Essential Java Interview Questions for Success
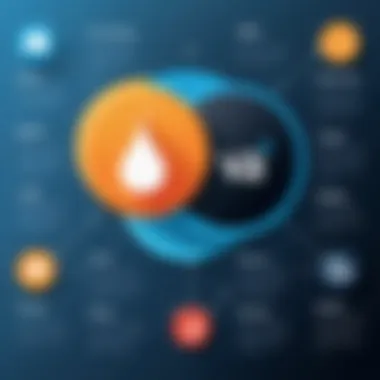
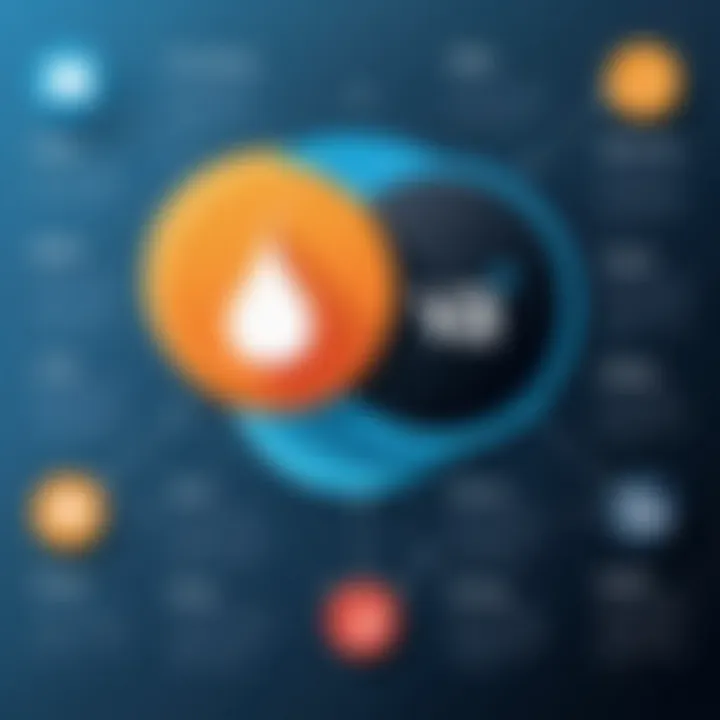
Foreword to Programming Language
Java, a widely used programming language, was originally developed by Sun Microsystems in 1995. Its main goal was to create a language that could run on various platforms. That led to the creation of the Java Virtual Machine, which allows Java applications to run on different operating systems without modification. This cross-platform capability is one of its defining features.
History and Background
Java has its roots in the C and C++ programming languages. It was designed to be simpler, more secure, and more portable. The release of Java 1.0 marked the beginning of its journey. Over the years, Java has evolved through various versions, enhancing its features, performance, and developer API.
Features and Uses
Java is known for its object-oriented approach, strong memory management, and robust security features. It is utilized in a variety of applications, from web development to enterprise systems and mobile applications.
Popularity and Scope
Today, Java continues to maintain a significant presence in the programming world. It is consistently ranked among the top programming languages due to its performance and widespread community support. Many large organizations rely on Java for backend development, mobile applications via Android, and cloud services.
Basic Syntax and Concepts
Understanding the fundamental concepts of Java is crucial for any aspiring developer. These basics form the groundwork for more advanced topics.
Variables and Data Types
In Java, variables are containers for storing data. Each variable has a specific data type, such as int for integers, double for floating-point numbers, and String for text. Proper use of variables and data types is essential for effective coding.
Operators and Expressions
Java includes several operators for performing various operations. These include arithmetic operations (+, -, *, /) as well as logical and relational operators. Expressions combine these operators with variables to perform calculations or comparisons.
Control Structures
Control structures govern the flow of execution in a program. Java provides conditional statements (if-else, switch) and loop constructs (for, while) to control how the program executes certain blocks of code based on specific conditions.
Advanced Topics
As you grow in your understanding of Java, you will encounter more complex concepts that are fundamental in real-world application development.
Functions and Methods
In Java, methods are blocks of code that perform specific tasks. They can accept parameters and return values. Learning to create and utilize methods is vital for organizing code and improving reusability.
Object-Oriented Programming
Java is fundamentally an object-oriented language. This paradigm utilizes ‘classes’ and ‘objects’ to facilitate code modeling. Understanding concepts like inheritance, encapsulation, and polymorphism is critical for advanced Java programming.
