Mastering Holiday Management in Java Programming
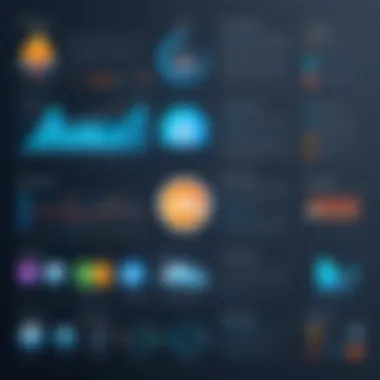
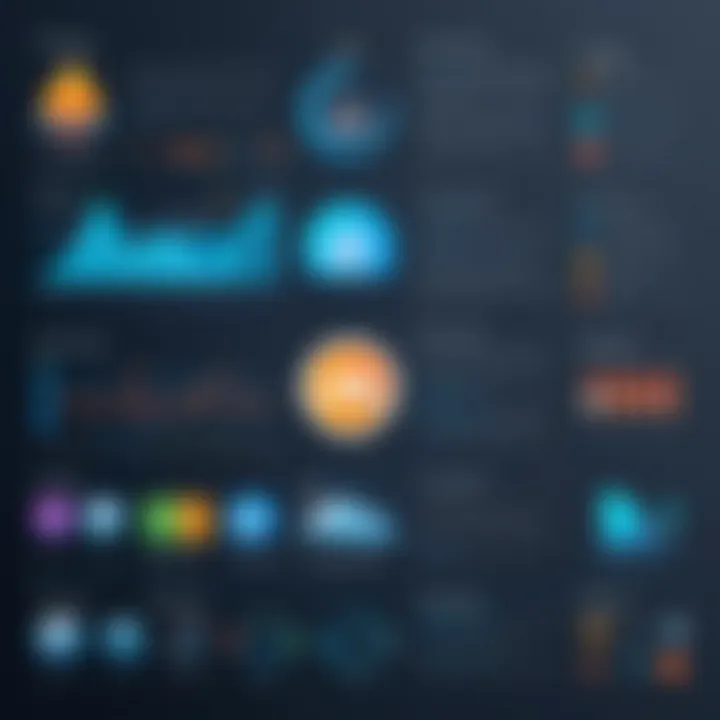
Intro
When one thinks about programming, the first things that come to mind are usually algorithms, data structures, and a flurry of code that seem like a foreign language. However, there’s another aspect that can greatly enrich your Java applications—holidays. Yes, understanding how to manage holidays within your application isn’t just a technical skill; it’s about infusing your software with cultural significance and user relevance.
As programmers, we dwell on the technicalities and logical structures, but let’s not forget that holidays can have a powerful influence on how software is integrated into people’s daily lives. Including holiday functionalities in your applications can enhance user experience, arbitrate meaningful interactions, and cater to the yearly rhythms that people celebrate.
The goal of this article is to shed light on how Java programmers can successfully implement holiday-related features. This includes exploring useful libraries, discussing coding strategies, and recognizing the cultural underpinnings that holidays carry. From novices just dipping their toes in programming to intermediate developers looking to level-up their game, there’s something here for everyone.
Let's take a leap into understanding the background and practical application of holidays in Java programming.
Prologue to Holidays in Java Programming
Understanding how to weave holidays into the fabric of Java applications isn’t just a nice touch; it's becoming essential in today’s diverse programming landscape. As developers, getting a grasp on how to manage holidays means acknowledging the real-world contexts in which our software operates. Whether you're developing an app for budgeting, event planning, or even social media scheduling, the importance of accommodating holidays can't be understated. They shape user behavior, affect business operations, and can often determine what features are critical.
Understanding Holidays in Context
Holidays vary widely across cultures and countries, creating a patchwork of observances that hold significance to different groups. For instance, in the United States, Thanksgiving is a major holiday that encourages travel and family gatherings, while in India, Diwali represents a festival of lights, bringing its own set of customs and celebrations. When programming, it's vital to embed these holidays meaningfully. This goes beyond simply marking dates on a calendar; it involves understanding the why behind these observances to create applications that resonate with users.
Consider how an application might manage bookings during major holidays. If you're not attuned to the dates that matter, you might end up with overbookings or inefficient services. For example, programming an application that simply ignores Christmas wouldn't sit well with users who rely on accurate scheduling.
