A Comprehensive Guide to Vanilla JavaScript Fundamentals
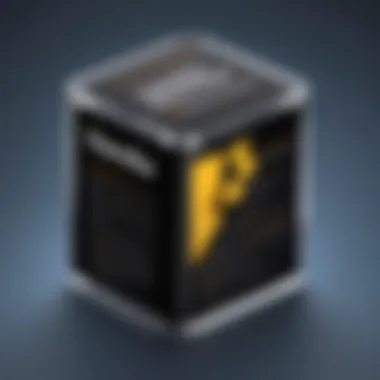
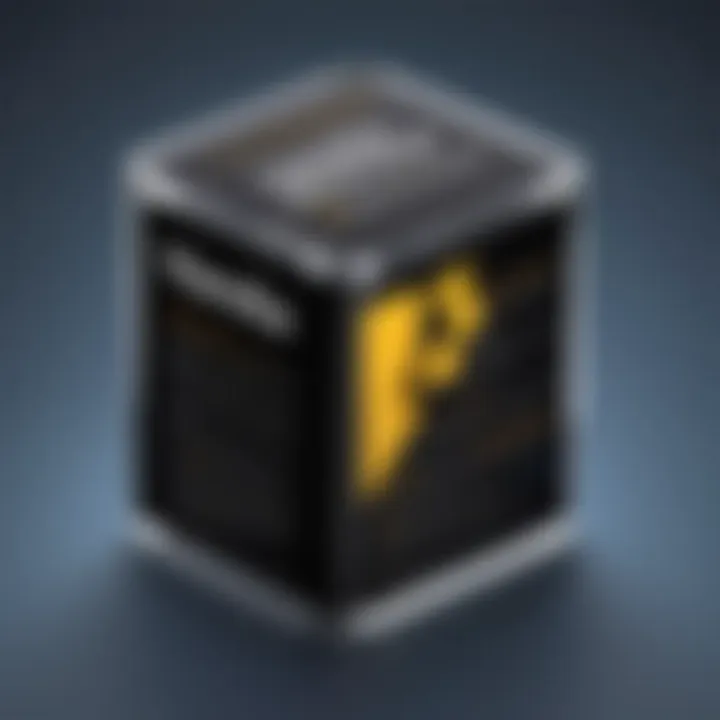
Intro
Prelude to Programming Language
JavaScript, a cornerstone of web development, is acquiring essential role in creating dynamic and interactive applications. Unlike its more expansive frameworks such as React and Angular, vanilla JavaScript refers to the pure form of the language, stripped of additional libraries or frameworks. Understanding this language is crucial due to its universality and foundational importance.
History and Background
JavaScript was created in 1995 by Brendan Eich while he was working at Netscape. Eich aimed to develop a lightweight scripting language that could enhance web pages. Initially named Mocha and later renamed to LiveScript, it finally became JavaScript, misleadingly connecting it to Java. Over the decades, JavaScript matured into a powerful programming language, widely adopted across the internet.
Features and Uses
The simplicity of syntax makes JavaScript approachable for beginners. Its features include
- Event handling
- Document Object Model (DOM) manipulation
- Asynchronous programming capabilities
These functionalities allow programmers to create highly responsive and engaging user experiences. Vanilla JavaScript is frequently used for adding interactive elements to websites, managing animations, and simpler game development.
Popularity and Scope
JavaScript is among the top programming languages globally. According to surveys, it stands as the most commonly used language among developers. The range of applications includes front-end development, back-end with Node.js, and even mobile app development through frameworks like React Native. Its importance continues to grow as the web communities expand.
Basic Syntax and Concepts
Having a firm grasp of basic syntax is critical to gaining proficiency in vanilla JavaScript.
Variables and Data Types
JavaScript refers to a few types of data:
- String: For text.
- Number: For numeric data.
- Boolean: True or false values.
- Object: Key-value pairs, for complex data structures.
Example of variable declaration:
Operators and Expressions
Operators perform actions on variables and values. Categories include:
- Arithmetic: , , ,
- Assignment: , ,
- Comparison: , , , ``,
Expressions can be simple or complex, depending on the requirement.
Control Structures
Control structures like if-else statements and loops govern how code executes. Statements determine which blocks to execute.
This code evaluates the , which results in a simple age filter.
Advanced Topics
For those seeking to deepen their understanding of vanilla JavaScript, advanced topics must be explored.
Functions and Methods
Functions are reusable blocks of code, which are vital for efficient programming. They can return values and take parameters.
Object-Oriented Programming
Object-oriented programming allows bundling data and functions. By creating objects, you can model real-world information.
