A Comprehensive Guide to C++ Programming Concepts
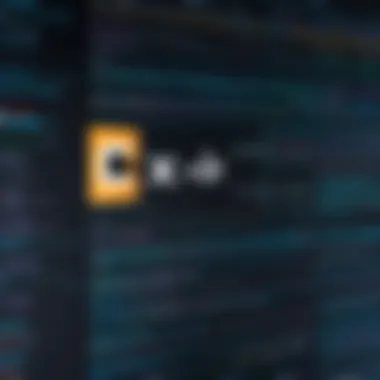
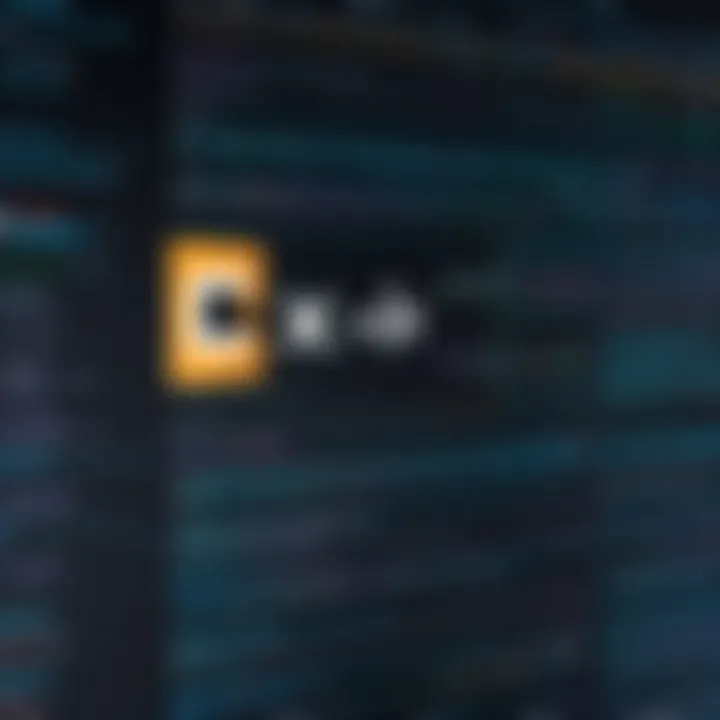
Foreword to Programming Language
Programming languages are the backbone of software development, laying out a framework for instructions that computers can understand. Among these languages, C++ stands tall as one of the most pervasive and influential, shaping the way we develop applications today.
History and Background
C++ was created by Bjarne Stroustrup at Bell Labs in the early 1980s. It evolved from C, a well-respected language renowned for its performance and efficiency. However, Stroustrup noticed a gap; he wanted a language that supported object-oriented programming while retaining C's powerful features. With that thought, C++ was born, and it rapidly gained traction in a variety of fields.
Features and Uses
C++ is diverse in its capability, blending low-level and high-level features. It supports various programming styles, including procedural, object-oriented, and even generic programming.
Some prominent features include:
- Object-oriented programming: Enabling code reusability through inheritance and polymorphism.
- Templates: Allowing for generic programming, thus writing code that can operate with various data types.
- Standard Library: Encompassing a powerful set of classes and functions that enhance development efficiency.
Applications of C++ span a broad spectrum, from game development using engines like Unreal Engine, to systems software, embedded programming, and high-performance applications in fields such as finance and telecommunications.
Popularity and Scope
C++ continues to stand out in the competitive landscape of programming languages, firmly establishing its place in academia and industry. According to surveys, it's consistently ranked among the top programming languages, a testament to its enduring relevance. Many companies, such as Google and Microsoft, rely on C++ for their performance-critical software solutions. Its capability in handling complex systems keeps it in demand, proving that learning C++ can be a significant asset in a programmer's toolkit.
"C++ is a language that genuinely combines high-level and low-level programming - an invaluable skill in the modern tech landscape."
As we progress through this article, we will uncover the basic syntax, advanced techniques, practical examples, and resources that can help students and aspiring programmers delve deeper into the world of C++.
Preface to ++ Programming
C++ programming stands as a foundational pillar in the world of software development. With roots embedded in the late 1970s, C++ swiftly transformed from a language for system software to a versatile tool used in a myriad of industries. This section aims to shed light on why understanding C++ is crucial for both budding programmers and seasoned developers alike. The nuanced features and robust capabilities of C++ make it a gold star in languages, particularly when it comes to performance-critical applications.
History and Evolution
To appreciate C++, one must traverse back to its inception. Developed by Bjarne Stroustrup at Bell Labs, C++ emerged as an enhancement to the C language, integrating object-oriented features that enabled programmers to design more manageable and scalable applications. These innovations allowed for better code organization, making it more efficient and easier to debug.
As the years rolled on, several updates birthed new standards like C++98, C++03, C++11, and C++14, each smoothing out kinks while introducing powerful new capabilities. These iterations echoed the voice of a dynamic programming culture—always adapting, never stagnating. Today, C++ boasts features like auto type deduction, move semantics, and lambda functions that showcase its inclination toward contemporary programming needs.
"C++ is not just a programming language; it’s a way of thinking about programming."
Core Principles of ++
Understanding C++ requires grasping its core principles which set it apart from many other languages. It combines both high-level and low-level programming features, giving developers the unique ability to tailor their applications precisely according to the hardware capabilities. This duality is expressed through four main principles:
- Object-Oriented Programming (OOP): This principle allows for encapsulating data and functions into cohesive units, making programs more robust and easier to manage.
