Integrating Web Pages into Android Applications for Enhanced User Experience
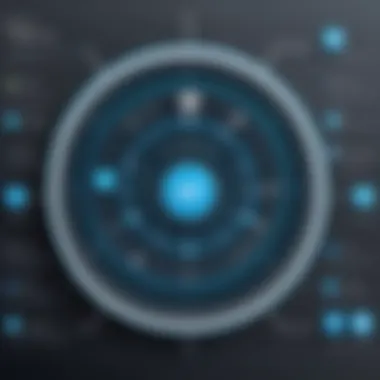
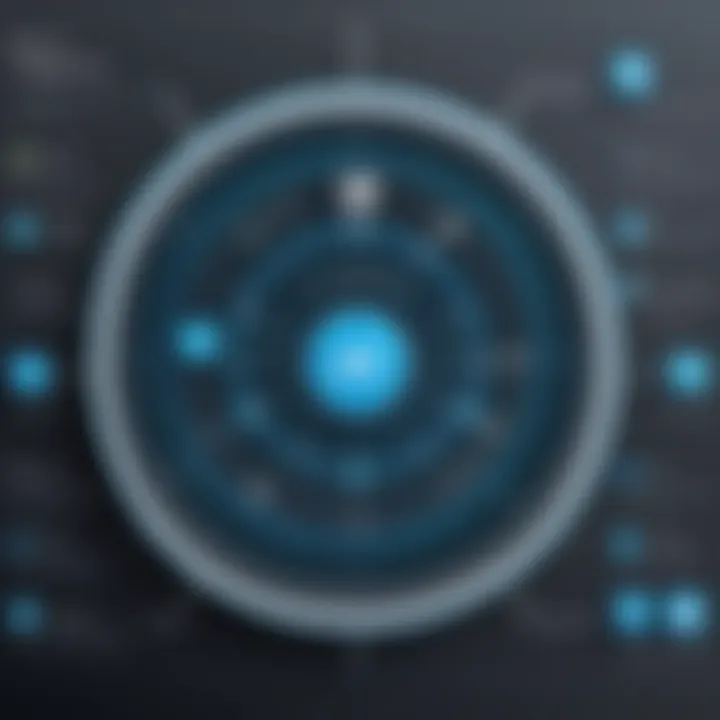
Intro
In this article, we will explore various methodologies tailored for smooth integration of web pages into Android environments. Relevant topics include technical discussions surrounding implementation strategies, specific frameworks, common challenges, and recommendations for best practices.
The significance of web integration in Android applications cannot be overstated. It allows for real-time content updates, interactive features, and overall flexibility. Whether one wishes to create a hybrid application or simply utilize web services within apps, understanding the framework is essential for success.
Before diving deeper into specific techniques, it's important to have a solid foundation. This includes comprehensive knowledge of Java and its syntax since basic Android development heavily relies on this programming language.
Throughout this document, each section will build upon the last to guide readers from conceptual underpinnings to hands-on implementation. So let us begin by breaking down the essential facets that lead to successful integration of web pages into Android applications.
Understanding the Context of Web Page Integration
Understanding the context is essential for grasping existing methodologies and why a cohesive integration is beneficial. This integration can lead to increased flexibility in app development. It also allows for real-time content updates without requiring frequent application updates through app stores.
There are multiple considerations to keep in mind when delving into web page integration. Among them, performance and user experience should be prioritized. If not handled properly, loading web content within an app may lead to increased load times or sluggish interfaces, ultimately frustrating users.
As the digital landscape evolves, a clear understanding of the unique challenges can help guide developers. Differences between web content and native app behavior demand attention to detail in design and implementation. This involves taking into consideration various user platforms and optimizing web pages accordingly.
Consider the figure of users today. Most are familiar with rapid page loads and smooth scrolling. As expectations grow, the blend between an app's functionnality and the website's presentability becomes pivotal in user retention.
"User engagement hinges significantly on how delicately integrated web contents cascade with native applications. The modern user expects much more than a basic functionality."
In summary, recognizing and implementing a solid web page integration framework not only enhances a developer's toolkit but also guarantees that end-user satisfaction is at the forefront of application development.
The Rise of Hybrid Applications
The popularity of hybrid applications stems from their unique capability to combine web-based and native capabilities within a single package. This merge optimizes the use of resources across platforms. The structure offers a compelling alternative to traditional application development methods, where either native or web applications were typically exclusive.
