An In-Depth Exploration of PostgreSQL Stored Procedures
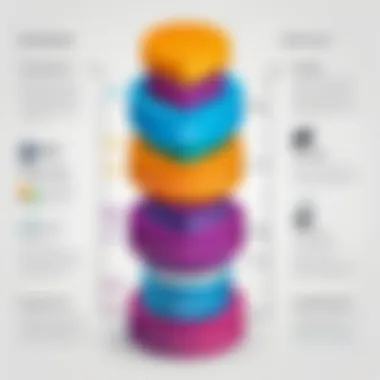
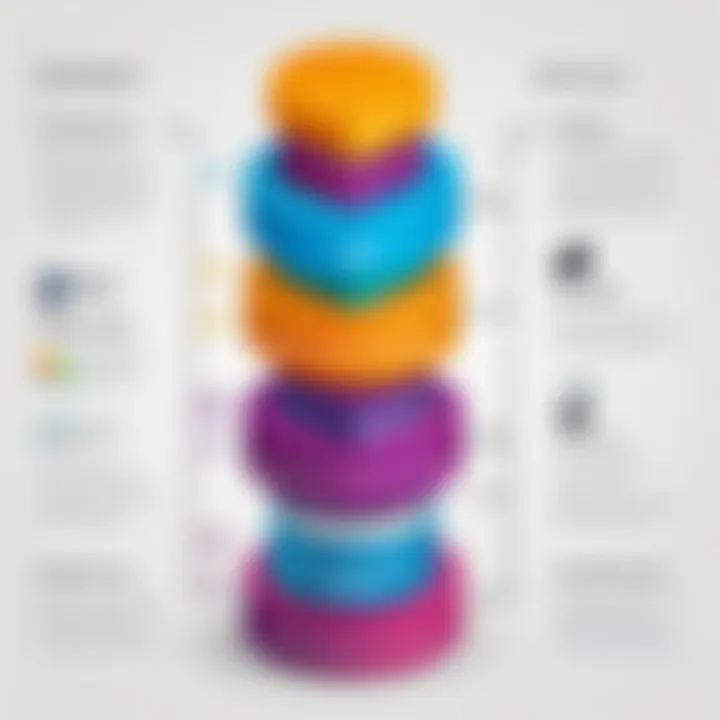
Intro
This article aims to delve into PostgreSQL stored procedures, central to enterprise-level database management. Defining what stored procedures are and understanding their syntax is critical for anyone wielding the power of databases. Stored procedures encapsulate SQL commands for reuse, improving efficiency and performance. They also allow complex operations to be executed within the database itself, minimizing data transfer across network boundaries.
Keywords like PostgreSQL, stored procedures, and database management will recur throughout the discussion. This comprehensive guide will equip readers with the knowledge necessary to maximize the utility of PostgreSQL stored procedures, from basic syntax to more intricate optimization techniques.
By illuminating the differences between stored procedures and functions, as well as the integration patterns with other database components, this article enriches the database management skillset of students and learners engaged in programming as a fundamental craft.
Prolusion to PostgreSQL Stored Procedures
Definition and Overview
PostgreSQL stored procedures are routines stored in the database that can execute a series of SQL commands. They can take parameters and return results. Understanding their role is crucial for efficient database management.
Key Benefits
Stored procedures offer several advantages:
- Efficiency: Reduce the amount of repetitive code by encapsulating logic.
- Security: Limit direct access to data. Procedures can restrict operations.
- Performance: Reduce network traffic by executing commands directly within the database.
- Maintenance: Afford easier updates to business logic without changing application code.
Basic Syntax and Concepts
Declaring a Stored Procedure
The basic syntax for declaring a PostgreSQL stored procedure includes defining its name, parameters, and body:
Parameters and Return Types
Stored procedures can accept input parameters. However, unlike functions, they do not directly return values. Instead, they can manipulate data within the database context.
Control Structures
Control structures, like and , extend procedural capabilities, permitting conditional logic and repetition within procedures, enhancing functionality.
Advanced Topics
Error Handling Techniques
Robust error handling is fundamental in stored procedures. Using the block, developers can define responses to different error conditions, ensuring procedures fail gracefully, maintaining database integrity.
Differences between Functions and Procedures
- Functions return values, whereas procedures do not.
- Stored procedures can manage transactions, allowing them to commit or rollback changes.
Hands-On Examples
A Simple Stored Procedure
Here’s an example of a simple stored procedure to insert a record:
Intermediate Use Case: Updating Records
In this example, a stored procedure updates user details:
Resources and Further Learning
Recommended Books and Tutorials
- "PostgreSQL: Up and Running"
- "Learning PostgreSQL"
Online Courses and Platforms
- Coursera
- Udemy
Community Forums and Groups
For further support and discussion, engaging with community forums on sites like Reddit can be beneficial: reddit.com.