- Data Abstraction: C++ promotes hiding complex realities while exposing only relevant parts. This leads to simplified program design and greater focus on the essential core.
- Inheritance: A powerful feature that enables the creation of new classes based on existing ones, thus fostering code reusability and a natural hierarchy in application design.
- Polymorphism: It allows treating different data types through a common interface. Essentially, you can use a single function to work with different types of data, thus reducing redundancy.
These core principles directly influence C++’s performance and adaptability, making it an ideal choice for various applications ranging from game development to system programming. Understanding these foundations will prepare aspiring programmers for a fruitful journey into the vast landscape of C++.
Setting Up the Development Environment
Setting up a development environment is a key first step in programming with C++. It often serves as the stage where your coding journey will either flourish or falter. A well-configured environment can streamline your coding experience, enhance productivity, and minimize errors. The right tools make coding smoother, and they also empower you to focus on writing effective code rather than spending time wrestling with setup issues.
One must consider several elements when setting up the environment. Choosing the correct tools, understanding the differences between compilers, and installing an IDE that fits your needs are crucial steps. Without a good grip on these elements, even seasoned programmers might find themselves hitting walls. Here are some considerations and benefits that arise from getting this aspect right:
- Efficiency: A setup that is tailored to your needs can drastically improve your coding speed. With everything at your fingertips, you won't waste time rummaging around for the right tools.
- Error Reduction: A properly configured environment often helps catch errors early in the coding process. Modern IDEs and compilers will highlight issues before you even run your code.
- Learning Curve: If you're just starting, having a simplified and intuitive setup lowers the barrier to entry. You can focus on learning C++ rather than getting caught in the weeds of configuration.
In this section, we'll explore two fundamental parts of setting up your C++ development environment: selecting the right compiler and installing Integrated Development Environments (IDEs). Both play critical roles in your programming experience.
Choosing the Right Compiler
When diving into C++, the compiler you choose can significantly impact your development workflow. Compilers transform your C++ code into executable programs, and different compilers come with their own sets of advantages and disadvantages.
GCC, the GNU Compiler Collection, is commonly favored among many programmers due to its open-source nature and extensive support for C++. However, if you are using Windows, Microsoft Visual C++ offers deep integration with Windows and robust debugging tools.
Here are some considerations to factor in:
- Performance: Certain compilers optimize code better than others. For example, some may produce faster executables, while others might offer quicker compile times.
- Compatibility: If you're planning on deploying your software across different systems, ensure your compiler is compatible with all target platforms.
- Support: Having access to a strong community or vendor support can be immensely beneficial, especially when you run into pitfalls or have questions during your development process.
A practical way to evaluate compilers is to try a few simple coding tasks on different platforms and observe the differences. You may find that one compiler fits your needs better than others.
Installing IDEs for ++
An Integrated Development Environment, or IDE, combines all the necessary tools you need in one place. This includes a code editor, a debugger, and sometimes even a compiler. For C++, there are numerous IDE options, ranging from lightweight text editors to full-fledged development environments.
Among the leading IDEs for C++ include Code::Blocks, Eclipse CDT, and Microsoft Visual Studio. Each comes with unique features that accommodate various programming styles and preferences. Here's a closer look at what you might consider:
- User Interface: A user-friendly interface can make life a lot easier for beginners. Look for IDEs that offer clear layouts and intuitive navigation.
- Debugging Capabilities: Debuggers integrated in IDEs can help you identify and fix bugs faster. Look for ones that provide real-time debugging and easy navigation between code and error messages.
- Additional Features: Some IDEs come with plugins or add-ons that can extend functionality. This could include features like version control, static analysis, or enhanced build tools.
Here’s a brief code snippet outlining how you might set a basic structure in an IDE:
This simple program will print "Hello, World!" to the console, but you can only run it effectively if your IDE is set up correctly to compile and execute C++.
As you embark on your journey in C++, taking time to establish a solid development environment will pay dividends down the road. It may seem tedious now, but in the long run, it allows you to create a productive and enjoyable coding experience.
Essential Syntax and Structure
Understanding the essential syntax and structure of C++ is akin to learning the grammar of a new language. This foundation is crucial for anyone embarking on their programming journey, as it influences not only the way code is written but also how effectively that code runs. In C++, the syntax dictates the way in which statements are formed, how data is manipulated, and how the commands are executed. This section lays out the groundwork you’ll need to become proficient in C++ programming.
Basic ++ Syntax
At its core, C++ syntax is a set of rules that dictates how programs are written. The syntax includes the ways in which declarations, expressions, statements, and comments are structured. For instance, every statement ends with a semicolon, acting like a stop sign that denotes the end of a command.
Here’s a simple example of how basic syntax looks in practice:
In this code snippet, is an inclusion directive that brings in standard input-output stream libraries. The function is where program execution begins. The outputs text to the console. Each command is neatly wrapped up with a semicolon, illustrating a fundamental aspect of C++ syntax.
Data Types and Variables
The next important element to grasp is data types and variables. In C++, a variable is like a container that holds data. However, it’s imperative to declare the data type first. Each data type has its own properties and rules:
- int: Represents integer values.
- float: For numbers with decimals.
- char: Represents a single character.
- bool: Can either be true or false.
Declaring variables correctly is crucial, as it enables the compiler to allocate memory appropriately. Here’s a simple example:
In this code, we declare an integer variable named and assign it the value of 25. Understanding this is vital as you begin to manipulate and interact with different types of data in your programs.
