Mastering Java GUI Development: A Comprehensive Guide
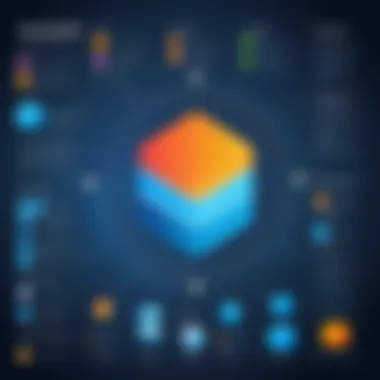
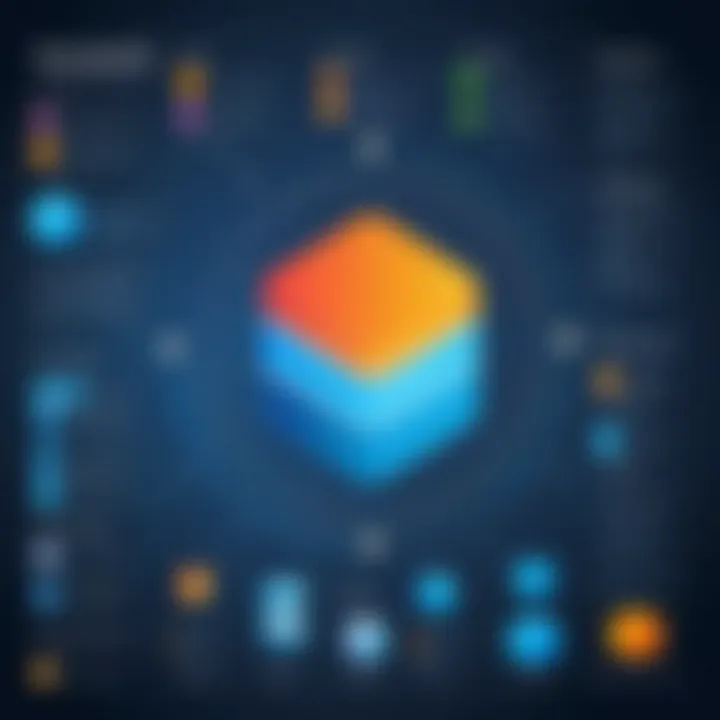
Intro
Java GUI development is a fascinating journey into the world of building user interfaces that are not just functional, but visually appealing and intuitive. Whether you are a beginner eager to learn or someone with a bit of experience under your belt, understanding the fundamental concepts and frameworks in this realm is crucial. This guide aims to make that exploration accessible and engaging.
Graphical user interfaces are at the heart of most modern applications. They enable users to interact with software in a more meaningful way than mere command line inputs. In Java, several libraries and frameworks facilitate the creation of GUIs, such as Swing, JavaFX, and AWT. Each of these tools has its own quirks and advantages, which we will delve into.
Most importantly, this guide is designed for students and those learning programming languages, as we will discuss essential tools and methodologies, all while highlighting best practices and common pitfalls. Let's dive into the depths of Java GUI development together!
Preface to Programming Language
History and Background
Java was created by Sun Microsystems in the mid-1990s with the goal of building platform-independent applications. The core philosophy behind Java was simple: āWrite once, run anywhere.ā This feature quickly garnered attention in the software industry, allowing developers to create applications that functioned seamlessly across different platforms. The Java programming language itself is rooted in C and C++, which makes it both powerful and somewhat familiar to many programmers.
Features and Uses
Java brings a bag of features that make it a solid choice for GUI development. These features include strong memory management, automatic garbage collection, and a rich set of APIs that provide functionalities beyond just building UIs. Moreover, Java is used widely, from mobile applications to enterprise-level solutions, allowing developers to innovate across various industries.
- Cross-platform compatibility: Applications run on any device with the Java runtime.
- Rich libraries: A plethora of libraries to facilitate rapid GUI development.
- Strong community support: Forums, tutorials, and an ever-evolving landscape of resources.
Popularity and Scope
The popularity of Java can be attributed to its versatility and robust community. According to various surveys, Java consistently ranks among the top programming languages in the world. As of today, Java is an omnipresent language that you can find almost everywhere ā from Android apps to large-scale enterprise systems. This widespread usage offers learners a solid reason to invest time in mastering Java GUI development.
"Java's ability to bridge the gap between server-side and client-side applications makes it one of the most sought after languages in the tech ecosystem."
Understanding Java's landscape, history, features, and compelling reasons for its popularity sets the foundation for building a strong skill set in GUI development. This comprehensive guide will further explore the syntax, core concepts, and advanced topics in Java programming, setting you on a path to create outstanding graphical user interfaces.
