Mastering Selenium: A Comprehensive Guide for Automation
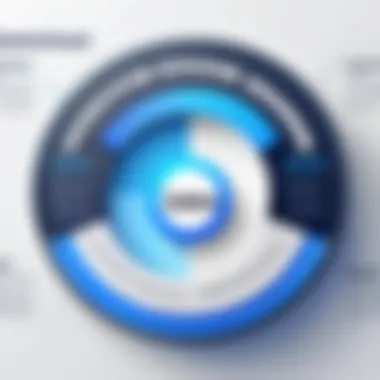
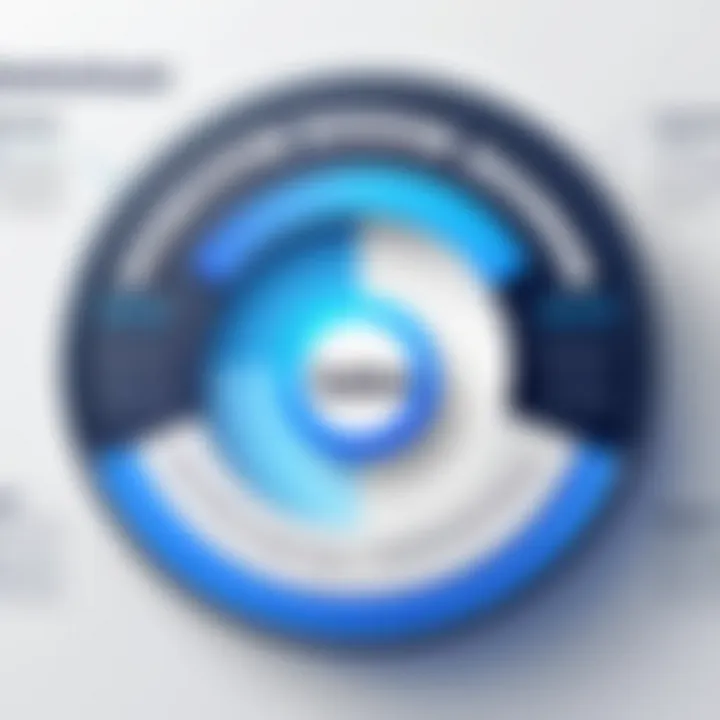
Intro
Selenium is a prominent tool designed for automating web applications for testing purposes. It is used globally by various developers and testers to simplify testing processes, enhance efficiency, and ensure applications function correctly across different browsers. This guide will address its core functionalities, installation, configurations, and various methodologies in depth.
Prologue to Selenium
Selenium started as a simple internal tool at ThoughtWorks in 2004, emerging as a response to the challenges faced in automated testing. Over the years, it has grown into a multifaceted framework comprised of multiple components. These include Selenium WebDriver, Selenium IDE, and Selenium Grid, each serving distinct yet overlapping purposes.
- Selenium WebDriver provides the means for direct control of web browser interactions, supporting various browsers such as Google Chrome, Mozilla Firefox, and Safari.
- Selenium IDE is a suitable tool for users wanting a no-cost entry point into automation, featuring a straightforward interface to record and playback scripts.
- Selenium Grid, on the other hand, facilitates the concurrent execution of tests in numerous browsers, enabling it to streamline workflows significantly.
Basic Syntax and Concepts
Understanding how to deploy Selenium necessitates familiarity with a programming language. Most users leverage languages like Python, Java, or C#. Below are essential concepts:
- Variables and Data Types: In programming, variables hold data values. For example, in Python, you could declare a variable like:
- Operators and Expressions: Selenium tests require logical expressions to dictate flow. Common operations include equality checks and Boolean evaluations.
- Control Structures: To create dynamic flow in tests, control structures such as , , or looping functions are essential.
Advanced Topics
Once comfortable with the basics, aspiring testers may choose to delve further into more advanced functionalities:
- Functions and Methods: To ensure code reusability and clarity. Defining functions can substantially improve the fluency of tests.
- Object-Oriented Programming: This programming style helps in organizing code. Utilizing classes to represent web elements can align code with user interactions.
- Exception Handling: A critical skill while using Selenium involves handling unexpected situations, such as element-not-found errors. Proper exception handling can protect against automation failings.
Hands-On Examples
Practical experience is essential in automating processes. Consider these typical examples to reinforce learning:
- Simple Programs: Initiating a web browser with a basic script.
- Intermediate Projects: Developing tests for a login functionality in a web application with user input
- Code Snippets: Offering pre-made pieces of code to perform specific actions like clicking buttons or filling out forms helps develop scripting skills.
Resources and Further Learning
To further develop skills in Selenium automation, consider these resources:
- Recommended Books and Tutorials: Look for titles like Selenium Testing Tools Cookbook or explore free online tutorials from platforms likeMozilla Developer Network.
- Online Courses and Platforms: Websites like Udemy and Coursera offer structured guides.
- Community Forums and Groups: Engaging on platforms like Reddit can provide valuable advice and peer support.
The integration of Selenium into regular development workflows focuses on enhancing automated testing and accelerating product releases.
Selenium serves as a crucial instrument in the field of web application testing. A clear and detailed understanding will equip readers with the necessary tools to apply Selenium in their respective endeavors efficiently.
Prelude to Selenium
In the context of web development and testing, Selenium has become an essential tool due to its versatile capabilities. Understanding Selenium provides significant advantages for automating user interactions with web applications, which proves efficient for both testing and scraping purposes. This section introduces the foundational aspects of Selenium, elaborating on relevant details about its definition, purpose, evolution, and its high impact on the automation landscape.
Definition and Purpose
Selenium can be defined as an open-source suite of tools prioritizing automation of web applications across various browsers and platforms. This open-source nature invites continuous improvement from contributors, which supports its reliability and user adoption. The purpose of Selenium extends particularly to testing—the chief use case, enabling developers and QA engineers to run automated test scripts effectively. Beyond testing, it also serves in web scraping, thus allowing users to optimize data collection from websites, offering a time-effective solution.