Operators in ++
Operators play a significant role in programming by allowing you to perform operations on variables and values. In C++, these can be categorized into several types:
- Arithmetic Operators: Such as , , , , which perform mathematical calculations.
- Relational Operators: Include , , , ``, which are used to compare values.
- Logical Operators: Such as , , , used to make decisions based on conditions.
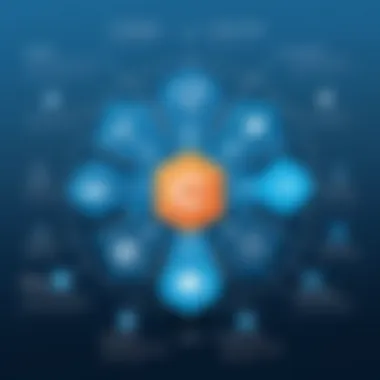
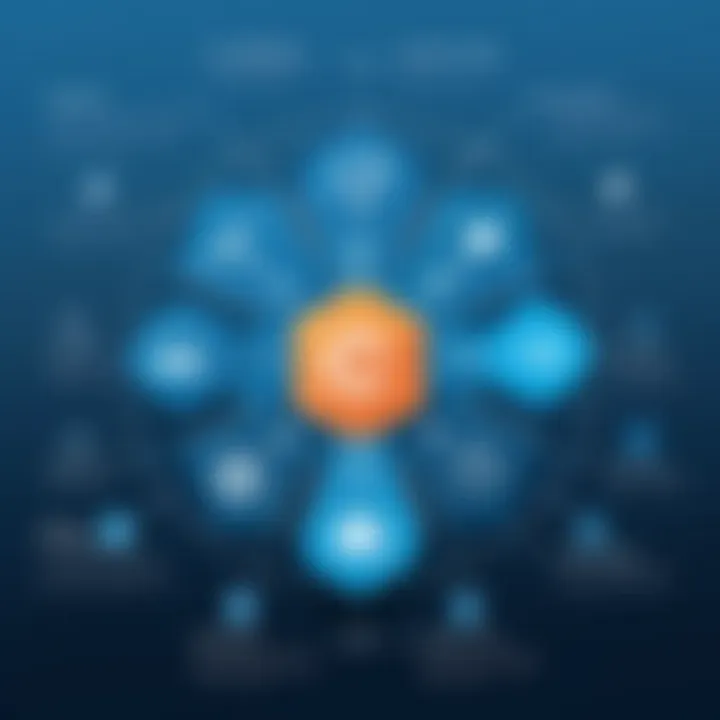
For instance, to add two integers in C++, you might write:
The above example demonstrates using the operator to add two integers, which showcases how smoothly these operators blend into the syntax of C++. Understanding these operators not only makes coding easier but also enhances your ability to develop complex logic within your programs.
Key Takeaway: Mastering the basics of syntax, variable declaration, and operators sets the stage for further exploration into the depths of C++ programming. Without this foundation, one may struggle with more advanced concepts and applications.
Control Structures
Control structures are essential in programming, especially in C++. They dictate the flow of execution in a program, making decisions based on conditions or repeating tasks until certain criteria are met. Without these structures, accomplishing complex tasks would be like trying to navigate through a maze with no signs or directions.
Conditional Statements
Conditional statements allow a program to execute different blocks of code based on specific conditions. This means that the program can make decisions, and not just follow a set path like a train on tracks. Think about it: you wouldn’t want your program to act the same way regardless of user inputs or changing circumstances. Conditional statements include constructs like , , and .
For example, let’s say you’re writing a program that grades students based on their scores. You wouldn’t want everyone to get the same grade even if they performed differently. Here’s a sample snippet of C++ code:
In this code, the program addresses different scores and assigns appropriate grades. If you input a score of 85, it will execute the condition for a grade of B. Just like that, conditional statements help programmers to efficiently handle multiple scenarios without convoluting the code.
Looping Constructs
Looping constructs are another cornerstone of control structures. They allow a block of code to run repeatedly until a certain condition is met. This can save time and effort, especially when dealing with repetitive tasks.
Common looping constructs in C++ include , , and . Imagine wanting to print a list of even numbers up to 100. Instead of writing 50 individual lines of code (which is quite tedious), you can use a loop:
Here, the loop iterates from 0 to 100, incrementing by 2 each time. This method eliminates redundancy and makes your code cleaner, which is always a good practice.
Functions in ++
Functions are a cornerstone of programming in C++. They serve as the building blocks for creating modular and efficient code. As you dive into the world of C++, understanding the role and functionality of functions is essential. Functions allow developers to break down complex problems into smaller, manageable chunks. This not only improves readability but also simplifies debugging and maintenance of the code.
Using functions effectively can enhance productivity. Programmers can write a function once and call it multiple times throughout their code, which promotes code reuse. Additionally, functions help to abstract away details, allowing users to focus on what the function does rather than how it does it. This aspect of C++ becomes particularly valuable when designing large applications where maintaining organization and clarity is paramount.
Defining Functions
Defining a function in C++ involves specifying its return type, name, and parameters (if any). The basic syntax resembles something like this:
For instance, if you want to create a simple function that adds two integers and returns the result, you would write:
It's crucial that the return type of the function matches the type of value you're returning. Otherwise, you may run into various issues, like mismatched types or compilation errors. Functions can also be defined without parameters and can return void if no value is needed.
Function Overloading
Function overloading is a unique feature of C++ which allows multiple functions to have the same name with different parameters. This ability to overload functions enhances flexibility and enables developers to create more intuitive interfaces.
