Kickstart Your Python Project: Essential Steps and Tools
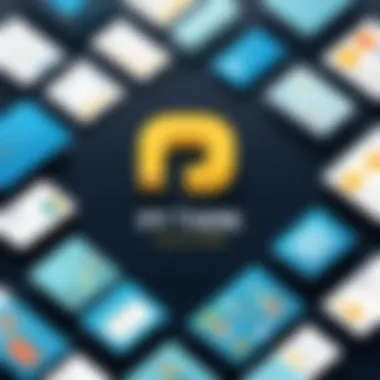
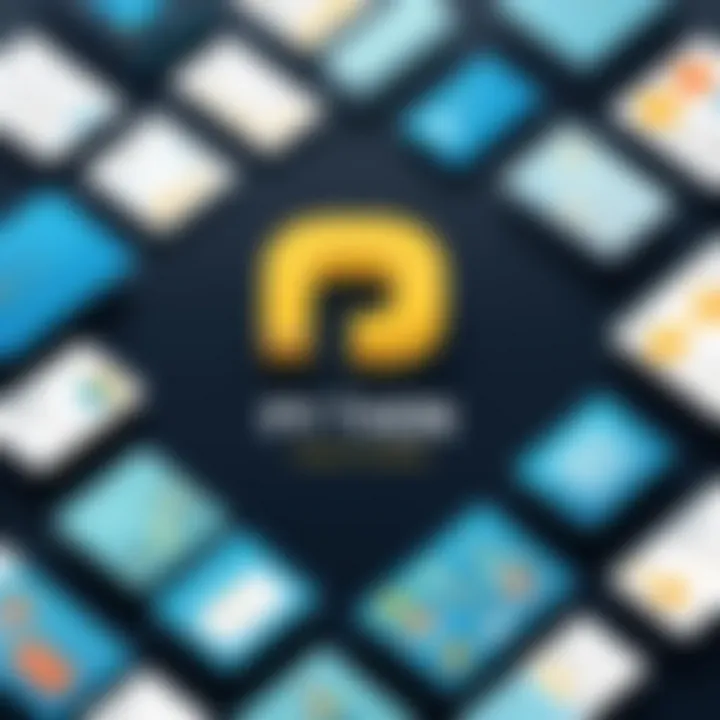
Intro
Preface to Programming Language
Python is a high-level programming language known for its clear syntax and robust versatility. Designed by Guido van Rossum, Python was first released in 1991. Over the decades, it has transformed into one of the most favored languages for various applications, from web development to data analysis.
History and Background
Python is built on the ideals of readability and simplicity, which are evident in its design and functionality. Its origin lies in the 1980s, focusing on interactivity and simplicity, leading to the conception of a language that developers could efficiently use. The usage of Python spiked during the 2000s as the internet began to grow extensively, and data manipulation became vital for companies.
Features and Uses
Some distinct features of Python are its easy-to-understand syntax, dynamic typing, and comprehensive standard libraries. These attributes make it conducive to rapid application development. Python is widely used in various fields, including:
- Web development (Ceml, Django)
- Data Science (Pandas, NumPy)
- Automation and scripting
- Machine Learning (TensorFlow, SciKit-Learn)
These roles further elevate its relevance and scope in burgeoning industries that depend on programming.
Popularity and Scope
In 2023, Python is consistently cited as a top programming language, appended by numerous sources like TIOBE and Stack Overflow reports. With a vast global community around it, Python is favored by both beginners and experienced developers alike. Robust libraries and frameworks, as well as community support, play a significant role in cementing its status.
Basic Syntax and Concepts
To begin your journey with Python programming, understanding its basic syntax is essential.
Variables and Data Types
Variables in Python serve as containers for data values. Key data types in Python include:
- Integers
- Floats
- Strings
- Lists
The simplicity of defining variable types makes it accessible for newcomers. For example, you could initiate a variable as such:
Using such data types intuitively integrates with operations one conducts on variables later.
Operators and Expressions
Python has various operators for performing arithmetic, logical, and comparative operations. These include:
- Arithmetic operators (, , , )
- Comparison operators (, , ``, )
Combining variables and operators creates expressions and computations conducive to the tasks at hand.