Evolution of Selenium
Selenium's journey began in 2004 when Jason Huggins created it at ThoughtWorks. Initially intended to solve simple web application testing issues, it quickly grew in utility and became a collaborative project to enhance its features. Several significant versions emerged sice the inception—Selenium 1, also known as Selenium RC, introduced remote control capabilities, and upon the release of Selenium 2, WebDriver was implemented, which greatly simplified the management of web elements.
Over the years Sonic has unveiled multiple iterations, enhancing performance and flexibility. Selenium Grid also wwas introduced, allowing simultaneous execution of tests across diverse environments, thereby significantly diminishing testing time. The progressive transformations in Selenium’s evolution reflect its adaptability to the changing web landscape and user requirements. Any proficient Web Developer or Tester should acknowledge these developments, advocating for the integration of Selenium’s utilities when dealing with modern web applications.
Components of Selenium
Understanding the components of Selenium is integral to leveraging its capabilities effectively for web automation and testing. Each component serves a unique purpose and caters to various needs in the automated testing framework. Knowing the benefits and functions of Selenium's different elements enhances a developer's skill and productivity.
Selenium WebDriver
Selenium WebDriver is one of the core components of Selenium. It enables the automation of web applications across various platforms and browsers. WebDriver interacts with web browsers dynamically by exposing a simple programming interface to facilitate the clicking of buttons, submitting forms, and navigating between pages. The significance of WebDriver lies in its versatility. It supports multiple programming languages including Java, C#, and Python, which allows developers to utilize the language they are most comfortable with.
In practical terms, developers appreciate WebDriver due to its simple API and the ability to write straightforward code to perform complex automation tasks. With support for multiple web drivers (like ChromeDriver for Google Chrome, GeckoDriver for Firefox, and Internet Explorer Driver Server), it enables easy browser switching and stringent testing across environments. This is essential in identifying browser-specific issues before a product goes live.
Learn the basics with a sample WebDriver setup:
Selenium IDE
Selenium IDE, or Integrated Development Environment, provides a user-friendly GUI (Graphical User Interface) that allows users to record and replay tests. One primary focus of Selenium IDE is to simplify the test creation process for those who may be less familiar with coding. Users can manually construct test cases easily by recording actions like clicking or typing, saving significant time while reducing the effort required for development.
While Selenium IDE is useful for beginners or for quickly prototyping tests, it comes with limitations. Its capability to handle complex test scenarios might be restricted when compared to other components like WebDriver. Nonetheless, it provides a stepping stone into the larger and more powerful Selenium ecosystem, making it easier to build foundational skills in test automation.
Selenium Grid
Selenium Grid facilitates the execution of tests in parallel across multiple machines and browsers. The need for parallel testing arises from the desire to minimize test execution time. With Selenium Grid, developers can scale their testing infrastructure and maximize efficiency, especially in continuous integration (CI) environments where speed and reliability are critical.
It involves a centralized hub that manages test scripts feeding across various remote nodes representing different browser configurations. This design minimizes bandwidth consumption while offering greater flexibility. To set up a grid, developers need to configure the hub and individual nodes to listen for requests before executing those tests.
Utilizing Selenium Grid is crucial when aiming for broader compatibility across multiple browser versions or operating systems. It demonstrates a proactive approach in determining how different environments will behave before deployment. Managing such setups can appear intimidating, but the trade-off of improved efficiency supports sustained development and innovation.
Keep in mind that knowing the difference between these techniques—WebDriver for scripting, the IDE for rapid prototyping, and Grid for parallel execution—is fundamental for leveraging the power of Selenium in the most effective manner.
Installation and Setup
The installation and setup of Selenium are crucial steps for anyone seeking to automate web applications efficiently. A proper installation ensures that the framework can interact seamlessly with different browsers. This step can significantly impact the performance and reliability of your automated tasks. Understanding various dependencies and configurations will be beneficial for anyone using Selenium for the first time.
Prerequisites
Before diving into the installation, certain prerequisites must be acknowledged. First, a stable internet connection is required, as Selenium and its dependencies are typically installed via downloads from the web. Additionally, a fundamental understanding of programming concepts will aid users in grasping the nuances of using Selenium.
Moreover, it is recommended to have one of the following programming languages set up: Java, Python, or C#, depending on your preference. Ensure that an Integrated Development Environment (IDE) is readily available, as it will facilitate coding and debugging processes. Ensure that the Java Development Kit (JDK) is installed, if using Java, or that the relevant package managers for Python or C# are properly configured.
Installation Process for Different Browsers
Installing Selenium involves different methods based on browser preferences. Below, we will discuss the installation processes for Chrome, Firefox, and Edge, emphasizing their individual characteristics and advantages.
Chrome
Google Chrome is a popular choice for web automation. Its consistent performance and extensive developer-friendly tools make it favorable for Selenium users. One of the key characteristics of Chrome is its support for the latest web standards, ensuring pages are rendered correctly during automated testing. Many users prefer Chrome due to its strong community and a plethora of extensions available to enhance productivity.
To install Selenium for Chrome, you must download the ChromeDriver that corresponds to the version of Chrome you have installed. This unique feature allows ChromeDriver to enable Selenium to control the Chrome Browser directly. A slight disadvantage is that updates to either Selenium or Chrome can necessitate regular updates to maintain compatibility, but this is a relatively minor inconvenience given its benefits.
Firefox
Mozilla Firefox is another favored browser, known for its privacy and developer-focused features. The key characteristic of Firefox is its open-source nature, which allows sustained community boosting across its use. In various testing scenarios, Firefox provides robust capabilities alongside Selenium.
For installation with Firefox, users will need to download the GeckoDriver. It allows Selenium to communicate with Firefox efficiently. The unique advantage of using Firefox lies in its performance with certain web applications. However, some users have reported inconsistencies in testing page elements which may require additional handling.
