Crucial Java Coding Interview Questions to Master
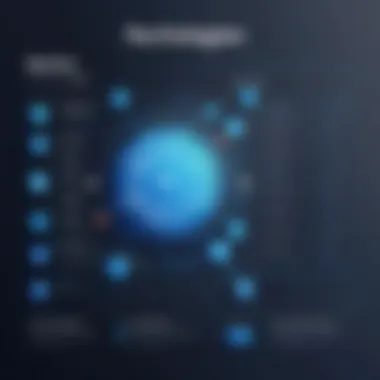
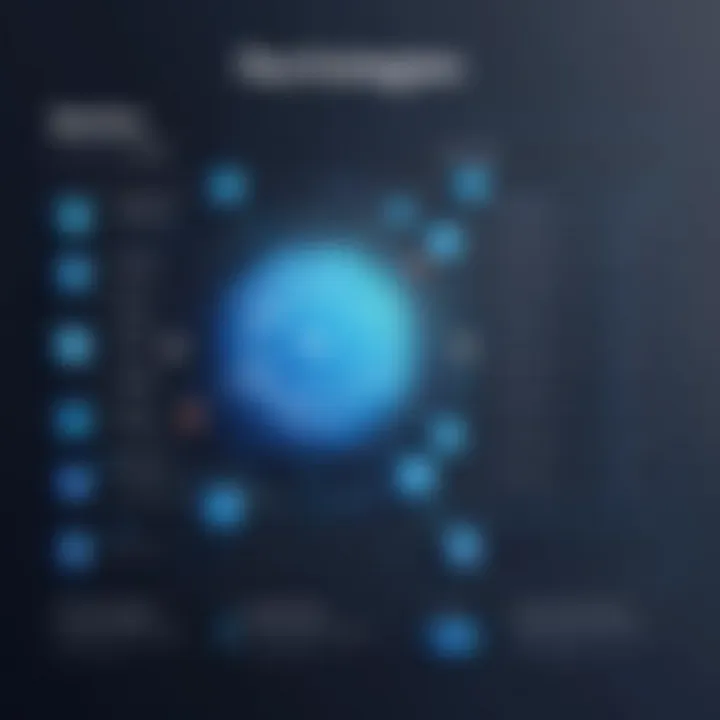
Intro
When exploring the world of technology, Java stands out as a vital programming language. It has solidified its place in the realm of software development, significantly impacting the way we create applications. Understanding Java is not merely an exercise in syntax; it's about grasping concepts that form the backbone of modern software engineering. In this article, we embark on a thorough examination of essential Java coding questions that are likely to pop up in interviews, and we will unpack the critical areas that prospective candidates must comprehend deeply.
Questions commonly posed during these interviews often probe algorithms, data structures, and system design. Preparing for these queries isn't just about cramming information; it's about fostering a mindset geared toward problem-solving and adaptability. This guide serves as a stepping stone for candidates eager to ace their Java job interviews by elucidating key concepts and providing practical examples.
"Success is where preparation and opportunity meet." — Bobby Unser
As we navigate through the intricacies of Java, we will touch upon various essential segments:
- Introduction to Programming Language
- Basic Syntax and Concepts
- Advanced Topics
- Hands-On Examples
- Resources and Further Learning
- History and Background
- Features and Uses
- Popularity and Scope
- Variables and Data Types
- Operators and Expressions
- Control Structures
- Functions and Methods
- Object-Oriented Programming
- Exception Handling
- Simple Programs
- Intermediate Projects
- Code Snippets
- Recommended Books and Tutorials
- Online Courses and Platforms
- Community Forums and Groups
With this roadmap in mind, let's delve into the first section—an Introduction to the Programming Language, setting the stage for better understanding of Java and how it fits into the wider context of coding interviews.
Preface to Java in Interviews
Java has carved out a significant place in the programming landscape. For many companies focusing on software development and technology solutions, Java remains a staple. Therefore, the significance of Java coding questions in interviews cannot be overstated. This section seeks to spotlight why Java proficiency is essential and to offer an overview of how interviews are structured around it.
The Importance of Java Proficiency
In the competitive world of tech interviews, having a solid grasp of Java can set candidates apart from the crowd. Mastery of this programming language demonstrates not just coding skills but also an understanding of core programming principles. Given its versatility, Java plays a significant role in various domains such as web, mobile, and enterprise applications.
Being proficient in Java can also mean a familiarity with key concepts like:
- Object-oriented programming (OOP) principles
- Exception handling strategies
- Frameworks and libraries like Spring or Hibernate
- Memory management and garbage collection concepts
Each of these elements can be the crux of interview discussions. Hence, brushing up on principles and applications can give a candidate a distinct edge. Recruiters desire developers who can not only write good code but who understand the underlying architecture and design patterns involved.