Control Structures
Python employs control structures, such as conditional statements and loops, aiding in controlling the flow of programs. Using , , and , developers can construct program logic seamlessly. For instance:
These statements help build dynamic applications, crucial for project development zoning.
Advanced Topics
Developers should explore advanced topics to skillfully navigate Pythonβs capabilities.
Functions and Methods
Functions are a fundamental part of programming in Python. They allow the encapsulation of reusable code blocks. A simple function can look like this:
Using functions promotes efficient coding practices and simplifies complex tasks intobits manageable portions.
Object-Oriented Programming
Python supports object-oriented programming, which reinforces the organization of code with classes and objects. This feature enhances reusability and lifespan management of elements in code. Classes are created as follows:
Understanding classes are paramount to structuring significant applications.
Exception Handling
Python offers exception handling to gracefully handle errors that inescapably arise in programming reality. Utilising and , developers can inform themselves and the user when errors are caught, maintaining user experience intact.
For instance:
This structure helps maintain standard application flow even in unexpected scenarios.
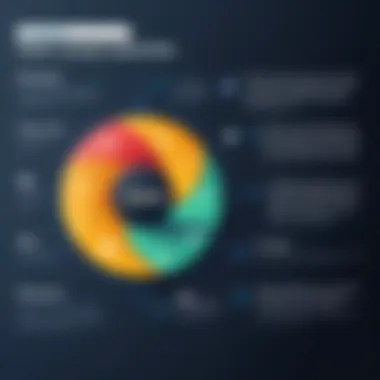
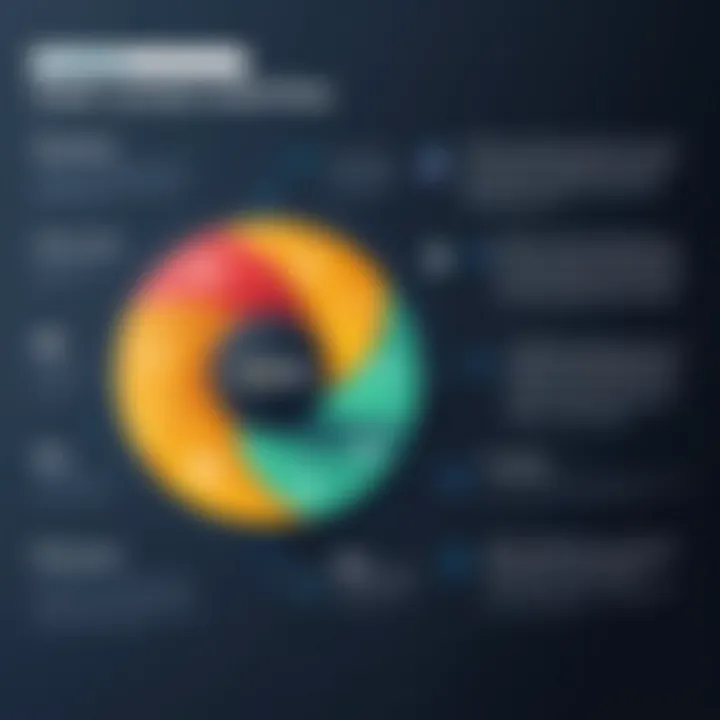
Hands-On Examples
Diving into practical applications offers an exceptional learning experience. Already learned concepts of communication get translated into reality here.
Simple Programs
Starting with small projects can be illuminating. A basic calculator functionality coded in Python elucidates operator engagement and provides incremental knowledge.
Intermediate Projects
Once foundational knowledge is acquired, progressing onto intermediate projects like web scraping with Beautiful Soup or simple GUI applications with Tkinter would elevate project experience.
Code Snippets
Objects, syntax references, and reusable code also epitomize essential markdowns approached within project design. For example, appending demo core loops facilitates understanding:
Resources and Further Learning
Seeking further resources could bolster skills and foster expertise in Python.
Recommended Books and Tutorials
Books like Automate the Boring Stuff with Python and online tutorials including Codecademy present potent preparatory tools for beginners.
Online Courses and Platforms
Mega resources like Coursera and edX provide comprehensive programming courses. Each course can numbers steps granularly, assisting in structured knowledge absorption.