Prelude to Java GUI Development
Java GUI development offers a unique blend of creativity and technical skill, allowing developers to construct visually appealing applications with interactive components. Understanding the principles behind it can be pivotal in enhancing the user experience.
Developers frequently grapple with the complexity of creating interfaces that are not only functional but also aesthetically pleasing. This section serves as the gateway to understanding the fundamental elements driving Java GUI design. The learning curve is often steep; however, embracing this journey can result in impactful software that resonates well with users.
Understanding User Interfaces
A user interface (UI) is the point of interaction between the user and the application. For Java developers, mastering the art of UI design is crucial. The essence of a good UI lies in its ability to communicate intuitively with users. Think of it this way: a great UI is like a well-traveled road; users navigate through it smoothly, without any bumps or unnecessary turns.
When diving into Java GUI, one must focus on several elements:
- Layout: The arrangement of various components should guide users naturally through the application.
- Responsiveness: Applications should adapt to various devices and screen sizes to retain user engagement.
- Accessibility: Designs should cater to all potential users, including those with disabilities.
The Java language supports several libraries and frameworks, and frameworks such as Swing and JavaFX are the primary players in constructing user interfaces. Each provides a set of components that are customizable, allowing developers to create unique experiences.
Importance of GUI in Software Development
Graphical User Interfaces (GUIs) are not just a luxury; they're a necessity in today's software landscape. Traditional command-line interfaces can leave users scratching their heads. A well-designed GUI can significantly improve user satisfaction and usability.
- User Engagement: A captivating interface keeps users coming back. Positive experiences often result in higher retention rates.
- Efficiency: Users can complete tasks faster when they can visualize their interaction with the software.
- Intuition: Good GUIs utilize familiar elements, making it easier for users to understand how to interact with the application.
A compelling GUI has the potential to turn a functional application into a masterpiece, enhancing its value in the competitive software market.
"In the realm of software, the GUI acts as the face of the application, shaping user perceptions."
In summary, Java GUI development serves as the crux of building user-friendly applications. Understanding UI intricacies and appreciating the importance of GUIs can elevate one's software design skills and facilitate a deeper connection between technology and humans.
Fundamentals of Java GUI Programming
Understanding the fundamentals of Java GUI programming is a critical step for any developer venturing into the world of graphical user interfaces. A strong foundation in these elements not only enhances your programming skills but also lays the groundwork for creating intuitive and engaging applications that resonate with users. By grasping the basic components, layout managers, and event handling mechanisms, developers can craft interfaces that respond seamlessly to user interactions.
The significance of mastering Java GUI fundamentals cannot be overstated. It promotes best practices in application design and helps avoid common pitfalls that can lead to a frustrating user experience. In short, getting a handle on the fundamentals gives you the ability to build applications that are not just functional but also user-friendly and visually appealing.
Overview of Java Swing and AWT
To dive deep into Java GUI programming, one must first understand the tools that enable developers to create these interfaces. Here is where Java's Abstract Window Toolkit (AWT) and Swing come into play. AWT was one of the earlier frameworks provided by Java for GUI development. It allows developers to create simple, lightweight components that directly interact with the userās device, granting them access to native GUI elements. However, AWT has limitations, particularly in terms of its component flexibility and appearance.
Swing, on the other hand, is a more advanced framework built on top of AWT. It offers a richer set of components that are more flexible and visually appealing. The fact that Swing components are written entirely in Java means they can be used across various platforms, maintaining their look and feel.
The choice between AWT and Swing is pivotal; Swing is generally recommended for most applications due to its extensive options and enhanced sophistication.
Key Components and Layout Managers
Understanding the key components of Swing is essential in Java GUI development. Some of the common components include:
- JFrame: This is the main window where other components reside.
- JButton: Represents a clickable button.
- JLabel: Used for displaying text or images.
- JTextField: Enables text input from users.
When it comes to arranging these components within a container, layout managers become an invaluable asset. They determine how components are sized and positioned in your interface. Here are a few widely used layout managers:
- FlowLayout: Positions components in a left-to-right flow, much like lines of text in a paragraph.
- BorderLayout: Divides the container into five regions: North, South, East, West, and Center.
- GridLayout: Arranges components in a grid of cells with equal size, making organizational tasks easier.