Importance of Holidays in Software Development
Incorporating holiday functionality in software isn't just about practicality; it enhances user experience significantly. When users find an application that respects their cultural practices, they are more likely to engage and remain loyal. This can influence app ratings, increase downloads, or drive more sales. Therefore, holiday management fosters not just a functional necessity, but also builds rapport and trust with users.
Here are a few key benefits of integrating holiday awareness into your Java applications:
- Enhanced User Experience: Tailoring functionalities around holidays provides a sense of familiarity to the user. This means adapting notifications, reminders, and features to align with what users value in their cultural context.
- Increased Engagement: When users feel that the software acknowledges important dates in their lives, they are more likely to interact with it and recommend it to others.
- Reduced Errors: By programming applications to recognize holidays, you mitigate the risks of operational errors that often occur when overlooking these significant dates.
"Software that respects cultural nuances and incorporates key holidays can transform user interaction from merely functional to profoundly personal."
In summary, understanding and implementing the concept of holidays in Java programming is crucial for modern software development. It reflects a comprehension of societal elements that govern the rhythm of people’s lives, directly enhancing software relevance and user satisfaction.
Java's Date and Time API
In the realm of Java programming, managing dates and times can often feel like navigating a labyrinth. The Java Date and Time API serves as a crucial guiding light through this complexity. This API addresses several pain points that developers face in date manipulation, offering a modern approach to working with time. It not only simplifies handling dates but also enhances code readability and reduces errors associated with date/time operations.
One of the key benefits of the Java Date and Time API is its ability to handle time zones effectively. Unlike previous versions, this API gives developers the tools to manage global applications that must consider users in varied geographical locations. Moreover, it promotes immutability, which means once an instance of a date or time is created, it can't be altered, thus bolstering reliability. For developers focused on holiday programming, these features are indispensable, allowing for smooth integrations without losing track of time characteristics.
Overview of the Java Date and Time API
The Java Date and Time API, introduced in Java 8, consists primarily of the package. This package brings together several key classes, such as , , , and . Each class plays a specific role in handling different aspects of time management.
- LocalDate: Represents a date without time-zone information, perfect for use cases like calculating holidays that don't require a specific time of day.
- LocalTime: Focuses solely on time without date or time-zone information; useful for defining office hours or events.
- LocalDateTime: Combines both date and time, ideal for logging and timestamping events.
- ZonedDateTime: Encapsulates the full date-time with a time-zone, crucial for global applications handling multiple time zones.
These elements together allow programmers to choose the right type for their needs, making it easier to keep track of dates, especially in holiday applications.
Handling Dates and Times Effectively
When it comes to effectively managing dates and times in Java, it is essential to leverage the versatility provided by the Java Date and Time API. Here are a few strategies programmers can implement:
- Use of Builder Patterns: For creating date instances, the builder pattern can make code more readable and less error-prone. Instead of manually specifying each component of a date or time, builders allow for a more intuitive construction process.
- Avoid Use of Deprecated Classes: It's important to steer clear of the older and classes. Not only are they less intuitive, but they also introduce complexities that can lead to confusion.
- Parsing and Formatting: Utilizing the class allows for standardizing formats when working with user inputs for holidays. It’s a useful tool to convert between Strings and date/time types seamlessly.
Here's an example:
- Time Zone Awareness: When coding holiday functionalities, consider the varying public holidays depending on regions. The ability to convert dates and times with would help accommodate users from different locales, making your application more user-friendly.
Identifying Holidays
Identifying holidays is a crucial aspect of programming that often gets overlooked, yet it holds significant importance in various applications. Developers need to consider holidays not just as days off from work but as key elements that can affect user experience, system functionality, and overall project planning. In any software that involves scheduling, booking, or events, recognizing holidays directly influences functionality and user satisfaction.
Moreover, understanding and implementing holiday recognition can help in reducing errors in transaction processing around holiday periods. Consideration of holidays creates a well-rounded application that can adapt to the users' needs and local cultures.
