Java Programming Test Insights for Interviews
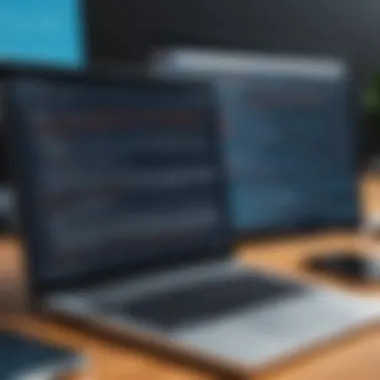
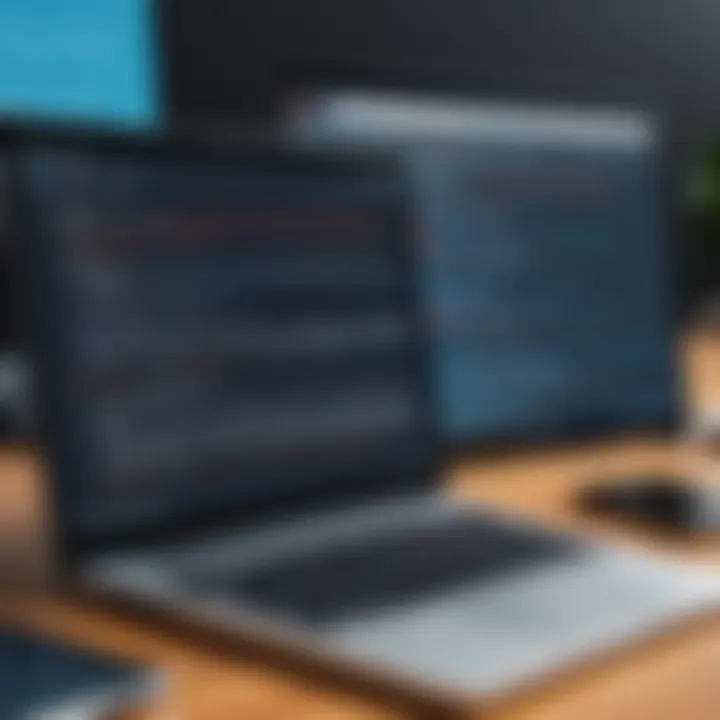
Intro
Java, initially released in 1995, has become a cornerstone in software development. Its design emphasizes portability, security, and robustness. Today, Java programming tests are critical components of technical interviews for positions in software engineering, web development, and related fields. Candidates should understand Java's fundamentals, coding challenges, and specific pitfalls that often arise during assessments.
History and Background
Java was conceived by James Gosling and his team at Sun Microsystems. The language was designed to have as few implementation dependencies as possible. This led to the creation of the slogan "Write Once, Run Anywhere" (WORA). As Java evolves, it has included features such as automatic memory management and support for multithreading.
Features and Uses
Java's primary features include:
- Platform independence: Java applications can run on any device that has a Java Virtual Machine (JVM).
- Strongly typed: It helps to catch errors at compile time rather than run time.
- Memory management: Automatic garbage collection helps manage memory and optimize resource use.
- Rich API: Java provides a comprehensive library that eases development across various domains such as web applications, mobile apps, and enterprise software.
Java is widely used in large systems and critical infrastructure. Industries such as banking, healthcare, and telecommunications choose Java for its reliability.
Popularity and Scope
According to recent surveys, Java consistently ranks among the top programming languages used worldwide. Its community is vast, providing access to numerous resources for learning and support.
The versatility of Java allows it to be utilized in numerous fields:
- Web Development (using frameworks like Spring and Hibernate)
- Mobile Development (Android applications)
- Enterprise Applications (Java EE)
In summary, understanding Java is vital not only for candidates preparing for interviews but also for those who are entering or advancing in the tech industry.
Basic Syntax and Concepts
Basic syntax and concepts form the building blocks for any programming language. For Java, these fundamentals are essential for successful test performance.
Variables and Data Types
In Java, variables are used to store data. The language has several built-in data types:
- Primitive Types: int, char, boolean, etc.
- Reference Types: Objects and arrays.
Declaring variables follows a clear format:
Operators and Expressions
Operators in Java can be classified as follows:
- Arithmetic Operators: +, -, *, /, %
- Relational Operators: ==, !=, >, , >=, =
- Logical Operators: &&, ||, !
Simple expressions can be formed using these operators, allowing candidates to solve basic problems quickly during interviews.
Control Structures
Control structures direct the flow of execution. Key structures include:
- Conditional Statements: if, switch
- Loops: for, while, do-while
Using control structures effectively allows candidates to demonstrate logical thinking and their ability to write efficient code.