Culmination
In summary, mastering PostgreSQL stored procedures significantly enhances database skills. Users become capable of creating efficient, secure, and maintainable database interactions. Understanding how to craft and utilize these procedures is paramount for effective database management.
Intro to PostgreSQL Stored Procedures
Stored procedures are a pivotal feature in PostgreSQL, enabling developers to encapsulate complex logic within the database itself. This strategic approach promotes efficiency, as it allows for the execution of pre-defined queries without transferring data over the network every time. Understanding stored procedures is essential for anyone looking to enhance their database management skills and implement robust data manipulation solutions.
Stored procedures serve multiple purposes. They can encapsulate complex SQL logic, enhancing maintainability and ensuring code reusability. Additionally, these procedures can simplify application development by offloading processing to the database. By utilizing stored procedures, database operations gain greater consistency and reliability, which is critical for large-scale applications.
Given that many modern applications rely heavily on data interaction, mastering the use of stored procedures can significantly elevate a developer’s capabilities. Moreover, the procedural nature of these features allows developers to integrate conditional logic, loop constructs, and various parameter types, making them highly versatile for multiple use cases.
Stored procedures are not just about encapsulating SQL commands; they're about optimizing processes and achieving seamless integration with application logic.
Definition and Purpose
A stored procedure is essentially a set of SQL statements stored in the database. It can be executed by applications or other procedures. The primary purpose of using stored procedures includes encapsulation of business logic, code reuse, and performance optimization. By defining business rules directly in the database layer, organizations can ensure that all applications follow the same logic when performing data operations.
Additionally, stored procedures support input and output parameters, allowing them to handle varying requirements dynamically. This feature alone enhances their utility, making them a powerful tool for developers when dealing with different data scenarios.
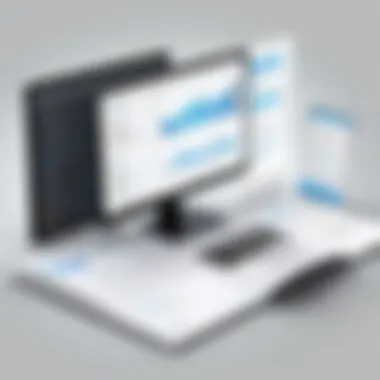
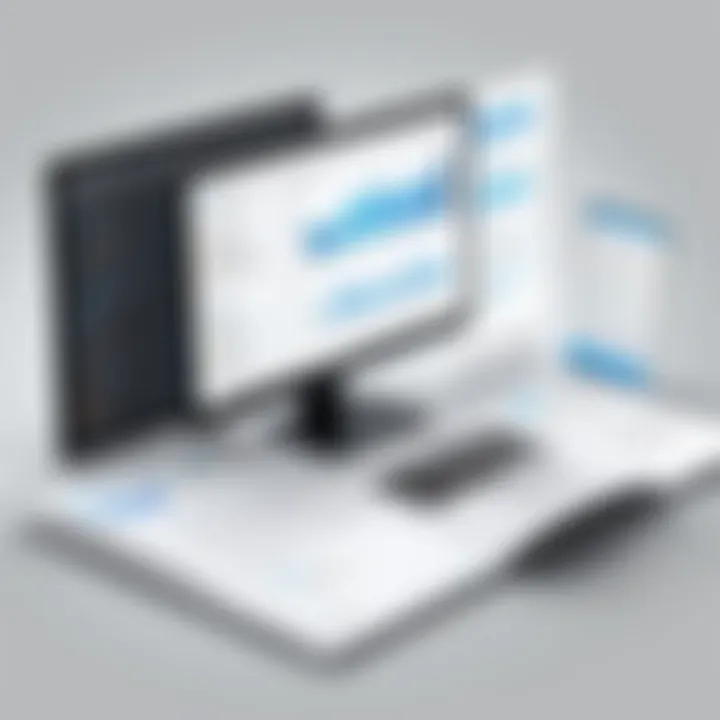
Historical Perspective
The evolution of stored procedures can be traced back to the earlier versions of relational database management systems. Initially, they were simple functions that helped improve performance by reducing the number of round trips between the application and the database server. Over the years, as databases became more complex, so did stored procedures. They evolved to support conditional logic, loops, and error handling, features which were essential for managing intricate business processes.
In PostgreSQL, stored procedures gained significant capabilities with version 11, introducing features such as named parameters and transaction control using and commands in procedures. These advancements marked a turning point, allowing developers to create more sophisticated logic within a simplified framework.
Understanding this historical context provides insight into why stored procedures are vital in today’s database management landscape. They not only help optimize query performance but also reduce the complexity of managing data interactions directly through application code.
