Mastering C: Your Guide to Online Coding Tests
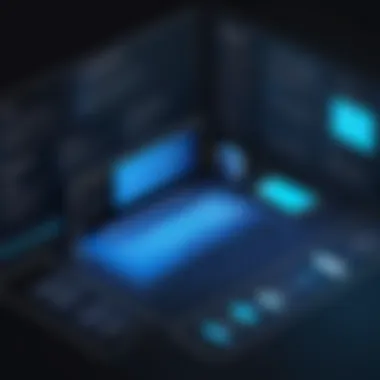
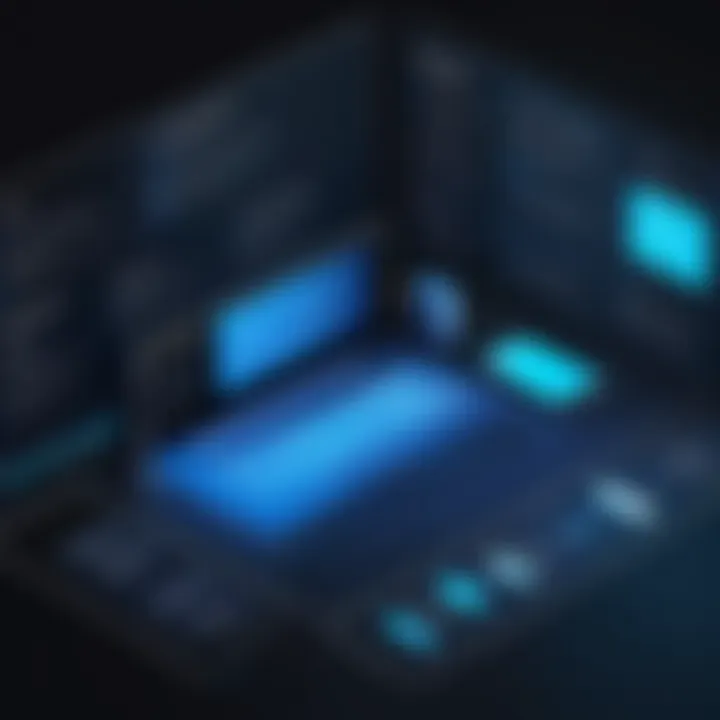
Intro
Online coding tests are becoming increasingly essential in the tech industry, especially when it comes to the C programming language. These assessments serve as a benchmark for both learners and professionals, allowing them to demonstrate their coding abilities and problem-solving skills. In this guide, we will explore the various aspects of coding tests in C, including their significance, typical structures, preparation strategies, and the top platforms that offer these assessments. By gaining insight into these elements, readers will better understand how to effectively navigate online coding tests.
Preface to Programming Language
History and Background
C is a foundational programming language developed in the early 1970s by Dennis Ritchie at Bell Labs. It was created to improve system programming and software development. The language gained immense popularity due to its efficiency and flexibility. It has influenced many other programming languages, including C++, Java, and Python. Knowing its history helps learners appreciate its significance in the programming landscape.
Features and Uses
C is known for its performance and close proximity to hardware. It provides low-level access to memory and allows for effective resource management. Features such as pointers, manual memory management, and a rich set of operators make C a powerful language for various applications, including system software, embedded systems, and game development. Its versatility is a key reason why learning C remains relevant in today’s tech environment.
Popularity and Scope
C is still widely used in both academic and professional settings. According to various surveys, it consistently ranks among the top programming languages. Many companies seek candidates with a strong grasp of C due to its foundational nature in software development. In addition, understanding C equips learners with skills applicable to other languages. This makes it a beneficial starting point for any coding journey.
Basic Syntax and Concepts
Variables and Data Types
In C, variables store data, and their types dictate the kind of data they can hold. Common data types include int, float, char, and double. Declaring a variable involves specifying the type and the variable name. For example:
Understanding data types is critical, as they affect memory usage and performance in programming.