Static vs. Dynamic Holiday Lists
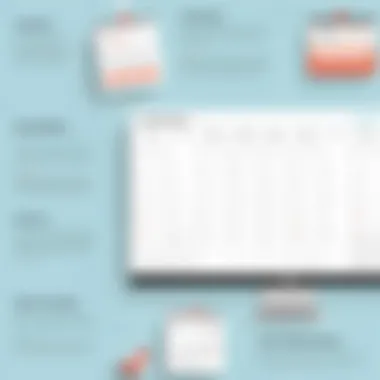
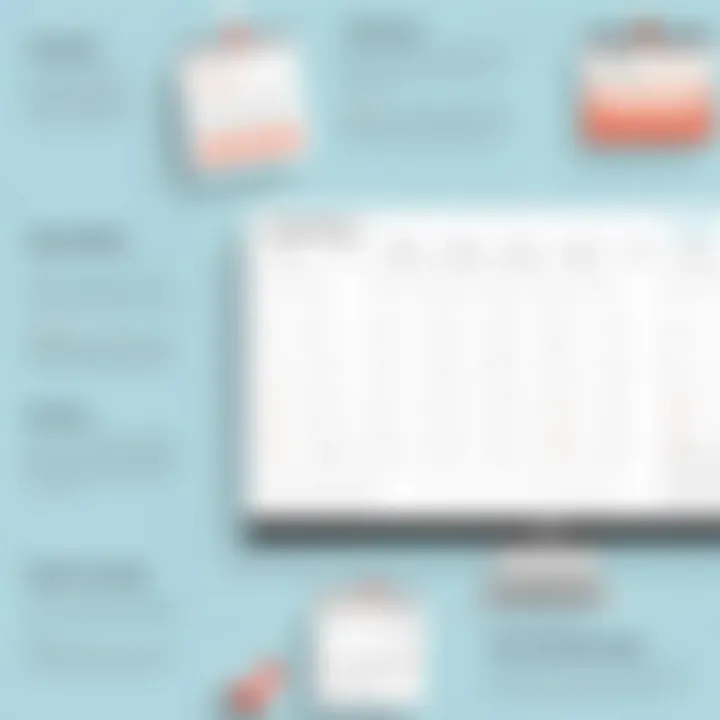
When it comes to managing holidays in programming, developers typically encounter two types of holiday lists: static and dynamic.
A static holiday list contains fixed dates and events that do not change year after year. For instance, Christmas, which is celebrated on December 25th, is always on the same date. This makes static lists relatively straightforward to implement as there is little that needs to be adjusted once they are set up. However, they lack flexibility to accommodate changes, such as regional observances or newly declared holidays.
On the other hand, a dynamic holiday list is more complicated but can cater to varying observances. For instance, Thanksgiving in the United States falls on the fourth Thursday of November each year, and it changes accordingly. Dynamic lists often require algorithms or external services to fetch the current data and reflect any changes. Adopting dynamic lists means that developers must ensure their application is robust enough to handle changes and updates effectively.
Here are key points to consider when choosing between static and dynamic:
- User Needs: If your target audience is specific to a region with fixed public holidays, a static list may suffice.
- Flexibility: Dynamic lists provide adaptability for growing applications that need to cater to a diverse user base.
- Maintenance: Static lists are easier to maintain, while dynamic lists may require APIs or data fetching processes.
Creating Holiday Calendars
Creating holiday calendars is a significant task for developers who want their applications to stay relevant in today's globalized world. A holiday calendar can serve many purposes: from managing employee leave to scheduling events, it provides the backbone that informs users about significant dates. Developing such a calendar involves several considerations.
- Customization: Users often have specific needs regarding the holidays they observe. Allowing customization helps cater to a wider audience.
- Localization: It's crucial to account for local holidays. For example, a user in the U.S. will need different holiday indicators compared to a user in India.
- Integration: Seamless integration with existing calendar APIs can be beneficial. For instance, the Google Calendar API provides a way to list and manage holidays efficiently.
- Visual Representation: A well-structured interface that visually represents holidays can enhance user engagement and understanding. Tables, color codes, or even simple graphical representations can go a long way.
By incorporating effective holiday calendars, programmers can enhance the functionality of their applications while addressing cultural sensitivities. In doing so, they ensure the software is not only technically sound but also socially aware.
"Holidays are not just days off; they represent culture, values, and the fabric of society that should be respected and integrated into our technologies."
