Java Code Questions and Answers: Comprehensive Guide
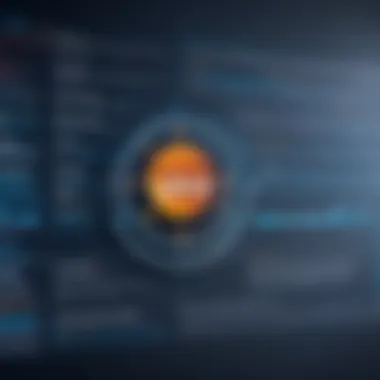
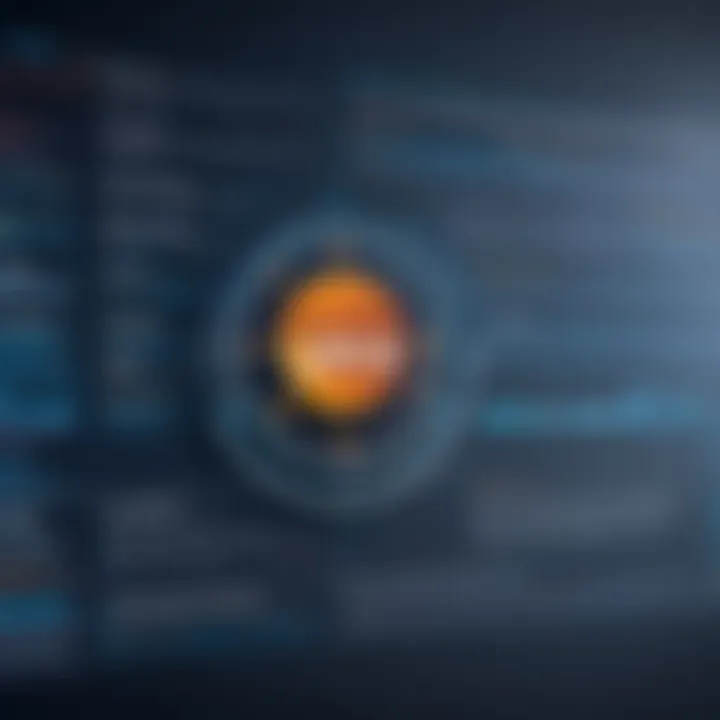
Prolusion to Programming Language
Java is a widely used programming language that emphasizes code readability and simplicity. It has a rich history that dates back to the mid-1990s. Java was developed by Sun Microsystems, and its first public release occurred in 1995. The motivation behind Java was to create a platform-independent language that could be used for cross-device applications. This foundational principle underlies Java’s design and has contributed to its sustained popularity.
History and Background
The journey of Java began as a project called Oak, initiated by James Gosling and his team. They aimed to create a language suitable for embedded systems. However, as the internet began to expand, the focus shifted toward web applications. Java's ability to run on multiple platforms led to its adoption for developing applets in web browsers.
Features and Uses
Java is known for several key features:
- Platform Independent: Java code can run on any device that has a Java Virtual Machine (JVM).
- Strongly Typed: This helps in catching errors during compile time, rather than at runtime.
- Object-Oriented: It encourages modular programming, making it beneficial for complex software projects.
- Multithreaded: Java can handle multiple threads of execution, which is vital for modern applications that require high performance.
Java is prominently used in various fields such as enterprise applications, mobile development with Android, and large web applications. Its versatility makes it a preferred choice for many developers.
Popularity and Scope
In recent years, Java has maintained a strong position in the programming world. According to the TIOBE index, it consistently ranks among the top programming languages. Its robustness, community support, and extensive libraries contribute to its wide usage.
Given its enduring presence, Java remains an essential skill for those entering the tech industry. The language's features support a wide range of applications, from small-scale projects to large enterprise solutions.
Basic Syntax and Concepts
Once you are familiar with the history and features, it is crucial to understand the basic syntax and concepts of Java. This foundation will aid learners in tackling coding challenges effectively.
Variables and Data Types
In Java, variables are used to store data. Each variable has a data type that defines what kind of data it can hold. Common data types include:
- int: for integers
- double: for floating-point numbers
- char: for individual characters
- String: for sequences of characters.