Operators and Expressions
Operators in C are symbols that perform operations on variables and constants. These include arithmetic operators such as +, -, *, and /, as well as relational and logical operators. Knowing how to manipulate these operators is essential for creating effective expressions and implementing logic in your programs.
Control Structures
Control structures guide the flow of the program. C uses various control structures, such as if-else statements, loops (for, while), and switch-case constructs. Each serves to control how the code executes based on certain conditions. Mastery of these is vital for programming tasks, allowing users to create complex logic with relative ease.
Advanced Topics
Functions and Methods
Functions in C are blocks of code designed to perform specific tasks. They improve code reusability and organization. Learning to define and utilize functions effectively is a significant skill in both coding tests and real-world applications. For instance, you can create a simple function as follows:
Object-Oriented Programming
Though C is primarily a procedural language, concepts from object-oriented programming (OOP) can be utilized through structures and functions. Understanding OOP principles such as encapsulation, inheritance, and polymorphism can enhance programmers’ ability to tackle complex problems and improve software design.
Exception Handling
While C does not have built-in exception handling like some other languages, it’s important for programmers to implement error-checking mechanisms. Functions like or manual checks can prevent unexpected behavior in code, particularly in critical applications. This knowledge is essential for coding tests that emphasize reliability.
Hands-On Examples
Simple Programs
Practicing simple programs is a cornerstone of learning. Examples may include tasks like printing a welcome message, calculating addition of two numbers, or determining whether a number is even or odd. These foundational exercises build confidence.
Intermediate Projects
As learners progress, they can tackle intermediate projects such as building a basic calculator, implementing a sorting algorithm, or creating a simple game. These projects often appear in coding tests, assessing both logic and creativity.
Code Snippets
Here's a simple code snippet for calculating the factorial of a number:
Resources and Further Learning
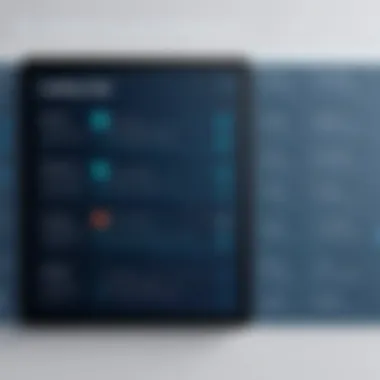
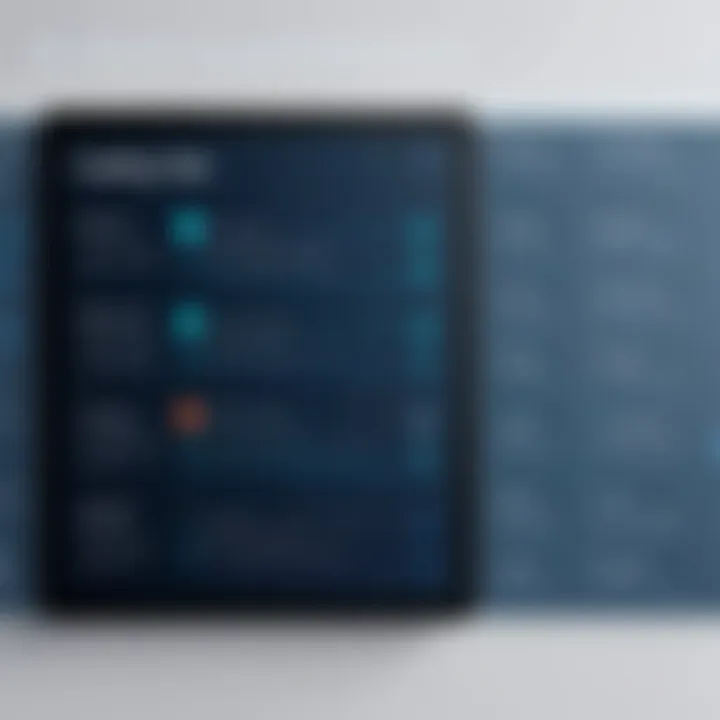
Recommended Books and Tutorials
- The C Programming Language by Brian W. Kernighan and Dennis M. Ritchie - A classic resource.