Edge
Microsoft Edge has emerged as a practical option for those within the Windows ecosystem. The familiarity many users have with Microsoft products can speed up the initial setup process. Key aspects of Edge include tighter integration with Windows, offering high performance on various web standards.
To effectively utilize Selenium with Edge, one should download the appropriate Edge WebDriver that matches their browser version. A unique feature of Edge provides enhanced security measures through improved compliance with enterprise policies, making this browser a solid pick for professional environments. However, some may find that it lacks the extensive developer tools available within Chrome or Firefox.
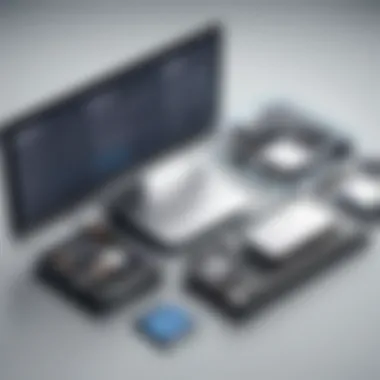
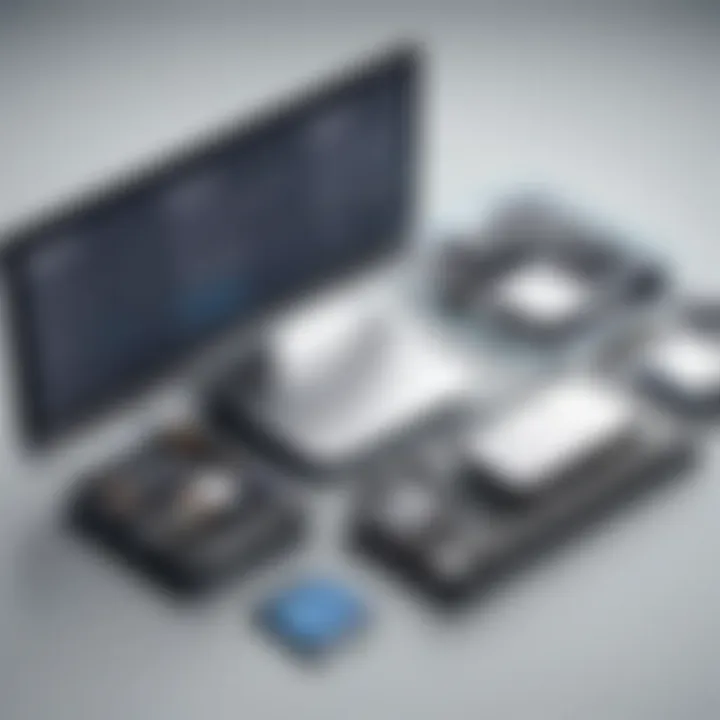
In summary, choosing the right browser for Selenium automation involves understanding the specific needs of your project. Each browser has its own strengths that can play a significant role in enhancing the efficiency of your automated tasks.
Basic Selenium Commands
Basic Selenium commands form the foundation of automating web interactions. Understanding these commands is crucial to leveraging Selenium’s capabilities effectively. This section aims to clarify these basic commands, such as launching a browser, navigating web pages, and interacting with page elements. By mastering these tasks, users can create robust automation scripts that interact with web elements efficiently.
Launching a Browser
Launching a browser is the very first step in utilizing Selenium. This command opens the specified browser session, allowing further interactions with web pages. By simulating user actions, Selenium can automate testing and site interaction more reliably than manual methods. This makes the launch command not just important but essential.
To launch a specific browser using Selenium WebDriver, users typically write code like this:
Navigating Web Pages
After the browser is launched, the next step is navigating to a specific URL. Proper navigation is vital for automation as it sets the context for the subsequent actions. Commands facilitate seamless transitions from page to page, mimicking actual user behavior. This structure allows tests to run smoothly across different environments, ensuring that applications work in various contexts.
Interacting with Page Elements
Interacting with page elements is where the capabilities of Selenium really shine. This set of commands enables users to manipulate the browser as a human would. It entails tasks such as finding elements, clicking buttons, and inputting text.
Finding Elements
Finding elements on a web page is a fundamental task in automated testing. Selenium allows users to select web elements by various criteria including ID, name, or class, depending on specific attributes. The flexibility in locating different elements makes this option particularly beneficial.
A key characteristic is the combination of multiple locators such as XPath and CSS Selectors. These provide precision when searches necessitate high accuracy. In scenarios that require complex interactions, using clear and efficient selectors allows for easier updates as website structures change. However, caution is necessary — over-reliance on fragile locators can lead to broken tests.
Clicking Buttons
Clicking buttons represents another core command that contributes significantly toward achieving user-like behaviors in automated scripts. This command typically uses the method on located elements. The ability to trigger actions like form submissions or navigation plays a vital role in how Selenium replicates user interactions on a web page.
A defining characteristic is the variety of methods to ensure that an element is clickable before performing an action. Using explicit waits to verify an element’s availability for interaction is often crucial. This increases the robustness of scripts and reduces the likelihood of test failures. Although powerful, if misused, it can lead to complications in terms of timing and performance during test execution.
Inputting Text
Inputting text into fields is a common aspect and can be performed using the command. This command enhances usability by allowing programmatic control over forms and data input processes. Its relevance is substantial, especially for tests dependent on examining form submissions or processed data on the web.
The unique feature that sets this command apart is its flexibility to receive various types of input, including keystrokes and special characters. This eases the testing process by emulating realistic user interactions. However, as different input types may require specific handling, it is essential to keep the context of usage in mind.
Asserting better control over your testing endeavors with these commands helps in executing comprehensive web automation. Efficient automation not only complements the testing process but enriches overall user experience during the life cycle of web developments.
Handling Dynamic Content
Handling dynamic content is crucial when using Selenium for automating web interactions. Many modern web applications utilize dynamic content, which refers to any data that changes without requiring a new page load. This agility creates challenges because elements may not be immediately available for interaction, causing tests to fail if they rely on static assumptions about element availability.