Operators and Expressions
Java offers a rich set of operators for manipulating data. These include arithmetic, relational, and logical operators. An expression is formed by using one or more operands and operators. For example:
Control Structures
Control structures are essential for directing the flow of the program. The main types are:
- If-else statements: determining conditions
- Switch statements: selecting between multiple paths
- Loops: such as for and while loops for repetitive tasks.
Advanced Topics
As one delves deeper into Java, it is important to explore more advanced topics that enhance programming skills.
Functions and Methods
Functions and methods are blocks of code designed to perform a specific task. They improve code reusability and organization.
Object-Oriented Programming
Java is inherently object-oriented. This paradigm promotes creating classes and objects, encapsulation, and inheritance. Understanding these concepts is vital for efficient software design.
Exception Handling
Managing errors is crucial in programming. Java's exception handling mechanism allows developers to gracefully handle runtime errors without crashing the application. It uses keywords like try, catch, and finally.
Hands-On Examples
Practical examples reinforce learning. Below are examples of different skill levels.
Simple Programs
A simple program might print "Hello, World!" to the console. This is often the first program written by learners.
Intermediate Projects
Intermediate learners may create a basic calculator that takes user input and performs arithmetic operations. This involves using control structures and functions.
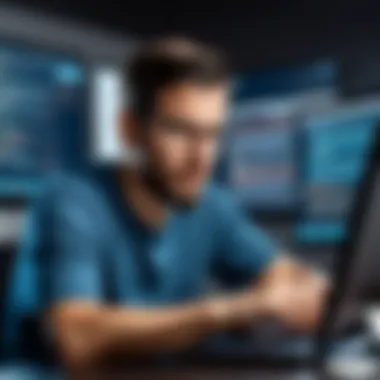
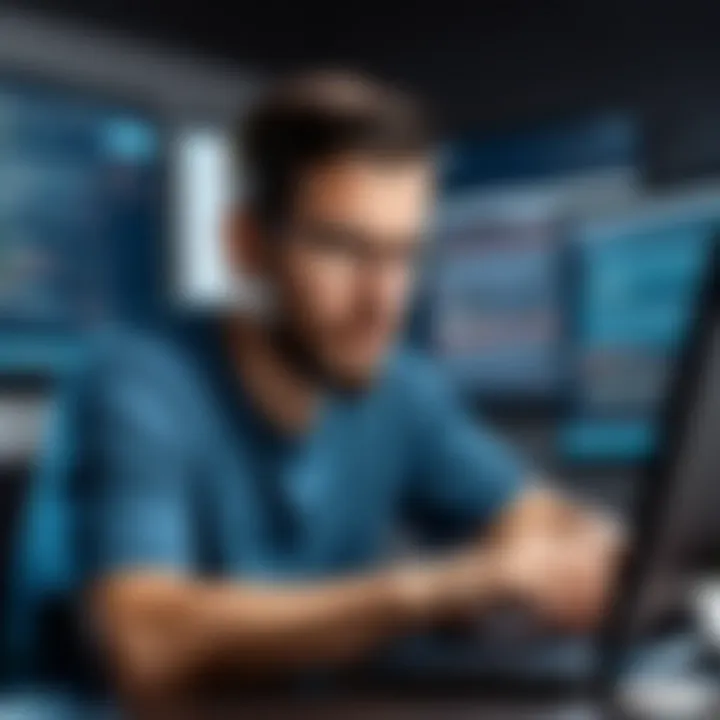
Code Snippets
Code snippets provide quick examples for common tasks. For instance, a snippet to find the largest number in an array:
Resources and Further Learning
Developing proficiency in Java involves utilizing various resources.
Recommended Books and Tutorials
Books like "Effective Java" by Joshua Bloch provide profound insights. Online tutorials also offer structured learning paths.
Online Courses and Platforms
Platforms like Coursera and Udemy provide courses tailored for different skill levels. These can supplement traditional learning methods.
Community Forums and Groups
Joining forums like Reddit or community groups on Facebook can enhance learning. Engaging with peers allows for sharing knowledge and solving problems collaboratively.
