Mastering Java Multi-Threading: A Complete Guide
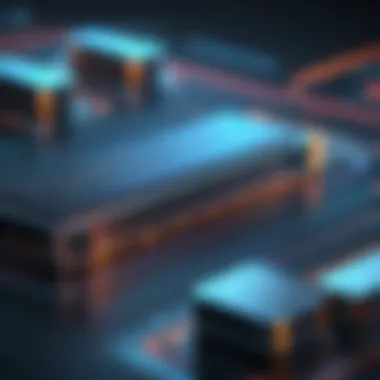
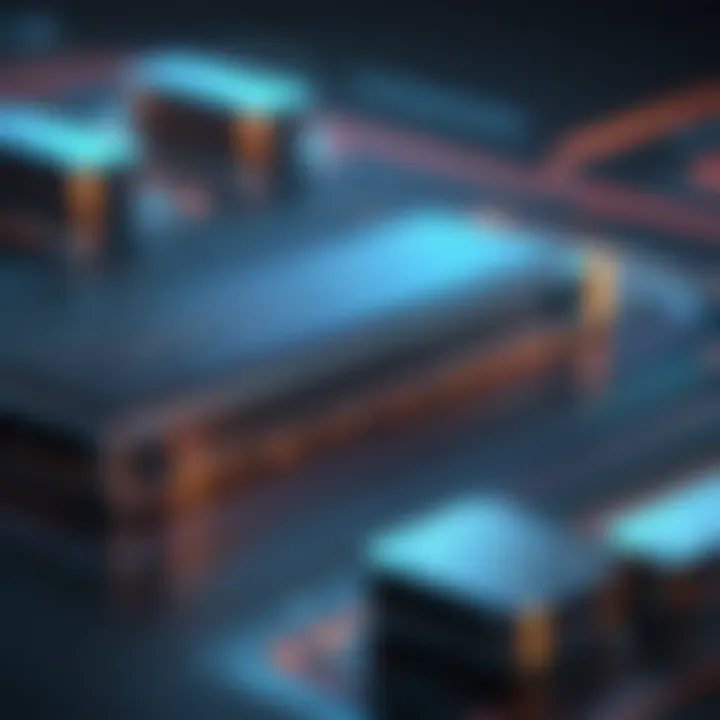
Intro
Java multi-threading is a significant facet of programming that supports concurrent task execution. Having a firm grasp on this topic can greatly enhance one's ability to write efficient and responsive applications. This section will set the stage for deeper exploration into the characteristics of Java threads, their operational phases, and synchronization methodologies.
History and Background
Java was created by Sun Microsystems and released in 1995. The goal was to provide a platform-independent language with a focus on ease of use and efficiency. Over the years, Java has evolved, introducing multi-threading capabilities to manage multiple tasks simultaneously. This feature allows developers to build applications that can perform several operations at once, making Java particularly useful for server-side applications, games, and real-time data processing.
Features and Uses
Multiple features streamline Javaโs multi-threading abilities:
- Lightweight Processes: Threads in Java are lightweight, enabling quick context switching and effective resource sharing.
- Synchronization: Java offers built-in synchronization methods to manage shared resources and prevent inconsistencies during concurrent executions.
- Thread Classes and Interfaces: The class and interface provide ways to implement and utilize threads easily.
The application of Java multi-threading spans various domains. Web servers utilize it to handle multiple requests concurrently. Games implement it to manage graphics rendering and user interactions simultaneously. Data processing applications exploit multi-threading to parse large datasets in a fraction of the time other single-threaded applications would require.
Popularity and Scope
Java remains one of the most popular programming languages in the world. Its versatility, cross-platform capabilities, and robust libraries for multi-threading contribute to its ongoing relevance. According to Wikipedia), Java continues to rank among the top programming languages, often used in academic settings and industries alike. The scope for Java's multi-threading is vast, extending across various platforms such as enterprise applications, mobile applications, and even cloud computing.
As we navigate through this tutorial, understanding the key components of Java multi-threading will be essential for mastering real-world applications and optimizing your programming tasks.
Preamble to Multi-Threading in Java
Multi-threading is a fundamental aspect of programming in Java, enabling applications to perform multiple tasks simultaneously. This is particularly important in todayโs computing landscape, where responsiveness and efficiency are critical factors in user experience. Understanding multi-threading allows programmers to build more robust applications that can make better use of system resources.
Definition of Multi-Threading
Multi-threading refers to the concurrent execution of two or more threads within a single process. Each thread represents a separate path of execution. This means that a Java program can perform different tasks at the same time without waiting for one task to complete before starting another.