Overview of Interview Structure
Entering a Java interview generally follows a structured path designed to gauge various competencies. Here's what candidates can typically expect:
- Initial Screening: This may involve a brief phone call or an online coding test focused on basic Java concepts. Candidates might face questions that center on syntax, basic programming paradigms, or Java APIs.
- Technical Interviews: Once through the initial screening, candidates usually proceed to technical interviews. Here, the focus shifts to problem-solving skills. Candidates may be asked to solve algorithms or data structure problems while writing code in real-time. The interviewer often asks follow-up questions to probe deeper into the candidate’s thought process and problem-solving strategies.
- System Design: For more advanced positions, particularly those involving backend systems or enterprise-level applications, candidates may face system design interviews. In these, they must consider the larger picture of application architecture, load balancing, database design, and scalability.
- Behavioral Interview: Lastly, behavioral interviews assess soft skills, teamwork compatibility, and cultural fit within the organization. Here, candidates are often asked to recount past experiences that show how they handled challenges or worked collaboratively.
"Technical skills are important, but cultural fit often makes the difference between a good hire and a great one."
In summary, the journey through a Java interview process is comprehensive, and understanding both the technical and interpersonal aspects can create a more favorable outcome for candidates.
Categories of Java Coding Questions
Understanding the categories of Java coding questions is crucial for anyone preparing for interviews in the tech industry. These categories not only guide the preparation process but also help candidates align their learning with the expectations of potential employers. Each category covers different aspects of Java programming that may be tested, ensuring a well-rounded understanding of the language. Moreover, familiarizing oneself with these categories can significantly enhance problem-solving skills. This section breaks down the key categories you should focus on while preparing for your Java interviews.
Fundamental Concepts
Fundamental concepts form the bedrock of Java programming. This category usually encompasses basic syntax, data types, control structures, and core object-oriented principles. Becoming fluent in these concepts is vital for every Java developer, as they are not just theoretical but applied in day-to-day programming.
Key Points:
- Understand primitive types, such as int, char, and boolean.
- Be comfortable with operators and expressions, as they appear frequently in coding challenges.
- Familiarize yourself with concepts like inheritance, encapsulation, and polymorphism, which are essential in object-oriented programming.
Grasping these principles can spell the difference between a greenhorn and a seasoned coder in interviews.
Algorithms
Algorithms are at the heart of programming. This category tackles how to approach problems methodically. Whether it's sorting arrays or calculating factorials, understanding various algorithms will allow you to derive more efficient solutions.
- Sorting Algorithms: Be prepared to implement or explain algorithms like Quick Sort, Merge Sort, or even Bubble Sort.
- Searching Algorithms: Knowing how to traverse through data efficiently is key; familiarize yourself with Binary Search and Linear Search.
- Dynamic Programming Problems: These are often riddles that value problem decomposition. Recognizing how to use recursion over iterations is fundamental.
A robust grasp of algorithms indicates to interviewers that you are equipped to handle complex coding challenges.
Data Structures
Data structures store and organize data, playing a vital role in how efficiently your programs run. Understanding various data structures can be critical during interviews, as interviewers often assess your knowledge in terms of performance and memory management.
- Linked Lists: Know how to manipulate singly and doubly linked lists.
- Stacks and Queues: Be ready to explain or implement stacks for LIFO operations and queues for FIFO.
- Binary Trees and Graphs: Understand traversals like in-order, pre-order, and post-order for trees and various graph search techniques like DFS and BFS.
Mastering data structures not only streamlines your coding but also shows your ability to analyze problems effectively.