Java Libraries for Holidays
When diving into the realm of holiday management in Java, we encounter a pivotal aspect that can significantly ease the coding burden — libraries. These libraries serve as ready-made tools, equipped with functionalities that let you handle various holiday-related tasks without reinventing the wheel. Using the right libraries can save you time, reduce errors, and help maintain clean code. Notably, Java boasts several libraries designed specifically for this purpose.
One of the primary considerations when selecting a library is its capability to handle both local and international holidays, which can vary significantly. This adaptability is crucial since holidays are not universal but are influenced by cultural, regional, and sometimes even historical factors. By leveraging these libraries, you can ensure that your application respects and recognizes diverse holidays, making it more user-friendly and relevant.
Using Joda-Time for Holiday Management
Joda-Time is revered in the Java community for its ability to simplify date and time handling. Unlike the built-in and , which can be cumbersome and error-prone, Joda-Time provides a more intuitive and fluent API. The library is particularly effective for managing holidays, thanks to its powerful date manipulation features and support for time zones.
Here’s how Joda-Time can be instrumental for holiday management:
- Flexibility: Joda-Time facilitates easy arithmetic on dates, so determining if a particular date is a holiday becomes straightforward.
- Localized Calendars: The library gives access to localized calendars, which can help in identifying holidays that vary from one region to another.
To exemplify:
This snippet provides a basic structure for a holiday checker, using Joda-Time's class.
Exploring Other Relevant Libraries
Aside from Joda-Time, there are other libraries worth considering for holiday management in Java. Each one brings unique features that can complement your project requirements:
- Java 8 Date and Time API: With the introduction of the package, Java 8 has its own sophisticated date and time handling. This package is great because it integrates seamlessly with the existing Java ecosystem, so you might not need to import additional libraries if JDK 8 or higher is being used.
- ICal4j: For those interested in working with iCalendar files, ICal4j is an excellent library to manage calendar data, including holidays. It supports creating, parsing, and generating ICalendar files, enabling integration with various applications.
- Holiday-Java: A tailored library explicitly created to hold holiday information for different countries. It's designed to be straightforward, enabling easy integration into existing applications.
Using libraries not only streamlines your development but also opens doors for integrating additional functionalities, such as reminders for holidays or displaying holiday-specific messages. As you explore these libraries, consider your specific requirements. Are you developing a global application? Or perhaps a localized one? This knowledge will guide your choice effectively.
Libraries are like a toolbox for the Java developer; having the right tools at your fingertips can make a world of difference in project outcomes.
Embracing these libraries can vastly enhance the capability and flexibility of holiday-related functionalities within your applications, making them not only efficient but also culturally attuned.
Implementation Techniques
Implementation techniques for handling holidays in Java programming are crucial for any developer looking to create robust applications. By understanding these practices, one can effectively manage date-related functionalities, enhancing both user experience and operational efficiency. The integration of holiday features can seem daunting at first, but grasping core concepts can simplify the approach significantly.
Building a Holiday Checker
A holiday checker serves as the backbone for verifying whether a given date falls on a public holiday. It’s especially useful in applications dealing with scheduling, planning, or any time-sensitive APIs. The primary benefit of having a dedicated holiday checker is to prevent conflicts and to enhance user satisfaction.
To build an effective holiday checker, a solid grasp of Java's Date and Time API is necessary. Here’s a basic outline of what the implementation might look like:
- Storing Holidays: You could create a simple list or use a map to maintain holiday data. This would typically involve using the LocalDate class for representing specific days.
- Checking Dates: The checker function would compare an input date against your list of holidays. Here’s a sample snippet:
- Custom Logic: Depending on user requirements, you might tailor the functionality further by including region-specific holidays.
This basic structure provides clarity on implementing a task that might seem overwhelmingly complex at first.
