How to Code in C: An In-Depth Guide for Programmers
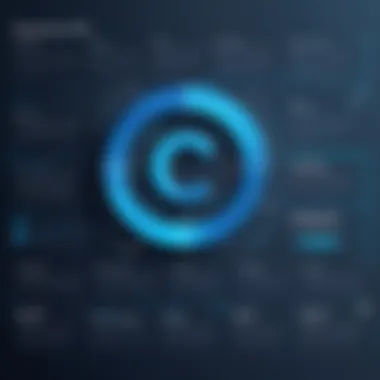
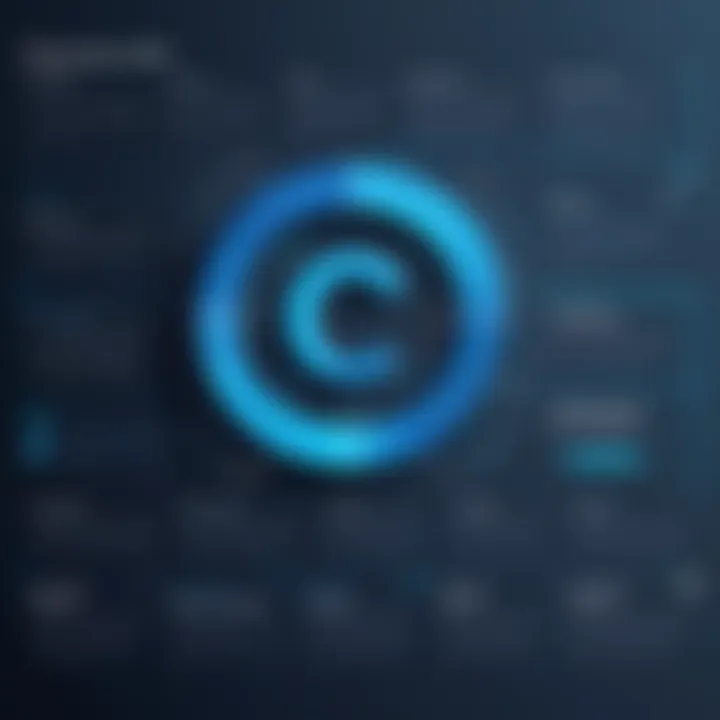
Intro
The programming language C stands as a significant milestone in the development of computer science. Created in the early 1970s, it has influenced many other languages and remains widely used today. Understanding the fundamentals of C can not only enhance programming skills but also offer insight into how software development operates under the hood. This guide aims to provide readers with structured learning covering both basic and advanced aspects of C programming.
Prologue to Programming Language
History and Background
C was developed by Dennis Ritchie at Bell Labs. It emerged from the need to write system software and applications. The language is closely tied to Unix operating system development, making it pivotal for many modern systems. Its simplicity and flexibility have made C a lasting choice for programmers.
Features and Uses
C is known for its efficient performance and close-to-hardware manipulation capabilities. Several key features make C appealing:
- Low-level access: C allows manipulation of bytes and memory, advantageous for system programming.
- Portability: Programs written in C can run on various platforms with minimal modifications.
- Rich set of operators: As a versatile language, it provides operators that enable fine data manipulation.
C is widely used in diverse applications, from operating systems to embedded systems. Industries such as telecommunications, automotive, and robotics extensively use C for its performance.
Popularity and Scope
The relevance of C remains substantial. Many universities still teach it as an introductory language. Its influence traces through languages like C++, Java, and Python. Understanding C enhances comprehension of these languages. The global demand for C developers continues to remain high, making it a valuable skill in the programming job market.
Basic Syntax and Concepts
Variables and Data Types
In C, variables must be declared before use. A variable is a symbolic name representing a value. Common data types include:
- int: For integers.
- float: For floating-point numbers.
- char: For single characters.
- double: For double-precision floating-point numbers.
Understanding these types is crucial as they determine how information is stored and manipulated in memory.
Operators and Expressions
C provides various operators for performing operations on data. Key operators include:
- Arithmetic operators: +, -, *, /.
- Relational operators: ==, !=, >, .
- Logical operators: &&, ||, !.
Expressions in C are combinations of variables, constants, and operators, producing a value.
Control Structures
Control structures dictate the flow of execution. They include:
- If statements: For conditional execution.
- For loops: For repeated execution.
- While loops: Conditional looping.
These structures facilitate decision-making and iteration in programming, which are vital for creating dynamic applications.
Advanced Topics
Functions and Methods
Functions are reusable blocks of code that perform specific tasks. They take inputs, execute the logic, and return outputs. This modular approach improves code organization and reuse. Example function declaration:
Object-Oriented Programming
While C is not inherently object-oriented, understanding the concepts can enhance code structure. Developers often employ techniques to simulate OOP through structures and pointers. This approach preparing programmers for languages that support OOP directly.
Exception Handling
C does not have built-in exception handling like some modern languages. Error management requires careful checking of function return values. Developers must validate input and maintain robust error-checking practices.
Hands-On Examples
Simple Programs
Starting with simple programs helps grasp the essentials. A basic program, such as printing "Hello World", illustrates syntax and structure:
Intermediate Projects
As skills progress, building simple calculators or text-based games provides practical experience. These projects often use conditional statements and loops.