Automation not only benefits from understanding dynamic content; it thrives on it. Effective handling of dynamic components enables more accurate tests, leading to improved software quality. Selenium provides tools to address these challenges, ensuring seamless interactions within your automation scripts. Consequently, understanding effective waiting strategies is paramount.
Wait Strategies
Wait strategies are fundamental in handling dynamic content within web applications using Selenium. They help ensure that the automation script waits for specific elements to become available before taking action. This section discusses three primary wait strategies: Implicit Wait, Explicit Wait, and Fluent Wait.
Implicit Wait
Implicit Wait is a straightforward approach that tells the Selenium WebDriver to wait for a certain amount of time when trying to find elements before throwing a . This wait is set once, and its effect remains constant throughout the lifetime of the WebDriver instance.
Key Characteristic: This wait is easy to implement and applicable globally to every element applied. It sets a standard time for all elements, thus simplifying scripts.
Benefit: It is beneficial for quick test scenarios where scripts need not be overly complex, as it allows the WebDriver an allowance when waiting for an element to appear.
Unique Feature: The primary advantage of using Implicit Wait is its automatic fashion – all elements use this setting uniformly. However, its disadvantage lies in potential inefficiency; it can wait longer than necessary because it is a 'set and forget' option, possibly delaying the automation in response to swiftly loading pages or elements.
Explicit Wait
Explicit Wait offers more precision as it lets you wait for a specific condition to occur before proceeding further in the code. When using it, you define the waiting conditions with ExpectedConditions class. Examples can include waiting for visibility, clickability, or specific attribute checks.
Key Characteristic: Its tailored approach allows waits for specific elements under predefined conditions, enhancing control over automation.
Benefit: Explicit Wait is favorable because it allows tailored relationships between tests and actual page performance. For cases with precise timing needs, it helps ensure timely interactions with dynamic content.
Unique Feature: A notable advantage of using Explicit Wait is its intelligence; it keeps checking the condition until either the condition is met or the timeout occurs. One downside is, fewer sync issues can become more challenging to evaluate along less circuitously scripted tests.
Fluent Wait
Fluent Wait modifsawat to the needs of the specific automation task whereas still informs Selenium to wait for elements. You can set multiple conditions for continuance, define the polling interval, and even ignore specific types of exceptions while still waiting for certain elements.
Key Characteristic: This wait is partly a combination of Peruervmatic trends and coordination flexibility.
Benefit: The versatility here makes it a popular choice. Automaters can test a larger variety of scenarios successfully including some which emboss inherent variable characteristics in threatening performance.
Unique Feature: The distinctive edge of Fluent Wait lies within its dynamic struggle against the failed attempts. It checks continuously based on your defined poling delay intervals while it struggles against Highlighting difficulties or unique variances for interactive performance much better and more visually communicative. One practical concern includes the potential climate founduq against common motion efforts — capitalising less against slowly maturing behaviors.
Selenium for Testing
Selenium is a vital tool in the realm of software testing. Its significance lies in automating web test cases, thereby increasing efficiency and accuracy. By utilizing Selenium for testing, teams can execute repetitive tasks quickly, which supports frequent and extensive testing of web applications. This automation helps identify bugs early in the development process, contributing to the overall quality of the software.
Utilizing Selenium allows developers and testers to conduct functional and regression tests and even more complex scenarios like user interaction simulations. Numerous benefits come with using Selenium, including cross-browser compatibilty, thus ensuring inconsistency across different platforms. Another compelling reason for choosing Selenium is its support for various programming languages like Java, Python, and C#. This flexibility facilitates a broader adoption, making it accessible for teams with different tech stacks.
Creating Automated Tests
Creating automated tests with Selenium is fundamental in expediting the testing process. Tests can be scripted easily using simple commands to navigate through the application interface. One gets to write tests in the programming language of choice, which allows integrating Selenium tests into existing development workflows.
The process of creating an automated test typically involves these steps:
- Identify the test scenario that needs automation.
- Use Selenium WebDriver to write the script for the test.
- Execute the script in the required browser and observe the test result.
- Debug and refine the script as necessary for accuracy.
It can significantly reduce the time required for manual and repetitive testing tasks.
Test Frameworks Compatibility
When automating tests with Selenium, it is essential to consider compatibility with various test frameworks. Such frameworks provide structured testing and enhance the functionality of test cases. Several important test frameworks compatible with Selenium are JUnit, TestNG, and Pytest.
JUnit
JUnit is a popular testing framework primarily used for unit testing in Java. Its most significant advantage is its @Before and @After notation, which allows the code to run before and after each test case. This increases structure and injects adaptability for creating one-off tests. A key characteristic of JUnit is its simplicity in configuration, making it favorable for beginners.
JUnit facilitates better test organization, which is essential for a coherent testing strategy.
However, it tends to lack some advanced features, leading teams to seek more sophisticated frameworks for larger projects.
TestNG
TestNG is another framework that builds on JUnit's ancient structure while delivering more advanced features. Amongst its prime characteristics, is its support for data-driven testing and powerful parallel execution capabilities. This makes executing large test suites faster and utilizing resources efficiently. Due to its flexibility in annotations, TestNG is particularly appealing to testers looking for extensive functionalities in reporting and grouping tests.
Nonetheless, the learning curve for TestNG may present challenges, causing delays in transitioning from simpler frameworks.
