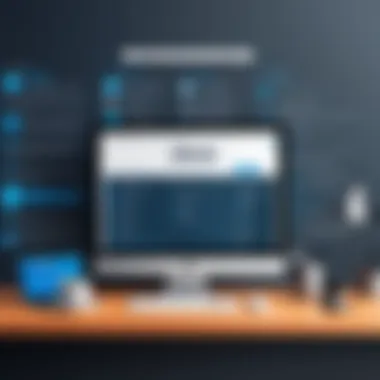
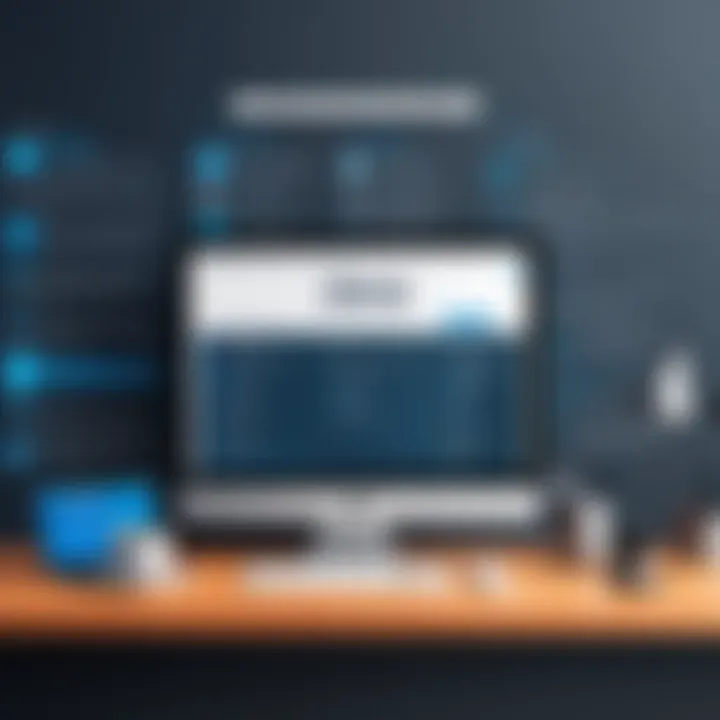
Now that we have dissected the components, understanding how Selenium WebDriver works in conjunction with Java is crucial. By harnessing Java's capabilities, such as its extensive libraries and support for object-oriented principles, Selenium tests can be turned into powerful applications. Java provides the framework necessary for error handling, data-driven testing, and setting up testing environments that are easily integrated with build and deployment tools.
As you start writing tests in Java, utilizing the WebDriver API becomes second nature. Integers, strings, and lists morph into powerful classes and objects that you employ within your tests. This synergy between Java and Selenium opens the door to a plethora of advanced testing scenarios, paving the way for a robust testing infrastructure.
Writing Your First Test
Writing your first test with Selenium and Java represents a crucial step in the journey of automated testing. Itâs not just about typing out code or executing scripts; it embodies an understanding of how these components function together to create reliable, repeatable tests. This experience helps to solidify both your Java programming skills and your comprehension of Seleniumâs capabilities. When you're confident in writing a basic test, it lays the groundwork for more complex scenarios in testing environments.
Equipped with the knowledge from prior sections, youâre now ready to take the plunge into practical applications. The benefit of writing your first test is twofold: it increases your technical skills, and it builds your problem-solving capabilities. As you write tests, you might encounter issues that push you to think critically and find solutions. With Java's object-oriented approach and Seleniumâs flexibility, the possibilities for future tests are exciting.
"The first step in testing automation is like learning to ride a bike: you may stumble at first, but once you find your balance, it opens up new avenues of speed and efficiency."
Creating a Simple Selenium Test in Java
Embarking on your first test in Java using Selenium doesnât have to feel like venturing into the unknown. It can be uncomplicated if you follow the right steps. A simple test generally includes:
- Setting Up WebDriver: The Selenium WebDriver acts as a bridge between your Java code and the browser. Make sure to import the necessary packages.
- Launching the Browser: This is where the magic begins. Initiate the desired browserâlet's say Chromeâand navigate to a webpage you want to test.