Is it clear that understanding Java is pivotal for any aspiring developer? Mastering this programming language opens doors to numerous career opportunities.
Foreword to Java Programming
Java plays a crucial role in the landscape of programming languages. Its versatility and robust features have made it a preferred choice for developers working across a variety of industries. In this section, we explore the beginnings of Java, its evolution, and understand why it is essential for both novice and experienced programmers.
The significance of Java programming cannot be understated. Java's design principles focus on portability, performance, and ease of use. These traits mean developers can write code once and run it anywhere, making Java an appealing option for various applications, from web servers to mobile applications.
History of Java
Java was created in the early 1990s by a group of Sun Microsystems engineers led by James Gosling. It was initially designed for interactive television, but the limitations of technology at that time halted its commercial application. Later, Java evolved into a general-purpose language, officially released in 1995.
From its inception, Java was built on principles that would resonate throughout its history:
- Write Once, Run Anywhere (WORA): Java code can run on any device that supports the Java Virtual Machine (JVM). This feature has transformed how applications are developed and distributed.
- Object-Oriented Design: This methodology allows developers to create modular programs, making them easier to maintain and understand.
- Strong Security Features: Java provided built-in security, which is crucial in networked environments.
As such, Java became a cornerstone for many large-scale applications and remains relevant today, aptly fitting into the modern developer's toolkit.
Key Features of Java
Several key features contribute to Java's ongoing popularity and effectiveness as a programming language:
- Platform Independence: Thanks to the JVM, Java offers unparalleled portability, allowing programs to be executed on various platforms without modification.
- Rich Standard Library: Java includes an extensive standard library, which provides a wide range of classes and functions that streamline programming tasks.
- Automatic Memory Management: Java includes a garbage collection system to manage memory allocation and deallocation automatically. This feature reduces memory leaks and optimizes resource use.
- Multithreading Capability: Java can handle multiple threads simultaneously, making it well-suited for applications that require concurrent processing.
- Robustness and Exception Handling: Java was designed with error-checking mechanisms that help in identifying problems at an early stage, making applications more reliable.
These features not only enhance the overall development experience but also ensure that the programs created are efficient and maintainable, resonating well with both new learners and seasoned developers.
"The versatility and adaptability of Java make it a critical language for modern programming practices."
Overall, this section underscores the foundational aspects of Java programming that inform the subsequent discussions on coding challenges and best practices. Understanding the history and features of Java sets the stage for learners to appreciate the depth and breadth of this language in the next sections.
Understanding Java Code Structure
Understanding the structure of Java code is crucial for anyone embarking on a journey in programming. This section lays the foundation for all subsequent learning by providing clarity on the basic components and organization of Java code. Grasping how Java is structured allows learners to write effective code, troubleshoot errors, and understand the logic behind complex programs.
When we discuss the structure of Java code, we refer to both its syntactical elements and its object-oriented paradigm. This knowledge is essential not only for writing functional code but also for maintaining and enhancing existing programs. As students progress through their learning, they will find that a solid understanding of Java's structure significantly eases the process of debugging and extending applications.
Basic Syntax of Java
Basic syntax refers to the rules that define how a valid Java statement is written and organized. Familiarity with Java's syntax is imperative for beginners as it is the first step in writing any code.
- Variables and Data Types: Java is statically typed, meaning every variable must be declared with a specific data type. This helps in managing memory efficiently and avoiding type-related errors.
- Control Statements: Java has various control statements, such as , , , and , which guide the flow of execution. Understanding these statements is fundamental for controlling program efficiency.
- Methods: Methods are blocks of code that perform specific tasks. They help in organizing code into manageable sections and promote reusability. Each method must have a return type and can accept parameters.
This simple program demonstrates the basic syntax of Java, showcasing class structure and the main method.
Classes and Objects
Java is designed as an object-oriented programming language, which revolves around the concepts of classes and objects. Understanding these concepts helps learners grasp the encapsulation, inheritance, and polymorphism principles that Java employs.