Community Forums and Groups
Partaking in forums like Reddit holds essential value for interacting with fellow learners. Engaging in discussions can deepen understanding and unveil new perspectives on hurdles faced during Python journey.
span>Further learning creativity crucial once getting comfy coding elements and development methodologies.span>
Understanding the Python Ecosystem
Understanding the Python ecosystem is crucial for anyone starting out with this programming language. It is a landscape filled with tools, libraries, frameworks, and communities that can greatly enhance your project development process. Engaging with this ecosystem will help you gain insights into Python's capabilities, allowing you to leverage them effectively for various purposes, be it web development, data analysis, or artificial intelligence.
Why Choose Python for Your Project
Python stands out as a popular choice for both beginner and seasoned programmers. Here are some reasons that contribute to its widespread adoption:
- Versatility: Python can be used in many fields, from web development to scientific computing, thanks to its extensive libraries.
- Simplicity: Its clean and readable syntax allows programmers to express concepts in fewer lines of code. This simplicity lowers the barrier for new developers.
- Strong Community Support: A large and active community means ample resources, discussions, and shared knowledge, which is invaluable for problem-solving and project growth.
In addition, Python's compatibility with other languages and its ease of integration make it a valuable asset in any tech stack. This multi-faceted nature simplifies processes, often saving time and resources.
Key Features of Python
Python boasts several features that enhance its effectiveness and efficiency for various projects:
- Interpreted Language: Code written in Python is executed line by line, making it easier to test and debug. This characteristic fosters rapid development.
- Dynamic Typing: There's no need to declare variables explicitly, allowing for more flexibility in coding.
- Rich Standard Library: Python provides built-in modules and packages, which can save you from writing boilerplate code and streamline functionality.
Python also supports multiple programming paradigms, such as procedural and object-oriented programming. This adaptability enables developers to choose their preferred approach when designing a system, contributing to better productivity.
Emphasis on Readability: One of Python's core philosophies is code readability. Programs remain simpler to manage and modify over time, fostering maintainability.
Setting Achievable Milestones
Setting significant milestones within your project facilitates structured progress tracking. It divides the overall project into manageable parts that can be tackled systematically. Every milestone acts like a checkpoint, allowing for assessments and adjustments based on accomplishments and bottlenecks that might occur.
When establishing these milestones, smart development practices encourage realism and feasibility. While ambition can drive innovation, unreachable goals lead to frustration and disillusionment. Break projects down into specific phases to ensure steady progress. Here are considerations that make your milestones more effective:
- Set short-term and long-term goals: Both perspectives boost maintainable project momentum.
- Utilize the SMART criteria: Specific, Measurable, Achievable, Relevant, and Time-bound markers help in clarifying your objectives.
- Demarcate dependencies: Recognizing related milestones heightens awareness of how choices impact project stages.
- Celebrate achievements: Acknowledging completion of significant parts can provide motivation and reinforce team cohesion.
Implementing effective milestones provides the framework that leads you toward successful project execution while allowing flexibility to adapt to changing circumstances.
Setting Up the Development Environment
Setting up the development environment is a crucial step in embarking on any Python project. A conducive environment facilitates smoother work flow and is critical for effective development habits. This section will discuss important elements such as choosing an appropriate IDE, installing Python and relevant libraries, and understanding version control with Git. Establishing a solid foundation for development aligns with achieving your project goals efficiently.
Choosing an Appropriate IDE
The Integrated Development Environment (IDE) you choose dramatically affects your coding experience. An appropriate IDE can enhance productivity and understanding of your code's structure. Popular IDEs like PyCharm, Visual Studio Code and Atom offer numerous features that improve coding efficiency. These features include syntax highlighting, code completion, and debugging tools.'
When selecting an IDE, consider factors such as:
- Usability: Choose an IDE that aligns with your coding style and habits. If you prefer simplicity, options like Atom might work, while if you enjoy a feature-rich environment, a choice like PyCharm could serve better.
- Extensions and Support: An IDE that has a facile extensions embrace can be advantageous. For instance, using plugins can add functionality that enhances your workflow.
- Community Support: Having access to a strong community means you can easily find solutions to issues that arise during coding.