Exception Handling
Effective Java programming requires managing errors gracefully. Exception handling allows developers to write robust code that can recover from unexpected conditions using try-catch blocks.
Hands-On Examples
Practical experience reinforces theoretical knowledge. Engaging in small projects or simple coding tasks can provide valuable insights into Java programming.
Simple Programs
Creating simple applications, such as a basic calculator or a number guessing game, can solidify understanding of Java's syntax and control structures.
Intermediate Projects
As skills progress, consider creating intermediate projects like a personal finance tracker or a weather application. These projects often require the integration of data structures and methods.
Code Snippets
Familiarizing oneself with common code snippets can accelerate learning. For example, the following code demonstrates a simple method to calculate the factorial of a number:
Resources and Further Learning
Continuous learning is key to mastering Java programming. Numerous resources exist to help aspiring developers enhance their skills.
Recommended Books and Tutorials
- "Effective Java" by Joshua Bloch
- "Java: The Complete Reference" by Herbert Schildt
Online Courses and Platforms
Websites like Coursera, Udemy, and Codecademy offer structured courses that cover a wide range of Java topics. These can help solidify knowledge through interactive learning.
Community Forums and Groups
Participating in forums like Reddit's r/java or engaging with groups on Facebook can provide a supportive environment to share knowledge and ask questions.
Remember, practicing coding regularly is essential for developing confidence and competence in Java programming.
By approaching your preparation for coding interviews with a thorough understanding of these concepts, you will feel better equipped to tackle the challenges that arise during technical interviews.
Prologue to Java Interviews
In today’s technology-driven landscape, Java remains one of the most sought-after programming languages. Its versatility in application development, from server-side solutions to mobile applications, makes it a staple in many companies. For candidates aspiring to work in roles that demand Java expertise, the interview process serves as a vital gateway. Thus, understanding the nuances of Java interviews is crucial for candidates.
Understanding the Importance of Java in Tech Interviews
Java has established itself as a foundational language in software engineering. Many organizations prioritize candidates with strong Java skills. It’s not solely about knowing how to write Java code; it’s about understanding principles such as object-oriented design, concurrency, and robust data handling. Mastery of these topics can significantly impact a candidate's performance in interviews. Interviews often probe into these aspects through targeted questions, compelling applicants to think critically and demonstrate their proficiency.
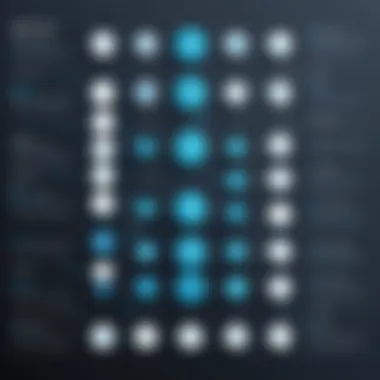
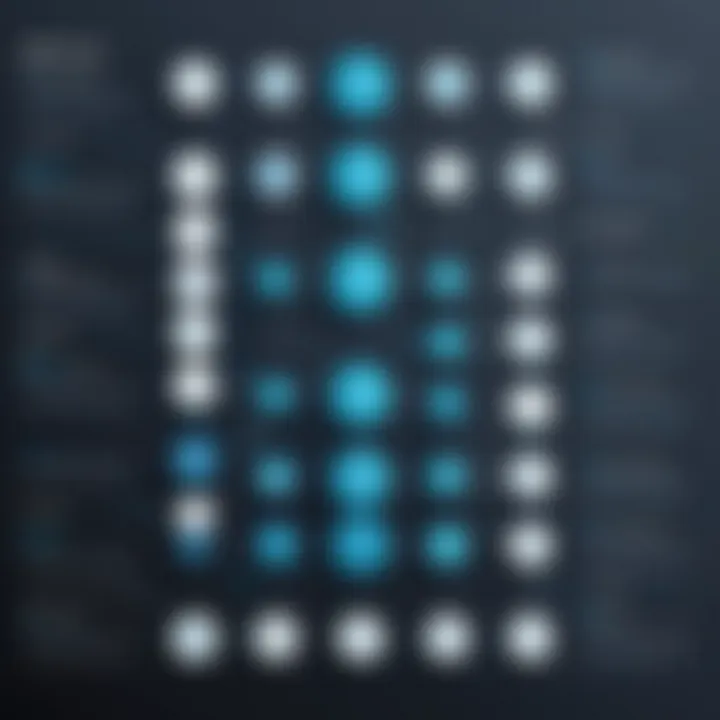
Moreover, Java's extensive libraries and frameworks add layers of complexity. Interviewers often expect candidates to integrate these tools effectively in real-world scenarios. This proficiency can differentiate candidates from their peers and is an essential element of any Java interview preparation.