- C Primer Plus by Stephen Prata - Good for beginners, covering fundamental concepts.
Online Courses and Platforms
Websites like Coursera, Udemy, and edX offer excellent courses specifically focused on C programming. These platforms provide structured learning paths for both beginners and experienced coders.
Community Forums and Groups
Engaging with communities on Reddit or specialized forums can greatly enhance learning. Sites like Stack Overflow offer invaluable support and resources for tackling programming challenges.
Online coding tests in C offer a pathway to validate your skills, gain experience, and prepare for real-world programming. Understand their structure and practice regularly to enhance your proficiency.
Foreword to Online Coding Tests
Online coding tests are becoming an essential part of the technical recruitment process. The rise of technology places emphasis on the ability to write clean, efficient code. Therefore, understanding the role and structure of coding tests is crucial for programmers, whether they are novices or seasoned professionals. This section introduces the concept of online coding tests, outlining their definitions, purposes, and importance in the tech industry.
Definition and Purpose
Online coding tests are assessments conducted over the internet to evaluate a candidate's programming skills. They are commonly used by employers during the hiring process. These tests serve multiple purposes, including:
- Skill Assessment: They allow companies to gauge a programmer's coding capabilities, logic, and problem-solving skills.
- Standardized Evaluation: By using coding tests, employers can have a consistent measure for all candidates. This eliminates bias during the interview process.
- Streamlined Recruitment: Coding tests help quickly filter candidates, enabling hiring teams to focus on those who meet the required technical skills.
In essence, online coding tests create a bridge between theoretical knowledge and practical ability. They assess how well candidates can apply their understanding of programming concepts in real-world scenarios.
Importance in the Tech Industry
The tech industry is highly competitive, with a continuous demand for skilled developers. Online coding tests play a crucial role in this landscape for several reasons:
- Competitive Advantage: Companies that utilize coding tests can identify top talent more effectively, ensuring they hire the best candidates.
- Efficient Resource Allocation: With the use of tests, hiring managers can allocate time efficiently. They can assess many candidates in a short period without extensive interviews.
- Skill Validation: Coding tests provide tangible proof of a candidate's abilities. This is especially important in tech, where practical skills often outweigh theoretical knowledge.
Moreover, coding tests are beneficial for candidates as well. They provide a platform for individuals to demonstrate their skills, which can lead to better job opportunities.
"In today’s job market, coding tests are a necessary hurdle for tech positions. They can elevate or eliminate candidates based on their programming proficiency."
Understanding Programming
Understanding C programming is critical for navigating the landscape of online coding tests effectively. C language is not just a tool for creating software; it serves as the foundation of programming concepts that are applicable across various languages. This comprehension includes grasping fundamental programming paradigms, memory management, and data structures—all vital for both personal skill development and success in coding assessments.
Overview of Language
C is a general-purpose programming language that was developed in the early 1970s. It is known for its efficiency and flexibility. Many modern programming languages, like C++ and Python, have roots in C, making it essential for anyone looking to delve deeper into software development.
C offers a middle ground between high-level programming and low-level machine languages. It provides constructs that map effectively to typical machine instructions, which allows for performance optimization. This is particularly crucial in scenarios where resource management is key, such as embedded systems or operating systems.
Moreover, understanding C helps in deciphering how other languages function. With a deep knowledge of C, a programmer can anticipate outcomes and behaviors in other programming environments, thereby solving problems more efficiently.
Key Features of Programming
C language possesses several key features that underscore its significance:
- Portability: C code can be executed on different types of machines with minimal modification, enhancing its utility across platforms.
- Efficiency: Its low-level capabilities allow for fine-tuned control over system resources, making it suitable for performance-critical applications.