Exception Handling
Error handling is vital when managing potential runtime issues. Using statements allows graceful failures to maintain user experiences.
Hands-On Examples
Implementing knowledge in practical scenarios aids skill enhancement.
Simple Programs
Creating basic functions or data calculators equips beginners with confidence in their coding.
Intermediate Projects
Calender apps or To-do apps exemplify using vanilla JavaScript to create functional utilities. These projects utilize both advanced topics mentioned previously.
Code Snippets
Code snippets could prove useful. Explore community resources or share common patterns.
Resources and Further Learning
Further self-education can lead to continued growth as a programmer.
Recommended Books and Tutorials
Several published works and online material serve programmers. StackOverflow and
Prolusion to Vanilla JavaScript
Vanilla JavaScript serves as the core foundation of web development. By understanding its principles, developers can effectively manipulate the behavior and aesthetics of their applications. In a world increasingly influenced by frameworks and libraries, it becomes essential to comprehend vanilla JavaScript. This knowledge not only informs best practices but also allows for a deeper grasp of how these libraries function beneath the surface.
Defining Vanilla JavaScript
Vanilla JavaScript is the term used for plain JavaScript, without any additional frameworks or libraries applied. It is simply the standard implementation recognized by all web browsers. In more technical terms, it corresponds to ECMAScript and allows developers to write highly performant code. Notably, when developers refer to vanilla JavaScript, they imply programming with native features without dependencies.
At its core, vanilla JavaScript comprises features like loops, functions, objects, and arrays that facilitate control over data. It does not cover the use of libraries such as jQuery or frameworks like Angular, React, or Vue.js. Instead, it presents a straight approach to coding, focused on learning the fundamental aspects of the language.
The Role of Vanilla JavaScript in Web Development
Vanilla JavaScript plays a vital role in modern web development, as it establishes the foundational building blocks for both simple and complex applications. Understanding these building blocks enhances one's capability to make libraries and frameworks more effective.
Importance in Performance
Using vanilla JavaScript removes additional overhead that frameworks often introduce, translating into better performance, particularly for straightforward tasks. This performance improvement is crucial especially in scenarios where page load time is critical. When reducing dependencies, the likelihood of encountering conflicts or bugs diminishes substantially.
Enhancing Job Readiness
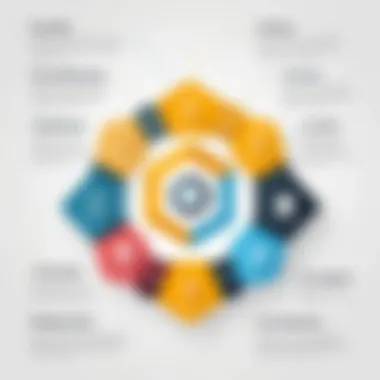
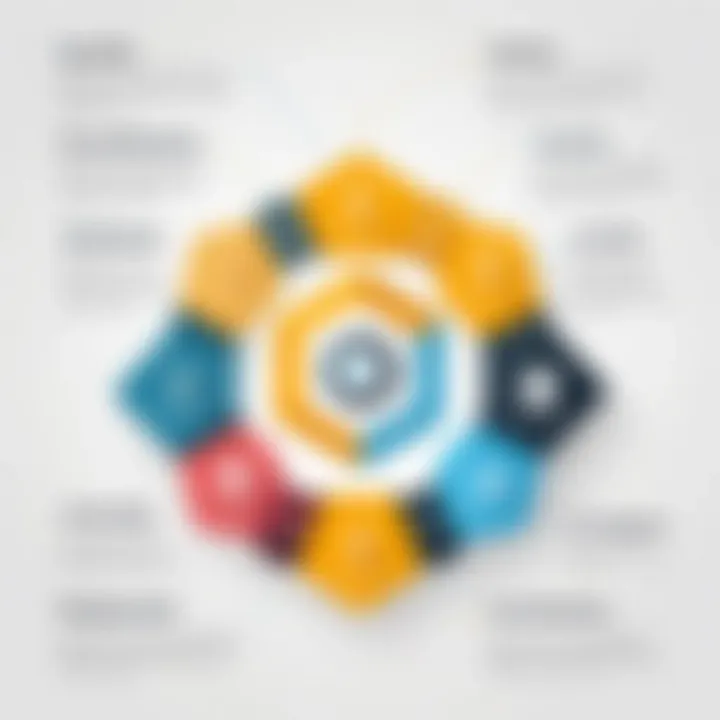
Employers value developers who have a strong knowledge of vanilla JavaScript. Many interview processes prioritize a deep understanding of core concepts. Without this knowledge, it can be challenging to address more complex tasks tackled within frameworks and libraries. Thus, laying down foundational skills is an essential step for any aspiring programmer.