Advanced Topics
Once candidates master the basics, they can explore more complex concepts, which are often the focus of technical tests.
Functions and Methods
Functions improve modularity and reusability. In Java, methods define operations performed on objects. They can take parameters and return values:
Object-Oriented Programming
Java is an object-oriented language, which allows for modeling real-world entities. Key principles include:
- Encapsulation
- Inheritance
- Polymorphism
Grasping these principles greatly enhances code structure and management skills for candidates.
Exception Handling
Handling exceptions is crucial in any language to ensure robustness. In Java, exceptions are managed using try, catch, and finally blocks:
Understanding exception handling is essential for writing resilient code and is often a focus during interviews.
Hands-On Examples
Practical application solidifies learning. These examples transition theoretical knowledge into functional programming.
Simple Programs
Creating simple programs can boost confidence. For example, a program to print the Fibonacci series demonstrates both loops and functions:
Intermediate Projects
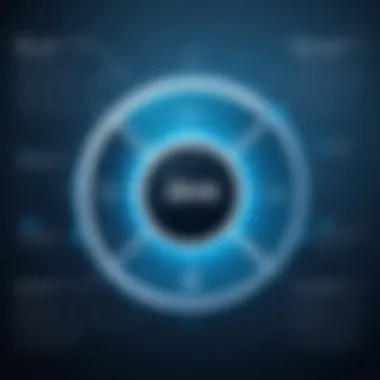
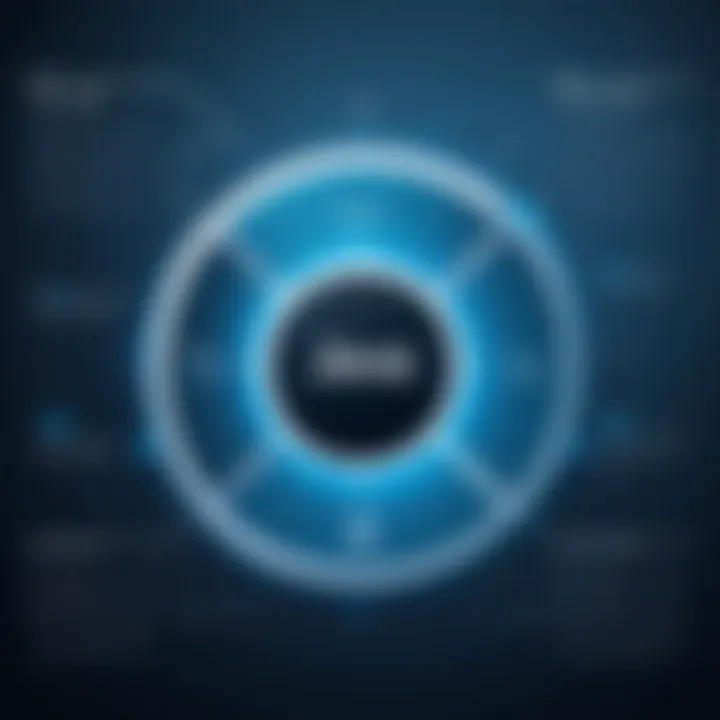
Candidates should attempt intermediate projects to master concepts. Projects like a calculator or a small game can cover various aspects of Java.
Code Snippets
Having short code snippets handy can aid in interviews. They should be clear, to the point, easy to modify, and reusable during assessments.
Resources and Further Learning
Continued education is vital in programming. Utilizing the right resources can make a considerable difference in preparation.
Recommended Books and Tutorials
- Effective Java by Joshua Bloch
- Java: The Complete Reference by Herbert Schildt
Online Courses and Platforms
- Coursera: Java Programming and Software Engineering Fundamentals
- edX: Introduction to Java Programming
Community Forums and Groups
Accessing community knowledge can provide additional support. Websites like Reddit have lively discussions around Java programming.
Remember, preparation is key. Understanding the core requirements of Java programming tests will boost your confidence and skills in technical interviews.
Preamble to Java Programming Tests
Java programming tests are crucial in today's technology-driven job market. Candidates often face these evaluations during interviews to demonstrate their coding abilities and understanding of the language. The purpose of these tests aligns with the goal of identifying skilled developers who can solve problems rapidly and efficiently. By ensuring a fair and structured assessment, interviewers can better gauge a candidate's potential.
Including a variety of test formats enhances the evaluation process. Different testing methods allow interviewers to assess different skills and aspects of programming in Java. This creates a more complete picture of a candidate's abilities. Moreover, understanding this area can aid candidates in preparing effectively and tailoring their study methods to the demands of the tests they may encounter.
In summary, Java programming tests not only reveal a candidate's technical skills but also showcase their problem-solving capabilities and adaptability. Familiarity with these tests is essential for both parties involved in the interview process.