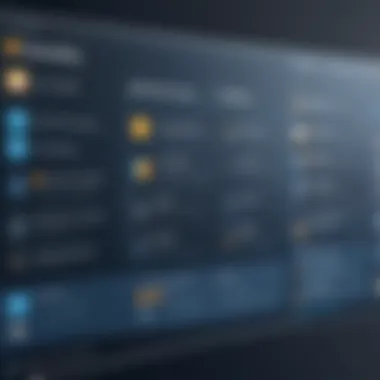
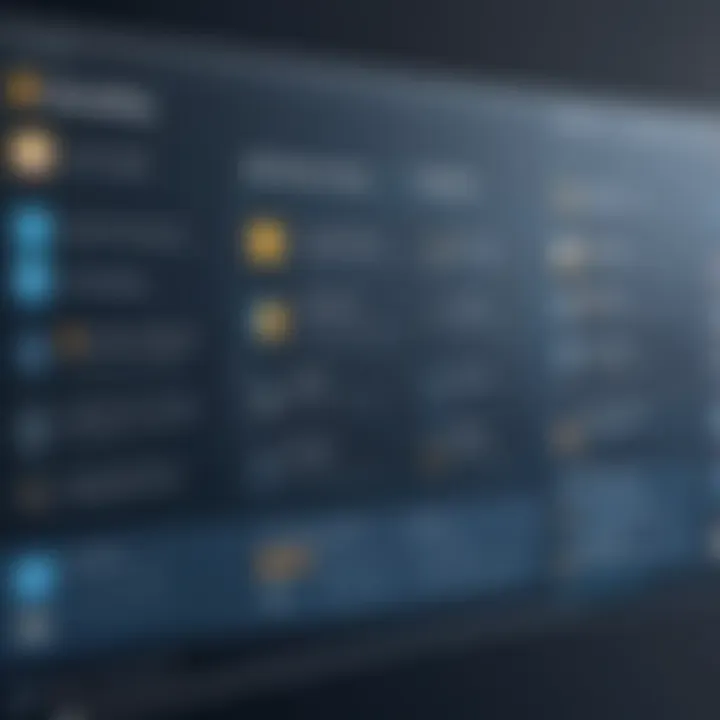
Event Handling Basics
In the realm of GUI programming, event handling is crucial. Simply put, it refers to the way that applications respond to user actions, such as clicks, keypresses, and mouse movements. In Java, every component can generate events that can be picked up by listeners. A listener is an interface that defines a method that must be implemented to handle a particular event type.
The event handling mechanism usually follows this pattern:
- Event Source: This could be a button that the user clicks.
- Event Listener: A class that implements specific listener interfaces to respond to events.
- Event Object: Contains information about the event, like the source and the type of event.
Here's a simple example of how this works in code:
This demonstrates adding an ActionListener to a button. When the button is clicked, it prints a message to the console. Understanding and implementing event handling effectively is vital for creating interactive and responsive applications, which are hallmarks of good GUI design.
Setting Up Your Development Environment
Setting up your development environment is a critical stage in the journey of Java GUI programming. If you think about it, itās like laying the foundation before erecting a building. No matter how grand your ideas or designs may be, a shaky base will lead to cracks down the line. The development environment encompasses all the software and tools you need, ensuring that your programming process is efficient and smooth. It also helps in streamlining your workflow and can significantly impact your productivity as you dive into building user-friendly applications.
Required Software and Tools
To get started, certain software and tools are absolutely essential for Java GUI development. First and foremost, you need a Java Development Kit (JDK). The JDK provides the necessary tools to compile and run Java applications, including GUIs. Additionally, you'll want to get familiar with Java's libraries such as Swing and AWT, which are foundational for building graphical user interfaces.
Alongside the JDK, having a good IDE is important. An Integrated Development Environment not only offers syntax highlighting and code completion but also simplifies debugging and testing. Programs like IntelliJ IDEA, Eclipse, and NetBeans are popular choices among developers. Each has its own merits and choosing one depends largely on personal preference.
Hereās a quick list of key tools:
- Java Development Kit (JDK) - essential for compiling and running your applications
- Integrated Development Environment (IDE) (like IntelliJ IDEA or Eclipse)
- Additional libraries - such as JavaFX for richer interfaces, if needed
- Version control software - Git is widely used for managing changes in your code
Installing Java Development Kit (JDK)
When it comes to installing the JDK, the process is often straightforward, but itās wise to follow it step by step. First, head over to ofļ¬cial Oracle's JDK download page. Choose the version suitable for your operating system. Whether youāre on Windows, macOS or Linux, each installation path varies slightly but the core ideas remain the same.
After you've downloaded the installer, follow these steps:
- Run the installer and go through the prompts. Accept the default settings unless you have a specific reason to change them.
- Once installed, set your environment variables. For Windows, this typically involves adding the path of your JDK to the variable.
