Mastering Recursive Formulas: A Comprehensive Guide
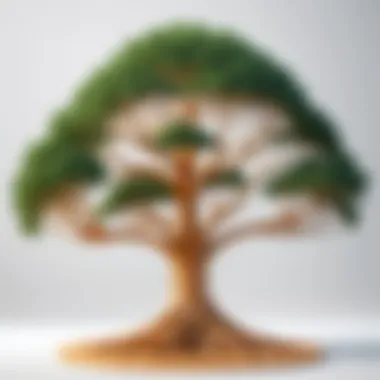
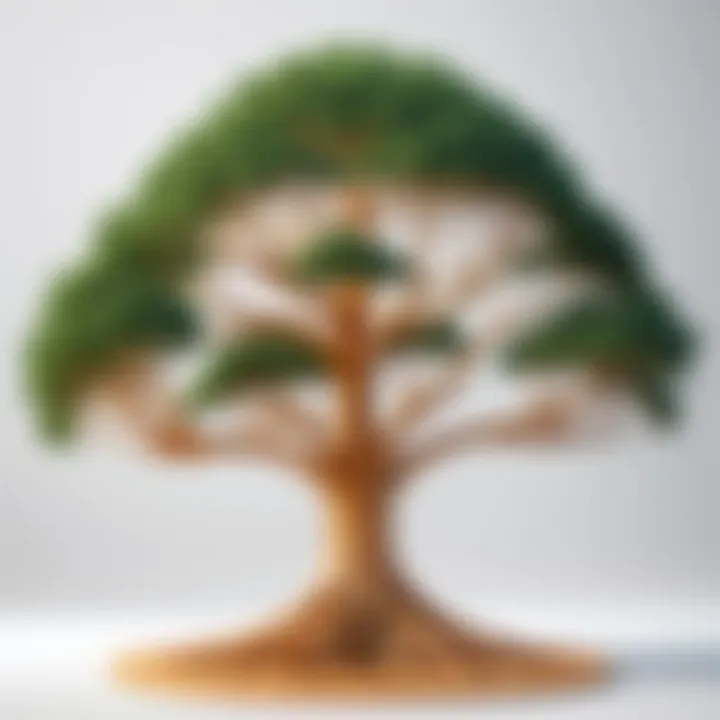
Intro
A recursive approach can simplify complex problems by breaking them down into smaller, manageable components. This guide aims to demystify the process of constructing recursive formulas, equipping learners with both foundational knowledge and practical examples that illustrate their applications in real-world scenarios. By the end of this article, readers will have a solid grasp of recursion and its syntax, enabling them to craft their own recursive definitions effectively.
Basic Concepts of Recursion
Recursion typically involves a base case and a recursive case.
- Base Case: This is the condition under which the recursion terminates. It provides a straightforward answer for a simple case.
- Recursive Case: This part of the formula expresses a larger problem in terms of smaller instances of the same problem.
Recursion can be succinct and elegant, but it requires careful consideration of the base and recursive cases to avoid infinite loops.
Writing a Recursive Formula
When creating a recursive formula, keep these steps in mind:
- Identify the Problem: Determine what needs to be solved recursively.
- Define the Base Case: Specify the simplest instance of the problem and its solution.
- Formulate the Recursive Case: Express the solution for larger instances in terms of the solutions to smaller instances.
- Test the Formula: Ensure that it works by applying it to the base case and progressively larger instances.
Practical Examples
To illustrate how these principles apply, consider the Fibonacci sequence. This is a popular example of recursion:
- Base Cases: F(0) = 0, F(1) = 1.
- Recursive Case: F(n) = F(n - 1) + F(n - 2).
When translated to code, this can look like:
Real-World Applications
Recursive formulas are not just academic. They have real-world applications, especially in computer science:
- Sorting algorithms, like QuickSort and MergeSort, utilize recursion to divide data into smaller subsets.
- Tree data structures often employ recursive formulas for traversing nodes.
Understanding recursion allows developers to optimize complex solutions, making them crucial in software development.
End
Constructing recursive formulas is an invaluable skill in programming and mathematics. By adhering to sound principles and continuous practice, learners can effectively approach recursive problems.
This guide provided a brief overview of how one can write recursive formulas.
