Mastering Java Interview Questions: An In-Depth Resource
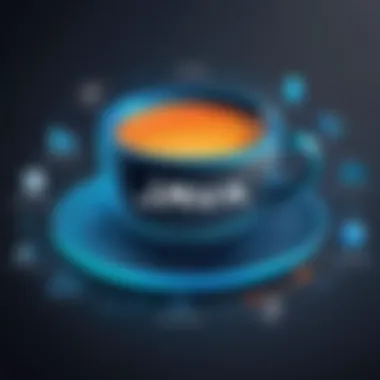
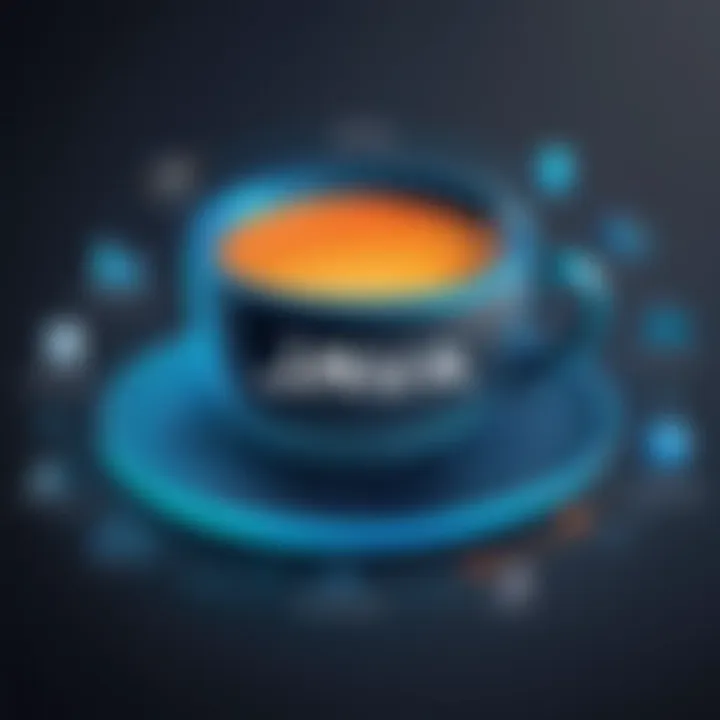
Intro
Navigating the intricacies of Java can be a challenging endeavor, particularly when preparing for interviews. Java, a programming language that has stood the test of time, is prized for its versatility and robust features. It serves as the backbone for a plethora of enterprise-level applications, mobile platforms, and web systems.
In this guide, we explore a variety of Java interview questions, designed to cover essential domains that candidates are expected to be well-versed in. From fundamental concepts like variables and data types to complex topics like data structures and algorithms, our aim is to create a strong framework to support both new learners and seasoned developers alike.
Importance of Java
"Java is to software what the Swiss Army knife is to tools — versatile and applicable to numerous fields."
With its long-standing influence, Java continues to be a go-to language in many development circles. Understanding the nuances of Java is not just beneficial for nailing the interview, but it’s also crucial for ensuring success in real-world programming environments.
The approach we take in this article is structured — categorizing questions based on fundamental knowledge areas, advanced topics, and practical applications. By parsing through these components, readers will gain insights not only into Java itself but also into how it integrates with broader programming concepts, preparing them to tackle interview questions with confidence.
As we delve into each section, we will emphasize key aspects that are likely to come up during interviews, integrating examples and best practices to solidify understanding. This will help bridge the gap between theory and application, encouraging you to think critically about Java’s role in software development.
Understanding Java Interviews
Understanding the intricacies of Java interviews is crucial for candidates aiming to prove their mettle in the competitive tech landscape. This section delves into the fundamentals of this process, emphasizing not just the mechanics of interviews but also the broader implications of the preparation phase.
The Importance of Preparation
Preparation stands at the forefront when it comes to Java interviews. Without a solid plan, candidates may find themselves floundering, akin to a ship lost at sea. Being well-prepared means more than knowing the syntax; it encompasses grasping concepts, practicing coding problems, and familiarizing oneself with potential interview questions.
With the right preparation, candidates significantly up their chances of success. Studies show that individuals who engage in targeted practice tend to perform better. So, while it may seem tempting to rely on intuition, preparation is the magic key that opens the door to triumph.
Types of Java Interviews
Java interviews come in various flavors, each tailored to assess different skills and competencies. Understanding these types not only prepares candidates for what to expect but also allows them to strategize their preparation effectively.
Technical Interviews
Technical interviews serve as the bedrock of the hiring process in many tech companies. These interviews focus on a candidate's coding abilities, algorithmic thinking, and problem-solving skills. During such interviews, candidates might face a whiteboard coding exercise, where they need to solve a problem on the spot.
One key characteristic of technical interviews is their emphasis on practical skills. This style is particularly beneficial as it enables hiring managers to gauge how candidates approach real-world problems.
However, technical interviews also come with unique challenges, such as the pressure of thinking on one's feet. Candidates might feel overwhelmed by the need to recall various concepts quickly, making mock interviews or practice sessions invaluable. The pros here—immediate feedback and the chance to work through problems under pressure—can outweigh the cons.