- Verify your installation by opening a command prompt and typing . You should see the version of Java you just installed.
This installation sets the stage to move into actual programming. Think of it as pulling all the ingredients together before cooking a fancy dish.
Choosing an Integrated Development Environment (IDE)
Choosing the right IDE can make or break your experience with Java GUI programming. Each IDE comes packed with features designed to make coding simpler, but they differ in terms of usability and functionality.
Eclipse, for instance, is highly customizable. Itās a favorite among many in the development community due to its extensibility with various plugins. However, it can sometimes feel overloaded for beginners. Meanwhile, IntelliJ IDEA provides intelligent code assistance and is user-friendly, making it a good choice for newcomers who want to focus on developing rather than configuring their tools.
Here are some things you might want to consider:
- Ease of use: This matters greatly, especially if youāre just starting out.
- Features offered: Look for debugging tools, version control integration, etc.
- Community support: A larger community can offer help and plugins.
Ultimately, your IDE is where you will spend most of your time during development, so it should feel comfortable for you. It's worth experimenting with a few to see which one jives best with your workflow.
"Choosing the right tools is half the battle when developing robust applications. Always opt for what feels intuitive to you."
Setting up your development environment is a stepping stone, and it's only by properly configuring it that you set yourself up for success in developing Java GUI applications.
Design Principles for Effective GUI
When embarking on the journey of Java GUI development, grasping the design principles for effective graphical user interfaces (GUIs) is paramount. Good design isnāt just about making things look appealing; it directly impacts user interaction and satisfaction. The goal is to create interfaces that make tasks easier, keep the users engaged, and provide a seamless experience. Failing to adhere to these principles can lead to confusion, frustration, and ultimately, user abandonment.
User-Centered Design Concepts
A user-centered design (UCD) approach is all about focusing on the needs and wants of the end-users. Before any coding begins, itās crucial to conduct thorough research on who will be using your application. This research informs the design decisions you make. For instance, if you're developing a tool for elderly users, larger buttons and simpler navigation are essential for ease of use.
- Understanding User Needs: Gather insights through surveys or direct conversations. Identify what features users deem necessary.
- Usability Testing: Once a prototype is developed, involve real users in testing. Observe how they interact with your application and gather feedback.
- Iterative Design: Consider feedback as a gift. Use it to constantly refine and improve the design. Iterate till you hit the sweet spot where the users feel comfortable.
Emphasizing that user needs drive design choices can create a sense of ownership that resonates well with your audience.
Consistency and Feedback
Another pillar of effective GUI design is ensuring consistency across the application. This principle touches on two major areas: visual consistency and functional consistency. Visual consistency means that elements like colors, fonts, and layouts should be uniform throughout the application. Users should feel a sense of familiarity, which greatly reduces the learning curve.
- Visual Consistency: Stick to a defined color palette and font style. Use the same button styles and icons across your application.
- Functional Consistency: This means that similar actions should yield similar results. For instance, if clicking a button opens a new window in one part of the application, it should do the same elsewhere.
Feedback is also crucial in guiding users through their journey in your application.
"Good feedback can mean the difference between a baffled user and a satisfied one."
- Immediate Responses: Simple actions like button clicks should give some form of immediate feedback. A color change or a subtle animation can signal that the command has been acknowledged.
- Notifications and Alerts: Use them wisely to inform users of actions they need to take, or errors they might have caused. But be careful not to overwhelm them with constant notifications.
By adopting these principles, developers can create user interfaces that feel intuitive and engaging. Implementing user-centered concepts and ensuring consistency alongside proper feedback makes a world of difference in user interaction. The result? A well-crafted application that users can appreciate, which stands the test of time.