Code Snippets
Code snippets can simplify tasks and improve efficiency. Regular use of snippets can increase coding proficiency and foster best practices in programming.
Resources and Further Learning
Recommended Books and Tutorials
Several books serve as excellent resources:
- "The C Programming Language" by Brian Kernighan and Dennis Ritchie.
- "C in a Nutshell" by Peter Prinz and Tony Larsen.
Online Courses and Platforms
Platforms like Coursera and edX offer courses on C programming. Engaging with structured online content can solidify knowledge and provide further insights.
Community Forums and Groups
Websites like Reddit have dedicated C programming communities where beginners and experts discuss ideas and share knowledge. Participating in such forums fosters learning and connection.
Utilizing available resources accelerates the learning curve and helps build a solid foundation in C programming.
Prelims to Programming
Understanding C programming is essential for anyone looking to delve into the field of computer science or software development. C is not just a language; it is a foundational stone upon which many other languages are built. Its influence is vast and significant, making it a critical subject in educational curricula and a valuable skill in the programming community.
C programming is known for its efficiency and control over system resources, which is why it is often used in systems programming, embedded systems, and the development of operating systems. Learning C equips programmers with a deep understanding of how computer science concepts work at a fundamental level. Here, we will explore its history, its relevance today, and its overall significance in the programming landscape.
History and Evolution of
C was developed in the early 1970s at Bell Labs by Dennis Ritchie. It evolved from a previous language called B, which was itself a descendant of the BCPL language. The main goal behind the design of C was to provide a simple, yet powerful tool for system programming. The first version of the C compiler was created in 1973, which allowed the Unix operating system to become more portable.
Over the years, C has undergone several revisions, notable among them the ANSI C standard in 1989 and later the ISO C standard in 1999. This evolution reflects the adaptability of C to new computing paradigms and hardware advancements while maintaining its core functionalities. Today, C serves as the backbone for various modern programming languages such as C++, C#, and Objective-C.
Significance of in Modern Programming
C continues to hold a significant position in modern programming for several reasons:
- Performance: C allows for close interaction with hardware, making it an excellent choice for programs that require high performance and low-level memory manipulation.
- Portability: Programs written in C can be compiled on various machines with little to no changes in code. This feature greatly enhances its usability.
- Foundation for Other Languages: Many high-level languages draw concepts and structure from C. This offers programmers a solid base if they choose to learn additional languages.
- Robust Libraries: C provides a wide array of libraries which are helpful in both scientific computing and general application development.
Through understanding C, programmers can better grasp essential programming concepts such as algorithms, memory management, and data structures. This deep dive into C programming empowers learners to tackle complex software projects.
"C programming is a critical skill for any aspiring computer scientist or software developer".
Setting Up the Development Environment
Setting up the development environment is a crucial step for anyone aiming to code in C. This phase lays the groundwork for all future programming activities. Selecting the right tools and platform not only enhances productivity but also improves the overall coding experience. A well-configured environment enables efficient debugging, ensures smooth compilation, and reduces the common frustrations that can arise from improper setup. Therefore, attention to detail in this stage is essential to establish a strong foundation for your programming journey.
Choosing a Suitable IDE
An Integrated Development Environment (IDE) plays a vital role in C programming. It provides a user-friendly interface that combines source code editor, build automation tools, and a debugger. By selecting the suitable IDE, you can significantly streamline your coding process.
Here are some popular IDEs to consider:
- Code::Blocks: An open-source option that offers a customizable interface and a wide range of plugins.
- Dev-C++: Lightweight and simple, it is an excellent choice for beginners who want to focus solely on coding.
- Eclipse CDT: A versatile IDE that supports many languages, including C, with robust features for advanced programmers.
- Visual Studio: Known for its powerful debugging tools, it is best suited for those seeking a professional development environment.
When choosing an IDE, consider factors such as ease of use, the availability of relevant plugins, and support for debugging tools. Compatibility with your operating system and personal development preferences will influence your choice as well. A good IDE ultimately allows you to configure and adapt settings according to your own workflow requirements.
Installing Necessary Tools
Once you have selected an IDE, the next step involves installing the necessary tools required for C programming. These typically include the C compiler, debugger, and any additional libraries that may support your coding activities.
The most commonly used compiler for C is GCC, which provides a powerful toolset for compiling and running your programs. To install it along with the IDE, follow these steps based on your operating system:
- Windows: You can use MinGW or MSYS2 to install GCC. They provide a straightforward installation process and command-line features.
- macOS: Install Xcode Command Line Tools, which includes GCC. Just run in your terminal to initiate.
- Linux: Most distributions come with GCC in their package manager. Use a command like to install it on Ubuntu.
Following installation, ensure that your IDE recognizes this compiler. Configure the IDE settings to point to the compiler paths correctly. This setup process is essential for seamless code execution and efficient problem-solving when errors arise.
"A wellconfigured development environment is the key to efficient coding and debugging."
This setup phase cannot be overlooked—spending the time ensuring that every component is correctly installed and configured paves the way for successful and enjoyable programming.