For further exploration, online courses on platforms like Coursera and tutorials on websites such as Khan Academy can be useful resources. Engaging with community forums, such as those found on Reddit, can also provide additional insights and guidance.
Understanding Recursion
Recursion is a fundamental concept in both mathematics and computer science. It lays the groundwork for many complex algorithms and problem-solving techniques. Gaining a thorough understanding of recursion is essential for anyone delving into programming, particularly for those who encounter data structures and algorithms.
The dual nature of recursion, involving both a base case and a recursive case, serves as the backbone of this principle. By grasping recursion, one learns how to break down intricate problems into simpler components, making it easier to arrive at a solution. This is not just theoretical knowledge; it has practical applications in various domains like artificial intelligence, game development, and data analysis.
Definition of Recursion
Recursion refers to the process where a function calls itself directly or indirectly to solve a problem. The key aspects of this definition are:
- Function Calls Itself: A recursive function will work on smaller instances of the same problem.
- Base Case: Every recursive function must include at least one base case, which stops the recursion.
- Recursive Case: This defines how the problem is broken down into smaller subproblems.
For example, consider the mathematical definition of the factorial function, where factorial of a number n, denoted as n!, is defined in terms of the factorial of (n-1). This definition illustrates the recursive nature clearly.
Origins of Recursive Thinking
The notion of recursion is not new. It has historical roots in mathematics and logic, tracing back to early thinkers who utilized recursive definitions in number theory. For instance, the concept is evident in the work of mathematicians like Euclid, who described methods of mathematical induction.
As computer science evolved, recursion became an integral part of programming languages. Many influential programming languages, such as Lisp and Scheme, were developed to embrace and enhance recursive approaches to problem-solving. Over time, recursion has been recognized for its ability to express complex computations in a clearer and more concise manner. This makes it not just a tool but a methodology for approaching challenges across various fields.
Understanding recursion provides clarity in problem-solving and stimulates innovative thinking in algorithm design.
The Structure of a Recursive Formula
Understanding the structure of a recursive formula is essential for anyone aiming to master recursion in mathematical or computational contexts. This structure forms the foundation of how recursion functions, allowing formulas to define a series of terms based on previous ones. By breaking down any mathematical problem or algorithm into manageable parts, it reveals the inner workings of recursive definitions. The importance of this topic cannot be overstated, as mastering it equips learners with the necessary skills to effectively tackle complex problems.
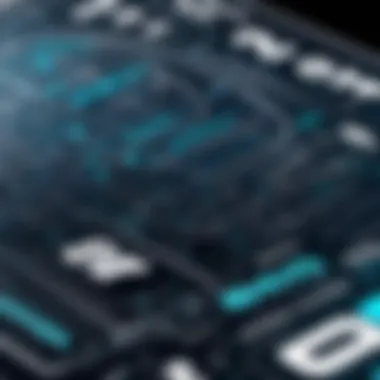
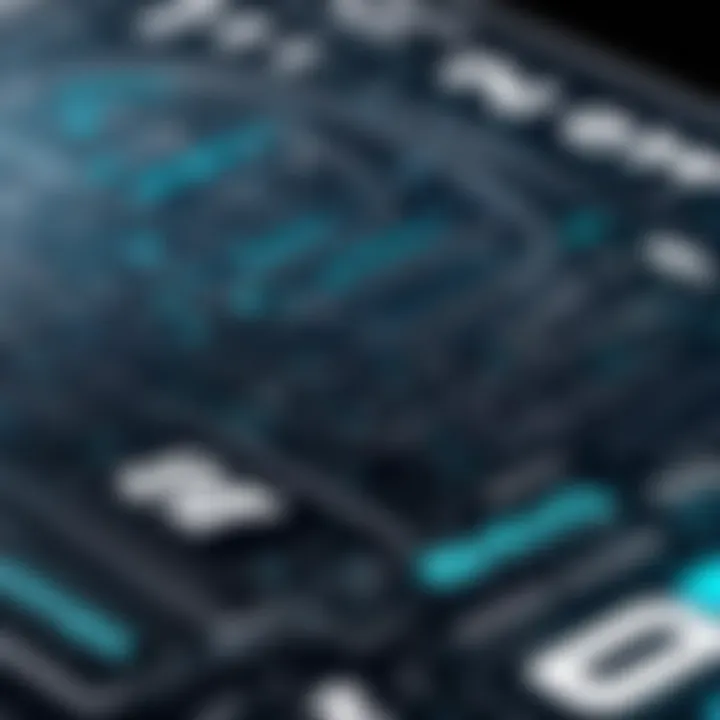
Base Case
A base case is a critical component of any recursive formula. It serves as the stopping condition that prevents infinite loops in a recursive process. Without a base case, the recursion will continue indefinitely, resulting in an overflow error or program crash. This characteristic makes it indispensable for the functionality of any recursive algorithm. A well-defined base case simplifies the logic necessary for understanding the entire recursive structure, making it a popular choice among programmers and mathematicians alike.