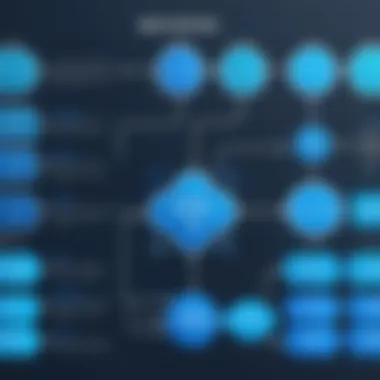
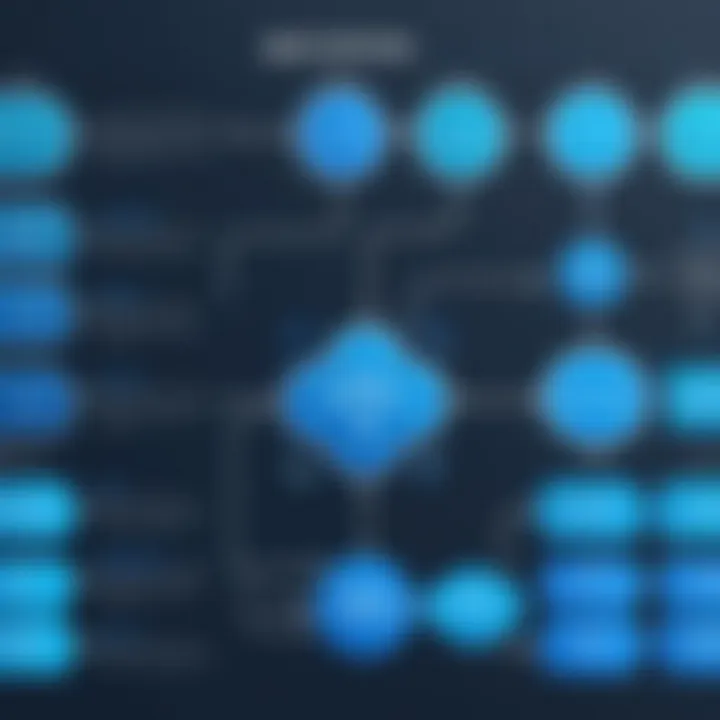
Installing Python and Relevant Libraries
The next step involves installing Python and selecting the relevant libraries for your project. To start, download the latest version of Python from python.org. Installing Python is quite straightforward and the website provides clear instructions. During the installation, it is advantageous to ensure that the install-tion path is added to system variables. This simplifies command-line access.
After setting up Python, determining the libraries essential for your project is important. Libraries make Python really powerful. Popular libraries to consider include:
- NumPy: Useful for mathematical functions and operations on arrays.
- Pandas: Extremely handy for data manipulation and analysis.
- Flask/Django: Great choices for web development projects.
- Requests: Excellent for making HTTP requests seamlessly.
To install libraries, you can use , Python's package installer. For example:
This single command will set your project with notable libraries promptly.
Version Control with Git
Utilizing version control with Git is indispensable for managing changes in your project over time. Git provides a safety net from accidental overwrites and can also assist in collaborative project settings.
With Git, several benefits emerge:
- Version Tracking: You can revert to previous versions if something goes wrong or if you realize a feature is introducing bugs.
- Branching: This allows you to develop features in isolation without disrupting the main code base. After successfully implementing a feature, merge it back into the main branch.
- Collaboration: If you're working with others, Git enables multiple developers to contribute changes. With platforms like GitHub or GitLab, collaboration becomes seamless and efficient.
To begin using Git, download it from git-scm.com. After installation, initializing a new Git repository requires running:
Structuring Your Python Project
Successful project development requires a well-thought-out structure. A clean structure helps in maintainability, collaboration, and scalability of your code. When starting a Python project, you must consider its organization. It serves not only as a roadmap but also supports logical flow and accessibility. Proper structure ensures different parts of your project are delegated their correct purpose, which helps to improve coding efficiency.
Organizing Your File Structure
Organizing your application's files is crucial in making your project easy to partner with and navigate. A clear hierarchy permits developers to find files quickly and understand their roles with high efficiency. Consequently, this can enhance teamwork among developers working on the same code base.
Common recommendations for structuring a Python file system are:
- Project Root: This is the central hub for your project.
- Modules: You should group associated functionalities together.
- Tests: Keep your tests in a separate directory. This can also include unit tests and integrative tests.