Building Your First Java GUI Application
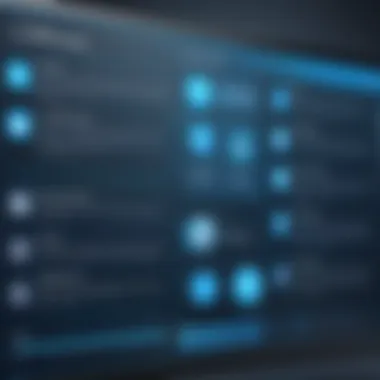
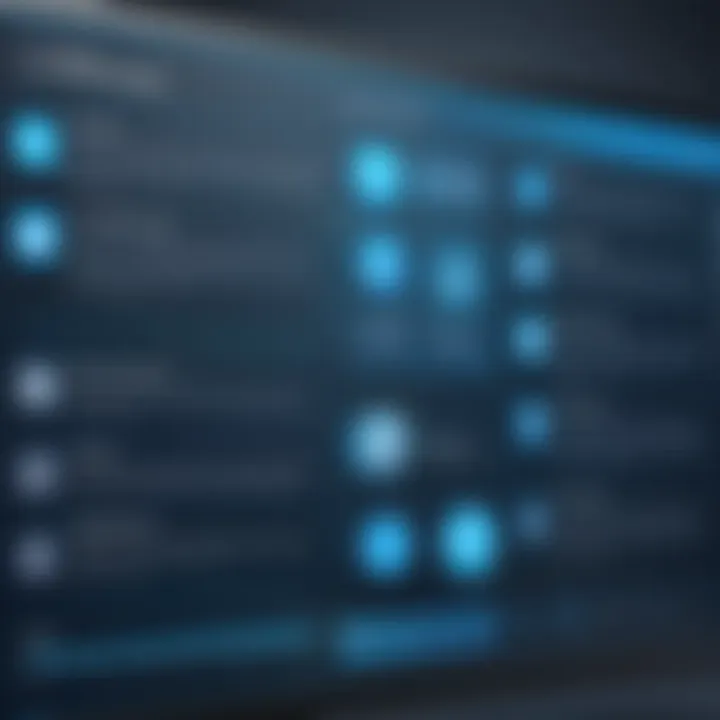
In the realm of software development, the creation of a graphical user interface (GUI) stands as a pivotal milestone. Building your first Java GUI application isn't just about throwing code together; it's a window into the practical implementation of your programming knowledge. This part of the guide serves as a critical juncture for aspiring programmers. Here, you'll grasp not only the mechanics of GUI development but also the underlying principles that bring your application to life.
Diving into Java GUI means embracing visual creativity alongside logical constructs. Youāll learn how to craft interactive interfaces that allow users to engage with your software in a palpable way. The hands-on experience gained here sets a solid foundation for deeper exploration into more advanced concepts down the line. Below, we break this process into more digestible parts, focusing on creating a simple window and adding essential components, along with event listeners that breathe functionality into your application.
Creating a Simple Window
To kick things off, letās start with the skeleton of your application: the simple window. Creating a window is akin to opening a blank canvas where you can showcase your features and components. In Java, this is achieved with the Swing framework, which provides a rich set of classes to create GUI elements. Here's how to create a basic window:
In this snippet, just three lines control your window's size and behavior. Notice how we specify . This line tells the program to exit when the user closes the window, a simple but crucial consideration. Also, setting the size is your first foray into visual dimensions, laying down the grounds for a structured interface.
A couple of points worth mentioning:
- Positioning the Window: It is often a good practice to center your window on the screen. You can do this by using
- Title Bar: The string parameter in the constructor sets the window's title. It's a small touch, but itās important for user experience.
This simple step of creating a window forms the backbone of your application. Once you have this down, you can explore integrations with various GUI components.
Adding Components and Event Listeners
Now that you have your window, it's time to add some life to your application by incorporating components like buttons, text fields, and other interface elements. These components are essential for user interaction and can transform your static window into a functional app.
Letās add a button with an event listener that reacts when the user clicks it. Below is an example:
In this code, a is created, and using an , the program handles button clicks. When the user presses the button, "Button was clicked!" is printed to the console. This straightforward functionality demonstrates how user actions can trigger responses within your application.
Here are a couple of things to keep in mind as you add components:
- Layout Managers: By default, Swing uses a , which stacks components. However, explore other layout managers like or for more refined arrangements.
- Usability: Pay attention to the size and visibility of components. They should be intuitive and easy to access for users.
As you progress, keep these basic elements in mind. Implementing a basic window and adding components with event listeners are foundational skills, paving the way for further complexities in Java GUI development. Each of these steps reinforces your understanding, allowing you to construct more sophisticated applications in the future.
Advanced GUI Features
In the realm of Java GUI development, mastering advanced features is akin to learning the finer points of a craft. As developers, we often find ourselves neck-deep in design elements that elevate user experience. Not merely sticking to basic functionalities, incorporating advanced GUI features opens up a world of possibilities.
Custom Painting and Graphics
Custom painting and graphics is where the pixelated world of Java Meets artistic expression. Rather than relying solely on built-in components, developers can draw custom shapes, images, and text that make applications truly unique. This is particularly vital when building applications that rely on complex data visualization or custom branding.
Imagine you are developing a drawing application. You might want to allow users to draw freely on a canvas. This involves overriding the method of a to perform custom drawing. For example:
Such customization enhances the aesthetics and function of your app, making it a canvas rather than just another software tool.