Importance of the Base Case
The significance of the base case lies in its role as the foundation for recursion. It provides the necessary anchor for the recursive process by clearly defining when to terminate further recursive calls. This unique feature enhances the reliability of recursive algorithms, ensuring they yield correct results without unnecessary complications. In this article, understanding the importance of the base case will help readers appreciate how it aids in the creation of robust recursive formulas, thereby strengthening their problem-solving capabilities.
Identifying Suitable Base Cases
Identifying suitable base cases can be challenging but is crucial for formulating effective recursive expressions. A proper base case must be simple and easily calculable. In many cases, it represents an obvious low point in the sequence being defined. This aspect is fundamentally beneficial, as it guides the recursive function towards a conclusive outcome. However, choosing a poorly defined base case can lead to incorrect calculations or unexpected behavior. Thus, learners need to understand how to recognize and specify suitable base cases for various situations in recursion.
Recursive Case
The recursive case is where the real power of recursion shines. This part of the formula defines how each term relates to one or more of its predecessors. It is vital for establishing a clear relationship within a sequence that can be executed repeatedly. By formulating the recursive step thoughtfully, programmers can leverage the concise nature of recursion to solve extensive problems efficiently.
Formulating the Recursive Step
Formulating the recursive step involves determining how to progress from one term to another based on the conditions defined by the recursive case. This part of the formula is essential for ensuring that each recursive call yields meaningful results. By carefully crafting the recursive step, one can exploit its advantages in reducing code complexity while increasing clarity. However, a poorly structured recursive step can result in incorrect calculations or inefficient code, underscoring the importance of precision in this aspect.
Relation between Current and Previous Terms
Understanding the relation between the current and previous terms helps to fill the gaps in recursion, connecting each term in the sequence. This connection is crucial for maintaining the integrity of the recursion, ensuring that progress is made toward the base case effectively. The unique characteristic of this relationship allows for the creation of elegant and efficient algorithms. Mismanaging this relationship can, however, lead to discrepancies in outcomes. Therefore, honing the ability to articulate these connections is vital in crafting successful recursive functions.
Examples of Recursive Formulas
Exploring recursive formulas surrounds not just their theoretical importance but also their practical applications in various fields. Recursive formulas allow students to express relationships between quantities in a streamlined manner. This can simplify complex problems, making calculations more efficient and intuitive.
Using concrete examples, like the Fibonacci sequence and factorial calculations, one brings the concept of recursion to life. These examples show how recursion can effectively break down larger problems into manageable parts, providing a clear path for solution development. Understanding these examples is crucial, as they lay the groundwork for applying recursion in programming and mathematical contexts.
Fibonacci Sequence
Defining the Fibonacci Recursive Formula
The Fibonacci recursive formula is foundational in many branches of mathematics and computer science. It defines each number in the sequence as the sum of the two preceding ones. Formally, this can be expressed as:
F(n) = F(n-1) + F(n-2),
with base cases F(0) = 0 and F(1) = 1.
This definition is elegant and highlights a key characteristic of the Fibonacci sequence: its simplicity. The efficiency of this representation allows one to see the recursive nature clearly. It is often chosen for educational examples because it models growth patterns observed in nature and is simple enough for students to understand.
Using this formula can have some disadvantages, especially in terms of computational efficiency. If implemented naively, it can lead to repeated calculations of the same values, causing performance slowdowns.