Here’s a quick example:
In this case, we have two functions named , one for calculating the area of a circle and the other for a rectangle. When calling , the compiler decides which version to execute based on the given parameters. This saves time and effort as you don’t have to remember numerous function names.
Recursion in ++
Recursion is when a function calls itself to solve a problem. It’s a compelling way to simplify code that would otherwise require complex loops. However, writing recursive functions demands careful attention to detail. A well-defined base case is crucial to prevent infinite loops.
A classic example is the calculation of the factorial of a number. Here’s how a recursive function might look:
In this example, the function calls itself until it reaches the base case of 1. While recursion can simplify the logic, it’s essential to understand the stack memory implications as deep recursion can lead to stack overflow errors.
Learning how to work with functions enhances not only your programming efficiency but also your ability to think logically about problems. Mastering these concepts is crucial for anyone aspiring to excel in C++ programming.
Object-Oriented Programming Concepts
Object-Oriented Programming (OOP) serves as a cornerstone in the landscape of software development, especially when it comes to C++. The paradigms and principles underlying OOP enable developers to build more manageable and scalable applications. This section explores the essential aspects of OOP in C++, focusing primarily on classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
OOP promotes an organized structure that mirrors real-world entities, thus simplifying complex problem-solving. By encapsulating data and functions, programmers can create modular code that is easier to maintain and extend. Utilizing OOP principles leads to better code reuse, improved collaboration, and increased efficiency in the development process.
Classes and Objects
In C++, a class acts as a blueprint for creating objects. An object is an instance of a class and represents a specific entity with attributes and behaviors. When defining a class, a programmer essentially describes the data structure and the operations that can be performed on it.
For example, consider a class named :
This code illustrates the foundational elements of a class: properties (color and model) and methods (functions to set properties and display them). This encapsulation allows the class to operate independently, thus encapsulating functionality within a well-defined boundary.
Inheritance and Polymorphism
Inheritance is a mechanism where a new class derives properties and behaviors from an existing class, known as the base class. This principle promotes code reuse and establishes relationships among classes. For instance, a class can inherit from the class. It can introduce additional features without altering the original class. Here's a simple illustration:
On the other hand, polymorphism allows methods to do different things based on the object that it is acting upon. This can be achieved either through method overriding in inheritance or by using function overloading. An implementation can look like this:
In this case, calling the method on a object will output . Such flexibility and functionality make it clear why inheritance and polymorphism are vital aspects of C++.
Encapsulation and Abstraction
Encapsulation combines data and methods into a single unit, the class, while restricting access to some of the object's components. This means that the internal representation of an object is hidden from the outside, exposing only what is necessary. This not only strengthens security but also makes the interface simpler to interact with.
Abstraction takes this a step further by focusing on the essential qualities of an object while hiding the irrelevant details. An example can be seen in the concept of a interface that allows different types of vehicles, whether a bike or a bus, to be treated similarly without exposing their specific implementations.
Here’s how a simple abstraction might look:
In this abstraction, we create a contract that all vehicles must follow, while the specific details of how each vehicle accelerates remain hidden within its respective class.
In summary, understanding Object-Oriented Programming Concepts in C++ is of paramount importance for anyone aiming to master the language. Classes and objects provide a structured foundation for building applications, while inheritance and polymorphism facilitate code reuse and adaptability. Encapsulation and abstraction contribute to data integrity and simplify interactions. Each of these elements acts as a building block to create robust and efficient software solutions.
Templates and Standard Template Library (STL)
Understanding templates and the Standard Template Library (STL) is crucial for any C++ programmer. These components not only enhance code efficiency but also bolster reusability, making the process of development smoother and more coherent. Templates enable developers to write functions and classes that work with any data type, leading to cleaner code and less duplication. The STL complements this by providing a rich set of ready-to-use classes and functions that simplify common tasks.
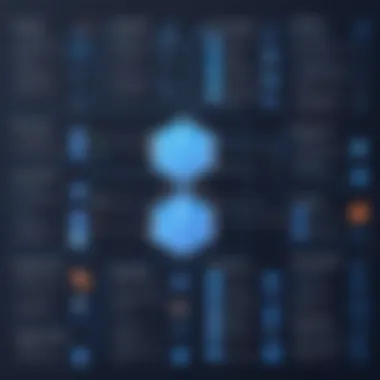
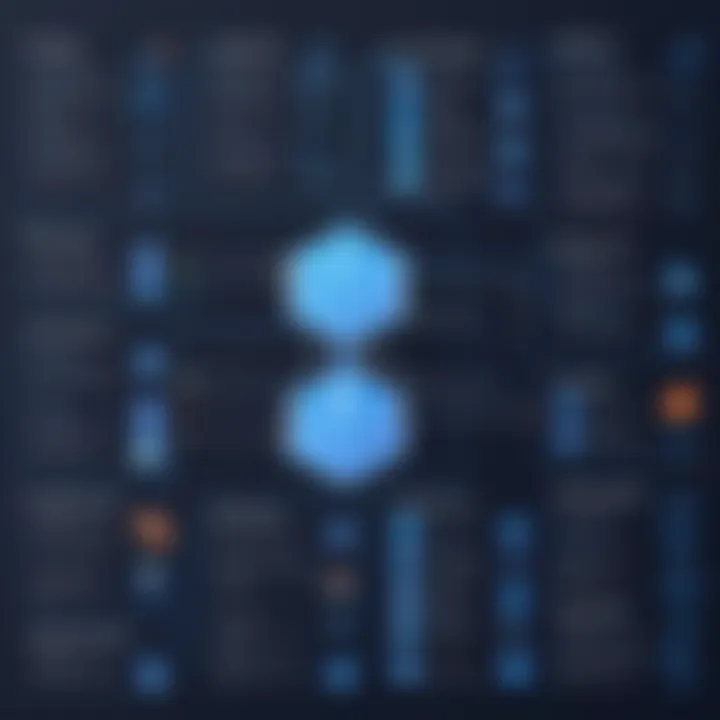
Preface to Templates
At its core, a template in C++ allows the creation of a blueprint for functions or classes without specifying the exact data type initially. It acts as a placeholder. This means you can write a single function or class to work with different types of data.