In Java, a thread is a lightweight subprocess, the smallest unit of processing that can be scheduled by the operating system. Threads share the same memory space but operate independently, which makes multi-threading a powerful feature.
Importance of Multi-Threading
The significance of multi-threading in Java can be observed in various scenarios:
- Improved Performance: Multi-threading allows for better CPU utilization as multiple threads can run in parallel. This is particularly beneficial for CPU-bound tasks.
- Responsiveness: Applications that require regular interaction with users, such as GUI applications, benefit from multi-threading. It helps keep the user interface responsive while executing complex background operations.
- Resource Sharing: Threads share the same resources of the process, which leads to less memory overhead compared to creating separate processes.
- Simplified Program Structure: Using multi-threading can simplify the structure of a program by allowing asynchronous execution and a clean separation of concerns.
Understanding multi-threading opens the door to various programming opportunities. It optimizes operations and enhances user experiences, making it an essential skill for Java developers.
"Multi-threading is essential for building high-performance, responsive applications in today's software development landscape."
By mastering multi-threading, programmers can create applications that not only function effectively but also meet the high demands of modern users.
Understanding Threads
Understanding threads is crucial in the realm of Java programming. Threads allow for the concurrent execution of code. This means that multiple tasks can be handled at once, which is essential for applications that require high performance and efficient resource management. In today's technology landscape, where user demands are continuously increasing, understanding how to manage threads effectively becomes a vital skill for developers.
By recognizing threads and how they operate, programmers can improve the responsiveness and speed of their applications. Moreover, thread management helps prevent common problems such as resource contention and thread starvation. As we delve into the specifics, we will explore what a thread is, how to create them, and the various methods we can use with threads to unlock their full potential.
What is a Thread?
A thread, in simple terms, is a lightweight process that is part of a larger process. Each thread has its own execution stack and program counter. Threads within the same process share memory and resources, making them efficient for tasks that require interaction. This efficiency is why multi-threading is favored in application design, particularly when we want to execute multiple tasks simultaneously or when handling network requests.
When a thread runs, it can be in one of several states, including running, waiting, or terminated. Understanding these states is fundamental to controlling thread behavior and optimizing performance.
Creating Threads in Java
Creating threads in Java is straightforward, and there are two primary methods to do so: using the Thread class or implementing the Runnable interface. Each approach has its own distinct benefits and use cases.
Using the Thread Class
Using the Thread class is one of the earliest methods to create threads in Java. By subclassing the Thread class, the programmer can override the method, which contains the code that will execute when the thread starts. The key characteristic here is simplicity. It allows developers to create a new thread easily by instantiating the Thread object and calling its method.
This method is popular because it is straightforward and easy to understand. However, there are disadvantages. One significant downside is that Java allows a class to extend only one class at a time. This means if a developer needs to inherit from another class, it cannot extend the Thread class. As a result, this approach is suitable for simpler applications but may not be ideal for more complex situations requiring flexibility.
Implementing the Runnable Interface
Implementing the Runnable interface provides more flexibility compared to extending the Thread class. By implementing the interface, a class can define the method that contains the task to be performed by the thread. This approach allows the class to extend another class while still being able to run in its own thread.
The key characteristic of using the Runnable interface is its versatility. It can encapsulate the task independently from the thread management, making it suitable for complex applications. The unique feature here is that it promotes better separation of concerns. However, one must remember that the Runnable interface does not allow for thread control directly, which sometimes requires additional management.
The choice between using the Thread class or the Runnable interface often depends on the specific needs of an application.
In summary, understanding threads and their creation methods is a foundational aspect of Java multi-threading. It enables developers to harness the capabilities of concurrent execution, enhancing overall application performance.
Thread Lifecycle
Understanding the Thread Lifecycle is essential in mastering Java multi-threading. A thread in Java goes through several states throughout its lifecycle. These states determine how a thread behaves and how it interacts with other threads in a multi-threaded environment. Knowing these states helps developers in troubleshooting issues, optimizing performance, and designing efficient applications.
