Mastering React Router: A Complete Guide
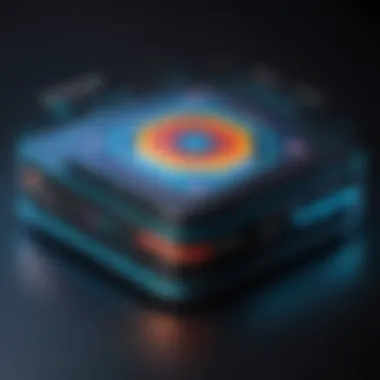
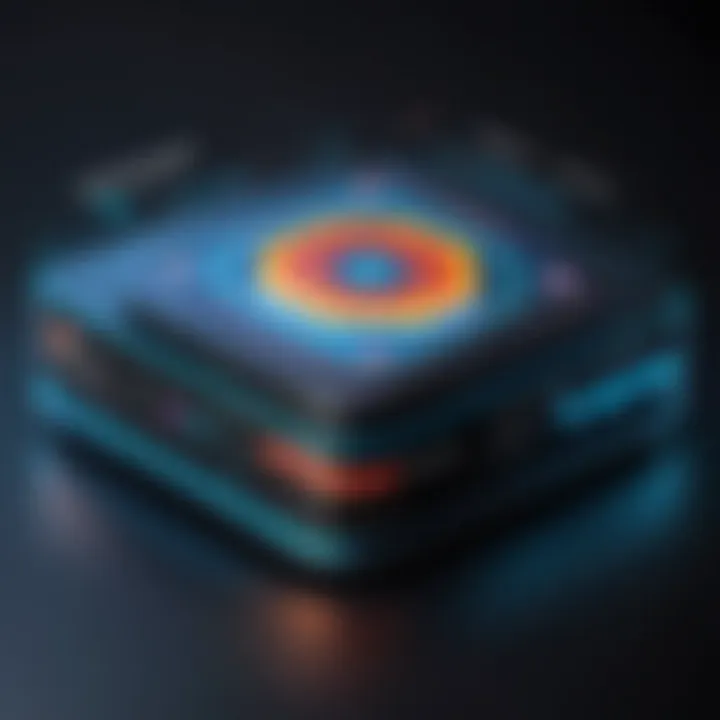
Intro
In the world of web development, managing navigation is essential. This is where React Router comes into play. It's a powerful library that is highly regarded in the React ecosystem. If you're looking to build apps that require multiple views and seamless transitions between them, understanding React Router is key. With its ability to maintain the history of your application's navigation, users can interact fluidly, which leads to a better experience.
What is React Router?
React Router is a standard for routing in React applications. It allows you to create single-page applications (SPAs) easily by letting users navigate between different components without refreshing the entire page. This leads to smoother performance and a more dynamic user interface.
Importance of Routing in React
Without proper routing, developers face challenges while creating interactive applications. With React Router, you can:
- Manage complex navigation structures.
- Keep user interfaces responsive.
- Maintain browser history for easier navigation.
- Split code for better performance.
Using routing effectively can dramatically improve your applications.
Users of React Router
Typically, React Router is utilized by:
- Front-end developers keen on enhancing web applications.
- Teams focused on building rich SPAs.
- Any developer wanting more control over navigation patterns.
By engaging with this guide, you will gain a stack of knowledge on how React Router can be implemented in real-world applications, making your development journey smoother and more efficient.
Intro to React Router
In the realm of modern web development, the ability to navigate seamlessly through a web application is critical. This is where React Router steps in as an essential library. Simply put, React Router enables routing in your React applications, guiding users through various components and pages without the need to refresh the entire browser. The need for smooth navigation is paramount, especially in Single Page Applications (SPAs) where dynamic changes are the norm rather than the exception.
Overview of React Router
React Router is a powerful routing library specifically designed for React applications. It allows developers to define multiple routes, map them to specific components, and manage history easily. At its core, React Router maintains a URL that reflects the current state of the application, creating an experience akin to that of a traditional multi-page site. This setup not only aids in enhancing user experience but also improves SEO performance for publicly accessible pages.
Some of the key features include:
- Dynamic Routing: Routes can change dynamically based on data, enabling a fluid user experience. This means you can display different components depending on the URL, which is currently relevant for user interaction.
- Nested Routing: This allows for more complex applications by enabling routes within other routes. The hierarchy of navigation can mirror your component structure, providing a clear path for users.
- Declarative Routing: React Router uses a declarative approach to routing, which means you define routes in a straightforward way. This translates your routing logic directly into the component structure, making it clean and easy to follow.
Importance of Routing in SPAs
In the context of Single Page Applications, routing makes all the difference. SPAs aim to provide a smooth, fluid experience, akin to using a native application. Without effective routing, a SPA might feel like a static site, where users have to navigate back and forth without clear visual cues.