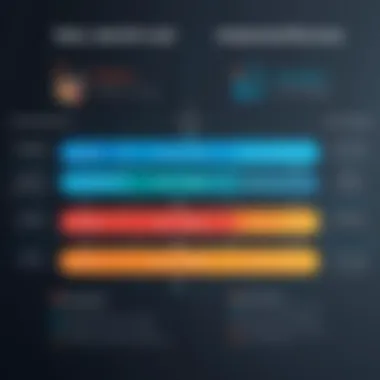
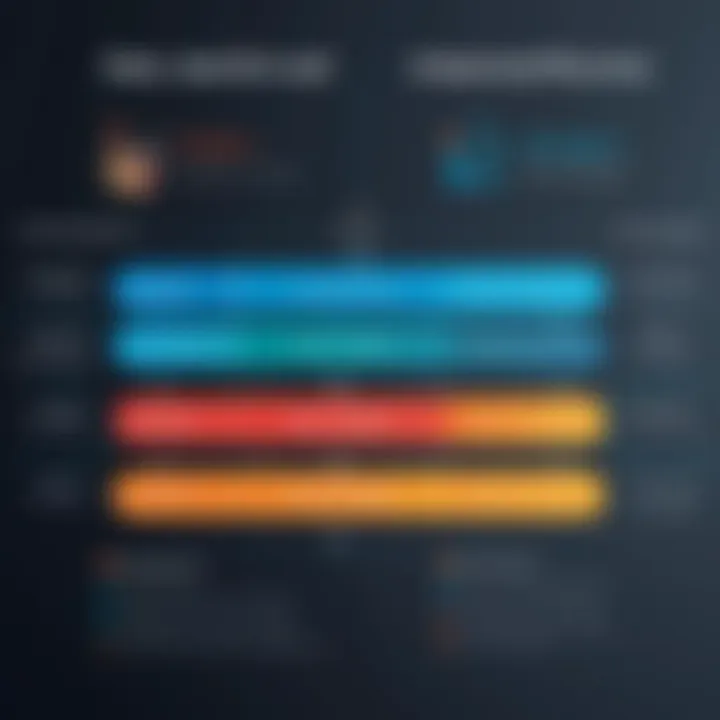
Object-Oriented Programming
Java is an object-oriented language, and its structure relies on OOP principles. Grasping these concepts is crucial for designing scalable applications.
- Classes and Objects: Be clear on the differences and how they interact.
- Inheritance and Interfaces: Know how to implement these features and when to use one over the other.
- Abstract Classes vs. Interfaces: Understanding their differences can help clarify many design decisions.
Demonstrating a robust understanding of OOP can help candidates stand out as it shows an ability to design complex, manageable systems.
Concurrency and Multithreading
This category often separates the wheat from the chaff in Java interviews. Understanding how to write programs that can handle multiple threads and process tasks concurrently is usually a must-have skill.
- Synchronization: Especially important when dealing with shared resources—it ensures that one thread does not interfere with another.
- Thread Lifecycle: Be familiar with the life cycle, states, and how to work with different thread-related classes from the Java API.
- Executors and Callable: Understand how these work in managing threads more efficiently than doing it manually.
Showing competence in concurrency can highlight your readiness for real-world applications where multi-threading is common.
System Design Questions
Although often viewed as advanced, system design questions are increasingly becoming a staple of Java interviews. This category tests your ability to architect a robust, scalable solution for real-world applications.
- High-Level Design: Be ready to sketch out the architecture of an application like a chat service.
- Low-Level Design: Focus on how you would design classes, interfaces, and interactions in smaller components.
- Handling Data Storage: Discuss options for databases, caching mechanisms, and choosing the right technology stack.
These kinds of questions assess not just technical knowledge but also strategic thinking, underscoring your readiness for complex challenges in software development.
Sample Coding Questions
The role of sample coding questions in the context of Java interviews cannot be overstated. They serve as a practical tool for both evaluators and candidates throughout the interview process. These questions give interviewers a glimpse into a candidate's problem-solving abilities and technical expertise. Additionally, they form a foundation upon which candidates can demonstrate their understanding of essential concepts in Java programming.
When prepping for interviews, candidates should become familiar with a variety of questions tailored to different categories. Not only do these questions prepare candidates for specific scenarios, but they also bolster their confidence when they step into the interview room. Here are some categories of questions that can structure a candidate’s preparation effectively.
Classic Algorithm Questions
Sorting Algorithms
Sorting algorithms are fundamental to computer science and a staple in coding interviews. They play a significant role in optimizing tasks that involve organizing data. Key algorithms like QuickSort, MergeSort, and Bubble Sort frequently come up in discussions, as they allow candidates to showcase their logical thinking and coding efficiency.
One prominent characteristic of these algorithms is their efficiency in handling different types of datasets, which speaks to their versatility. For instance, MergeSort is known for its stability and performance on larger datasets, while QuickSort shines with smaller arrays.
The unique feature of sorting algorithms lies in their various time complexities, which can influence performance dramatically depending on the input data. A candidate's ability to discuss these intricacies can make all the difference in an interview setting. However, they can be tedious to implement if not practiced, as some sorting techniques involve complex recursive steps.
Searching Algorithms
Searching algorithms, much like sorting algorithms, are paramount in programming due to their utility in retrieving data from collections. These questions typically cover traditional methods like Linear Search and Binary Search. The core advantage of Binary Search, particularly when dealing with sorted arrays, is its logarithmic time complexity, making it incredibly efficient and a favorite among interviewers.