For example, if you have a need to create a function that finds the maximum of two values, you can design a template that can handle both integers and floats—or even user-defined types:
This template approach means you avoid redundancy; you need only one implementation, and the compiler generates the necessary types when you use it.
Using STL Containers
The STL is a powerful suite in C++ that includes various data structures known as containers. These containers store collections of objects and help manage data efficiently. The most common types of containers include:
- Vector: A dynamic array that can grow in size automatically.
- List: A doubly linked list for efficient insertions and deletions.
- Map: An associative container that stores elements formed by a combination of a key value and a mapped value, essentially a dictionary.
Using STL containers can lead to a significant reduction in code complexity. Let’s look at an example to illustrate a simple usage of a vector:
In this snippet, elements can be added seamlessly, and it’s quite elegant compared to manually managing an array.
Algorithms in STL
An often-underappreciated facet of the STL are the algorithms. These are pre-built functions that provide commonly used operations on data within the STL containers. They range from sorting and searching to transforming data.
For instance, the algorithm can sort the elements of a vector effectively:
Furthermore, the use of algorithms promotes the concept of generic programming, where one can pass any compatible container and apply the same operations. This leads to significant flexibility in code design.
In summary, the importance of templates and the STL cannot be overstated for anyone looking to excel in C++. They offer robust strategies for writing clean, efficient, and reusable code. As one delves deeper, the nuances of these concepts knit together the fabric of proficient C++ programming.
"C++ templates and STL are not just tools of the trade; they are bridges to writing elegant and efficient code that speaks volumes about your programming acumen."
By employing these skills, programmers can elevate their C++ projects, be it for performance needs or code maintainability.
File Handling in ++
File handling is a pivotal aspect of programming that allows developers to interact with files residing on storage media. In the context of C++, this means both reading from and writing to files, which can be crucial for various applications ranging from simple data logging to complex data manipulation tasks. The essence of file handling lies in its ability to enable programs to store state or retrieve data even after execution has ended. Thus, understanding how to effectively manage files can significantly enhance the functionality of your C++ programs.
First off, keeping data persistent is crucial. When you write an application that needs to maintain user preferences, logging error information, or even saving state in a game, file handling comes to the forefront. It is the mechanism through which applications can communicate with external data, making it a core skill for any C++ programmer aiming to create robust applications.
Moreover, the benefits of mastering file handling extend beyond mere technical proficiency. Being adept in file operations can lead to better organization of data, improved efficiency, and ultimately a smoother user experience. However, engaging in file handling also brings a set of considerations, such as managing different file formats, ensuring data integrity, and handling errors like file not found or access permissions. These challenges necessitate a proactive approach to understanding the inner workings of file input and output in C++.
Reading from Files
When it comes to reading from files in C++, the library comes into play, specifically the class. This class facilitates the opening and reading of files, enabling developers to extract the data they need with ease.
To dive deeper, here’s how reading from a file typically works:
- Opening the File: Use to create an input file stream object and open the desired file.
- Reading Data: Employ various methods such as for strings or the extraction operator () for other data types, depending on the type of content in the file.
- Handling Errors: Always check if the file opens successfully and remain prepared to deal with unexpected end-of-file conditions or read errors.
For example, the following code demonstrates a basic approach to reading data from a text file:
This snippet opens a file named , reads each line until the end of the file, and prints it to the console. Notably, proper error handling ensures that your program can manage scenarios where the file might be missing or unreadable.
Writing to Files
Writing to files in C++ can be accomplished using the class, which allows you to create an output file stream. Through this, programs can generate new files or append content to existing ones, acting as a bridge between your application’s data and persistent storage.
Here’s a rundown of how to effectively write to a file:
- Opening the File: You can create a new file or open an existing file for writing. Be sure to handle the possible situations where the file could be read-only or if it doesn’t exist.
- Writing Data: Utilize the insertion operator (``) to send data to the file stream.
- Closing the File: It’s good practice to close the file after writing to release the resources.
Consider the following C++ code snippet, which illustrates how to write to a file:
This example creates and writes a couple of lines to it. Again, the inclusion of proper error handling is key to ensuring a smooth execution.
Important Note: Remember to always validate file operations. It’s not just about getting it right; it’s about getting it safe.
Error Handling and Debugging
In the world of programming, errors can creep in like unwelcome guests—unpredictable and often troublesome. Error handling and debugging are critical skills for C++ programmers that ensure software runs smoothly and effectively. Without them, coding becomes a guessing game fraught with missed opportunities for enhancement and optimization.
Understanding errors is the first step toward becoming adept in handling them. There are various types of errors in C++. Some are syntax errors, which arise when the code does not conform to the grammatical rules of the language. Others are logical errors, which occur when the program runs but does not produce the expected result. Runtime errors fall somewhere in between, surfacing during execution and causing programs to crash or behave unexpectedly. By learning how to identify these errors, programmers can streamline their code and enhance functionality.
