Java Programming Interview Code Examples
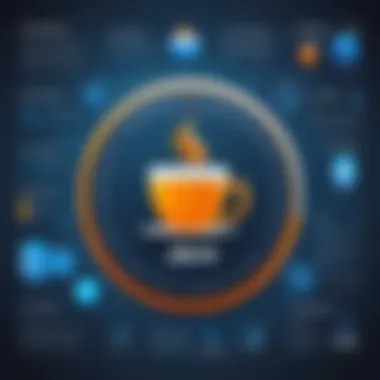
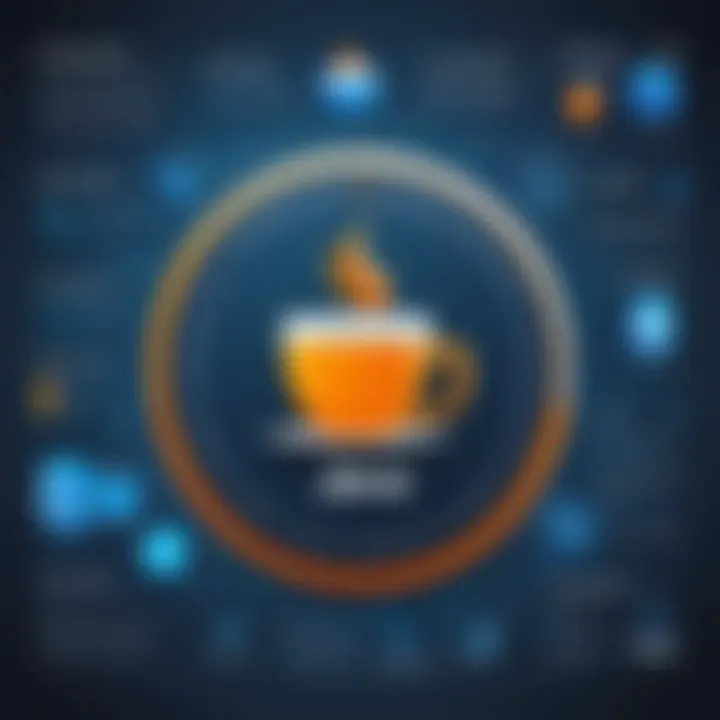
Preamble to Programming Language
Java is more than just a programming language; it embodies a philosophy of flexibility, efficiency, and compatibility. Since its inception in the mid-1990s, Java has consistently been a pioneer in the software development landscape.
History and Background
The roots of Java trace back to a project initiated by James Gosling and his team at Sun Microsystems. Originally launched under the name Oak, the language was designed for interactive television but quickly pivoted towards the burgeoning world of the internet. In 1995, it was officially rebranded as Java, and this shift marked the beginning of its journey into the hearts and minds of developers.
Features and Uses
Java offers a robust toolbox for creating application software. Key features include:
- Platform Independence: Write once, run anywhere (WORA) is a cornerstone principle of Java, made possible by the Java Virtual Machine (JVM).
- Object-Oriented: Java is built around the object-oriented programming paradigm, which fosters cleaner and more manageable codebases.
- Memory Management: Automatic garbage collection frees developers from the burden of manual memory management.
These features make Java the go-to language for web applications, enterprise software, mobile apps, and even cloud computing solutions. It is prevalent in many fields, such as finance, healthcare, and education.
Popularity and Scope
As of recent years, Java ranks among the top programming languages globally. According to various programming surveys, it remains a favorite for developers. Its widespread use in large scale systems and Android development ensures its relevance well into the future. On platforms like Reddit, enthusiasts and professionals converge to discuss tips, ask questions, and share insights about using Java.
In summary, understanding Java is not just about learning syntax and code; it’s about grasping its widespread utility in real-world applications.
Basic Syntax and Concepts
Diving into Java involves familiarizing yourself with its basic syntax and concepts, which set the stage for more complex programming tasks.
Variables and Data Types
Variables in Java act as placeholders for data. The language offers several data types, including:
- Primitive types: like , , , and , each serving distinct purposes based on the type of data stored.
- Reference types: such as objects and arrays, which hold references to their data.
Declaring a variable is straightforward:
Operators and Expressions
Operators in Java help manipulate data and variables. Common operators include arithmetic, relational, and logical operators. Expressions combine these operators and operands to produce a value. For instance:
Control Structures
Control structures govern the flow of your programs. Java employs constructs like , , and loops such as and .
Example of an if structure:
Control structures are essential for guiding your program's logic and handling different outcomes effectively.
Advanced Topics
Once you’re comfortable with the basics, Java opens up with a host of advanced topics that enhance your coding toolkit.
Functions and Methods
Functions, or methods in Java, let you encapsulate code for specific tasks. They promote reusability and modular design. Defining a method might look like this:
Object-Oriented Programming
Object-oriented programming (OOP) is a monumental aspect of Java. Concepts like encapsulation, inheritance, and polymorphism allow for effective structuring of your code. For instance, you could create a class for a and derive subclasses for different types.
Exception Handling
Java manages errors through exception handling, ensuring your program can recover from unexpected events. Using try-catch blocks is a common way to handle errors gracefully:
Hands-On Examples
Without practice, knowledge is fleeting. The following examples range from simple programs to more involved projects.