What sets searching algorithms apart is their direct application in real-world scenarios, such as finding specific data within a massive database. However, candidates should be careful when discussing their implementations, as a misstep in understanding array indices can lead to off-the-mark solutions that can raise eyebrows.
Dynamic Programming Problems
Dynamic programming is an advanced technique for solving complex problems by breaking them down into simpler subproblems. This method often surfaces in interviews, especially for candidates vying for positions that require robust problem-solving skills. An important characteristic associated with dynamic programming is its ability to save on computation by storing the results of subproblems, thus avoiding duplicate calculations.
Candidates often highlight how this technique improves the efficiency of algorithms that might otherwise have exponential time complexity. However, candidates should remain vigilant, as dynamic programming can be intricate, making it easy to become tangled in overly complex solutions if not approached systematically.
Data Structure Implementation Questions
Linked Lists
Linked lists present a dynamic storage model, crucial in programming as they allow for efficient memory usage. An essential feature of linked lists is their ability to grow and shrink as needed, which enables developers to manipulate data efficiently without the need for frequent memory allocation and deallocation.
This flexibility becomes especially useful in situations where the size of data can fluctuate. Candidates can expect to be quizzed on the basic operations of linked lists, such as insertion and deletion. The main disadvantage, however, is that they can offer poor cache performance compared to arrays due to their non-contiguous memory allocation.
Stacks and Queues
Stacks and queues, fundamental data structures, provide distinct functionalities that candidates must grasp. A stack operates on a Last In, First Out (LIFO) basis, which lends itself well to use cases like recursion and undo mechanisms in applications. Similarly, queues operate on a First In, First Out (FIFO) principle, making them ideal for managing processes in systems like task scheduling.
What sets these concepts apart is their straightforward implementation and versatile applications. However, candidates should be prepared to explain scenarios where one would choose a stack over a queue and vice versa, as interviewers often seek depth in understanding.
Binary Trees and Graphs
Binary trees and graphs take data organization a notch higher, providing structured ways to manage hierarchical data relations and complex networks, respectively. A binary tree, with its parent-child relationship, is often utilized for searching and sorting operations. This structure is pivotal in various algorithms like traversals (in-order, pre-order), which interviewers may ask candidates to implement.
Graphs, on the other hand, display relationships through nodes and edges, representing interconnected data effectively. This characteristic opens up a wealth of real-world applications, from social networking to navigation systems. Still, candidates should be wary of complexity; grasping traversal methods such as Depth First Search and Breadth First Search is crucial when tackling interview questions involving graphs.
Real-World Scenario Questions
Designing a Traffic Management System
Designing a traffic management system captures candidates’ abilities to engage in system design and architectural planning. This type of question evaluates a candidate's thought process in tackling real-world issues, applying Java to develop a feasible and efficient solution. Key characteristics include scalability and reliability, elements that are essential when considering variable traffic conditions.
The unique feature of these scenarios often involves integrating multiple systems, such as sensors and databases, to produce a cohesive solution, demonstrating the candidate's understanding of both coding and system design principles. However, complexity may arise, and interviewers might be keen to assess how candidates break down the challenges.
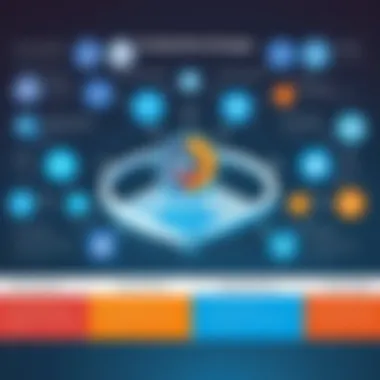
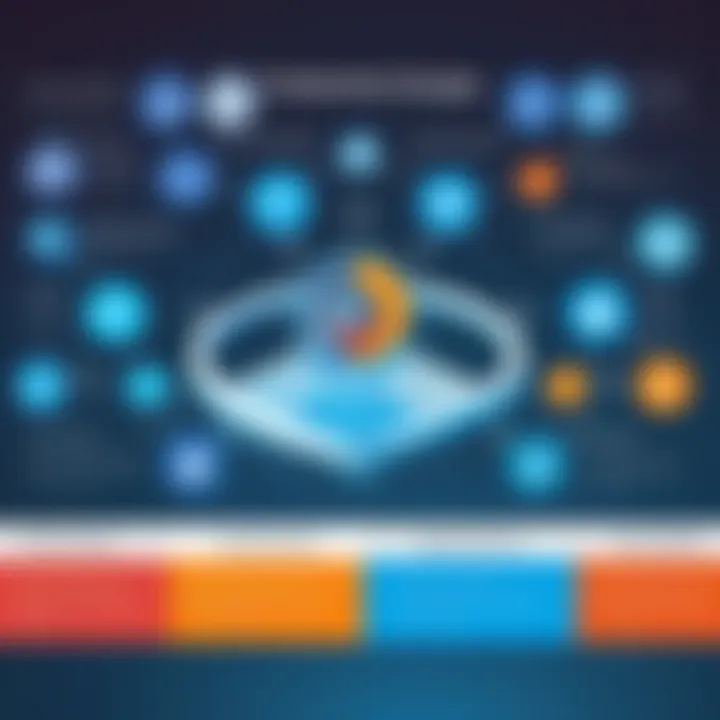
Building an Online Booking System
An online booking system is another common use case that tests candidates’ understanding of web-based applications. This scenario opens discussions on database management, user authentication, and concurrency, showcasing a candidate’s ability to design intuitively and with user-experience in mind.