Behavioral Interviews
Behavioral interviews, a staple in many hiring processes, delve into a candidate's experiences and interpersonal skills. Employers use these interviews to understand how past behaviors predict future performance. A common format involves the STAR method (Situation, Task, Action, Result), where candidates recount specific situations.
One notable aspect of behavioral interviews is their focus on soft skills—crucial in a collaborative environment. This approach is beneficial as it highlights a candidate's teamwork, communication, and adaptability. Behavioral interviews allow interviewers to see how candidates fit into a company's culture, going beyond technical know-how.
However, these interviews might be nerve-wracking for some, as recalling past experiences may not always be straightforward. The unique advantage here is the chance to showcase one's personality and thought process, which could tip the scales in favor of a candidate.
System Design Interviews
System design interviews target a candidate's ability to architect and design software systems. These interviews require candidates to break down high-level requirements and propose scalable solutions. It's not just about coding; it's about thinking critically and creating a holistic view of the software ecosystem.
What sets system design interviews apart is their focus on scalability, performance, and real-world application. Candidates are often asked to design systems that could handle substantial loads, like a social media platform or an e-commerce site. This aspect makes them essential for senior roles where complexities are higher.
However, these interviews can be daunting, especially for those less familiar with design principles. Candidates need to prepare extensively for them, potentially studying design patterns and architecture principles. The advantage lies in the opportunity to demonstrate strategic thinking, which can impress potential employers.
The knowledge and understanding of these varied interview types prepare candidates for a comprehensive interview process. It’s crucial to recognize that various skills will be assessed, influencing how one should gear up for the challenge.
Fundamental Java Concepts
Understanding Fundamental Java Concepts is essential for anyone preparing for a Java interview. This section lays the groundwork for more advanced topics and helps candidates answer questions with clarity and confidence. Without a firm grasp on these basics, it can be quite challenging to tackle the more complex subjects that often arise during technical interviews. Thus, mastering these concepts not only enhances coding skills but also boosts overall problem-solving abilities.
Core Java Features
Core Java features form the building blocks of the language and are crucial in any Java-based role. These features are what enable effective programming practices.
Class and Object
Class and Object are two of the most fundamental aspects of Java's object-oriented programming paradigm. A class serves as a blueprint for creating objects, which are instances of the class. This setup promotes encapsulation—an essential principle of OOP where data and methods are bundled together.
- Key characteristic: Classes provide a structured way to organize code, making it easier to manage larger programs.
- Benefits in the article: Focusing on classes and objects aids in understanding how to approach problems from a modular perspective, which aligns with industry practices.
- Unique feature: The ability to create multiple objects from a single class offers flexibility. However, this can lead to complexity if not managed properly, particularly in larger systems.
Data Types in Java
Data Types in Java dictate the kind of data a variable can hold. This includes primitives like integers and floats along with more complex types like arrays and strings. The importance of understanding data types cannot be overstated, as it fundamentally influences the choice of algorithms and data structures, which directly correlates with performance and usability.
- Key characteristic: Java's strong typing system helps prevent many common errors, such as type mismatches, during compilation rather than at runtime.
- Benefits in the article: Clear knowledge of data types can significantly improve coding efficiency by reducing the debug time and highlighting potential issues.
- Unique feature: The distinction between primitive (like , ) and reference types (like , ) aids in memory management. Yet, it requires careful handling to prevent issues like .
Control Structures
Control Structures in Java determine the flow of execution of code. They include if-else statements, loops, and switch cases. These structures allow for decisions to be made in response to various conditions, which is fundamental for implementing logic in any program.
- Key characteristic: They provide the capability to control the behavior of the program dynamically.
- Benefits in the article: Mastery of control structures is vital to optimizing performance and improving readability in code.
- Unique feature: Java's enhanced for-loop is designed specifically for iterating over collections and arrays easily. Nevertheless, improper use can lead to inefficiencies.
Java Syntax and Semantics
Java Syntax and Semantics encompass the rules and meanings behind the code written in Java. A solid understanding of both is crucial for effective coding and debugging practices. Syntax refers to the structure of the code, while semantics deals with the meaning of code constructs.
Understanding these facets will not only prepare candidates for typical languages questions but also help distinguish them in interviews. By paying close attention to writing clean code following proper syntax and keeping semantics in mind, a programmer enhances their chances of successfully passing interviews and excelling in real-world applications.
Object-Oriented Programming in Java
Object-Oriented Programming (OOP) forms the backbone of Java and understanding it is crucial for any aspiring developer. OOP enhances code organization, reusability, and maintainability—key aspects that are often scrutinized in interviews. The principles of OOP not only aid in building robust applications but also simplify complex problems by breaking them down into manageable objects. This section will dive into the core principles that dictate the design and functionality of Java programs.