Overview of Common Interview Formats
When preparing for Java interviews, candidates will likely encounter various formats. Understanding these helps in tailoring preparation effectively:
- Technical Screening: This initial phase may involve a coding test, often conducted online. Candidates can expect questions that assess their understanding of core Java concepts and data structures. An ability to code efficiently under time constraints is critical here.
- Behavioral Interviews: In these sessions, interviewers explore a candidate’s past experiences. They may ask about previous projects or challenges in coding. This format aims to assess problem-solving abilities and cultural fit.
- System Design Interviews: For candidates aspiring to senior roles, system design questions are prevalent. Interviewers may ask candidates to design a system using Java, evaluating their ability to architect solutions that are scalable and maintainable.
- Pair Programming: This format allows candidates to code alongside an interviewer. It assesses real-time problem-solving skills and communication, as candidates explain their thought processes.
Overall, a robust preparation strategy must encompass all these formats. Specific focus on improving coding skills, understanding design principles, and being able to articulate thoughts during interviews is fundamental for success.
Core Java Concepts
Core Java concepts form the backbone of Java programming and are critical to mastering the language. Understanding these concepts ensures that candidates have a strong grasp of the fundamental building blocks necessary for writing efficient and maintainable code. The focus on these topics during interviews is due to their foundational nature and wide application in real-world projects.
Fundamentals of Java
Data Types
Data types in Java define the type of data that can be stored in a variable. They are essential for efficient memory management and type safety. The key characteristic of Java's data types is that they are strongly typed, meaning each variable must be defined with a specific data type. This can help avoid common programming errors.
Unique features of data types include primitive types, such as , , and , as well as reference types like arrays and objects. The advantage of using reference types is that they can hold more complex data structures, whereas primitive types offer better performance due to their simplicity.
Operators
Operators are symbols used to perform operations on variables and values. They include arithmetic, relational, and logical operators. One important aspect of operators is how they provide the ability to manipulate data effectively. Their most significant quality is their versatility, enabling developers to write complex expressions in a clear manner.
The unique feature of operators, especially in Java, is the use of operator overloading which allows custom definitions for specific data types. However, while overloading can increase code flexibility, it can also lead to confusion if not used judiciously.
Control Structures
Control structures are used to dictate the flow of execution in a program. This category includes conditional statements like , loops such as and , and switch statements. The importance of control structures lies in their ability to handle decision-making within the code. They are fundamental because they allow for dynamic execution paths based on varying conditions.
A unique aspect of control structures is their ability to create complex control flows efficiently. However, excessive use of nested structures can result in code that is hard to read and maintain.
Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm based on the concept of objects. Java is inherently built on OOP principles, making an understanding of this area crucial for candidates.
Encapsulation
Encapsulation refers to the bundling of data (variables) and methods (functions) that operate on that data within a single unit, or class. This is vital as it promotes modularity and data hiding. The key characteristic of encapsulation is that it safeguards the state of an object by restricting unnecessary access.
The unique feature of encapsulation lies in the use of access modifiers like , , and to control visibility. While encapsulation enhances security, it can potentially introduce complexity in accessing the properties of objects if not managed well.
Inheritance
Inheritance is a mechanism by which one class can acquire properties and behaviors from another class. This promotes code reusability and helps in creating a hierarchical classification. The key advantage of inheritance is that it allows developers to extend existing class functionality without modifying the original code.
A unique aspect of inheritance in Java is its single inheritance model, where a class can inherit from only one superclass. Though this ensures simplicity, it may also hinder certain design patterns that require multiple inheritance.
Polymorphism
Polymorphism enables a single interface to be used for different data types. It enhances flexibility in programming by allowing methods to take many forms. The key characteristic of polymorphism is its ability to support both method overloading and method overriding. This is beneficial for maintaining cleaner and more understandable code.
One unique feature is that polymorphism can lead to runtime efficiency, as method calls in subclass types can be resolved dynamically. However, it may introduce overhead in terms of performance if not applied judiciously.