Candidates are usually expected to highlight essential aspects, such as user flow and data integrity, while also addressing scalability as more users come onboard. Nevertheless, the interview may steer towards the challenges of implementing an efficient system with high availability, forcing candidates to think critically.
Creating a Chat Application
Developing a chat application explores candidates’ knowledge in real-time communication technologies. This can include discussions about WebSockets or REST APIs involved in messaging. Key features to emphasize include user authentication, data encryption, and high availability to maintain seamless communication across platforms.
The challenge lies in addressing latency and designing a system that can handle numerous users simultaneously. Candidates should prepare to discuss these limitations to display their problem-solving capabilities.
In Java interviews, the capacity to articulate one’s approach and understanding during discussions of sample coding questions is pivotal in demonstrating knowledge and technical aptitude.
Problem-Solving Strategies
In the high-stakes environment of Java programming interviews, having a robust strategy for tackling problems can set candidates apart. This section explores three essential aspects: understanding the problem, breaking down potential solutions, and optimizing the code. Each of these elements plays a pivotal role in not just answering interview questions but also in fostering a deeper comprehension of programming concepts.
Understanding the Problem
Recognizing the problem at hand is the initial step towards crafting a solution. When faced with a coding question, candidates should take a moment to fully grasp the requirements. This stage involves dissecting the problem statement to identify key components, which can include:
- Input and Output: What are the expected inputs? What should the output look like? Understanding these elements can eliminate confusion later on.
- Constraints: Are there specific limitations or requirements? For example, must the solution run within a certain time frame or with a particular space complexity?
- Edge Cases: Think beyond the common scenarios. What about empty inputs, large datasets, or unexpected characters?
By seeking clarity on these points, a candidate can ground their approach in a solid understanding, ultimately making their solution more effective.
Breaking Down the Solution
After grasping the problem, it’s time to develop a solution. This involves breaking down the problem into manageable steps. Candidates should think about the logical sequence needed to arrive at a solution. For instance:
- Plan: Sketch out the steps logically; this could mean laying out algorithms in pseudocode or flowcharting the logic.
- Choose Data Structures: Depending on the problem, various data structures might be appropriate. It could be a list for sequential processing, a hash map for quick lookups, or maybe a binary tree for hierarchical data.
- Iterative Refinement: It's rare to get it perfect on the first try. So, candidates should be ready to iterate on their solutions based on what works and what doesn't.
This breakdown facilitates a structured approach to problem-solving that makes the process less daunting.
Optimizing the Code
Once the initial solution is crafted, optimization becomes the next logical step. Interviewers often look for candidates who not only find solutions but also present efficient ones. Focusing on performance can save time especially during interviews. Here are several strategies to think about while optimizing:
- Time Complexity: Evaluate the algorithm's performance and see if it can be improved from, let’s say, O(n^2) to O(n log n) for sorting tasks.
- Space Complexity: Determine whether the memory usage can be minimized. For example, instead of using an additional array for storing intermediate results, is it possible to compute results on-the-fly?
- Code Clarity: While optimizing, maintain readability. Remember, a balance must be struck; overly convoluted solutions might win in speed but fail to communicate intent.
By emphasizing on these strategies, candidates can sharpen their coding skills and improve their chances of impressing interviewers.
"A great programmer is not a magician; they think in structure. Having a plan is half the journey."
In short, mastering problem-solving strategies in Java interviews isn’t just about memorizing answers. It’s about developing a mindset and skillset that allows individuals to tackle challenges confidently and efficiently.