Principles of OOP
Encapsulation
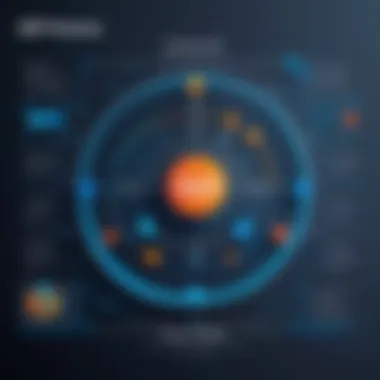
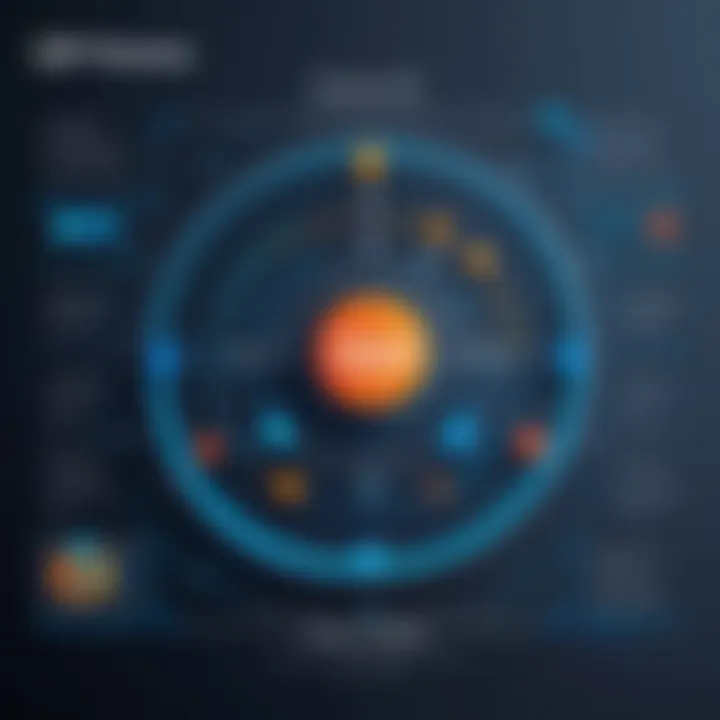
Encapsulation is akin to putting your valuable items inside a locked box. It allows developers to restrict access to certain components of an object while exposing only what is necessary. The key characteristic of encapsulation is the bundling of data (attributes) and methods (functions) that operate on the data into a single unit or class. This principle contributes significantly to data hiding, which ensures that the internal representation of an object is hidden from the outside.
The main advantage of encapsulation is that it protects the object’s integrity by preventing external interference and misuse. However, encapsulation can also lead to increased complexity in the codebase. If too many layers of encapsulation are used, it may hinder the performance or complicate debugging processes. Nonetheless, it remains a popular choice for maintaining clean architecture within Java applications.
Abstraction
Abstraction simplifies complex reality by highlighting the relevant details while hiding the unnecessary ones. In Java, it can be realized through abstract classes and interfaces. The beauty of abstraction lies in creating a well-defined contract for what the classes should do without detailing how they do it.
This principle is especially beneficial for programmers as it allows them to focus on high-level functionalities rather than the minute implementation details. However, while abstraction reduces code complexity, it requires careful planning of data and behaviors, which may not be intuitive for all developers.
Inheritance
Inheritance is a way through which one class can inherit properties and methods from another class, promoting code reusability. Think of it as a family tree, where a child class inherits traits from its parent class. This principle helps in extending existing code without modification, which can significantly cut down on development time.
The main advantage of inheritance is that it fosters a hierarchical classification of classes, making code organization and maintenance easier. Yet, it can sometimes introduce complications. Over-relying on inheritance can lead to a rigid structure in code and can make updates or changes to parent classes disruptive, possibly breaking child classes. Despite this, inheritance is a valuable concept for creating modular and flexible code.
Polymorphism
Polymorphism allows objects to be treated as instances of their parent class, enabling one interface to be used for different underlying forms (data types). This principle can manifest in two ways: method overriding and method overloading. The versatility of polymorphism is like having a Swiss army knife, capable of performing various functions based on the context.
The key characteristic of polymorphism is its power in building scalable and maintainable applications. It is beneficial because it leads to cleaner and more understandable code since developers can use a unified interface for interacting with various data types. However, polymorphism can sometimes lead to unpredictable behavior if not handled with care, especially when method overriding is involved. Thus, even though its utility is undeniable, it introduces a layer of complexity that requires thorough understanding.
Common OOP Questions
Understanding these fundamental principles will set a strong foundation for interviews. Candidates might encounter questions like:
- Can you explain the four pillars of OOP?
- What are the advantages of encapsulation?
- How does inheritance work in Java?
- Can you provide an example of polymorphism?
These questions assess not just knowledge but the ability to apply these concepts in practical scenarios. One should be prepared to elaborate on principles clearly and relate them to real-world coding problems, making the discussion engaging and insightful.