- Rich Library Support: C provides a robust standard library, offering pre-written functions that accelerate development.
- Structured Programming: The language promotes structured programming through functions, which enhances clarity and maintainability.
"C encourages fault-free coding, which directly translates to better quality software."
- Manual Memory Management: Programmers in C have control over memory allocation and deallocation, which is empowering but also requires responsibility to prevent memory leaks.
- Categorical Language: C is often implemented to create other programming languages and operating systems, further showcasing its versatility.
Structure of Online Coding Tests
Understanding the structure of online coding tests is crucial for preparation and performance in these assessments. The layout of a test typically influences the overall experience for candidates, directly affecting their ability to demonstrate their programming skills effectively. With so many variations of tests, comprehending their common elements enables better strategic planning and efficient utilization of time during the test. Moreover, gaining insight into this structure can help candidates to build confidence, allowing them to approach coding tests with clarity and purpose.
Types of Questions
In online coding tests, questions usually come in different formats. Each format has its own focus and benefits, which contribute to the overall assessment of a candidate's abilities. Below we examine three common types of questions found in coding tests:
Multiple Choice Questions
Multiple choice questions (MCQs) are a common feature of online coding tests. These questions assess theoretical understanding and knowledge of specific C programming concepts. The key characteristic of MCQs is their ability to quickly gauge a candidate's knowledge base without requiring extensive coding. They provide an efficient way to cover a wide range of topics in just a short amount of time.
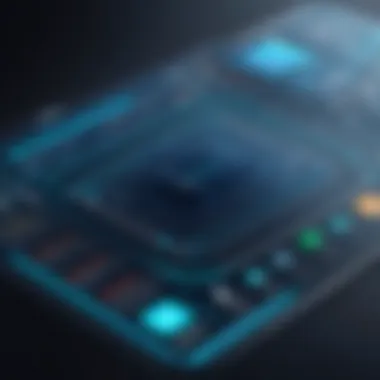
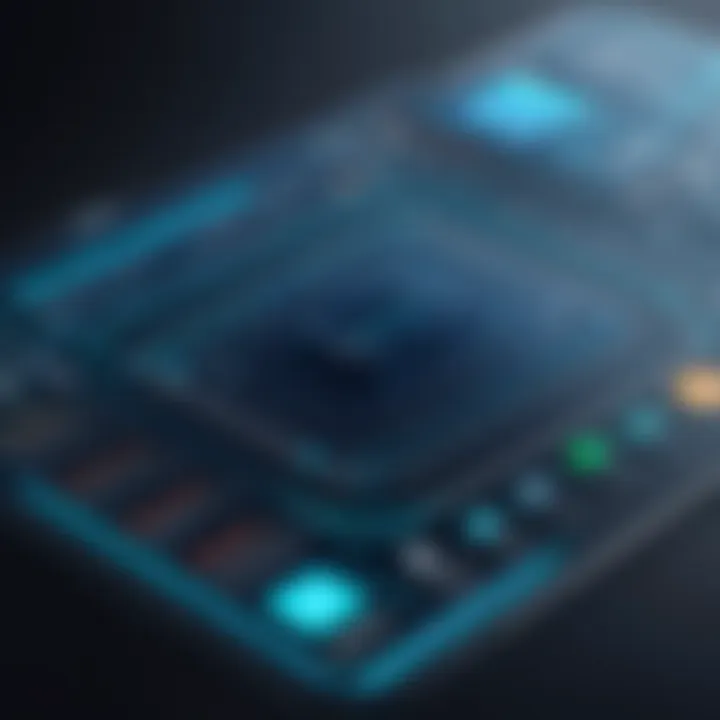
One unique feature of MCQs is that they can be designed to include misleading options. This encourages candidates to think critically and truly understand the material rather than relying on memorization. However, the disadvantage is that candidates may guess answers, which skews their actual comprehension level.