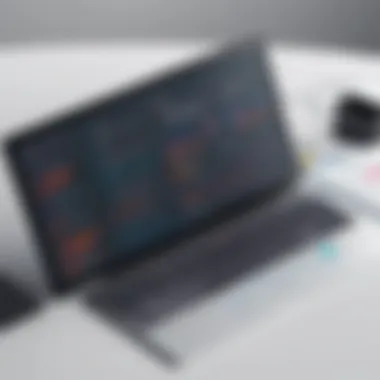
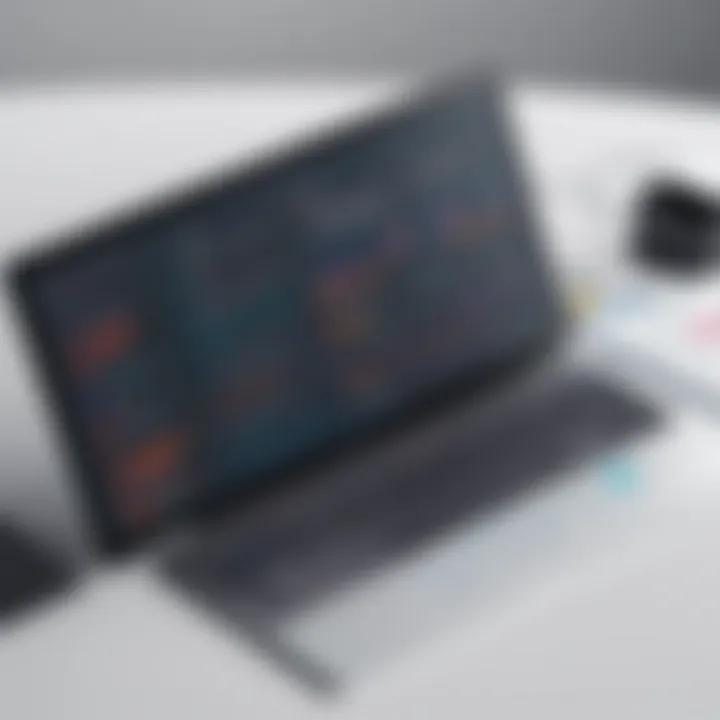
Basic Syntax and Structure
Understanding the basic syntax and structure of C programming is essential for learners at all levels. Syntax refers to the set of rules that define the combinations of symbols that are considered to be correctly structured programs in C. A solid grasp of this foundation enables programmers to write code that is not only functional but also understandable. This section addresses why syntax matters, its benefits, and important considerations to keep in mind as one navigates through C programming.
Understanding Syntax
C syntax comprises several elements that form the building blocks of any successful C program. Each statement in C is typically terminated by a semicolon, which serves as a clear indicator that a complete command has been given. Key elements include keywords, identifiers, literals, and operators.
Keywords are reserved words in C that have special meaning, such as , , and . They cannot be used as identifiers. An identifier is a name given to entities like variables and functions, forming the means to distinguish one from another. C also has literals, which represent fixed values like numbers or characters, and operators, used for performing operations on variables and values.
Beyond these fundamental elements, whitespace management is also a critical aspect of syntax. While the C compiler largely ignores extra spaces and newlines, using them effectively can significantly improve readability. Well-structured code allows for easier debugging and future modification.
Breaking Down a Program
When dissecting a C program, understanding its structure is paramount. A typical C program follows a systematic approach:
- Preprocessor Directives: These are lines that begin with a symbol, indicating to the compiler to include files or define constants before the actual compilation begins. For example, imports standard input-output functions.
- Main Function: Every C program must have one function, which serves as the entry point. This is where execution begins. The definition looks like:
- Variable Declarations: Variables must be declared before they are used. Here one specifies the data type, such as or , and then assigns a variable name.
- Statements and Expressions: After declaring variables, the program executes statements that perform actions, like calculations or outputting results. For example, prints a message to the screen.
- Return Statement: Most programs conclude with a statement, indicating the successful completion of the program.
By recognizing these sections and their purposes, beginners can develop their first programs more effectively and avoid common pitfalls. Understanding these components provides a strong base for future learning in C.
"A solid grasp of syntax leads to clearer logic and better problem-solving in coding."
When coding in C, adhering to these syntax rules and structuring a program logically is vital for creating code that is both effective and maintainable.
Data Types and Variables
Understanding data types and variables is fundamental in C programming. These concepts serve as the building blocks for any application you will create. Data types determine the kind of data that can be stored in a variable, while variables act as containers for these values. A deep comprehension of these elements is essential to write efficient and reliable code in C.
Selecting the correct data type impacts memory use, performance, and the overall behavior of your application. Wrong choices can lead to data corruption and unexpected results, increasing the complexity of debugging.
Primitive Data Types
C supports several primitive data types, which are categories of data that act as the core types for variables. The most commonly used primitive types in C are:
- int: This type is used for integers. The size can vary based on the compiler but is generally 4 bytes.
- float: A single-precision floating-point number, typically using 4 bytes.
- double: A double-precision floating-point, usually taking 8 bytes. It offers a larger range and more precision than float.
- char: This type holds a single character and generally takes 1 byte.
Understanding these types helps to write more efficient code. Choosing, for instance, a float instead of a double when high precision is not required can save memory and enhance performance.
Declaring and Initializing Variables
In C, declaring a variable is essential for stating its type before you use it. A variable declaration informs the compiler about the variable type and how much memory to allocate. Here's how you can declare a variable in C:
Initialising a variable means giving it an initial value. Common approaches involve assigning a value directly at declaration or using the assignment operator later. For example:
This initialization ensures that your variables have defined values when your program starts running. Not initialising a variable could lead to undefined behavior, which is something to avoid.