Abstraction
Abstraction is the concept of hiding the complex implementation details and showing only the essential features of an object. This is important in simplifying interactions in software design. The key benefit of abstraction is that it reduces complexity by allowing programmers to focus on interactions at a higher level.
A unique feature of abstraction in Java is its ability through abstract classes and interfaces. While abstraction provides clarity in design, it can sometimes result in under-utilization of capabilities if abstract methods lack clear implementation guidelines.
Exception Handling
Exception handling is essential for writing robust Java applications. It helps manage errors gracefully, ensuring that the program can continue operation even in adverse circumstances. In Java, exceptions are objects that describe an error condition. They allow developers to foresee potential issues and handle them through blocks. Understanding this is crucial for developing resilient applications.
The key characteristics of exception handling include checked and unchecked exceptions, which determine whether or not a compilation error occurs when exceptions are not handled. The unique feature of Java’s exception handling is the necessity of handling checked exceptions. This forces developers to actively consider error conditions, although it increases code complexity due to additional boilerplate code.
Data Structures and Algorithms
Data structures and algorithms form the fundamental building blocks of programming. They are essential for organizing, managing, and processing data efficiently. In the context of Java coding interviews, understanding these concepts can significantly influence a candidate's ability to solve problems logically and effectively.
Familiarity with various data structures allows candidates to choose the right tool for a specific situation. Similarly, mastering algorithms aids in optimizing solutions for better performance. These areas are not just academic; they also reflect real-world applications where efficiency and speed matter.
Common Data Structures
Arrays
Arrays are one of the simplest and most widely used data structures. They store elements of the same type in contiguous memory locations. The primary characteristic of arrays is their fixed size, which means that the size must be defined at the time of creation. This property is both a benefit and a limitation.
The fixed size allows for quick access to elements via an index, making operations like searching and sorting straightforward. However, the inflexible size can lead to issues if the number of elements exceeds the initial allocation. This can require additional memory management, becoming a disadvantage for dynamic applications where data size can vary.
Linked Lists
Linked lists consist of nodes where each node contains data and a reference to the next node. This structure allows for dynamic memory allocation, making linked lists more flexible than arrays. A critical advantage of linked lists is their ability to easily insert and delete nodes, which can enhance performance in certain contexts.
However, since linked lists do not allow random access, searching for an element can be slower compared to arrays. Each node contains an extra reference, which can increase memory usage inefficiently depending on the linked list's size.
Stacks
Stacks are built on a Last In, First Out (LIFO) principle, where the most recent element added is the first one to be removed. This characteristic makes stacks beneficial for scenarios like function calls and backtracking algorithms. Their simplicity and the ease of implementation are major factors that make stacks popular choices in programming.
However, the LIFO ordering can be a disadvantage in situations requiring quick access to arbitrary elements. Additionally, stack overflow can occur if the maximum capacity is exceeded, limiting practicality in extensive data applications.
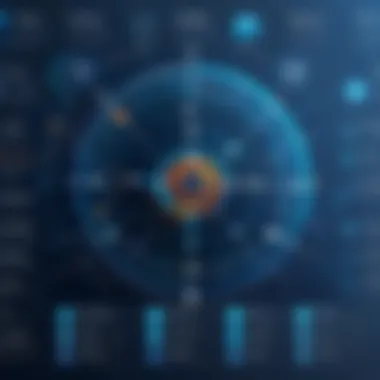
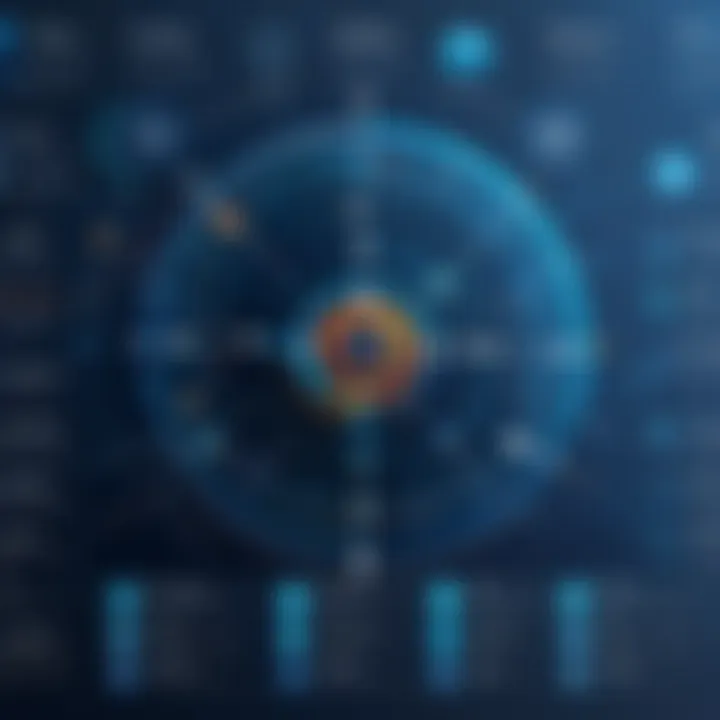
Queues
Queues employ a First In, First Out (FIFO) mechanism, where the first element added is the first to be removed. This property is essential for managing tasks in scenarios like task scheduling and resource sharing. Queues provide a clean and efficient way to handle data flows and processes sequentially.