Programming Challenges
Programming challenges form the core of many online coding tests and are focused on practical coding skills. They typically require candidates to write code that solves a specific problem, reflecting their ability to apply theoretical knowledge in practical scenarios. The key characteristic of programming challenges is that they often simulate real-world problems that programmers face.
This format is beneficial because it allows evaluators to assess not only problem-solving skills but also coding style and efficiency. A unique aspect of programming challenges is the potential to run multiple test cases against submitted solutions. However, such tasks can be demanding, as they require both depth of knowledge and the ability to articulate code under time constraints.
Debugging Tasks
Debugging tasks are another essential component of online coding tests. They focus on identifying and fixing issues in provided code snippets. This assesses a candidate's analytical skills, attention to detail, and understanding of programming logic. The key characteristic of debugging tasks is that they typically require more careful examination of code, demanding both comprehension and troubleshooting aptitude.
Debugging is a beneficial choice for coding tests because it reflects real-life work scenarios where developers often need to troubleshoot issues. Nevertheless, the challenge lies in the limited time most tests provide, which can lead to frustration if candidates struggle with a particular bug.
Test Duration and Scoring
The duration and scoring of online coding tests are significant factors affecting performance outcomes. Test length can vary widely, often ranging from 30 minutes to several hours, depending on the complexity and number of questions. Awareness of the time allotment is essential so candidates can pace themselves appropriately during the test.
As for scoring, tests can be graded on a variety of criteria. Some are straightforward, with points allocated per correctly answered question. Others consider code efficiency, clarity, and even style. Thus, understanding the scoring model can help candidates tailor their approach and focus areas accordingly. Knowing these details prior to starting the test is key to optimizing performance.
Popular Platforms for Coding Tests
In the realm of online coding tests, platforms play a critical role. They provide a structured way to assess programming skills, offering various types of coding challenges suitable for different levels of learners. Each platform has unique features that could influence a candidate’s performance and preparation. Understanding these platforms is essential for anyone looking to excel in coding assessments. By familiarizing oneself with multiple options, you can select the platform that best fits your learning style and needs. Here are three prominent platforms that are frequently used for coding tests in C.
LeetCode
LeetCode is among the most popular platforms for coding practice, especially in terms of preparing for technical interviews. It offers a vast collection of coding problems ranging from easy to hard, which allows users to gradually build their skills. The platform also provides a mock interview feature, simulating real interview scenarios.
Key Features of LeetCode:
- Diverse Problem Set: Thousands of coding challenges across various topics and difficulty levels.
- Discussion Forum: Users can discuss solutions and strategies, fostering a community of learners.
- Company-Specific Questions: Real coding questions asked during interviews at big tech companies, which can be especially helpful for targeted preparation.
Having a subscription can unlock premium features, including additional problems and solutions. This makes LeetCode a preferred choice for many students and professionals looking to refine their skills in C programming.
HackerRank
HackerRank is another leading platform that caters not just to individual learners but also organizations looking to evaluate developers’ coding skills. The platform offers numerous coding challenges, competitions, and tutorials, making it a versatile learning tool. It also has assessments designed for employers, helping them gauge candidates during the hiring process.
Highlights of HackerRank:
- Skill-Based Assessments: Users can take assessments specifically tailored to their skills.
- Real-World Projects: In addition to coding challenges, it allows users to work on projects that reflect real industry tasks.
- Leaderboard and Community: An interactive community where users can compete against each other and see their rankings.
HackerRank's focus on both learning and assessment makes it a comprehensive tool for anyone serious about improving their coding abilities.
CodinGame
CodinGame presents a more gamified approach to coding challenges. It allows users to solve problems while competing in a game-like environment, which can make learning programming feel less daunting. This platform is especially beneficial for those interested in enhancing their problem-solving skills through fun and engaging means.
Features of CodinGame:
- Game Mechanics: Solve coding puzzles to progress in gaming style, appealing to both new and advanced programmers.