Communicating Solutions
In today's technical interviews, showcasing your coding skills is only half the battle. The ability to communicate your thought process effectively is equally vital. Candidates often underestimate how articulating their solutions can influence an interviewer's perception of their expertise. Clear communication helps bridge the gap between coded logic and human understanding, allowing your interviewer to follow your reasoning and appreciate your problem-solving prowess.
When you articulate your approach, you not only demonstrate your technical knowledge but also your collaborative spirit. In many teams, developers must work together to navigate challenges, and being able to convey your ideas can facilitate smoother collaboration down the line. Your communication lays the groundwork for how potential colleagues perceive you as a collaborator.
Additionally, effective communication in interviews reduces potential misinterpretations. By explaining your logic thoroughly, you decrease the likelihood of the interviewer guessing your intent or misjudging your capabilities. After all, they are not just assessing your final output, but also evaluating how you arrived at your conclusions.
"Clear explanations build trust and understanding, both in interviews and in future collaborations."
Articulating Thought Processes
This section ties back to the heart of coding interviews. When presented with a coding problem, take a moment to internalize it. Start by summarizing it in your own words, laying the groundwork for effective explanation. For example, if you're asked to reverse a String in Java, you might say, "I need to take the input string and return it in reverse order."
Next, engage in a brief brainstorming session. Discuss different approaches you might use, such as using built-in functions or creating a loop structure to swap characters manually. Each time you consider an option, articulate why that method could be beneficial or what its drawbacks might be. This shows the interviewer you think critically about options.
Consider using examples or analogies to clarify your points. If you are tackling a data structure issue, you could relate it to organizing books on a shelf. This method helps solidify your explanation, making it more relatable. Remember, context is key.
Handling Questions from Interviewers
Interviews aren't just a one-way street. Be prepared for follow-up questions that might challenge your assertions or dive deeper into your reasoning. An interviewer might query your choice of an algorithm, or they may present a related problem. Don’t shy away from these inquiries; view them as opportunities to expand the conversation.
Stay calm and think before responding. If you’re uncertain, it’s perfectly acceptable to admit it. Propose potential directions you could explore to find an answer. For instance, if asked about optimizing a sorting algorithm, you might say, "I'm not sure off the top of my head, but I could look into using quicksort or mergesort based on the data set size."
Encouraging feedback also hints at your willingness to accept constructive criticism, another essential trait in a developer. Adaptability is valued highly in tech. Don’t forget to ask questions, too, if you need clarification on what the interviewer is specifically interested in regarding your solution.
Preparing for Java Interviews
When it comes to cracking a Java coding interview, preparation is half the battle. This article aims to underline the significance of laying solid groundwork before stepping into the interview call. Proper preparation can make the difference between a confident code-whisperer and someone lost in syntax.
Importance of Preparation
Understanding Java doesn’t end with knowing its syntax and key concepts. It’s about how you can apply that knowledge in real-world scenarios during interviews. Interviewers often look for problem-solvers who can demonstrate both coding prowess and critical thinking skills. That’s where preparation takes center stage.
Being familiar with common types of coding questions can ease nerves. It’s like having a roadmap that guides you through the maze of expected queries. Moreover, thoroughly practicing coding can pinpoint areas needing improvement, ensuring candidates walk in with confidence.
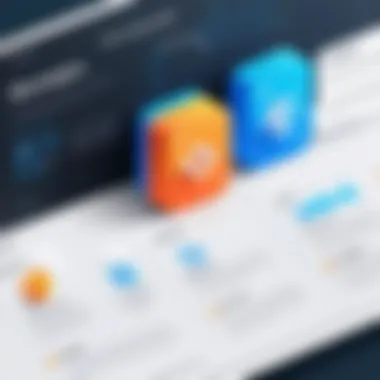
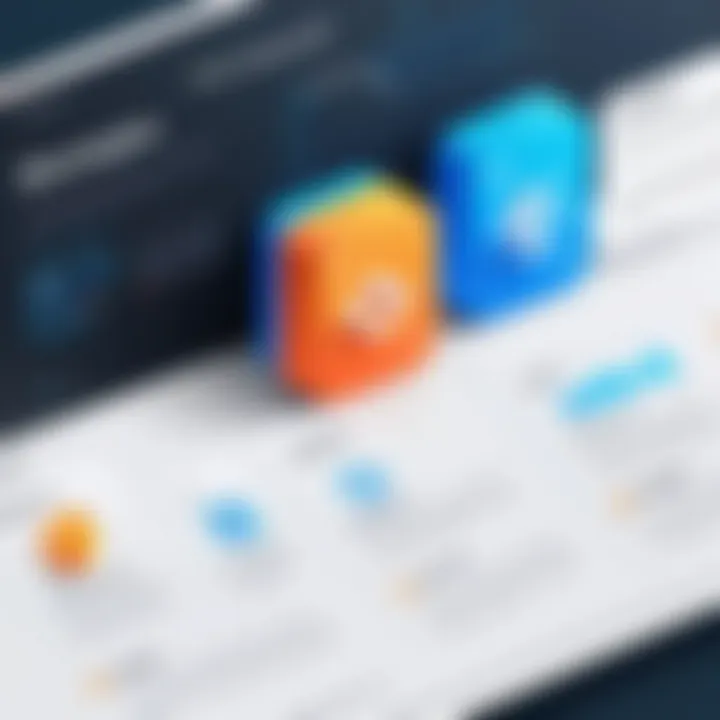
Benefits of Good Preparation
- Enhances Confidence: Knowing you’ve practiced and reviewed helps decrease anxiety.
