Mastering Java OOP Concepts for Technical Interviews
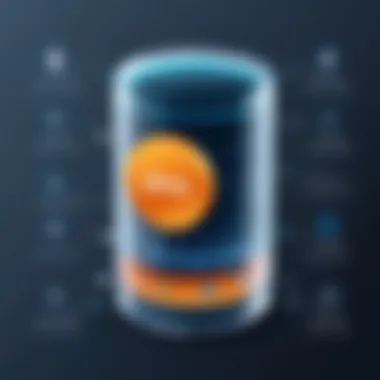
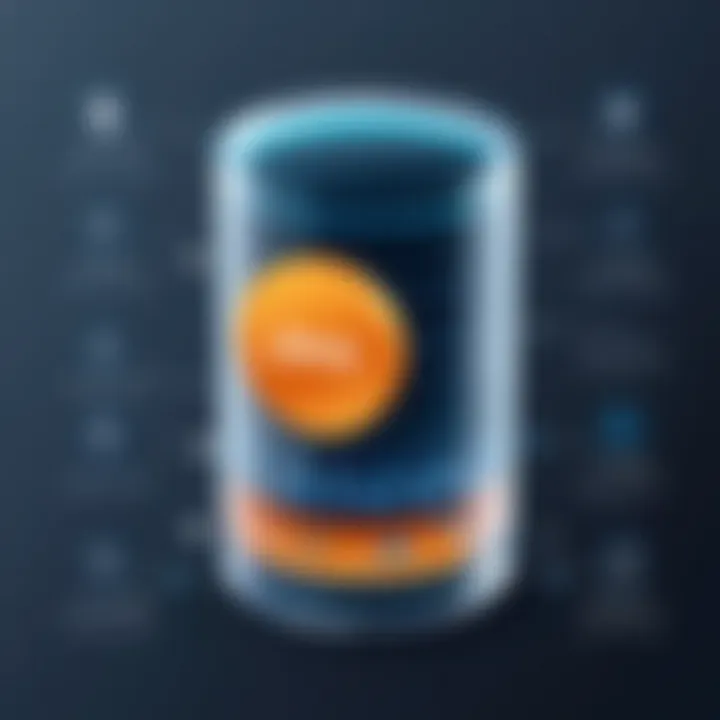
Intro
Navigating the realm of Object-Oriented Programming (OOP) with Java is no small feat. As students and budding programmers gear up for interviews, it becomes imperative to grasp the core concepts that underpin Java OOP. Having a firm foundation will not just make you a strong candidate but will also allow you to think critically about problem-solving in Java.
Understanding OOP involves breaking down its four pillars: encapsulation, inheritance, polymorphism, and abstraction. These principles are not merely academic; they form the backbone of Java programming and will likely surface in one form or another during interviews.
So, let’s dive deep into these concepts, explore their relevance, and provide instances that can aid your learning experience.
Preamble to Programming Language
History and Background
Java first hit the stage in the mid-1990s, introduced by Sun Microsystems. Originally intended for interactive television, it soon found its fame in web applications. The design of Java prioritized portability, allowing programs to run on any device without modification via the well-known phrase "Write Once, Run Anywhere". While it was a radical idea then, this principle is foundational in today's software development landscape.
Features and Uses
Java is often celebrated for its simplicity and versatility. It boasts features like:
- Platform independence: Thanks to the Java Virtual Machine (JVM), Java applications can run on any operating system.
- Strongly typed: Java’s type-checking at compile time can help reduce runtime errors.
- Rich API: There’s a massive collection of libraries and frameworks that enhance development speed.
From web development frameworks like Spring to Android app development, the language finds its applications in numerous domains. Businesses turn to Java not just for these reasons but also due to its scalability and maintainability.
Popularity and Scope
In recent years, Java's popularity continues to soar. It remains a preferred choice for enterprise-level applications. According to various surveys, Java consistently ranks among the top programming languages. As industries evolve and focus on data processing, Java also adapts through frameworks like Apache Hadoop.
"Java is the most popular programming language in the world for building applications, both small and large."