Mastering Vanilla JavaScript is akin to grounding yourself before exploring more advanced languages or frameworks. It is a phase every skilled developer goes through.
Epilogue
In summary, mastering vanilla JavaScript is an invaluable asset for web developers. By understanding both the definitions and the roles algaeortaal unique trends, one can produce reliable and efficient code."
Why Use Vanilla JavaScript?
Understanding why to use vanilla JavaScript is crucial in a world teeming with frameworks and libraries. Vanilla JavaScript refers to using the pure, unmodified version of the language. By relying on vanilla JavaScript, developers can not only enhance performance but also reduce the complexity often associated with using multiple frameworks.
Performance Advantages
Performance optimization stands as a strong argument for employing vanilla JavaScript. Libraries like React or Angular introduce overhead, which can slow down applications. In contrast, code written in vanilla JavaScript typically runs faster due to its simplicity.
- Lightweight: Without dependencies on third-party libraries, the code remains lighter. This can lead to quicker load times and better performance.
- Execution Speed: Native JavaScript implementations execute faster than abstractions provided by libraries. Browsers optimize vanilla JavaScript very well.
- Fewer Resources: Using vanilla JavaScript requires less CPU and memory, benefiting performance-sensitive applications.
Ultimately, focusing on performance can set applications apart in the competitive tech landscape.
Reducing Dependency on Frameworks
Dependency on frameworks can bind developers to specific methodologies and upgrade cycles. By embracing vanilla JavaScript, developers free themselves from these confines.
- Flexibility: Vanilla JavaScript allows for intuitive programming without adhering to a library's cascading rules. This enables unique solutions tailored to project requirements.
- Maintenance: Relying solely on vanilla JavaScript facilitates easier updates and modifications in the project codebase. Frameworks can often lead to complex maintenance issues with versioning.
- Better Understanding: When developers build with vanilla JavaScript, they gain a clearer understanding of the language itself. This foundational knowledge strengthens problem-solving capabilities and reduces the learning curve when using frameworks later.
In the end, leveraging vanilla JavaScript connects developers closer to the mechanics of browser interactions, paving way for better mastery overall.
Utilizing vanilla JavaScript provides a firm foundation, enabling more informed decisions when addressing programming challenges.
By recognizing the significance of these factors, developers can make more strategic choices about when and how to leverage the extensive ecosystem that exists around vanilla JavaScript.
Getting Started with Vanilla JavaScript
Getting started with Vanilla JavaScript is crucial for both beginners and those looking to solidify their foundation in web development. This segment of the guide emphasizes the core tenets of Vanilla JavaScript and its usage in real-world projects. Mastering the basics can vastly improve proficiency in writing clean, efficient code. Furthermore, understanding its rules and setups fosters a profoundly deeper appreciation for how web features are constructed.
Setting Up Your Development Environment
Before writing any code, it’s essential to establish an appropriate development environment. This smooths the learning curve and avoids potential frustrations later on. Here are the significant elements to consider:
- Text Editor Software: Useful editors include Visual Studio Code, Sublime Text, or Atom. Each editor achieves similar goals but presents unique user experiences.
- Web Browsers: Choose a modern browser for testing purposes. Google Chrome and Mozilla Firefox are examples that offer developer tools.
- Folder Structure: Create a tidy file organization for your projects. Whether it includes folders for scripts, styles, or images, aptly naming folders can eliminate confusion.
Once set up, one can focus on coding without continually worrying about the environment itself.
Basic Syntax and Structure
Understanding the syntax and structure of Vanilla JavaScript remains essential. Clarity in writing code enhances readability for anyone involved in the project. Here are critical aspects to focus on:
- Variables: Declaring variables can be done using , , or . They hold data, whether strings, numbers, or more complex objects.
- Data Types: JavaScript handles different data types such as string, number, boolean, null, undefined, and object. Recognizing which type to use will improve logical flow in programs.
- Functions: Define functions using the function keyword, arrow functions, or other expressions. The ability to encapsulate functionality promotes code reusability.
- Basic Control Flow: Mastery of if statements, loops, and switch cases delivers control over execution flow based on conditions.
_"Establishing a solid grasp of syntax sets the stage for more advanced features down the road."
On foundational ground, students can now explore more recondite areas of advanced JavaScript and enjoy better security and performance in their web applications.