Developers appreciate the hybrid model because it allows for code reuse between platforms. For example, a single codebase can serve Android and iOS environments. Time and cost efficiency are huge factors that influence the growing preference towards hybrid solutions.
This progressive movement can be attributed to several factors:
- Rapid Development Cycles: Developers can produce and modify applications more quickly.
- Cross-Platform Consistency: It helps maintain uniform user experience across devices and platforms.
- Development Cost Reduction: Fewer resources are needed when maintaining only one codebase.
Thus, the demands for hybrid applications will only increase, making integration with web pages fundamental for businesses aiming to retain competitiveness in their market spaces.
User Expectations for Modern Apps
User expectations and behavior are evolving increasingly fast. Users today anticipate applications not only to function correctly but also to respond instantaneously and look appealing. This expediency extends to integrated web pages within applications.
When developers fail to meet such expectations, apps risk losing user engagement. It has become widely acknowledged that the modern user views elevated functionality as non-negotiable. The application’s ability to pull real-time data and provide an intuitive user experience becomes directive in its authenticity in the user’s daily chaos.
Important considerations include:
- Load Time: Users commonly accept that a delay of seconds feels significant, leading to unhappiness.
- User Interface: It is recommended that blended elements maintain familiarity. Mixing outmoded web design with fresh app UI often leads to confusion.
- Compatibility Across Devices: From smartphones to tablets, stability across all devices allows users to attempt diverse accessing points effectively.
In closing, the significance of meeting user expectations remains pivotal in integrating web pages within mobile applications. It not only ensures retention but builds positive reputation, ultimately contributing back to long-term growth and success of an application.
Key Technical Components
WebView Overview
WebView is a fundamental building block for displaying web content within an Android application. It acts as a mini-browser embedded in the app, allowing for the seamless integration of web pages while providing control over key aspects of how they operate.
The benefits of utilizing WebView include:
- Cross-Platform Functionality: Developers can leverage existing web technologies, making it easier to port webpages without extensive adaptation.
- User Interface Consistency: WebView helps maintain a cohesive look and feel across both native and web components.
- Ease of Updates: Changes to web content often do not necessitate an application update. Content shifts can occur instantly when the app is online.
Key considerations include performance. Developers need to optimize load times and consider connectivity issues that might affect user engagement. The implementation of proper settings for WebView is also paramount to ensure a smooth user experience.
A simple implementation of WebView in an Android application can be depicted as:
JavaScript Interface
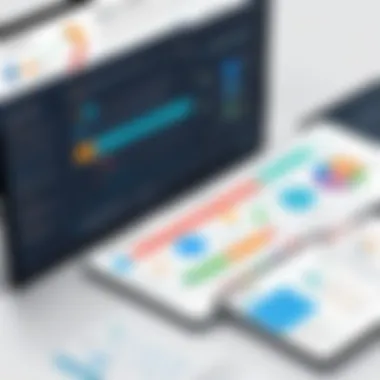
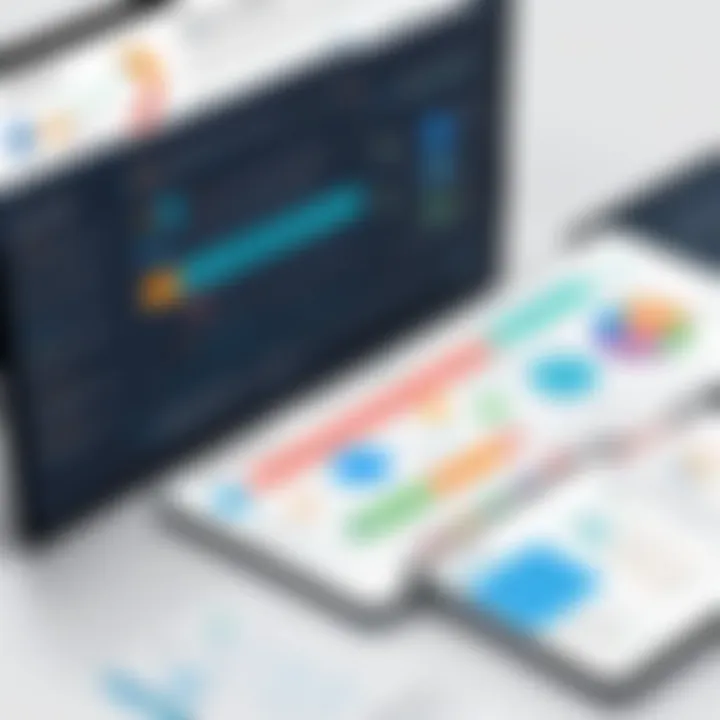
The JavaScript Interface creates a bridge between web content and native Android code. By enabling JavaScript within a WebView, developers can allow the web page to interact with the underlying app functionalities, significantly enhancing user experience.