Looking at recent trends, learning Java opens doors to opportunities in software engineering, mobile development, and more.
Basic Syntax and Concepts
Variables and Data Types
Understanding variables and data types is like learning your ABCs when it comes to programming. In Java, every variable has a data type, which dictates its nature. A few primary categories include:
- Primitive Types: Such as , , , etc.
- Reference Types: Which involve classes, arrays, and interfaces.
Each data type brings its own properties and usage. For instance, an holds whole numbers, whereas a is for decimals, making your choice pivotal based on the context.
Operators and Expressions
To manipulate data, Java provides a variety of operators, including:
- Arithmetic (e.g., +, -, *, /)
- Relational (e.g., ==, !=, >, )
- Logical (e.g., &&, ||, !)
Expressions combine these operators to perform calculations or evaluations, forming the very logic of your programs.
Control Structures
Control structures guide the flow of your program. Key types include:
- Conditional Statements: , , and .
- Loops: , , and .
These structures help in making decisions and repeating actions, crucial for creating dynamic applications that react to varying conditions.
Advanced Topics
Functions and Methods
Functions in Java, termed as methods, play an instrumental role in defining the actions your objects can undertake. Understanding how to declare and invoke methods effectively is critical. A method can be as simple as returning a sum of two integers, or as complex as processing user input. Below is a simple method declaration:
Object-Oriented Programming
At the heart of Java lies OOP—a paradigm that utilizes objects to model real-world concepts. By learning core OOP principles, candidates can convey their understanding of software design patterns that employers often look for. The four pillars previously mentioned will frame your understanding of how objects interact.
Exception Handling
Now and again, things might go awry while coding. Java offers a structured approach to handle exceptions gracefully. This involves using , , and blocks to manage errors without crashing your program. Mastering exception handling not only makes your code robust but also highlights your preparedness to tackle unexpected issues.
Hands-On Examples
Simple Programs
Engaging in straightforward coding challenges can solidify your skills. Start with simple programs such as a basic calculator or a number guessing game. Each mini-project serves as practice in applying concepts learned.
Intermediate Projects
Once comfortable, branch out to intermediate projects like creating a simple banking system or a personal library inventory. Projects like these leverage multiple OOP principles and provide a more comprehensive experience.
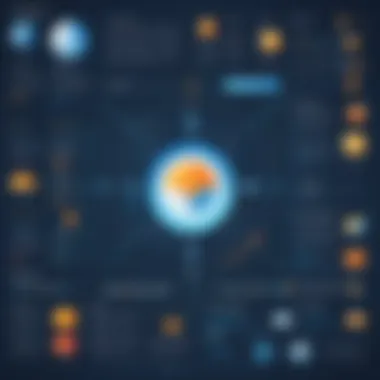
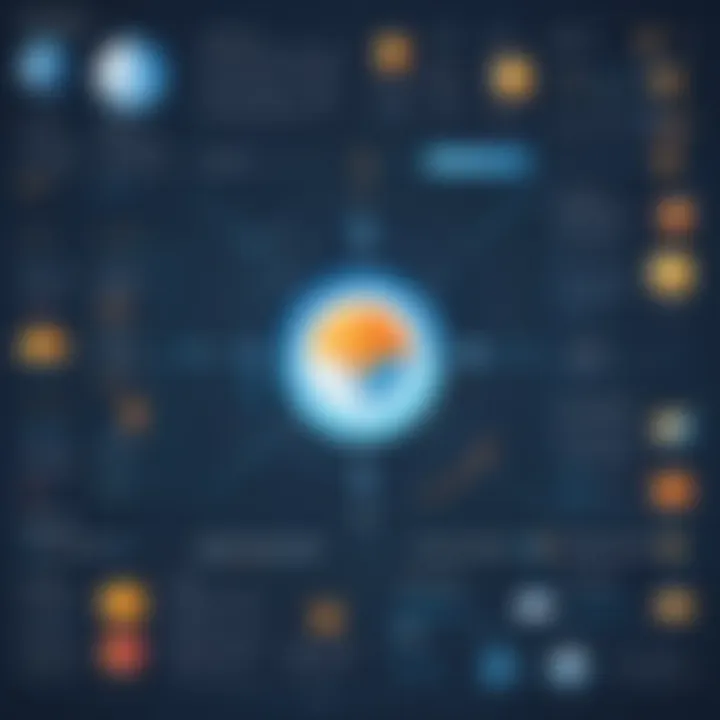
Code Snippets
Here’s a snippet that exemplifies encapsulation in action:
Resources and Further Learning
Recommended Books and Tutorials
- Effective Java by Joshua Bloch
- Java: The Complete Reference by Herbert Schildt
Online Courses and Platforms
- Coursera
- Udacity
Community Forums and Groups
In summary, mastering Java OOP concepts sets the stage for success in technical interviews. By absorbing theoretical knowledge combined with practical applications, candidates can confidently articulate their solutions, leaving a lasting impression. Armed with these tools and insights, you should be well on your path to interview readiness.