Utilizing efficient error handling not only guards the application against abrupt failures but also boosts overall user experience. In poorly managed applications, a single error can lead to a complete system shutdown. However, with proper exception handling, the application can gracefully alert the user to the issue and continue operating, minimizing disruption.
Understanding Exceptions
Exceptions are a fundamental concept in error handling in C++. When an error occurs within a program, an exception is raised to signal that something has gone awry. Think of it as a fireworks display, where the sudden explosion of color alerts you to the problem. Programmers can utilize exceptions to manage errors efficiently without cluttering the main logic of their programs with error-checking code.
Here’s a basic breakdown of how exceptions work in C++:
- Throwing an Exception: This is the act of generating the exception when an error condition arises, using the keyword.
- Catching an Exception: This technique involves using the and keywords to handle the exception gracefully without crashing the program.
- Propagation: If an exception goes uncaught, it propagates back up the call stack, which can lead to program termination if not properly handled.
Here is a simplified code sample to illustrate:
This example exhibits a simple throw and catch mechanism. In it, when generates a runtime error, it’s caught in the function and handled appropriately, avoiding a crash and maintaining control over the program’s flow.
Debugging Techniques
Debugging is the art of tracking down bugs, and in C++, it becomes imperative for all developers. There are several effective strategies that one can employ when searching for these elusive errors:
- Print Statements: Sometimes, the simplest tactic is to use print statements to display the state of variables at various points in the program, helping locate where things begin to go wrong.
- Debugger Tools: Many IDEs offer built-in debugger tools that allow programmers to step through the code line by line. This method gives a clear view of variable values and program flow, which can illuminate hidden bugs.
- Unit Testing: Writing tests for your functions can unveil bugs right away. It’s a proactive approach that exposes issues under varying conditions.
- Static Analysis Tools: These can analyze source code without executing it. They flag potential errors or code smells that might lead to bugs down the road.
As one ventures further into C++, mastering error handling and debugging isn’t merely beneficial; it's essential. These tools create resilience in your software design, increasing reliability and user satisfaction. To stay informed about best practices and updates in tools, resources like Wikipedia and Reddit can be invaluable.
Proper error handling is like building a strong seatbelt. It won’t prevent accidents, but it will ensure you walk away relatively unscathed when things go sideways.
Best Practices in ++ Programming
When diving into the world of C++, it's crucial to grasp the best practices that can elevate your coding skills from mediocre to exceptional. These practices aren't mere suggestions; they form the backbone of robust, maintainable, and efficient programs. As C++ is a powerful language, neglecting best practices could lead to unmanageable code, hinder collaboration, and even introduce hard-to-track bugs.
Key elements to consider in best practices include code readability, consistency, efficiency, and organizational structure. By adhering to these principles, developers ensure that their code is not only functional but also clear to other programmers, which is essential in any collaborative environment.
Code Readability and Maintenance
Code readability is often the hallmark of effective programming. Well-structured and clear code can save time in the long run—both for the original creator and for anyone who might handle the code later on. Imagine pouring hours into writing a piece of software, only to find that a simple bug requires hours of deciphering your own work.
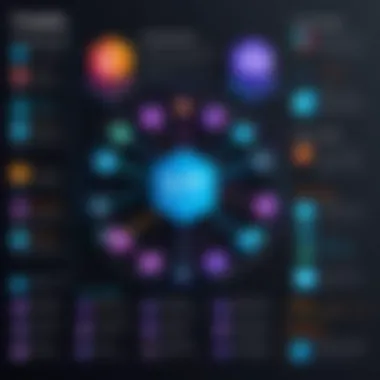
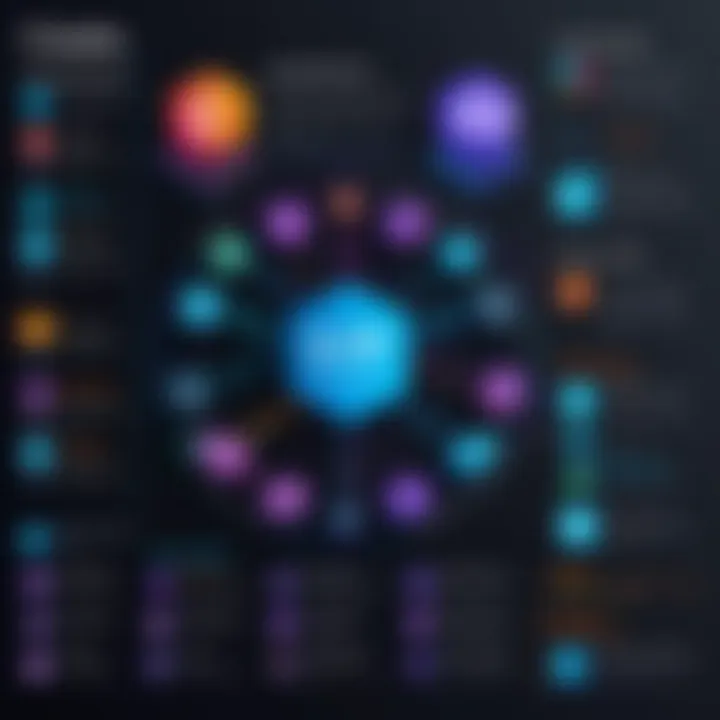
Here’s why code readability is vital:
- Easier Debugging: When code is written clearly, it becomes simpler to locate and fix issues. This can drastically speed up the debugging process, allowing developers to pivot quickly when problems arise.