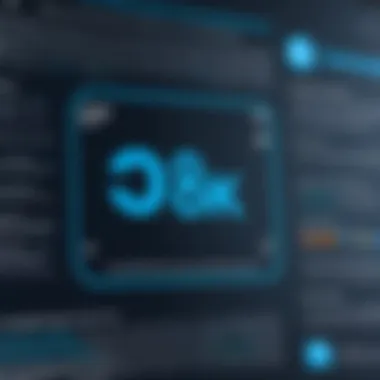
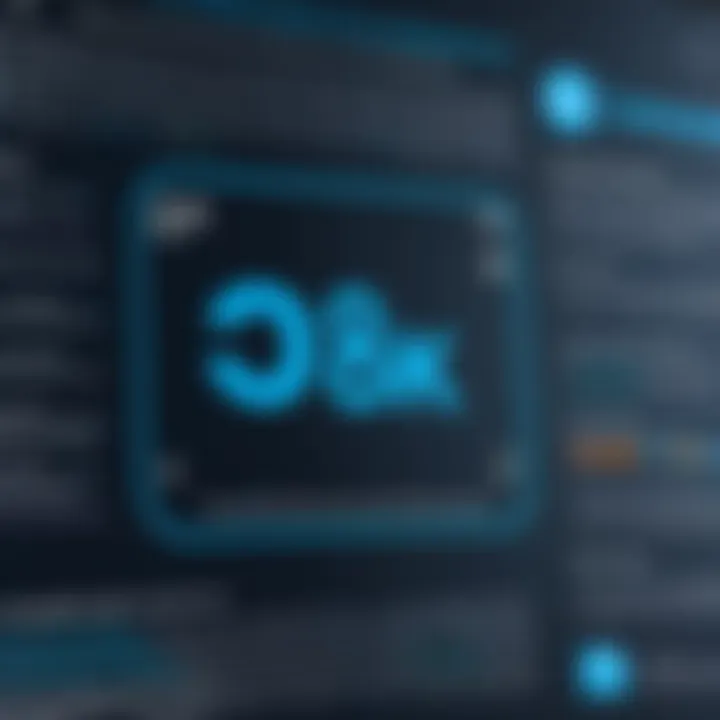
- Classes: A class is a blueprint for creating objects. It can contain fields (attributes) and methods (functions). Defining a class allows for creating multiple instances of objects that share the same structure and behavior.
- Objects: An object is an instance of a class. Each object has its own state and can perform operations defined by the class methods. This allows developers to model real-world entities and interactions in a structured way.
Through practical use, students will learn how classes and objects interact to form more complex applications. This understanding is critical for effective programming and software design.
Common Java Code Questions
Java remains one of the most widely used programming languages. Understanding common Java code questions is vital for those who are learning or wish to solidify their programming skills. Addressing these questions provides insight into practical programming logic and concepts. It encourages developers to think critically, leading to improved coding abilities and problem solving.
Fundamentals of Programming Questions
Fundamental programming questions cover the basic concepts that every beginner must grasp. Understanding data types, control statements, and basic syntax forms the foundation of Java programming.
- Data Types: Java has various data types. Learn the differences between primitive types like and , and reference types like and arrays. Knowing data types is essential for memory allocation and performance.
- Control Statements: Control statements dictate the flow of execution in a program. This includes , , , and looping constructs like and . Understanding how to properly use control statements is crucial for writing functional and efficient code.
For instance, consider a simple program that checks if a number is even or odd. The question can be framed as:
This example highlights the importance of conditional logic in decision-making within code.
Object-Oriented Programming Questions
Object-oriented programming (OOP) is a significant paradigm in Java. Questions in this area often focus on class design, inheritance, and polymorphism. Understanding these concepts will help learners grasp Java's object-oriented nature.
- Classes and Objects: At its core, OOP revolves around classes and objects. A class acts as a blueprint to create objects. Being able to define and utilize classes effectively creates robust applications.
- Inheritance: Inheritance allows one class to inherit fields and methods from another. This leads to code reuse and a clearer architecture. For example, a class named can be extended by and , allowing them to showcase specific behaviors.
Advanced Java Questions
Advanced Java questions delve deeper into the language's more complex concepts such as threading, networking, and Java APIs. These topics are essential for developers looking to create sophisticated applications that require performance and concurrency.