Understanding Java OOP
Diving into the world of Java Object-Oriented Programming (OOP) is vital for anyone gearing up for software development and technical interviews. Java OOP is more than just a coding style; it lays the foundation for creating robust and maintainable code. Understanding these concepts can help as a compass during real-world scenarios and interviews alike.
One of the biggest benefits of mastering Java OOP principles is that it nurtures the ability to conceptualize real-world problems into code. By doing this, candidates can demonstrate their problem-solving skills, which is often a focal point in interviews. It’s not merely about knowing the syntax or the theory; it’s about applying these principles in practical situations that truly shines in interviews.
Moreover, a grasp on Java OOP makes it easier to collaborate with other developers. Being familiar with common concepts like encapsulation and inheritance allows for smoother teamwork when building software solutions. Additionally, understanding Java OOP can aid in navigating frameworks and libraries that rely heavily on these principles. Having this knowledge puts candidates in a favorable position, equipping them to tackle interview questions with confidence and precision.
Defining Object-Oriented Programming
Object-Oriented Programming (OOP) refers to a programming paradigm that uses "objects" to represent data and methods. These objects consist of both state (attributes) and behavior (methods). In the context of Java, OOP promotes modularity and code reusability, leading to easier maintenance and development.
At the heart of OOP is the idea of encapsulating the data and operations together, which paves the way for organized and more understandable code. It’s similar to how beings have their unique traits and behaviors, making it a natural and relatable approach to coding.
Key Features of Java OOP
Encapsulation
Encapsulation is all about bundling the data and methods that operate on that data within a single unit or class. This characteristic allows controlling access to the internal state of an object, hence keeping the data safe from unauthorized access.
- Why Encapsulation? It creates a clear separation between the interface and the implementation. This can be a real game changer when adjusting parts of the code without affecting other components.
One unique feature of encapsulation is its utilization of access modifiers, such as private and public, to define visibility. It prevents external code from altering the state of an object directly, which could lead to erratic or unpredictable behavior in large systems. It's worth noting that while encapsulation promotes safeguard, it may sometimes lead to complexity when designing interfaces.
Inheritance
Inheritance allows classes to inherit properties and behaviors of other classes, fostering a hierarchical relationship between them. This promotes code reuse, reducing redundancy and encouraging the development of more generalizable code. A suitable analogy here is the family tree, where children inherit traits from their parents.
- Key characteristic: It empowers the creation of a base class that can encompass common functionality, while specialized subclasses can add or modify features without rewriting code.
A caveat of inheritance is tightly coupled code. If changes are made to a parent class, it might unintentionally affect child classes, so it’s vital to tread carefully when making adjustments.
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon, even though the method calls look the same. This feature can be particularly empowering in interviews, as it highlights adaptability and flexibility in coding practices.
- Key characteristic: It can be categorized into compile-time polymorphism (method overloading) and runtime polymorphism (method overriding).
A unique aspect of polymorphism is its capacity to enhance code readability and facilitate future expansions. However, this can also introduce complexity, especially in understanding which method is being executed at runtime, necessitating a deeper grasp of the system.