- Interacting with Web Elements: Use Selenium's methods to find elements on the webpage. You can locate elements using different strategies such as by ID, name, or CSS selector.
- Performing Assertions: Check whether the expected outcome matches the actual outcome to determine if the test passes or fails.
Here's how a basic test can look in Java:
This snippet covers the essentials of starting your testing journey. Make sure to adjust the path for your ChromeDriver installation. Running this code will open a Chrome window, navigate to the predefined URL, and check the title against what's expected.
Analyzing Test Results
Analyzing the results of your test is just as significant as writing it. This step enhances your understanding of how effective your tests are and helps you make necessary adjustments for improvement. When the test has completed its run, you should delve into the outcomes meticulously.
To analyze test results, consider the following:
- Compare Expected and Actual Results: Identify any discrepancies. If the test fails, dig deeper into the code to understand what went wrong and whether it is due to a bug in the application or an error in your test logic.
- Review Logs: Often, Selenium WebDriver will provide logs that denote the actions executed, helping pinpoint failures.
- Refactor Tests: Regularly revisiting tests is prudent. If certain elements or functionalities are consistently failing, that may indicate a need for adjustments in your approach.
Key Points of Analyzing
- Keep an open mind towards failure; itâs a teacher.
- Document findings from each test run.
- Establish a feedback loop to enhance test design.
By wrapping your head around this critical phase, you set the stage for better-designed tests, creating a solid foundation for future automation endeavors. Learning to extract value from your test results will foster a testing culture that emphasizes quality and reliability in software development.
Advanced Selenium Features
Exploring advanced features of Selenium is like diving into a treasure chest for any software tester or developer. These features not only enhance the testing process but also streamline it, making complex tasks more manageable. Understanding these advanced functionalities is integral to mastering Selenium and effectively harnessing its full potential. Let's dig into the specifics of the advanced Selenium elements to shed light on their importance and use cases.
Handling Dynamic Web Elements
One of the paramount challenges in automation testing is dealing with dynamic web elements. These elements appear or change state during the test's execution, often in ways not apparent at the moment of script writing. Consider a dropdown that only shows options after a user clicks on it. Not addressing these dynamic elements can lead to flaky or failed tests, which might frustrate testers and derail software release schedules.
To handle these dynamic elements adequately, one can employ several strategies:
- Explicit Waits: Using Selenium's WebDriverWait class allows testers to pause the execution until a particular condition is met, making it easier to interact with elements that take time to appear.
- Fluent Waits: This strategy provides more flexibility than explicit waits, allowing for a customizable polling interval and the ability to ignore specific exceptions.
Utilizing these waits provides a more reliable test execution.
This snippet shows how to wait for an element's visibility before interacting with it, significantly reducing the risk of timing-related errors.
Managing Timeouts and Waits
Timeout management is another critical aspect when working with Selenium. The speed of web applications can vary broadly, and tests need to be resilient enough to handle those variations. Improperly set timeouts may lead to tests failing when they simply need more time to complete their execution due to slower response from server or application load.
Two main types of timeouts should be configured in Selenium:
- Page Load Timeout: This timeout applies to the time it takes for a page to load fully.
- Implicit Wait: This timeout sets a default waiting time for the WebDriver to poll the DOM for elements.
Along with these, balancing the waits is essential to create a seamless user experience during testing. Overusing waits might slow down tests excessively, while underusing them might lead to missed interactions.
Using Page Object Model for Test Design
The Page Object Model (POM) is a design pattern that enhances test automation by separating the representation of web pages from test scripts. This method not only clarifies code organization but also promotes reusability and maintenance, which are vital as applications evolve.
Under POM, each page in the application is represented by a separate class. This structure allows developers to encapsulate the page's elements and actions into the respective class, promoting modularity. Some benefits of using POM are:
- Ease of Maintenance: If elements change on a page, testers only need to update that specific page object, instead of each test case that interacts with it.
- Improved Readability: Tests become cleaner and easier to understand since actions on the page are abstracted away into methods within the page classes.