- Documentation: Documentation remains crucial for stating the project specifics, installation method, and usage instructions. Proper directories for these ensure they're readily available for reference.
For example:
This example highlights ease of access and clarity, ultimately fostering a smoother development process. You should also consider naming conventions. Keep filenames descriptive and avoid confusion.
Creating Virtual Environments
Virtual environments play a significant role in Python project management. They provide isolated environments that keep dependencies minimal and tailored for radiating efficiency for your project. This isolation means you can selectively maintain specific package versions for each project without conflicts.
To create a virtual environment, you can use the built-in venv module.
Hereβs a simple guide to setting up a virtual environment:
- Open your command line interface.
- Navigate to your project directory.
- Execute the command:
- Activate the virtual environment:
- You can now install project dependencies using pip, which will reflect solely within this environment.
- On Windows:
- On macOS/Linux:
Creating a virtual environment ensures clarity and maintains clean project spaces, making it an indispensable practice within Python development.
Remember, a logical structure helps make any larger Python project manageable and flexible, easing eventual debugging and updates.
Writing Your First Lines of Code
Writing your first lines of code is a critical moment in any Python project. This phase authentically transforms your project plans into tangible solutions. You have defined your project goals, selected your tools, and now comes the time to let your ideas come to life through code. It is in this step that you transition from theoretical concepts to practical implementation.
When you start writing code, remember that clarity should be your utmost priority. It is essential to implement logic in a way that is not just functional, but also understandable. Each function you create should serve a purpose in the larger framework of your project. As such, it is imperative to mist your creative processes with a systematic structure.
Implementing Project Logic
Implementing the logic of your project requires careful consideration of the objectives you want to achieve. This part involves translating your project requirements into algorithms, which ultimately dictate how your code will work.
- Break Down Problems: Dividing your project into smaller, more manageable tasks can help you focus on one aspect at a time. This ensures that each section works correctly before you combine them into a whole.
- Use Control Structures: Emphasize the importance of using control structures like loops and conditionals. They will form the backbone of your program's logic by allowing your code to make decisions and perform actions repetitively.
- Define Functions Clearly: Each function should have a specific role. Be clear about its purpose and parameters.
For example:
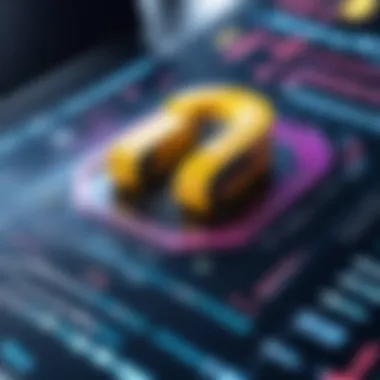
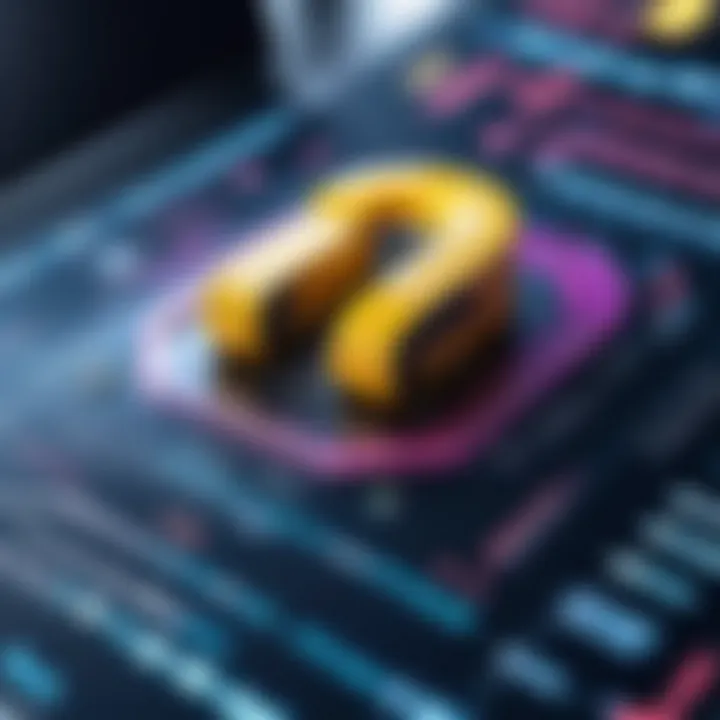
This simple function obeys a clear need β to calculate the area of a circle given its radius. Simplicity in implementation enhances long-term maintainability.