- Multithreading: Java's multithreading capability allows multiple threads to run simultaneously. This is crucial for applications that require concurrent processing.
- Java Collections Framework: This core feature helps manage groups of objects. Understanding the different collections like , , and is essential for efficient data handling and programming.
"Mastering multiple aspects of Java leads to producing efficient and high-quality applications."
In summary, common Java code questions offer essential insights into the language. Covering fundamentals sets the stage for more complex topics in programming. An understanding of both basic and advanced concepts provides the tools needed to build effective Java applications.
Java Code Answering Techniques
Java programming encompasses not only writing solutions but also effectively answering questions related to those codes. Understanding is crucial for anyone aiming to master the language, whether you are a novice or an intermediate learner. This section aims to break down essential elements, strategies, and practical approaches that improve one’s ability to articulate Java-related solutions.
Effective Problem Solving Strategies
Problem solving in programming requires a structured approach. Here are some strategies that can be useful:
- Understand the Problem: Before writing code, ensure you comprehend the question fully. Break down what is being asked. Take time to analyze the requirements and constraints.
- Plan Your Approach: After understanding the problem, outline a solution. You can sketch algorithms on paper or use pseudocode to visualize your approach. This makes coding easier.
- Break the Problem Into Smaller Parts: Divide complex problems into smaller, manageable sections. Tackle each part independently and combine them into the final solution.
- Test Incrementally: As you code, continuously test small parts of your code. This helps catch errors early, making debugging easier later on.
Effective problem solving is foundational. It saves time and reduces frustration, especially in a language like Java with its vast libraries and frameworks.
"The essence of good programming lies in effective problem solving."
Debugging Techniques
Debugging is an unavoidable part of programming. Having effective debugging skills enhances your ability to find and fix issues in Java code. Below are some common debugging techniques:
- Print Statements: Insert print statements in your code to display variable values and execution flow. This helps to trace where errors occur.
- Use a Debugger: Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse have built-in debuggers. These tools allow you to step through your code line-by-line, inspect variables, and understand the program's execution state.
- Check Stack Traces: When an error occurs, Java generates a stack trace. Reading the stack trace can give you insights about where the error originated.
- Peer Review: Sometimes a fresh perspective can identify problems quickly. Have someone else review your code. They might spot mistakes that you have overlooked.
- Unit Tests: Implementing unit tests for Java code will help you check the functionality of individual components. If a failing test reveals a mistake, it is usually easier to locate the source of the problem.
Debugging requires patience and an analytical mind. Utilizing various debugging tools and techniques sharpens your Java skills and leads to more polished code.
Practical Java Code Examples
Practical Java code examples serve as the backbone of understanding how Java programming functions in real-world scenarios. Such examples are crucial for learners since they bridge the gap between theory and practice. They allow students to see tangible applications of concepts they have studied, reinforcing learning through implementation. In this section, we will delve into two primary categories: Basic Java Programs and Intermediate Java Challenges.
Basic Java Programs
Basic Java programs are fundamental building blocks for those new to the language. These programs help establish a solid foundation of programming principles. Often, they cover essential tasks, such as creating simple outputs, performing arithmetic calculations, and managing user inputs. Below is a list of important elements typically involved in basic programs:
- Hello World Program: This is often the first program written by beginners, showcasing how to print output to the console.
- Simple Arithmetic: Programs that perform addition, subtraction, multiplication, and division illustrate how to use variables and operators.
- Data Input: Incorporating user input through the Scanner class allows learners to understand how to interact with users effectively.
The benefits of completing basic Java programs include enhancing confidence in coding skills and cultivating a deeper understanding of syntax. Students learn how to structure their code, apply control statements, and execute methods—all vital skills that form the basis for more complex programming tasks.