Key Concepts in Vanilla JavaScript
Understanding the key concepts in Vanilla JavaScript is vital for anyone who aims to work effectively with this programming language. These fundamental elements serve as the building blocks for developing web applications and features. By mastering these concepts, you improve your ability to write efficient and readable code, making the experience of learning JavaScript much more significant.
Variables and Data Types
Variables in JavaScript are containers that store data values. They are essential as they allow developers to manipulate and reference values throughout the code. JavaScript has a dynamic nature, which means you don’t need to declare the type of a variable explicitly.
There are three main ways to declare variables: var, let, and const. Each has its own scope and usage requirements:
- var: This keyword permits variable declarations to be scoped to the function and can be reassigned. However, hoisting can lead to unexpected behavior.
- let: This is block-scoped, meaning the variable will only exist in the curly brackets it is defined in. or are now generally favored over .
- const: Used to define variables that cannot be reassigned. This is ideal for constants.
Data types in JavaScript range from primitives to reference types. They include:
- String: This data type represents textual data.
- Number: Represents both integer and floating-point numbers, all in a single type.
- Boolean: Represents or values.
- Null: This is assigned to a variable that has no specific value.
- Undefined: This signifies a declared variable that has not yet been assigned a value.
- Objects: Used to store more complex entities, group key-value pairs together.
These basic components will create detection structures that translate human requirements to machine-understandable data.
Operators and Expressions
Operators and expressions are central to performing computations in JavaScript. Every programming language uses operators, and their significance cannot be understated.
Operators:
- Arithmetic Operators: Include addition (+), subtraction (-), multiplication (*), division (/), etc. They form the basic calculations in your code.
- Comparative Operators: Allow developers to compare values, utilizing equality (==), inequality, greater than (>), among others. These are often used in control flow to make decisions based on conditions.
- Logical Operators: Include AND (&&), OR (||), and NOT (!). They enable building more complex conditions in the code.
Expressions:
An expression is a combination of values, variables, operators, and function calls that evaluate to a value. For example, is an expression where is the operator that combines two values.
Using these tools, one can carry out various operations necessary for web functionalities.
Functions and Scope
Functions are powerful tools in JavaScript. They simplify code re-use and organization, promoting the DRY (Don't Repeat Yourself) principle. A function is a block of code designed to perform a particular task.
Functions can also accept parameters, allowing them to process inputs and return results. In JavaScript, you can declare functions in several ways: using the function keyword, arrow functions, and immediately invoked function expressions (IIFE).
When it comes to Scope, it defines the visibility of variables. Variables triggered in a function won’t be accessibile outside that function, ensuring encapsulation.
- Global Scope: Variables available throughout the code.
- Function Scope: Restricted to the function it is defined in.
Being adept in functions and their scopes offers developers knowledge to dodge pitfalls during programming.
Control Structures
Control structures are essential as they decide the flow of code execution. They form the logic behind making decisions and conducting operations based on varying conditions.
Primarily, you have:
- Conditional Statements: Like , , and . These help in executing specific blocks of code based on whether a condition evaluates as true or false.
- Switch Case: This control structure is helpful when you want to test a value against different cases. It’s tidy and reduces long if statements.
- Loops: Such as and , allow executing a code block multiple times, limited by a specific condition. This is crucial when dealing with datasets and repetitive tasks.
Understanding these elements provides the frameworks needed to control how scripts are handled, allowing for dynamic interactions within web applications.