Implementation in Code
Translating the Fibonacci sequence into code showcases how recursion works in programming. Here is a simple Python implementation:
This code clearly illustrates the recursive process. It starts with the base cases and builds up from there. This implementation is beneficial because it closely follows the mathematical definition of the sequence. However, it suffers from the same inefficiencies faced in recursion, as it calculates Fibonacci numbers multiple times.
To optimize, one might consider memoization, an approach which stores the result of expensive function calls and returns the cached result when the same inputs occur again. This alteration can substantially reduce execution time while maintaining the recursive structure.
Factorial Calculation
Recursive Definition of Factorial
The recursive definition of factorial serves as another classic example of recursion. It defines the factorial of a non-negative integer n as follows:
n! = n Γ (n-1)!,
with the base case being 0! = 1.
This definition is both concise and powerful. The recursive nature highlights how factorials can be computed by breaking them down into simpler components. Understanding this concept can provide insight into more complex recursive processes.
The main strength of this definition lies in its clarity and direct process. However, with larger integers, one might encounter issues regarding stack overflow and computation time, as the recursion depth increases with large inputs.
Programming Implementation
The programming implementation of the factorial function showcases the algorithm's recursive nature in a clear manner. Below is an example in Python:
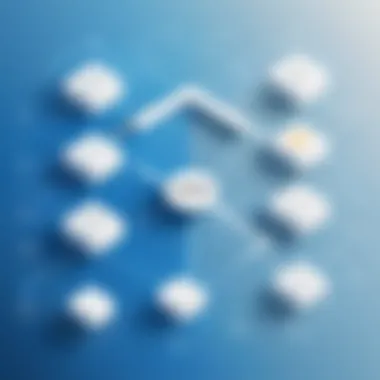
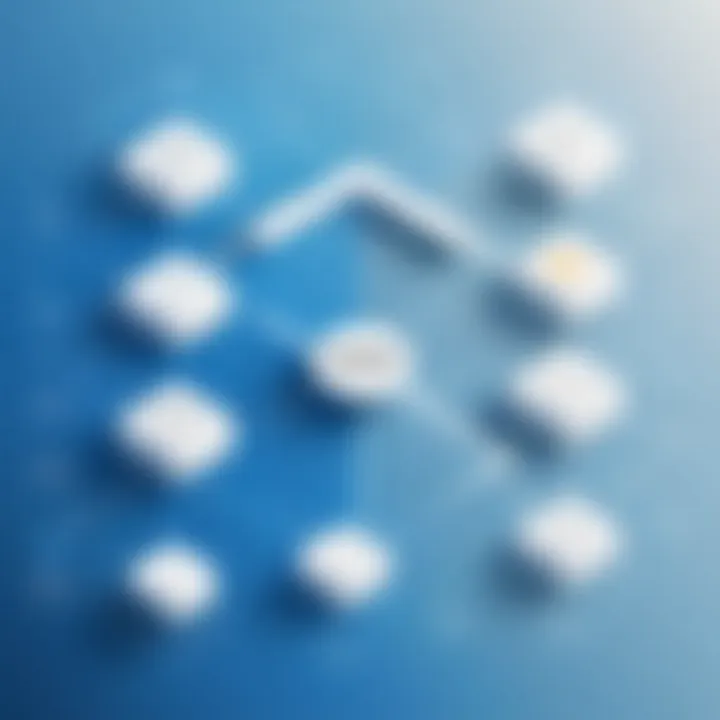
This simple code not only shows the recursive nature of factorials but also adheres to mathematical principles. It is a popular choice for illustrating recursive functions due to its straightforward logic. But, as with the Fibonacci implementation, deep recursion can lead to inefficiencies that may require optimization strategies like tail recursion to mitigate potential problems.
Common Pitfalls in Recursive Formulas
A well-crafted recursive formula is an essential component of effective problem solving in both programming and mathematics. However, the process of creating these formulas is rife with potential missteps. Understanding common pitfalls is crucial for anyone embarking on writing recursive definitions. This section will delve into specific aspects that often lead to issues and provide considerations that can help navigate these challenges.
By addressing the pitfalls related to infinite recursion and misunderstandings of base cases, readers will gain valuable insights on how to avoid these errors, leading to more reliable and efficient recursive formulas.