States of a Thread
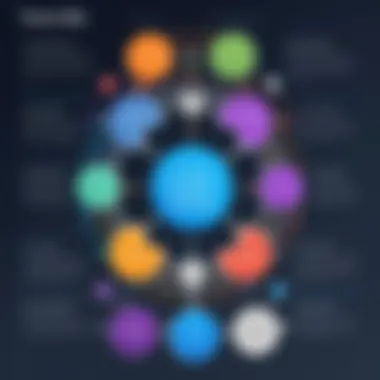
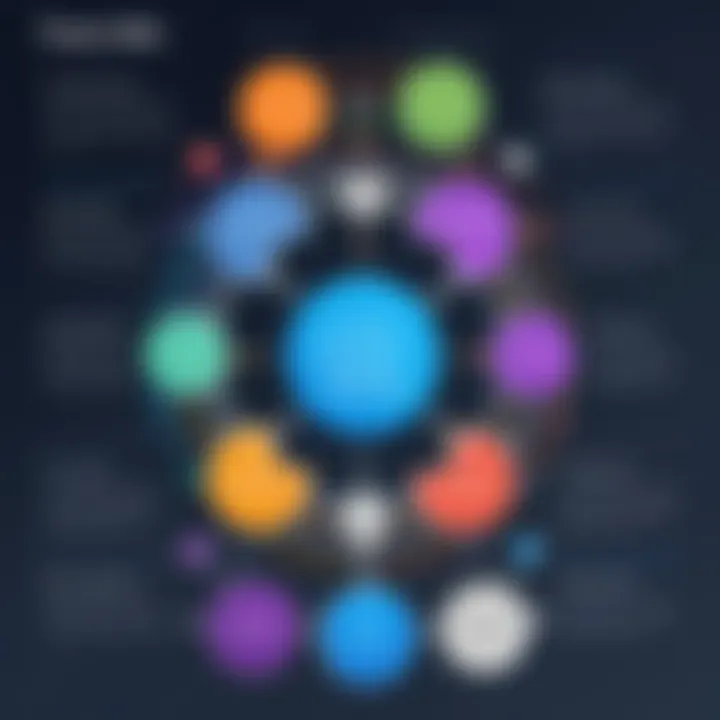
New
The New state is the initial stage of a thread's lifecycle. When a thread object is created but not yet started, it is in the New state. The main characteristic of this state is that the thread is not active yet. It can be created by instantiating the class or implementing the interface. A key advantage of this state is that it allows developers to prepare parameters before the thread begins executing. However, until the method is called, no thread-specific resources are allocated, which can be a disadvantage if resources are needed immediately.
Runnable
Once the thread is started, it enters the Runnable state. In this state, a thread is eligible for execution but not guaranteed that it will run immediately. The unique feature of this state is that the thread can be in this condition even when it is not actively utilizing the CPU. When multiple threads are in this state, the thread scheduler determines which thread among them gets CPU time. This state is beneficial for allowing threads to compete for CPU, but it can create scenarios where a thread does not get immediate execution, leading to delays.
Blocked
The Blocked state occurs when a thread is trying to access a resource that another thread has locked. In this state, the thread cannot continue until the lock is released. This state is significant in managing concurrency since it ensures that shared resources are accessed in a thread-safe manner. The primary disadvantage of this state is that it can lead to performance hits, especially if threads are blocked for extended periods, causing increased wait times.
Waiting
When a thread enters the Waiting state, it is waiting indefinitely for another thread to perform a particular action. This state is marked by calls such as or , where the waiting thread releases the lock it holds. This is beneficial as it helps in resource management and synchronization between threads. However, because the waiting state can last indefinitely without guarantees of resumption, it can also introduce potential deadlock situations if not handled properly.
Terminated
The Terminated state signifies that the thread has completed its execution or has been forcefully stopped. Once in this state, the thread cannot be started again, and its resources are reclaimed by the Java Virtual Machine. The significance of this state lies in proper management of thread lifecycle, ensuring that resources are freed and not left hanging. While it represents the end of thread activity, developers must ensure that application logic accommodates for thread termination, as this can affect overall application flow.
Transition Between States
The transition between these states is vital for understanding the thread lifecycle. Each state logically flows into another as various actions take place. For example, starting a thread transitions it from New to Runnable. Similarly, acquiring resources can move it from Runnable to Blocked or Waiting. This dynamic nature of threads illustrates the complexity of multi-threading and highlights the need for careful design and debugging practices.
Synchronization in Java
In Java, synchronization is a crucial concept that allows multiple threads to operate without conflict. It ensures that only one thread can access a resource at a time, which prevents data inconsistency caused by concurrent modifications. When multiple threads try to access the same resources simultaneously, it can lead to unpredictable behavior. Hence, synchronization makes sure threads do not corrupt data and maintain integrity.
Need for Synchronization
The primary reason for synchronization is to avoid data inconsistency. Shared resources, such as variables, files, or databases, can be accessed by multiple threads. If one thread updates a shared resource while another thread is reading it, the reading thread might receive incomplete or corrupted data. This is especially problematic in applications that require high accuracy, such as financial software, where every calculation must be precise.