For implementation, a typical page class might look like this:
With this class, a test case can become much more readable:
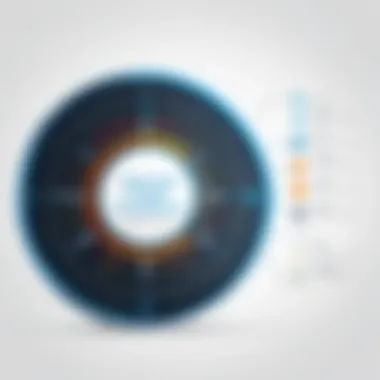
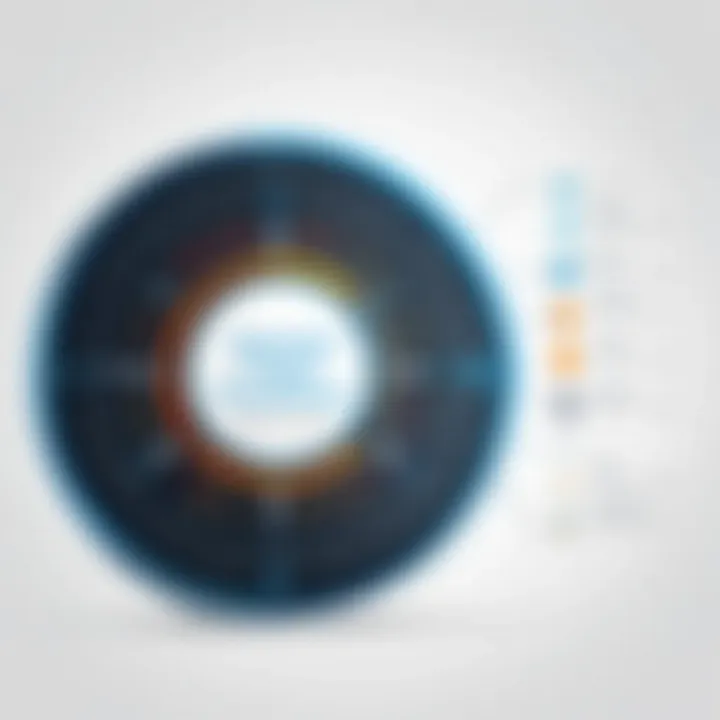
By adopting this model, tests become less tangled and easier to comprehend, enabling quicker adaptation to changes in testing requirements or application structure.
"Using advanced features in Selenium, like dynamic element handling and the Page Object Model, transforms how we approach automated testing, paving the way for smoother and more efficient workflows."
As we enhance our practice with these advanced features, we can not only improve the overall integrity of our testing process but also position ourselves at the forefront of automated testing approaches.
Best Practices in Automated Testing
Automated testing has become an essential component in the software development lifecycle. When integrating Java and Selenium, adhering to best practices not only enhances the quality of your tests but also streamlines the entire testing process. These practices help in minimizing errors, increasing code reliability, and ensuring that tests are maintainable and scalable over time.
When discussing best practices, it's essential to shift the focus on the underlying principles that guide the testing process. For instance, structuring tests properly, maintaining clean test scripts, and integrating with continuous integration systems play foundational roles in achieving effective automated testing. These areas not only improve workflow but also allow for easy troubleshooting and faster iterations in response to feedback.
"Well-structured tests serve as documentation for the code they validate, effectively communicating your intentions to others."
Structuring Your Tests
The structure of your test cases significantly affects your ability to maintain and expand your test suite over time. A logical organization allows both new and experienced developers to navigate the tests effectively. To achieve this, consider the following:
- Use of Naming Conventions: Clear and descriptive names for your test methods and classes can serve as self-documentation. Avoid vague titles like or . For example, name a test for checking user login as .
- Test Independence: Structure each test to be independent of others. This means it should set up its environment and state. This prevents failures in one test from cascading into another, ensuring that tests can be run in isolation.
- Grouping Related Tests: Use test suites to group similar tests together. This can improve clarity and organization, especially when tests share the same setup or teardown logic.
By focusing on these elements, you create a more robust foundation for your automated tests, making it easier to manage and adapt as your project evolves.