Constants in
Constants are variables whose values cannot be modified after their initial assignment. In C, the const keyword is used to declare a constant. This can help prevent accidental changes to variable values that should remain static.
Declaring constants looks like this:
Using constants in C offers several advantages, including:
- Clarity: Constants make your code easier to understand by indicating fixed values.
- Safety: They help reduce errors by ensuring that variable values do not change during execution.
- Maintainability: Changes to the value can be made in one place, simplifying updates to the code.
Using constants helps to improve code reliability and makes it easier for other programmers to comprehend your code logic.
Control Structures
Control structures are essential elements in C programming that dictate the flow of control in a program. They enable programmers to make decisions, execute repetitive tasks, and branch execution paths. Understanding these constructs is crucial for creating efficient and logical code. Without control structures, programs would operate sequentially, limiting their functionality and adaptability. Therefore, mastery of conditional statements, looping constructs, and the switch case statement is vital for both beginners and experienced programmers.
Conditional Statements
Conditional statements allow programmers to execute different code blocks based on certain conditions. The primary conditional statement in C is the statement, which evaluates a boolean expression and executes the corresponding block of code if the condition is true. An example of an if statement is as follows:
There are also and statements to handle alternative conditions and default scenarios. This way, conditional statements provide a straightforward method for implementing decision-making logic. They are powerful tools for handling user input and defining program behavior based on varying situations.
Looping Constructs
Looping constructs are vital for executing a block of code multiple times. The common types of loops in C are , , and .
- The loop is often used when the number of iterations is known beforehand. The syntax is as follows:
- The loop continues executing as long as a given condition remains true. Its structure is:
- The loop is similar to the while loop but ensures at least one execution of the code block, regardless of the condition:
These constructs allow for efficient code reuse and facilitate the processing of tasks that require iteration, such as traversing arrays or handling repetitive user inputs.
Switch Case Statement
The switch case statement offers a clean, concise way to execute different blocks of code based on the value of a variable. It functions as a multi-way branch, eliminating the need for multiple statements. The general syntax is:
Each corresponds to a potential match of the expression, and the statement prevents fall-through to subsequent cases. If none of the cases match, the code in the section will execute. This device is particularly useful for handling multiple conditions that relate to a single variable, ensuring the code remains organized and easy to read.
Functions in
Functions are a cornerstone of programming in C. They enable developers to write modular and reusable code. This modular nature leads to improved code maintainability and readability. In larger programs, where complexity can quickly spiral, functions provide a means to break down tasks into manageable parts. Each function can perform a specific task, allowing programmers to divide their work into smaller, more understandable segments.
Using functions offers several benefits. First, it promotes the DRY principle—"Don't Repeat Yourself". By encapsulating code in functions, programmers can avoid redundancy, leading to fewer bugs and easier modifications. Second, functions facilitate clearer organization of code, making it simpler to navigate through the main program. Lastly, they allow for easier testing and debugging since individual functions can be tested in isolation.
Defining and Calling Functions
In C, defining a function involves specifying its return type, name, and parameters, if any. The basic syntax is:
An example of a simple function definition might be:
This function, named , takes two integer parameters, and , and returns their sum. Note how the return type is specified before the function name.
To use this function within your program, you would call it by its name and pass the required arguments. For example:
This line calls the function, passing and , and stores the result in the variable .
Function Parameters and Return Types
Parameters in a function allow you to pass values into it, which can be utilized within its body. A function can have zero or more parameters. The type of each parameter must be declared. This makes it clear what kind of arguments the function expects. If a function does not return a value, its return type is specified as .
For instance, consider a function that calculates square:
In this case, the function has a single parameter and does not return a value.
When you define functions, ensure that the return type aligns with the value being returned. Many programmers often overlook this aspect, which can lead to undefined behavior in C.
Scope and Lifetime of Variables
The scope of a variable refers to the region of the program where it can be accessed. Variables defined within a function are local to that function. Once the function completes, these variables cease to exist. They cannot be accessed outside, which helps prevent unintended interference from other parts of the program.
Additionally, there are different storage classes in C that define both the scope and lifetime of variables. , , , and are common examples.
- : Default storage class. Local variables that are not static.
- : Retains its value even after the function exits.
- : Accesses variables declared outside of the function.
- : Suggests to place variable in a CPU register for faster access.