However, like stacks, queues can also face capacity limitations, especially if a fixed-size structure is utilized. The inability to access elements randomly is another limitation that can recall some performance issues in certain problems.
Hash Tables
Hash tables offer efficient data retrieval using a key-value pairing mechanism. This structure allows for average-case constant time complexity for search operations, making it a highly efficient choice for many applications. One unique feature of hash tables is their ability to handle large amounts of data while keeping the access speed high.
On the downside, hash tables can suffer from collisions, where two keys hash to the same index. This can complicate retrieval processes. If not handled properly, collisions can degrade the performance close to that of linked lists in the worst case.
Algorithmic Techniques
Sorting Algorithms
Sorting algorithms play a crucial role in arranging data in a specific order, helping in efficient search operations. Common algorithms include Quick Sort, Merge Sort, and Bubble Sort. Each has unique characteristics and performance metrics making them suitable for different scenarios.
For example, Quick Sort is notable for its efficiency in large datasets due to its average case O(n log n) complexity. However, its worst-case performance can degrade to O(n²), particularly with poorly chosen pivot elements.
Searching Algorithms
Searching algorithms, like Binary Search and Linear Search, are fundamental for finding elements within data structures. The primary characteristic is the speed with which they can locate data.
Binary Search, particularly, is highly efficient with O(log n) complexity but requires sorted data. In contrast, Linear Search does not necessitate a sorted list but operates with O(n) complexity, making it less efficient for large datasets.
Recursion
Recursion is a powerful technique where a function calls itself to solve smaller subproblems. This method is beneficial for tasks that can be divided into similar sub-tasks, such as tree traversals or solving mathematical problems like Fibonacci series.
However, recursion can lead to stack overflow if the recursion depth is too large. It can also be less efficient unless optimized with techniques like memoization.
Complexity Analysis
Time Complexity
Time complexity assesses how the computational time of an algorithm grows relative to the input size. Understanding time complexity is crucial for selecting the right algorithms in interviews. A common way to express time complexity is Big O notation.
For instance, O(1) represents constant time complexity, while O(n) signifies linear growth concerning the input size. Knowing these helps candidates to evaluate the efficiency of their solutions.
Space Complexity
Space complexity measures the amount of memory an algorithm consumes during its execution. It is another critical aspect when discussing algorithmic efficiency. Just like time complexity, it is often expressed in Big O notation. Understanding how algorithms utilize memory helps in optimizing programs, especially in environments with resource limitations.
Both time and space complexities are essential knowledge areas in preparing for Java coding interviews, as they provide a framework for evaluating the efficiency of different solutions.
Java Collections Framework
The Java Collections Framework is a pivotal component of the Java programming language. It provides a set of classes and interfaces that enable developers to store and manipulate groups of related elements. This framework encapsulates several well-known data structures and algorithms, making it easier for Java developers to implement dynamic collections in their applications.
Accurate knowledge of the Java Collections Framework enhances a candidate's technical skills, as it addresses how to effectively organize and manipulate data. When preparing for coding interviews, understanding collections is essential since interviewers often focus on algorithms and performance. This section expands on the basic principles of collections and their various implementations, offering clarity on their specific uses and benefits.
Overview of Collections
Collections in Java can be categorized into various types, each serving a particular purpose. They help manage data efficiently, allowing developers to store, retrieve, and manipulate data with ease. The main types of collections include Lists, Sets, and Maps. Each collection type provides specific characteristics that define how it behaves in terms of performance and storage.