Simple Programs
Starting with simple exercises can help cement your basic understanding. For example, writing a program to print "Hello, World!" sets the groundwork for your journey:
Intermediate Projects
Once you're comfortable, try tackling more challenging projects like creating a basic calculator or a small guessing game. Such tasks will refine your skills and deepen your understanding of Java.
Code Snippets
Throughout interviews, candidates can be asked to quickly write snippets. Familiarity with useful code snippets can bolster confidence. For instance, a quick sort implementation can showcase your grasp of algorithms:
Resources and Further Learning
To deepen your understanding, rely on quality learning resources:
- Recommended Books and Tutorials: Books like "Effective Java" by Joshua Bloch and "Head First Java" provide profound insights.
- Online Courses and Platforms: Platforms like Coursera and Udemy have comprehensive Java courses tailored to various levels.
- Community Forums and Groups: Engaging with others through forums such as Facebook Groups or Reddit can provide support and new perspectives.
Continual learning and practice are essential to mastering Java. Explore resources, build projects, and engage with the community. The journey is just as important as the destination.
Preface to Java for Interviews
Diving into the world of Java for interviews is like opening a door to a treasure trove of opportunities. Java is not just a programming language; it's a versatile tool that many modern companies value. In today's tech-driven job market, understanding Java can be the key that unlocks career prospects. This section emphasizes why having a solid grasp of Java is essential and what candidates should focus on to succeed in technical interviews.
Importance of Java Knowledge in Modern Interviews
Java occupies a pivotal place in the landscape of programming languages, widely used across various industries for web, mobile, and enterprise applications. The real-world demand for Java skills makes it a hot commodity in job interviews. Companies like Google, Amazon, and IBM actively seek candidates proficient in Java. The language's reliability, scalability, and extensive library support contribute to its widespread acceptance among employers.
When preparing for interviews, being able to discuss and demonstrate Java concepts can significantly increase one's chances of landing a job. It shows potential employers that a candidate can not only write code fluently but can also tackle complex problems. Understanding Java allows candidates to answer questions related to frameworks like Spring and Hibernate, delve into database handling with Java Database Connectivity (JDBC), and explain their design decisions during technical discussions.
"Knowledge of Java is akin to having a golden ticket in the tech world."
Overview of Common Java Concepts
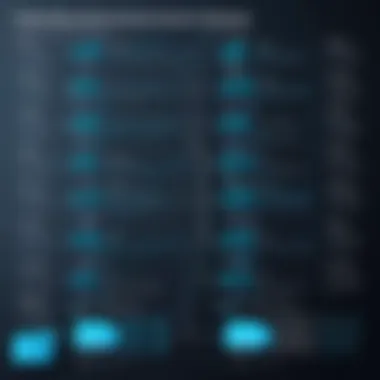
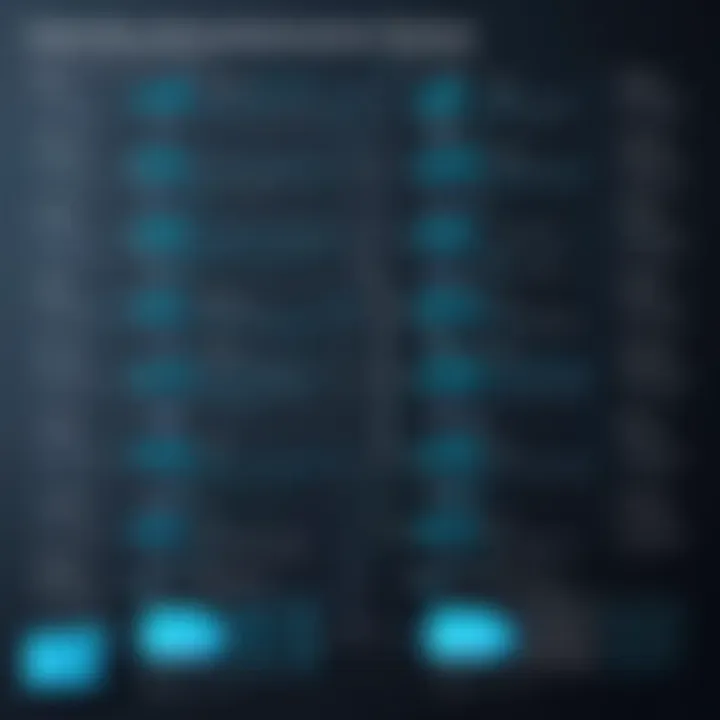
To get a handle on what interviewers might ask, candidates must familiarize themselves with fundamental Java concepts that form the building blocks of the language. Here are some key areas to focus on:
- Data Types and Variables: Understanding the types of data Java can handle is crucial when it comes to memory management and performance. This includes both primitive types such as , , , and reference types like arrays and objects.
- Control Structures: Java's decision-making constructs, including if-else statements, switch cases, and loops, are essential for writing efficient programs. A solid grasp of these is critical for solving algorithm-based questions in interviews.
- Object-Oriented Programming (OOP): Since Java is deeply rooted in OOP principles, candidates should be well-versed in concepts like classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
- Exception Handling: Java's approach to error management is vital for building robust applications. Knowing how to use try-catch blocks, and understanding the differences between checked and unchecked exceptions, can showcase a candidate’s coding proficiency.
- Collections Framework: Java provides a rich API that includes various data structures such as lists, sets, and maps. Familiarity with these can come in handy during coding challenges, as efficient data handling directly affects performance.