Some of the primary benefits of using a JavaScript Interface include:
- Increased Engagement: JavaScript can trigger native actions, such as displaying notifications or changing app behavior based on web interactions.
- Feature Richness: It allows websites to utilize app features, where functions like camera access or data storage enhance overall functionality.
To implement a JavaScript Interface, developers must annotate the interactivity methods correctly. For example:
This opens up the possibility for the matched HTML/JavaScript commands within the WebView.
Through a solid understanding of both WebView and the JavaScript Interface, developers position themselves to synthesize web and native elements effectively.
Development Environment Setup
Given the broad scope of web page integration, adequate preparation in terms of tools and settings becomes essential. Here are the main elements that developers should take into account:
- Configuration of Android Studio: As the primary IDE for Android development, Android Studio provides the necessary features to manage coding, resources, and build processes efficiently.
- Necessary SDKs: Installing the correct Software Development Kits ensures compatibility with various devices and delivers optimally functioning applications.
- Emulator or Real Device: It's crucial to test on actual devices or emulators that represent user scenarios accurately.
Setting up a development environment might seem trivial, but overlooking missteps in this phase can lead to various issues later on in the project lifecycle.
Tools and Software Requirements
Establishing the right tools and software must come first in the development environment setup. Without the appropriate resources, development work can stall or contain bugs that can be hard to trace. A combination of both software and hardware is useful here:
- Android Studio – The official integrated development environment designed specifically for Android.
- Java Development Kit – Required for coding Java-based applications.
- Gradle – A robust build automation tool that manages dependencies.
- Android Emulator – Enables testing applications in a simulated environment, closely mimicking real-device performance.
- Version Control System – Tools like Git aid in code management, keeping track of changes.
These elements establish the foundational layer of the development environment, aiding in efficient workflows and easier problem resolution.
Creating an Android Project
Once the tools are in place, creating an Android project is the next logical step. This task sets the framework where coding takes place. Below is a clear guide for initiating a new android project:
- Launch Android Studio. From the welcome screen, choose Start a new Android Studio project.
- Choose Project Template. Selecting the right template helps frame the project. Templates can be 'Empty Activity', 'Basic Activity', or others depending on project requirements.
- Fill in Application Details. This includes the application name, package name, and save location on your system, making it identifiable and easy to locate later.
- Select Language. You can choose between Java or Kotlin, which are currently the supported languages for Android development.
- Choose Minimum API Level. Selecting the minimum API level enables compatibility across devices.
After confirming the settings, Android Studio will generate the necessary files and folders for your project, allowing further customization and development.
Developing mobile applications with web pages integration requires discipline and attention at every stage, starting from setting up the right environment to potentially refining the implementation.
Remember, the success of integration heavily relies on consistent updates and seeking assistance from communities such as reddit.com when needed.
Implementing Web Pages in Android Apps
Implementing web pages in Android applications is essential for modern app development. This process allows developers to blend the vast capabilities of the web with native app environments. By doing so, they can offers richer experiences and functionalities to users. Utilizing WebView and other related components opens the door for displaying dynamic web content inside an app smoothly.
The benefits of implementing web pages in app aren't limited to functionality. Users expect seamless navigation and a responsive interface. Convincingly, well-implemented web features can elevate an Android app's performance. Cross-pollination of content and experiences enhances user satisfaction. This section will guide developers on how to effectively integrate web pages.
Adding WebView to Layout
To successfully integrate web content, the first step is inserting a WebView into the app's layout. A WebView acts as a mini-browser within the application. This component renders dynamic HTML, CSS, and even JavaScript.
To include a WebView in your layout file, follow these simple steps.
- Open the XML layout file where you want to add the WebView.