- Effective Collaboration: Teams thrive on clear communication, and code is no exception. When your code is readable, team members can understand it without needing a translator.
- Future-proof Code: A project may evolve over time, and what seemed clear today might not be as straightforward weeks down the line. Code that is easy to read can adapt to changes with minimal strain.
Here are some tips to enhance code readability:
- Consistent Naming Conventions: Adopting a set naming structure (like CamelCase or snake_case) helps maintain consistency. For instance, using descriptive variable names such as instead of provides clarity.
- Indentation and Spacing: Properly formatting code with consistent indentation makes it visually organized. A well-spaced code block is easier for the eye to navigate.
- Logical Segmentation: Breaking down code into functions or classes enhances its structure. Each function should have a clear purpose—this way, readers can follow the logic without getting lost in the weeds.
Commenting and Documentation
While code should ideally be self-explanatory, comments serve as a safety net to clarify complex logic or decisions that might not be immediately apparent. Effective commenting practices can bridge the gap between current and future developers.
Important aspects to consider when commenting include:
- Clarification of Intention: Explaining why certain choices were made can be more critical than what the code does. Understanding the rationale behind logic can guide future modifications.
- Documentation of Interfaces: Comments should outline how functions or classes interact. For example, documenting what parameters a function accepts—and their types—is invaluable for future users.
- Avoid Over-commenting: While comments are helpful, they should not clutter the code. Simple and straightforward remarks are best. If a line of code is doing what it suggests, it often doesn’t need a comment. If it’s complicated, a comment is warranted.
You might find comments formatted like this helpful:
"Good code is its own best documentation."
By adhering to effective commenting and maintaining a focus on readability, C++ developers can not only improve their own work but also contribute positively to their teams. As software evolves, well-commented and legible code can save countless hours of headaches—a vital practice in today's fast-paced tech landscape.
Through these best practices, you’ll strengthen your C++ skills, making your journey through coding more productive and enjoyable. Whether you’re working solo or within a team, these principles lay the groundwork for success in software development.
Advanced ++ Features
Advanced C++ features play a critical role in enhancing the capabilities of the language, enabling developers to write more efficient and expressive code. Understanding these features isn't just about adding extra tools to the toolbox; it involves grasping ideas that can significantly influence the way programming tasks are approached. This section focuses on two key aspects: smart pointers and lambda expressions, each offering unique advantages and considerations.
Smart Pointers
Smart pointers simplify memory management in C++. Unlike traditional pointers, smart pointers manage the lifetime of an object automatically. They help eliminate common problems such as memory leaks, dangling pointers, and double deletions. In C++, three primary smart pointers are commonly used: , , and .
- : This type of smart pointer maintains exclusive ownership of an object. When a goes out of scope, it automatically deletes the associated object. This frees resources without needing a manual delete, reducing the risk of leaks. Here’s a quick example:
- : This allows shared ownership of an object. Multiple instances can point to the same resource, and the object is destroyed only when the last is destroyed. However, this can lead to cyclic references if not handled properly, requiring careful consideration during design.
- : This smart pointer is used alongside to avoid reference cycles. A does not affect the reference count of the shared object, thus preventing it from being deleted while still allowing access.
Using smart pointers can lead to cleaner code and enhanced safety, making them essential for modern C++ programming.
Lambda Expressions
Lambda expressions are a powerful feature in C++, introduced in C++11. They offer an elegant way to define anonymous functions, which can be used to encapsulate behavior that can be passed around or stored in variables. This is particularly useful in scenarios involving algorithms or event handling, where defining separate functions may add unnecessary complexity.
A basic lambda expression looks like this:
In this example, is a lambda function that takes two integers and returns their sum. The syntax consists of:
- Capture list: Specifies which variables from the surrounding scope are accessible within the lambda.
- Parameter list: Similar to normal functions, defines inputs.
- Return type: Optional, inferred by compiler.
- Function body: Contains the logic.
For instance, you can leverage lambda functions with standard algorithms:
This usage shows how lambda expressions contribute to concise code. They allow for inline function definitions, reducing the need for verbose function declarations. Moreover, because lambdas can capture state, they enable closures, facilitating functional programming paradigms in C++.
In essence, advanced C++ features like smart pointers and lambda expressions not only improve code safety and efficiency but also empower developers to embrace modern programming paradigms that streamline the development process.
In summary, embracing these advanced features is essential for anyone looking to master C++. They embody the language's evolution, providing powerful tools that simplify common challenges, ultimately contributing to cleaner and more maintainable code.
Real-World Applications of ++
C++ has carved its niche across various domains, proving itself to be not just a programming language, but a versatile tool for problem-solving and efficiency. The real-world applications of C++ showcase its weaknesses and strengths and serve as practical considerations for developers and organizations alike. The language's performance, scalability, and the ability to handle low-level operations make it particularly valuable in sectors that require robust and efficient solutions.
"C++ is not just about writing code; it is about crafting solutions that are efficient and powerful."
++ in Game Development
Perhaps one of the most notable applications of C++ is in game development. The gaming industry, with its demand for high-performance graphics and real-time processing, benefits enormously from C++. Major game engines like Unreal Engine and Unity incorporate C++. The language's ability to enact complex algorithms quickly while maintaining performance is crucial. Here are some key points highlighting why C++ is favored in gaming:
- Performance: C++ allows developers to write code that is optimized for speed. This is crucial in gaming, where every millisecond counts, especially in fast-paced environments.