Maintaining Test Scripts
Maintaining test scripts is often overlooked, yet it is critical for the longevity and effectiveness of your testing efforts. As changes occur in the application, test scripts should be updated collaboratively. Key points to consider include:
- Regular Refactoring: Periodically review and refactor your test scripts. Like any code, they can become messy as features evolve. Implementing changes like removing redundant steps or reorganizing logic can enhance readability and performance.
- Version Control: Utilize version control systems like Git to track changes to your test scripts. This way, you can revert to previous versions if new tests introduce issues.
- Documentation and Comments: While the code should generally be self-explanatory, situations will arise where additional comments are beneficial. Use them to clarify complex tests or important decisions made during the scripting process.
By putting in the effort to maintain your test scripts, youâll save yourself headaches and time down the line.
Integrating with Continuous Integration Systems
Utilizing Continuous Integration (CI) systems is a game changer in automated testing. Integration helps catch bugs earlier in the development cycle, reducing overall costs and enhancing the product quality. Hereâs how to effectively utilize CI environments:
- Automated Test Execution: Set up your CI environment to automatically run tests on new commits. This ensures that any newly introduced bugs are quickly identified.
- Build Feedback Loops: Configure your CI system to provide immediate feedback on builds and test outcomes. Teams can respond to failures promptly, avoiding the accumulation of errors that often occurs in longer feedback loops.
- Reporting and Metrics Tracking: Integrate reporting tools with your CI pipeline. Capturing metrics on test execution times and failure rates can offer valuable insights for improving your test suite.
Incorporating these practices into your testing strategy should bolster your projectsâ resilience and efficiency in ways that are unmistakable. Engaging with Java and Selenium, while adhering to these guidelines, can elevate the quality and consistency of automated testing greatly.
Debugging and Troubleshooting
Debugging and troubleshooting play a critical role in the journey of anyone working with Java and Selenium. When dealing with automated tests, itâs not just about writing scripts; it's about ensuring those scripts run smoothly and deliver the intended results. The importance of debugging lies in its potential to pinpoint errors that could render tests useless or, worse, lead to missed defects in your application. A well-structured debugging process can save time, improve efficiency, and bolster the overall quality of the software being tested.
There are key elements associated with debugging that every tester should recognize. First is the ability to understand the error messages produced during test failures. Instead of diving headfirst into the code, itâs imperative to take a step back. Analyzing those messages can often provide a roadmap to the root cause. Secondly, the practice of isolating variables can simplify the troubleshooting process. This involves testing smaller sections of code individually to narrow down issues without being overwhelmed by the breadth of the entire script.
Benefits of effective debugging are manifold. Among them, improved accuracy in automated tests tops the list. When tests are properly debugged, they yield reliable results that reflect genuine application performance. Moreover, honing your debugging skills can increase your programming prowess. With practice, the ability to spot errors quickly becomes second nature, leading to more productive coding sessions.
While debugging is essential, it also requires a degree of patience and consideration. Often, the simplest oversightâsuch as a missed semicolon or an unrecognized variableâcan derail an otherwise perfect test script. Recognizing this fact reminds us that attention to detail is paramount in this field.
"Mistakes are a fact of life. It is the response to the error that counts."
Common Issues and Solutions
In the world of automated testing with Selenium, various issues can arise, challenging even the most seasoned testers. Below are some common problems along with their solutions:
- Element Not Found: This is perhaps one of the most frequent issues when writing Selenium tests. The reason could range from timing issues (elements taking longer to load) to incorrect locators. The solution here often involves implementing explicit waits to give the elements time to appear before interacting.
- Stale Element Reference: This occurs when a web element youâre trying to interact with is no longer attached to the DOM. If the page is refreshed or navigated away, you may encounter this error. Using to re-find the element can help resolve this issue.
- Timeout Exceptions: Tests might fail due to timeouts in locating elements. This could be due to elements taking longer to load. The fix involves adjusting timeouts based on the expected load time of your application or using appropriate wait strategies.
- Technical Misconfiguration: Often, issues arise simply from improper setup, like mismatched versions of Selenium WebDriver and your browser. Ensuring that versions are compatible can prevent many headaches.
