Java Profiling Techniques and Best Practices Guide
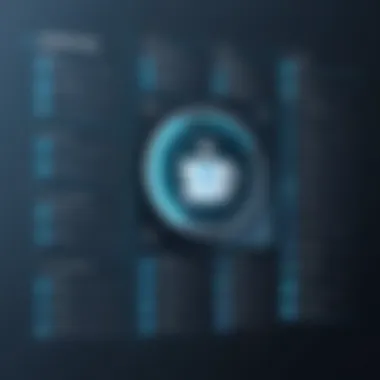
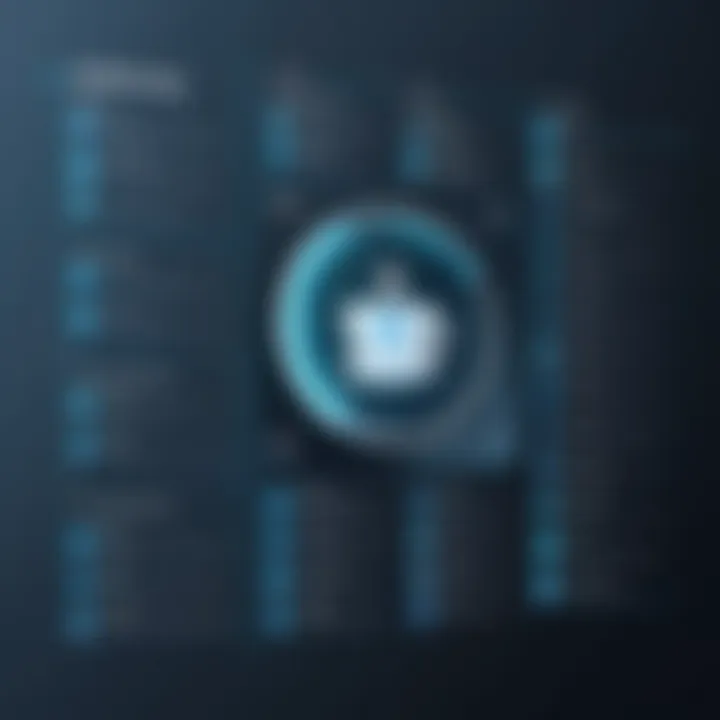
Intro
Java profiling is a critical practice for optimizing performance in Java applications. Given the complexities of modern software development, understanding how to effectively profile your code can mean the difference between an application that runs smoothly and one that struggles under the weight of its tasks. In this guide, we will explore various profiling methodologies, dive into tools available, and highlight key performance analysis aspects that every developer should consider.
It’s essential to establish a few baseline definitions around profiling. Simply put, profiling helps developers understand where their application spends most of its time and resources, including memory and CPU usage. Without profiling, pinpointing bottlenecks can feel like looking for a needle in a haystack.
Understanding Java Profiling
Why is Profiling Necessary?
Profiling isn’t just a luxury for seasoned professionals; it’s an integral part of software development. By identifying performance issues early on, developers can optimize their applications proactively. This not only enhances user experience but also ensures that applications can scale without sacrificing performance.
- Faster Response Times: Users expect applications to run smoothly. Profiling reveals sluggish areas so you can address them promptly.
- Better Resource Management: Efficient use of resources minimizes waste, which is tantamount to savings in operating costs.
- Enhanced Scalability: As applications grow, unoptimized code can hinder their potential. Profiling paves the way for better scalability.
Key Techniques in Profiling
When it comes to profiling Java applications, several techniques can be employed. A selection of those includes:
- Sampling: This method periodically checks the state of an application, providing a statistical overview of where time is being spent.
- Instrumentation: By inserting additional code into your application, it can reveal detailed insights into execution paths and performance metrics.
- Tracing: Tracing captures detailed execution information about function calls and is valuable for understanding problems in more complex scenarios.
Tools for Java Profiling
To navigate the profiling landscape effectively, various tools are available. Here’s a brief overview of some noteworthy ones:
- VisualVM: It’s a free tool that provides real-time monitoring and profiling functionalities integrated into the JDK.
- YourKit Java Profiler: A commercial profiler known for its user-friendly interface and powerful insights into memory usage and CPU performance.
- Java Mission Control: Part of the JDK, it offers advanced monitoring capabilities and is designed for analyzing Java applications with minimal overhead.
Each of these tools caters to specific needs and preferences. When choosing a profiler, consider factors like ease of use, integration capabilities, and the specific performance metrics that matter most for your project.
Best Practices in Java Profiling
Knowing how to utilize profiling tools is one thing, but having a solid strategy is another. Here are some best practices to keep in mind:
- Profile Early and Often: Don’t wait until the end of a project to start profiling. Integrate it into your development process from the outset.
- Focus on Critical Paths: Identify and prioritize areas of code that have the most significant impact on performance.
- Analyze Contextually: Understanding which components interact with each other can help reveal deeper performance issues.