Data Structures in Java
Data structures form the backbone of data organization, manipulation, and retrieval in programming. In the context of Java, understanding these structures is vital not just for coding but for cracking interviews where this knowledge often separates the wheat from the chaff. With a myriad of interview questions pointedly probing into candidates' grasp of data structures, becoming well-versed in Java’s approach can lead to significant advantages.
A solid foundation in data structures allows developers to tackle algorithmic problems methodically. Familiarity with how to store, access, and modify data effectively can lead to optimized performance in applications. Students and aspiring developers should take this seriously; the right data structure can drastically reduce the time complexity of an algorithm, transforming an unwieldy task into a manageable one.
Arrays and Strings
When discussing foundational data structures in Java, arrays and strings are often where it all begins. Arrays are a collection of variables, often of the same type, stored in contiguous memory locations. This facilitates indexed access, which makes retrieval operations quite fast. An array's fixed size can be both its strength—allowing for efficient memory usage—and its weakness, as it doesn’t adapt to changing data needs.
Strings in Java, however, deserve special attention. They are, in essence, a sequence of characters, and while they appear as a simple data type, they encapsulate powerful functionalities. Remember, every time you manipulate a string in Java, you're primarily dealing with an object from the class. The immutability of strings is a double-edged sword. On one hand, it enhances security and thread-safety, but it can lead to inefficiencies when frequent modifications are necessary.
"An effective data structure is not just a collection of data; it’s a dynamic way to represent real-world problems."
Collections Framework
The Java Collections Framework is an extensive set of classes and interfaces providing a sophisticated way to handle groups of objects. Unlike arrays, the collections framework excels in flexibility and ease of use. This section focuses on the primary interfaces: the List, Set, and Map interfaces, each serving a unique purpose in helping Java developers build efficient applications.
List Interface
The List interface is one of the most vital components of the collections framework. It allows for the storage of ordered collections, providing access to elements through indices. Key characteristics of lists include their ability to allow duplicates and maintain the order in which elements are inserted. This makes the List interface a popular choice when order matters, as in applications that involve user-generated content.
A unique feature of the List interface is its dynamic resizing. Unlike regular arrays, you can add or remove items without needing to define a maximum size upfront. This flexibility proves beneficial in real-world applications.
Advantages:
- Dynamic sizing allows adaptation to data growth.
- Built-in methods simplify operations like insertion, deletion, and search.
Disadvantages:
- If not managed properly, performance can degrade, particularly with larger lists as shifting elements is needed after removals.
Set Interface
Diving into the Set interface, it’s important to recognize that this collection allows storage of unique elements, which means no duplicates are permitted. This uniqueness is beneficial when you need to maintain distinct entries, such as in the context of user IDs or product codes.
The Set interface provides a more theoretical approach to data organization. It doesn’t maintain the order of elements, which can be handy in scenarios where this attribute might be unnecessary.
Advantages:
- Automatic handling of duplicates streamlines data integrity.
- Often more efficient than lists for membership checks due to its underlying implementations.
Disadvantages:
- Lack of indexed access means searching through the set can be less efficient, depending on the implementation.
Map Interface
Maps are an essential part of Java’s collections framework, holding a collection of key-value pairs. The Map interface serves as a cornerstone for all such collection types, allowing for the fast retrieval of values based on specified keys. A prominent characteristic of maps is their ability not to allow duplicate keys, ensuring that each key points to a single value.
Maps become integral when context and relationships matter. They effectively model real-world situations where entities are associated with specific attributes or identifiers.
Advantages:
- Fast retrieval of values via keys makes accessing information efficient.
- Supports custom key objects, enabling complex data structuring.
Disadvantages:
- Memory consumption can be high with large volumes due to the overhead of storing keys and values together.
Understanding the nuances of data structures like arrays, strings, and Java Collections Framework is fundamental for any Java developer. The intersection of scalable, efficient data management techniques with well-rounded programming knowledge can easily become a decisive factor in job interviews.