Understanding the Syntax
Understanding the syntax of PostgreSQL stored procedures is fundamental for anyone looking to write effective and efficient procedures. A well-structured syntax not only ensures that the code compiles correctly but also increases readability and maintainability. This is crucial in collaborative environments where multiple developers may work on the same codebase. Clear syntax helps in understanding the flow of the stored procedure, making it easier to debug and enhance.
Moreover, grasping the syntax allows for better optimization. Knowing how to structure SQL commands, use parameters, and define return types can significantly affect performance. This knowledge empowers developers to write stored procedures that execute swiftly and consume minimal resources.
Basic Syntax Overview
The basic syntax for creating a stored procedure in PostgreSQL can be broken down into several key components. The fundamental structure generally includes the statement, followed by the procedure name, a list of parameters, and the procedure body.
When writing any stored procedure, keep in mind:
- Procedure Name: This should be unique in the context of the database, identifying what the procedure does.
- Parameter List: Each parameter must have a name and a data type, reinforcing how data flows into the procedure.
- Language: Specifying the programming language used, usually PL/pgSQL for PostgreSQL.`
Parameter Types
Parameters are crucial as they allow input values to be passed into the stored procedure. Each parameter must have a defined data type, which could be common types like , , , etc. For example:
In this code snippet, receives an input value, while serves as an output parameter. Understanding how to declare parameters properly aids in improving the robustness and reusability of stored procedures.
Return Types
The return type in a stored procedure is less common compared to functions, but it can still play a vital role. While stored procedures do not typically return values as functions do, they can utilize OUT parameters or perform actions that affect the database state. It is essential to understand the distinction. When you want to return a value, you can either use OUT or REF cursor. Defining the intended output helps clarify what the procedure aims to achieve.
In summary, mastering the syntax of PostgreSQL stored procedures is essential for executing complex tasks efficiently, facilitating error handling, and building a robust database architecture. The clarity of syntax enhances communication among team members and promotes code longevity.
Key Functionalities
Understanding the key functionalities of stored procedures is essential for effectively leveraging PostgreSQL in various applications. These functions serve as crucial tools for optimizing database operations and enhancing overall performance.
Executing SQL Commands
Stored procedures are specifically designed to encapsulate SQL commands that can be executed on demand. This functionality streamlines operations. By grouping multiple SQL statements into a single callable unit, a developer reduces the amount of code needed to perform complex tasks. For instance, a stored procedure can execute several DML operations, such as INSERT, UPDATE, or DELETE, all in one go. This approach reduces the communication overhead between the application and the database, which is particularly beneficial in high-load scenarios. Additionally, encapsulating logic in stored procedures makes the database interactions more manageable and secure, as it limits direct exposure of the underlying data structure.
Data Manipulation Capability
The ability to manipulate data efficiently is one of the distinguishing features of stored procedures. Users can write procedures that accept parameters for dynamic data handling. This functionality allows for versatile data operations. For example, a stored procedure can be tailored to update specific records based on input parameters, thus allowing flexible data management. Furthermore, stored procedures can return data sets which makes them powerful when integration with applications is required. Here are some important aspects:
- Parameterization: Using parameters allows dynamic filtering and updating of data based on user input.
- Reduced Repetition: Developers can reuse stored procedures across different applications, minimizing redundancy in code.
- Improved Security: By limiting direct access to tables, stored procedures decrease the risk of SQL injection attacks.
Conditional Logic Implementation
Conditional logic is another significant aspect of stored procedures. By incorporating control structures, such as IF statements or CASE conditions, stored procedures can effectively manage the flow of execution based on data conditions. This capability is crucial for businesses that require complex business logic to be executed within the database. For example, a procedure can decide whether to commit or roll back transactions based on the outcome of previous operations, thus preserving data integrity. Here are key points regarding its utility:
- Dynamic Execution Paths: Depending on input conditions, different code branches can execute. This provides flexibility in operations.
- Error Handling Integration: Conditional logic can be coupled with error-handling mechanisms to reinforce data reliability.
- Business Logic Enforcement: Complex rules can be enforced directly at the database level, ensuring consistency across applications.
"The utilization of stored procedures enables efficient data operations, enhancing both performance and security in PostgreSQL environments."
In summary, the key functionalities of stored procedures—executing SQL commands, handling data manipulation, and implementing conditional logic—are foundational elements that empower developers and administrators to design effective, secure, and scalable database applications.
Creating Your First Stored Procedure
Creating your first stored procedure is a pivotal step in understanding the powerful features offered by PostgreSQL. Stored procedures allow for the encapsulation of complex business logic directly within the database, promoting both efficiency and maintainability. They can simplify repetitive tasks, reduce network traffic by executing logic on the database server, and can enhance performance through optimized execution plans. Therefore, knowing how to create such procedures is essential for anyone who aims to master PostgreSQL.