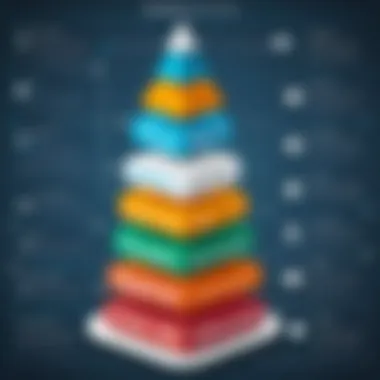
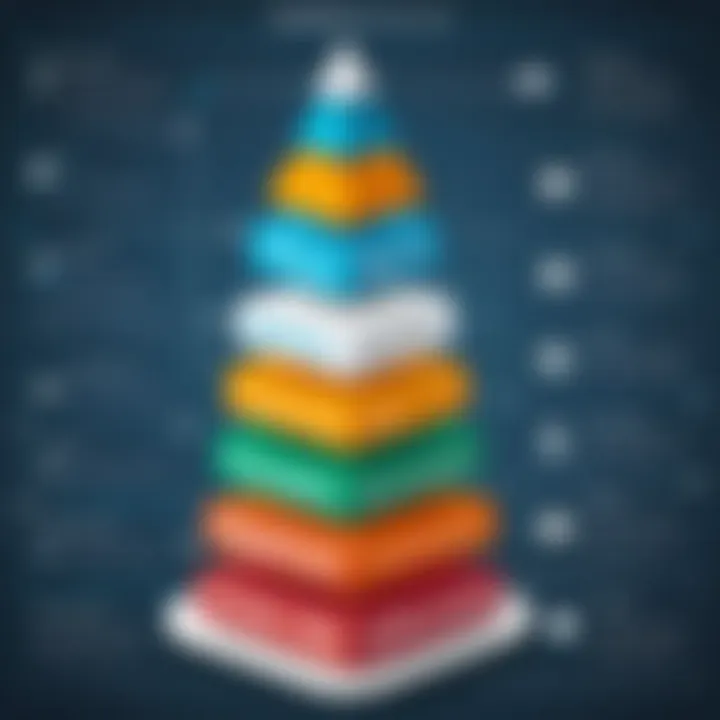
Integrating Holidays into Applications
Once you have the holiday checker in place, the next step involves integrating it within larger applications. This could be within a scheduling tool, for example. The idea here is to not only check for holidays but also to adapt the application's behavior accordingly.
Here’s where it gets interesting. Integrating holiday logic means your application needs to be aware of when users are planning their activities. How this aligns with holidays could change workflows dramatically.
- Event Scheduling: When creating events, incorporating the holiday checker ensures users are informed of holiday overlaps. Imagine booking a meeting on Christmas Day—nobody likes those last-minute notifications!
- Dynamic UI Feedback: If a user selects a holiday period, your application can dynamically suggest alternatives or warn against scheduling conflicts.
- Localization: When catering to a global audience, you might want to fetch holiday data based on the user's location. There are APIs available that can help with this by providing holiday details tailored to countries or regions. This promotes diversity and helps your software resonate with different cultures.
Implementing holiday features fosters a more inclusive and user-friendly design. As developers, it’s essential to be aware of these functional aspects, considering them not just as technical tasks but as opportunities to enhance user interaction.
User Input and Customization
In the realm of software development, tailoring applications to fit user needs can significantly enhance user experience. This is particularly true when it comes to holidays—an aspect that isn't merely a backdrop but an integral part of how individuals interact with technology. User Input and Customization allows programmers to create flexible applications that adapt to diverse cultural contexts and personal preferences. Acknowledging the fact that holidays are not one-size-fits-all is vital. Different users celebrate a broad spectrum of holidays, and enabling them to manage these through customizable options garners a greater sense of engagement.
Allowing User Preferences for Holidays
When building applications that consider holidays, allowing users to define their preferences is paramount. Imagine a global app where a user in the U.S. wants to mark Thanksgiving, while a user in India may prefer Diwali. Allowing for such customization adds value, making the software more relatable. By implementing a user preference module, developers can offer settings that include:
- Country Selection: Users can choose their country to auto-populate holiday lists relevant to their location.
- Personalized Reminders: Options for users to set reminders before holidays can greatly enhance usability.
- Adding Local Holidays: Users should have the right to add local holidays that may not be listed in the main database. This personal touch can foster a connection between the user and the application.
The challenge here is ensuring that the solution remains user-friendly yet comprehensive. A simple interface to add or remove holidays could streamline the process. If the settings become too complex, users could become frustrated rather than empowered.
Custom Holiday Definitions
Custom holiday definitions elevate personalization to another level. Having the ability to not only adjust the list of holidays but also define them opens a whole new world of possibilities. Users should be able to create holidays that reflect their cultural or organizational practices. For instance, an educational institution may observe Teacher's Day, which might not be a recognized holiday in many regions.