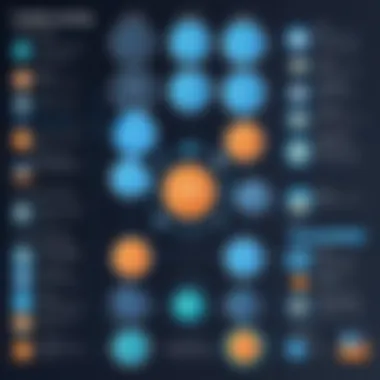
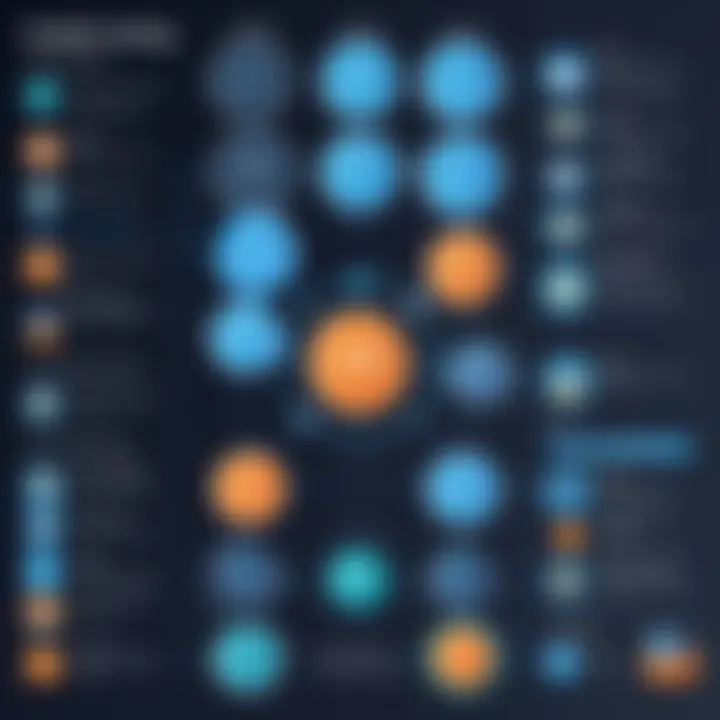
Intermediate Java Challenges
As students progress, they often face intermediate Java challenges that require more critical thinking and problem-solving skills. These challenges might include working with arrays, collections, and fundamental algorithms like sorting and searching. Engaging with intermediate content helps reinforce coding proficiency and introduces more complex data structures.
Common challenges found in this category include:
- Array Manipulation: Problems involving the creation and modification of arrays.
- Sorting Algorithms: Implementing sorting techniques such as bubble sort, quick sort, and merge sort helps students understand efficiency.
- Search Algorithms: Developing programs that utilize linear search and binary search approaches allows them to explore algorithm optimization.
Completing these intermediate tasks not only enhances a learner's ability to think algorithmically but also prepares them for real-world scenarios such as software development and data analysis. Through practical application of skills, students solidify their understanding and gain insight into Java's capabilities.
"Engagement with practical coding examples is essential for aspiring programmers. It builds the technical skills required and enhances problem-solving abilities."
By the end of this section, learners should recognize the significance of hands-on experience in reinforcing theoretical knowledge acquired throughout their study of Java programming.
Accessing Additional Resources
Accessing additional resources is a crucial step for anyone looking to deepen their understanding of Java programming. This section serves to illuminate how supplementary materials can facilitate a more robust learning experience. By utilizing external resources, students can access diverse perspectives, examples, and methodologies that enhance their coding skills. Additionally, these resources often provide updated information that can keep learners informed of the latest trends and best practices in Java development. Overall, by embracing these tools, learners ensure they are well-equipped to tackle coding challenges and effectively apply Java concepts.
Books and Online Resources
Books and online resources remain fundamental for learners at various stages in their programming journey. They offer not just theoretical insights, but practical applications that help in solidifying concepts. Some notable books include:
- Effective Java by Joshua Bloch: This renowned book provides essential programming best practices.
- Java: The Complete Reference by Herbert Schildt: A comprehensive guide covering all facets of the Java language.
- Head First Java by Kathy Sierra and Bert Bates: It presents fundamental concepts in an engaging and visually rich format.
In addition to these books, various online platforms can be indispensable. Websites like Oracle's Java Documentation offer official guides and tutorials. Interactive platform like Codecademy and LeetCode provide coding exercises that enhance practical application. Online communities, including Reddit, host discussions where learners can ask questions and share knowledge.