Step-by-Step Guide
To create your first stored procedure in PostgreSQL, follow these essential steps:
- Set Up Your Environment: Ensure that you are connected to your PostgreSQL database instance using a database tool like pgAdmin or via the command line. Having a suitable environment is crucial.
- Define the Procedure: Start by defining the procedure's name and its parameters. The syntax usually follows the pattern:For example, a simple procedure to add two numbers could be:
- Implement Logic: Inside the and block, incorporate the SQL commands and logic needed to perform the desired tasks. For the preceding example, the logic is merely a simple raise notice command demonstrating how to add numbers.
- Save the Procedure: Execute the command to save the procedure in the database.
- Verify Creation: Check through a query to ensure your procedure is now part of the database object structure. You can query to verify.
Testing Your Procedure
Once you have created your stored procedure, the next step is testing it to ensure it performs as expected. Testing serves multiple purposes, foremost among which are validating the logic, checking for potential errors, and ensuring optimal performance.
To test your procedure, you can follow these steps:
- Call the Procedure: Use the statement to invoke your created procedure. For example:This execution should raise the notice with the correct sum.
- Check Output: Observe the output, which in this case should confirm the expected sum of 15. Ensure that the stored procedure handles a variety of inputs, including edge cases, to guarantee robust performance.
- Debugging: If the procedure does not work as intended, review the logic inside the defined blocks. Using logging or raises for debugging output can be immensely helpful in honing in on the source of any problems.
In summary, creating and testing your first stored procedure involves meticulous attention to the definition, logic implementation, and verification. This process not only fortifies your understanding of PostgreSQL but also lays the foundation for crafting more complex database solutions.
Optimization Techniques for Stored Procedures
Optimization techniques for stored procedures are crucial for achieving efficient database operations. Proper optimization can significantly improve performance, reduce resource consumption, and ultimately lead to a smoother user experience. In the context of PostgreSQL, where complex queries may be run frequently, optimizing stored procedures is not just beneficial—it's essential.
Performance Tuning
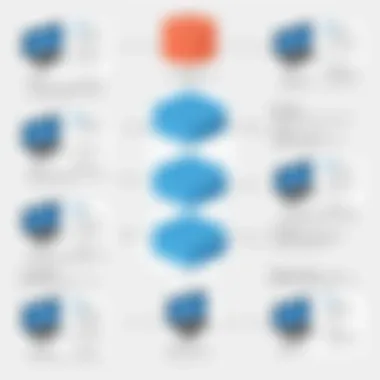
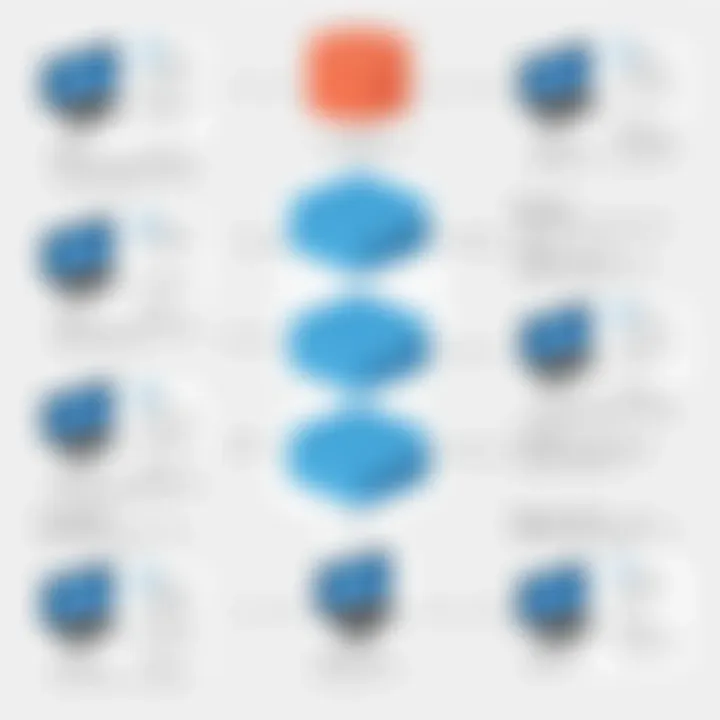
Performance tuning involves adjusting various parameters to enhance the execution efficiency of stored procedures. This process may include optimizing SQL queries, proper indexing, and caching. Specific actions to consider include:
- Query Optimization: Analyze the SQL queries within the stored procedure. Rewrite them for clarity and efficiency. Use to understand the query execution plan. This helps identify slow parts that may need adjustment.