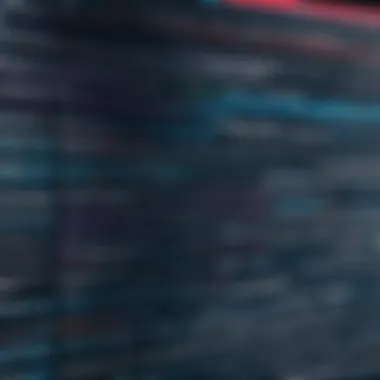
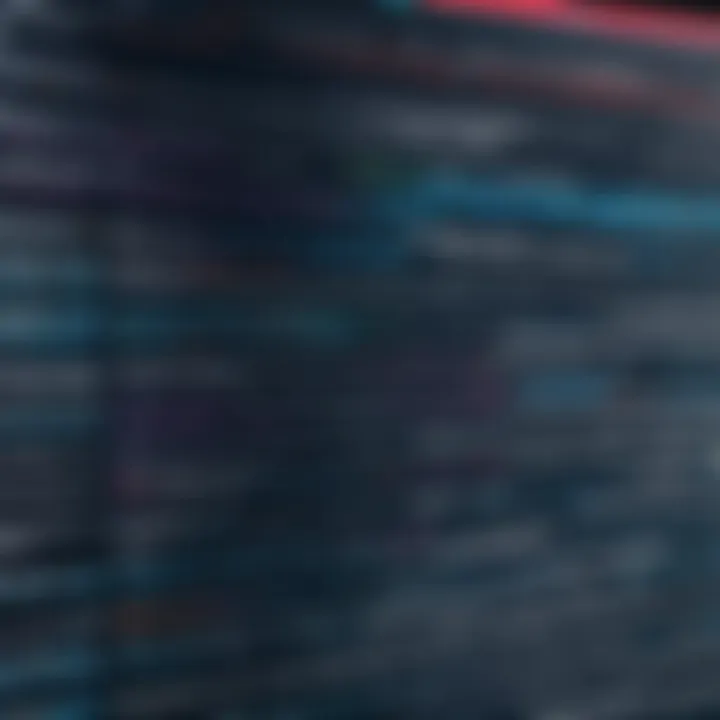
Understanding these concepts is vital when working with functions in C. Managing the scope and lifetime of variables effectively ensures your programs function correctly and efficiently.
"Functions in C programming are essential for creating clean and maintainable code, reinforcing the principle of reusability within your programming tasks."
Pointers and Memory Management
Memory management is a cornerstone of efficient programming. In C, pointers play a vital role in this process. Pointers are variables that store the address of another variable. They provide a powerful way of accessing and manipulating memory directly. Understanding how pointers work allows programmers to optimize performance by controlling memory use more effectively.
Pointers are essential for dynamic memory allocation. This concept enables allocation and deallocation of memory at runtime. This flexibility gives programmers the ability to manage memory without the constraints of static memory allocation. In contrast to languages with automatic memory management, C puts the responsibility on the developer to handle memory explicitly. This requires awareness of how memory is allocated, used, and freed.
Understanding Pointers
A pointer is a variable that points to a memory address of another variable in C. To declare a pointer, you use an asterisk (*) before the variable name. For example:
Here, is a pointer that can hold the address of an integer variable. To assign a memory address to a pointer, you use the operator, which retrieves the address of a variable. For example:
This assigns the address of to . You can access the value stored in a variable that the pointer points to by using the dereference operator (*):
Pointers enhance the efficiency of several processes, including function parameter passing and array manipulation. Passing parameters by address allows functions to modify the original variable without creating a copy. This results in less memory usage and faster execution times.
Dynamic Memory Allocation
Dynamic memory allocation enables the allocation of memory at runtime using functions like , , , and . For example, allocating memory for an integer dynamically would look like:
This line allocates memory for an array of five integers. The return value of is a pointer to the allocated memory, which you must cast to the appropriate type.
It is important to check if the allocation was successful:
Once you are done using dynamically allocated memory, it is crucial to free that memory using the function to prevent memory leaks.
Memory Leaks and Cleanup
Memory leaks occur when dynamically allocated memory is not properly deallocated. This can lead to increased memory usage over time, and if severe, it may cause the application to crash or slow down. To avoid memory leaks, every call to or related functions should be matched with a corresponding call to .
Always ensure to set a pointer to after freeing it:
This prevents accidental access to freed memory, which can lead to undefined behavior in your program.
Proper handling of pointers and memory is crucial for creating stable and efficient applications in C.
Arrays and Strings
Arrays and strings are fundamental concepts in C programming that play a crucial role in data management and manipulation. Understanding how to use arrays and strings is essential for writing efficient C code. Arrays provide a means to store multiple items of the same type in a structured manner, while strings, which are essentially arrays of characters, allow for text processing. Both of these features enable programmers to manage collections of data effectively.
One major benefit of using arrays is their ability to provide direct access to individual elements. This is achieved via indexing, making it easy to read and modify data. Strings are equally important as they facilitate operations like input and output of textual data, which is often required in software development. Knowing how to define and use arrays and strings enhances programming skills in C and provides a solid foundation for more complex data structures.
Defining and Using Arrays
In C, an array is defined as a collection of elements that are all of the same type. This allows for grouping related data under a single name, facilitating easier management. For instance, if you want to store the daily temperatures of a week, you can define an array of floats. The syntax for defining an array involves specifying the data type, followed by the array name and its size. Here is an example:
This code snippet creates an array that can hold seven floating-point numbers. Once defined, elements can be accessed using their index numbers, which start at 0. For example, to set the temperature of the first day, you would write:
Furthermore, you can initialize an array at the time of declaration, like this:
Using arrays in loops allows for efficient data processing. For example, you could calculate the average score from the array easily. Overall, arrays are a powerful tool for managing data in C, allowing for organized storage and efficient data manipulation.
String Manipulation in
Strings in C are sequences of characters terminated by a null character (). They are used for handling textual data. Manipulating strings is a common task in programming, and C provides several functions for this purpose.
To define a string, you would typically use an array of characters. For instance:
In this example, the string can hold up to 19 characters plus the null terminator. C offers a range of standard library functions to perform various string operations. Here are some commonly used functions:
- : This function returns the length of a string.