Manipulating the Document Object Model (DOM)
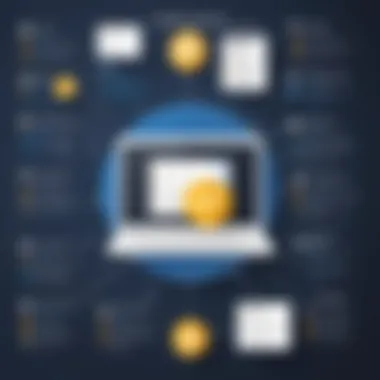
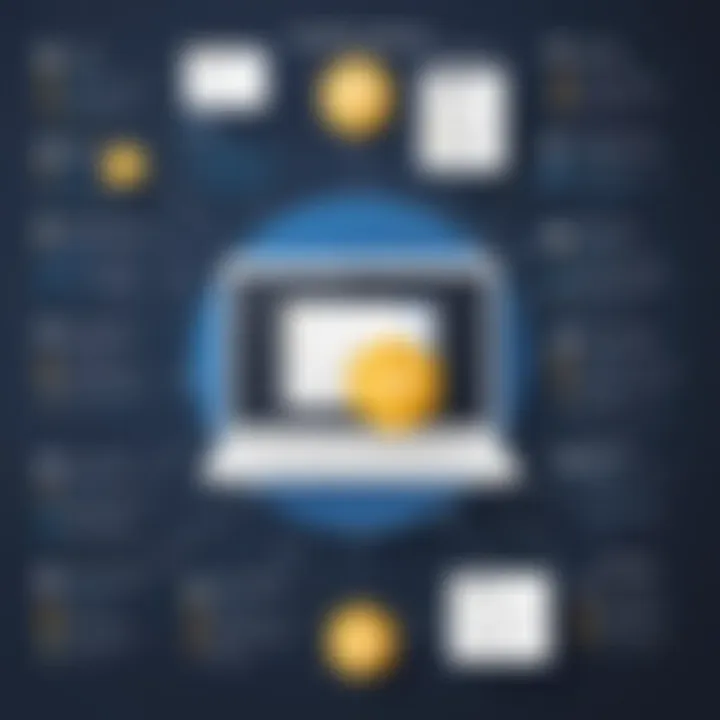
Manipulating the Document Object Model, commonly known as the DOM, is central to understanding how web pages communicate with the browser. The DOM represents the structure of a web document as a tree of objects. Each node in the tree represents a part of the document, such as elements, attributes, and text. Learning to navigate and manipulate the DOM effectively allows developers to create dynamic and interactive web applications using vanilla JavaScript.
Understanding the DOM Hierarchy
The DOM hierarchy organizes elements from the top of the document. At the root sits the object, which can be thought of as the starting point for accessing any element within the web page. From there, elements such as , , and branch out to display content. Each subsequent child describes parts of that structure in terms of parent and child relationships.
Understanding this hierarchy helps developers find and manipulate elements more efficiently. As an example, one can access the title of a page through the hierarchy as follows:
This command accesses the element by first traversing through the child of the object. Knowing how to navigate the DOM automatically enhances a developer's speed and precision.
Selecting and Modifying Elements
Selecting and modifying elements in the DOM is vital for achieving dynamic interactions. Developers can select elements using methods such as , , or even . Each of these methods offers unique advantages depending on the context of use. For instance:
- returns a single element with the specified ID, which is often necessary for targeting unique elements.
- allows for more flexibility as it accepts any CSS selector for more complex searches.
Once selected, modifications can easily be made to any element
- Changing the content can be done with or properties.
- Updates to styles or classes are achievable with properties such as or .
For example:
With this capability, developers can respond to user interactions and create a seamless experience across web applications.
Event Handling
Event handling is essential for responding to user interactions in a webpage. In JavaScript, events signify user actions such as clicks, keypresses, or changes in an input field. Setting up event handlers allows developers to execute specific functions when these actions occur, drastically enhancing user experience.
For instance, to handle a click on a button:
In this example, when a button is clicked, an alert pops up, showcasing that seamless interaction achieved via events. It's important to attach these handlers responsibly, however, ensuring they do not lead to performance issues by creating unnecessary listeners or neglecting eventual removals.
Understanding how to manipulate the DOM and handle events forms the bedrock of effective web development. These skills not only enhance user experience but also provide a practical foundation for learning more complex frameworks later.
In summary, effective DOM manipulation is vital not only for web applications but also for creating a more interactive and responsive user interface. Mastery of this topic empowers developers, whether seasoned in their careers or just starting their journey into the world of programming.
Asynchronous JavaScript
Asynchronous JavaScript is a fundamental aspect of modern web development, preventing the browser from freezing while it waits for processes like API responses. Mastering asynchronous programming becomes crucial for developing interactive and responsive applications. It ensures operations run smoothly, improving user experience and performance. Developers frequently face scenarios where an operation takes time, such as fetching data. Understanding how to handle such situations effectively enhances programming skills.
Understanding Callbacks
Callbacks are functions that are passed as arguments to other functions. They are essential in handling asynchronous operations. When an operation completes, it invokes the callback function to execute certain tasks without blocking the main thread.
Consider the following example:
In this code, simulates fetching data and then uses a callback to log the result. If it's not handled properly, callback functions can lead to callback hell, a situation where code becomes nested and difficult to read. To manage complexity, it's essential to structure your callbacks and possibly favor techniques like promises instead.
Promises and Asynchronous Functions
Promises are a cleaner way to manage asynchronous operations. A promise represents a task that may complete in the future, either by resolving with a value or rejecting with an error. They help flatten the structure of asynchronous code, making it easier to follow.
Creating a promise looks like this:
In this snippet, the function returns a promise. If the operation is successful, it resolves; otherwise, it rejects. Using promises makes the code more manageable, enabling easier error handling with . Additionally, async/await syntax, introduced in ES2017, builds on promises, allowing naive synchronous-looking code that's clean and efficient.