Purpose of Java Programming Tests
The primary goal of Java programming tests is to evaluate a candidate's proficiency in writing Java code. They assess not only syntax knowledge but also a wider grasp of logic and algorithms. This can help companies make informed decisions regarding hiring, as candidates showcase their ability to tackle real-world programming tasks.
Furthermore, these tests serve a dual purpose. For candidates, they provide an opportunity to demonstrate their understanding of Java's core principles and practices. Completing these evaluations well can significantly boost a candidate's confidence and improve their chances of landing a desirable position.
Common Formats of Java Tests
Java programming tests can take various forms, each with unique advantages. Some common formats include:
- Multiple-Choice Questions: Often used to test theoretical knowledge about Java fundamentals.
- Coding Challenges: Candidates write code to solve specific problems. This demonstrates their practical coding skills and familiarity with algorithms.
- Take-Home Assignments: These allow candidates to complete coding tasks at their own pace. They often provide insight into the quality of code, documentation, and approach taken by the candidate.
- Live Coding Interviews: In these sessions, candidates code in real-time while interviewers observe. This format tests both coding ability and communication skills under pressure.
Understanding the formats helps candidates prepare. Adapting study techniques to suit the specific type of test can improve performance.
Key Concepts in Java
Understanding key concepts in Java is paramount for success in coding interviews. These principles form the foundation of Java programming and greatly enhance the candidate's ability to solve problems effectively. Knowing these concepts allows candidates to better showcase their skills and understanding of Java. Moreover, interviewers use them to evaluate the depth of knowledge in a candidate, which is crucial for making informed hiring decisions.
Object-Oriented Programming Principles
Java is designed around the principles of object-oriented programming (OOP), which includes four fundamental concepts: encapsulation, inheritance, polymorphism, and abstraction. Each of these principles plays a crucial role in creating a strong and maintainable code structure.
Encapsulation
Encapsulation involves bundling the data (attributes) and the methods (functions) that operate on data into a single unit called a class. This principle helps protect the internal state of an object from unintended interference and misuse. By restricting direct access to some components, encapsulation promotes modularity and controlled access. It is beneficial in this article because it highlights the importance of robust data management in Java applications.
The unique feature of encapsulation is the use of access modifiers, such as , , and , which dictate the level of access to class members. This can be advantageous as it enhances security and reduces complexity by keeping the internal workings hidden from the outside. However, encapsulation can also introduce additional layers of complexity if not managed properly.
Inheritance
Inheritance is a mechanism that allows one class to inherit fields and methods from another class. This principle promotes code reusability and establishes a hierarchical relationship between classes. In Java, inheritance encourages the use of abstract classes and interfaces which can significantly improve the design of a system. Its use in this article showcases how effective inheritance can minimize redundancy in coding.
The unique feature of inheritance is that it allows subclasses to override methods of the parent class, which is useful for achieving polymorphic behavior. A person might see this as advantageous because it enhances flexibility in code modification. However, improper use of inheritance can lead to a convoluted class hierarchy that is difficult to maintain.
Polymorphism
Polymorphism enables a single interface to represent different data types. In Java, it primarily manifests as method overloading and method overriding. This principle allows for flexibility and the ability to define multiple behaviors for the same action. Its inclusion is valuable in this article since it highlights how polymorphism can simplify code and improve its readability.
Polymorphism's unique feature is its ability to allow different classes to be treated as instances of the same class through a common interface. This is beneficial for maintaining cleaner and more modular code. On the downside, abuse of polymorphism can make code harder to understand because the actual method being invoked might not be clear without tracing through several layers of implementation.
Abstraction
Abstraction simplifies complex reality by modeling classes based on essential qualities. In Java, abstraction can be achieved with abstract classes and interfaces, providing a way to define the core behaviors without detailing implementation. This is significant in this article as it emphasizes the need for creating simplified models that allow for easier management of complex systems. The unique feature of abstraction is that it helps enhance the focus on the important aspects of object design while hiding unnecessary details. Such a focus is advantageous as it leads to better application architecture. Nevertheless, over-abstraction can lead to design that is too complex and can hinder the development process.
Core Java Syntax
Core Java syntax consists of the rules and regulations for writing the Java programming language. This syntax includes variable declarations, control statements (if-else, switch, loops), class and method definitions, and more. Understanding Java syntax is crucial for any developer, as it acts as the foundation for writing functional Java programs. Correct syntax ensures that the code is interpretable and executable by the Java compiler. Mistakes or incorrect usage can lead to compilation errors and runtime exceptions, which programming candidates must be aware of during interviews.
Data Types and Variables
Data types in Java define the types of data that can be stored and manipulated within a program. Java has two primary data types: primitive types and reference types.