Moreover, understanding the Graphics2D class can unlock more complex designs. With this class, you can manage advanced features like gradients, transformations, and anti-aliasing. Diving into these capabilities allows for smoother and more visually appealing graphics. However, one must remain cautiousāoverdoing custom painting can lead to performance hits if not managed wisely.
Using Third-Party Libraries for Enhancements
When you're developing Java GUIs, you might feel a bit like a chef trying to whip up a three-course meal with a tiny spice rack. Fortunately, third-party libraries swoop in like a trusted sous-chef, providing a treasure trove of utilities that can significantly enhance your applications.
Libraries such as JFoenix for Material Design or ControlsFX can be integrated seamlessly to elevate the interface design. They provide pre-built components that can save you time and add functionality that might otherwise take ages to develop on your own. For instance:
- JFoenix: Offers sleek material design components that lend a contemporary touch to your application.
- JavaFX: Provides controls like buttons, lists, and charts that excel in aesthetics and usability.
Using these libraries does come with considerations. It's important to evaluate factors like community support, documentation, and compatibility with your existing codebase. Some libraries might have frequent updates that necessitate adjustments on your part. But when used effectively, these tools can propel your Java application to impressive heights, transforming it from a basic setup to a robust user-friendly experience.
Remember: The user interface isn't just the face of your application; it's also a crucial element that defines how users understand and interact with technology.
Testing and Debugging GUI Applications
Before you can present a shiny new Java GUI application to users, youāve got to know it works like a charm. Testing and debugging become vital cogs in the development wheel, ensuring everything operates smoothly. A GUI application, unlike a barebones console app, requires a nuanced approach during testing because it has many interactive components. Each button click, mouse hover, and keyboard event should act as expected. When testing a GUI, you're not just checking functionality; you're also paying attention to how intuitive the applications feel for users.
In terms of benefits, effective testing reduces frustration for end-users, saves time and resources, and builds a product that's robust in real-world situations. Additionally, finding and fixing issues early can prevent costly changes later when a project may be further along in its timeline. Thus, testing and debugging can often mean the difference between a successful product launch and a lukewarm reception.
Best Practices in Testing GUI
When it comes to testing your Java GUI applications, there are a few best practices to keep in mind:
- Automate Where Possible: While manual testing has its place, especially for exploratory testing, automating repetitive tasks lets you focus on complex scenarios that are far less predictable.
- Incorporate Different Test Levels: Use unit tests for specific components, integration tests for interactions between them, and user acceptance tests to ensure it meets requirements.
- Pay Attention to Usability: Test not just for functionality but also for usability. Observing real users interacting with your application can unearth various improvements that might have flew under the radar during development.
- Cross-Platform Testing: Make sure the application runs smoothly across different operating systems and screen sizes. The same interface can behave dramatically differently on Windows versus macOS.
"Testing is not just about finding bugs; it's about building confidence that the software behaves as intended."
Common Debugging Techniques
Debugging is an essential skill in the toolkit of a Java GUI developer and comes with several techniques that can prove helpful:
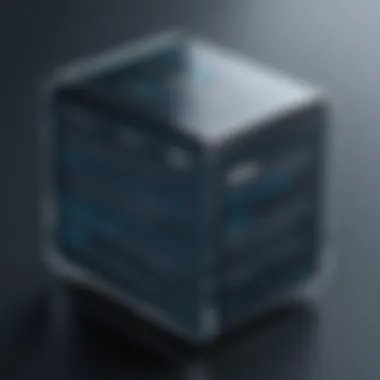
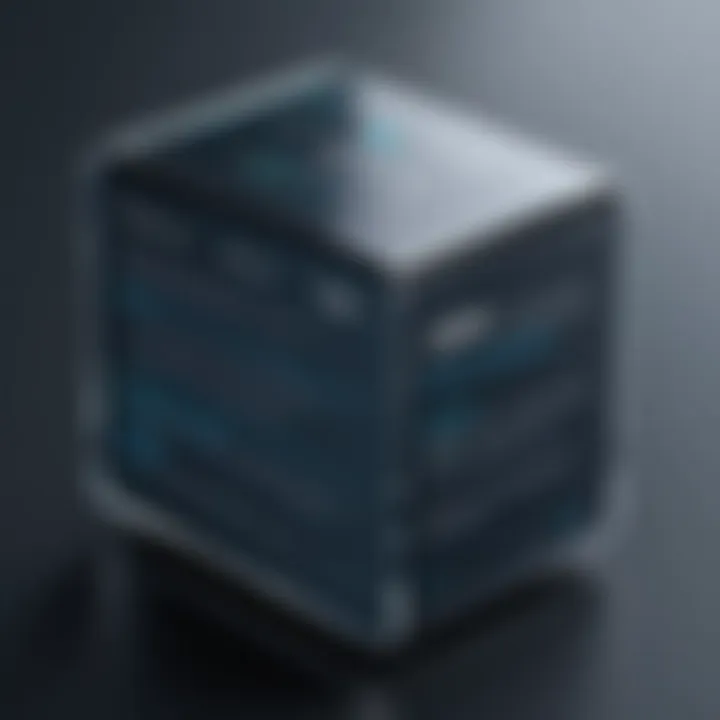
- Use Logging Strategically: Implement logging within your application to track down issues more efficiently. It can give you clues about the internal state when something goes awry.