Routing in SPAs offers several significant advantages:
- User Experience: A well-structured routing mechanism provides a smooth and intuitive navigation experience. Users can move seamlessly between sections of the app, engaging with content fully.
- Reduced Load Time: By avoiding full page refreshes, SPAs leverage browser history and maintain state in a lighter, faster manner. This not only speeds up the perceived load time but enhances performance metrics.
- Enhanced Bookmarking: With proper routing, users can directly link to specific views within the application. This opens up opportunities for sharing and revisiting content effectively, just like traditional websites do.
- Centralized Navigation: Routing simplifies the management of global states across an application, allowing developers to focus on building components without worrying about individual state management intricacies.
As we venture deeper into this comprehensive guide on React Router, itās important to appreciate these foundational elements. They set the stage for understanding not just how routing operates but why it's indispensable in modern web applications.
By using React Router, you empower your applications with the navigation they need, making every click count toward an enriched user experience.
With this context in mind, letās explore how to set up React Router and delve into its core functionalities.
Installation and Setup
Getting React Router up and running is a fundamental step in creating an effective single-page application (SPA). This section not only discusses how to install it but also emphasizes the importance of understanding the setup process. Having a solid knowledge of installation and setup can save you time and frustration later. When you start implementing routing in your app, a smooth installation can help you avoid a tangled web of issues down the line.
Prerequisites for Using React Router
Before diving into installing React Router, there are a few prerequisites to keep in mind. First and foremost, you need a basic understanding of React itself. If you are not familiar with React, it might be wise to familiarize yourself with its core concepts. Here are some essential points to note:
- Node.js and npm: Ensure you have Node.js installed. npm, which comes with Node.js, serves as your package manager, enabling you to install React Router easily.
- React App: If you haven't created a React app yet, use Create React App. It's a one-stop solution for bootstrapping a new React application with zero configuration needed, which is convenient.
- Basic React Knowledge: You should understand components, props, and basic state management in React. This knowledge will help you grasp how React Router integrates with your overall application structure.
By ensuring you're equipped with these prerequisites, you can avoid head-scratching moments later on.
Installing React Router Library
Now that you are prepped and primed, it's time to install React Router. The process is straightforward, yet itās the little details that can often trip you up. Hereās how to get it done:
- Open Your Terminal: If you're using a terminal or command prompt, navigate to your React application directory. If you just created a new app using Create React App, it will already be there.
- Run the Installation Command: Type the following command:This command fetches React Router's core library and makes it available in your project. Remember, is specifically for web applications, while can be used for mobile apps built with React Native.
- Verify Installation: After running the command, check your file to ensure that now appears in your dependencies. Also, itās a good habit to run the application to ensure everything is working smoothly.
Quick Tip: Keep your packages up to date. As React Router evolves, newer versions could offer performance improvements, bug fixes, or new features. Always refer to the official documentation for the latest details.
With installation and setup squared away, you can now dive deeper into the features of React Router and start building routes for your application. Understanding these foundations not only boosts your confidence but also lays the groundwork for more advanced routing techniques in the subsequent sections.
Basic Routing Concepts
In the landscape of web development, understanding the fundamentals of routing is like learning the ABCs before crafting an essay. Routing defines how users move through an application, and therefore, itās crucial for managing application flow and user experience. At its core, React Router provides the tools necessary for implementing navigation within single-page applications (SPAs), ensuring that users can traverse between different views seamlessly, without page reloads.
The importance of grasping basic routing concepts cannot be overstated. The success of an application often hinges upon how intuitively it guides users from one component to another. This effectiveness is directly linked to the architecture of your routes. Routing not only helps in directing users but also augments the overall user satisfaction by preventing confusion during navigation. Think of it as designing a roadmapāif your paths are clear, users will easily find their way around.
Understanding Route Component
Hereās an overview of how the Route component works:
- Path Matching: Each Route listens for changes in location URL and matches it against the specified path. If thereās a match, it renders the corresponding component.
- Component Rendering: You can specify which component to render directly through the prop. For example:This would render the component whenever the user navigates to .
- Exact Matching: Sometimes, the default behavior of Route is to match partially. If you want a path to match exactly, the prop should be employed. For instance:
- Redirects: You can also use Route to redirect users from one URL to another with the help of the component, which helps manage navigation paths smoothly.
"The Route component is essentially asking, 'What do you want to see today?' based on your current location."