Key features to consider for custom holiday definitions include:
- Custom Naming: Users should be able to name their custom holidays, allowing for unique identifiers.
- Date Selection: An intuitive calendar selector can facilitate picking the date for these custom holidays.
- Recurring Options: Providing options for holidays that occur annually can save users from having to re-enter holidays every year.
Implementing these custom definitions serves two purposes: it promotes a sense of ownership over the application and allows for unique use cases that developers may not have considered.
By integrating user preferences and custom holiday definitions into Java applications, developers not only enhance functionality but also foster a sense of belonging among users—their holidays matter, and their voices can be heard.
Such a focus on user input ensures that software becomes more than just tools; they become a part of the user’s cultural tapestry. Understanding this aspect of development can help programmers create more meaningful applications.
Testing and Validation
When it comes to programming features related to holidays in Java, having robust testing mechanisms in place is not just a luxury—it's a necessity. Testing and validation ensure that your holiday functionalities operate as intended, thereby enhancing both user experience and system reliability. These processes help detect bugs, improper logic, and incorrect return values before your software gets into the hands of end users. In a diverse programming landscape, where various regions celebrate unique holidays, it’s important to validate that your application recognizes them accurately.
Key benefits of thorough testing and validation in the context of holiday-related functionalities include:
- Accuracy in Date Handling: Ensuring that your code accurately identifies holidays, accounting for regional variations.
- User Satisfaction: Reducing errors leads to a smoother experience, keeping users content and minimizing the need for updates.
- Flexibility and Scalability: A well-tested foundation allows for easier incorporation of new features as programming needs evolve.
Overall, incorporating best practices in testing and validation safeguards your code and builds trust among your application’s users.
Best Practices for Testing Holiday Functions
To keep your holiday functions in top shape, it's prudent to follow a few best practices for testing:
- Unit Testing: Write unit tests for every function that identifies or processes holiday data. This can often save you time down the road by ensuring each unit behaves as expected.
- Date Combinations: Test a combination of standard dates (like New Year's Day or Christmas) and less common holidays. This can reveal whether your function mishandles edge cases.
- Cultural Considerations: Factor in regional differences. For example, the import of holidays in the United States may differ substantially from those in Europe. Having a variety of test cases will help.
- Automated Testing Tools: Utilize tools like JUnit to facilitate your testing process. Automating your tests ensures they're run consistently, reducing the chance for human error.
- Regular Updates: As holidays may change or new observances may be added, keep your holiday functions up to date with regular testing intervals.
By applying these best practices, you set a solid foundation for error-free holiday functionalities.
Handling Errors and Edge Cases
Errors in holiday-related functionality can range from simple mistakes—like misidentifying the date of a holiday—to more complex issues that could lead to application crashes or incorrect data processing. It’s paramount to anticipate these types of errors and edge cases.
Start by identifying potential errors which might include:
- Incorrectly Configured Time Zones: A common pitfall is neglecting timezone variability; holidays celebrated at different times in different locations can throw a wrench in your program.
- Missing Holidays: As local or cultural holidays vary, ensure your application can handle inputs for regional observances that might not be hard-coded.
- Leap Years and Special Cases: Ensure your functionality works appropriately during leap years, particularly around dates like February 29th.
"If you don't test, it's like gambling with your code. You never know when it might fail."