Algorithms and Problem Solving
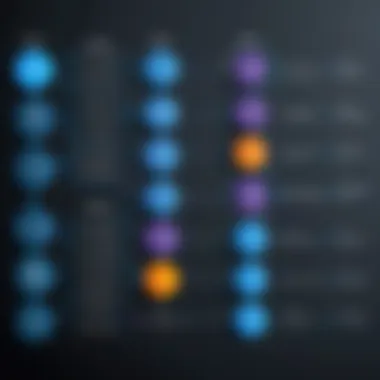
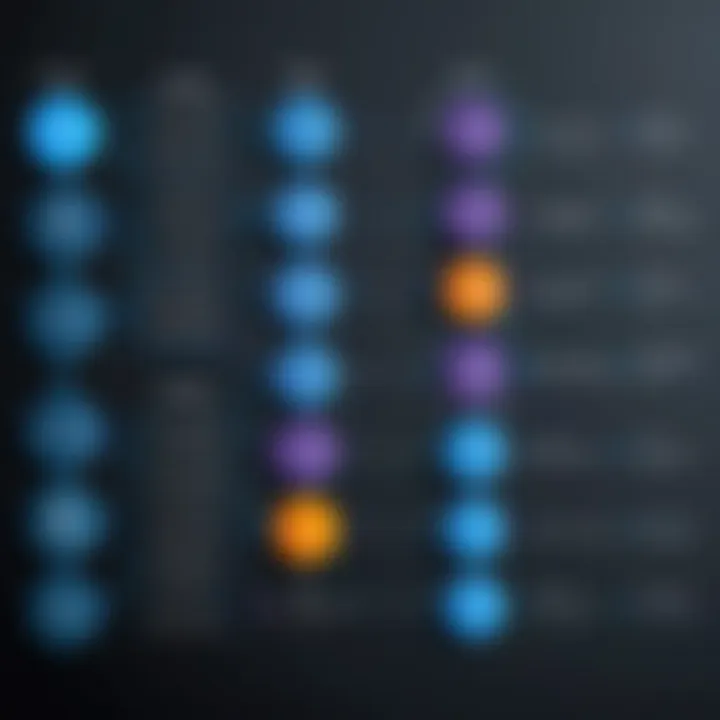
Grasping the essentials of algorithms and problem-solving techniques is pivotal for anyone looking to crack Java interviews. Employers often want not just to see if candidates know their stuff but also how they approach issues. Algorithms define the step-by-step procedure for solving a particular problem, acting as the backbone for efficient programming, and problem-solving skills highlight a developer's analytical prowess. Hence, having a good grasp of these topics can bolster a candidate's performance significantly.
The benefits of mastering algorithms include enhanced efficiency and the ability to optimize code to meet specific constraints. As companies face larger datasets and increased performance requirements, being proficient in algorithms allows developers to write faster and more efficient code. Considerations, then, revolve around understanding time and space complexity, as this knowledge is often tested during interviews. Knowing how to choose the appropriate algorithm for a given problem can set candidates apart from the crowd.
Sorting Algorithms
Sorting algorithms are fundamental for organizing data. The way these algorithms manipulate data plays a significant role in the performance of applications, and interviewers tend to highlight them due to their frequent use in real-life applications.
Quick Sort
Quick Sort is notable for its low average-case time complexity of O(n log n), making it a go-to choice for many when speed is critical. This algorithm employs the divide-and-conquer strategy by partitioning the data into subarrays. The main characteristic that sets Quick Sort apart is its in-place sorting, which helps in reducing the overhead associated with auxiliary array space.
One of the unique features of Quick Sort is that it generally performs better than other O(n log n) sorting algorithms, particularly in practice. However, there are cases where its worst-case performance can degrade to O(n²) if a poor pivot choice is made. Despite this potential downside, its average-case benefits make Quick Sort a commonly favored option in the realm of algorithms.
Merge Sort
Merge Sort shines when it comes to stability and performance with linked lists. Its characteristic of always operating at O(n log n) time complexity, regardless of input state, makes it dependable. The way it works is by dividing the list into halves, sorting each half, and then merging those sorted halves back together, which contributes to its name.
A unique feature of Merge Sort is its auxiliary space requirement, which can be seen as a disadvantage. Unlike Quick Sort, it requires additional space for merging operations, which can lead to inefficiencies when working with large datasets. Nevertheless, its predictable performance and stable sorting behavior cement its place as a key player in algorithm discussions.
Searching Algorithms
Searching algorithms facilitate the retrieval of data, playing a critical role in software development. Mastering different searching techniques can also demonstrate problem-solving abilities and efficiency in fetching required data.
Binary Search
Binary Search is a powerhouse in terms of efficiency because it operates on sorted data, achieving a time complexity of O(log n). This searching algorithm works by continually dividing the sorted dataset in half, effectively narrowing down the searched element's location. The key characteristic of Binary Search is its logarithmic time complexity, allowing for rapid data retrieval compared to slower searching methods.
One unique feature is that Binary Search requires the dataset to be sorted beforehand, which may be seen as a disadvantage in real-time scenarios where sorting isn't feasible. However, when used appropriately, it can escalate performance significantly, making it a crucial skill in coding interviews.
Linear Search
Linear Search takes a different approach by checking each element in the dataset sequentially. This searching method offers simplicity and operates best on small or unsorted datasets, showcasing a time complexity of O(n). Its main characteristic—the straightforwardness of implementation—makes it accessible for early learners in Java.
While its simplicity can be beneficial, Linear Search can turn into a bottleneck when dealing with large datasets, offering no optimization advantages. Although not as efficient as its counterparts, understanding Linear Search is fundamental as it lays the groundwork for more advanced searching techniques.
"A good programmer is someone who always looks both ways before crossing a one-way street."
Mastering algorithms, particularly sorting and searching, provides a solid foundation for tackling complex Java problems in interviews and beyond. With practice and understanding, candidates can build the necessary skills to impress potential employers.
Exception Handling in Java
Understanding how to manage exceptions is key in Java development. It serves as a safety net, catching runtime anomalies that may otherwise bring a program crashing down. Exception handling ensures that your application runs smoothly, providing a better user experience. Not only does this topic come up frequently in interviews, but it also reflects a programmer's capacity to foresee potential problems and mitigate risks. This is a vital skill, particularly when dealing with large or complex systems.