- Streams and Lambda Expressions: With the rise of functional programming in Java 8, candidates should also be familiar with streams and lambda expressions, as they simplify data processing tasks.
Focusing on these topics can help interviewees to enter the interview room with confidence, armed with the knowledge required to impress potential employers. The journey through Java may seem daunting, but with determination and the right resources, one can navigate it successfully.
Fundamental Java Concepts
Fundamental Java concepts are the backbone of understanding the language's core functionality. A solid grasp of these basic elements paves the way for mastering more advanced topics. In interviews, the ability to demonstrate this foundational knowledge can set a candidate apart. Not only does it display the depth of one’s understanding, but it also highlights problem-solving skills in coding challenges.
Data Types and Variables in Java
Primitive Data Types
Primitive data types in Java refer to the most basic types of data built into the language. These data types include boolean, char, byte, short, int, long, float, and double. They serve as a fundamental tool for a programmer since they represent simple values directly. One key characteristic of primitive data types is their efficiency in terms of memory usage. For instance, an takes up 4 bytes of memory, which makes calculations quick and straightforward. This efficiency is a huge benefit especially when performance is key in coding interviews.
A unique feature of primitive data types is that they hold data values directly, unlike reference types that point to objects in memory. While using primitive types can lead to more memory-efficient programs, they lack methods and properties that objects possess. However, in simple scenarios, their simplicity might just be what you need to get the job done.
Reference Data Types
Reference data types, on the other hand, allow you to create complex data structures. Unlike primitive data types, which hold their values directly, reference data types hold references to their data, which means they point to the actual data stored in the heap memory. Common examples include arrays, Strings, and user-defined classes. Their ability to encapsulate and manage multiple values makes them an indispensable part of Java programming.
One of the distinguishing features of reference data types is their ability to interact with methods and have properties associated with them. This capability allows developers to create modular and scalable applications, making reference data types a popular choice in coding examples. However, since they involve additional overhead for memory management, they may not always be as performant as primitive types in every use case.
Control Structures
Control structures are a vital aspect of Java programming, guiding the flow of execution based on conditions. Understanding how to implement them effectively can drastically improve the logic of your solutions.
If-Else Statements
If-else statements facilitate decision-making processes in code. They allow programmers to execute different blocks of code based on boolean conditions. The beauty of if-else statements lies in their simplicity and clarity. They can be employed to handle various scenarios—from processing user inputs to branching logic based on complex business rules. An important characteristic is their straightforward use case: if the condition holds true, execute one block; otherwise, run another.
This approach can be immensely beneficial for structuring code in a readable way. However, they can quickly become unwieldy if not managed properly. Overusing nested if-else statements may lead to what’s famously called “spaghetti code,” which is challenging to read and maintain.
Switch Cases
Switch cases are another tool for handling multiple conditions, facilitating a cleaner syntax when one must deal with numerous possibilities. This structure is generally favored when evaluating a single variable against a list of potential values. Its organization allows for more readable and maintainable code compared to extensive if-else chains.
A notable feature of switch cases is that they provide a break statement to exit the switch block. This design prevents the execution from inadvertently flowing into subsequent case statements. On the downside, switch cases are less flexible than if-else statements since they work best with specific data types, like enumerated types and integers.
Loops
Loops form an essential part of Java programming, particularly for tasks that involve repeated execution of code blocks. There are several types, including for loops, while loops, and do-while loops, each serving its own purpose. Loops contribute significantly to efficiency, allowing you to automate repetitive tasks such as iterating through arrays or lists.
A key characteristic of loops is their ability to reduce redundancy in your code. Instead of writing repetitive instructions multiple times, a single loop can do the heavy lifting. However, care must be taken to manage the loop's termination condition effectively. Infinite loops can occur if exit conditions are neglected, leading to resource consumption and program crashes.
In summary, mastering these fundamental concepts in Java equips candidates with vital skills that are often scrutinized in interviews. The depth of knowledge reflected in these areas often showcases a candidate’s readiness for tackling more complex programming challenges.
Object-Oriented Programming in Java
Object-Oriented Programming (OOP) is a cornerstone for software development in Java. Understanding OOP is not just crucial for mastering Java itself, but also for performing well in interviews, where a solid grasp of these concepts often sets top candidates apart. OOP provides several inherent benefits, such as enhanced code reusability, improved maintainability, and a clearer structure, making it the go-to paradigm for many modern software applications.
Classes and Objects
Class Definition
At its core, a class in Java is a blueprint for creating objects. It defines a collection of attributes and behaviors encapsulated in a single structure. This encapsulation is not only crucial for organizing code but also for instilling a strong sense of modularization. A defining characteristic of class definition is that it allows programmers to group related data and methods a way that reflects real-world problems.
The key characteristic of class definition is its ability to encapsulate state and behavior together. Unlike simple function-based paradigms, classes lead to cleaner and more intuitive designs. For the article's focus, this encapsulation is beneficial as it results in code that can be easily understood and modified, crucial aspects during interviews.
A unique feature to consider is how classes support constructors, which are special methods to initialize new objects. Constructors can accept parameters, making class instantiation flexible and powerful. There’s an advantage here; however, it can also lead to complexity if not managed properly.
Object Creation
The act of creating an object is a fundamental aspect that ties into the core principle of OOP: instantiation. When a class is defined, objects are created from this blueprint which brings the class to life. A notable attribute of object creation in Java is its simplicity and the clarity it provides. Using the keyword, developers can instantiate objects with ease, ensuring that those objects are independent and maintain their own states.