To handle these errors:
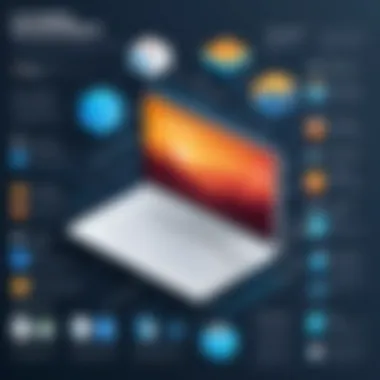
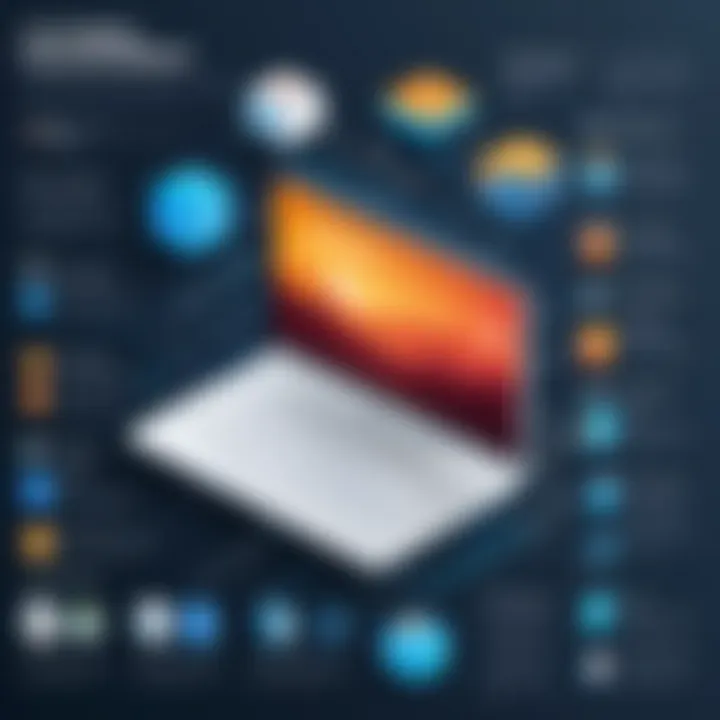
- Graceful Degradation: Instead of crashing, allow the application to fall back to a default function or a user-friendly error message.
- Logging and Monitoring: Keep logs of any inconsistencies or failures that users encounter, as this can guide future improvements.
- User Feedback Loops: Encourage users to report any issues they find, allowing for a continuous cycle of testing and improvement.
In the end, maintaining meticulous attention to handling errors and edge cases will not only save you headaches down the line but also ensure your holiday functionalities are reliable and user-focused.
Cultural Significance of Holidays in Software
In the realm of software development, holidays are more than just time off from work; they are moments steeped in significance that resonate across cultures and communities. Understanding the cultural significance of holidays in software is vital for developers aiming to create applications that are both functional and culturally sensitive. This consideration not only enhances user experience but also reflects a broader understanding of global diversity in programming practice.
Holidays often bring with them data that programmers need to account for in their applications. This includes special dates, traditions, and corresponding cultural practices that might vary from region to region. Ignoring these aspects can lead to user dissatisfaction and misunderstanding. Thus, a keen awareness of holidays impacts how software is constructed and how users engage with it.
Impact of Cultural Holidays in Programming
Cultural holidays influence programming decisions in numerous ways. For one, they dictate the flow of user interaction with software, especially applications relating to e-commerce, travel, or event planning. Consider how eagerly users expect to see festive themes or deal offers around holidays such as Christmas or Eid. Developers must adjust their systems to recognize these dates and adjust promotions accordingly.
Furthermore, there are crucial technical implementations that need to be made. For instance, recognizing when major holidays fall during the year can affect data processing, server load, and application responsiveness. Ensure you factor in public holidays while designing APIs that may need to return holiday-specific data. An example of this could be adding a filter to allow a user to check product availability based on local holidays.
- Holiday calendars become essential in these scenarios, as they help integrate cultural understanding into software design.
- It’s vital to take into account different time zones, especially for global applications.
"Ignoring cultural holidays is akin to programming in a void; it separates your application from the real world, which thrives on diverse experiences."
Embracing Diversity through Software
Embracing diversity through software goes beyond merely integrating holiday functionalities; it’s about honoring the unique practices and beliefs that different cultures bring to the table. This approach not only fosters inclusivity but also draws in a broader audience, as users are more inclined to engage with applications that recognize and celebrate their diverse backgrounds.
Diverse representation in software often leads to innovation in how technology serves various communities. For instance, incorporating features that allow users to select their cultural holidays can personalize user experiences. Such enhancements can include:
- Customizable holiday alerts that notify users of upcoming cultural events.
- The option to choose specific themes or designs that reflect the holiday in question.
- Features that allow users to share cultural content within the software, thus encouraging community building.
A nifty example can be found in social media platforms like Facebook, where users can interact through special holiday frames or stickers that echo their cultural celebrations. Such initiatives not only celebrate diversity but also highlight the programmer's dedication to understanding their user base.