- Leverage Java Debugger: Tools like JDB offer real-time debugging capabilities. You can set breakpoints to pause execution and examine the stack, variables, and threads effectively.
- Isolation Testing: If a UI component misbehaves, test it in isolation to pinpoint whether the issue lies within that component or stems from interactions with others.
- Methodical Troubleshooting: Tackling bugs one at a time can be effective. Try to reproduce the bug consistently before you start tweaking things, ensuring you understand exactly what the problem is.
These techniques will aid developers in pinpointing and resolving issues. Adopting rigorous testing and debugging practices sets the stage for creating reliable Java GUI applications that deliver value to users.
Performance Optimization
When developing Java GUI applications, the importance of performance optimization cannot be overstated. As applications grow more complex and feature-rich, minding performance becomes crucial for providing a smooth user experience. Users expect applications not only to function correctly but also to respond swiftly to their commands. If an application lags or freezes, it can frustrate users and may diminish their trust in the software. Moreover, optimizing performance can lead to more efficient resource utilization, which is beneficial in reducing operational costsāparticularly important in enterprise environments.
In this section, we will explore various strategies that can enhance the performance of Java GUI applications. This will encompass identifying performance bottlenecks and practicing efficient resource management. It's about fine-tuning every facet of your application to ensure it runs like a well-oiled machine.
Identifying Performance Bottlenecks
The first step in performance optimization is identifying where the bottlenecks lie. Usually, these are parts of the code that slow down the entire application. Potential sources of these bottlenecks can be numerousāinefficient algorithms, excessive use of system resources, or even a poorly designed user interface can be culprits.
To effectively identify these bottlenecks, developers can employ a variety of tools and techniques:
- Profiling Tools: Java provides tools such as VisualVM and YourKit that help analyze application performance by monitoring CPU usage, memory consumption, and method invocation counts. These tools offer visual feedback that can pinpoint which methods or components are causing lag.
- Logging and Monitoring: Implementing logging at strategic points in the application can help capture performance data in real time. This data can be analyzed to assess how long specific tasks take and where problems might occur.
Once bottlenecks are identified, the next logical step is to optimize them. Often, this might involve rewriting certain sections of code using more efficient algorithms or reducing unnecessary computations.
Efficient Resource Management
Once performance bottlenecks are identified, the next focus should be on resource management. This aspect of performance optimization is about ensuring the efficient use of system resources, such as memory, CPU, and network bandwidth.
Here are some key strategies to consider:
- Memory Management: Java automatically manages memory with its garbage collection mechanism, but it's still essential to assess how memory is utilized within your GUI. Using too many unnecessary components can increase memory usage and strain the application. Be judicious in your choice of components and dispose of those that are no longer needed.
- Lazy Loading: Implementing lazy loadingāwhere components are only loaded when they are requiredācan help reduce the initial load time and distribute the resource demand over time.
- Thread Management: Using multiple threads effectively can greatly boost performance. The SwingWorker class allows background tasks to be executed without blocking the UI thread, ensuring that the interface remains responsive even while performing heavy computations.
By employing these strategies, developers can see a marked improvement in the responsiveness of their Java GUI applications.
"Getting the performance right is like learning to ride a bicycle; you don't know just how much it matters until you've wobbled for a while."
Deploying Java GUI Applications
Deploying Java GUI applications is a critical step in the development process. Itās where all the hard work comes together, turning lines of code into a fully functional program that users can interact with. Ultimately, how you approach deployment can greatly affect the end-user experience. Hence, understanding this phase is indispensable for any developer aiming for success with their applications.
When discussing deployment, there are several specific elements to consider. These include the desired platforms for your application, the packaging method, user environment, and ongoing support and updates. Choosing the right deployment strategy is not a one-size-fits-all solution. Factors such as performance requirements, user accessibility, and update frequency can significantly influence your approach.