- Multiplayer Competitions: Users can compete against others in real-time coding challenges, which encourages a sense of community.
- Support for Multiple Languages: While this guide focuses on C, CodinGame supports several programming languages, offering flexibility.
Incorporating such playful elements may enhance the user experience and can lead to a deeper understanding of coding logic and creativity.
Ultimately, selecting the right platform can significantly impact your coding preparation journey. Each one has its unique strengths and benefits tailored to different needs, ensuring that every programmer can find a suitable environment to thrive in.
By leveraging these platforms wisely, you can elevate your coding skills and prepare thoroughly for online coding tests. Choosing where to practice effectively can lead to better performance in actual assessments.
Preparing for Online Coding Tests
Preparing for online coding tests is a fundamental aspect that significantly impacts your performance. Understanding the structure and requirements of these tests can provide a competitive edge. When you familiarize yourself with common coding challenges, you increase your confidence. Moreover, solid preparation helps in reducing anxiety and allows a clear focus during the test.
Fundamental Concepts to Review
Before taking online coding tests, reviewing key concepts within the C programming language is crucial. These fundamental concepts include variables, data types, control structures, functions, and pointers. Each concept plays a vital role in understanding how to tackle problems presented in the tests. You should ensure your knowledge of the standard libraries provided by C, such as and , as they often become instrumental in solving coding challenges efficiently.
Practice Strategies
Effective practice is an integral part of preparing for coding tests. It helps solidify your understanding and enhances your problem-solving abilities. Here are specific strategies you can implement:
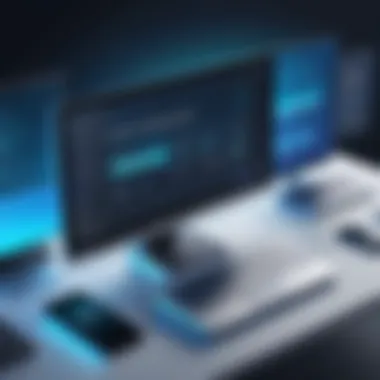
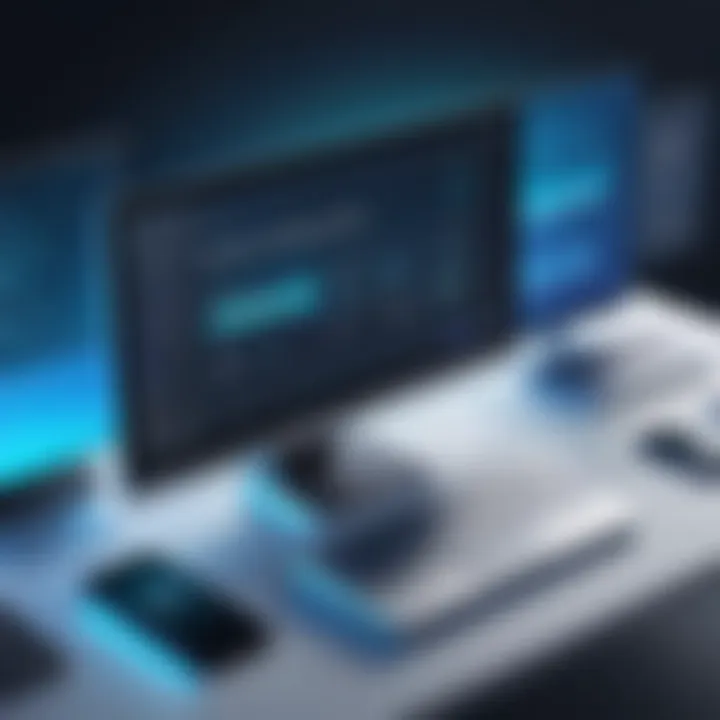
Timed Practice Sessions
Timed practice sessions are a critical component of preparation. They simulate the real test environment and help you manage pressure. The main characteristic of these practice sessions is that they set strict time constraints for problem-solving. This is beneficial because it trains you to think quickly and focus on efficient coding. A unique feature of timed sessions is the feeling of urgency they create, which can mimic actual testing conditions.