PDF Resources for Java Preparation
PDF resources can be particularly beneficial for those preparing for exams or interviews. These documents typically compile important topics and questions in a concise format, allowing for focused study. Some recommended PDF resources include:
- Java Programming Interview Questions: A comprehensive guide with frequently asked questions.
- Java SE 8 Programmer Study Guide: Offering insights tailored to the certification exam, this guide is essential for serious candidates.
To further aid learning, many educational institutions provide freely accessible PDFs on Java fundamentals and advanced concepts. Searching online for resources through educational sites can yield helpful guides that serve as both study aids and reference materials.
"Accessing a wide array of resources ensures that learners are not confined to a single perspective and fosters a broader understanding of Java programming."
Overall, engaging with books, online platforms, and PDF documents enriches the learning process for programming students. Each resource contributes to a well-rounded knowledge base, helping to ensure success in mastering Java.
Case Studies in Java Programming
Case studies in Java programming are essential as they highlight the practical use and impact of Java in various industries. They not only provide insight into how Java is applied in real-world situations but also allow learners to see the tangible benefits of mastering the language. In this section, we will explore the significance of case studies, how they inform the learning process, and the unique aspects they contribute to understanding Java.
Real-World Applications of Java
Java is used in countless applications across diverse sectors. Large-scale systems, mobile apps, and web applications frequently rely on Java due to its scalability and reliability. For instance, enterprises often choose Java for building backend applications because of its robustness and vast framework ecosystem.
Some key sectors that prominently use Java are:
- Finance: Banks and financial institutions utilize Java for secure transactions and complex backend processing.
- Healthcare: Java is used for managing patient records and hospital management systems.
- E-commerce: Online retailers rely on Java for handling high traffic, ensuring smooth transactions and customer interactions.
These applications of Java showcase its versatility and reliability. Additionally, understanding the context in which Java operates helps learners grasp the concepts better, as they can relate theory to practice.
Analysis of Java Projects
Analyzing successful Java projects offers valuable lessons to learners by showcasing effective coding techniques, architectural decisions, and the overall software development lifecycle. When examining notable Java projects, one should consider the following factors:
- Project Objective: What problems does the project aim to solve? Understanding the objectives can guide the learning process about relevant coding practices.
- Architecture: Analyzing the project's architecture helps comprehend how Java's features are utilized to overcome challenges or enhance performance.
- Code Quality: Evaluating the code written can reveal effective coding standards, patterns, and best practices in Java development.
For example, examining a well-known open-source project like Apache Hadoop can provide insights into effective data processing and storage solutions. It delivers practical knowledge about how Java handles big data across distributed systems.
"Case studies enhance not just understanding but also inspiration for budding developers, showing what can be accomplished with Java."
In summary, case studies in Java programming play a critical role in bridging the gap between theoretical knowledge and real-world application. They serve as a powerful tool for aspiring programmers, enriching their learning experience and preparing them for practical challenges ahead.
End
The conclusion of this article serves as a critical touchpoint for readers, emphasizing the essential learnings acquired through the exploration of Java code questions and their corresponding answers. It consolidates the insights gained, offering a reflection on the significance of understanding Java programming in both academic and practical contexts.
One cannot overlook the importance of synthesizing previous sections. As readers navigate through topics such as Java’s code structure, common programming questions, and effective coding strategies, they build a foundation that not only supports their understanding of Java but also enhances their problem-solving abilities. These are crucial skills for any aspiring developer.
Additionally, by engaging with practical examples and case studies, readers gain real-world insights into how Java operates in various applications. This not only aids in cementing theoretical knowledge but also equips individuals with the capacity to apply what they have learned in hands-on scenarios.
In the domain of programming, especially Java, continuous learning and practice are vital. The comprehensive nature of the materials discussed encourages readers to revisit the core concepts, thus fostering a cycle of learning and improvement.
"Education is the most powerful weapon which you can use to change the world." – Nelson Mandela
This statement resonates strongly within the context of learning Java, where understanding and applying coding practices can lead to significant professional opportunities.