- Primitive Types: These include , , , and , among others. They hold simple values and are non-object types.
- Reference Types: This category includes arrays and objects, which reference a memory location that holds the actual data.
Understanding both data types and how to declare and use variables effectively is essential in this article. Variables serve as containers for storing data values, and knowing their scope and lifetime can significantly impact the performance and reliability of Java applications. Poor use of data types and variables can lead to memory leaks and unwanted behaviors in programs.
Common Java Interview Questions
Understanding common Java interview questions is crucial for candidates preparing for technical interviews. These questions not only evaluate a candidate's technical abilities but also test their problem-solving approach, critical thinking, and adaptability in coding real-world solutions.
Benefits of mastering these questions include:
- Assessment of skills: Technical questions reveal the depth of a candidate's knowledge in Java programming and their ability to apply concepts effectively.
- Increased confidence: Knowing what to expect helps in reducing anxiety during the interview process, allowing candidates to focus on their performance.
- Preparation for various roles: Different positions may require different levels of expertise. Recognizing common themes in questions helps candidates tailor their learning and preparation.
Technical Questions
Technical questions often center around key Java concepts, algorithms, and data structures. They might include inquiries about specific Java libraries or syntax peculiarities. Sample questions could involve:
- Explain the difference between an interface and an abstract class in Java.
- How does garbage collection work in Java?
- Can you describe the Java Collections Framework?
- Write a method to find the largest number in an array.
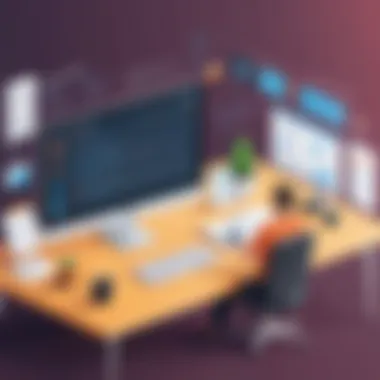
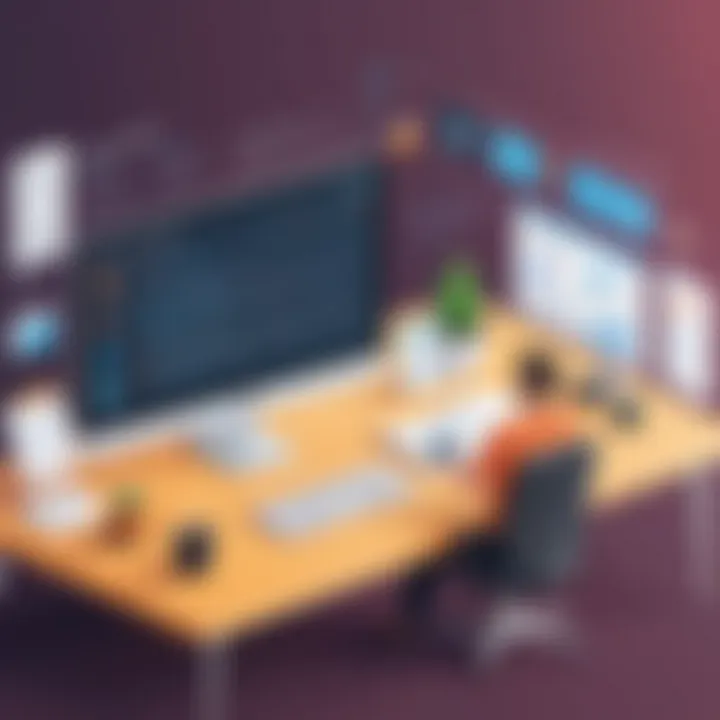
Candidates should focus not only on providing correct answers but also on articulating their thought process. For instance, when asked about sorting algorithms, explaining the differences between QuickSort and MergeSort showcasing both time and space complexity demonstrates a deeper understanding.
"Technical questions are not just tests of knowledge, but opportunities to showcase problem-solving skills."
Behavioral Questions
Behavioral questions are equally important as technical ones. These questions aim to understand how a candidate handles challenges and works within a team. They may include:
- Describe a time when you faced a difficult problem in coding. How did you solve it?
- How do you prioritize tasks when you have multiple deadlines?
- Can you share an experience where you had to debug an issue?
Candidates should highlight their experience, illustrating their approach to challenges and collaboration. Providing specific examples and outcomes can greatly enhance responses.
Tips for answering behavioral questions:
- Use the STAR method (Situation, Task, Action, Result) to structure answers.
- Be honest about challenges faced and what was learned.
- Display emotional intelligence and communication skills when discussing experiences.