- Index Usage: Ensure that appropriate indexes are in place. An index can significantly reduce the search space for a query, speeding up data retrieval. However, keep in mind that while too many indexes can slow down data modification operations, the right balance is key.
- Configuration Parameters: PostgreSQL’s configuration can be fine-tuned to affect how it handles workloads. Tweaking parameters like , , and can lead to notable performance improvements.
Tuning these parameters allows stored procedures to execute with reduced overhead. It reduces the time taken for database operations and improves overall application responsiveness.
Reducing Execution Time
Reducing execution time is a direct outcome of effective optimization. Here are several strategies to minimize execution duration of stored procedures:
- Limit Cursor Usage: While cursors may seem useful for iterative processing, they can introduce significant overhead. Aim to replace cursors with set-based operations whenever possible. Processing data in bulk is generally faster than processing row by row.
- Batch Operations: If a stored procedure is set to perform repetitive actions, consider batching operations. This minimizes round trips to the database and consolidates work into fewer transactions.
- Transaction Management: Efficient transaction management is vital. Use transactions judiciously to prevent locking issues but ensure they are not left open longer than needed.
"Efficient execution can lead to performance gains that are easily visible in applications and databases where user experience relies on responsiveness."
- Profiling Tools: Utilize PostgreSQL profiling and monitoring tools to identify bottlenecks in stored procedures. Tools such as can offer insights into execution times and help target specific areas for improvement.
By applying these techniques, developers can achieve significant reductions in execution times. The result is faster access to the data and a better experience for users interacting with the databases. Optimization is an ongoing process. Regular review and adjustments are necessary for maintaining high performance as applications and data needs evolve.
Differences Between Stored Procedures and Functions
Understanding the differences between stored procedures and functions is crucial for effective database development and management. While both serve to encapsulate SQL code for reuse, their purposes, behaviors, and use cases differ significantly. By grasping these distinctions, developers can make informed decisions on when to employ each within their database architecture.
Use Cases
Stored procedures are best suited for scenarios where a series of operations need to be performed on the database. This includes data manipulation like inserts, updates, or deletes. For instance, in an e-commerce setting, a stored procedure can automate the process of updating inventory levels after a successful transaction.
Functions, on the other hand, are typically used for computations or to return single values. They are well suited for situations where a value needs to be retrieved based on input parameters. For example, a function might compute the total price of an order by applying discounts and taxes based on user input.
Some practical use cases include:
- Stored Procedures:
- Functions:
- Batch processing of sales data.
- Complex data manipulations or multiple updates in one execution.
- Calculating user ratings based on current reviews.
- Returning daily sales figures for reporting.
Implementation Criteria
When considering whether to use a stored procedure or a function, several factors come into play. First, it’s important to decide the need for returning a result. Functions return a single value, while stored procedures may not return a value at all. This distinction influences how they are called within SQL statements.
Another factor is transaction control. Stored procedures can control transactions, allowing developers to commit or roll back changes based on the success of execution. Functions cannot handle transactions, which limits their use in complex operations requiring multi-step processes.
Security is also a concern. Stored procedures can encapsulate sensitive operations, limiting direct access to the underlying tables. In contrast, functions are less flexible in this respect. Thus, it’s essential to evaluate the security requirements of your application when choosing between the two.
In summary, distinguishing between stored procedures and functions equips developers with the necessary insights to optimize their SQL coding practices. With well-defined use cases and careful consideration of implementation criteria, developers can leverage the strengths of both features in PostgreSQL effectively.
Error Handling in Stored Procedures
Error handling is an essential component of developing reliable and effective stored procedures in PostgreSQL. This aspect of programming, often overlooked by beginners, can significantly influence the robustness of the database processes. Effectively managing errors ensures that the stored procedures can handle unexpected scenarios gracefully without crashing the application.
When errors arise during execution, they can disrupt the flow of commands, lead to data inconsistencies, or even compromise the integrity of the database. Being proactive about error handling helps developers anticipate future challenges and build resilience into their stored procedures. Furthermore, a well-structured error handling approach provides clarity to users, facilitating debugging and maintenance.
Common Errors
Stored procedures can encounter various types of errors. Understanding these will greatly assist in crafting effective error management strategies. Here are some common issues:
- Syntax Errors: These occur when the SQL commands within the stored procedure are not written correctly. Syntax errors can be easily avoided by thorough review and testing before deployment.
- Runtime Errors: These errors occur during the execution of the stored procedure, often due to issues like invalid data types or division by zero. They can, however, be effectively managed using proper error handling techniques.
- Logic Errors: Sometimes, the stored procedures will run without syntactic issues but return incorrect results due to logical flaws. Identifying these requires careful examination and testing of the logic behind the stored procedure.