Understanding these collections is crucial as they offer unique advantages depending on the context of the programming task. Moreover, Java’s collections classes implement algorithms that can be used directly, resulting in quicker and more efficient coding practices.
Commonly Used Collections
List
The List interface is an essential part of the Java Collections Framework. It represents an ordered collection of elements, meaning that they can be accessed by their positions in the list. The key characteristic of a List is its ability to maintain the order of insertion, which is particularly beneficial when the sequence of elements matters.
A popular option among Lists is the ArrayList, known for its dynamic array capabilities. It allows elements to be added and removed, resizing the underlying array when necessary. The main advantage of using an ArrayList is its efficient random access. However, its performance can decrease during frequent insertions and deletions as it requires shifting elements.
Set
A Set is another fundamental collection in Java, designed to hold unique elements without duplicates. The key characteristic of a Set is its guarantee of uniqueness. This is beneficial when the need arises to store a collection of items without considering repeated values.
Implementations like HashSet and TreeSet are commonly used; HashSet offers constant time performance for basic operations like add, remove, and contains. On the other hand, TreeSet provides ordered storage, which allows for easy retrieval of elements in natural order. One disadvantage of Sets is that they do not allow positional access, which can complicate certain scenarios where order matters.
Map
The Map interface provides a collection structure that pairs keys with values, enabling efficient data retrieval based on keys. The key characteristic of a Map is its ability to store data in key-value pairs, making it highly efficient for lookups. The most commonly used implementation, HashMap, offers constant time complexity for basic operations.
A unique feature of HashMap is that it allows null values and one null key. However, a challenge may arise with the usage of null keys in certain contexts, as it may lead to exceptions if not handled properly. Choosing the right implementation of Map based on the requirements of order and performance is critical for maximizing efficiency.
Understanding the nuances of each collection type allows Java developers to make informed decisions, leading to optimized code.
By diving deep into these collections, candidates can show their ability to handle data manipulation challenges effectively during interviews. Familiarity with the Java Collections Framework sets a strong foundation for solving complex problem statements in coding interviews.
Multithreading and Concurrency
Multithreading and concurrency are vital concepts in Java programming. They allow more than one thread to execute independently but share common resources. This is crucial in real-world applications where efficiency and performance play a central role. Being skilled in these areas is not just an asset for developers but a requirement for many roles in software development. Understanding these topics helps candidates excel in interviews, especially as the demand for responsive applications grows.
Understanding Multithreading
Multithreading enables concurrent execution of two or more threads. In Java, this is achieved through the class or implementing . Threads can be running, ready, waiting, or terminated. This approach leads to better resource utilization and improved performance in applications. When coding, it's essential to understand how multithreading can optimize tasks such as background operations and responsive UIs.
Concurrency in Java
For managing multiple threads effectively, Java provides several tools and techniques.
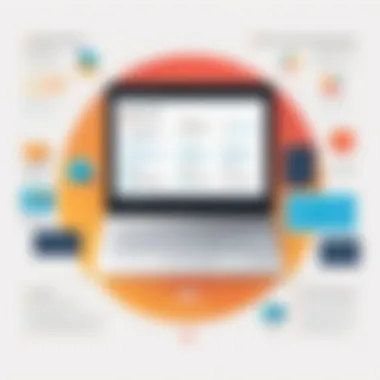
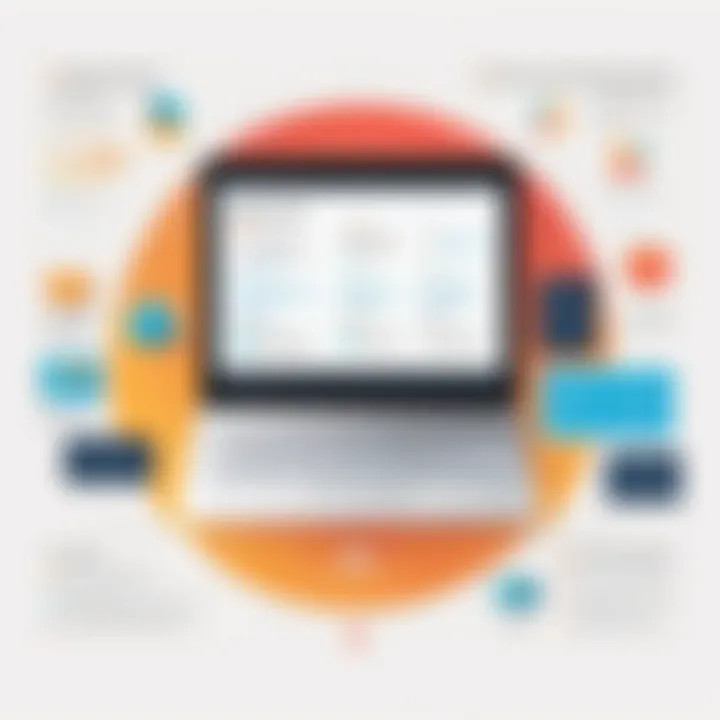
Synchronized Blocks
Synchronized blocks are a mechanism in Java to ensure that only one thread can execute a block of code at a time. This prevents data inconsistency when multiple threads access shared resources. The use of synchronized blocks is straightforward: any block of code can be wrapped to restrict concurrent access.