An example with async/await:
This streamlined approach enhances readability and maintainability in asynchronous programming. Thus, mastering callbacks and promises is integral as they form the backbone of modern JavaScript practices, setting a robust foundation for handling concurrency in applications.
Object-Oriented Programming in Vanilla JavaScript
Object-oriented programming (OOP) is an essential paradigm in programming languages, including vanilla JavaScript. It focuses on organizing code using objects, which can encapsulate both data and behaviors. Understanding how OOP functions in vanilla JavaScript is important. It enables better code organization and reusability. As developers approach complex applications, adopting this approach becomes necessary to maintain scalability and structure.
Creating Objects and Prototypes
In vanilla JavaScript, creating objects is essential for reducing code duplication and enhancing modularity. Objects in JavaScript are created through various methods such as object literals and constructor functions.
Object Literals
The object literal notation is a straightforward method to create a new object:
This example shows a simple object representing a car. It has properties for make, model, and year, alongside a method to start the car.
Constructor Functions
An alternative to object literals is constructor functions. This method is useful when creating multiple similar objects. Here is how you can define a constructor function:
To create new instances, you would use the keyword:
Through prototypes, additional functionality can be added. For example, you can define a method on the prototype like this:
This approach fosters a clear method of adding shared behaviors to all instances, minimizing memory usage and creating consistency.
Class Syntax and Inheritance
ES6 introduced a more straightforward and cleaner syntax for classes in JavaScript. The keyword offers a syntactical sugar over the traditional constructor functions. It simplifies OOP by providing a clear framework for encapsulating data and methods.
Creating a Class
Here is how you can define a simple class:
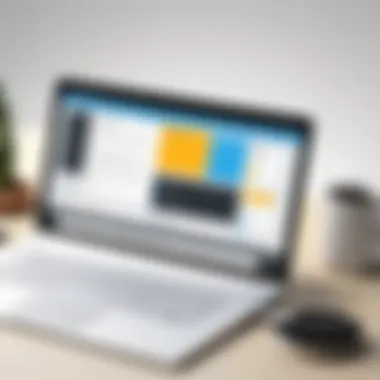
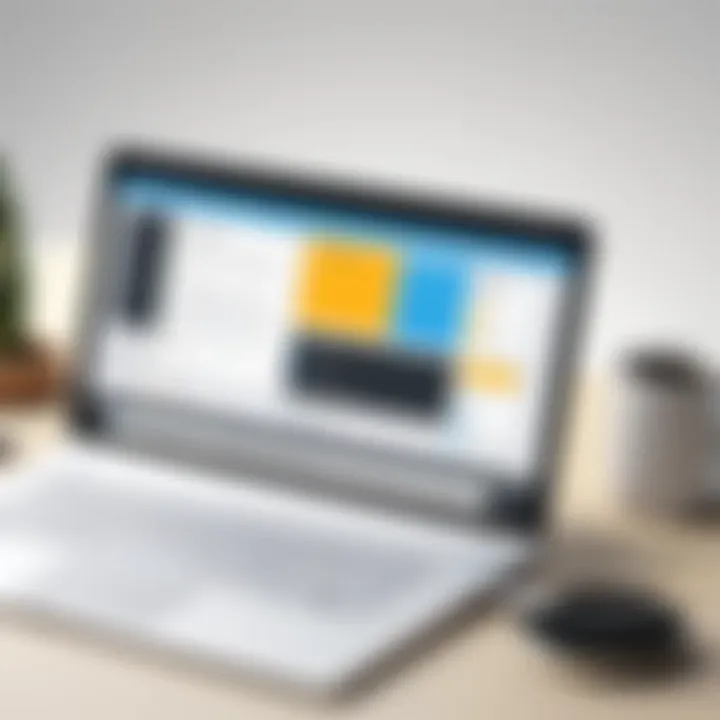
This class includes a constructor and a method, . You can then instantiate it similarly to functions.function:
Inheritance
Inheritance allows a class to inherit properties and methods from another class, providing an organization to common behaviors among related entities. You can use the keyword to create a derived class:
The class takes the properties of the class and adds its own. When you create an instance like this:
The instance can access from the base class as well as the new method defined in the class.
Summary: OOP in vanilla JavaScript greatly enhances code modularity and maintainability. Creating objects effectively and utilizing class syntax allows developers to articulate their intentions clearly. Doing so contributes directly to mastering complex systems in JavaScript.