Moreover, synchronization helps in maintaining the sequence of operations. When threads are synchronized, the execution of one thread can be made contingent upon the completion of another. This controlled execution order can be critical for multidimensional tasks where certain actions depend on the results of others. Without synchronization, the outcome could be erroneous.
Methods of Synchronization
Synchronization in Java can be achieved through various methods. The two main techniques are Synchronized Methods and Synchronized Blocks. Both methods aim to block concurrent access to shared resources but in different contexts.
Synchronized Methods
Synchronized methods provide a simple way to ensure that only one thread executes a method at a time. When a method is marked with the keyword, the Java Virtual Machine locks the object before the method executes. This means if one thread is executing a synchronized method, other threads that attempt to execute any synchronized method on the same object must wait until the first thread releases the lock.
- Key Characteristic: The method itself is locked, restricting access based on the object instance.
- Benefits: This method is easy to implement and understand, making it a popular choice for synchronizing critical sections of the code.
- Disadvantages: However, synchronized methods can lead to performance issues due to waiting threads, especially if the method takes a long time to execute. Additionally, if overused, they can lead to thread contention and deadlocks, where two or more threads are waiting indefinitely.
Synchronized Blocks
Synchronized blocks are a more flexible approach to controlling access to resources. Instead of locking the entire method, you can choose to lock only a portion of the code. This can improve performance as not every part of the method needs to be synchronized.
- Key Characteristic: The code block itself is locked, allowing finer control over synchronization.
- Benefits: Synchronized blocks minimize the scope of synchronization. They allow the developer to lock only the critical sections, reducing waiting time for other threads.
- Disadvantages: On the downside, they can increase complexity in the code as developers must ensure the correct sections are synchronized, which may introduce bugs if not handled carefully.
In summary, synchronization is essential in Java to prevent data inconsistency and ensure thread safety. Understanding the various methods of synchronization helps developers choose the right approach for their applications.
Inter-thread Communication
Inter-thread communication is a vital concept in multi-threading that allows threads to communicate with each other effectively. This mechanism is crucial when threads are working on a shared resource or need to synchronize their actions. The ability to exchange signals between threads can enhance both performance and efficiency. Without proper communication, issues such as data inconsistency can arise, leading to unpredictable behavior of applications.
Understanding Wait and Notify
The and methods are integral to enabling inter-thread communication in Java. These methods provide a way for threads to block their execution until they are notified to continue. When a thread calls , it releases the lock it holds on an object, allowing other threads to acquire that lock. This is significant in scenarios where multiple threads must coordinate their actions to avoid conflicts and ensure data integrity.
In practice, suppose you have a producer and a consumer thread. The producer needs to wait until there is space in a buffer before producing more items, while the consumer will wait for items to be available. When the conditions are met, these threads can notify one another to proceed with their tasks.
Using Wait, Notify, and NotifyAll Methods
The methods , , and are used in conjunction with an object's monitor in Java.
- wait(): When a thread calls this method, it goes into a waiting state until another thread sends a notification. It is essential to call within a synchronized context.
- notify(): This method wakes up a single thread that is waiting on the object's monitor. If multiple threads are waiting, one will be randomly selected.
- notifyAll(): Unlike , this method wakes up all threads that are waiting on the object's monitor, allowing them to contend for the lock once it is released.
Here is a basic example of using these methods:
Understanding these methods provides a strong foundation for implementing effective communication between threads, ensuring better management of shared resources. Proper use of these methods can significantly improve the responsiveness of applications, making them more efficient.
"Effective inter-thread communication is crucial to building robust multi-threaded applications."
In summary, the mechanisms of waiting and notifying are essential for synchronizing actions of threads. Mastering these concepts enables developers to write more reliable and efficient multi-threaded applications.
Java Concurrency Utilities
In the realm of multi-threading, one must grasp the importance of Java Concurrency Utilities. These utilities simplify the complexity of managing multiple threads. They offer a framework designed to facilitate the development of multi-threaded applications, thus enhancing the performance and scalability of Java applications.