"A stitch in time saves nine" – the same sentiment applies to profiling. Regular profiling can help prevent performance degeneration later in the software lifecycle.
In summary, profiling is an essential practice that every Java developer should embrace. By combining the right tools and techniques with sound best practices, you can optimize your applications and ensure they thrive in a competitive environment. In the following sections, we will delve deeper into the specifics of various profiling methodologies, along with practical examples to illuminate these concepts further.
Ending
Java profiling is not just about fixing problems; it’s about understanding the intricate dance of code execution and resource management. By embracing a profiling mindset early in development, you set the stage for a smooth-running application that meets user expectations. Whether you are a novice or a seasoned developer, the principles of profiling are universally beneficial.
Intro to Java Profiling
Profiling is the compass that guides developers toward the optimization of their code. In the realm of Java, profiling is not just an afterthought; it's a way of life for anyone intent on crafting high-performance applications. Understanding this process helps in identifying bottlenecks, memory leaks, and inefficient algorithms—essential components that can mean the difference between an app that hums along smoothly and one that lags like a turtle on a leisurely stroll.
Understanding Profiling in Software Development
In software development, profiling refers to the analysis of a program's behavior. This can encompass a variety of metrics, focusing on aspects like CPU and memory usage as well as response times. Developers often utilize profiling tools to gather data on how an application executes under different conditions.
For instance, imagine you have a Java application that is supposed to handle multiple requests. You might think everything is running fine, but when you dive into profiling, you discover unnecessary thread waits and high CPU consumption during peak usage. Suddenly, the whole scenario changes. Profiling puts you in the driver’s seat. It lets you see under the hood, revealing what happens when your application hits the asphalt.
Some key aspects of understanding profiling include:
- Data collection: Gathering relevant metrics that provide insights into performance.
- Visualization: Making sense of raw data through graphical representations.
- Action plan: Developing strategies based on findings to enhance application performance.
Importance of Profiling for Java Applications
Java, one of the most widely-used programming languages, powers a multitude of enterprise applications. Given this extensive use, profiling becomes crucial because it allows developers to tune their systems for better performance. For example, a common situation in enterprise apps involves handling vast amounts of data. Without profiling, developers are often left shooting in the dark, not knowing if their code is the root cause of slowdowns or memory issues.
Key reasons why profiling is particularly important for Java applications include:
- Enhanced performance: Profiling sheds light on critical areas that need attention, enabling optimizations that lead to improved speed and responsiveness.
- Resource management: It assists in identifying how efficiently resources are being utilized, which is vital given the high demand for memory in Java applications.
- Improved maintainability: When developers know the ins and outs of their code’s performance, they can write cleaner, more efficient code that can be easily understood and modified by others down the line.
"Profiling is like putting your Java application under a microscope; it reveals the tiny issues that can grow into massive problems if left unchecked."
In summary, Java profiling stands as a cornerstone practice that guides developers in refining and optimizing their applications. Setting aside time to profile can yield substantial benefits, including better performance and reduced resource consumption. As the tech landscape continues to evolve, mastering these techniques becomes not just beneficial, but essential for a successful Java development career.
Key Concepts in Java Profiling
Understanding the core concepts of Java profiling is essential for anyone looking to analyze and optimize the performance of Java applications. Just like a skilled mechanic needs to understand how an engine works to fix it, developers must grasp these concepts to effectively diagnose performance issues. This section will paint a clearer picture of what profiling entails and why it is fundamental in the software development lifecycle.
Profiling is not just about running tests and looking at metrics; it involves collecting, analyzing, and interpreting data that reflects an application's behavior at runtime. So, what specific elements should one look at?
Performance Metrics and Indicators
When venturing into the world of Java profiling, performance metrics are your navigation tools. They provide insight into how applications utilize resources like CPU, memory, and threads. Some standard performance indicators include:
- CPU Utilization: Measures how much CPU resources your application consumes. Abnormal spikes can indicate inefficiencies or bottlenecks.
- Memory Usage: Indicates how much memory your application utilizes during its execution. High memory consumption might suggest memory leaks or unnecessary object creation.
- Thread Count: Reflects the number of threads in use. Excessive threads can lead to overhead and performance degradation.