- Insert the following code:
- Ensure you have the necessary Internet permissions in the :
Loading Web Content in WebView
After adding the WebView to your layout, you can now load web content into it. This commonly includes calling URLs in a few lines of code. A typical code snippet in Kotlin looks like this:
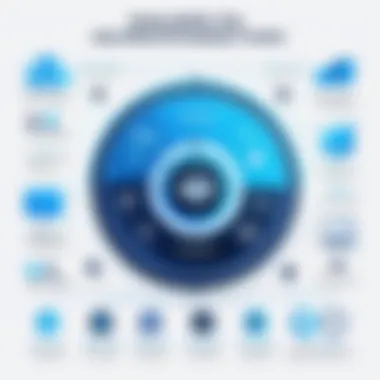
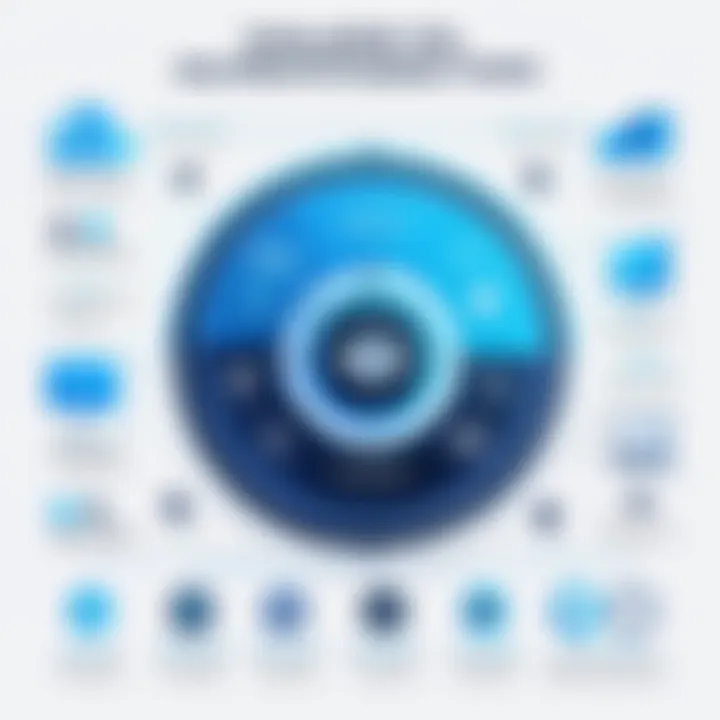
Handling various types of content is essential. WebView allows for loading local HTML or even text strings. Having the ability to navigate efficiently boosts app interactions. However, developers must keep an eye on loading times and any external dependencies as these factors affect performance. For best results, prioritize optimizing web content to suit mobile environments.
Managing Navigation Within the WebView
Encouraging a fluid user experience requires effective navigation, particularly inside the WebView. Users expect to move between different links dynamically. The Android WebView provides built-in methods to handle navigation behavior. Capable developers often establish a custom allowing for seamless management of URLs.
For instance, beneath is how you handle URL loading:
Additionally, back stack management is crucial. When users hit the back button, it's often desired that they return to the previous web page, not exit the app suddenly. Using allows you to create a back navigation mechanism.
Optimized management within the WebView enhances overall user satisfaction, leading to robust app retention levels. It creates an intuitive navigation experience, often leading users to explore more content.
Enhancing User Experience
First, loading speed is critical. Users expect seamless navigation and quick access to information. If a web page takes too long to load, users may abandon the app altogether. Therefore, optimizing loading times becomes essential. Using strategies like caching and asynchronous loading can significantly improve responsiveness.
Next, the visual presentation of web content is vital. Adapting the design to be mobile-friendly helps ensure that content displays correctly on different screen sizes. The layout should support easy scrolling and touch interactions, ensuring that users do not have to struggle with small buttons or navigational elements. This can be achieved through strategies such as using fluid grid layouts and simple navigation menus.
There is also the consideration of accessibility. Making web content accessible for users with disabilities expands reach and improves overall user experience. Guidelines for developing accessible web content, such as the Web Content Accessibility Guidelines (WCAG), can provide useful frameworks to follow. This shows a commitment to inclusivity, satisfying a broader user base.
Incorporating customization options can also enhance user experiences. Allowing users to alter the appearance, such as themes or font sizes, caters to personal preferences and makes the app more enjoyable to use. Tools that support user control can result in higher engagement.