Intro to java.util.concurrent
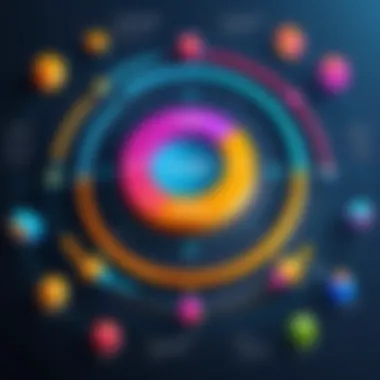
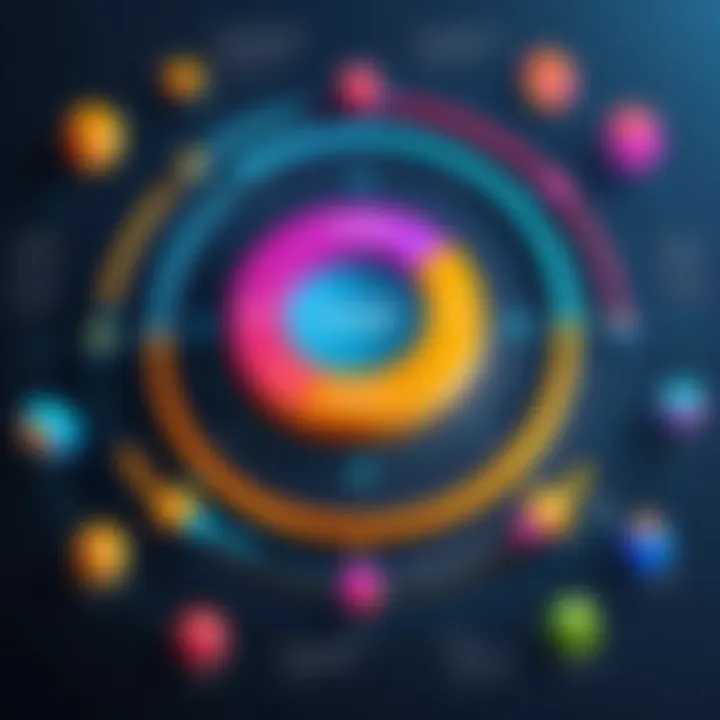
The package is a vital part of Java. It provides a set of tools that streamline concurrent programming. This package includes powerful features that make thread management easier. Within this package, developers can find structures like concurrent collections, executors, and various synchronization aids.
All these tools reduce the risk of errors in multi-threading environments. For instance, using concurrent collections can help avoid race conditions, which are common pitfalls when multiple threads manipulate shared data. This makes programs more robust and easier to maintain.
Executor Framework
The Executor Framework is a central component of the package. It abstracts thread management and takes control of the low-level details of creating and managing threads. The key aspect of this framework is that it allows developers to focus on the tasks that need to be performed rather than the intricacies of thread life cycles.
Creating a Thread Pool
Creating a thread pool is a common practice when using the Executor framework. A thread pool is a collection of pre-initialized threads ready to execute tasks. This characteristic makes managing resources more effective. Instead of creating a new thread for each task, which can be resource-intensive, a thread pool reuses existing threads. This leads to reduced latency and improved performance, especially in applications with many short-lived tasks.
A unique feature of thread pools is their ability to limit the number of concurrent threads. This helps to prevent resource exhaustion, leading to smoother application performance.
Advantages of using a thread pool include:
- Efficient use of resources
- Enhanced performance due to reduced thread creation overhead
However, there are also disadvantages. For example, if tasks require more computation time than expected, the fixed-size pool can lead to delayed task execution.
Executing Tasks in Parallel
Executing tasks in parallel is another crucial benefit of the Executor framework. This feature allows multiple tasks to be handled simultaneously. The parallel execution can significantly improve application responsiveness and throughput, especially in data-intensive applications.
The key characteristic of parallel execution is its ability to distribute workloads across threads. This is particularly useful in modern multi-core environments, where each core can handle a separate thread.
A unique feature of task execution in parallel is that it abstracts the complexities involved in synchronizing threads. This makes the implementation much smoother for developers.
Advantages of executing tasks in parallel:
- Improved application performance
- Greater efficiency in resource utilization
However, the trade-offs must be considered. For example, working with shared resources can still introduce concurrency challenges.
Common Problems in Multi-Threading
Multi-threading in Java brings both efficiency and performance, but it is not without its pitfalls. Understanding the common problems that arise during multi-threaded programming is crucial for developers. Addressing these issues helps maintain application stability, improves response time, and ensures accurate data processing. In this section, we will explore three primary issues: deadlock, starvation, and race conditions. Each of these problems can significantly affect the behavior of your application, leading to unexpected results if not properly managed.
Deadlock
Deadlock occurs when two or more threads block each other by holding resources that the other threads require. For instance, thread A holds resource X and waits for resource Y, while thread B holds resource Y and waits for resource X. This situation creates a standstill, leading to a complete halt in execution. Recognizing a deadlock is essential because it can make your application unresponsive.