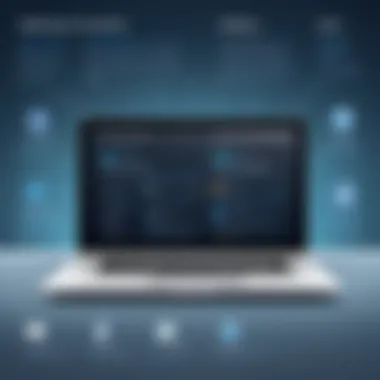
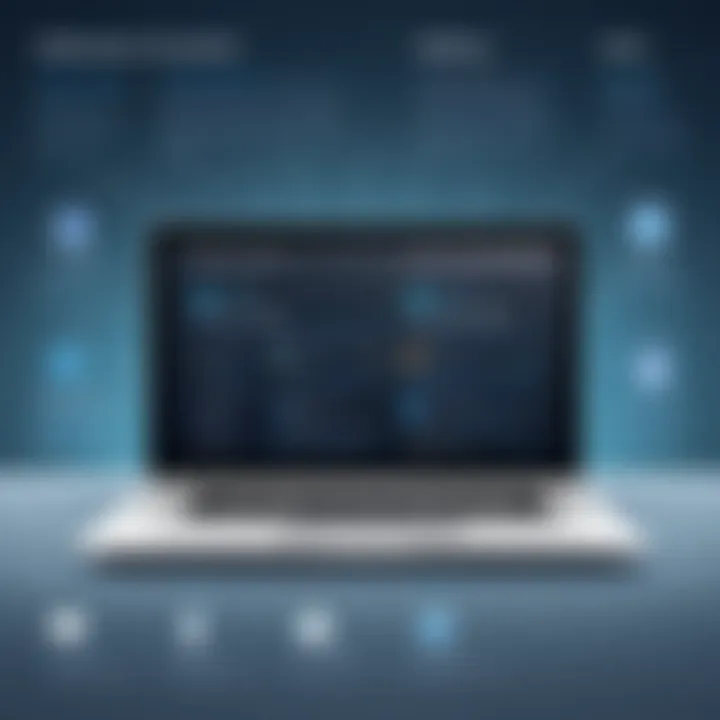
Pytest
Pytest is well-known for its simplicity and scalability. Built mainly for Python applications, it effectively handles simple unit tests and complex functional testing. Its keyword-driven and fixture implementation stands out, providing unique advantages for developing intricate test setups. Ease of writing concise test cases makes it a beneficial tool for both beginners and seasoned professionals.
On the flip side, Pytest may require additional configuration for its use with Selenium, raising barriers for some users.
Each framework invites its advantages and drawbacks. Choice should depend on team skills and project demands.
Best Practices in Selenium Usage
Using Selenium effectively involves adhering to some well-defined best practices. These practices assist developers and testers in building robust and efficient automation scripts, leading to improved maintainability and reduced errors. Following best practices can also enhance the readability of your code, making life easier for future contributors and collaborators.
Code Organization
Organizing your code is vital in Selenium scripting. Poorly structured code can lead to confusion and increased testing time. Consider using a consistent framework that separates the different layers of your automation process. By implementing modules or classes, you can encapsulate related functionality. For instance:
- Page Object Model (POM): This design pattern helps you separate the representation of your web pages. Each web page is represented by a single class containing its methods and elements. This encapsulation simplifies updates as changes in the UI need only be updated within the class responsible for that page.
- Maintain Integrity: Ensure that methods can be reused across tests. This reduces redundancy and makes it easier to manage the test suite. Functions like logging in, navigating to certain pages, or checking elements state should all be methods in your POM classes.
Organizing your code in this matter promotes scalability, as additional tests can be added with ease without needing to rewrite existing code.
Error Handling and Reporting
Error handling is another crucial aspect in Selenium. Scripts will frequently encounter unexpected situations. Handling these gracefully ensures robustness in your tests. Incorporate strategies using try-catch blocks to manage exceptions.
Key considerations for error handling:
- Visibility of Errors: Rather than allowing tests to fail quietly, employing proper logging helps you understand why a test fails. Use frameworks that offer clear error reporting mechanisms.
- Adding Assertions: Integrate assertions into your scripts to validate expected outcomes actively. For instance, if a web element does not exist when it should, raise an error to halt the execution immediately. This saves time realizing an error later.
- Reporting: Utilize reporting frameworks, which automatically document the results of your tests. Tools such as Allure or Extent Reports provide detailed summaries, facilitating easier understanding of what tests passed or failed and why.
Implementing consistent error handling can drastically improve the professional polish of your Selenium tests.
Debugging and Troubleshooting
Debugging and troubleshooting play a crucial role in the effective utilization of Selenium. Complex web applications often have dynamic behavior, leading to unpredictable elements that can disrupt automated tests. Proper debugging techniques are essential for identifying and resolving issues that arise during the testing process. By focusing on these practices, developers can ensure robust, dependable scripts that enhance the overall quality of their application.
Issues in Selenium can stem from various sources including incorrect configurations, unsupported browser versions, or code errors, among others. These stumbling blocks can hinder a tester's progress and complicate the development workflow. Recognizing common issues and mastering debugging techniques can significantly reduce downtime and increase productivity.
Common Issues
Selenium users often encounter a variety of challenges. Understanding these can justfiy quicker resolutions. Here are prevalent issues developers should be aware of:
- Element Not Found: Tests fail when elements specified in the script cannot be located on the web page. This issue might stem from changes in the application’s layout or incorrect locator strategies.
- Timeout Exceptions: Scripts may throw timeout exceptions due to elements being too slow to load. This is common with dynamic content or websites with heavy scripts.
- Stale Element Reference: This happens when the desired element has been changed or removed from the web page after it has been located initially. Scripts may error out as a result.
- Web Driver Issues: Compatibility problems between the Selenium WebDriver and the browser can also lead to failures. Proper installation of the WebDriver as per browser version is necessary.
- False Positives: Occasionally, Selenium may report that an operation completed successfully when it did not. This can lead to overlooking actual failures.
Being aware of these common issues primes developers to address them attentively and efficiently.
Debugging Techniques
Utilizing effective debugging techniques is vital for promptly solving issues. A few recommended methods are:
- Logs and Console Outputs: Selenium provides access to logs that can help in understanding what went wrong during execution. It is wise to incorporate logging methods in the scripts for deeper insights.