Common errors can derail procedures, making knowledge of potential pitfalls crucial. Any developer faced with these challenges must have a plan in place to handle them smoothly to maintain operational integrity.
Using EXCEPTION Blocks
In PostgreSQL, the EXCEPTION clause is a powerful tool for error handling in stored procedures. It allows developers to define how the procedure should respond if an error occurs. By integrating EXCEPTION blocks, developers can specify alternative actions to take, thus preventing the procedure from failing completely. Here’s how it works:
- Define the EXCEPTION Block: At the end of the procedure, the EXCEPTION clause is added to specify error handling operations. This block allows you to catch specific errors and respond accordingly.
- Specify Error Handling Logic: Within the EXCEPTION block, you can define custom logic for different types of errors. This could involve rolling back transactions, logging errors, or even sending alerts to system administrators.
- Use SQLSTATE Codes: PostgreSQL utilizes SQLSTATE codes to classify errors. Familiarity with these codes enables precise error handling. For instance, using code signifies a no data found scenario, which developers can address accordingly in the EXCEPTION block.
The following code demonstrates how to use EXCEPTION blocks in a PostgreSQL stored procedure:
Implementing EXCEPTION blocks not only aids in graceful handling of unexpected events but also strengthens the overall design of stored procedures. It provides a structured approach to addressing errors, enhancing the reliability of programmed database operations.
Effective error handling is vital. The success of database applications often depends on how well developers anticipate and manage errors.
Best Practices for Effective Stored Procedure Design
Designing effective stored procedures is crucial for optimal database performance and maintainability. Stored procedures are precompiled, database-specific routines that enhance the performance of security and encapsulation of logic for repetitive tasks. Following best practices ensures database projects are both scalable and efficient.
Maintainability Considerations
Maintainability is a key aspect of designing stored procedures. It is essential to make stored procedures easy to read, modify, and debug. Here are some key points to consider:
- Clear Naming Conventions: Use meaningful names that indicate the purpose of the stored procedure clearly. For instance, using names like instead of vague names like makes it easier for other developers to understand the code.
- Documentation: Comment extensively within the logic. Providing context for the logic, parameters, and expected outcomes can drastically improve maintainability.
- Modular Design: Break large stored procedures into smaller, reusable ones. This reduces complexity, making each piece easier to test and update without affecting the entire system.
- Error Handling: Implement robust error handling to effectively manage exceptions. This can prevent unexpected behaviors from affecting the overall program.
Proper maintenance saves time and resources in the long run. Following these considerations helps identify issues early.
Security Implications
When designing stored procedures, security is an ever-important factor. Poorly designed procedures can become gateways for vulnerabilities. Here are several points to consider regarding security implications:
- Parameterization: Always use parameterized queries within stored procedures to prevent SQL injection. This separates data from commands, making it harder for attackers to influence queries.
- Least Privilege Principle: Grant users the minimum permissions necessary to execute a stored procedure. This limits potential damage in case of exploitation.
- Audit and Logging: Implement audit mechanisms to record the execution of stored procedures. Knowing who, when, and how procedures are executed is vital in identifying security breaches.
- Using Roles: Assign roles instead of individual permissions to manage access control more effectively. This simplifies permission management over an evolving team.
In summary, focusing on maintainability and security during the design phase contributes to the overall success of stored procedures. These best practices not only enhance performance but protect the integrity of the database system.