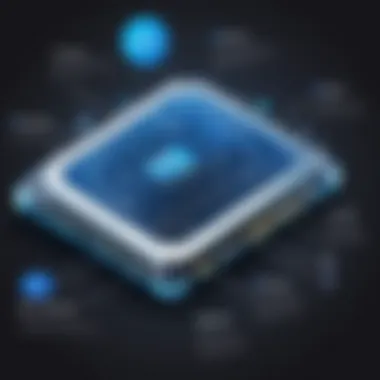
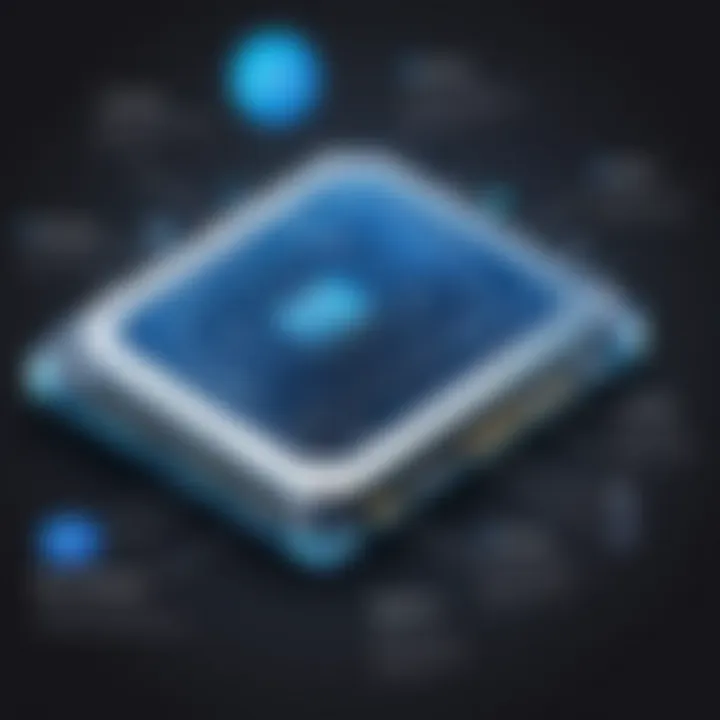
These metrics help identify performance issues swiftly. However, remember that metrics alone don't tell the full story. It's critical to place these indicators in context. For instance, high CPU usage could be benign if your application is performing intensive calculations, but it might raise red flags during idle periods.
"Metrics are like a map; they guide your journey but don't reveal the entire landscape."
Types of Profiling: Sampling vs Instrumentation
In the profiling landscape, sampling and instrumentation represent two distinct methodologies, each with its strengths and weaknesses. Understanding these differences is crucial for selecting the most effective approach for your profiling needs.
- Sampling Profiling: This method collects data at regular intervals, allowing developers to understand which methods are consuming heavy CPU cycles. It’s like glancing at a watch every few minutes to estimate how long you have been working. This approach often introduces less overhead than instrumentation, making it suitable for performance-critical applications that require minimal impact during profiling.
- Instrumentation Profiling: This involves modifying the bytecode of the application to record detailed execution information. Think of it as a fly on the wall, observing everything happening within your code. While instrumentation provides granular data, it can also slow down your application, making it less suitable for production environments.
Each method has its place in profiling. Choosing the right one depends on the context of the application and the nature of the performance issues at hand. From novice students to seasoned developers, being acquainted with these key concepts will enhance your Java profiling skills.
Identifying the root causes of performance issues can often save significant time and resources, ensuring both application efficiency and user satisfaction. By focusing on these fundamental aspects, you're well on your path to mastering Java profiling.
Profiling Techniques
In the ever-evolving landscape of software development, profiling techniques form the backbone of performance optimization. These methods enable developers to capture and analyze how their applications operate under varying conditions. Understanding how these techniques work is crucial; not only do they reveal performance bottlenecks, they also uncover underlying issues that could lead to inefficient resource usage. Adopting effective profiling techniques allows developers to make informed decisions when optimizing their code, ultimately leading to enhanced application performance and user satisfaction.
CPU Profiling Methods
When it comes to profiling CPU usage, you’re looking at an essential aspect of performance analysis. CPU profiling focuses on understanding how processor time is distributed across different parts of an application. This can reveal hot spots where the CPU is spending an inordinate amount of time, signaling an opportunity for optimization.
One common method for CPU profiling is sampling, where the profiler captures the call stack at regular intervals. This gives a statistically representative view of CPU usage over time. Alternatively, instrumentation can be utilized, where the code is modified to include profiling hooks. This method tends to provide more precise information, but at the cost of introducing overhead into the application, which can sometimes skew results.
Factors to consider in CPU profiling include:
- Execution context: Identifying which input sets lead to high CPU usage.
- Time of measurement: Profilers are more effective when used during typical usage scenarios rather than during stress tests, as the latter might present an unbalanced picture.
Memory Profiling Techniques
Memory profiling stands as a cornerstone of application optimization. It is devoted to analyzing how applications allocate, access, and free memory, which can directly impact performance and stability. Identifying and fixing memory leaks—where memory that is no longer used remains allocated—can significantly improve an application's performance.
Common techniques in memory profiling include using memory dump analysis tools and real-time monitoring tools. Tools like YourKit Java Profiler offer insightful visualizations of memory usage, helping detect leaks or inspect object creation patterns. While using memory profiling methods, developers should pay attention to:
- Allocated memory size versus the actual usage size: This gives indicators of inefficient memory use.