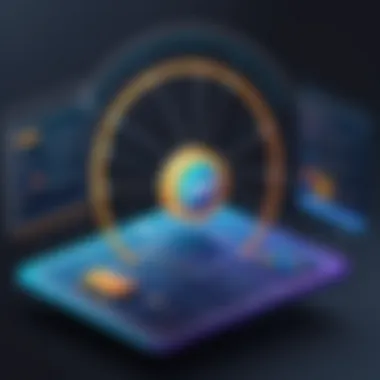
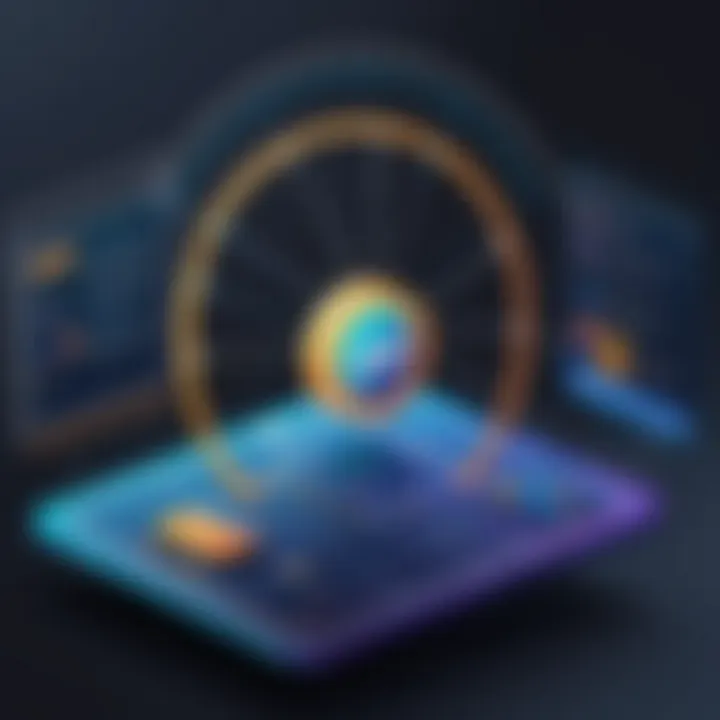
Navigating with Link Component
Navigating through your application goes beyond just defining routes. It involves creating a user interface that allows users to traverse those routes efficiently. Thatās where the Link component comes into play. Unlike the traditional tags, which cause full page reloads, Links provide a more seamless experience in SPAs.
- Creating Navigation Links: To create a navigation link, you simply wrap your text or elements within a component and specify a property, which denotes the target route. For example:When users click on this link, theyāre taken to the route without refreshing the page.
- Styling Links: You can even style the Link to give users feedback on which route they are on. By using the prop, you could add active styles:
- Handling Complex Navigation: For more complex navigation needs, such as using nested routes, the Link component integrates smoothly with those setups too, ensuring a fluid user experience regardless of complexity.
In summary, the Route and Link components serve as your navigational compass and pathways in your application, respectively. Without these, directing users through your app could become as tricky as navigating a maze without a map.
Advanced Routing Techniques
In the dynamic landscape of web development, the ability to manage routes effectively is paramount, especially when using React Router. As applications grow in complexity, so does the necessity for advanced routing techniques. This section digs deep into two key concepts: implementing nested routes and dynamic routing with URL parameters. These concepts arenāt just fancy tricks; they can significantly enhance both user experience and application structure.
Implementing Nested Routes
Nested routes are a powerful feature that allows developers to create a more organized routing structure within a React application. This technique enables the building of routes that are hierarchically related. For instance, consider a dashboard for a web application where the main route leads to a dashboard component, but within it, there exist several sub-components like user settings, profile page, and admin options. Utilizing nested routes facilitates a clear hierarchy and separation of concerns.
Implementing nested routes can be as simple as including a component that renders child components under a parent route. Hereās a basic approach:
In this example, the component can wrap its children's components without crowding its own definition. The primary advantage here is clarity and maintainability in routing logic. Each child route can independently manage its internal logic, making it easier for developers to scale the project in the future.
Moreover, by nesting routes, developers can pass down props, access additional data, or even share state between child components effortlessly. Additionally, it allows more seamless transitions for users, as they remain within the same parent context rather than being flashed out and back again through the main navigation.
Dynamic Routing with URL Parameters
Dynamic routing introduces the capability to create URLs that are parameterized, enabling real-time data retrieval based on user inputs or application state. This is particularly useful in scenarios where data is fetched based on unique identifiersāthink of a user profile page that loads data based on the user ID.
React Router permits this through URL parameters. Hereās an illustration:
In this instance, serves as a placeholder for the actual user ID. When a user navigates to , the component can extract from the URL, allowing it to fetch data specific to that user. This flexibility elevates the application to a new level of interactivity.
Dynamic routing also aids in creating comprehensive and intuitive user experiences. For instance, one can construct an e-commerce site where clicking on a product leads to a dedicated product page, pulling in relevant details by the product ID embedded within the route. This not only streamlines data management but also enhances SEO capabilities as clean, descriptive routes improve crawlersā understanding of site architecture.
"To learn to use advanced routing techniques effectively is to wield the power of seamless navigation and data integrity."
As we dive deeper into this guide, remember that mastering these concepts will set the stage for your journey in React application development.