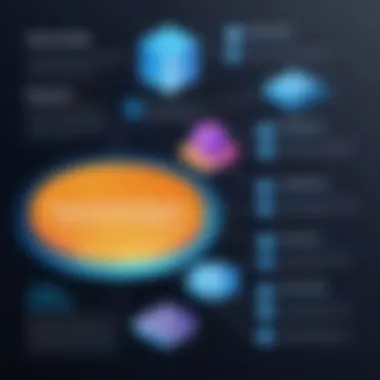
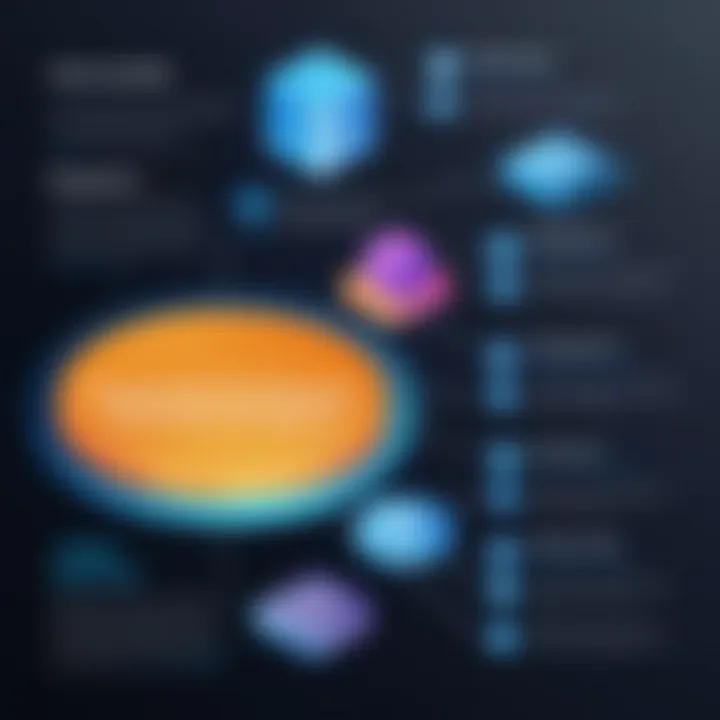
Integrating Stored Procedures with Applications
Integrating stored procedures within applications is essential for enhancing the overall functionality and performance of database interactions. Applications rely heavily on efficient database management, and stored procedures play a significant role in achieving this. Stored procedures encapsulate SQL code, which means that complex operations can be executed on the server rather than the application layer. This transition reduces network traffic and improves data handling efficiency. Moreover, by centralizing logic within the database, stored procedures promote code reuse, ensuring that developers can maintain consistency across various applications.
Connection Protocols
Connection protocols are critical when integrating stored procedures with applications. These protocols streamline the communication between the application and the PostgreSQL database. Commonly used protocols include TCP/IP and Unix sockets.
When applications communicate with the database via TCP/IP, the data travels over a network. This method is flexible and suitable for remote database connections. Alternatively, Unix sockets provide faster local connections as they operate directly through the file system.
Both protocols allow efficient execution of stored procedures, enabling applications to send parameters and receive results seamlessly. Here are some considerations regarding the choice of connection protocol:
- Performance: Unix sockets generally offer lower latency for local connections, while TCP/IP may have overhead for remote access.
- Security: Ensure that appropriate security measures are in place, particularly for TCP/IP connections, to protect data in transit.
- Scalability: Consider how the choice of protocol will affect the application's ability to scale as demand increases.
Interfacing with Various Programming Languages
Interfacing stored procedures with different programming languages is vital for application development. Developers can utilize various programming languages such as Python, Java, PHP, or C# to interact with PostgreSQL stored procedures. Each language provides libraries or frameworks that simplify the connection and execution of stored procedures.
For example, in Python, the library enables developers to execute stored procedures easily. A simple code snippet for executing a stored procedure might look like this:
With this approach, developers can leverage the power of stored procedures within their applications much more effectively. Some benefits include:
- Ease of Use: Libraries available in various programming languages make it straightforward to work with stored procedures.
- Code Simplicity: Developers can focus on application logic, reducing the complexity associated with direct SQL commands.
- Flexibility: Businesses can choose the technology stack that best suits their needs, all while maintaining database interactions via stored procedures.
Utilizing stored procedures enhances not just performance but also the maintainability of the application architecture.
Real-World Use Cases
In practical settings, realizing the utility of PostgreSQL stored procedures can enhance the operational efficiency of applications. These procedures allow for complex business logic to be encapsulated within the database itself. This significantly reduces the amount of data sent over the network, as operations become executed directly on the server. By doing so, applications can experience marked improvements in performance and lower latency. Moreover, stored procedures serve as a single access point for data operations, which simplifies maintenance and security management.
E-commerce Applications
The e-commerce sector is an excellent domain for employing stored procedures. These procedures streamline various processes, such as order processing, inventory management, and user authentication. For instance, when a customer places an order, a stored procedure can handle tasks like checking inventory levels, calculating total costs, and updating relevant records in a single call.
Here are several specific benefits to utilizing stored procedures in e-commerce applications:
- Efficiency: Performing multiple SQL commands in one procedure can reduce round trips to the database, improving speed.
- Consistency: Business rules can be enforced uniformly within the procedure, ensuring that all transactions adhere to the same logic.
- Security: By limiting direct access to the database, stored procedures act as an additional security layer. Users interact with the procedures rather than directly with the tables.
A typical example might include a stored procedure that combines user data retrieval and order generation. Here is a basic code outline for such a procedure:
This simple procedure exemplifies how clear and concise logic can be implemented to facilitate e-commerce transactions.
Data Warehousing Solutions
Data warehousing represents another significant area where stored procedures play a crucial role. Typically, data warehousing concerns the collection, storage, and analysis of large volumes of data. Here, stored procedures can handle data extraction, transformation, and loading processes, often referred to as ETL.