Abstraction
Abstraction focuses on hiding complex realities while exposing only the necessary parts of an object. It essentially allows developers to reduce complexity by emphasizing what an object does instead of how it does it. Think of it like driving a car—most drivers don’t need to know the intricate workings of the engine; they just need to know how to operate it.
- Why Abstraction? It simplifies interaction with complex systems by offering a simpler interface through abstract classes or interfaces. This can lead to cleaner code and quicker project advancement.
One distinctive feature is that abstraction often employs both abstract classes and interfaces to ensure a relevant structure. The downside, however, lies in the possible upfront work required to set up these abstractions, which some may find challenging.
Common OOP Principles in Java
Java's Object-Oriented Programming (OOP) principles serve as the backbone for developing robust, maintainable, and reusable code. Understanding these principles is not just useful; it's essential for anyone preparing for technical interviews. These fundamentals give a framework for thinking about software design, and they provide the guidelines that help in structuring applications clearly and effectively.
The key elements of OOP—encapsulation, inheritance, polymorphism, and abstraction—work in harmony to simplify complex problems. This section will explore each principle, providing clarity on their roles, advantages, and how they interconnect.
Encapsulation Explained
Encapsulation is primarily about bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class. This approach offers a measure of protection to the data from unauthorized access, effectively reducing vulnerabilities in code.
Access Modifiers
Access modifiers are crucial in achieving encapsulation. They define the visibility and accessibility of class members. The primary modifier types are , , , and the default (package-private) access.
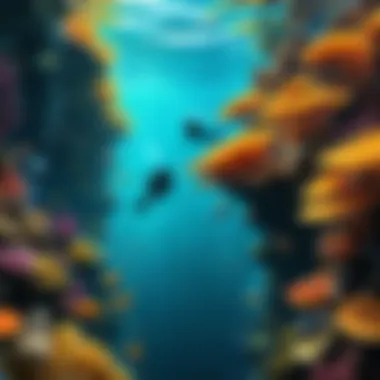
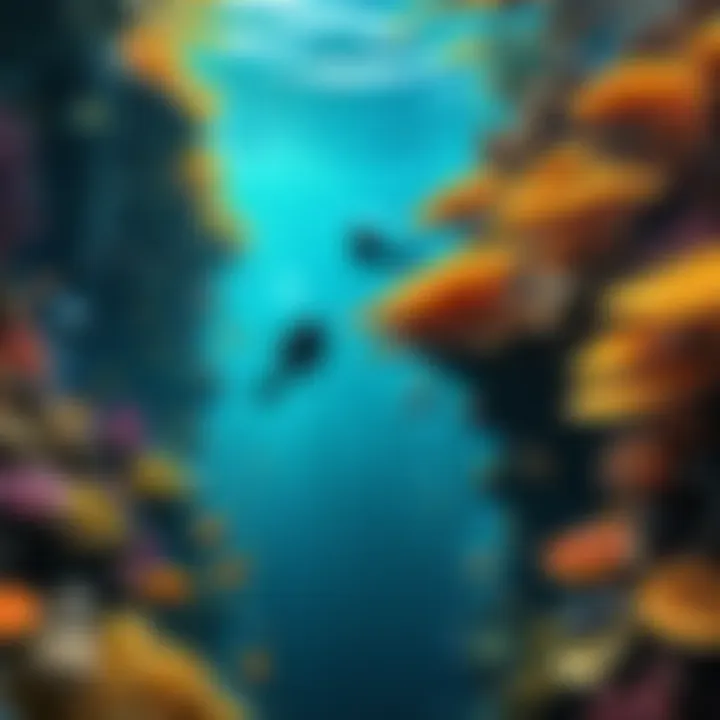
One of the standout characteristics of access modifiers is that they allow for detailed control over who can access data within a class. This feature makes them a preferred choice in our discussion. For example, if a class's variable is marked as , it cannot be accessed directly from outside the class, ensuring data integrity.
However, this ruling has its disadvantages. If not managed properly, it could lead to code that’s overly restrictive or difficult to navigate.