- Breakpoints: Incorporate breakpoints in your code to pause execution and inspect the state of variables or the runtime environment. This helps in understanding the flow and identifying failure points.
- Assertions: Assertions can help validate whether the expected outcome of a test matches the actual results. Conditional checks during runtime lead to quick identification of logic errors.
- Screenshots: Capture screenshots during execution failure. These images reveal the state of the application right before or during an error, helping clarify problems that need addressing.
- Debugger Tools: Adopting integrated Debugger tools within IDEs allows for a step-by-step execution of code, providing a high level of interaction to grasp every detail of what’s happening.
Address debugging as an ongoing practice. Engaging in regular code reviews may surface issues even before launching tests into execution. Regular updates to trials and continual improvement can bolster testing reliability.
Improving debugging practices along with a clear approach to troubleshooting offers profound benefits. Efficiency in this area may dramatically enhance the user experience and the automation framework overall.
Advanced Selenium Features
Advanced Selenium features are crucial for improving the efficiency and effectiveness of automated testing. These capabilities offer deeper interactions with web browsers, allowing developers to perform actions that enhance testing scenarios. Two essential components in this domain are cookies management and browser options and profiles. Understanding these topics unlocks better control and customization, which is vital in modern web application testing.
Working with Cookies
Cookies are small pieces of data that websites store on a user's computer. They are fundamental in maintaining a session state, managing user preferences, and tracking user behavior. In Selenium, working with cookies allows testers to simulate user actions that require persistent data.
By manipulating cookies, you can:
- Authenticate a user session: Login actions can be bypassed by directly setting the authentication cookie.
- Handle application states: Restore a specific state by loading cookies saved from a previous session.
- Test with various user profiles: Simulate scenarios where different users have distinct cookie-based preferences or setting.
Executing cookies management in Selenium is relatively straightforward. Below is a brief outline on how you can manage cookies using the Selenium API:
With this manipulation, you establish a more robust control over user sessions, ultimately leading to detailed and thorough testing processes.
Browser Options and Profiles
Bulk of the web applications can exhibit diverse behaviors across various browsers. Thus, leveraging browser options and profiles can considerably enhance automation schemes.
- Customizing browser settings allows for environment configurations suited to specific requirements. For example, you can:
- Profiles are quite useful to automate setups that would usually demand manual interference. Say, for example, needed plugins for certain tests can be pre-installed in a designated profile, eliminating setup times before each test iteration.
- Set up headless mode in browsers to execute tests without a graphical interface, saving resources for environments like CI/CD pipelines.
- Adjust the window size or display options to simulate various device environments.
This flexibility in browser options can distinguish a novice from a proficient test engineer. Such features significantly enhance the potential for writing quality tests that can run smoothly without manual offloading.
To achieve optimal performance and successful testing, integrating cookies and browser profiles is essential, reflecting consistent behavior across testing environments. Employ these features for better and more efficient web application automation.
Integrating Selenium with /
Integrating Selenium into Continuous Integration (CI) and Continuous Deployment (CD) processes is essential for maximizing the effectiveness of automated testing. This topic emphasizes the connection between testing automation and streamlined software delivery. By utilizing Selenium within CI/CD pipelines, teams can achieve faster development cycles and more reliable releases.
This section focuses specifically on how automation tools such as Jenkins and Travis CI can facilitate the seamless transition of test cases from development to production environments. Benefits of this integration include better testing coverage, earlier detection of bugs, and overall improved quality of software products.
It is crucial to consider how automated tests complement CI/CD practices. Test scripts run automatically in CI pipelines notify developers of issues early. Additionally, managing tests through these tools allows for reusability and faster execution, which is significant in agile environments. Effective execution requires setting up configurations wisely, ensuring that resource allocations meet the demands of testing workloads.
Continuous Integration Tools
To explore the integration of Selenium with CI/CD, we will focus on two prominently-used Continuous Integration tools: Jenkins and Travis CI.
Jenkins
Jenkins is an open-source automation server widely adopted by development teams for building, testing, and deploying software. One key characteristic of Jenkins is its extensive plugin ecosystem, which allows users to integrate Selenium testing easily. This versatility makes it a popular choice for automated testing environments.
A unique feature of Jenkins is its ability to manage job scheduling and track different versions of projects. The use of Jenkins supports not only continuous integration but also continuous delivery practices. One notable advantage of Jenkins is its community support and thorough documentation, providing users with ample resources to configure Selenium tests effectively.
However, there can be disadvantages as well. Jenkins requires regular maintenance and updates due to its complex nature, and setup can be demanding for newcomers. The requirement for server management may also be a consideration for teams without dedicated DevOps resources.
Travis
Travis CI is another tool that facilitates Continuous Integration and is particularly known for its simplicity and GitHub integration. Its key characteristic is that it provides easy setup right within project repositories. Users can deploy projects with just configuration files, skipping heavy setup processes that other tools may require.
An unique feature of Travis CI is its capability for parallel testing. This allows developers to execute Selenium tests in different environments simultaneously, significantly reducing overall test execution times. As a benefit, this parallel setup leads to increased confidence in the quality of the build being deployed.
On the downside, Travis CI's free plan can have limitations based on the rates of public repositories, which some teams may find restrictive and less flexible compared to other platforms. Not all configurations may be supported as extensively compared to specialized alternatives like Jenkins, which could hinder custom configurations that certain teams may need.
Integrating Selenium with tools like Jenkins and Travis CI embodies the attributes of modern software practices - automation, speed, and a commitment to quality.
Overall, selecting the best Continuous Integration tool greatly influences the success of Selenium test implementations in a CI/CD framework. Proper tool configuration allows teams to harness the full potential of automated browser testing, thereby enhancing development cycles and ensuring high-quality deliverables.