The key characteristic here is that every object maintains its unique data. This independence enhances the modular nature of Java applications. The article promotes this aspect since it encourages candidates to think in terms of discreet components during interviews.
However, object creation can also pose challenges. For instance, excessive object creation can lead to poor performance issues, such as increased memory consumption, which is something candidates must be aware of.
Inheritance and Polymorphism
Single and Multiple Inheritance
Inheritance allows a new class to inherit attributes and methods from an existing class. In Java, it primarily supports single inheritance; a class can inherit from one superclass. This feature promotes a clear hierarchy and simplifies code. The single inheritance model proves beneficial as it keeps the class structure straightforward, aiding comprehension during interviews.
However, Java does have interfaces that facilitate multiple inheritance. Using interfaces, a class can implement multiple types, which effectively bypasses the restrictions of single inheritance. This unique feature is important for candidates to understand, as it showcases Java’s flexibility and depth in handling complex relationships.
One downside of single inheritance could be the inflexibility in extending multiple features. In contrast, interfaces offer a workaround but may complicate class structures if not applied judiciously.
Method Overloading and Overriding
Method overloading and overriding are two powerful features of OOP that allow for greater flexibility and functionality. Overloading permits a class to have multiple methods with the same name, differentiated by parameters, which streamlines code and promotes ease of use without altering method names each time. This characteristic is a powerful tool in an interview setting, as it demonstrates a candidate's ability to write concise and efficient code.
On the other hand, method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This capability is key in achieving polymorphism, a concept where a single action can perform different tasks based on the object's data type.
Both features play into the article’s theme by emphasizing adaptability and efficient coding practices. Nevertheless, candidates must understand when to use these features appropriately, as overloading or overriding without clear intent can lead to convoluted code that detracts from readability.
Encapsulation and Abstraction
Access Modifiers
Access modifiers control the visibility of class members, a significant aspect of encapsulation. In Java, there are four access modifiers: , , , and . This multitude allows developers to finely tune the access level to their class attributes and methods.
The key characteristic of these modifiers is that they protect the integrity of the data, ensuring that objects interact in consistent ways. This is not only beneficial from a security standpoint but also instills good coding practices for interview candidates.
However, using too many modifiers can lead to overly complicated class structures. It's essential to strike a balance, making sure that encapsulation doesn’t turn into excessive hiding of information.
Abstract Classes and Interfaces
Abstract classes and interfaces in Java provide a means to achieve abstraction, leading to cleaner designs and reducing complexity. An abstract class can provide a base for other classes, allowing code reuse while also defining a template for subclasses. In contrast, interfaces define a contract that implementing classes must fulfill. This characteristic encourages programmers to think about shared behavior and design objectives.
In the context of the article, understanding these concepts allows candidates to structure their code with reusability and maintainability in mind. They also serve as significant markers for interviewers looking at how well candidates grasp abstraction. A distinct downside could be that while abstract classes can provide some method implementations, interfaces are fully abstract, which may lead to more boilerplate code.
Ultimately, mastering Object-Oriented Programming principles in Java is not just about writing code. It’s about understanding the underlying concepts, methodologies, and the reasoning behind them, which is pivotal for successfully navigating interviews.
Exception Handling in Java
Exception handling is a critical aspect of Java programming, especially when preparing for interviews. Understanding how to manage exceptions effectively not only helps in writing robust applications but also shows prospective employers that you are proficient in handling error situations gracefully. The concept underlines the importance of maintaining a smooth user experience even when something goes awry during program execution.
When a program encounters unexpected situations, such as user input errors or network issues, exceptions come into play. Mastery of this topic can distinguish a competent candidate from the rest. Interviewers often look for candidates who can explain how they would handle various types of errors and exceptions in real-world applications.
Understanding Exceptions
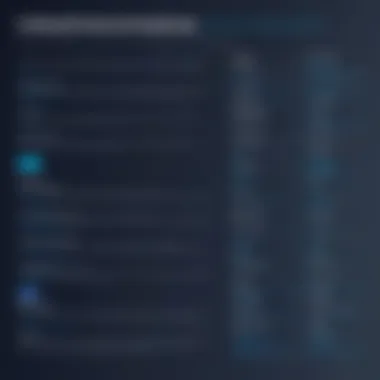
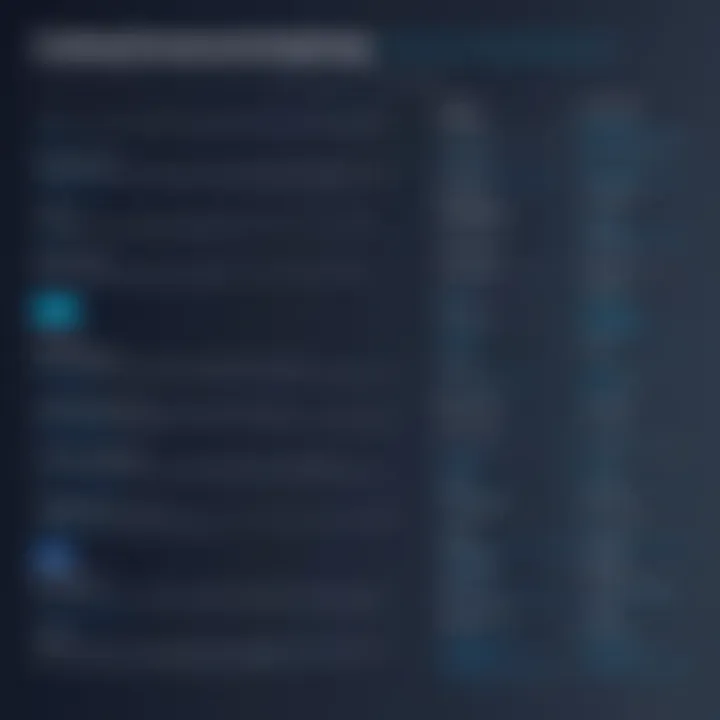
In Java, exceptions are events that disrupt the normal flow of the program's instructions. They can arise from a myriad of situations, ranging from programming mistakes to external issues. Grasping the nuances between checked and unchecked exceptions is foundational for any Java developer.