Practicing Coding Challenges
Practicing coding challenges is a fundamental aspect of preparing for Java programming interviews. The purpose of these challenges is not only to assess candidatesβ coding abilities but also to measure their problem-solving skills and logical thinking. A well-structured practice routine can provide candidates with valuable insights into their strengths and weaknesses, allowing them to focus their efforts on the areas that require improvement. Moreover, tackling coding challenges can help in familiarizing candidates with the types of questions they may encounter during interviews.
Engaging in these challenges helps candidates build confidence. Confronting a wide array of problems prepares them for the unpredictability of interview scenarios. This preparation also deepens understanding in key Java concepts, which is crucial for expressing technical knowledge convincingly during discussions.
Types of Coding Challenges
Algorithm Problems
Algorithm problems focus on the core principles of computer science such as complexity and data manipulation. They are critical for understanding the underlying mechanics of how data is processed and optimized within software applications. A key characteristic of algorithm problems is their focus on efficiency. Candidates often need to devise solutions that not only work but do so in the most efficient manner possible. This aspect makes them a beneficial choice in this article.
The unique feature of algorithm problems lies in their emphasis on logic and reasoning. Candidates can enhance their analytical thinking abilities by dedicating time to solve these issues, which also translates well into real-world software development. However, a disadvantage is that they may be challenging for beginners, necessitating a solid foundation in programming concepts before grappling with complex algorithms.
System Design Questions
System design questions pertain to creating scalable software architecture. They challenge candidates to think about components, data flow, and system interactions, which are essential in real-world applications. A key characteristic of these questions is their breadth, requiring candidates to address various aspects of system construction, such as performance and reliability. This makes them a popular choice for this article.
The unique feature of system design questions is their practical application. Candidates can draw from their experiences and understand the full scope of what constitutes a robust software solution. However, a downside is that thorough preparation requires time and prior knowledge of design patterns and architectural principles.
Real-World Scenarios
Real-world scenarios depict situations candidates may face in software development. These challenges allow candidates to apply Java skills in contexts similar to what they would encounter in their jobs. A vital characteristic of real-world scenarios is their relevance, connecting theoretical knowledge to practical application. This alignment makes them a useful aspect of this article.
The unique feature of real-world scenarios is their emphasis on application over mere theoretical knowledge. They push candidates to develop solutions that are not only functional but also meet user needs. One disadvantage is that these scenarios can be situationally specific, potentially leading to confusion if candidates are unfamiliar with the context of the problem.
Resources for Practice
Practicing coding challenges effectively requires quality resources. Websites like LeetCode and HackerRank offer vast repositories of coding problems tailored for various skill levels. Additionally, reviewing community discussions on platforms such as Reddit can provide insights into common pitfalls and best practices. For deeper learning, books like "Cracking the Coding Interview" can be incredibly beneficial.
In summary, engaging in various coding challenges, from algorithm problems to real-world scenarios, fosters essential skills that are critical for success in Java programming interviews. Such practice reinforces theoretical knowledge and enhances problem-solving abilities, ultimately contributing to a more rounded preparation for candidates.
Using Integrated Development Environments
The role of Integrated Development Environments (IDEs) in Java programming tests is crucial. IDEs provide a comprehensive platform where developers can write, debug, and compile code efficiently. For those preparing for interviews, understanding how to leverage an IDE can significantly enhance productivity and confidence during coding tasks. The right IDE not only aids in writing cleaner code but also helps manage project files, version control, and testing processes effectively. Thus, familiarity with IDEs is essential to navigate technical interviews seamlessly.
Choosing the Right IDE
Selecting an appropriate IDE can impact your coding efficiency during an interview. Here are three notable options:
Eclipse
Eclipse is a widely used IDE known for its extensibility and robust plugin system. It supports various programming languages, but its strength lies in Java development. One key characteristic of Eclipse is its powerful project management capabilities, which allow for easy organization of multiple files. This is beneficial for candidates who might work on larger projects as part of their interview tests.
A unique feature of Eclipse is the integrated debugging tool, which streamlines error checking and performance analysis. It does have a steeper learning curve for beginners, but investing time in mastering Eclipse can lead to significant long-term benefits.
IntelliJ IDEA
IntelliJ IDEA is renowned for its intelligent coding assistance and exceptional refactoring capabilities. This IDE stands out due to its intuitive interface and smart code completion features that enhance the coding experience. It is a popular choice among developers because it reduces the time required to write boilerplate code, allowing you to focus more on solving the problem at hand in an interview.
One significant feature of IntelliJ IDEA is the built-in version control system integration, enabling seamless collaboration and easy code management. However, it can be resource-intensive, requiring a more powerful machine for optimal performance.