Real-World Applications of Vanilla JavaScript
Understanding the real-world applications of vanilla JavaScript is vital. This knowledge bridges the gap between theory and practice, providing clarity on how this programming language enhances web results. Proficiency in vanilla JavaScript empowers developers to create more than just a basic website—it facilitates the development of dynamic, responsive web features and applications.
Building Interactive Web Features
Incorporating interactivity is a foundational aspect of modern web design. Features such as image galleries, modal pop-ups, and real-time content updates heavily rely on JavaScript.
Vanilla JavaScript allows developers to rethink user experience. Simple elements can become visually appealing and engaging with some additions. For example, instead of static text, you can leverage focal interactions to reveal hidden content without page reloads. This engages users by improving the overall website experience.
Consider incorporating event listeners to recognize user actions. Providing instant feedback transforms how users interact with web applications. You could do this by implementing a click listener and corresponding handler functions using vanilla JavaScript. This fosters a sense of real-time communication and enhances the intuitiveness of the website.
Furthermore, mapping the interaction accurately allows users to navigate projects seamlessly, ultimately leading to higher user retention rates. Overall, building interactive web features not only heightens user satisfaction but also improves the site's practical functionality.
Here, a brief code sample illustrates adding an interactive button:
This simple interaction can efgectively highlight moments and services effectively.
Creating Simple Web Applications
Web applications have revolutionized how users access data through the internet. Frameworks abound but starting with vanilla JavaScript provides advantages at its core, beginning with understanding foundational principles. Emerging in a climate rich with complexity and overhead, developers can harness uncomplicated frameworks as a powerful tool.
Vanilla JavaScript promotes comprehension over frustration. Simulate more complex functionality by animating objects or transitioning states using straightforward coding. Within this framework of logic, developers can realize basic phenomena with clarity. A web application like a task manager illustrates this principle well. You can maintain task information despite the simplicity of functionalities by utilizing well-structured object management.
With this means, errors occur less frequently. For students building skill, anything serving to broaden perspective can rejuvenate gaps of understanding. Gaining fluency with vanilla JavaScript enhances one’s capacity down the line when engaging complex programming with more robust frameworks.
Consequently, developing simplified applications reinforces important theories, improving your programming journey.
To wrap up, diving deeper into JavaScript's real-world applications fosters foundational insights essential for any aspiring web developer. It's meritorious to analyze interactions achieved through basic features. Such simplicity has flourishing implications, instilling the importance evident in further expedition into JavaScript skill sets.
Understanding the balance between simplicity in design and user affects remains a key advantage when using vanilla JavaScript.
Testing and Debugging Vanilla JavaScript
Testing and debugging are crucial aspects of programming in Vanilla JavaScript. Without them, the code written can become prone to problems and errors, which lead to unforeseen disruptions in web applications. It ensures the functionality meets the expected results. This section focuses on common pitfalls, errors, and effective debugging techniques.
Common Pitfalls and Errors
When working with Vanilla JavaScript, developers frequently encounter several specific pitfalls and errors. Being aware of these can help improve the quality and performance of the code.
Here are some common mistakes:
- Typographical Errors: Mistakes often occur with misspelled variable names or functions, which can lead to reference errors.
- Variable Hoisting: JavaScript hoists variable declarations, which can cause unexpected issues if a variable is accessed before it is defined.
- Asynchronous Code: Overlooking the behavior of asynchronous functions may lead to callbacks executing in an unintended sequence.
- Type Coercion: JavaScript are weakly typed. It may implicitly convert types, resulting in unintentional bugs when comparing different data types.
It is essential to understand that recognizing typical errors early makes debugging easier and more efficient.
Using Developer Tools for Debugging
Developer tools in modern web browsers offer powerful features for diagnosing issues in your code. With insightful debugging capabilities, these tools help streamline the process. Here’s how they benefit your development process:
- Console Logging: Using efficiently is a primary way to capture outputs or variable states at specific execution points.
- Breakpoints: Developer tools allow you to set breakpoints. This slows down the execution, letting you investigate variable values at a particular line of code.
- Error Messages: Warnings and error messages displayed in the console offer direct insight into problems in your code. Writing corrected responses to these messages aids in improving code quality.
- Live Reloading: Many tools enable live reloading of pages. You will immediately see impacts of changes without refreshing the entire page.
In summary, taking advantage of testing and debugging safeguards your project against common errors and enhances your coding practice, crucial for anyone learning programming.
Best Practices in Vanilla JavaScript Development
Best practices in vanilla JavaScript development are essential for ensuring code quality, consistency, and ease of maintenance. Applying these strategies leads to an improvement in the overall codebase, making it easier for other developers to understand and collaborate on projects. It also minimizes bugs and enhances performance.