- Garbage collection overhead: This aspect tells you how often the garbage collector runs and whether or not it is hampering performance.
Thread Profiling Approaches
Thread profiling tackles the complexity that comes with multi-threaded applications. Given that Java is naturally parallel, understanding thread behavior is key to achieving optimal performance. This method reveals how threads interact, whether there are any contention issues, and how well the application scales under concurrent loads.
Two primary methodologies for thread profiling exist: thread state profiling, which observes the state of threads (such as runnable, blocked, or waiting) at a given point in time, and contention analysis, which assesses how threads are competing for resources.
Key considerations when profiling threads include:
- Monitoring thread contention during peak operation: This can help identify bottlenecks in resource access.
- Shortening thread lifetimes: Long-lived threads might keep resources in use longer than necessary, causing delays for other threads.
Understanding the intricacies of CPU, memory, and thread profiling can be a game changer for developers striving for top-notch performance. Optimizing these aspects can ensure applications run smoothly and efficiently.
By administering various profiling techniques and applying the insights gained, developers can not only enhance performance but also ensure their applications are ready to meet user demands.
Profiling Tools for Java
When it comes to unraveling the complexities of Java applications, profiling tools are like the magnifying glasses developers use to peer into the inner workings of their code. These tools provide insights that go beyond what a casual glance might reveal, allowing programmers to pinpoint performance bottlenecks, memory leaks, and other critical issues that could affect the application’s efficiency and responsiveness. Using profiling tools is not merely optional; it’s a fundamental aspect of ensuring that Java applications run smoothly and effectively.
Built-in Java Profilers: JVisualVM and JConsole
JVisualVM and JConsole represent two of the crown jewels in the toolbox of any Java developer. Built into the Java Development Kit, both tools offer indispensable functionality for analyzing performance.
JVisualVM is particularly noteworthy for its comprehensive set of features. It provides a user-friendly graphical interface that allows developers to monitor application performance in real time. Through JVisualVM, users can observe CPU and memory usage, inspect threads, and even analyze heap dumps. This multifaceted overview empowers developers to make data-driven decisions and optimize their applications accordingly.
JConsole, on the other hand, leans more toward a straightforward approach. This command-line-based tool allows users to connect with Java applications running on the Java Virtual Machine (JVM). It excels at monitoring the performance of the applications via the Java Management Extensions (JMX). While it may lack the extensive graphical capabilities of JVisualVM, its utility in providing essential performance metrics should not be underestimated. Developers often appreciate how lightweight and responsive JConsole can be, making it an excellent choice for quick analyses.
Third-Party Profiling Tools
While built-in profilers are effective, third-party tools take Java profiling capabilities several notches higher. Here, we explore three reputable options from the field—YourKit Java Profiler, Java Mission Control, and NetBeans Profiler. Each of these tools brings a distinct flair to the process of performance analysis, promising to enrich the profiling experience.
YourKit Java Profiler
YourKit Java Profiler stands out for its robust feature set and versatility. This profiler not only provides standard CPU and memory profiling but also offers advanced analysis options such as allocations, call trees, and thread analysis. What makes YourKit a sought-after choice among developers is its ability to navigate complex performance problems swiftly. One defining characteristic is its lightweight agent architecture, which means less overhead when analyzing running applications. Because it minimizes performance impact, developers often find it an attractive option, especially in production environments.
YourKit allows developers to dive deep into problems without the added burden of degrading application performance.
However, it’s essential to keep in mind that while YourKit is a powerful tool, it is a commercial product requiring a license, which may deter some developers, especially those who are just starting out.
Java Mission Control
Java Mission Control is another heavyweight in the realm of Java profiling tools. It is best known for its analysis features, enabling developers to explore JVM performance like never before. One of its principal aspects is its ability to analyze profiling data captured using Java Flight Recorder, a tool built into the JVM for low-overhead data collection. This aspect allows developers to gather detailed insights without significantly affecting application performance.