Route Guards and Navigation
Route guards are a crucial concept in React Router that helps to control access to certain parts of an application based on specific conditions. They play a significant role in securing routes and ensuring that users can only access the data or functionality that they are authorized to. Without proper regulations in place, user experience could be compromised, leading to potential security risks. Given the pervasive nature of web applications today, understanding route guards is essential for any developer striving to build robust, user-friendly applications.
Creating Protected Routes
Creating protected routes ensures that sensitive or crucial areas of your application are shielded from unauthorized access. This functionality is vital when developing applications with varying user roles or permissions. For example, if you're building a healthcare platform, you might want patients to access their medical records while preventing unauthorized users from viewing this sensitive information.
To implement a protected route in React Router, you can leverage a combination of components and hooks. Hereās a simplified illustration of how it can be executed:
In this snippet, we create a component that accepts another component as a prop. Inside, we check for authentication status. If the user is authenticated, the component renders. If not, it redirects the user to a login page. This way, you can maintain clear navigation while safeguarding sensitive areas of your application.
Handling User Authentication
In any application, effective user authentication is the bedrock of security. In the realm of React Router, managing user authentication and its associated states becomes paramount to safeguard user data and enhance navigation flow. Authentication is not merely about logging in; it's also about maintaining state throughout the user experience.
Using a combination of local storage, cookies, or even session management can be beneficial in handling user authentication. Developers often implement context APIs combined with reducers for managing state seamlessly across the application. Below are some considerations for effective user authentication:
- Ensuring Secure Data Transmission: Always utilize HTTPS to encrypt data sent between the client and server. This protects sensitive information from being intercepted.
- Session Management: Leverage session storage or cookies for maintaining user session. Choose mechanisms aligned with your security requirements, considering the implications of each.
- Error Handling: Implement robust error handling throughout the authentication workflow. This will improve user experience by providing feedback during failed attempts.
- Best Practices: Regularly update and patch any external libraries to mitigate vulnerabilities associated with user authentication.
Should everything go pear-shaped and a user becomes unable to access a part of your app due to an authentication failure, it's vital to keep them informed. Provide clear messaging about why access was denied and offer pathways to rectify the situation.
"Security is a process, not a product." ā Bruce Schneier
Keeping in mind these fundamental elements can substantially enhance the security and usability of routes within your React applications, ensuring that users can navigate through their options without undue risk.
Managing Routes with State
Managing routes with state is crucial for creating a dynamic user experience in React applications. As applications grow in complexity, the need to maintain and manage state effectively while navigating between different pages becomes vital. This section delves into the key aspects of state management, focusing on two fundamental components: React Context and Navigation History.
Using React Context for Routing
React Context provides a powerful means to pass data through a component tree without having to manually pass props at every level. This becomes particularly useful for routing as it allows your application to share routing state across various components seamlessly. By using Context, you can manage route-related state globally, which enhances the maintainability of your application.
For example, suppose you have a multi-step form spread across different routes. Here, React Context can store user inputs and navigation progress, enabling users to jump between steps without losing their data. This not only improves user experience but also simplifies managing state across components that donāt share direct parent-child relationships.
"With React Context, itās like having a shared room where all components can communicate without shouting over each other."
To implement this, you can create a Context wrapper around your application. Hereās a snippet of how you might define a context for routing:
Storing Navigation History
Navigating effectively within your app often involves keeping track of the user's journey through various routes. This is where storing navigation history comes into play. By maintaining a log of users' visited routes, you can create features like back buttons or even breadcrumbs that enhance user experience.
Storing navigation history allows users to easily return to previous pages, which is especially important in applications with deep hierarchies or many subroutes. Additionally, you can analyze this history to gather insights on user behaviors, thereby making informed decisions for further development.