NetBeans
NetBeans offers an all-in-one solution for Java development with an easy setup process. Its key characteristic is the support for multiple languages, including Java, which makes it a versatile option. NetBeans is beneficial for students and beginners since it comes with excellent documentation and tutorials to ease the learning process.
A unique feature of NetBeans is its GUI builder, which allows for rapid development of user interfaces. This is especially advantageous in interviews focused on building applications with graphical elements. However, NetBeans may not match the extensibility of Eclipse or IntelliJ IDEA, limiting advanced customization options.
Setting Up the Environment
Setting up the IDE environment is a fundamental step before delving into coding questions. Candidates must ensure they have the necessary software installed and properly configured. This involves selecting the appropriate version of the JDK, configuring build paths, and ensuring all libraries are correctly referenced.
Properly configured IDEs can save time and reduce errors during coding assessments, allowing candidates to focus on demonstrating their programming skills.
Once the environment is set up, familiarity with the IDE's features is paramount. Practice using debugging tools, version control systems, and code navigation features. Being comfortable with these tools will enhance problem-solving speed during interviews.
Understanding Java Libraries and Frameworks
Java libraries and frameworks are essential components in Java programming. They provide developers with pre-written code to facilitate common tasks. Understanding these can enhance productivity and code quality. Each library and framework has specific purposes, addressing unique needs in application development.
Popular Java Libraries
Apache Commons
Apache Commons is a widely-used library that offers reusable components. Its primary contribution is to streamline code development by providing commonly used functionalities. The key characteristic of Apache Commons is its variety of utilities, from file manipulation to object manipulation. This versatility makes it a beneficial choice for developers looking to avoid reinventing the wheel. A unique feature of Apache Commons is its modular design, allowing the integration of only required components, which can help reduce project size. One advantage is its extensive documentation and community support, while a possible disadvantage is the learning curve associated with its various components.
Google Guava
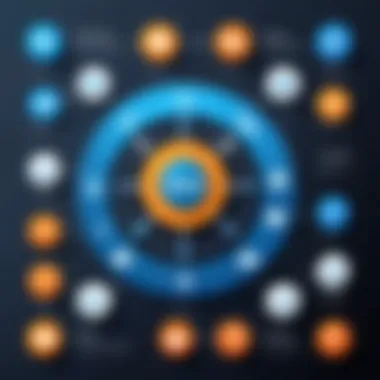
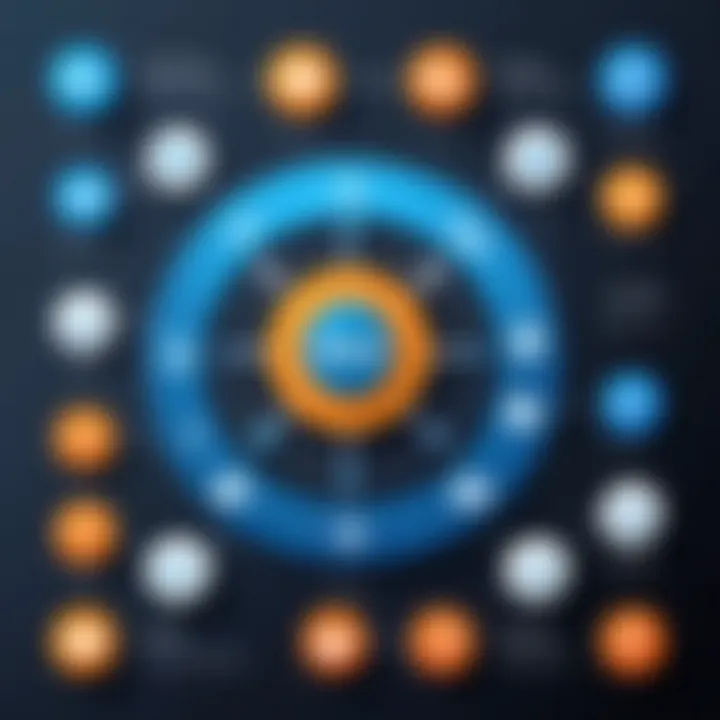
Google Guava is another powerful library that enhances Java's standard library. It focuses on the collection framework and provides additional utilities for developers. The key characteristic of Google Guava is its focus on immutability and functional programming aspects in Java. It is a popular choice due to its advanced collections and caching capabilities. A unique feature of Guava is its ability to simplify complex data handling with rich APIs. While its advantages include improved performance and ease of use, its disadvantages may stem from added complexity in understanding its extensive features for beginners.
JUnit
JUnit is a framework used for unit testing in Java. Testing is crucial in software development, and JUnit provides a structured way to ensure code reliability. The key characteristic of JUnit is its annotation-based testing structure, which allows tests to be organized easily. This makes it a beneficial tool for maintaining code quality. A unique feature is its support for test suites and various assertion methods, which helps write robust tests. Its advantages include wide adoption and integration with many IDEs, while a potential disadvantage is the initial setup before getting to testing.