When candidates demonstrate a solid grasp of exception handling, they signal their readiness to tackle real-world challenges. An in-depth understanding of exception management helps in constructing robust applications that don’t just fail silently but inform the user of issues, thus enhancing maintainability. Taking the time to grasp these concepts can give candidates an edge in scenarios where performance and reliability are prioritized by potential employers.
Understanding Exceptions
Essentially, exceptions are events that disrupt the normal flow of execution in a program. They can arise from various issues, including hardware failures, invalid input, or even bugs in the program itself. Java provides a built-in framework for managing these issues through the use of , , and blocks.
Here’s how they generally work:
- Try Block: You wrap the code that might throw an exception in a try statement.
- Catch Block: If an exception occurs, control passes to the catch block, where you can specify how to handle that particular exception.
- Finally Block: This block, if present, executes after the try and catch blocks, whether or not an exception occurred, making it perfect for cleanup code.
Using exception handling wisely not only helps in debugging but also brings clarity to code. For instance, when reading a file, if it doesn’t exist, a well-placed exception can notify the developer or system operator rather than causing the application to stall.
Common Exception Questions
In Java interviews, candidates might frequently encounter specific questions around exception handling to gauge their understanding and experience. Here are some of the common queries that may arise:
- What is the difference between checked and unchecked exceptions?
Checked exceptions are those that a method must either handle or declare, like . Unchecked exceptions, on the other hand, are not required to be caught or declared and include and . - How do you create a custom exception?
You can create a custom exception by extending the class. This enables you to throw and catch exceptions specific to your application’s needs.
- Why is it critical to use finally blocks?
Finally blocks are usually employed for resource cleanup, such as closing files or database connections. Even if an exception is raised, the code in the finally block runs, ensuring that resources are freed as anticipated.
Java Multithreading and Concurrency
Understanding Java Multithreading and Concurrency is crucial for any developer aiming to work with Java-based applications. These concepts not only enhance a program’s performance but also ensure efficient resource management in a multi-core processing environment. Multithreading allows for parallel execution of code, meaning operations that could normally block or slow down an application can proceed without waiting on others. This results in a smoother experience for users, as responsiveness is maintained even under heavy loads.
Moreover, as systems grow more complex, handling multiple tasks concurrently becomes a necessity. Java's built-in features significantly simplify these processes, but familiarity with the underlying principles is essential. Developers armed with this knowledge can address challenges related to thread safety, race conditions, and deadlocks effectively.
Thread Management
Thread management is at the heart of achieving optimal performance through concurrency in Java. It encompasses the creation, scheduling, and management of threads during program execution. Java, with its class and the interface, offers straightforward ways to manage threads. For instance, a developer can create threads for different tasks that run simultaneously, thus maximizing CPU usage.
Additionally, it’s vital to monitor thread states—running, waiting, or blocked—to effectively diagnose issues that may arise during execution. A classic pitfall in thread management is improper handling of shared resources which can lead to inconsistent states or crashes. By employing synchronization techniques, developers can avoid such failures and ensure safe access to shared resources.
Concurrency Utilities
Java provides a range of concurrency utilities that truly elevate the ability to work with multithreaded environments.
Executor Framework
The Executor Framework is a powerful tool designed to manage threads effectively in Java. It abstracts away the complexities involved in direct thread management. Instead of manually creating and managing threads, developers can utilize the framework to handle this more efficiently. One key characteristic of the Executor Framework is its ability to pool threads, which minimizes the overhead of frequently creating and destroying threads.
The Executor Framework streamlines the execution of asynchronous tasks while offering scalability and performance, making it a favored choice among developers.
Among its several features, the ability to queue tasks allows developers to control how tasks are executed and processed. However, using this framework is not without its challenges. Mismanagement of task queues or pools can lead to thread starvation and reduced performance. Understanding how to configure and tune parameters is paramount for optimal usage.
Synchronization
Synchronization in Java is another essential aspect that helps maintain thread safety when multiple threads access shared resources. It allows developers to prevent thread interference by ensuring that only one thread can execute a block of code at any given time. The keyword is fundamentally significant in this context, locking the resources as needed. This characteristic is beneficial because it guarantees consistency in applications that require precise control over data access.