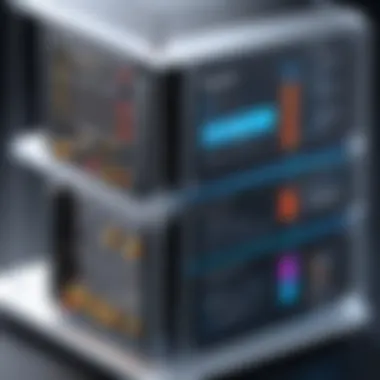
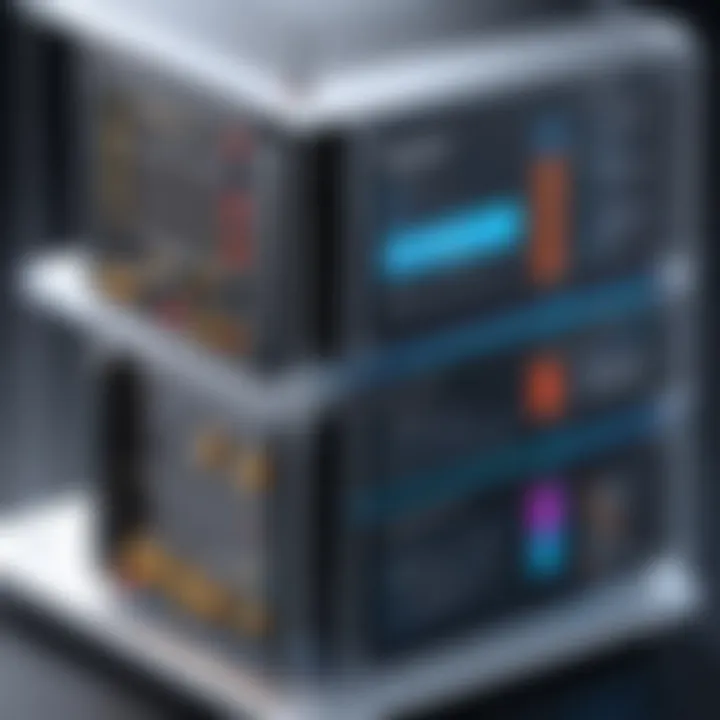
A simple way to achieve this is by utilizing the hook in conjunction with your routing logic. Hereās a quick outline on how it's done:
- Initialize State: Use a state variable to keep track of the history array.
- Update History on Navigation: Every time a user navigates, push the new route into the history array.
- Back Navigation: Provide a mechanism to pop the latest entry from the history when users hit the back button.
Here's how you could structure this in your component:
Styling and Animating Routes
When building applications with React Router, the experience doesn't just lie in the functionality but also in how it feels to the user. Styling and animating routes not only enhances usability but also adds a layer of professionalism to your application. Users today expect smooth transitions and visually appealing interfaces, so addressing these aspects can make your application stand out amidst the competition.
Applying CSS for Route Transitions
One of the crucial elements of styling routes is creating effective transitions between them. Transition effects provide a smooth user experience, signaling to the user that a change is happening. This can be done using CSS properties like , , and . Hereās a brief rundown on how to implement this:
- Defining a Base Style: Start with basic styles for your components. For instance, you can set the initial to zero.
- Setting Active Style: Once the route changes, you may want to increase the opacity to one.
- Logic for Class Application: You could manage transitions with state in your component. Based on the route change, add or remove the class.
This simple approach not only ensures smooth transitions but can also help in maintaining a consistent aesthetic across your application. Another aspect to consider is responsiveness; transitions should look good on any device, so testing on various screen sizes is essential.
"In design, simplicity is the ultimate sophistication." ā Leonardo Da Vinci
Integrating Animation Libraries
While pure CSS techniques have their merits, sometimes you need more complex animations that CSS may not easily cover. This is where animation libraries come into play. Libraries like or can significantly simplify the animation process and offer advanced features.
Benefits of Using Animation Libraries:
- Easier Implementation: They often provide out-of-the-box functionality for transitions, reducing the need to code from scratch.
- Rich Animations: Libraries often offer more complex animations that can be visually engaging and enhance user experience.
- Interactivity: Many libraries provide options to tie animations to user interactions, creating a more dynamic application.
For example, integrating for route transitions may look something like this:
Here, the component takes care of the transition while you simply focus on rendering your children. Incorporating animation can transform an ordinary app into something appealing. However, always remember not to overdo itāexcessive animations can lead to distractions rather than enhancements. Striking a balance is key.
Best Practices for Managing Routes
Managing routes efficiently in a React application is crucial for a seamless user experience. Best practices around routing not only enhance the performance of the application but also make the codebase more maintainable. Proper route management aids in preventing common pitfalls such as duplication, confusing navigation, and slow load times. In this section, we will discuss key considerations that can help developers design clean and efficient routing structures.
Maintaining Clean Navigation Structures
Maintaining a clean navigation structure helps both developers and users. A well-organized navigation layout can significantly improve usability. Itās like keeping your desk tidy; when everything has its place, itās easier to find what you need. Here are some strategies:
- Use Nested Routing: Create a hierarchy of routes to reflect the applicationās structure. It adds clarity and keeps related components grouped, reducing confusion.
- Employ Descriptive Naming: Route paths should be intuitive. Instead of using arbitrary names, choose names that clearly identify the function or content of the page, such as instead of just .
- Central Routing File: Keeping routes centralized helps in managing the routes easily. Having a single reference point simplifies debugging and modifications. A dedicated file for routing can save time and reduce errors when changes are needed.
By following these principles, developers can craft navigation flows that are both logical and straightforward, resulting in a more pleasant experience for end users.
"The navigation structure you build must be as easy to follow as a well-marked hiking trail."
Optimizing Performance with Lazy Loading
Performance is paramount in web applications. A sluggish application can drive users away faster than you can say "404 error." One effective way to enhance performance is lazy loading. This technique involves loading components only when theyāre needed, rather than all at once at the beginning.