To avoid deadlock:
- Implement a Lock hierarchy to control the order in which threads request locks.
- Use timed locks to limit how long a thread waits for a resource.
- Reduce the time a thread holds on to a lock.
An example of deadlock can be:
Ensure proper coordination among threads to prevent them from entering a deadlock.
Starvation
Starvation happens when a thread is perpetually denied access to the resources it needs for execution. This can occur when high-priority threads monopolize the CPU, leaving lower-priority threads unable to proceed. Starvation can lead to severe inefficiencies in system performance, especially in a multi-threaded environment.
To mitigate starvation:
- Use a fair scheduling algorithm to ensure that all threads are given an opportunity to execute.
- Avoid setting excessively high priorities for certain threads at the expense of others.
Consider the following scenario:
- If you have a low-priority thread waiting for resources held by high-priority threads, it may never get executed if those high-priority threads continue to create new work.
Race Conditions
Race conditions occur when two or more threads attempt to change shared data at the same time, leading to inconsistent results. The behavior of your program may vary depending on the timing of thread execution, which makes debugging particularly challenging. For example, if two threads try to update a shared variable at the same time without proper synchronization, it can result in unpredictable outcomes.
To prevent race conditions:
- Always use synchronization mechanisms such as synchronized methods or blocks to control access to shared resources.
- Consider using higher-level concurrency utilities like java.util.concurrent package to manage multiple threads more safely and easily.
In summary, handling these common problems effectively is vital for any Java developer working with multi-threading. Understanding how to identify and prevent deadlocks, starvation, and race conditions will lead to more robust applications.
Best Practices for Multi-Threading
When working with multi-threading in Java, adhering to best practices is vital. These practices streamline operations, enhance performance, and reduce potential problems, such as deadlocks and race conditions. In an environment where multiple threads operate simultaneously, a lack of structure can lead to complications and inefficiencies. By integrating effective strategies, developers can truly maximize the performance and reliability of their concurrent applications.
Minimizing Synchronization Overhead
Synchronization is necessary to maintain data integrity in a multi-threaded environment. However, overusing synchronization can lead to significant overhead. This overhead occurs because synchronized methods and blocks force threads to wait for access to shared resources, causing reduced throughput and increased latency. To mitigate these issues, one can adopt several key strategies:
- Consider using finer granularity: Rather than synchronizing an entire method or block, only synchronize the specific lines of code that access the shared resource. This approach minimizes the time a thread holds on to the lock.
- Prefer concurrent data structures: Java provides a set of concurrent collections in the package, which are optimized for concurrent modifications. Using these collections can reduce the need for synchronization significantly.
- Utilize Lock objects: The class provides more flexible lock semantics than synchronized blocks. It allows features like try-lock and timed-locking, which can help mitigate waiting time in some cases.
Implementing these techniques can effectively reduce synchronization overhead, enhancing not only performance but the overall responsiveness of applications.
Using Thread-Safe Collections
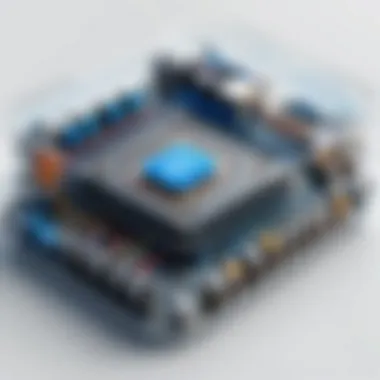
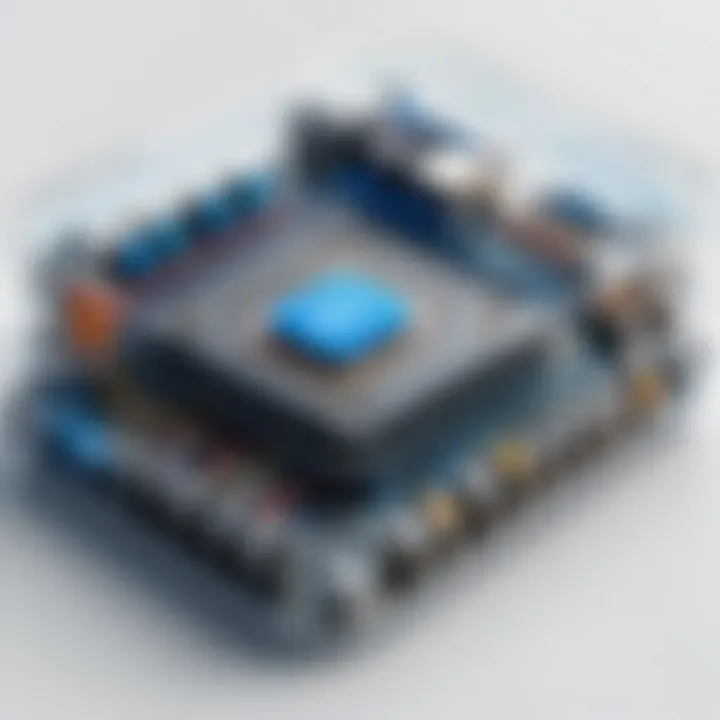
Thread-safe collections are integral to managing concurrent processes smoothly. These collections are designed to allow multiple threads to read from and write to them without corrupting the data or causing exceptions. There are several advantages to using thread-safe collections, including:
- Data integrity: Operations on thread-safe collections ensure that the data remains consistent, even when multiple threads are accessing it concurrently. This reduces the risk of encountering issues like race conditions.