Checked vs Unchecked Exceptions
One critical distinction in exceptions is between checked and unchecked exceptions. Checked exceptions are checked at compile-time, meaning the programmer needs to either handle these exceptions through a block or declare them in the method signature using the keyword. This characteristic encourages developers to think proactively about potential issues during development.
On the flip side, unchecked exceptions occur at runtime. They do not need explicit handling, which makes them somewhat easier but could lead to runtime errors if not anticipated. This makes unchecked exceptions a common source of bugs if a programmer isn't diligent in their practices.
Using both types strategically can enhance your code's robustness. For example, by using checked exceptions to enforce handling of known issues like file not found errors while leaving unchecked exceptions to address programming mistakes allows for a clean approach.
Common Exception Types
Recognizing common exception types is also essential, as it helps in understanding how to deal with various scenarios.
- NullPointerException: Occurs when trying to use a null reference.
- ArrayIndexOutOfBoundsException: Happens when attempting to access an invalid index in an array.
- ArithmeticException: Arises from illegal arithmetic operations, like division by zero.
These exceptions are frequently encountered in coding interviews and real-world applications alike. Knowing when and how these exceptions occur will significantly improve your problem-solving skills.
Try-Catch Blocks
The mechanism is the backbone of exception handling in Java. It allows developers to write code that will "try" to execute and "catch" exceptions if they happen.
Nesting Try-Catch
Nesting blocks can be handy in situations where you expect multiple exceptions across different sections of a code. This flexibility can enhance your error handling strategy by separating concerns.
For instance, one can catch exceptions for file I/O operations in one block while handling database exceptions in another, thus keeping the logic clear and manageable. However, overusing this feature could lead to code that is hard to read and maintain.
Finally Block
A crucial part of the exception handling strategy in Java is the block. This block will always execute after a block finishes, regardless of whether an exception was thrown or caught. This makes it an excellent place for cleanup activities, like closing a file or releasing database connections.
Utilizing a block simplifies resource management and greatly enhances the reliability of your applications. For example, if you open a file for reading, placing the close operation in the block ensures it gets executed even if an exception occurs during reading.
"Proper exception handling can mean the difference between a stable application and one that crashes at the first hurdle."
Ultimately, being adept at exception handling is a skill that showcases a developer's robustness in dealing with the unpredictable world of programming. This not only opens doors during interviews but also contributes to writing code that stands the test of time.
Collections Framework in Java
In the realm of Java programming, the Collections Framework stands as a cornerstone for managing groups of objects. This framework brings forth a structured approach to handle data in terms of lists, sets, and maps, allowing developers to create flexible and efficient applications. During interviews, showcasing a solid understanding of the Collections Framework can highlight one’s programming prowess, as it encompasses not only the ability to manipulate data but also the understanding of complexity and performance trade-offs. Thus, a candidate's familiarity with these tools reflects their readiness for real-world coding challenges.
Prelims to Collections
In Java, Collections offer a standard way to store, retrieve, and manipulate data. They include various interfaces and classes specifically designed to handle groups of objects. When tackling coding interviews, being well-versed in Collections helps candidates quickly implement solutions that are both effective and elegant.
List, Set, and Map Interfaces
The List, Set, and Map interfaces are key players in the Collections Framework. Each of these interfaces serves a different purpose, tailored to specific needs of data handling.
- List: This interface allows duplicate elements and maintains the order of insertion. Its versatility makes it a popular choice for situations where the order is critical, such as maintaining a playlist or user actions in an application.
- Set: Unlike List, the Set interface prevents duplicate values. It’s useful in scenarios where uniqueness is vital, like storing unique user IDs or distinct items in a database.
- Map: This interface represents key-value pairs and allows for efficient lookups. It’s especially handy for situations where associations between objects are required, such as maintaining user profiles or configurations.
The key characteristic that sets aside List, Set, and Map is how they handle data so differently, catering to various requirements in a program. A well-designed application often relies on a combination of these interfaces, enabling efficient data organization and manipulation.
However, their unique features also come with trade-offs. For instance, while List offers ordered storage, it may incur performance overhead when searching for elements compared to a Set. Similarly, while Maps provide quick lookups, they usually consume more memory than a List.
Common Implementations
When diving deeper into the Java Collections Framework, it’s important to also understand the common implementations of these interfaces.
- For List, implementations such as ArrayList and LinkedList are standard. ArrayList gives quicker access to elements, but a LinkedList shines in scenarios where insertion and deletion operations are frequent.