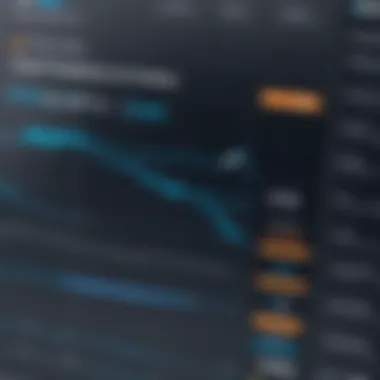
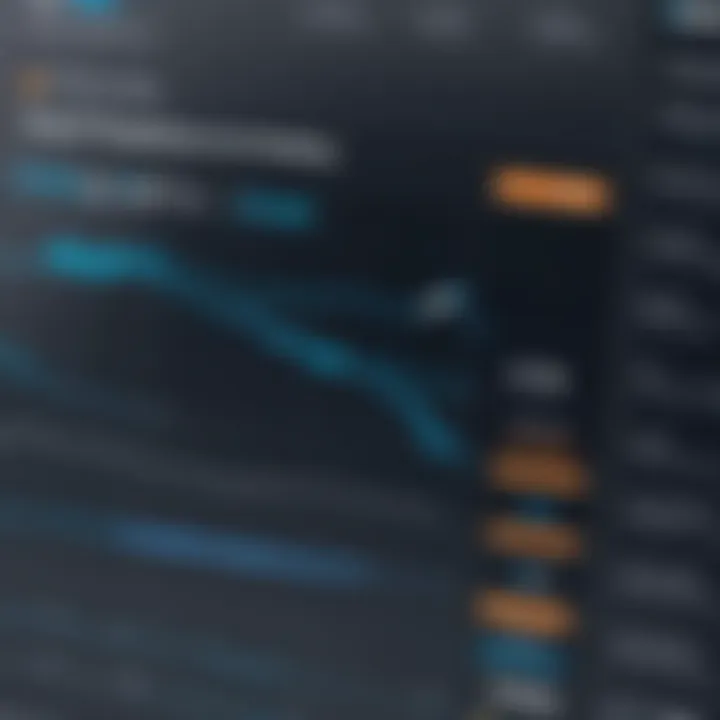
Moreover, Java Mission Control features a sophisticated user interface that helps in visualizing essential application metrics over time. Its ability to conduct root cause analysis effectively makes it an attractive choice for developers looking to optimize their code. Yet, one should be aware that navigating through its features may require a learning curve, potentially posing challenges for newcomers.
NetBeans Profiler
Next up is the NetBeans Profiler, which is part of the NetBeans Integrated Development Environment (IDE). This tool is particularly appealing for developers who prefer an integrated solution, as it seamlessly works within the NetBeans environment. One significant advantage is its easy accessibility; users can profile their applications without switching to a separate tool.
The profiling capabilities include monitoring memory usage, method execution time, and thread behavior, making it quite comprehensive for an IDE tool. However, it's worth noting that the primary audience for this profiler is those already invested in the NetBeans ecosystem, which could limit its adoption by developers using other IDEs.
Analyzing Profiling Results
Analyzing profiling results is akin to piecing together a puzzle to see the full picture of an application's performance. This phase is tremendously vital because it provides insights into how various components contribute to the overall behavior of a program. By interpreting these results effectively, developers can make informed decisions that lead to improved application performance, better resource management, and ultimately a more delightful user experience. Here are a few key considerations when diving into profiling results:
- Identify Bottlenecks: The primary objective is pinpointing parts of the application that slow it down. These can be methods that take too long to execute or blocks that wait on resources.
- Understand Resource Utilization: Profiling data can reveal how much CPU or memory an application uses. Understanding these metrics helps in making decisions on whether to optimize specific sections or refactor entire processes.
- Continuous Learning: The more a developer engages with the profiling process, the more adept they become at recognizing patterns and potential issues early on.
"The results you see in profiling are only as good as the questions you ask."
Interpreting CPU Utilization Data
To effectively interpret CPU utilization data, developers should first grasp how different methods consume CPU resources during their execution. This type of profiling provides a detailed picture of what the CPU is doing while the application runs. High CPU usage might indicate:
- Inefficient Algorithms: Look out for methods that exhibit poor complexity characteristics. For instance, if you have a sorting algorithm that runs in O(n^2) time, it’s probably a prime candidate for optimization.
- Excessive Context Switching: Too many threads vying for the CPU's attention can lead to context switching, which is not only costly but can also degrade performance significantly.
By concentrating efforts on this data, one can refine the code to utilize fewer CPU cycles, resulting in faster execution times and better scalability.
Understanding Memory Leak Patterns
Memory leaks can plague any Java application, but spotting them early can save developers from headaches later. During the analysis of profiling results, some key patterns to look for include:
- Unreleased Resources: Instances where objects are retained in memory because references are still held, even when not needed. A common example is when static collections grow over time without being cleared when data is no longer required.
- Long-lived Objects Keep Alive: If an object exists throughout the lifecycle of an application but is only needed temporarily, it can block garbage collection.
By identifying these leakage patterns, effective mitigation strategies can be devised, such as revisiting object lifecycles and ensuring resources are released appropriately.
Performance Optimization Strategies
Once the profiling data has been analyzed and interpreted, the next step is implementing performance optimization strategies that can transform findings into tangible improvements. Here are some strategies to consider:
- Refactor Inefficient Code: Take the time to restructure code that has been marked as a performance hindrance. Make sure to opt for algorithms with lower computational complexity.
- Adjust Threading Models: If the thread pool is overloaded, consider adjusting the number of threads to match the workload more closely or utilize asynchronous programming where applicable.
- Implement Caching: Use caching systems to store frequently accessed data and reduce the load on memory or databases.