Getters and Setters
Getters and Setters are methods that allow you to retrieve and modify the values of private properties. They act as intermediaries between the class's internal data and the outside world.
In our context, one key characteristic of getters and setters is that they preserve encapsulation, as they provide controlled access rather than direct data exposure. They are popular choices among developers because they enable validation of new data before it's set, ensuring data integrity.
Yet, there’s a flip side. Relying too heavily on getters and setters can lead to cumbersome code structures, making the code base harder to maintain in the long run.
Inheritance in Java
Inheritance is a powerful feature that allows a new class (derived or child class) to inherit properties and behaviors (methods) from an existing class (base or parent class). This principle is fundamental to promoting code reuse, avoiding redundancy.
Single and Multiple Inheritance
Java supports single inheritance, meaning that a class can only inherit from one direct superclass. This design choice simplifies the inheritance tree and avoids complexities linked to multiple inheritance, such as the
Interview Questions Related to OOP Concepts
In the context of preparing for technical interviews, understanding OOP (Object-Oriented Programming) concepts is paramount. Interview questions centered around OOP principles gauge not just theoretical knowledge but the ability to apply these concepts in practical scenarios. As candidates navigate through the interview landscape, showcasing a solid grasp of OOP can set them apart from the pack. It's crucial for demonstrating one's problem-solving skills and coding proficiency. This section will cover basic and advanced OOP questions, along with practical problem-solving tasks, setting a comprehensive framework for aspiring programmers.
Basic OOP Questions
Explain the four pillars of OOP.
The four pillars of Object-Oriented Programming are encapsulation, inheritance, polymorphism, and abstraction. Each pillar plays a unique role in shaping how applications are designed and implemented.
- Encapsulation secures data by restricting direct access to some of an object's components, which enhances data integrity.
- Inheritance allows new classes to inherit properties and methods from existing classes, promoting code reusability.
- Polymorphism enables methods to do different things based on the object it is acting upon, making programs more flexible.
- Abstraction simplifies complex systems by presenting only the necessary details while hiding implementation complexities.
All in all, these pillars collectively create a powerful framework that makes Java a popular choice in software development. Understanding their core characteristics and interconnections is essential for clear communication during interviews. Demonstrating awareness of these principles can empower candidates to effectively tackle complex problems, thus providing a meaningful edge in interviews.
What is encapsulation with an example?
Encapsulation refers to the technique of bundling the data (attributes) and methods (functions) that work on the data into a single unit or class. A classic example is a class for a bank account. In such a class, sensitive data like the account balance would be private, while public methods would allow safe manipulation of that data, like depositing or withdrawing money.
This method of bundling not only provides a way to protect data but also adds a layer of abstraction, making the interface clear to the user of the class. Encapsulation promotes control over the data by regulating how it is accessed. It benefits code maintainability and reduces the risk of accidental interference with the internals.
Advanced OOP Questions
Discuss method overriding and its significance.
Method overriding is a feature that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This reinforces the concept of polymorphism, as it enables a call to an overridden method to resolve at runtime, depending on the object's instance rather than its reference type.
In this example, the class overrides the method of the class. This capability of overriding allows designers to implement concrete behaviors in subclasses while maintaining a common interface through the superclass. Candidates who effectively discuss this feature during interviews demonstrate not only their understanding of inheritance but also a nuanced grasp of leveraging polymorphism, which is vital in real-world programming.
How does Java support polymorphism?
Java supports polymorphism through method overloading and method overriding. With polymorphism, the same method can behave differently based on the context in which it is called. In Java, two types of polymorphism emerge: compile-time (or static) and runtime (or dynamic).
- Compile-time polymorphism happens when the method signature differs within the same class, enabling multiple methods with the same name but different parameters.
- Runtime polymorphism arises through method overriding, where the method invoked is determined by the object's actual type during execution.
This dual approach enhances flexibility and capability within Java programs, enabling them to act differently based on varying data types. When candidates discuss how Java implements polymorphism, it illustrates their ability to design adaptable systems—an undeniably attractive quality for any hiring manager.
Practical OOP Problem-Solving Questions
Design a class for a library system.