Frameworks to Know
Spring Framework
Spring Framework is an influential framework designed for enterprise applications. Its contribution lies in simplifying Java development through features like dependency injection. The key characteristic of Spring is its modular architecture, allowing developers to pick and choose components. This flexibility makes it a popular choice for developing large applications. A unique feature of Spring is its support for aspect-oriented programming, enhancing code modularity. Key advantages include a rich ecosystem of tools and libraries, but it may introduce disadvantages, such as the complexity of configuration, especially for beginners.
Hibernate
Hibernate is primarily a data access framework that simplifies database interactions. It addresses the complexities of object-relational mapping (ORM) in Java applications. The key characteristic of Hibernate is its ability to map Java objects to database tables seamlessly. This makes it a beneficial choice for developing data-driven applications. A unique feature is its caching mechanism, which improves performance by reducing database access. One of its advantages is the time saved in managing database interactions, though a disadvantage could be the overhead of learning proper configuration and mappings.
JavaFX
JavaFX is a framework for building rich user interfaces in Java applications. Its contribution lies in providing a versatile platform for desktop applications. The key characteristic of JavaFX is its ability to create visually appealing graphics with ease. This makes it a beneficial choice for developers targeting desktop environments. A unique feature of JavaFX is its scene graph, which simplifies layout management and rendering. While its advantages include modern UI capabilities, one potential disadvantage may be limited community support compared to older frameworks like Swing.
Understanding these libraries and frameworks is crucial for any Java developer. They can significantly enhance productivity and the practical application of Java in various projects.
Soft Skills for Interview Success
In the realm of technical interviews, particularly in Java programming assessments, candidates often focus heavily on their technical abilities. However, it is vital to recognize the significance of soft skills in shaping interview success. These interpersonal skills complement technical knowledge, enabling candidates to present themselves effectively and integrate into a team environment. The importance of soft skills cannot be overstated, as they often differentiate candidates with similar technical qualifications.
Soft skills encompass a variety of competencies such as teamwork, emotional intelligence, and adaptability. They enhance communication, ensuring that candidates can articulate their thought processes clearly during problem-solving situations. Additionally, these skills facilitate rapport-building with interviewers, creating a more engaging and memorable experience.
Another benefit of strong soft skills is their influence on performance during collaborative projects. Many development roles require candidates to work within teams. Therefore, demonstrating proficiency in these areas signals to employers that candidates will contribute positively to workplace dynamics.
Moreover, candidates who exhibit strong soft skills often portray an eagerness to learn and grow. This adaptability is crucial in the ever-evolving tech landscape, where staying current with trends and technologies, especially in Java, is essential. Candidates should strive to hone these skills alongside their technical abilities to present a well-rounded profile.
"Software development is not just about writing code. It's about communication and collaboration."
Communication Skills
Communication skills play a critical role in the success of any interview. They involve the ability to clearly express ideas, listen effectively, and engage in meaningful dialogue with others. In a Java programming test, being able to articulate oneβs thought process when solving coding challenges can significantly impact the impression made on interviewers.
Firstly, clear communication helps interviewers understand a candidate's technical reasoning. When discussing code, explaining choices made during problem-solving, or elaborating on design decisions, clarity is essential. If a candidate stumbles over explanations, it may raise concerns about their competence.
Active listening is also important. Candidates must pay careful attention to interview questions and instructions. Misunderstanding a question can lead to irrelevant answers or missed opportunities to showcase relevant skills. Thus, candidates should practice summarizing questions to confirm understanding.
Lastly, effective communication extends beyond verbal skills. Non-verbal cues, such as body language and eye contact, enhance confidence and engagement. Being aware of these elements can create a positive interaction during the interview.
Problem-Solving Approach
A strong problem-solving approach is crucial for candidates undergoing Java programming tests. Interviews often involve algorithmic challenges or design scenarios, meant to evaluate a candidate's ability to tackle practical problems. Adopting a systematic method can make a significant difference in successfully navigating these challenges.
First, problem-solving begins with understanding the issue at hand. Candidates should take time to dissect the problem into smaller components. This approach not only clarifies the requirements but may also highlight edge cases that need to be addressed.
Next, candidates should formulate a plan. This may involve brainstorming possible solutions and outlining a strategy before diving into coding. Sharing this thought process with the interviewer provides insight into the candidate's logical reasoning and planning abilities.
Execution is the next step. Candidates must not only write correct code but also consider efficiency and potential optimizations. Discussing time complexity and space complexity demonstrates a deeper understanding of algorithms.