- Better Time Management: Prior preparation allows you to allocate interview time wisely.
- Improved Problem-Solving Skills: You develop strategies for tackling Java problems effectively.
- Insight into Real-World Applications: Understanding how concepts apply in practice is vital.
- Articulation of Thought Process: A strong grasp of coding principles aids in explaining your approach during the interview.
Practical Coding Exercises
Doing practical exercises becomes an essential part of preparation. Not only do they familiarize you with the code itself, but they also simulate the interview environment. Engaging in daily coding drills keeps your skills sharp and allows you to understand the nuances of Java better.
- Daily Challenges: Utilize websites like LeetCode and HackerRank for practice problems tailored to your skill level. These challenges boil down core concepts into bite-size problems.
- Focus Areas: Concentrate on algorithms, data structures, and system design principles—key topics that pop up frequently in interviews.
Also, try your hand at pair programming with a colleague or use online platforms to engage with others undertaking similar paths. This could provide beneficial perspectives and reveal common pitfalls.
Mock Interviews and Feedback
Mock interviews play a pivotal role in preparing for the real deal. They simulate the pressure of an actual interview and get you accustomed to articulating your thought processes clearly.
- Structural Practice: Conduct mock interviews with friends from the industry or utilize platforms like Pramp or Interviewing.io. This act not only tests your preparedness but allows you to refine your oral communication as you explain your approach.
- Constructive Feedback: Seek detailed feedback from your mock interviews. Understanding where you stumbled can illuminate areas that still need polishing. A reviewer might find flaws that might slip your notice.
- Resilience in Failure: It’s part of the journey. Failures during mock interviews can serve as learning opportunities. Reflect on what choices could yield different outcomes, enhancing your skills and strategy moving forward.
"Preparation is the key to success" - Benjamin Franklin.
Remember, each practice session unfolds like a stepping stone along your career path, enabling you to strategize your approach better for future interviews.
Common Challenges in Java Coding Interviews
Java coding interviews present unique challenges that can hamper a candidate’s performance. Understanding these common hurdles helps in preparation, making it possible to navigate through them effectively. Acknowledging these issues not only sheds light on typical pitfalls but also equips candidates with strategies to counteract possible negative impacts during the interview process.
Time Management Issues
Managing time during coding interviews can feel overwhelming, often causing even the most proficient programmers to falter. During these high-pressure environments, candidates typically face numerous coding tasks within strict time constraints. Candidates may find themselves struggling to grasp the problem, leading to wasted minutes.
To tackle this challenge, practicing under simulated conditions is essential. The goal is to mimic the interview environment as closely as possible—limit the time for each question and focus on completing as much as possible within that timeframe. Here are a few techniques to manage time better:
- Prioritize Understanding: Before rushing into your solution, take a minute to break down the problem. A clear understanding reduces the time spent figuring things out mid-way.
- Set Time Limits: Give yourself strict times for each segment of your coding. For instance, allocate the first five minutes to plan, then about 10 minutes for coding.
- Use Pseudocode: Quickly jotting down your thought process in pseudocode can streamline your coding phase. It will help in visualizing the whole approach before diving into syntax.
"Preparation may not guarantee success, but it surely maximizes your chances of performing well."
This thought resonates well when it comes to managing time effectively.
Inadequate Understanding of Concepts
Many candidates underestimate the theoretical aspects of Java, finding themselves unprepared to discuss or implement fundamental concepts during their interviews. Simply memorizing code will not suffice; a deep-seated understanding of the hows and whys is crucial.