Utilizing Python Libraries
The broad functionality of Python comes largely from its vast array of libraries. Utilizing these libraries can save you significant time while increasing the robustness of your project.
- Leverage Existing Solutions: Many common tasks have already been solved by libraries such as NumPy for numerical computations, Pandas for data manipulation, and Flask for web applications. Integrating them may prevent duplicating efforts.
- Familiarize Yourself with Package Management: Use package managers like pip to easily install and manage libraries. Keeping your dependencies organized is crucial for a clean working environment.
- Regularly Consult Documentation: Whenever engaging with a library, refer to its official documentation. Good documentation will provide examples and guide you on how to achieve your programming goals smoothly.
By specializing in your project logic and effectively utilizing available Python libraries, you set a solid foundation for your application. This approach enables you to build powerful, maintainable projects that align well with your original goals, which, ultimately, is how practical programming makes profound impact.
Ultimately, testing and commenting serve as foundational parts of development, fostering an environment of clarity that not only benefits the programmers but elevates the entire project.
Documenting Your Project
Documenting your Python project is not just about creating notes for yourself. It serves several vital functions that ultimately enhance the overall quality and usability of your work. Thoughtful documentation plays a crucial role in making your project approachable for yourself and others. It often acts as the connecting bridge between intention and implementation. Through documentation, you outline your project's aim, structure, and how to use it effectively. Readers not familiar with your project should be able to grasp what your code accomplishes simply through the documentation.
One of the main benefits of thorough documentation is clarity. It allows anyone diving into your code to understand the purpose and functionality behind various components. This is especially important when you or others return to the code after an extended break. Projects can become challenging to return to, and attentive notes can clarify logic that seemed obvious at first glance.
Aside from supporting comprehension, documenting specfically can also enhance collaboration. When working in team settings, clear directives on project objectives, installed dependencies, and usage instructions streamline the onboarding process for new contributors. Neglecting proper documentation can lead to confusion and inefficiencies. Moreover, maintaining robust documentation helps instill credibility in your projects, allowing future developers to review your design choices and logic. It translates thoughts into an articulate format, fostering constructive conversation around your work.
Furthermore, taking time to document Invests directly in your learning journey. Explaining complex functionalities preperly requires you to grasp them deeply. This iterative process can reinforce concepts that you may not have mastered fully yet.
In Python, one of the most prevalent and simple ways to document your project is through README files. Letβs explore some crucial elements in creating these essential documents.
Creating README Files and Documentation
A README file is often the first impression of your project. It should be a shining example of what your project is and how it works. Having a well-structured and informative README not only guides users but also assists other developers who may use your code in future projects.
A good README often includes the following components:
- Project Title: State the name of your project simply and clearly.
- Project Description: A concise overview explaining what the project aims to accomplish.
- Installation Instructions: Step-by-step guidance on how others can install your project easily. Note any dependencies or system requirements.
- Usage Instructions: Examples demonstrating how to use your project can be exceptionally helpful. Incorporating clear examples showcases its functionality.
- Contributing: Briefly outline how others can contribute to your project, including any guidelines to follow.
- License: Clearly state the terms under which your project may be used.
Creating these instructional and supportive sections is not a hold up. Rather, they prevent painful confusions later. They also increase the credibility of your work.
Proper documentation takes time, but its long-term benefits hugely outweigh the short-term commitment.
To wrap things up, always remember that concise, clear, and valuable documentation soars high in importance. As you pour time into code, dedicate sufficient care for processing the clarifications. Effective structuring ensures future self or working colleagues can use and contribute to the project smoothly. A well-crafted README can serve as an inviting front door, bringing others into your programming endeavors.
Deploying Your Python Project
Deploying your Python project is a pivotal step that bridges the gap between development and real-world application. This stage not only defines how users will access your project but also determines the scalability and future viability of your work. Proper deployment can enhance user engagement, ensure reliability, and make ongoing maintenance smoother.