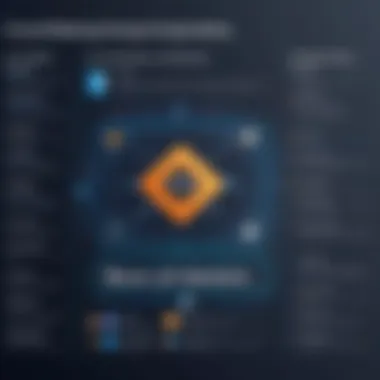
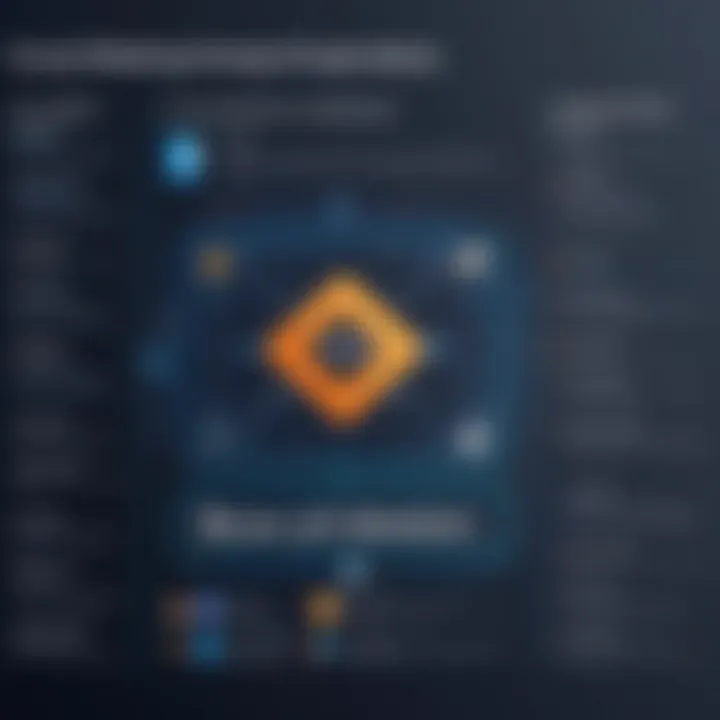
However, excessive synchronization can result in performance bottlenecks, leading to decreased application efficiency. Balancing the need for data integrity against the performance cost of synchronization is a delicate dance. Prudent use of synchronization mechanisms such as or can help mitigate the risks while maintaining system performance.
By understanding these key elements—thread management, the Executor Framework, and synchronization—developers can navigate the complexities of Java multithreading and concurrency. The insights gained here serve as a solid foundation for tackling more sophisticated concepts in the realm of Java programming.
Advanced Java Concepts
Understanding advanced concepts in Java is key for developers aiming to elevate their skills and prepare effectively for interviews. Mastering these concepts not only enhances coding proficiency but also opens up pathways to tackling more complex problems. In the fast-paced world of technology, being adept in advanced Java features can make a significant difference in a candidate’s employability.
Java Streams API
The Java Streams API is a powerful feature introduced in Java 8 that allows for functional-style operations on collections. It provides a clear and concise way to process sequences of elements—like lists, sets or arrays—without the need for boilerplate code. This feature is particularly significant in interview scenarios, as it encapsulates modern programming principles, illustrating how developers can write cleaner and more efficient code.
A few of the key benefits include:
- Efficient Data Processing: Streams enable bulk operations on collections, which can lead to performance improvements by utilizing internal iterations over external iterations.
- Readability and Expressiveness: By using method chaining and lambda expressions, the code clarity is noticeably enhanced compared to traditional approaches.
- Parallel Processing: For data-intensive applications, streams can be run in parallel with minimal overhead, leveraging multicore architecture to improve performance.
"Utilizing the Streams API can drastically simplify operations and enhance performance, embodying the core principles of functional programming."
Functional Programming in Java
Functional programming is an essential paradigm that emphasizes immutability, first-class functions, and pure functions. Java, while traditionally object-oriented, has integrated functional programming principles that can significantly improve code clarity and reusability. Understanding this paradigm can set candidates apart in interviews, as it showcases their ability to adapt to evolving programming styles.
Some highlights of functional programming in Java include:
- Lambda Expressions: This feature allows you to write instances of single-method interfaces (functional interfaces) more succinctly, facilitating concise implementations for methods like , , or within streams.
- Higher-Order Functions: Java supports passing functions as arguments, enabling more abstract code writing, where functions can be saved in variables or passed as parameters.
- Immutability: By focusing on immutable data, developers reduce the likelihood of side effects, enhancing the integrity and maintainability of the code.
In summary, advanced Java concepts and the understanding of Java Streams API along with functional programming principles are undeniably crucial for candidates aiming to pass their Java interviews. Being versed in these areas not only prepares individuals for technical questions but also equips them with the tools to solve problems effectively and elegantly.
Best Practices for Interview Preparation
Preparing for a Java interview is not just about crunching numbers or memorizing syntax; it’s an art that blends knowledge with strategy. Adequate preparation can make the difference between landing your dream job and walking out of an interview with your head hung low. Knowing this, candidates should approach their preparation with careful consideration of best practices tailored for Java interviews.
A well-structured plan allows candidates to identify areas that need attention and to hone their skills effectively. One vital aspect revolves around maintaining a balance between theoretical knowledge and practical application. It’s not enough to understand object-oriented programming concepts in isolation. Candidates must also be adept at applying these in problem-solving scenarios. This is where hands-on coding and practice come into play, providing the opportunity to grapple with real-world problems and develop a clear, intuitive grasp of Java.
Key Elements of Best Practices:
- Continuous Learning: Stay updated with the latest Java versions and frameworks. The language evolves, and so should you.
- Understand Job Descriptions: Tailor your study to match the specific technologies and methods mentioned in targeted job descriptions. This refines your focus.