- For Set, options like HashSet and TreeSet come into play. HashSet is great for quick lookups but does not maintain order. Meanwhile, TreeSet keeps the elements in sorted order, providing easy access to the lowest and highest values.
- In terms of Map, the HashMap offers fast retrieval based on keys, while TreeMap ensures sorted key values.
Each of these implementations demonstrates a different approach to handling the underlying data structure, allowing programmers to select the one best suited to their task. The unique feature of these implementations lies in how they balance efficiency with functionality, letting developers optimize their applications.
Iterating over Collections
When dealing with Collections, an essential skill is the ability to effectively iterate through these data structures. The chosen method of iteration can significantly impact the readability and performance of code.
For-each Loop
The for-each loop (or enhanced for loop) provides a clean and readable way to iterate through elements in a Collection. This method eliminates the need for an index variable, simplifying the code.
- Its key characteristic is that it does away with boilerplate code that one might find in a standard for-loop. By using this loop, programmers can write concise, less error-prone code.
- However, a limitation of the for-each loop is its inability to modify the Collection during iteration, which could lead to exceptions if one tries to alter the structure mid-loop. A programmer must plan accordingly to avoid pitfalls when using this approach.
Iterators
For more complex scenarios where Collection modification is required, Iterators are invaluable. An Iterator allows both navigation through the elements and modification of the Collection as you loop through.
- The key characteristic of Iterators is their ability to traverse through any Collection type with a consistent API, making them a universal tool in a programmer's kit.
- Unlike the for-each loop, Iterators permit removing elements during iteration by using the proper methods, making them more flexible for certain use-cases.
While Iterators provide robustness, they also add a bit of complexity compared to the for-each loop. Managing the state and ensuring that the Collection isn't modified improperly requires extra caution from a developer, which is sometimes challenging, especially for beginners.
Understanding how to leverage the various components of the Collections Framework can vastly influence the performance and efficiency of Java applications.
In summary, whether it’s through Lists, Sets, Maps, or the various means of iteration, the Collections Framework offers a rich set of tools that every Java developer should grasp for both interviews and real-world applications.
Java Streams and Lambda Expressions
Java Streams and Lambda Expressions mark a significant evolutionary step for Java programming. Their introduction brought a functional programming approach to a language traditionally known for its object-oriented principles. Understanding these concepts is crucial, especially for technical interviews where efficient coding is paramount.
Prelude to Functional Programming in Java
Functional programming encourages immutability and the use of functions as first-class citizens. This paradigm shift allows developers to write clearer, more concise, and maintainable code. Java Streams and Lambda Expressions take advantage of this approach, making operations with collections much simpler. For instance, operations that once required loops can now be accomplished in one or two lines of code, enhancing both readability and efficiency. In the fast-paced world of technology, these features provide a competitive edge in interviews.
Working with Streams
Creating Streams
Creating Streams in Java simplifies the handling of a sequence of elements, whether they're from a collection, array, or even I/O channels. The ability to implement a process without manually iterating through lists is one of its standout features. This not only saves time but also minimizes the chances for bugs which commonly arise in traditional loop constructs. A Stream can be created in several ways:
- From Collections: You can easily create a stream from any collection using method.
- From Arrays: Utilizing allows one to convert arrays to streams directly.
- Generating Streams: The or methods offer a chance to create infinite streams, providing flexibility based on needs.
Here's an example:
This characteristic not only leads to less code but also promotes more readable solutions, making it a preferred choice among developers.
Stream Operations
Once a Stream is created, various operations can be performed on it. These operations can be categorized into two types: intermediate and terminal operations. Intermediate operations might include operations such as , , or , which build a new stream without altering the original. Terminal operations, on the other hand, like or , produce a result or a side effect, concluding the stream.
The use of Stream Operations is beneficial when one desires an efficient and expressive means to handle data. For example, filtering out specific entries from a collection becomes intuitive with Streams:
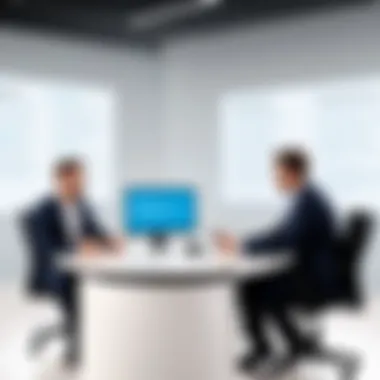
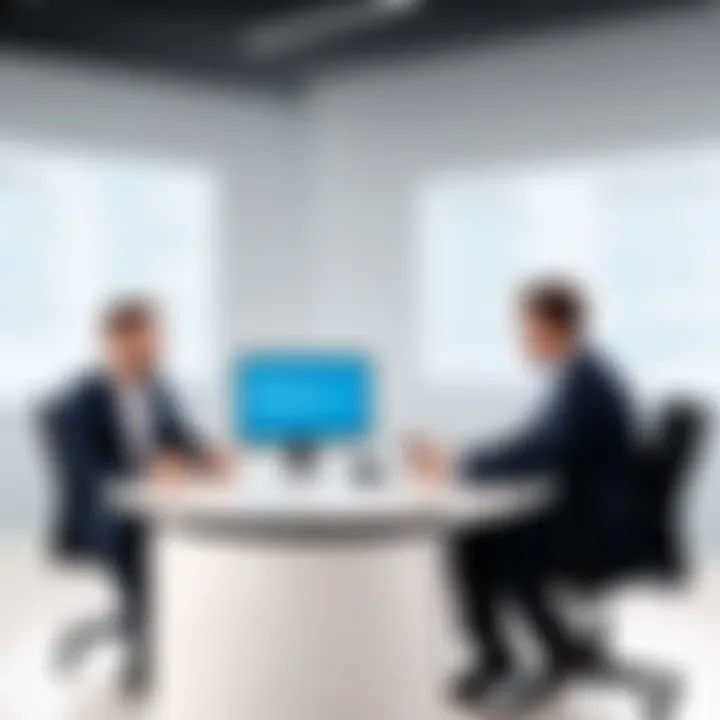
Streams embrace laziness which means they only process the data when absolutely necessary. This can lead to performance improvements, especially when working with large data sets. However, it can also lead to pitfalls like too much reliance on this feature can lead to obscured logic for those who are not well-versed with functional programming.