Tools for Debugging Selenium Tests
Several tools can enhance the debugging process when working with Selenium. Some of the most notable ones include:
- Selenium IDE: A powerful tool that allows you to record and playback your tests. Itâs perfect for beginners and provides a platform to understand the flow of tests more clearly.
- Chrome DevTools: This built-in tool is invaluable when working with Google Chrome. It allows you to inspect elements, monitor changes in the DOM, and view error messages that can provide deeper insights into issues that Selenium encounters.
- JUnit or TestNG: These are testing frameworks that provide annotations for logging, assertions, and setup methods for managing test cases. They can help catch issues at various stages of test execution.
- Eclipse Debugger: If you're using Eclipse as your IDE, the built-in debugging feature allows you to set breakpoints and step through your code line by line, making the isolation of issues much easier.
Future of Java and Selenium in Software Testing
The landscape of software testing is constantly evolving, and the relationship between Java and Selenium is no exception to this trend. As new technologies emerge and evolve, their integration into existing systems becomes imperative for maintaining efficiency and relevance. The future of Java and Selenium in automated testing not only looks promising but also brings with it a myriad of opportunities that can significantly improve testing practices.
One key aspect worth noting is the ongoing shift towards adopting cloud-based testing environments. With the proliferation of software products and an ever-increasing number of devices, having the ability to run tests in the cloud allows developers and testers to achieve greater scalability. Java, known for its platform independence, complements Seleniumâs versatility, enabling testers to operate their frameworks across a variety of cloud infrastructures seamlessly.
Moreover, the increasing focus on continuous integration and delivery (CI/CD) practices heavily influences the future of testing. As teams adopt agile methodologies, the need for automated tests that can integrate smoothly into CI pipelines is critical. Selenium's ability to quickly run browser-based tests makes it a staple for such integrations. Java supports this by providing powerful libraries that facilitate automation with flexible configurations and community support thatâs hard to match.
"Automation in testing isnât just about speed; itâs about achieving precision while embracing emerging technologies."
In addition, a noteworthy trend is the growing emphasis on visual and exploratory testing. As applications become more interactive and user-centric, the tools need to keep up. Innovative plugins and tools are being developed that leverage Java with Selenium to enhance visual testing capabilities, allowing for better user interface evaluations.
Emerging Trends in Automated Testing
With automated testing playing a crucial role in software development, itâs vital to stay abreast of whatâs changing. Some emerging trends include:
- AI-Driven Testing: The infusion of artificial intelligence in automation tools is gaining traction. AI can enhance test case generation, maintenance, and optimization.
- Robust Browser Testing Tools: As browsers evolve, so too must the testing tools. Tools that ensure compatibility with multiple browser versions and setups are on the rise.
- Shift-Left Testing: This approach encourages earlier testing in the software development lifecycle. Emphasizing prevention rather than detection leads to fewer bugs in the later stages of development.
- Containerization: Using technologies like Docker for test environments enables better consistency across different staging environments, further solidifying the reliability of tests.
Staying connected with these emerging trends enables developers to enhance their automation strategies and embrace necessary changes.
The Role of Artificial Intelligence in Testing
Artificial Intelligence is transforming how we view software testing. Its role is becoming increasingly pertinent for several reasons:
- Enhanced Test Design: AI can analyze existing test scripts, predict defects, and suggest improvements. This leads to the creation of smarter tests that cover more ground without additional manual input.
- Automated Maintenance: AI can autonomously maintain test scripts, making the testing process smoother as applications are updated with minimal human intervention.
- Performance Optimization: Through pattern recognition and machine learning, AI can help optimize test execution times and resource usage. This contributes to faster feedback cycles, allowing for quicker releases.
- User Experience Monitoring: By simulating real user interactions, AI can gauge performance and usability in a manner that traditional automated testing often falls short of.
In summary, embracing AI within Java and Selenium environments presents vast advantages. As testing evolves, utilizing AI not only addresses the increasing complexity of applications but also streamlines processes. Overall, the future of Java and Selenium in software testing is bright and teeming with potential, driven by technological advancements and the shifting needs of the software development community.