Infinite Recursion
Identifying Infinite Loops
Infinite recursion occurs when a recursive function continues to call itself without ever reaching a terminating condition. This issue can lead to system crashes or excessive memory use due to stack overflow. A key characteristic of infinite loops is their unbounded nature, which traps the program in a continuous cycle. Recognizing these loops is vital for debugging.
When constructing recursive formulas, careful definition of base cases is needed to prevent infinite recursion. The contributors to this issue can stem from incorrectly implemented conditions or missed conditions. A beneficial choice for addressing this pitfall is to develop test cases that simulate edge conditions to see if the function behaves as expected.
Preventative Measures
To prevent infinite recursion, a good practice is to clearly define the stopping condition in the recursive clause. This step involves ensuring that for each recursive call, progress is made toward reaching the base case. The unique feature of these preventative measures is their emphasis on structured design in formula creation.
The advantages include improved reliability of the recursive function, which makes it easier to maintain and debug in the long run. Constraints on inputs and thorough pre-condition checks can serve as safeguard against entering infinite loops.
Misunderstanding the Base Case
Consequences of Incorrect Base Cases
A misdefined base case can lead to unexpected behavior in recursive functions. When the base case is incorrect, the subsequent calculations may not terminate correctly. Key characteristic of this pitfall includes failure to provide a clear stopping point for the recursion, often resulting in infinite recursion discussed earlier.
Understanding the implications of incorrect base cases is a vital aspect of successfully navigating recursion, as these errors can cause cascading failures in algorithm logic.
Debugging Base Cases
Debugging base cases involves scrutinizing the initial conditions of a recursive formula to ensure they are accurate. This process is crucial, as an inaccurate base case will lead to incorrect results. Highlighting the importance of this practice stems from the foundational role that base cases play in the recursive process.
The unique feature of debugging base cases lies in systematically testing different segments of the recursion. Utilizing debugging tools and adding print statements can help trace the execution path, thereby revealing where the base case may have faltered. The advantage of this approach is that it not only clarifies the structure of the recursive function but also enhances overall problem-solving skills.
Optimizing Recursive Functions
Optimizing recursive functions is crucial for enhancing performance and efficiency. Recursive algorithms are elegant, but they can sometimes lead to excessive resource usage. Hence, understanding optimization techniques is vital for students and budding programmers.
One of the key issues with standard recursion is that it may involve overlapping subproblems. This can result in recalculating the same outcomes multiple times, leading to a performance bottleneck. By employing optimization strategies, programmers can avoid such redundancies and improve the execution speed of their algorithms. The two main techniques we will discuss here are memoization and tail recursion.
Memoization Techniques
Memoization is a powerful optimization method. This technique involves storing the results of expensive function calls and reusing the cached results when the same inputs occur again. In a recursive context, it dramatically cuts down on computation time.
When implementing memoization, the function will check if the result for a particular input is already computed. If it is, the function will return the cached result instead of executing all calculations again. This is particularly effective in scenarios such as calculating Fibonacci numbers.
Using memoization can transform an exponential time complexity to a linear one. The use of space to store results is largely outweighed by the gain in efficiency. While implementing memoization, it is essential to consider how to implement an effective cache that suits the specific problem.
Tail Recursion
Defining Tail Recursion
Tail recursion is a specialized form of recursion where the recursive call is the final action performed in the function. This characteristic allows some programming languages to optimize the recursion process, so that it can be transformed into an iterative process internally. The primary contribution of tail recursion is that it prevents stacking multiple frames in memory, hence avoiding potential stack overflow problems.
A key feature of tail recursion is simplicity. When a function is designed tail-recursively, it often results in code that is clearer and easier to reason about. However, not all programming languages support tail call optimization, so the benefits can vary.
Benefits of Tail Recursion
The benefits of tail recursion are significant, particularly in terms of performance and resource management. Since it avoids the accumulation of stack frames, tail recursion can efficiently handle very deep recursions without the risk of exceeding stack limits. This can be beneficial when the recursion depth is unpredictable or substantial.
Moreover, adapting algorithms to utilize tail recursion may make them faster. This is because the execution context may remain consistent instead of fluctuating between different states. Also, it may enhance the readability of the code. By focusing on the end result rather than intermediate states, tail-recursive functions often convey intent more clearly.