To design a class representing a library system, consider how various entities interact. A potential starting point could be a class that holds a collection of objects. Here’s a simple model:
This approach captures the relationship between a library and its collection. Candidates can showcase their understanding of OOP principles through such designs, illustrating encapsulation by having private attributes and public methods, alongside the use of collections.
Create a simple interface for a vehicle.
In Java, an interface can define a contract for classes without providing implementation details. For instance, consider a simple interface for a vehicle:
This demonstrates abstraction by outlining vehicle behavior without restricting specific implementations to cars, bikes, or trucks. It promotes design flexibility. Understanding and creating interfaces is a crucial skill in Java programming that interviewers look for, as it reflects a candidate's comprehension of OOP and design patterns.
Ultimately, showcasing the ability to not only understand but also apply OOP concepts through practical examples can significantly bolster a candidate's interview performance in software development roles.
Common Pitfalls in Java OOP Interviews
In the world of technical interviews, especially those focusing on Java Object-Oriented Programming (OOP), candidates can encounter a variety of challenges. These challenges, or common pitfalls, can often be the difference between success and failure. Understanding these potential missteps is crucial, as it allows candidates to navigate through the interview landscape with confidence. This section highlights key areas where candidates might stumble and offers insights on how to tackle these issues.
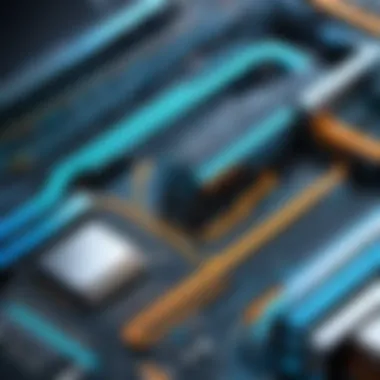
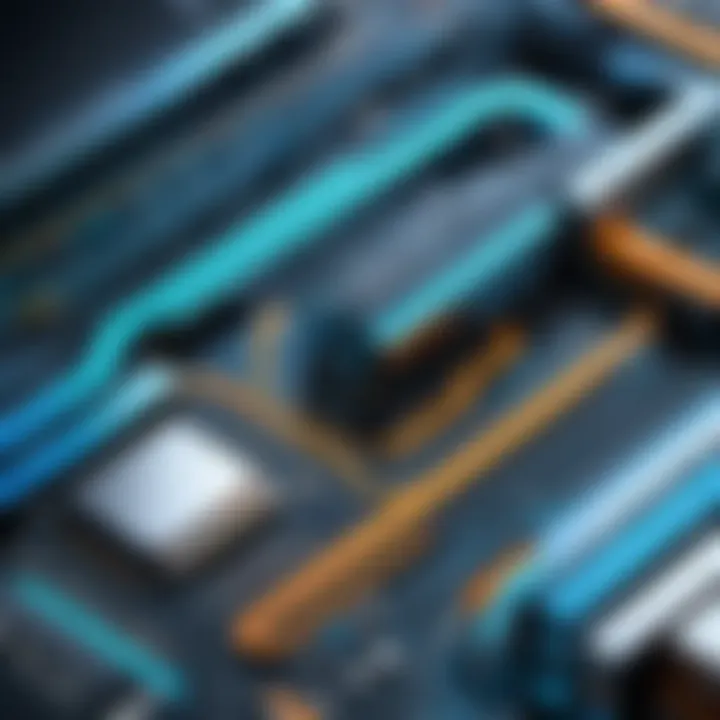
Misunderstanding Key Concepts
One of the most frequent traps candidates fall into during interviews is the misunderstanding of fundamental OOP concepts. It’s easy to mix up encapsulation with abstraction or inheritance with composition. Each of these concepts plays a distinct role in shaping an object-oriented design.
Importance of Clarity: Failing to explain these concepts clearly can raise red flags for interviewers. Suppose a candidate confuses encapsulation, which is about bundling data and methods that operate on that data, with abstraction, which focuses on simplifying complex realities by modeling classes based on their essential properties and behaviors. In such a case, it might suggest a lack of comprehensive understanding, which interviewers are keen to avoid.