Lastly, testing and validating the code is crucial. Candidates should speak through their testing approach, checking against sample inputs and discussing potential failure points. This final step reinforces a thorough problem-solving approach and highlights a commitment to quality.
By focusing on these soft skills, candidates can enhance their Java programming interview performance significantly. It is not solely about the technical knowledge but also about how candidates communicate and solve problems.
Common Pitfalls to Avoid
Navigating the realm of Java programming tests can be challenging, especially in an interview setting. As candidates prepare for these assessments, it is crucial to be aware of common pitfalls. By understanding these mistakes, candidates can enhance their performance and avoid unnecessary errors. Recognizing these issues not only boosts confidence but also promotes a deeper comprehension of Java concepts. Below are two significant pitfalls to be mindful of.
Neglecting Fundamentals
The foundation of any programming language lies in its fundamentals. In Java, this includes understanding basic syntax, data structures, and core principles of object-oriented programming. Neglecting these essentials can result in poor performance during interviews. Candidates might focus too much on complex problems or advanced libraries, thus skipping over basic concepts. This can lead to a lack of clarity when answering questions or solving coding challenges.
As a strategy, candidates should allocate time to review basic concepts. In-depth knowledge of topics like loops, conditional statements, and data types are vital. Mastering these areas allows for better problem-solving skills during technical assessments. Practicing simple problems can reinforce these concepts. It can also help in recognizing patterns commonly found in coding questions.
"A solid grasp of fundamental principles is indispensable for tackling more advanced topics effectively."
Overcomplicating Solutions
Another common failure is the tendency to overcomplicate solutions. Many candidates think that more complex solutions demonstrate greater skill. However, this could not be further from the truth. Interviewers value clarity and elegance in code. An overly complicated solution may indicate confusion or a lack of understanding of the problem at hand.
To avoid this pitfall, candidates should focus on simplicity. A clear, direct solution is often more effective than a convoluted one. Break the problem down into manageable parts. Use straightforward logic when approaching coding challenges. Additionally, think through the implications of each choice made in the code. Candidates should also practice articulating their thought process during mock interviews to enhance their ability to communicate effective solutions.
By focusing on fundamentals and aiming for simplicity, candidates can significantly improve their performance in Java programming tests. It ensures a streamlined approach to problem solving and enhances their overall coding skills.
End and Next Steps
In concluding this article on Java programming tests for interviews, it is crucial to recognize the significance of adequate preparation and continuous learning in the evolving field of software development. As technology advances, the expectations for candidates change. Understanding how to prepare effectively for interviews can dramatically enhance one's chances of success.
Being well-versed in Java's foundational concepts and practical applications places candidates at an advantageous position. This includes familiarity with coding challenges, libraries, and frameworks typically encountered during interviews. Moreover, acknowledging the potential pitfalls, such as neglecting core programming principles or overcomplicating solutions, helps candidates approach problems with clarity and confidence.
Preparing for Interviews
Preparation for interviews should not be an afterthought; it is a vital component of the overall process. Here are some strategies that candidates can employ:
- Mock Interviews: Engaging in practice sessions with peers or mentors can provide realistic insights. Mock interviews help refine answers and improve delivery under pressure.
- Review Core Concepts: Regularly review fundamental Java concepts such as object-oriented programming, data types, and error handling. This knowledge is critical when responding to technical questions.
- Coding Exercises: Utilize online platforms to solve coding problems. Websites like LeetCode or HackerRank offer extensive practice sets specifically for Java.
- Understand Common Libraries: Familiarising oneself with libraries like the Java Collections Framework or JUnit can give a significant edge in understanding the practical applications of Java.
In addition to these methods, candidates should also consider the company culture and the specific technologies they use. Researching the organization's tech stack may help tailor answers and demonstrate genuine interest.
Continuous Learning in Java
The journey of a Java developer does not end with securing a job. Continuous learning is essential for staying relevant in a competitive job market. Here are some recommendations for ongoing development:
- Online Courses: Platforms like Coursera or Udemy provide courses that cover advanced topics in Java, including concurrent programming and Java EE frameworks.
- Join Coding Communities: Participating in forums on Reddit or Stack Overflow can provide insights into common challenges faced by developers and solutions from experienced professionals.
- Attend Meetups and Conferences: Engaging with other developers and industry leaders can offer networking opportunities and insights into the latest Java trends and technologies.
- Contribute to Open Source Projects: Working on real-world projects can improve coding skills and understanding of collaborative programming environments. This experience can also be a valuable addition to oneβs resume.
In summary, the landscape of Java programming is constantly changing. Candidates should take advantage of various resources and strategies to prepare for interviews thoroughly, and pursue continuous learning to thrive in their careers.