Key Characteristic: The ability to control access to methods or blocks of code is what makes synchronized blocks a popular choice in concurrency handling.
A unique feature of synchronized blocks is that they can improve application performance significantly. However, improper use can lead to issues like deadlocks, where two or more threads are waiting indefinitely for each other to release resources. Therefore, careful design is needed when employing synchronized blocks to maintain application reliability and performance.
Thread Pools
Thread pools are another important aspect of concurrency in Java. They manage a pool of threads to execute multiple tasks concurrently, rather than creating new threads for each task. The use of thread pools enhances application performance and resource management by reusing a number of existing threads.
Key Characteristic: A primary advantage of thread pools is that they reduce the overhead associated with thread creation and destruction.
The unique feature of thread pools is their ability to queue tasks when all threads are busy. This mechanism ensures that incoming tasks are not lost but waited on. While this improves efficiency, a downside is that it could lead to increased latency if the pool is not sized appropriately for the workload. Registering the maximum thread limit is crucial to balance workload and system resources effectively.
Efficient use of multithreading and concurrency techniques can elevate application performance and reliability, values much sought in modern software development.
By understanding these aspects, candidates can demonstrate their knowledge and problem-solving skills during their interviews and, ultimately, in their future roles.
Common Java Frameworks and Libraries
In the realm of Java development, frameworks and libraries play a crucial role in enhancing productivity and simplifying complex tasks. These tools have been meticulously designed to address common programming challenges, providing developers with pre-written code that can be reused. This is especially important during interviews, as understanding these frameworks can showcase a candidate's familiarity with the broader Java ecosystem. Furthermore, many employers expect candidates to have practical experience with notable frameworks, making knowledge in this area a significant asset.
Spring Framework
The Spring Framework is one of the most widely used frameworks among Java developers. Its comprehensive programming model offers a wide range of functionalities, making it suitable for building enterprise applications. The core of Spring is based on the Inversion of Control (IoC) principle, which promotes loose coupling between components. This results in more manageable and testable code.
Key Features of Spring Framework:
- Dependency Injection: This feature allows for better management of dependencies between components. Developers can define how objects interrelate, and the framework takes care of the object creation.
- Aspect-Oriented Programming (AOP): AOP helps in separating cross-cutting concerns, such as logging and transaction management, from the business logic, leading to cleaner code.
- Spring Boot: This simplifies the process of setting up and developing applications. With pre-configured templates, developers can get applications running quickly without extensive configuration.
Understanding Spring is vital during interviews as many organizations utilize it for building scalable and robust applications. Interview questions might focus on aspects such as Dependency Injection patterns or how to configure an application using Spring Boot.
Hibernate
Hibernate is a powerful Object-Relational Mapping (ORM) library that streamlines database interactions in Java applications. It allows developers to work with database entities as Java objects, significantly reducing the amount of boilerplate code required for database operations.
Benefits of Using Hibernate:
- Simplified Data Handling: Boone data can be easily mapped to Java classes, allowing developers to focus on business logic rather than SQL queries.
- Automatic Schema Generation: Hibernate can automatically create database schemas based on Java classes, saving time in setup.
- Caching Mechanisms: Hibernate provides built-in caching options, improving application performance.