- Practice Mock Interviews: Simulating real interview conditions helps build confidence and identifies areas needing improvement.
"Practice is the best of all instructors." – Publilius Syrus
Following these practices not only boosts your readiness but also instills a degree of confidence that is palpable in interviews.
Hands-On Coding Practice
Hands-on coding practice is the bread and butter of effective Java interview preparation. Theoretical knowledge often fails to translate effectively in a high-stakes environment, and practical coding can bridge that gap. Engaging in coding challenges on platforms specifically tailored for this purpose can sharpen your skills and expose you to different problem-solving approaches.
Candidates can benefit immensely from utilizing resources like LeetCode, HackerRank, and CodeSignal, which focus on diverse coding challenges ranging from simple syntax to advanced algorithms.
- Identify Key Areas:
- Build Small Projects:
- Focus on data structures like arrays, lists, and maps.
- Be prepared to implement sorting and searching algorithms through coding exercises.
- Create mini-projects using Java to enhance understanding of concepts and frameworks, such as Spring or Hibernate.
By regularly engaging in coding exercises, you become familiar with common pitfalls and develop a more fluid coding style, which in turn enhances your performance during the interview itself.
Study Resources
Selecting the right study resources is just as crucial as the practice itself. The digital age offers an abundance of material that can help Java candidates prepare thoroughly. It’s essential to sift through the available resources to pinpoint those that offer comprehensive coverage of Java topics relevant to interviews.
A few reputable resources include:
- Books:
- Online Courses:
- Online Communities:
- "Effective Java" by Joshua Bloch provides best practices and insights for writing efficient Java code.
- "Java: The Complete Reference" by Herbert Schildt covers everything from the basics to advanced topics in Java.
- Platforms like Coursera and Udemy offer curated Java courses that cater to various skill levels.
- Participating in discussions on Reddit or Stack Overflow can provide real-world context to theoretical knowledge, along with networking opportunities.
In essence, a mixture of practical exercises and solid theoretical grounding equips candidates for interviews. Keep in mind that dedication and consistency in your studies ultimately pave the path to success.
Simulating Java Interviews
In the journey to mastering Java and landing a desirable position, practicing through simulations can be a game changer. Simulating Java interviews provides candidates with the opportunity to immerse themselves in an interview-like environment. This practice focuses on refining one’s ability to articulate thoughts clearly and efficiently under pressure, mimicking the actual queries they may face in a real interview situation. Contextualizing questions within simulated scenarios prepares candidates for both common and unexpected inquiries.
The key benefits of simulating Java interviews include not only enhancement of technical skills but also bolstering confidence. When candidates engage in mock sessions, it often highlights areas that need improvement. Being aware of one's weaknesses can transform anxiety into an actionable plan for growth. Furthermore, candidates can develop essential soft skills such as communication, which many hiring managers prioritize.
It’s important to keep a few considerations in mind. First, the quality of the practice matters significantly. Using realistic questions that reflect the current industry standards ensures that candidates are truly prepared. Candidates should also seek feedback post-simulation to optimize learning outcomes. Ultimately, the goal is not just to get through the simulation but to ensure that improved performance translates into actual interview success.
Mock Interviews
Mock interviews act as practice run-throughs that encompass a range of Java-related questions and problems. They can either mimic a panel situation or resemble a one-on-one conversation with a potential employer. Often, the critical aspect of these mock interviews lies in their ability to offer immediate feedback. Candidates can learn how to approach difficult questions and provide articulate responses, simulating the pressure of a real-world technical discussion.
In preparing for a mock interview, candidates should consider the following:
- Define the scope: Focus on specific topics such as algorithms, OOP principles, or data structures depending on one’s expertise level.
- Time management: Practicing within a set time constraint allows for a more realistic experience. It helps to simulate the time pressure that usually exists in interviews.
- Engage in peer or mentor reviews: Getting insights from someone perceived to be more knowledgeable can provide direction on what areas need more focus.
Utilizing platforms such as reddit.com can help candidates connect with others looking to practice mock interviews, encouraging a sense of community and shared learning.
Peer-to-Peer Practice
Incorporating peer-to-peer practice into interview preparations can add tremendous value. This method allows candidates with similar goals to pair up and conduct practice interviews with each other. It’s about mutual growth; each participant takes turns in playing the role of both interviewee and interviewer. This dual role helps to foster a deeper understanding of what questions may arise and how best to address them.
Self-directed learning through peer interactions often yields a more enriching experience due to diverse perspectives. Participants might have different strengths which can aid each other effectively. Moreover, when friends or colleagues practice together, they are more likely to share constructive criticism that could prove invaluable.
To maximize the experience, participants should keep these pointers in mind:
- Create structured scenarios: Define clear roles, criterial, and specific areas to cover for each session.
- Rotate partners regularly: Practicing with multiple peers exposes candidates to a variety of questioning styles and problem-solving approaches.
- Follow-up discussions: Post-interview feedback sessions are crucial, where partners can discuss what went well and what needed improvement.