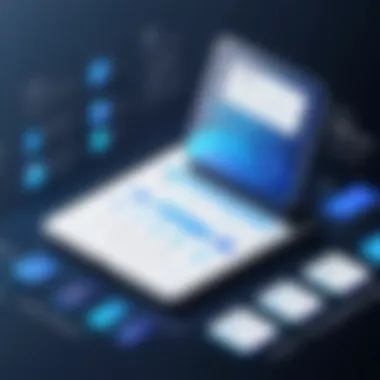
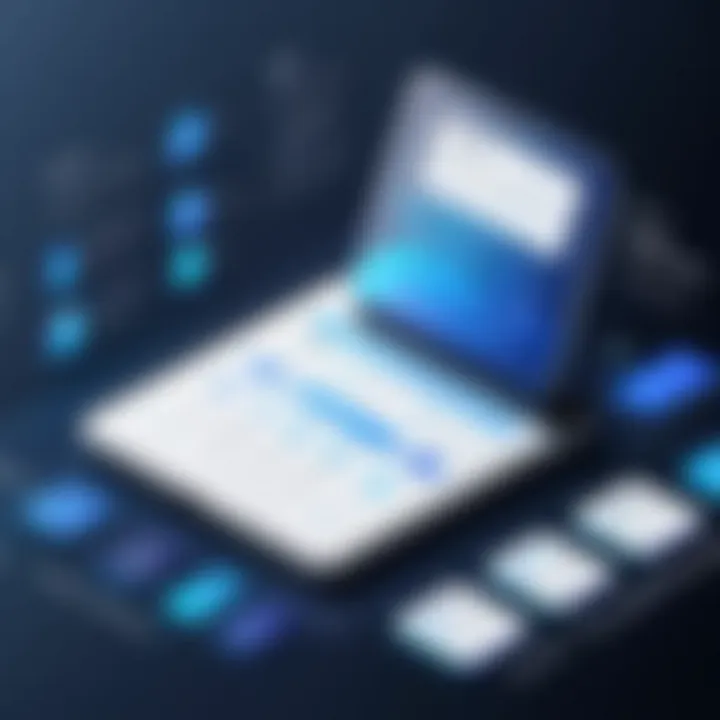
Selenium in Different Programming Languages
Selenium has become a pivotal tool for automating web applications, promising a standardized way to interact with web elements beyond just browsing capabilities. Understanding how Selenium works across different programming languages is important because it provides developers the ability to utilize pre-existing skill sets while engaging with their preferred environment. Attributes such as community support, various frameworks, and specific implementation peculiarities define how effectively Selenium can be integrated within projects.
Java API
Java is one of the most popular programming languages and a primary language for Selenium. Using the Java API allows developers to create effective automated tests for web applications. The rich ecosystem surrounding Java provides a plethora of libraries and tools that enhance the capabilities of Selenium. With Java, various testing frameworks like JUnit and TestNG can seamlessly integrate.
Some notable features of the Java Selenium bindings include:
- Strong community support helps in finding quick solutions to problems.
- An extensive repository of utilities and functions for enhanced functionality.
- Parallel test execution capabilities when combined with TestNG.
Code Example
To kick off, here is a fundamental example of how to launch a website using Selenium with the Java API:
Python API
Python's syntax is clean and its libraries, like , can indeed make test automation simple and effective. Python offers dynamic typing and rapid application development, making it popular especially among beginners in programming.
When using Python with Selenium, it benefits from a wealth of libraries meant for data manipulation, automated scripting, and its approachable syntax. Moreover, popular open-source frameworks such as pytest are easily integrated.
Advantages of Python API:
- Simple syntax which makes it easy for learners to grasp.
- Faster execution and production timelines lead to higher efficiency.
- Wide array of libraries for different needs like data processing or HTML parsing.
Code Example
Here's a simple Flask with Selenium example in Python that opens a web page:
API
The C# API opens up opportunities for those heavily invested in the .NET framework. C provides a statically typed environment and strong object-oriented capabilities that can facilitate the structuring of test automation projects largely.
With the need for .NET components in many enterprise-level applications, integrating Selenium in a C# environment can optimize workflows. Additionally, tools like NUnit enhance testing principles within C#.
Key notes regarding C# API:
- Leverages strong typing for improved error management during development.
- It enables autocompletion features in IDEs for faster coding.
- Benefits from tight integration with Microsoft's ecosystem allowing for comprehensive test automation solutions.
Code Example
An example of using Selenium with C# is as follows:
Closure
Understanding Selenium in different programming languages improves flexibility in choosing an appropriate testing stacked suited best for a project. Each language presents its distinct advantages and integration benefits. Therefore, mastering Selenium not just in a singular language format enables programmers to adapt to diverse project needs, increasing overall productivity.
Security and Selenium
In an increasingly interconnected world, understanding security in the context of Selenium is paramount. Automation tools like Selenium provide tremendous benefits for testing web applications. However, with these capabilities come various security risks that developers must navigate. Failing to address security concerns can lead to vulnerabilities that could expose sensitive information or compromise application integrity.
With effective use of Selenium, developers can create automated tests for web applications that help in identifying security flaws. Yet, it's crucial to recognize the specific elements of security related to automated testing processes. By being aware of security implications, developers can build robust automated solutions that respect privacy and remain secure. Below, we will look more closely at the risks and best practices to mitigate them.
Understanding Security Risks
When utilizing Selenium for test automation, several potential security risks arise. It's essential to be familiar with these to protect the applications you are testing
- Data Exposure: Automated scripts may collect information from web pages. If not handled correctly, this data can be exposed to unauthorized sources. Developers should ensure environmental isolation to minimize this risk.
- Insecure Dependencies: Sometimes, automated tests rely on third-party libraries. If any of these libraries contain malware or vulnerabilities, they can pose security risks to the application spying.
- Cross-Site Scripting (XSS): If a Selenium script interacts with dynamic content unvalidated properly, it could inject harmful scripts. Always validate inputs before passing them along.
In summary, the risks involved with Selenium automation primarily stem from data handling, dependencies, and scripts' interaction with web pages. Mitigating these risks involves implementing a secure mindset when developing test scripts.