Programming does require some level of trade-offs, and understanding these optimizations is pivotal for developing robust algorithms.
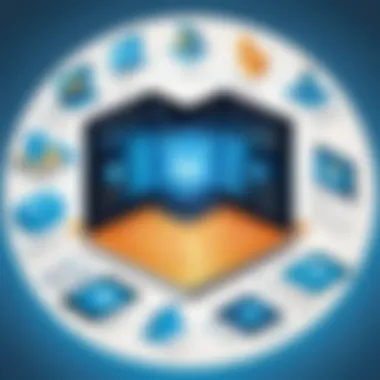
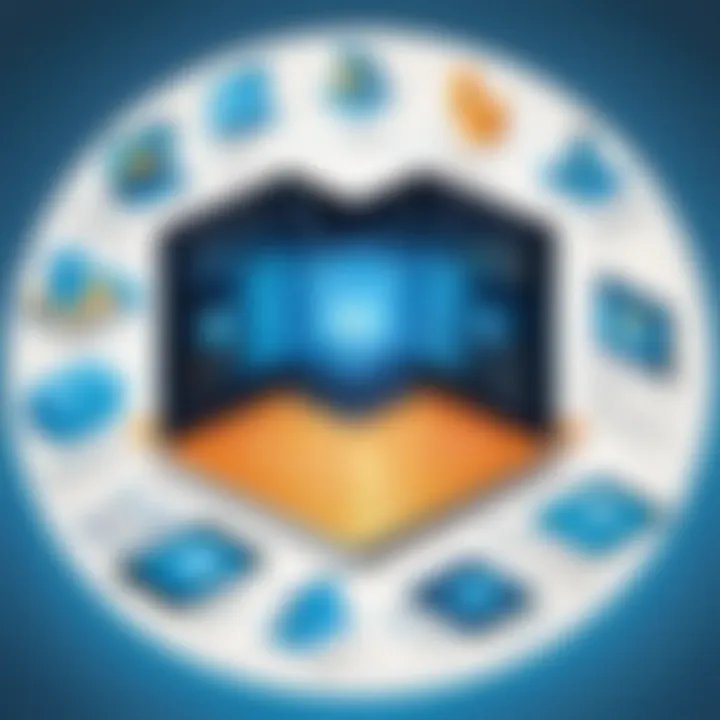
Applications of Recursive Formulas
Recursive formulas are not only powerful theoretical tools in mathematics, but they also have significant practical applications in the realms of computer science and programming. By allowing problems to be solved by breaking them down into simpler subproblems, recursion greatly enhances both algorithm efficiency and problem-solving capabilities.
When working with recursive formulas, several key benefits emerge, such as:
- Clarity and Simplicity: Recursive formulas often present clear and elegant solutions to complex problems. This format can make understanding the overall logic easier compared to iterative approaches.
- Efficiency in Problem Solving: Recursive approaches can eliminate the need for cumbersome loops, thus streamlining the coding process. This can lead to cleaner and more maintainable code.
- Natural Fit for Certain Problem Types: Some problems, such as those involving trees or fractals, lend themselves inherently to recursive solutions. In these cases, recursion seamlessly matches the problem structure, making it a suitable choice.
However, it is crucial to use recursion judiciously, considering both performance implications and the possibility of stack overflow errors in certain implementations. The balance between usability and efficiency must be maintained for recursion to be beneficial.
Recursive Algorithms in Sorting
Recursive algorithms are commonly used in sorting techniques, allowing for efficient data organization. Two prominent examples are Merge Sort and Quick Sort.
Merge Sort
Merge Sort is a classic example of a recursive sorting algorithm. It operates by dividing the array into smaller subarrays until each subarray consists of a single element. This division is followed by the merging step where all elements are combined back together in sorted order.
One key characteristic of Merge Sort is its divide and conquer strategy. It ensures that the worst-case time complexity remains consistent at O(n log n), making it a favorable option for large datasets. This reliability contributes to its popularity.
A unique feature of Merge Sort is its stability; when two elements are equal, their order is preserved. Stability is advantageous in many applications where the original order of equivalent elements matters, such as sorting records of the same value based on an additional criterion.
However, Merge Sort does have a disadvantage. It requires additional space for the temporary arrays used during the merging process, which can be a concern in memory-limited environments.