To prevent these misunderstandings:
- Practice articulation: Use simple language to explain these concepts to someone not familiar with them, which helps clarify your own understanding.
- Visual aids: Sketch class diagrams or flow charts if possible. Visualizing how these components interact can deepen comprehension.
"Understanding the core concepts of OOP is the backbone of effectively communicating and demonstrating your knowledge during interviews."
Neglecting Practical Examples
Another major pitfall is the tendency to overlook practical examples that demonstrate the application of OOP principles. Theory is important, but without practical illustrations, it can fall flat. When asked about polymorphism, for instance, a candidate might explain the definition but fail to give a real-world analogy or code sample to highlight its use.
Elevate Your Response: To avoid this, candidates should prepare a repertoire of practical examples from their own experience or common scenarios. Here’s how to approach this:
- Relate to real-world applications: For instance, discussing a class structure that represents different types of vehicles (car, bike, truck) can easily showcase inheritance and polymorphism in action.
- Hands-on coding: Share snippets of code you’ve written, pointing out how you’ve utilized encapsulation or abstract classes in a project. This demonstrates not just theoretical knowledge but practical application.
Thinking through how to frame your examples can make a significant difference in how your responses are received. Those who indulge in practical scenarios tend to leave a more lasting impression on interviewers, showcasing that they not only know the theory but can also apply it effectively.
In summary, avoiding misunderstandings of key concepts and incorporating practical examples can greatly enhance a candidate's performance in Java OOP interviews. Thus, candidates must engage with these areas to showcase their true potential and readiness for the challenges that lie ahead.
Tips for Interview Success
Securing a job in technology, particularly in software development, hinges substantially on how well you grasp Java OOP concepts. Not only does a deep understanding bolster your confidence, but it also facilitates clearer communication during interviews. This section aims to provide key strategies for presenting your knowledge effectively, ensuring you stand out from the crowd.
Articulating OOP Concepts Clearly
When it comes to interviews, effectively communicating your understanding of Object-Oriented Programming in Java can be a game changer. It's not just about knowing the definitions; it's about how you convey this knowledge. Using clear and concise language, while avoiding jargon, helps bridge the gap between technical complexity and interviewer comprehension.
For example, instead of simply saying that encapsulation protects the state of an object, you might say:
"Encapsulation is like a protective shell around my object, keeping its data safe from unauthorized access. I expose only what I need through getter and setter methods."
This analogy not only clarifies your understanding but also makes your explanation relatable. While preparing, aim to practice explaining these concepts aloud; it mimics the interview environment. You can even record yourself to identify areas for improvement.
Practicing Coding Examples
In the technical interview realm, practical application is paramount. Employers often present coding challenges to assess not just your understanding of concepts, but your ability to apply them under pressure. Regular practice with coding examples ensures you're ready for these situations.
Start with basic exercises, such as:
- Implementing a class to model a bank account: include methods for deposits and withdrawals that demonstrate encapsulation.
- Creating an interface for drawing shapes that can be implemented by various classes like Circle and Square, showcasing abstraction.
As you gain confidence, tackle more complex scenarios, like designing a simple e-commerce system. This hands-on practice helps solidify your knowledge and provides concrete examples to discuss in your interview. Furthermore, consider using online platforms like LeetCode or HackerRank to find specific challenges focused on OOP principles.
Researching Company-Specific OOP Applications
Understanding how a particular company employs OOP principles can give you a significant leg up in interviews. Each organization may have unique implementations that cater to their business needs. Prior to your interview, dedicate time to research the company, focusing on how they utilize Java and OOP concepts.
This might involve:
- Reading through the company’s job postings for hints about required skills.
- Exploring their official website or tech blogs to find relevant case studies or projects.
- Engaging on platforms like Reddit or Facebook to seek insights from current or past employees about their experiences.
By grasping how the company leverages OOP, you can tailor your responses to reflect that knowledge, demonstrating not just awareness but also genuine enthusiasm for contributing to their projects.
The more informed you are about a company's practices, the greater your ability to align yourself with their goals during an interview.