- Simplified code: Developers can write code without the need to manage synchronization manually. This reduces complexity and improves maintenance.
- Optimized performance: Many thread-safe collections employ advanced techniques like lock striping, which allows multiple threads to operate simultaneously on different parts of the collection, improving speed.
Some common thread-safe collections include the , , and . Choosing the right collection based on your specific scenario can further enhance the efficiency of multi-threading practices in Java.
Implementing these strategies is not merely a suggestion; it is a necessity. Understanding these principles can significantly reduce common pitfalls in multi-threading, promoting a more seamless coding experience for developers.
Practical Applications of Multi-Threading
Understanding multi-threading is essential for developers, as it allows them to create more efficient and responsive applications. The practical applications of multi-threading in Java are extensive, and they offer numerous benefits across different domains. By leveraging the power of multiple threads, developers can maximize resource utilization, improve performance, and provide better user experiences.
Web Applications
Multi-threading is crucial in web application development, especially when handling numerous simultaneous user requests. In web servers, each user request can be processed in its own thread. This concurrent execution enables the application to manage multiple tasks at once, significantly enhancing its responsiveness.
For instance, when a web server receives numerous requests, it can spawn a new thread for each request. This prevents blocking and ensures that users do not experience delays. Popular frameworks, such as Spring and Java EE, use multi-threading to create scalable applications.
Moreover, when implementing features like real-time updates or notifications, threads can be utilized to handle background tasks without interrupting the main user interface. This allows the application to remain fluid and interactive, a critical aspect in modern web development.
Gaming Applications
In the gaming industry, multi-threading significantly improves performance and user experience. Games often require the processing of numerous tasks simultaneously. For example, physics calculations, AI behavior, and rendering graphics can operate in separate threads.
By dividing these processes, games can run smoothly without lag, providing a better experience for the player. In multiplayer settings, each user can be handled in their own thread, simplifying synchronization and communication between players.
Using Javaโs concurrency utilities, developers can efficiently manage game states. A well-implemented multi-threading strategy ensures that games remain responsive, even with complex calculations happening in the background.
Data Processing Techniques
Data processing often involves handling large volumes of information. Multi-threading can effectively improve the performance of data-intensive applications. For instance, when processing large datasets or performing complex calculations, different parts of the data can be processed in parallel threads, reducing the overall execution time.
In applications that require data streaming, such as financial or meteorological systems, threads can manage incoming data, analyze it, and store results without delay. This capability is essential for applications that demand real-time data analysis.
The combination of Javaโs robust libraries for multi-threading with efficient algorithms makes it possible to build high-performing data processing applications. This also allows for better resource management and improved throughput.
"Multi-threading can be seen as the backbone of many modern applications, enabling them to scale and perform efficiently in various environments."
Debugging Multi-Threaded Applications
Debugging multi-threaded applications presents unique challenges. Understanding how threads interact and how they can introduce complexity into an applicationโs behavior is essential for developers. With the rise of multi-core processors, utilizing multi-threading has become common, making debugging skills necessary. Improperly managed threads can lead to issues such as race conditions, deadlocking, and inconsistent states. Addressing these problems effectively requires a good grasp of both tools and strategies specific to the multi-threaded environment.
Effective debugging can prevent costly errors and improve application stability. Furthermore, the performance of an application is often reliant on how well its threads operate. Developers need to adopt appropriate debugging practices to diagnose and fix issues promptly. This ensures that multi-threaded applications run efficiently and correctly, providing a better end-user experience.
Tools for Debugging
There are various tools available that can aid in debugging multi-threaded applications. Here are a few that are particularly valuable:
- Java Debugger (JDB): A command-line tool that allows you to set breakpoints, inspect variables, and control program execution.