Improved user experience directly correlates with better app retention and user loyalty.
Ultimately, the importance of enhancing user experience cannot be overstated. By focusing on elements like performance, visual design, accessibility, and customization, developers can create an engaging product that meets—and exceeds—user expectations.
Customizing WebView Settings
Customizing WebView settings is crucial for achieving optimal performance and responsiveness in Android applications. Developers have various options to adjust configurations for improved results. Key settings include enabling JavaScript, debugging options, cache mode, and mixed-content settings. Enabling JavaScript allows interactive content to function correctly, while appropriately adjusting the cache mode can help with faster browsing experiences. Here are a few crucial settings:
- JavaScript Enabled: Most modern web applications rely on JavaScript. This should be enabled within the WebView settings to improve functionality.
- Debugging: Android’s WebView has built-in debugging support, aiding developers to diagnose issues effectively, which enhances overall execution during tests.
- Cache Management: Setting cache modes correctly optimizes loading times. This can involve turning on caching for improved speed, depending on users’ internet connections.
- Mixed Content Mode: Handling secure and insecure content is important, especially in ensuring users remain secure while browsing.
By customizing these settings, developers can provide a more robust experience for app users.
Handling User Interaction
Handling user interactions in an Android app that includes web pages is critical to enriching the overall experience. Effective interaction handling means recognizing how users navigate, engage, and provide input. Ensuring that these elements work smoothly influences satisfaction.
To enhance user interactions, developers can implement several strategies. For instance, intercepting URL loads can help manage how users navigate through the app's web content. Developers may set certain URLs to open within the application's built-in browser, while others redirect to the device's external browser. This kind of thoughtful navigation control directly improves user flow.
Moreover, customizing touch events provides additional levels of convenience. For example, detecting swipe gestures can enable users to revisit previous pages with ease. Also, by appropriately managing long-press actions, developers can open context menus, providing instant access to functions like bookmarking or sharing.
Feedback calls upon user interactions are also vital. Providing clear indications, whether through haptic feedback or visual cues, enhances the user's confidence in their actions. Communication through helpful prompts, when they occur, can clarify further context.
By effectively managing user interactions, developers can craft a more responsive and enjoyable experience. This not only leads to more significant user engagement but fosters overall retention, critical for any successful Android application.
Addressing Common Challenges
Integrating web content into Android applications brings specific challenges that developers must consider. This section emphasizes why it is crucial to address these issues, highlighting the potential pitfalls that could affect both app performance and security.
Performance Considerations
Performance is a foundational aspect of any app, impacting user retention and satisfaction significantly. When integrating web pages, load times can become a pivotal concern. If web content takes too long to display, users may abandon the app in favor of alternatives. Moreover, a sluggish app can lead to negative reviews, affecting overall ratings on app stores. Therefore, developers must ensure that their web content is optimized for speed and efficiency.
Some strategies to enhance performance include:
- Minimizing Requests: Ensure that the minimum number of requests are made to load web content. This reduces lag time.
- Efficient Caching: Using caching mechanisms can store frequently accessed data, minimizing load times and improving user experience.
- Image Optimization: Ensure that images are compressed and responsive, meaning they can adjust according to different screen sizes. This action not only enhances loading speed but also improves the app’s aesthetics.
Developers should regularly test performance metrics using tools like Google Lighthouse or WebPageTest, which can provide insights into load times, responsiveness, and diagnostics for improving web content rendering within apps.
Security Implications of Web Content
The inclusion of web content introduces various security risks. When users consume web data, apps become potentially vulnerable to attacks such as Cross-Site Scripting (XSS) or data leakage. Understanding these risks is imperative to provide a smooth and secure experience.