- : It copies one string to another.
- : This concatenates two strings.
- : It compares two strings.
Using these functions, string manipulation becomes straightforward. Utilizing to copy a string is done as follows:
Effective string handling enhances program functionality, particularly when dealing with user input or generating textual output. Therefore, a solid grasp of both defining arrays and manipulating strings is vital for any aspiring C programmer. Understanding these concepts not only improves coding proficiency but also prepares one for handling more complex data structures down the line.
Structures and Unions
In C programming, structures and unions are essential tools for developers. They allow grouping of different data types under a single identifier. This can simplify code management and enhance readability. Understanding how to define and use structures and unions is key for efficient memory use and data organization.
Structures enable developers to create a collection of related variables, often of different types. This capability is useful for defining entities that have multiple attributes. For example, a structure can encapsulate the properties of a student such as name, age, and grades. With structures, developers can handle complex data more intuitively.
Unions, on the other hand, provide a way to store different data types in the same memory location. A union can contain multiple members, but only one member can contain a value at any time. This feature makes unions lightweight and efficient in memory use, especially when the size of the data types is significant. However, developers must be careful with unions to avoid data corruption because accessing a member that has not been initialized can lead to undefined behavior.
In this section, we will define structures and explore their application, followed by a discussion on unions and their efficiency in memory management.
Defining Structures
Structures are defined using the keyword. To declare a structure, you follow this syntax:
For example, to define a structure:
Once defined, you can create structure variables:
This structure allows for clear handling of related data as a single entity. Using structures helps keep your code organized and makes it easier to manage multiple related variables. By logically grouping data, you increase the clarity of your intentions in the code and facilitate easier debugging.
Using Unions for Memory Efficiency
Unions are defined similarly to structures but use the keyword. The syntax follows:
For instance, if you define a union for a variable that can be either an integer or a float:
When you use this union, it requires enough space to store the largest member:
It’s important to note that when writing to a sample
File Handling in
File handling is a crucial aspect of programming in C. It allows programs to read and write data to files, enabling persistent storage of information beyond the program’s execution time. Understanding file operations is essential for tasks ranging from data logging to configuration management. Being proficient in file handling ensures that programs can interface with external data sources, handle large datasets, and perform necessary data maintenance effectively.
Not only does file handling increase the functionality of applications, but it also adds a layer of complexity that allows developers to create dynamic and interactive software. Knowing the correct methods for opening, reading, writing, and closing files is vital for managing file resources without causing memory leaks or data corruption.
Moreover, the C programming language provides several built-in functions for facilitating file management, which makes it relatively straightforward to implement. With proper file handling techniques, programmers can streamline data processing, enhance user experience, and build robust applications that meet real-world needs.
Opening and Closing Files
Opening and closing files are foundational operations in file handling. In C, files can be opened using the function, which establishes a connection between a file on disk and the program.
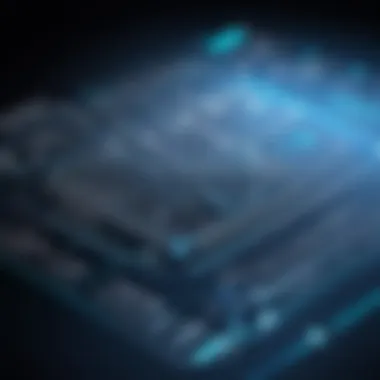
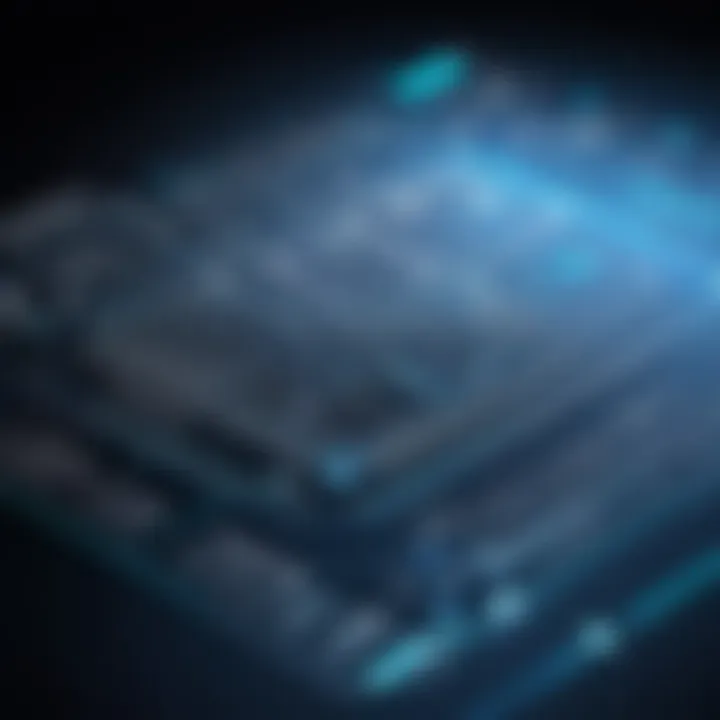
The first parameter is the name of the file, while the second defines the mode of access, such as read (), write (), or append (). It is crucial for programmers to check if the file was opened successfully by validating the returned pointer:
Closing the file is done using the function, which ensures that all buffers are flushed, and resources are released appropriately.