Packaging Applications for Distribution
Packaging your Java GUI application for distribution involves bundling all necessary files and resources to ensure that your application runs smoothly on the user's machine. This process can include the Java Runtime Environment (JRE), any libraries your application depends on, and, of course, the applicationās own files.
- Creating Executable Packages:
- Cross-Platform Packaging Methods:
- Consider using tools like Jar (Java Archive) files to encapsulate your application.
- In bundling your app, don't forget to include manifest files which help in defining entry points.
- For broader reach, think about creating installers using tools like Launcj or Inno Setup. These can help ease the installation process for users on different operating systems.
By carefully curating the packaging, developers can ensure that the final product aligns with user expectations and system requirements, thus providing a seamless installation experience.
Cross-Platform Considerations
In todayās tech landscape, users can run applications on various operating systems. This means cross-platform considerations canāt be brushed off lightly when deploying a Java GUI application.
- Javaās Role:
Java provides a unique advantage here. Because Java applications run on the Java Virtual Machine (JVM), the code can generally function across different platforms without modifications. This feature is a cornerstone of Javaās allure, simplifying much of the hassle typically associated with cross-platform development. - User Experience:
Yet, itās crucial to ensure that the application looks and behaves consistently across different OS environments. Alterations in appearance or functionality can frustrate users. Always run thorough testing within various operating systems, such as Windows, macOS, and Linux, to detect discrepancies and address them before distribution. - Dependency Management:
Donāt forget about dependencies! Ensure that all external libraries are accessible on any platform the application supports. Tools like Maven or Gradle can help in managing these dependencies effectively.
"A well-prepared deployment is just as vital as the code that underpins it. Great applications lose their shine if they donāt deliver a consistent user experience across platforms."
In summary, deployment is not merely a formality. It requires strategic planning that encompasses packaging, cross-platform compatibility, and user experience. This crucial phase can define how widely your Java GUI application will be adopted and enjoyed.
Future Trends in Java GUI Development
As the digital landscape continues to evolve at a breakneck pace, understanding how Java GUI development is adapting becomes crucial for modern developers. With every passing day, new technologies and frameworks emerge, and the need for seamless integration into diverse environments increases. Recognizing these trends not only empowers developers to stay ahead of the curve but also ensures they can deliver applications that resonate well with users' expectations.
Emerging Technologies and Frameworks
In recent years, a fascinating wave of technologies has begun to reshape the Java GUI landscape. Among these is JavaFX, which has become the preferred option for developers looking to create rich internet applications with stunning graphics and advanced UI controls. The shift towards JavaFX represents a pivotal change from the traditional Swing and AWT, which are often seen as outdated.
JavaFX embraces modern development principles such as:
- Responsive Design: With the increasing demand for applications that work across different devices, JavaFX provides tools for building dynamic layouts that adapt to various screen sizes.
- CSS Styling: Leveraging CSS for styling offers more flexibility and enables developers to separate design from functionality, simplifying code maintenance.
- FXML: This XML-based language allows users to define the user interface in a declarative way, making it easier to manage complex UIs.
Additionally, frameworks like Gluon are bridging the gap between mobile and desktop applications, allowing developers to write code once and deploy it across multiple platforms. These emerging technologies highlight the importance of adaptability as development practices evolve.
Java in the Age of Web and Mobile Development
The ubiquitous presence of web and mobile applications demands that Java GUI developers rethink how they approach user interface design. Today, it's not enough for applications to merely function; they must also provide an engaging and fluid user experience.
Java's versatility is showcased through its various deployment options, from desktop applications to powerful web services. Technologies such as Spring Boot for back-end support combined with JavaFX or Vaadin for rich web interfaces exemplify how developers can take advantage of Java's robustness in the face of growing competition from frameworks like React and Angular.
Furthermore, mobile development has seen a rise, where tools such as Codename One and JFoenix enable Java developers to build native applications that work on both iOS and Android. This is particularly important as the world leans heavily into mobile-first solutions; as such, staying updated with these advancements ensures Java remains relevant in this dynamic market.
With this shift, considerations around cross-platform consistency, performance optimization, and user accessibility become paramount, as users expect seamless experiences regardless of the device they are using. In summary, being aware of these trends allows developers to proactively harness new tools and skills, ensuring they remain competitive and customer-focused.
"Staying up-to-date with emerging technologies is not just beneficial, it's essential for survival in the competitive field of software development."
The future of Java GUI development is bright. By embracing these trends, developers can create applications that are not only functional but also enjoyable to use, paving the way for a new generation of Java-based software.