In terms of best practices, remember to measure the improvements with additional profiling rounds to validate the effectiveness of your changes. Optimization is an iterative journey, not a one-off sprint.
Best Practices for Effective Profiling
When engaging in Java profiling, adhering to best practices becomes pivotal. A structured approach not only helps in producing coherent profiling data but also maximizes the benefits drawn from profiling. Here’s a closer look at important elements that can elevate the profiling process, making it more effective and insightful.
Establishing Baseline Metrics
Before diving into tuning and optimizing your Java applications, it’s essential to set a standard against which to measure performance. Establishing baseline metrics serves as a foundation for your profiling efforts. This involves collecting performance data in a stable state of the application, reflecting its typical operational characteristics. Without these baseline figures, any enhancements made could be misguided.
For instance, if you’re running a web application, take note of response times and resource usage under normal load. If you change anything later, you’ll have concrete data to track the differences. This allows you to observe the effects of your optimizations objectively, ensuring that you make informed decisions based on real data rather than instinct.
Incremental Profiling Strategy
Rather than conducting extensive profiling in one go — which might overwhelm both the profiler and the developers — it’s wise to adopt an incremental profiling strategy. This method focuses on profiling small sections of the application one piece at a time. The advantage here is twofold. First, it makes it easier to identify specific issues as they arise. Second, it's a lot less resource-intensive, helping avoid performance dips that might occur when profiling an entire application at once.
To implement this, start profiling critical components first, such as database interactions or resource-intensive algorithms. After identifying bottlenecks in these areas, work through the profiling of additional sections iteratively. This systematic approach can lead to clearer insights on how to optimize each part of the application effectively.
Maintaining Code Quality during Profiling
Profiling often requires modifying code — whether you're inserting profiling hooks or using specific profiling tools. However, it’s crucial to maintain code quality throughout this process. Messy code can lead to skewed profiling results, making it harder to pinpoint problems and enhancements.
Here are a few tips to keep your code in check:
- Version Control: Always use version control systems to manage your code. This allows you to revert changes that may compromise quality.
- Code Reviews: Engage in regular reviews. Other developers can spot potential issues induced by profiling insertions.
- Clean Up: After profiling, ensure the code is cleaned of any temporary changes. Unused profiling hooks or commented-out debug statements should be removed.
When done right, profiling should enhance rather than detract from code quality. In essence, by following these best practices, developers can ensure a balanced approach to profiling without compromising software integrity.
Common Pitfalls in Java Profiling
In the realm of Java profiling, it’s crucial to be mindful of common pitfalls that can derail your performance optimization efforts. The process, while fundamental for achieving great software efficiency, is sometimes fraught with misunderstandings that lead to suboptimal results or worse, performance degradation. By recognizing these pitfalls and understanding their implications, developers can navigate profiling with greater effectiveness, ensuring that the insights gleaned from analysis translate into real-world improvements.
Ignoring Profiling Results
One of the most significant missteps a developer can make is to simply ignore the profiling results. Even in a world buzzing with data, some still treat findings like an unnecessary footnote, assuming that the application runs fine and brushing aside alarming statistics. This oversight can stem from several reasons:
- Underestimating Performance Bottlenecks: Some developers may think, "It’s not that bad!" without really examining the numbers. They might ignore spikes in CPU usage or memory allocation patterns that, if left unaddressed, will snowball into more significant challenges down the road.
- Overconfidence in Code Quality: A rich history of successfully deployed applications might lull a programmer into a false sense of security. They may assume that since the previous versions ran smoothly, the current iteration couldn't possibly have issues worthy of concern.
- Disregarding Recommendations: When profiling tools present suggestions based on their findings, it can be easy to dismiss them. However, these tools are designed to highlight potential areas for improvement and should not be taken lightly.
Ignoring such findings can lead to performance degradation, increased resource consumption, and ultimately a poor user experience. It is imperative to keep an open mind and take the time to thoroughly analyze what the data presents.