Summary
Java Streams and Lambda Expressions represent modern Java programming at its finest. Their ability to simplify coding practices while maintaining performance is critical, particularly for candidates eager to make their mark during technical interviews. Learning how to effectively use these tools can define one's preparation, and understanding their nuances can differentiate an adept coder from a novice. In a world that values concise and readable code, mastering these features is indeed a worthy investment.
Common Java Interview Questions
The realm of Java programming interviews often beckons candidates with a diverse range of inquiries. Common Java interview questions not only assess a candidate's technical knowledge but reflect their problem-solving capabilities as well. These questions can range from algorithmic puzzles to practical coding exercises, each tailored to unravel the depth of the interviewee's understanding of Java.
Understanding this topic is crucial for candidates. It highlights specific elements like coding efficiency, logical reasoning, and familiarity with core Java concepts. When diving into this section, you will uncover how to tackle algorithmic questions, essential sorting techniques, and searching methodologies. Learning about practical coding exercises is also paramount as they offer a platform to showcase your programming finesse and versatility in problem-solving.
Algorithmic Questions
Algorithmic questions form a substantial portion of technical interviews. They test not only one's ability to code but also their problem-solving aptitude and understanding of algorithm efficiency.
Sorting Algorithms
Sorting algorithms are significant because they are fundamental in organizing data. When sorting arrays or lists, algorithms like Quick Sort, Merge Sort, and Bubble Sort come to the forefront.
The major characteristic of sorting algorithms is their ability to efficiently arrange data, which is crucial for later retrieval operations. For example, if you need to find an element in a sorted array, using binary search drastically minimizes search time compared to unsorted data. This is why sorting algorithms are favored in this article.
A unique feature of sorting algorithms lies in their varying complexities. For instance, while Bubble Sort is easy to implement, it's very inefficient for large datasets. On the other hand, algorithms like Merge Sort are more complex but result in vastly improved performance.
Advantages of sorting algorithms include improved data handling and increased performance in search operations, while disadvantages often stem from their computational cost in terms of time and space.
Searching Algorithms
Searching algorithms complement sorting algorithms, focusing on locating specific elements within datasets. Two primary searching strategies are linear search and binary search.
The key characteristic of searching algorithms is that they attempt to optimize the retrieval process. For example, binary search operates on sorted datasets and drastically reduces search time compared to linear search, which is more straightforward but inefficient for larger arrays.
A unique feature of searching algorithms is the trade-off between simplicity and efficiency. While linear search is easier to grasp, binary search offers far superior performance in sorted arrangements.
Benefits of searching algorithms include rapid data retrieval and effective data organization. However, certain disadvantages can arise, particularly if the data structure isn't sorted. For instance, binary search requires an initial sorting step, which adds an overhead.
Practical Coding Exercises
Moving beyond theoretical knowledge, practical coding exercises are crucial for honing programming skills. They challenge candidates to apply concepts in real-world scenarios and measure their coding capabilities under timed pressures.
Problem-solving Challenges
Problem-solving challenges encourage candidates to think critically and creatively. These exercises often test the breadth of knowledge in Java and require a comprehensive understanding of algorithms and data structures.
A standout characteristic of problem-solving challenges is their diversity. They may range from simple string manipulations to complex graph algorithms. This variability makes them a beneficial component of our article.
One unique feature of these challenges is that they mirror real-world problems in software development. For instance, developing a login system or an inventory management tool can be encapsulated in these exercises.
Advantages include practicing coding under pressure while disadvantages could be that not all challenges strictly reflect everyday programming tasks, sometimes leading to excessive abstraction.
System Design Questions
System design questions delve into high-level architecture and scalability of applications. They often ask candidates to design complex systems such as distributed databases or microservices architecture.
A key characteristic of system design questions is the emphasis on scalability and efficiency. Candidates must demonstrate their understanding of how different systems interact, reflecting real-world picture of enterprise-level applications.
This article favors system design questions because they reveal a candidate's strategic thinking regarding system organization and future-proofing applications against growth.
One unique element of system design is its abstract nature, often requiring candidates to think about trade-offs between performance and resource usage. On the plus side, successful navigation through these questions often correlates with successful job placements. But they can also be quite intimidating and challenging.