By adhering to these best practices, you can enhance your programming workflow. For students and learners, particularly, it is helpful to instill these practices early in their coding journey for solid foundations.
Code Readability and Maintenance
Code readability refers to how easily a developer can read and understand code. Writing clear and well-structured code is not just a matter of style, it's a necessity for maintenance.
- Use Descriptive Variable Names: Choose variable names that clearly describe their purpose. For instance, using a name like over a generic name like provides clarity about what the variable represents.
- Consistent Formatting: Maintain consistent indentation and line spacing. Most developers appreciate a uniform look. This could involve aligning braces, consistently using either single or double quotes, and keeping lines to a reasonable length.
- Break Codes into Functions: Logic should be separated into functions, avoiding large blocks of code. This promotes reusability and simplifies the testing process.
- Limit Global Variables: Overusing global variables increases the risk of naming conflicts. Be judicious with their use; prefer local scope when possible.
- Adopt Conventional Structures: For instance, using an MV* design pattern can greatly improve the organization of your code.
Clearly expressing the logic and flows through these practices allows other programmers or future you to quickly grasp what has been done. You'll avoid the frustration that often accompanies cryptic code where a great focus is always on understanding rather than actually working on solutions.
Using Comments and Documentation
Comments in the code improve long-term maintainability. This guideline guides not only defining how code works but also the why behind it.
- Explain Complex Logic: When encountering convoluted pieces of logic, provide insight into why you made certain decisions in comments.
- Comment Functions and Modules: Brief overviews on what your functions do should precede the actual code implementation. It's more useful for others (and your future self).
- Update Comments as Code Changes: A best practice is to update comments when the related code changes. Stale comments become misleading, causing confusion.
- Use In-Line Comments Sparingly: Keep in-line comments concise, ensuring not to clutter the code. Instead, partition complex ideas with logical spacing.
Followers of these techniques can lead to a significantly more accessible code that anyone can work with expeditiously both during development and when maintaining the project later on.
Adopting best practices in coding creates lesser chaos during collaborations, promoting harmony amidst teams.
Overall, cultivating good habits like code readability and descriptive commenting can catalyze your development projects into remarkable cohesive products. Prioritize these aspects, and they can enhance not only individual efforts but also collective productivity.
Resources for Further Learning
The journey of mastering vanilla JavaScript does not end with understanding basic concepts or even implementing intermediate projects. Continuous learning through various resources is crucial to deepen your knowledge and improve your skills. Accessing the right resources can provide diverse methodologies, updates on best practices, and ecxhanded clarity on advanced topics.
When diving into resources for further learning, consider what will most impact your growth. Here are two primary areas where learners should invest time: books and online courses, as well as active engagement with developer communities.
Recommended Books and Online Courses
Books and online courses serve different learning needs. Well-written books can present fundamental theories and methodologies clearly. They often provide context to the coding practices you encounter on a daily basis. For online courses, they enable you to practice what you have learned with guided projects under professional instruction. Here are some notable recommendations:
- "Eloquent JavaScript by Marijn Haverbeke": This book is an authoritative book for JavaScript teachers to recommend. Its approach combines theory with practical examples, making it useful for both beginners and intermediate learners.
- "JavaScript: The Good Parts by Douglas Crockford": This book delineates what criticisms are overwrought and which JavaScript features bring true value.
- Online Platforms like Codecademy offer practical, interactive lessons focused on vanillla JavaScript, which can be incredibly helpful in getting hands-on experience. Youtube also hosts many tradiational as well as non-didiatic sources focusing on bad habits to avoid and best practices for clean coding.
By integrating different resources, you ensure a multi-faceted learning path, thus reflecting diverse professional experiences.
Engaging with Developer Communities
Being part of a network of learners and professionals opens up avenues for discussion, collaboration, and inspiration. Engaging with developer communities provides insight you might miss if learning solo. These communities might include online forums, social media platforms, or even local coding groups. Each offers a unique way to connect with others on the same path.
- Platforms like reddit.com/r/learnjavascript contain threads full of peer discussions that could spark essential learnings. You can ask questions or share projects and solicit feedback.
- Also, Facebook and LinkedIn groups might have local presence advantages. Engaging here juxtaposes myriad skill levels, allowing you to both give back and obtain useful information. Learning via collective dialogue highlights your imperfections and illuminates fresh perspectives on problem-solving.
Developers and learners from various backgrounds provide different views that multiply your understanding. This environment nurtures curiosity and encourages knowledge.