An inadequate grasp of core principles can create barriers, preventing candidates from answering questions satisfactorily. Employers often look for candidates who not only code proficiently but also understand the backbone of their coding decisions. Common areas of confusion include:
- Object-Oriented Programming: Understanding concepts like inheritance, encapsulation, and polymorphism.
- Concurrency: Grasping how to manage threads and processes in Java is essential, particularly in multi-threaded applications.
- Data Structures: Knowing which data structures to use for different scenarios directly impacts code efficiency.
To overcome this challenge, candidates should focus on:
- Reviewing Core Concepts: Regularly revisiting fundamental concepts through online tutorials, textbooks, or coding platforms can deepen understanding.
- Engaging in Peer Discussions: Joining study groups or forums on platforms like Reddit can also assist in gaining insights from others who have navigated similar hurdles.
- Hands-On Practice: Building simple projects can solidify understanding and provide practical applications for theoretical knowledge.
Equipping oneself with a solid foundation in core Java concepts will significantly alleviate anxiety and uncertainty in interviews, paving the way for clearer thinking and more refined coding solutions.
Building Java Projects for Experience
Building Java projects is not just a way to polish your coding skills; it also opens doors to real-world applications of what you’ve learned. When stepping into the world of programming, hands-on experience is key. The projects you develop serve as concrete evidence of your coding abilities. Employers look for candidates who can translate theory into practice, and working on projects showcases your capability to do just that.
Choosing the Right Project
Selecting a project that aligns with your interests and skill level can greatly influence your learning experience. Here are some considerations to keep in mind:
- Align with your goals: Consider whether you aim to strengthen your understanding of core concepts or delve into more complex areas. A project that resonates with your career aspirations can be motivating.
- Start simple: If you’re new to Java, pick a project that isn't overly complex. A simple calculator or a to-do list application can provide a foundation. Such projects reinforce fundamental programming principles and keep you from feeling overwhelmed.
- Challenge yourself: Once you've gained some confidence, consider moving on to something more challenging, like a web application or a game. Projects like these require more thought and planning but they can demonstrate your growth.
- Community feedback: Seek out open-source projects or those with collaborative elements. Engaging with other developers can enhance your learning and provide diverse perspectives.
Documenting Your Work
Proper documentation of your projects is as crucial as the code you write. It not only helps others understand your thought process but also serves as a valuable reference material for yourself in the future. Here are a few tips to communicate effectively:
- README files: At the very least, you should include a README file within your project repository. This should explain the project’s purpose, how to run it, and a brief description of your design choices.
- Comment your code: Within your code, use comments to clarify complex sections. This will aid both yourself and others who may read your code later. Avoid extensive comments on straightforward sections though; that could clutter your thoughts.
- Version control: Utilize Git for version control. It offers a way to track changes and document the evolution of your project. Your commit messages can narrate the journey of your work, showcasing your problem-solving methods.
- Share your work: Platforms like GitHub not only facilitate collaboration but also act as a portfolio for your projects. Potential employers can see not just the final product, but the process behind it as well.
Documenting your projects not only boosts your learning but also serves as a bridge between you and potential employers. It demonstrates that you can communicate your ideas clearly.
Building Java projects provides a playground for experimentation. Remember, it’s not only about the destination but also the journey you take to get there. Each project you build is a stepping stone toward mastering Java and excelling in interviews.
End
One of the key elements to grasp here is the notion of continuous improvement. Programming and technology evolve rapidly, and keeping pace with these changes is critical. Candidates should embrace the idea that preparation does not stop at the interview. Every coding question faced is an opportunity for growth and learning.
When considering the benefits, there are several that come to mind:
- Skill Enhancement: Review of concepts strengthens coding skills.
- Confidence Building: Understanding answers to various questions boosts self-assurance during interviews.
- Networking Opportunities: Engaging with communities on platforms like Reddit or Facebook can provide insights and additional resources.
Candidates should also be mindful of the considerations involved in this continuous learning journey. Balancing theory with practical application is essential. While it’s easy to memorize answers, a deep understanding of why a solution works is more beneficial in the long run.
Moreover, exam charm aside, candidates must reflect on their unique problem-solving approaches. Everyone tackles challenges differently, and personal experience adds value to technical discussions during interviews.
"The more total understanding you have of a subject, the easier it is to weave through challenges it presents."
In summary, the conclusion is not merely an end but a bridge to a larger journey of learning and adaptation in the field of Java programming. As candidates navigate their paths through code challenges, they should remember that each step taken in mastering Java today will pay dividends in tomorrow's opportunities.