However, a potential disadvantage is that excessive pressure may affect your performance negatively if not managed well. It's crucial to strike a balance between practicing under time constraints and allowing sufficient time to learn the material.
Mock Interviews
Mock interviews provide a realistic experience that aids in preparation. They usually involve solving problems in front of an interviewer, which helps you develop your communication skills. The key aspect of mock interviews is that they offer feedback. This feedback can highlight your strengths and areas that need improvement. A unique feature of mock interviews is the interactive element, where you explain your thought process. While they are a great preparation method, one disadvantage may be the lack of genuine pressure compared to actual assessments.
Peer Collaboration
Peer collaboration can greatly enhance your learning experience. Working with others allows for the exchange of knowledge and diverse problem-solving approaches. One key characteristic is that it fosters a supportive environment, making difficult concepts easier to grasp. Collaborative coding improves understanding and keeps motivation levels high. A unique aspect of peer collaboration is the opportunity for joint practice, which can lead to discovering new techniques. However, one downside is that it might lead to dependence on others, which is not ideal when you need to perform independently during tests.
Through these strategies, you can create a robust framework for preparing for online coding tests. By focusing on understanding fundamental concepts and employing effective practice techniques like timed sessions, mock interviews, and peer collaboration, you significantly enhance your readiness.
Executing Tests Effectively
Executing tests effectively is a pivotal aspect of succeeding in online coding assessments. Mastering this not only enhances performance, but also builds confidence. Proper execution can make the difference between passing with flying colors or missing essential points. This section will delve into crucial techniques for managing your time efficiently during tests and will highlight common pitfalls that programmers often encounter.
Time Management Techniques
Time management is vital in an online coding test. Many candidates underestimate how quickly the clock can run down. Here are several strategies to ensure that time is used wisely:
- Prioritize Questions: At the beginning of the test, take a moment to review all questions. Identify which ones you can tackle quickly. Focus first on easier questions that will secure you points.
- Set Time Limits: Assign a specific amount of time for each question. For instance, if you have an hour for ten questions, aim to spend no more than six minutes on each. If you find a question particularly tricky, mark it for review and move on.
- Practice with a Timer: Regular timed practice can simulate real test conditions. Use platforms like LeetCode or HackerRank to get accustomed to solving problems under time restraints.
- Stay Calm and Focused: Anxiety can cause time to slip away unnoticed. Take deep breaths and maintain focus, reminding yourself that staying calm can enhance your performance.
Common Pitfalls to Avoid
When it comes to executing online coding tests, certain mistakes can detract from overall performance. Being aware of these pitfalls is key to improvement:
- Ignoring Problem Constraints: Many candidates miss critical details about what is asked. Read the problem statement thoroughly to avoid unnecessary mistakes.
- Overcomplicating Solutions: Sometimes the simplest solution is the best. Avoid the trap of overthinking and remember that often, straightforward algorithms yield effective results.
- Failure to Test Code: Writing code without testing can lead to overlook bugs. Always allocate time to run your solutions against test cases, both provided and edge cases of your own.
- Neglecting Code Clarity: While passing tests is essential, it is equally important that your code is readably written. This becomes significant if the test also evaluates functionality and clarity. Ensure use of proper formatting and variable naming that aligns with C programming conventions.
"Effective execution is as much about clarity in coding as it is about finding the right solutions."
By applying these time management techniques and being conscious of common pitfalls, candidates can improve their performance in online coding tests. Preparing effectively can ensure that the challenging nature of these assessments does not become overwhelming.
Post-Test Analysis
Post-test analysis plays a crucial role in online coding tests. Once you finish a test, your results are more than just numbers or an overall score. They are a rich source of feedback that helps you identify your coding strengths and weaknesses. By understanding your results, you can formulate an action plan for continued learning and improvement.