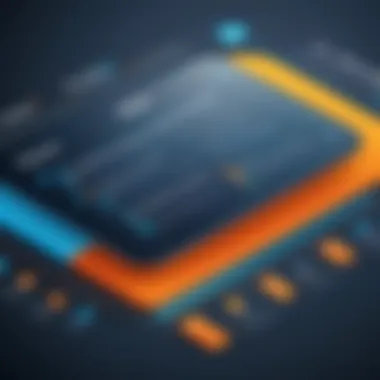
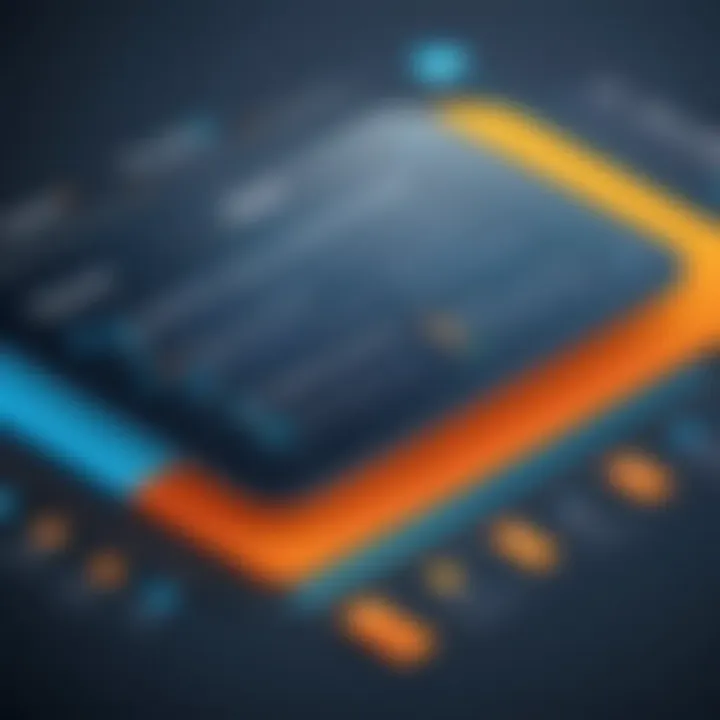
"Data is not just numbers. It’s a story begging to be told."
Over-Profiling Impact on Performance
Equally damaging is the practice of over-profiling, which refers to the relentless pursuit of data, often without a clear objective. While gathering comprehensive profiling information might seem like a good idea, it can actually hinder more than help:
- Performance Degradation: Some profiling tools introduce overhead that can impede application performance. When you continually profile an application, instead of gaining clarity, you might be slowing it down. It's essential to find a balance—profiling too frequently can lead to skewed results and inaccurate baselines.
- Data Overload: With more data comes greater responsibility. Over-profiling can lead to huge volumes of information that must be analyzed and interpreted. This can result in confusion and indecision. If one is drowned in numbers, the key insights can easily be obscured.
- Lack of Focused Optimizations: Profiling should be targeted to specific issues or phases of development. A scattershot approach may distract from addressing genuine performance vulnerabilities that need urgent attention.
Integrating Profiling into Development Workflow
Integrating profiling into the development workflow is like fitting a key into a lock; it’s essential for unlocking the full potential of Java applications. When profiling becomes a routine in your development practices, it reveals insights that can substantially refine application performance. It’s important not only to identify performance bottlenecks but also to make profiling a central component of your coding life.
The benefits of seamlessly incorporating profiling are numerous:
- Proactive Problem Identification: Instead of discovering issues after deployment, continuous profiling helps catch them during the development phase. This can save developers time and effort, while also enhancing user satisfaction.
- Enhanced Decision Making: By having real-time profiling data, developers are better equipped to make informed decisions regarding code optimization. This concrete data serves as a guide when fixing performance problems or experimenting with new features.
- Unified Team Understanding: If profiling is integrated into the workflow, all team members can easily access performance insights. This collective knowledge can lead to more innovative solutions and cohesive project advancements.
Continuous Performance Monitoring
In the ever-changing landscape of software development, continuous performance monitoring is the bedrock upon which robust applications are built. It ensures that as new features are added or modifications are made, performance is not compromised. By employing continuous performance monitoring, developers remain vigilant.
Monitoring should not be a one-off task. It is essential to adopt a cycle of measurement, analysis, and action, which can include:
- Regular Profiling Sessions: Schedule profiling throughout the development lifecycle, particularly after significant changes to the code, to catch anomalies early.
- Alert Systems: Utilize tools that can alert developers when performance thresholds are exceeded, making it possible to react promptly rather than retrospectively.
- Utilizing Metrics: Focus on performance metrics like CPU usage, memory consumption, and response times to identify trends that may indicate underlying issues.
This practice helps to cultivate performance awareness among developers. It’s not just about watching the numbers; it’s about understanding what they say about the application’s health.
Automating Profiling in / Pipelines
Automating profiling within CI/CD pipelines can bridge the gap between coding and deploying, ensuring performance is maintained without adding significant manual overhead. With frequent code changes in a CI/CD setup, manually tracking performance can quickly become a burden. Automation can take performance checks from a chore to a smooth, integrated process.
- Integration of Profilers into Build Steps: By incorporating profiling into each build step, any drastic changes in performance can be flagged immediately, allowing teams to address issues head-on.
- Automated Reporting: Automate performance reports as part of the build process. This enables developers to view profiling results without navigating through numerous tools, simplifying the workflow.
- Gating Pull Requests: Implement rules that prevent merging pull requests into the primary branch if performance declines beyond specified parameters. This can uphold the integrity of application performance standards.
By embedding profiling deeply into the development workflow, we not only create more efficient applications but we also promote a culture of performance awareness that resonates throughout the entire development process.
"Performance monitoring should not be considered an isolated activity, but part of the DNA of software development"
Thus, when profiling becomes habitual, it genuinely transforms coding competencies and leads to high-performance Java applications.