Quick Sort
Quick Sort is another recursive sorting algorithm known for its efficiency and performance. It selects a 'pivot' element and partitions the array into two subsets, one with elements less than the pivot and the other with elements greater than it. The recursive process then applies the same strategy on these subsets.
The key characteristic of Quick Sort is its average-case time complexity, which is often better than Merge Sort, clocking in at O(n log n). Quick Sort effectively handles larger datasets efficiently, making it a popular choice among programmers.
One unique feature of Quick Sort is its in-place sorting capability. This means it doesnβt require significant additional memory, which can be a significant advantage when working with large data arrays.
However, Quick Sort can suffer from poor performance if the pivot selection is not optimal, leading to O(nΒ²) complexity in the worst case. This vulnerability to performance degradation emphasizes the importance of good implementation practices.
Data Structure Traversal
Data structure traversal techniques rely heavily on recursion. These methods are essential for navigating complex structures like trees and graphs, yielding significant benefits.
Tree Traversal Techniques
Tree Traversal Techniques, including in-order, pre-order, and post-order traversal, are fundamentally recursive in nature. This allows for streamlined navigation through tree-like structures, making it easy to access and manipulate data.
The key characteristic of tree traversal techniques is their elegant handling of hierarchical data. Recursive methods can efficiently traverse and process each node without the need for additional data structures, which can simplify implementation.
The unique feature of recursive tree traversal is its ability to naturally reflect the structure of the data being represented. This alignment provides clarity and can reduce the likelihood of errors during coding.
One downside to consider is the potential for a stack overflow in deeply nested structures. Programmers should balance the depth of recursion with resource availability.
Graph Traversal Benefits
Graph Traversal is another application of recursion that allows for systematic exploration of nodes and edges. Common algorithms, such as Depth-First Search (DFS) and Breadth-First Search (BFS), utilize recursion to navigate through graphs effectively.
A key characteristic of graph traversal benefits is the comprehensive approach to exploring all reachable cycles and pathways within a graph. This allows for detailed analysis of graph structure.
The unique feature of recursion in graph traversal enables efficient pathfinding and connectivity checks. This is vital in various applications, from network routing to social network analysis.
However, similar to tree traversal, recursion in graph traversal can lead to performance issues with large graphs, particularly in cases with excessive node depth. Managing resources effectively is critical for success.
Understanding the applications of recursive formulas enriches oneβs programming toolkit. By exploring these examples, a solid foundation in both theory and practice can be laid, enhancing problem-solving skills.
End
The conclusion of this article serves as a vital summation of all previously discussed elements related to recursive formulas. Recursion is not simply an abstract concept confined to academia; it has practical applications in numerous fields, including mathematics and computer science, that extend beyond theoretical perspectives. Mastering this idea can enhance problem-solving skills and algorithm design competence.
Recap of Key Concepts
To aid retention, let us recap the key concepts covered:
- Definition of Recursion: We defined recursion as a method where the solution to a problem depends on solutions to smaller instances of the same problem. This builds a foundational understanding necessary for constructing recursive formulas.
- Base and Recursive Cases: Clear identification of both the base case and the recursive case is crucial. The base case provides a stopping point, while the recursive case demonstrates how to move between stages or levels of the recursion.
- Common Pitfalls: Awareness of issues like infinite recursion and misunderstanding base cases prepares readers to avoid prevalent errors in their implementations.
- Optimization Techniques: Learning about memoization and tail recursion exposes readers to strategies for enhancing the efficiency of recursive calls.
Future Learning Paths
- Explore Advanced Algorithms: Delve deeper into complex algorithms that utilize recursion, such as dynamic programming and divide-and-conquer strategies. These advanced techniques can further refine algorithmic thinking.
- Data Structures: Understanding how recursion interacts with different data structures can also be beneficial. For instance, tree and graph traversal offers insightful use-cases where recursion shines.
- Programming Languages: Study how various programming languages implement recursive features and optimizations. Languages like Python and JavaScript have unique approaches that can enhance your coding proficiency.
- Problem-Solving Platforms: Engage with online problem-solving platforms like LeetCode or HackerRank. These platforms provide a variety of problems that require recursion, allowing for practical application of learned concepts.