Benefits of lazy loading include:
- Reduced Initial Load Time: Since only essential parts of the app load first, users can start interacting with the app quicker. This is especially important for data-heavy applications.
- Decreased Resource Usage: By loading components on demand, the application consumes fewer resources, optimizing overall performance.
Considerations for implementing lazy loading include:
- Code Splitting: Utilize React's built-in support for dynamic imports. With code splitting, large chunks of JavaScript can be executed only when the user navigates to specific routes.
- Error Handling: Always implement error boundaries when you're lazily loading components. Mistakes happen; ensuring that errors in loading do not crash the entire app is vital.
- Progressive Enhancement: Make sure that essential features are still accessible without all components loaded. This way, users can get at least some functionality as they wait for the rest to load.
By taking advantage of lazy loading, you not only enhance the user experience but also ensure your app runs smoothly even under heavy traffic. Itās a win-win scenario.
Debugging Routing Issues
Debugging routing issues is something that can be a real pain. However, itās critical for developers to understand how to tackle these challenges effectively. When you rely on React Router for navigation, it becomes your lifeline for managing the applicationās state and rendering components. If things go sideways, a poorly functioning router could lead to a frustrating experience for users, ultimately affecting the overall perception of the application. Understanding common routing errors and how to resolve them, as well as using the right tools for debugging, provides a solid foundation for maintaining robust applications.
Common Routing Errors and Solutions
Plenty of hurdles can crop up when dealing with routing in React applications. Here are some of the most frequent issues you might encounter:
- 404 Errors: A classic blunderāfor instance, if users enter a URL that doesn't match any defined routes, they'll hit a 404 error. Ensure that a catch-all route is configured to manage these routes gracefully. A component that provides a user-friendly message can help your users know they need to return home instead of leaving confused.
- Redirects Gone Awry: Sometimes you might intend to reroute a user, but due to misplaced routes or unhandled props, they find themselves in the wrong destination. Use the correct order in your route hierarchy to prioritize paths effectively.
- Mismatch in Paths: If component paths are incorrectly specified, the expected component may not render. Double-check your syntax; using a precise path, like , isnāt the same as due to JavaScript's case-sensitivity.
- State Loss During Navigation: Loss of state while navigating between routes can lead to data inconsistencies. To remedy this, consider using state management libraries like Redux or Context API to maintain state across routes seamlessly.
"A simple yet effective approach is to keep a close eye on the console logs. Often, the errors will be spelled out right there, making it easier to connect the dots."
By listening to these frequent faux pas, you can save both time and headaches.
Using React DevTools for Debugging
When it comes to debugging routing issues, React DevTools can be your best ally. It provides a comprehensive interface, making it straightforward to track down problems without pulling your hair out. Hereās how you can leverage React DevTools effectively:
- Inspect Components: From your applicationās component tree, you can dive in and see the props related to routing. Are they what youād expect? If not, you can trace back to your routing setup.
- View Router State: React Router maintains its own state, and DevTools allows you to check whether itās functioning as intended. Ensure that the state reflects current routes after navigation changes.
- Monitor Renders: Being able to see which components re-render can help pinpoint inefficiencies or accidental calls that may contribute to unwanted behavior in your routing logic.
- Debugging Performance: If your app feels sluggish, using React DevTools to profile your application can reveal whether unnecessary renders are happening as a result of routing paths being mishandled.
Utilizing these capabilities will not only help you in identifying and resolving routing bugs but also enhance your overall development efficiency.
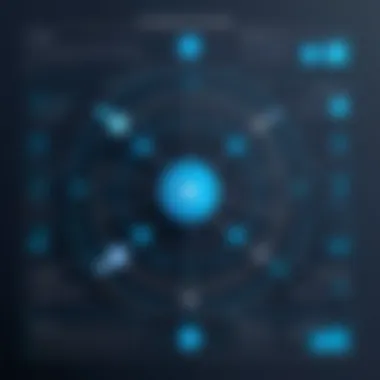
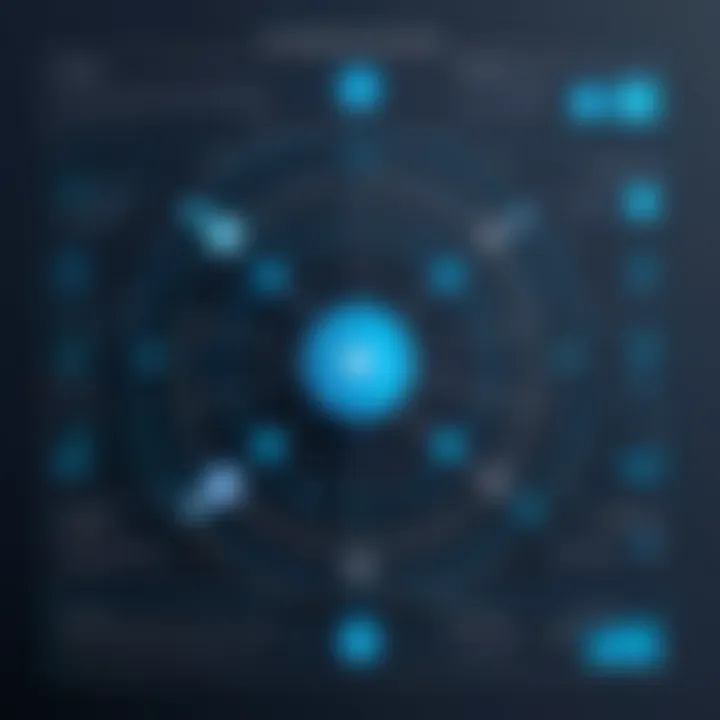
Whether itās a simple redirect issue or a more complex state management problem, being able to debug effectively can make your development process smoother. Understanding and employing these tools and solutions elevate your skill set in a significant way.