- Memory Management: Controls over memory allocation and deallocation offers developers fine-tuning capabilities that are crucial in ensuring smooth gameplay.
- Object-Oriented Programming: The principles of classes and objects in C++ align well with the architecture of complex game systems, enabling code reuse and personality in character development.
However, game development in C++ also comes with its challenges. The language has a steep learning curve, and debugging can be more complex compared to higher-level languages. Yet, for those who master it, the rewards in terms of performance and capabilities are substantial. Notable titles like "Call of Duty" and "World of Warcraft" are testaments to the power of C++ in bringing intricate worlds to life, pushing the boundaries of technology and creativity.
++ for Systems Programming
Another significant area where C++ finds its footing is systems programming. The language is commonly used in developing operating systems, device drivers, and embedded software. Here’s why C++ plays a crucial role in this domain:
- Low-Level Manipulation: C++ provides the ability to interact with hardware, setting it apart from many higher-level languages. This feature allows for the creation of efficient systems-level software.
- Resource Management: Effective use of memory and resource management is key in systems programming, and C++ allows developers to manage hardware resources carefully.
- Compatibility and Portability: C++ code can run on various platforms with minimal alterations, which is invaluable for systems programming where hardware configurations vary.
Though C++ might not be the only option for systems programming, its ability to provide a balance between high-level abstractions and low-level control has made it a preferred choice for many programmers worldwide. Established operating systems like Windows and UNIX have parts written in C++, drawing on its efficiency and reliability.
The Future of ++
As we venture deeper into the realms of software development and technology, it becomes increasingly evident that C++ continues to hold a significant place in the programming landscape. Even as new languages pop up like daffodils in spring, C++ maintains its relevance thanks to its performance, efficiency, and a vast ecosystem that continues to evolve. This section examines the trends and innovations shaping the future of C++, highlighting its enduring importance.
"C++ is not just a programming language; it's a foundation for numerous applications and solutions in the tech domain."
Current Trends and Innovations
C++ is undergoing notable advancements, paralleling changes in the technology industry. One of the most significant trends is the integration of modern C++ features, primarily introduced from C++11 onwards. These changes, like smart pointers and lambda expressions, enable developers to write cleaner and safer code. A key point to recognize is that these updates are not just about syntax; they are about improving developer experience and system performance.
Another trend is the continuous enhancement of the Standard Template Library (STL), which is getting richer and more efficient. Libraries such as Boost and Qt are pushing boundaries, offering sophisticated functionalities that simplify programming tasks.
Moreover, with the rise of high-performance computing (HPC), C++ remains a prime choice for performance-intensive applications. Industries dealing in simulations, real-time processing, and extensive data computation are heavily leaning on C++ due to its unmatched speed and flexibility. It’s no surprise that many tech giants like Google and Microsoft invest significantly in C++ optimization and tooling.
++ in Emerging Technologies
As businesses pivot towards new technologies like artificial intelligence, machine learning, and the Internet of Things (IoT), C++ proves to be a pivotal player. The language's inherent efficiency makes it a natural ally for systems where performance metrics are critical.
For instance, in machine learning frameworks, C++ is often employed at the core for computational aspects, while higher-level languages handle user interactions. Popular libraries such as TensorFlow benefit from C++ to optimize their performance. Similarly, the burgeoning field of IoT relies on C++ due to its ability to manage hardware resources effectively. This synergy allows IoT devices to operate with limited resources while maintaining responsiveness.
Closure
As we wrap up our thorough examination of C++ programming, it's essential to reflect on the critical facets that not only highlight the significance of this powerful language, but also underscore its continual relevance in today's fast-paced tech environment. The conclusion serves as a capstone, providing a moment to consolidate the myriad nuances explored throughout this piece.
Recap of Key Points
- History and Evolution: C++ has evolved significantly since its inception, influenced by the growing demands of modern software development.
- Core Principles: Key principles such as object-oriented programming, templates, and the Standard Template Library showcase C++’s versatility and strength.
- Control Structures and Functions: Understanding control structures, including loops and conditional statements, alongside the diverse array of functions underscores the language's ability to handle complex tasks efficiently.
- OOP Concepts: The concepts of classes, inheritance, and polymorphism reveal how C++ encapsulates real-world modeling into programming paradigms.
- File Handling, Error Management, and Best Practices: Effective file handling and robust error management ensure reliability, while best practices in code maintenance elevate future development.
- Advanced Features and Applications: The use of smart pointers, lambda expressions, and real-world applications in systems programming and game development provide proof of C++’s impact across various domains.
"C++ remains a cornerstone language, adept at merging efficiency with powerful abstraction, essential for high-performance applications."
Encouragement for Continuous Learning
The journey through C++ is akin to navigating through a dense forest—every pathway can lead to new discoveries. The tech industry is dynamic, constantly evolving and presenting new challenges. For students and aspiring developers, embracing a mindset of continuous learning is crucial. This means not only keeping up with the latest version updates and features, but also experimenting with advanced concepts through practical applications.
Consider engaging with communities on platforms such as reddit.com or even discussions on professional networks like facebook.com, where real-world C++ enthusiasts share knowledge and resources.
By leveraging these resources and committing to hands-on practice, you can deepen your fluency in C++. This will empower you to tackle complex problems and innovate solutions, transforming your coding skills from basic to remarkable. So, ready your coding environment and dive back into the world of C!