Understanding Your Results
After completing a coding test, the results typically include metrics such as accuracy, speed, and problem-solving approach. Most platforms provide a breakdown of your performance on different question types. Such an analysis is vital because it shows how well you understood the core concepts of C programming.
To effectively interpret your results:
- Analyze the Score: Look past the overall score. Understand which areas impacted it the most. If you scored low, was it due to incorrect logic in your programs or syntax errors?
- Review Feedback: Many platforms offer feedback on your solutions, including sample answers and explanations for correct responses. This information can expose gaps in your understanding.
- Check Timing: Evaluate whether you managed your time effectively. In coding tests, time management is important, and a slow pace can lead to unfinished questions. Losing points due to time constraints can be avoided in the future with practice.
This critical inspection of your results can empower you to make better choices for additional learning resources, such as textbooks or online courses relevant to the areas identified as weak.
Identifying Areas for Improvement
Identifying areas for improvement goes a step further than understanding results. It involves engaging with your outcome to ensure progress in upcoming tests. The following strategies can help:
- Focus on Weak Topics: If your results indicate struggles with specific C concepts, allocate more study time to them. Topics often include pointer operations, memory management, or recursion. Understanding these areas deeply can enhance your test performance significantly.
- Practice with Purpose: Utilize platforms like LeetCode or HackerRank, focusing on problems that mirror your weaknesses. Tracking your progress over time can highlight improvements and motivate you.
- Peer Review: Discuss your results and solutions with peers or mentors. They might offer insights that you hadn’t considered.
- Mock Tests: Regularly complete mock tests to simulate the test environment. Keep refining your strategy for tackling questions based on your previous tests.
Reflecting on your performance and creating a defined improvement plan can set you on the path toward becoming proficient in C programming and excelling in online coding tests.
Epilogue
In the realm of coding assessments, the conclusion marks a crucial point where thoughts coalesce into actionable insights. This section synthesizes the varied discussions seen throughout the article, presenting a consolidated view on online coding tests in C. The significance of this conclusion cannot be overstated. It serves not only as a recap of what has been learned, but also as a guide to applying this knowledge effectively.
The benefits of engaging with online coding tests are manifold. First, these tests provide a solid benchmark for skill evaluation. They measure not just theoretical knowledge but practical application in coding scenarios. Engaging with such tests strengthens problem-solving abilities in a real-world context, preparing candidates for future challenges in their professional lives.
Additionally, it is imperative to consider the continuous enhancement of skills through practice. These tests present numerous opportunities for learners to identify their strengths and weaknesses. Ultimately, the conclusion is where practitioners find clarity in their coding journey, equipping them with the tools necessary to tackle complex programming tasks with confidence.
Recap of Key Points
- Significance of Online Coding Tests: Key for both learning and validating skills in C programming.
- Types of Questions: Includes multiple choice, programming challenges, and debugging tasks, leading to comprehensive skill assessments.
- Popular Platforms: LeetCode, HackerRank, and CodinGame offer structured environments for practice.
- Preparation Strategies: Utilization of timed sessions, mock interviews, and collaboration strengthens readiness.
- Post-Test Analysis: Understanding results is essential for targeted improvement.
Final Thoughts on Online Coding Tests in
As the landscape of technology evolves, so too does the role and relevance of coding tests. For students and emerging professionals, these assessments bridge the gap between academic knowledge and industry expectations. Online coding tests in C serve as a platform for self-assessment, enabling one's growth as a proficient programmer.
The journey does not end with passing a test; it is a continuous cycle of learning, practicing, and growing. Those who invest time in understanding and honing their skills through online coding tests are better prepared for the complexities that await in the tech industry.
For further exploration into programming and coding practices, consider visiting resources like Wikipedia) or engaging in discussions on platforms such as Reddit. These avenues can enrich your understanding and facilitate a broader perspective on your coding endeavors.