Best Practices for Secure Automation
To effectively secure automated Selenium tests, developers can follow a range of practices:
- Limit Access to Data: Ensure that your automated test scripts run in environments with minimized access to sensitive data. This can involve configuring accounts with lower privilege levels.
- Use Secure Libraries: Regularly update dependencies and use trusted libraries. Regular updates are crucial as vulnerabilities in older versions can be a significant risk.
- Test the Security of the Tests: Employ penetration testing techniques against the test automation environment and modules each stage to identify and fix potential security issues.
- Secure Development Practices: Follow encryption protocols for sensitive information. For cookies, ensure that scripts manage them through secure configurations.
- Logging and Monitoring: Implement comprehensive logging mechanisms that audit automated interactions with web applications. Real-time monitoring can help identify anomalous behavior instantly.
- Stay Informed: Participate in forums and updated resources to keep abreast of the latest vulnerabilities and security patches. Checking in regularly on platforms like Reddit or Stack Overflow can be beneficial for user-generated advice and experiences.
By adhering to these best practices, developers can significantly reduce the risk associated with using Selenium, ensuring secure automation while effectively testing web applications and preserving data privacy.
Selenium Community and Resources
Selenium has fostered a vibrant community that can significantly aid developers in their automation efforts. Engaging with this community provides access to a wealth of knowledge, problem-solving resources, and valuable connections with professional peers and enthusiasts alike. Being part of such a network encourages a culture of collaboration and support that can enhance the skill set of both novice and experienced users.
Access to the Selenium community opens the door to various resources that can accelerate learning. Whether through online forums or official documentation, users can easily find answers to their queries and insights to improve their testing strategies. Participating in community discussions not only clarifies doubts but also builds confidence in using Selenium to its fullest. The inclusion of modern tools and techniques from other users can expand one’s ability to tackle diverse challenges that arise during testing tasks.
Online Forums and Groups
Online forums and groups play a crucial role in nurturing the Selenium community. Platforms like Reddit, Facebook, and dedicated testing forums facilitate interactive discussions about troubleshooting, best practices, and real-world applications.
- Tips and Tricks: Users often share their unique hacks and solutions to overcome common issues faced while using Selenium. This collective wisdom can shorten the learning curve and provide ideas that save time.
- Networking Opportunities: Interacting in forums allows developers to connect, which can lead to job opportunities, partnerships, or mentors that guide them on their journey in automation testing.
- Bootstrapping Projects: Many seasoned Selenium users contribute by posting open-source projects or tutorials which can serve as reference points for those who wish to start from a solid foundation.
Engaging actively in community-driven forums can lead to discovering concepts and approaches not covered in official resources.
Documentation and Guides
Comprehensive documentation is one of the key aspects of effectively utilizing Selenium. Official guides and documentation provide thorough insights into every element of the tool, facilitating a seamless learning experience. Thoughtful exploration of complete guides can highlight functionalities, declarative methods, and scalable implementations.
- Clarity and Reliability: Designed for user comprehension, Selenium’s documentation serves both as a foundational resource for beginners and a detailed reference for more advanced users.
- Up-to-Date Information: Official documentation is regularly updated, keeping users informed about changes, newly released features, and deprecated elements. The special attention to detail ensures that developers possess an accurate understanding of the tool.
- Examples and Tutorials: Each section of the documentation typically includes practical examples that illustrate various commands and their usage. Handling practical parts through guided examples promotes grounded learning while experimenting directly with Selenium.
In summary, the resources found within the Selenium community are invaluable assets for users looking to deepen their understanding and capabilities within web automation and testing. Taking advantage of forums, groups, and Selenim's documentation can profoundly shape one’s journey in mastering this robust framework.
Finale
The conclusion serves as a crucial component in this article. It provides a final summary of the insights discussed, encapsulating the essence of Selenium’s applications in a clear manner. By reflecting on the key topics, readers solidify their understanding and can assess how Selenium fits into their own projects.
In consolidating what they have learned, users can recognize the practical significance of Selenium. Incorporating automation in web applications enhances productivity in diverse environments like UI testing and web scraping. It allows developers to implement efficient testing as part of the development cycle. This reduces the time allocated for QA processes and allows faster product iterations.
When considering implementation, it’s vital to factor in particular aspects, such as the selection of environments and programming languages. Grasping how Selenium integrates with continuous integration tools elevates its utility further.
"Utilizing Selenium empowers teams to respond promptly to user feedback through quick enhancements."
In this concluding section, readers will begin to see Selenium not only as a tool but as a vital aspect of modern software development that leads towards a more adaptive development culture.
Summary of Key Points
- Selenium automates web application testing, ensuring thorough testing and early detection of bugs.
- Different components such as WebDriver, IDE, and Grid cater to various testing needs.
- Installation is straightforward across different browsers, making it accessible for diverse users.
- The article emphasizes on best practices for code organization and practical debugging techniques.
- Continuous integration compatibility with tools such as Jenkins enhances the efficacy of Selenium.
As a visitor returns to this article, the core objectives established from the beginning manifest in practical scenarios at the forefront of web automation and testing satisfaction.
Future of Selenium
The future of Selenium unfolds as an intriguing subject, attracting both developers and organizations alike. Undoubtedly, as web technologies evolve, Selenium must also grow to meet new challenges. A primary focus for future development could revolve around accommodating more complex web applications and ever-changing browser features. Automating sophisticated interactive interfaces may require constant updates and agility in framework design.
Furthermore, the sustainability of communities that support Selenium ensures a continuous stream of enhancements and innovative solutions. Collaboration within the community can lead to faster troubleshooting responses and shared solutions, which is invaluable.
There might be a noticeable inclination towards educational content and resources focused on simplifying use cases and boosting usability beyond programming expertise. Efforts on better documentation could improve adoption within the educational sector. The balance between maintaining robust technical features while offering approachable guidelines will solidify Selenium's position as the preferred automation framework.