This step is essential to prevent memory leaks and maintain the integrity of the file system. Not closing a file can lead to undefined behavior, including data loss or corruption.
Reading from and Writing to Files
Reading from and writing to files is where the true power of file handling is showcased. In C, functions like for reading lines and for writing formatted output are commonly utilized.
To read from a file, the following example uses :
This code snippet reads each line from the opened file until the end is reached. Similarly, for writing data, the function offers flexibility to format data:
Using these functions, programmers can manipulate data efficiently. However, attention must be paid to error handling when reading from or writing to files. Ensuring that the operations are successful preserves data integrity and avoids runtime errors.
Remember: Always verify if the file operations succeed by checking the values returned by these functions. This practice helps maintain robust code.
In summary, mastering file handling in C is essential. It enables the development of applications that interact with user data effectively, ensuring that programs can execute beyond their initial scope. By properly opening, reading, writing, and closing files, developers can create more complex and useful software.
Error Handling and Debugging
Error handling and debugging are critical aspects of coding, particularly in C programming. With a robust understanding of these topics, developers can efficiently identify and rectify issues that arise during the software development process. This leads to stable and reliable applications, enhancing user experience and minimizing downtime.
The significance of effective error handling cannot be overstated. Errors can originate from various sources, such as invalid user inputs, unexpected system behavior, or logical flaws in the code. When developers handle these errors gracefully, they can maintain control over program execution and prevent abrupt terminations. This enhances the overall robustness of the software and provides users with a seamless experience.
Debugging, on the other hand, is the process of identifying and fixing bugs in the code. It is an essential skill for programmers. Debugging techniques allow developers to step through their code to observe operations in real-time, making it easier to understand where relations break down or specifications fail. Mastering these techniques, therefore, empowers programmers to improve their problem-solving skills and ultimately leads to cleaner, more efficient code.
Common Errors in
C programming is known for its rich feature set but also for its complexities. Consequently, various errors can occur. Understanding these common errors is crucial for anyone learning to code in C.
Here are some of the prevalent errors encountered in C:
- Syntax Errors: These occur when the code violates the language rules. Missing semicolons, typos, or incorrect use of brackets fall into this category.
- Runtime Errors: These manifest when the program is running. Examples include dereferencing null pointers or attempting to divide by zero where it leads to program termination.
- Logical Errors: These errors are not syntax-related but stem from incorrect logic. The program runs but provides incorrect results.
- Segmentation Fault: This occurs when the program tries to access a memory location that it is not allowed to, often leading to unexpected crashes.
A deep understanding of these errors helps developers anticipate problems and deal with them proactively.
Debugging Techniques
To rectify code issues effectively, programmers employ various debugging techniques. A few key techniques are:
- Print Debugging: The simplest method involves inserting print statements in the code to understand the program flow and variable values at certain points.
- Using Debuggers: Tools like GDB (GNU Debugger) allow developers to set breakpoints, step through code, and inspect memory. This facilitates more in-depth analysis of the code's behavior.
- Code Reviews: Often, a second set of eyes can identify errors overlooked by the original developer. Peer reviews can be an invaluable debugging technique.
- Unit Testing: Implementing tests for individual components of the program can ensure that each part operates as expected, catching errors early in the development process.
Through these debugging techniques, programmers can systematically address issues and improve the quality of their code.
"Debugging is twice as hard as writing the code in the first place. Therefore, if you write the code as cleverly as possible, you are, by definition, not smart enough to debug it." - Brian Kernighan
Advanced Programming Concepts
Understanding advanced C programming concepts is essential for any developer who aims to master the language. This section delves into specific techniques that can significantly enhance your coding capabilities. By grasping these concepts, programmers can write more efficient, cleaner, and more maintainable code. Let’s explore some key areas that will elevate your proficiency in C.
Using Macros and Preprocessor Directives
Macros and preprocessor directives play a crucial role in C programming. They allow developers to define constants and create reusable sections of code. The preprocessor handles these directives before compilation, making it possible to include headers and define macros that simplify programming tasks.
Using , you can create a macro for constant values. For instance:
Using throughout your code can make it more readable. Another important directive is , which allows you to manage external libraries. This capability enhances the flexibility of your programs and fosters code reuse.
Benefits of using macros include:
- Improved code readability.
- Simplified maintenance.
- Reduced risk of errors from hardcoded values.
However, it is essential to use them judiciously. Over-reliance on macros can lead to unclear code. Thus, knowing when to use them effectively is important.
Multithreading in
In the realm of modern programming, multithreading has become a vital component, especially for performance optimization. Multithreading allows your program to perform several operations simultaneously, which can significantly enhance application performance on multicore processors.
To utilize multithreading in C, you typically make use of the POSIX threads, known as pthreads. This library provides a rich set of functions for managing threads and synchronization between them. Here’s a simple example of creating a thread:
Key considerations when using multithreading include:
- Race conditions: Ensure shared variables are accessed safely.
- Deadlocks: Avoid situations where two or more threads are waiting for each other to release resources.
Understanding how to effectively implement multithreading can lead to significant advancements in program efficiency and responsiveness.
Understanding Standard Library
The C Standard Library is a powerful collection of functions that provides essential programming tools. It includes functions for handling input and output, memory allocation, string manipulation, and more. Leveraging this library can dramatically speed up development and reduce bugs.