Choosing a Deployment Platform
The choice of a deployment platform should align with the needs of your project. Various hosting services offer ready-to-go environments designed specifically for Python applications. Here are some options worth considering:
- Heroku: Known for its user-friendly interface and straightforward setup, Heroku offers built-in tools for scaling and maintaining Python web applications.
- AWS: Amazon Web Services provides powerful infrastructure options, allowing for extensive customization. It may have steeper learning curve but is backed by vast resources.
- DigitalOcean: Popular among developers, it provides cost-effective solutions with flexible plans suitable for both small and large applications.
- PythonAnywhere: As the name suggests, this is a hosting service specifically designed for Python projects, allowing easy deployment with minimal configuration.
When selecting a platform, consider factors like cost, ease of use, available resources, and customer support. Look for documentation and user feedback as they provide insights into potential pitfalls and benefits.
Registering and Setting Up an Application
Once you choose a platform, the next important step is registering and setting up your application. This stage typically involves a few common steps:
- Create an Account: Sign up with the chosen platform. Ensure you read their terms of service and privacy policies. Different platforms may offer free trials or tiered pricing.
- Provision Resources: Depending on your application, you may need to allocate resources such as a database, web server, or load balancers. Take note of recommendations from your platformβs guidelines.
- Deploy the Code: Most platforms allow you to push your code through command line tools or through integrations via Git. Follow the specific steps outlined in deployment documentation of the platform.
- Environment Variables: This is crucial for securing sensitive data such as API keys, database passwords, etc. Set environment variables according to the platform protocols.
- Test the Deployment: Before announcing your project, consider conducting thorough tests to see how it operates in its new environment. Ensure all features work as expected.
Continuous Improvement and Learning
In software development, the journey does not stop with project initiation or even deployment. Continuous improvement and learning are essential elements for maintaining the relevance and functionality of your Python project. This timely approach benefits you, your project, and ultimately the user community. To remain competitive and useful, developers must adopt a mindset of ongoing enhancement.
To truly understand the importance of continuous improvement, consider the fast pace of technology evolution. Tools, frameworks, and user expectations change rapidly. Without a process for adapting and reevaluating, you face a risk of obsolescence. Embracing continuous learning ensures that you not only keep up with technological advancements but also learn from real-world usage and user feedback. This feedback cycle is critical in realizing the true potential of your work.
Gathering User Feedback
When you finish your project and it goes live, collecting user feedback becomes vital. Users hold valuable insights that help in knowing both strengths and weaknesses. By establishing structured methods for gathering this feedback, you can derive meaningful insights to enhance your project.
Some useful methods to gather feedback include:
- Surveys and Questionnaires: Create simple surveys post-deployment to assess user experiences.
- Beta Testing: Invite a select user group to trial your project before wide release and gather insights focused on real operational challenges.
- User Forums: Facilitate open discussion on platforms like Reddit to encourage conversation on issues, feature requests, and improvements.
- Support Channels: Implement support channels where users can report problems which can pave the way for further upgrades.
Focusing on relevant issues reported by users will help reinforce engagement, ensuring you build a product that continues to meet their needs.
Updating Your Project Based on Insights
Feedback is useless without action. To capitalize on the information gathered, efficient updates are crucial. Adopt a revision approach that augments your core objectives while remaining attuned to user expectations.
Key actions might include:
- Prioritize Feedback: Not all feedback will necessitate immediate attention. Use criteria related to user impact and project vision to prioritize.
- Iterative Releases: Plan updates in cycles while introducing enhancements and keeping stability as a priority. This approach minimizes risks associated with introducing too many changes at once.
- **Documentation can also play an integral role. Updating your documentation in-line with changes keeps your user community engaged and well-informed which strengthens trust.
- Reflect on Metrics: Track progress carefully with analytics tools. Ffor example, tools like Google Analytics can guide your decisions based on user interaction.
Continuous learning further creates a streamlined flow of knowledge, aiding in better decision-making in follow-up projects. Make learning from both successes and failures a recurring part of your development practices. This ensures your work not only serves its immediate purpose but remains viable in the evolving technological brief.
In the world of software development, staying stagnant is equivalent to falling behind. Continuous improvement and active updating strategies based on user feedback can breathe new life into your projects and sustain user interest over time.