The advantages in a data warehousing context include:
- Batch Processing: Stored procedures can efficiently manage ETL processes by pulling data from various sources, transforming it accordingly, and loading it into warehouse tables.
- Simplified Data Governance: With centralized logic within stored procedures, it becomes easier to enforce data governance policies.
- Scheduled Execution: Procedures can be scheduled to run at off-peak hours, optimizing system resources.
For instance, a stored procedure can perform nightly data aggregations and load results into summary tables for reporting purposes. A simplified example may look like this:
This procedure exemplifies efficiency and automation in data warehousing, illustrating how stored procedures can greatly enhance overall data management strategies.
Future Trends in PostgreSQL Stored Procedures
The future of PostgreSQL stored procedures is a critical area to explore, especially considering the evolving technology and database requirements. Understanding future trends helps developers and database administrators prepare for enhancements and optimizations in their workflows. Analyzing these trends encourages better practices and broadens the scope for innovative database solutions. With the development of PostgreSQL constantly progressing, examining what lies ahead in stored procedures can yield several benefits.
Emerging Features
PostgreSQL is known for its robust feature set, and this includes stored procedures. As the community develops, new functionalities continuously emerge. Among the anticipated features, some will improve usability, while others will focus on performance enhancements. Recent updates indicate a probable integration of improved debugging tools. These tools may simplify the troubleshooting process when issues arise within stored procedures.
Another emerging feature is the support for additional procedural languages. Recent updates hint at expanding the languages supported within stored procedures beyond PL/pgSQL. This allows developers to utilize languages they are already fluent in, potentially speeding up the development process.
Moreover, the introduction of features that enhance parallelism and concurrency will be essential. Optimizing execution paths and enabling simultaneous process handling can significantly improve performance, especially for large datasets.
Community Contributions
The PostgreSQL community plays a vital role in shaping the future of stored procedures. The open-source nature of this database system allows users to propose modifications and improvements. This leads to a form of collective wisdom where multiple perspectives on problem-solving converge. Many emerging features stem from community feedback and needs recognized during database consultations.
Participating in forums such as Reddit or contributing to documentation on platforms like Wikipedia enables users to share experiences and highlight gaps in the current feature set. Additionally, as more businesses adopt PostgreSQL, their specific use cases expose areas needing improvement. This input becomes crucial for developers focusing on the future.
Furthermore, community-driven projects and discussions can drive feature addition, ensuring that PostgreSQL remains flexible and innovative. Engagements through platforms like Facebook promote conversations around enhancements and future directions. This opens up possibilities for networking, collaborations, and knowledge sharing within the community.
"The innovative nature of PostgreSQL is a testament to its active user community and the continuous quest for improvement amongst its developers."
In summary, the future of PostgreSQL stored procedures showcases emerging features fueled by community contributions. Understanding these aspects prepares users for advanced database management, ultimately enhancing performance and usability.
The End
The conclusion of this article serves as a pivotal point that encapsulates the core ideas discussed regarding PostgreSQL stored procedures. Understanding the implications and benefits of stored procedures is essential for anyone aspiring to manage databases effectively. Stored procedures streamline database operations, ensuring that complex tasks can be executed quickly and efficiently. They also foster code reusability, which simplifies development and reduces errors during implementation.
Summary of Key Points
- Definition of Stored Procedures: Stored procedures are predefined SQL statements stored in the database.
- Syntax Understanding: The syntax requires familiarity with parameters and return types, which are critical to writing effective stored procedures.
- Key Functionalities: Stored procedures can execute SQL commands, manipulate data, and incorporate conditional logic. This flexibility is advantageous for any database management tasks.
- Creating and Testing: A systematic approach to creating stored procedures enhances their effectiveness. Testing procedures is also essential to catch errors before deployment.
- Best Practices: Emphasizing maintainability and security in design helps to avoid potential pitfalls that could arise later.
- Real-World Applications: Stored procedures find robust applications in various sectors such as e-commerce and data warehousing. The versatility in these environments emphasizes their importance.
Final Thoughts on Stored Procedures
As we conclude, it’s critical to appreciate the evolving nature of PostgreSQL and its stored procedures. The community continually contributes to enhancements and adapts future trends to meet demand. Mastering stored procedures not only improves efficiency but also arms developers with the knowledge to create scalable and secure database applications. In an era where data management is paramount, the ability to utilize stored procedures effectively will be a significant factor in a programmer’s success. Understanding and applying the insights from this article will surely augment your database management capabilities, preparing you for the challenges ahead.
"Proficiency in stored procedures is an invaluable asset in the toolkit of a proficient database developer."
With the right approach and continued learning, embracing stored procedures offers a pathway to more efficient and robust database interactions.