In coding interviews, candidates can expect questions regarding how to configure Hibernate, the caching mechanisms, and how Hibernate handles transactions. Understanding its features and how it integrates with the Spring Framework can also be helpful, as many projects employ both technologies together.
Familiarizing yourself with Spring and Hibernate is essential for modern Java development, as these frameworks represent industry standards and are commonly required skills in job descriptions.
Preparing for Coding Interviews
Preparing for coding interviews is a crucial phase for any Java developer looking to secure a position in today's competitive tech industry. This section emphasizes the significance of a systematic approach to interview preparation. First, understanding the landscape of commonly asked questions is key, as this can help you familiarize yourself with coding problems that you may face. Moreover, practicing these questions can build your confidence and refine your problem-solving skills, which are invaluable during live interviews.
Practice with Sample Questions
Engaging with sample questions is one of the most effective strategies for preparation. These questions can range from simple to complex, covering various topics within Java. Candidates should focus on the most common types of questions, such as:
- Core Java concepts
- Data structures
- Algorithms
- Object-oriented programming principles
Practicing with sample questions allows individuals to develop a better understanding of how to apply theoretical knowledge to practical scenarios. It also highlights areas that may require further study. Ultimately, going through sample questions helps candidates to approach problems more strategically, preparing them to articulate their thought processes during interviews.
Utilizing Online Resources
The internet provides an abundance of tools and resources to assist candidates in preparing for interviews. These resources can be broadly categorized into two types: mock interviews and coding platforms.
Mock Interviews
Mock interviews offer candidates a chance to simulate real interview conditions. This methodical practice is invaluable and provides insight into how to present oneself effectively. A key characteristic of mock interviews is the variability in format. They can be structured or unstructured, depending on the platform used.
Mock interviews are a popular choice for candidates looking to refine not just technical skills but also soft skills like communication. One unique feature is that many platforms offer feedback after the interview, which is crucial for improvement. However, it is important to choose mock interview partners who are knowledgeable and able to give constructive feedback to ensure a beneficial experience.
Coding Platforms
Coding platforms, such as LeetCode or HackerRank, offer a variety of challenges to practice coding skills. These platforms are beneficial for developing problem-solving techniques and can help candidates build a routine around coding practice. A key characteristic of these platforms is their vast repository of questions covering different programming topics, including Java-specific challenges.
One unique feature is that they often include community discussions and solutions, providing different approaches to the same problem. The advantage of using these platforms is that they can track your progress over time, allowing you to focus on weaker areas. A potential disadvantage, however, is that some candidates may become solely reliant on platforms, neglecting to apply knowledge in real-life scenarios.
Tailoring Your Preparation
Tailoring your preparation means aligning your study plan with your target job requirements. Researching specific companies and noting their preferred Java frameworks or libraries can guide your focus. Additionally, collaborating with peers for study sessions adds a social element and allows for the sharing of diverse perspectives and resources.
Final Thoughts
As we conclude our examination of Java coding interview questions, it is vital to reflect on the significance of continuous learning and maintaining a growth mindset. Navigating through technical interviews can be challenging. However, the skills and knowledge acquired in the process are invaluable.
Continuous Learning
In the realm of technology, continuous learning is paramount. Java, while established, constantly evolves. New frameworks, tools, and techniques emerge frequently. Keeping pace with these changes is essential for developers aiming for long-term success. Engaging with online courses, textbooks, or Java communities can strengthen your understanding of advanced topics.
Furthermore, learning does not end when you secure a job. Many resources are available to facilitate ongoing growth. Participate in hackathons, code reviews, and open source projects.
- Leverage online platforms: Websites like Wikipedia) and Reddit provide valuable insights.
- Read articles and blogs: Follow Java expert blogs that discuss updates and new practices.
The journey of a developer is lifelong. Staying informed helps not just in interviews but in actual job performance as well.
Growth Mindset in Coding
Embracing a growth mindset means understanding that abilities can improve through dedication and effort. This philosophy is crucial during your preparation for coding interviews.
When faced with difficult coding puzzles, interpreting failure as a learning opportunity fosters resilience. A growth mindset encourages you to seek feedback and collaborate with others. Engaging with peers can provide different perspectives on problem-solving.
Consider the following aspects to cultivate a growth mindset:
- Be open to challenges: Instead of avoiding difficult problems, tackle them head-on.
- Accept constructive criticism: Use feedback to refine your skills and knowledge.
- Stay curious: Explore various aspects of Java and related technologies.