Case Studies: Successful Java Profiling
In the realm of Java development, case studies stand as a testament to the efficacy and application of profiling techniques. They serve not only to showcase successful outcomes but also to provide valuable insights into methodologies that can be utilized in your own projects. This section highlights how implementing profiling can lead to enhanced performance and smoother user experiences. Individuals facing overhead from memory issues or sluggish operations can glean techniques from real-world examples. Collectively, these case studies inform best practices, demonstrating both successes and pitfalls to avoid.
Profiling a Web Application
When embarking on a Java web application, the first item on the agenda should be identifying performance issues. A practical example can be seen with a popular e-commerce site facing latency problems during seasonal sales. By utilizing tools like YourKit Java Profiler, the developers captured CPU consumption patterns. Here’s what came to light:
- High CPU Usage: Peak load times showed that certain servlet operations consumed excessive CPU resources.
- Memory Bottlenecks: Active user sessions were not being released properly, leading to memory leaks.
Once the data was analyzed, the team employed a strategy to optimize their session management and decrease the strain on server resources. By isolating specific bottlenecks and addressing them with targeted code adjustments, they achieved a significant performance boost. Load testing before and after these modifications showed a reduction in response time by over 50%. This case highlights the importance of systematic profiling that reveals actionable insights, helping developers fine-tune their web applications effectively.
Optimizing a Backend Service
Another eye-opening case involves a financial institution that relied heavily on a Java-based backend service for transaction processing. As transaction volumes surged, the backend service began to slow down, causing frustration and potential revenue loss. Looking for solutions, the engineers decided to turn to JVisualVM for thorough profiling.
Here’s a breakdown of their findings:
- Thread Contention Issues: Many threads were competing for the same resources, leading to delays.
- Database Connection Leaks: Several connections to the database were not being closed promptly, creating a backlog in transaction processing.
Armed with this knowledge, the team refactored their database connection pooling configuration, ensuring that connections were properly managed. The thread contention issue was addressed by optimizing synchronization mechanisms. Post-implementation analyses showed marked improvements, with transaction processing speeds increasing by nearly 75%.
Through these case studies, it becomes evident that Java profiling transcends mere technical analysis. It is about creating an agile development environment, capable of navigating complexities with clarity.
Future Trends in Java Profiling
Exploring the future of Java profiling is essential for programmers and developers alike, as it directly impacts application performance and efficiency. With the continuous evolution of technology and changes in how software is developed and deployed, understanding the forthcoming trends in profiling can greatly enhance one's ability to create high-performance Java applications. Here, we will take a look at advancements in profiling techniques and how cloud computing is reshaping the landscape of Java profiling.
Advancements in Profiling Techniques
As we step deeper into the era of automation and optimization, profiling techniques are evolving rapidly. One significant advancement is the integration of machine learning algorithms with profiling tools. By utilizing predictive analytics, developers can gain deeper insights into application behavior under load and identify potential bottlenecks before they become problematic. This proactive approach helps prevent issues and ensures smoother user experiences.
Another noteworthy trend is the development of real-time profiling systems. Traditional profiling methods often require tests to be run in controlled environments, which can delay feedback. With real-time analysis, profiling can take place in production environments, providing immediate data on performance metrics. This shift allows developers to act swiftly, tweaking their applications based on live feedback.
"The future of Java profiling embraces a balance between precision and real-time responsiveness, aiming for a seamless integration into the development lifecycle."
Additionally, improved visualization tools are making it easier for developers to interpret profiling data. Graphical representations of performance metrics aid in quickly identifying performance hotspots. Instead of sifting through raw logs, users can now leverage dashboards tailored for intuitive understanding of the application performance.
Impact of Cloud Computing on Profiling
Cloud computing is playing a pivotal role in transforming how Java applications are profiled. As more organizations transition to cloud environments, the profiling landscape must adapt. One significant impact is the shift towards distributed profiling. In the past, profiling typically occurred within a single machine. However, with the rise of microservices and serverless architectures, profiling needs to encompass a more extensive ecosystem. Tools that can analyze performance across multiple services in real-time are becoming indispensable.
This distributed approach not only gives a broader view of application performance but also highlights interactions between different services, uncovering issues that may not be evident in isolation. Moreover, as cloud environments often come with dynamic scaling capabilities, profiling tools must be able to provide insights in varying workloads and help developers understand how their applications behave under different conditions.
Furthermore, security considerations are becoming paramount in the cloud profiling space. As applications become more complex and multitenant, profiling tools will need to weave security monitoring with performance evaluations, ensuring both application security and efficiency.
In summary, staying ahead of the curve in Java profiling requires an understanding of where technology is headed. With advancements like machine learning integrations, real-time analytics, and cloud-focused profiling methodologies, developers can better equip themselves to tackle the challenges of performance optimization in their Java applications.