In summary, unifying clear communication, practical coding experience, and targeted research not only prepares you for interviews but enhances your overall understanding of Java OOP concepts. With these tips, you'll be well on your way to making a strong impression.
Resources for Further Learning
Understanding Java Object-Oriented Programming (OOP) isn't merely about grasping the concepts; it's also about continuous learning. The landscape of technology changes rapidly and keeping abreast of the latest information is vital. This section showcases resources that will support your journey into the depths of OOP in Java. By utilizing these materials, candidates can reinforce theoretical knowledge, gain practical insights, and feel more prepared for interviews.
Books on OOP in Java
Books are often a great starting point when diving deep into a subject. For OOP in Java, there are countless texts, but a few stand out due to their thorough explanations and practical examples:
- Head First Java by Kathy Sierra and Bert Bates: This book is not just about theory; it combines illustrations with engaging explanations to keep readers fueled. Although it doesn’t stick strictly to OOP principles, it surely lays down a strong foundation in Java.
- Effective Java by Joshua Bloch: This book moves into the advanced territory, providing a nuanced approach with best practices and design patterns in Java that equip developers to apply OOP principles effectively.
- Java: The Complete Reference by Herbert Schildt: A behemoth of a book that covers not only OOP but the entire Java language itself. It's like a treasure chest of information, making it incredibly useful for beginners and seasoned developers alike.
These books not only provide detailed knowledge of OOP principles but also enhance problem-solving skills.
Online Courses and Tutorials
In a world gone digital, online courses have dramatically changed how one can learn Java OOP concepts. A few noteworthy platforms include:
- Coursera: Offers a variety of courses from top universities. Courses like "Object Oriented Programming in Java" provide structured content and flexible schedules.
- Udemy: A treasure trove of courses, many of which focus on practical skills. Look for courses such as "Java Programming Masterclass" which dive deep into OOP and provide exercises to solidify concepts.
- Codecademy: Known for interactive learning, Codecademy’s Java course incorporates OOP principles through hands-on tasks that help reinforce learning by doing.
These platforms often come with quizzes and projects, making it easier to apply what you learn directly.
Community Forums and Discussion Boards
The importance of community cannot be overstated. Engagement with fellow learners and developers can refine one's understanding and provide fresh perspectives. Some notable forums include:
- Stack Overflow: An invaluable resource for troubleshooting issues. You can ask specific questions related to Java OOP concepts or search the vast database for similar queries.
- Reddit: Subreddits like r/learnjava and r/java are gems where users share experiences, tips, and resources. Many seasoned developers are willing to help newcomers navigate the challenges of learning Java OOP.
- Facebook Groups: There are numerous groups dedicated to Java programming. Engaging in discussions here can provide support and practical advice from peers who were once in your shoes.
Each of these resources not only builds capability but also fosters a community around your learning journey.
Making the most of these resources will ensure that you are not just equipped for interviews but really develop a deep understanding of object-oriented programming in Java. Knowledge is a dynamic and ongoing process; keep learning and stay curious.
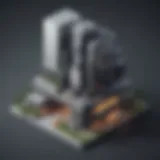
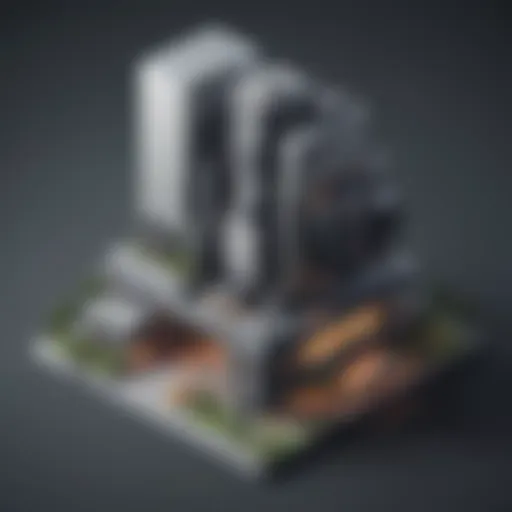