Case Studies and Practical Examples
When treating complex concepts like routing in React, it's often said that seeing is believing. Case studies and practical examples serve not just as helpful illustrations but as essential devices for mastering the inner workings of React Router. They enable learners to grasp theoretical knowledge in tangible ways, making the transition from concepts to actual implementation smoother.
Incorporating real-world applications into your learning journey engages the mind differently compared to reading abstract instructions. It allows you to visualize how routing mechanics play out in various contexts, whether you're navigating a simple app or a more complicated website. Let's dive into a couple of practical examples, breaking down how you can apply React Router in everyday development scenarios.
Building a Simple Multi-page Application
Creating a simple multi-page application with React Router showcases the power of structured routing in practice. Imagine a scenario where you have a website that includes a homepage, an about page, and a contact page. With React Router, itās straightforward to manage navigation between these various components, keeping your application organized and user-friendly.
You begin by setting up your project. After installing React Router, you can create three distinct components: , , and . Each component will house the content relevant to that particular route. Hereās a snippet for how you might set this up:
Here, sets up the routing environment, while is essential as it renders the first that matches the current location. With this structure in place, users can now navigate seamlessly between the pages with corresponding URL paths. Simple yet effective, this pattern sets the groundwork for more sophisticated routing techniques.
Implementing a Blog with Dynamic Routing
As complexity increases, so does the necessity for dynamic routing, especially in a blog application. When dealing with a blog, each post typically has a unique URL, making dynamic routing crucial for rendering specific content based on paths. This setup not only enhances the user experience but also improves SEO by providing meaningful URLs.
To illustrate, letās say you have a list of blog posts and want users to navigate to individual ones. You can create a route that accepts a parameter from the URL, such as a post ID or title. Hereās how you might tackle this with React Router:
In the above code, the component will fetch data based on the parameter in the URL, allowing for a unique view for each blog post. This dynamic routing approach means you can easily add, remove, or alter posts without changing the fundamental structure of your routes.
"Dynamic routing not only facilitates smooth navigation but also allows for content that resonates with the userās journey through your application."
By leveraging practical case studies like building a multi-page application and implementing dynamic routing for a blog, developers can firmly grasp the utility and strength of React Router. This hands-on approach also emphasizes best practices, making the learning process an engaging endeavor.
In closing, practical examples are not merely supplements to your learning; they are integral to understanding how React Router can effectively manage navigation in varying application contexts. Such insights not only facilitate current projects but also lay the groundwork for future endeavors as you traverse the landscape of React development.
Integrating with Backend Services
Integrating backend services into a React application is a crucial step in building dynamic and responsive applications. The relationship between the frontend and the backend is like peanut butter and jelly; one complements the other to create a complete sandwich. Without a proper connection, the user experience might feel flat or lacking in data. This section will illuminate the importance of integrating backend services, outlining how developers can efficiently manage data fetching and form submissions based on routes.
Fetching Data Based on Routes
When building a single-page application (SPA) with React Router, routing plays a key role in determining what data is presented at any given time. Fetching data based on routes is not just about grabbing information when the user navigates; it's about delivering the right content at the right moment.
For example, consider a blog application where each post has its own unique URL. When a user navigates to , it's important that your application fetches the details of the post corresponding to that specific ID.
To implement this, you can leverage React's useEffect hook alongside React Router to trigger data fetching when a route is activated. Hereās how you might structure that:
Here, gives access to the route parameters, allowing you to fetch data dynamically. This keeps your data and UI in sync, enhancing the user experience significantly. Users no longer need to refresh the page to see updated content; it's all just a smooth transition from one route to another.
Handling Form Submissions with Routes
Submitting a form in a React application is not merely about sending data to a server; it is about ensuring a seamless flow of information along with a robust user experience. Routes can facilitate different actions based on form submission, which is essential for applications requiring user input.