Some core areas of the C Standard Library are:
- String handling: Functions such as , , and facilitate the manipulation of strings.
- Memory management: Functions like , , and handle dynamic memory efficiently.
- Mathematics: The library includes numerous mathematical functions, providing easy access to complex calculations.
The C Standard Library not only simplifies coding but also enhances code quality through well-tested and efficient implementations.
Learning to use the C Standard Library effectively is a fundamental skill in C programming. By familiarizing yourself with its various components, you can write concise and efficient code, allowing you to focus more on the problem at hand rather than low-level implementation details.
Best Practices in Coding
Best practices in C coding are critical for both novice and experienced programmers. They enhance code readability, maintainability, and efficiency. In a language where precise syntax and semantics are vital, adhering to best practices can significantly impact the quality of your code and the ease with which it can be understood or modified.
A well-structured C program can save time during debugging, learning, and collaboration. Moreover, as projects grow and evolve, the importance of following consistent coding conventions becomes even more pronounced. Below, we explore two critical aspects of best practices in C coding: writing readable code and code optimization techniques.
Writing Readable Code
Readable code is fundamental for collaborative environments. When others look at your code, clear structure and naming conventions can convey your intent quickly. Here are several guidelines that aid in achieving this goal:
- Use Descriptive Names: Variables, functions, and classes should have names that clearly describe their purpose. For example, instead of naming a variable , use .
- Consistent Indentation: Maintain consistent indentation, typically four spaces per indent level. This visually separates code blocks, making it easier to follow.
- Comment Generously: Place comments at key parts of the code, especially for complex logic. However, avoid stating the obvious, as this can clutter the code.
- Limit Function Length: Functions should be concise and purpose-oriented, performing a single task when possible. This aids future modifications and debugging.
- Organize Code Logically: Group related functions and variables together. Segment your code into distinct sections with clear headings if necessary.
Good readability prevents confusion and promotes better collaborative practices.
Code Optimization Techniques
Optimizing C code is essential for improving performance. Here are a few techniques that can be employed:
- Use of Efficient Algorithms: Analyze the time complexity of your algorithms. Consider using algorithms with better complexity to reduce overall execution time.
- Minimize Resource Usage: When possible, free memory after use to avoid memory leaks. This is particularly applicable when using dynamic memory allocation.
- Inline Functions: If certain functions are called frequently, consider using inline functions to reduce overhead.
- Profile Your Code: Utilize profiling tools to identify bottlenecks in your application. This helps you focus optimization efforts on the most critical areas of your code.
- Avoid Premature Optimization: While optimization is important, it's better to prioritize code readability and correctness first. Optimize only after ensuring that the code functions as intended.
A well-written C program is not merely a collection of commands; it is a carefully orchestrated arrangement of instructions that communicates clear intent.
Final Thoughts on Learning
Learning C programming can be a transformative journey for any aspiring programmer. It lays a solid foundation for understanding more complex languages and helps sharpen problem-solving skills. This article has delved into various aspects of C, emphasizing key principles that enhance both coding competence and confidence.
Importance of Learning
C is known for its performance and efficacy. While newer languages offer convenience, C remains relevant due to its close proximity to hardware and its influence on modern programming. Mastering C can deepen your understanding of low-level processes and memory management, aspects often abstracted away in higher-level languages.
Key Benefits of Learning C:
- Understanding Underlying Mechanics: In C, programmers deal with memory directly. This teaches essential concepts like pointers and memory allocation.
- Improved Problem Solving: Tackling C programming challenges enhances logical thinking and structured problem-solving approaches.
- Basis for Other Languages: Many languages like C++, Java, and Python have roots in C. A strong footing in C can ease transitions to these languages.
- Portable and Efficient Code: C produces efficient programs that can run on various platforms with minimal changes, making it a versatile language to learn.
"In programming, it is often the strong understanding of fundamentals that separates the proficient from the proficiently confused."
Moreover, C is often the first language taught in computer science programs due to its simplicity and relevance. This article has outlined essential coding practices and concepts ranging from control structures to advanced topics like multithreading.
Resources for Continued Learning
As you embark on or continue your journey with C, several resources can enhance your learning experience. Utilizing diverse materials can keep your studies engaging and provide a comprehensive grasp of coding in C. Here are a few recommended resources:
- Books:
- Online Courses:
- Forums and Communities:
- Online Documentation:
- The C Programming Language by Brian W. Kernighan and Dennis M. Ritchie - A classic textbook that covers the basics thoroughly.
- Head First C by David Griffiths and Dawn Griffiths - Offers a visually rich format that's great for beginners.
- Coursera - C for Everyone - A beginner-friendly course that covers the fundamentals.
- edX - Introduction to C Programming - A comprehensive introduction that includes hands-on coding exercises.
- Reddit's r/C_Programming - A place to ask questions and share insights about C programming.
- Stack Overflow - Get help with specific coding issues and browse existing solutions.
- C Programming Documentation) - An overview of C's features and standards.
- The GNU C Library Documentation - A detailed reference for C functions and libraries.
Exploring these resources will solidify your understanding of C and keep you updated on the latest developments in programming. Each step forward builds a robust programming skillset that can greatly benefit your future endeavors.