Future Trends in Holiday Programming
The realm of holiday programming is rapidly evolving, with advancements in technology reshaping how developers approach the integration of holiday functionalities into Java applications. This section explores the significance of these trends, emphasizing how staying up-to-date can grant programmers a competitive edge.
As cultures intertwine and global travel becomes more commonplace, software developers must recognize the importance of accommodating a diverse array of holidays. Programs that respect and adapt to various cultural observances not only enhance user experience but also strengthen international business relations. In this landscape, understanding future trends in holiday programming is crucial. Here’s what developers should keep an eye on:
- Increased demand for user-centric applications that consider local and global holidays.
- The necessity for efficient data processing capabilities to manage dynamic holiday modifications.
- An emphasis on providing a seamless integration of holiday features within existing applications.
"In programming, keeping pace with trends can determine whether your application meets user expectations or falls flat."
AI and Machine Learning in Holiday Management
The incorporation of artificial intelligence and machine learning into holiday management introduces a game-changing element for developers. These technologies facilitate the creation of smart applications capable of adapting to new holiday-related data effortlessly. Imagine a Java application that learns user preferences for holiday activities over time, eventually suggesting personalized holiday calendars. This is not just a far-fetched idea, but a real possibility.
Developers can leverage machine learning algorithms to analyze patterns in holiday usage, enabling applications to make educated guesses about holidays likely to be important to users. Here’s how AI can enhance holiday management:
- Predictive Analytics: By analyzing historical data and user behavior, applications can anticipate upcoming holidays and notify users in advance.
- Dynamic Functionality: AI-driven features can allow users to customize their holiday experiences based on cultural relevance or personal preferences.
- Data Integration: Automated data collection from various sources helps in keeping the holiday information fresh and up-to-date.
The Role of APIs in Holiday Integration
With the proliferation of applications requiring holiday functionalities, the role of APIs becomes paramount. APIs—Application Programming Interfaces—act as bridges, enabling different software systems to communicate and share data. For holiday programming, APIs can streamline the integration of holiday information with applications without the burden of manual updates. Here’s a closer look at their significance:
- Seamless Data Access: APIs can provide access to centralized holiday databases, ensuring that applications always have the latest holiday data.
- Customization and Flexibility: Developers can create tailored experiences for users by using APIs to fetch region-specific holiday data, adapting the application accordingly.
- Community Insights: Integrating community-driven platforms where users share their holiday experiences or suggestions can enhance the application's relevance.
Using APIs effectively can make a world of difference in how holiday data is managed in Java applications, transforming static holiday lists into dynamic and interactive features that truly resonate with users.
Closure
In wrapping up our exploration of holidays in Java programming, it becomes clear that the integration of holidays into software systems is not merely a technical endeavor but also a reflection of cultural awareness and user experience. Understanding how to manage holiday functionalities can significantly enhance the relevance and usability of applications. As we have seen, effectively recognizing holidays allows developers to accommodate varying user needs and expectations, which ultimately leads to a more engaged user base.
Synthesizing Knowledge on Holidays in Java
Throughout the article, we have discussed various aspects of handling holidays within Java applications. Key points include the importance of choosing between static and dynamic holiday lists, harnessing libraries like Joda-Time, and best practices for testing holiday functions. These insights are vital for ensuring that your application behaves intelligently and respectfully throughout different festive periods.
By synthesizing this knowledge, developers not only streamline their coding practices but enhance the broader software landscape. When you create applications that recognize and celebrate holidays, you contribute to a more inclusive tech culture. This is not just 'nice to have'—it can be vital for user satisfaction and engagement.
Encouraging Further Exploration
Given the complexities and cultural significance surrounding holidays, there is much more to delve into. Think about exploring APIs that provide holiday data for various regions. Such resources can expand the linguistic and cultural reach of your applications, leading you to think outside the box in your programming journey.
Additionally, consider collaborating with other developers to share insights or even contribute to open-source projects focused on holiday functionalities. Engaging in community discussions, perhaps on platforms like Reddit or specialized programming forums, can further foster your knowledge and understanding of this niche in software development.
As a closing note, whether you're a novice or someone more seasoned in Java, the horizon is broad and promising. Don't hesitate to dig deeper into the realm of holiday programming, as it holds the potential to make applications more relevant and harmonious with users’ lives across various cultures.