- VisualVM: A visual tool integrating several command-line JDK tools and lightweight profiling capabilities. It helps monitor application performance, which can be crucial during debugging.
- Eclipse IDE: An integrated development environment that provides debugging features like remote debugging, breakpoints, and thread analysis, making it suitable for Java development.
- IntelliJ IDEA: Another powerful IDE that offers advanced debugging features, including visualizing threads and their states during execution.
Using these tools helps developers gain insight into their applications and identify problems more effectively.
Common Debugging Strategies
Implementing the right strategies when debugging multi-threaded applications can save significant time and effort. Here are some common strategies:
- Thread Monitoring: Use monitoring tools to keep an eye on thread states and their activities. This can help identify bottlenecks or deadlocks.
- Logging: Incorporate logging throughout the application. Logs provide a trail of what threads are doing and help in tracing the thread execution flow. This practice offers insights into timing and order of operations.
- Reproducing Problems: Try to create scenarios where the issue can occur. This involves providing specific conditions that lead to the problem.
- Code Review: Carefully review the code that involves thread interactions. Look for potential areas where things can go wrong, such as synchronized blocks or shared resources that are not adequately managed.
- Isolate Components: If possible, isolate and run components independently to determine if the issue lies within a specific area or interaction.
- Focus on how threads are interacting.
- Look for any threads that may be waiting indefinitely.
- Use different log levels to capture significant events.
- Ensure logs are thread-safe to avoid mixed output.
- Make use of debugging tools to reproduce issues in a controlled environment.
- Understand the necessary sequence of events that leads to the error.
- Discuss with peers to get different perspectives on potential issues.
- Refactoring code can sometimes simplify the problem and make it easier to see where issues reside.
Debugging multi-threaded applications can be complex, but with the right tools and strategies, developers can manage and resolve issues effectively. Understanding how to utilize these resources greatly enhances the ability to create stable, responsive, multi-threaded applications that perform well under various conditions.
End
In the realm of Java programming, understanding multi-threading stands as a cornerstone for creating efficient and responsive applications. This conclusion section encapsulates the significance of the concepts discussed throughout the article. It highlights the myriad benefits that come with mastering multi-threading while considering the specific elements and considerations unique to this subject.
Key benefits of multi-threading include improved performance and resource utilization. By allowing multiple threads to execute concurrently, Java applications can take full advantage of multi-core processors. This parallel execution reduces latency in applications, which is especially important in scenarios such as web applications and real-time data processing. Additionally, multi-threading can enhance user experience, as it allows applications to remain responsive, providing a smoother interaction for users.
Moreover, understanding the challenges of multi-threading is critical for robust application development. As mentioned earlier, developers must navigate issues such as deadlock, race conditions, and starvation. Being aware of these problems enables programmers to implement effective solutions and debuggings strategies. Thus, debugging tools are crucial to identify and resolve these issues, improving overall application reliability.
Ultimately, this conclusion reinforces the idea that mastering multi-threading in Java offers significant advantages. The knowledge obtained from this tutorial equips learners with the tools necessary to implement concurrent programming effectively. Educating oneself about the underlying concepts facilitates a deeper understanding of not just Java, but also programming paradigms in general.
Summary of Key Points
- Java multi-threading enables concurrent execution of tasks, enhancing performance.
- Thread lifecycle consists of various states: new, runnable, blocked, waiting, and terminated.
- Proper synchronization is essential to avoid issues like deadlock and race conditions.
- Java provides useful utilities under for managing concurrency.
- Best practices include minimizing synchronization overhead and using thread-safe collections.
This article serves as a comprehensive guide, emphasizing not only the theoretical aspects but also applying practical examples to enhance learners' coding skills.
Future of Multi-Threading in Java
The future of multi-threading in Java looks promising, especially with the continuous evolution of programming languages and paradigms. As more applications move toward cloud computing and distributed systems, the need for efficient concurrent execution grows.
While traditional multi-threading techniques remain significant, newer approaches are emerging. Reactive programming and the use of asynchronous APIs are gaining traction, enabling developers to handle a multitude of tasks without the complexity associated with managing threads directly. Javaโs integration with frameworks such as CompletableFuture signifies a shift toward more streamlined concurrency management.
As Java continues to evolve, so too will the tools and methodologies designed for multi-threading. Developers will likely see enhanced libraries and frameworks that simplify the complexities of managing concurrency. These advancements will empower programmers to leverage the full potential of modern processors, adapting to the increasing demands of sophisticated applications.