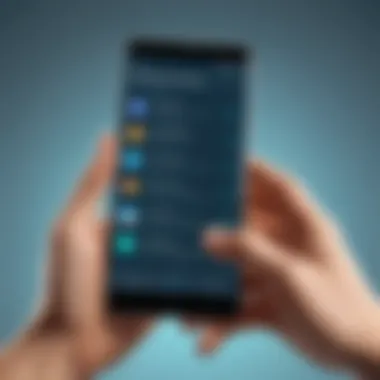
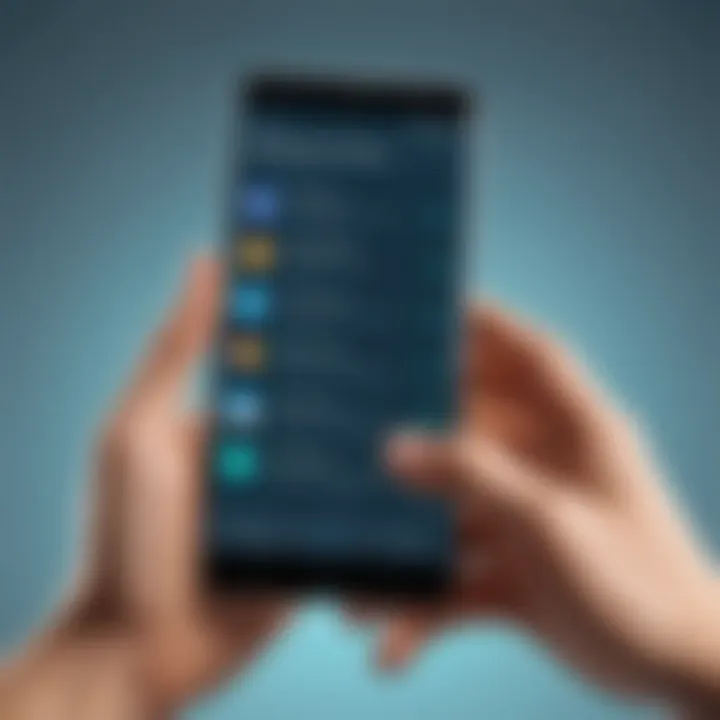
Security measures that developers should adopt include:
- Implementing Content Security Policy: Ensuring that policies are in place to control what data is allowed to load can help mitigate risks.
- User Input Handling: Any user input directed to the web pages should be sanitized to prevent malicious code execution.
- SSL Certificates: Secure your web connections through HTTPS, establishing encrypted communication channels, which are crucial for safeguarding user data.
Proper security assessments are vital. Developers should consider employing tools like OWASP ZAP to analyze vulnerabilities. Constant vigilance and periodic auditing can ensure that web integrations do not become backdoors for malicious activities.
By addressing performance and security implications, developers can enhance the integrity and reliability of their apps while delivering an unimpeded experience for users.
Testing and Debugging
Unit Testing WebView Integrations
Unit testing in the context of WebView integrations focuses on testing individual components of your application in isolation. This is vital for ensuring that each piece functions correctly before conducting more comprehensive tests. Here are some key aspects to consider:
- Frameworks Support: Unit testing in Android can be effectively conducted using frameworks like JUnit and Mockito. Both frameworks allow developers to mock dependencies for their tests, making the process smoother.
- Integration Test Cases: Develop unit tests that cover key functionality like loading URLs, managing redirects, and handling user interactions. This helps ensure that each component behaves as expected across different scenarios.
- Automation: Utilizing tools like Espresso can automate the testing process, allowing the developer to simulate user interactions within the WebView. This automation is advantageous as it saves time and enhances test coverage.
Implementing and conducting these unit tests systematically ensures that your WebView integrations adhere to quality standards, which is essential for user trust.
Debugging Techniques for WebView
Debugging is an ongoing process that cannot be neglected. Efficiently debugging WebView can correct issues arising from integration with web content or app responses. Here are some techniques to consider:
- Logcat: Utilize Android's Logcat tool for capturing logs during runtime. Examining logs provides insights into errors occurring within WebView, making it easier to diagnose problems.
- Chrome DevTools: Chrome DevTools offers developers a way to debug web content loaded in WebView. By enabling debugging over USB, developers can connect their device to Chrome and use the DevTools to inspect elements, view JavaScript console messages, and analyze network traffic.
- JavaScript debugging: For issues within web content, employ statements in your JavaScript code, which lets you pause execution at certain points. This is particularly useful for browser-type debugging in the WebView.
- Error Handling Mechanisms: Implement error handling within the WebView by listening to error events. This can alert the app that an issue needs addressing instead of failing silently.
By applying these debugging techniques, developers can swiftly identify and rectify issues within WebView, progressively building a more robust application.
Effective testing and debugging procedures ultimately lead to smoother, more effective Android application experiences.