Think about a scenario where users need to create new posts. After filling out a form on a specific route, like , submitting this form might redirect them to to see the updated list of posts. This showcases how routes can significantly enhance usability.
Here is an example of handling a form submission that takes the user to a different route upon successful completion:
In this case, allows you to programmatically navigate to the posts page after a successful submission. This not only improves user navigation but also creates a more interactive experience, keeping users engaged with the application.
Integrating backend services effectively addresses challenges such as data management and dynamic user interactions. It lays a foundation for creating more sophisticated applications with a focus on user experience, which is invaluable for developers aiming to influence the digital landscape.
Future of Routing in React
As we look toward the horizon of web development, the role of routing in React is evolving rapidly. The future of routing in React isn't merely about creating paths for navigation; it's also about enhancing the user experience through increasingly sophisticated techniques and tools. Routing frameworks must adapt to the dynamic needs of applications today, balancing functionality with ease of use. This section will explore the new developments on the blocks, highlight their significance, and provide insights into how these changes will shape developers' approaches to routing in their upcoming projects.
Emerging Trends in React Development
In todayās fast-paced digital landscape, emerging trends often dictate how developers workflows and tools evolve. A few notable trends shaping React routing include:
- Server-side Routing: With the rise of frameworks like Next.js, server-side rendering (SSR) is changing the way we think about routing. It offers improved load times and better SEO capabilities. Developers are keen to leverage SSR in combination with client-side routing, leading to a smoother experience for users.
- Micro Frontends: This architectural style allows teams to develop independent sections of a larger app, which can have separate routing needs. As applications grow in complexity, micro frontends can enhance manageability and scalability by avoiding the pitfalls of monolithic architectures.
- Declarative Routing: This approach simplifies how developers define routes through a more intuitive syntax. Libraries are increasingly embracing a declarative paradigm, allowing for readable code that's easier to maintain.
- Routing as Code: By integrating routing logic directly into development processes and version control, teams can enhance collaboration. This trend aligns with the DevOps culture and encourages better design practices for routing.
"The future of routing embraces flexibility, aiming to adapt to the developer's needs while still providing an optimal user experience."
Predictions for React Router Evolution
As the landscape continues to shift under our feet, itās enlightening to consider what the future may hold for React Router. A few predictions that seem likely include:
- Increased Integration with State Management Libraries: As applications become more interactive and complex, tools will likely emerge that integrate routing more tightly with state management libraries like Redux or MobX. This could provide better control over when routes change in relation to state.
- Focus on Performance Optimization: The development community is now more aware of performance metrics. The React community will likely push for optimizations in loading times and memory usage, such as merging route definition with lazy loading mechanisms for components.
- Cross-framework Compatibility: As the web ecosystem becomes more interconnected, routing solutions might evolve to support different frameworks. This could mean that developers will have a universal routing layer applicable across various UI libraries, reducing the time spent switching contexts and learning different routing paradigms.
- Emerging React Standards: As React continues to mature, official guidance and standards around routing are likely to develop. This could include more comprehensive recommendations tailored for various application scenarios, streamlining the routing landscape.
In summary, the future of routing in React seems vibrant and holds the promise of enhanced capabilities. As developers gear up to tackle new challenges, understanding these trends and predictions will empower them to embrace innovative approaches, ensuring their apps remain relevant and user-friendly.
Closure
In this final section, we will underline the significance of wrapping up the knowledge and insights gained from this guide. Reflecting on the fundamental elements of routing using React Router can enhance how you structure user navigation. Not only does effective routing improve user experience, but it also streamlines the development process. By mastering React Router, developers can create fluid single-page applications that present users with organized and intuitive navigation.
Recap of Key Takeaways
To synthesize our discussions, here are some pivotal takeaways that should linger in your mind as you continue to explore React development:
- Understanding of Routing: Routing is not just about URLs. It dictates how users interact with various parts of your application.
- Iterate on Best Practices: Remember, maintaining clean navigation structures and optimizing with lazy loading can significantly enhance performance.
- Real-world Implementation: The case studies we've reviewed demonstrate the power of dynamic and nested routing in practical scenarios.
- Future Trends: With the rapid evolution of technology, staying updated on trends and potential developments in React Router will position you ahead of the curve.
Encouragement for Further Learning
As you stand on the cusp of your journey into the world of React Router, consider this an open invitation to delve deeper. The landscape of web development is ever-evolving. There are ample resources available for honing your skills. Engaging with communities on platforms like Reddit or exploring newer materials on websites such as Wikipedia can provide additional perspectives and insights.
- Dive into Advanced Topics: Look into more complex routing scenarios and integrations.
- Explore New Libraries: Don't shy away from experimenting with additional libraries that complement React Router.
- Build Projects: Practical experience always outweighs theory. Consider tackling projects that impose real-world challenges, such as integrating with a backend or optimizing for mobile.