Best Practices for Java Code in Interviews
In the realm of programming, proficiency isn't just a matter of knowing syntax or understanding algorithms; how you present your code matters a great deal too. Best practices in Java coding for interviews can set a candidate apart, making a significant impression on recruiters who are often inundated with resumes. Thus, having a solid grasp of these practices is crucial if one aims to succeed in technical interviews. Respecting these conventions not only boosts personal credibility but also communicates an understanding of industry standards.
Writing Clean Code
In this section, we dig into two main features of writing clean code — code readability and documentation and comments. Each of these elements plays a distinct role in developing maintainable and understandable code.
Code Readability
Code readability refers to how easily a person can scan and understand the code. A key characteristic of high readability is its logical consistency. When code aligns with the natural flow of language and common programming practices, it becomes significantly easier to interpret. This feature is beneficial as it enhances collaboration among multiple developers working on the same project.
For instance, using meaningful variable names and following naming conventions can dramatically improve clarity. Rather than naming a variable arbitrarily, naming it keeps intentions clear and states its purpose directly.
A unique feature of code readability is the arranging of code blocks. By breaking down complex methods into simpler ones, one enhances comprehension. The disadvantage could be that overemphasis on readability might sometimes lead to longer code than necessary. However, in interview scenarios, prioritizing clarity is generally a wise choice.
Documentation and Comments
Documentation and comments serve as the guiding light for understanding code. One of the key characteristics is that they provide context that might not be evident from the code alone. They are invaluable in explaining why certain decisions were made within the codebase. This practice tends to be beneficial because it assists not only the original developer in recalling their thought process but also others who might work on the code later.
For example, effective use of comments can clarify the purpose of a specific algorithm or the expected results of a method. A unique feature of comments in this context is the ability to outline potential pitfalls or bugs, which can avert future misunderstandings. However, too many comments or poorly written ones can clutter the code and detract from its readability.
Testing and Debugging Techniques
Testing and debugging play pivotal roles in delivering robust Java applications. This section discusses unit testing and debugging tools, focusing on how they contribute to reliable code and showcasing their importance for interview preparation.
Unit Testing
Unit testing is a critical aspect of the software development process. It focuses on testing individual components of the software to ensure each part functions as intended. A key characteristic of unit testing is its ability to catch bugs at an early stage, saving time and cost later in the development cycle. This practice is popular because it reinforces confidence in the code’s functionality before integration.
One unique feature of unit testing is that it promotes better design principles and leads to cleaner, more modular code. Writing unit tests often necessitates breaking code down into smaller, testable units, which naturally enhances clarity and functionality. An advantage of unit testing is it makes refactoring a safer process; however, it can lead to an overhead of additional code which might seem daunting at first.
Debugging Tools
Debugging tools provide developers a way to assess their code’s performance while it runs. The key characteristic of debugging tools is that they grant visibility into the program's operation. This makes it easier to spot and fix errors than sifting through lines of code manually. Using such tools can greatly improve efficiency, which is crucial in a real-time interview scenario.
A unique feature of debugging tools is their ability to inspect variables and view the program's call stack while it executes, giving developers deeper insight into runtime behavior. However, they might not always mimic production environments, leading to discrepancies that can confuse new programmers.
In summary, adhering to best practices for Java code in interviews is not just about knowing the right syntax. It also requires an understanding of how to write clear, maintainable code while leveraging effective testing and debugging techniques. This approach will impress interviewers and bolster personal confidence in coding abilities.
End
In wrapping up this guide, it's essential to recognize the pivotal role that mastering Java code examples can play in interviews. This thorough comprehension not only equips candidates with practical skills, but also cultivates a mindset tuned to problem-solving—an invaluable asset in the tech landscape.
Summary of Key Points
- Proficiency Matters: Knowing Java well goes beyond just syntax; it's about understanding how to implement concepts effectively. Companies look for candidates who can demonstrate coding proficiency during tests and discussions.
- Practice Makes Perfect: Regularly tackling algorithmic questions and practical exercises sharpens your coding ability. This consistent practice is crucial for interview readiness.
- Clean Code Philosophy: Writing clean and maintainable code reflects a developer's professionalism. Evaluators appreciate adherence to coding standards and best practices because it shows respect for collaborative projects in the work environment.
- Ask Questions: During interviews, it's beneficial to engage with your interviewers. Asking clarifying questions displays your critical thinking and eagerness to understand the problem at hand.
Final Thoughts on Preparing for Interviews
As the job market becomes increasingly competitive, the significance of thorough preparation cannot be overstated. Here are some tips to keep in mind:
- Simulate the Interview Environment: Practice coding problems in conditions similar to those you will face in interviews. Time yourself, use a whiteboard or simply code on paper without relying on any digital aids.
- Know Your Basics: Revisit fundamental concepts like data structures and algorithms. A solid foundation is often tested in interviews, and understanding these will allow you to solve more complex problems.
- Be Adaptive: Different organizations value different skills. Tailor your preparation based on the role you’re applying for. If the job description highlights knowledge in a specific area of Java, hone in on that aspect.
- Stay Calm and Confident: Nerves can get the best of anyone. Remember to breathe and approach each question methodically, showcasing your thought process rather than just racing to the solution.
"Preparation is key to success in any endeavor. It’s your first step towards grabbing that opportunity you’ve been waiting for."
Through a blend of preparation, understanding concepts, and developing solid coding habits, candidates can enter and thrive in interviews with confidence. The insights shared within this article serve as a toolkit for aspiring programmers aiming to elevate their skills in the world of Java.