Best Practices for Integration
Optimizing Web Content for Mobile
Mobile optimization serves as a crucial element when integrating web content into Android apps. Modern users expect fast and smooth experiences. Here are key points to consider:
- Performance: Ensure that web content loads quickly. Heavy images, unnecessary scripts, and excessive third-party resources can slow down loading times.
- Accessibility: Optimize web pages for touch interactions. Buttons and navigation links should be easy to tap, offering clear visibility on mobile devices.
- Content Format: Resize and adjust content layout tailored to smaller screens. Articles, for instance, should offer shorter paragraphs and sufficient spacing to improve readability.
By focusing on mobile optimization, developers can ensure that the web content is not only accessible but also enhances the overall app experience.
Leveraging Responsive Design
Responsive design is essential for effective integration of web pages. The principle behind responsive design is to make optimal use of various screen sizes and dimensions.
Important considerations for leveraging responsive design include:
- Flexibility: Page layout configurations must flex smoothly to fit various device screens without losing content integrity.
- Media Queries: Utilize CSS media queries to apply different styling rules based on the device's characteristics. This responsive technique allows for an exceptionally flexible design approach.
- Fluid Grids and Images: Avoid fixed widths. Use percentages or relative units to size and position elements on a web page. Fluid images must scale within their containing elements.
Utilizing responsive design effectively will not only enhance user experience, but also ensure that the web pages look consistent across all devices and orientations.
The importance of adhering to optimization and responsive design cannot be overstated; they prepare your app for the diverse mobile landscape, broadening its reach and functionality.
Future of Web and Mobile Integration
The integration of web pages into mobile applications is not just a trend; it is a fundamental shift in how users interact with digital content. This section discusses the evolving relationship between web and mobile platforms. As technology progresses, developers must adapt to ensure robust user experiences that effectively leverage the strengths of both paradigms. The upcoming trends and techniques play a crucial role in shaping future applications and their functionalities. Below, we explore emerging trends and the role of progressive web apps.
Emerging Trends in Hybrid Applications
Hybrid applications combine elements of both web and native experiences. They leverage the strengths of web technologies while delivering the performance benefits of native apps. Here are some current trends driving this development:
- Increased Use of JavaScript Frameworks: Frameworks like React Native and Flutter streamline hybrid application development. They allow developers to build cross-platform apps using familiar JavaScript features.
- Focus on User Interface and User Experience: Design practices are evolving to create more intuitive and fluid interfaces. Ease of use is paramount; designers are prioritizing seamless interactions within applications.
- Enhanced Performance Capabilities: Technologies such as cached APIs and native plugins contribute to improved loading times and responsiveness. Reductions in latency and increased speed enrich user experience.
- Integration of AR and VR: Augmented Reality and Virtual Reality applications are gaining traction. Developers utilize hybrid frameworks to expand these experiences, engaging users in ways previously thought not possible.
Adaptations in hybrid mobile apps are critical for transitioning Web standards to newer mobile technologies. This allows applications to grow more versatile, responding more efficiently to the evolving nature of user needs.
The Role of Progressive Web Apps
As the name suggests, Progressive Web Apps (PWAs) represent a step forward in web page integration within Android applications. They build upon traditional web applications, providing enhanced capabilities that mimic a native app experience. Among the components that define their impact are:
- Offline Accessibility: Using service workers, PWAs can be accessible even without internet connectivity. Users enjoy the same functionalities and experience regardless of their access to a network.
- Responsive Design: PWAs feature adaptive designs that provide optimal viewing experiences across a wide range of devices. From smartphones to tablets, this flexibility assures usability.
- Installation and App-Like Interfaces: Users can install PWAs directly from their browsers, offering quick access without navigating an app store. This installation process simplifies engagements while rotating consumption rapidly.
- Faster Page Loads: Load speeds greatly improve by caching content and pre-fetching resources. Users benefit from faster interactions, reducing abandonment rates and enhancing overall satisfaction.
Progressive Web Apps transform the approach to web development, marrying reliable web design with the fundamental features traditionally reserved for native mobile apps. As adoption increases, the implications on the mobile landscape are profound, making understanding this role essential for developers.
Continued integration of these methodologies is essential for staying relevant in a fast-evolving tech landscape. Keeping abreast with changes will aid developers in building user-centric applications capable of operational crossovers in both mobile and web domains.