Integrating Google Maps API with Java: A Comprehensive Guide
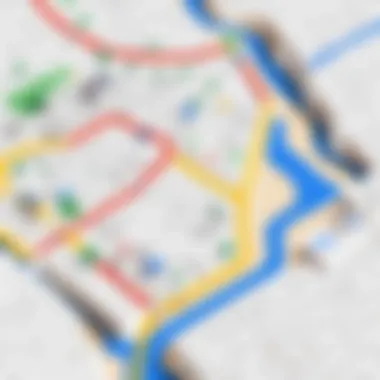
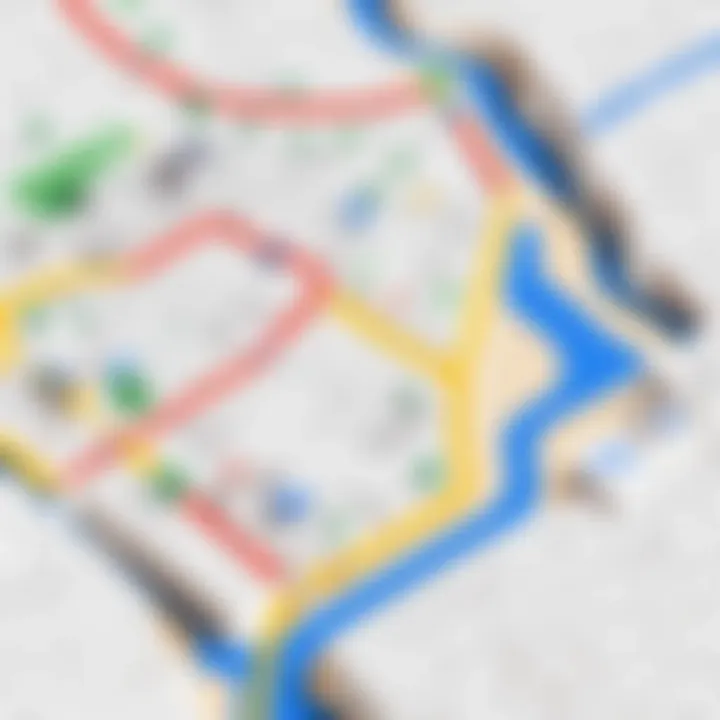
Intro
Understanding how to work with APIs has become essential for developers today. One of the most popular APIs available is Google Maps API. It allows developers to embed Google Maps on webpages and applications, enriching user experiences with location-based features. Integrating this API with Java can unlock a lot of potential for any application, making spatial data accessible and interactive. This guide aims to shed light on practical strategies necessary for the successful incorporation of Google Maps API with Java.
Foreword to Programming Language
History and Background
Java was introduced by Sun Microsystems in 1995. It was designed with portability in mind, enabling developers to write code once and run it anywhere. Over the years, Java has evolved significantly, becoming a foremost choice for Web applications, mobile applications, and enterprise software. Its capacity for handling large-scale applications makes it the programming language of choice for many developers.
Features and Uses
Java is an object-oriented programming language known for its robustness, security features, and multi-threading capability. This means that software can perform various tasks simultaneously, a significant advantage in applications like those using Google Maps. Moreover, its rich API and large community further enhance its versatility.
Popularity and Scope
According to various surveys, Java consistently ranks among the top programming languages globally. Its usage spans across many industries. This widespread popularity stems from its reliability and the extensive ecosystem surrounding it. For those looking to work with powerful APIs such as Google Maps, having a strong grasp of Java is beneficial.
Basic Syntax and Concepts
Variables and Data Types
In Java, variables store data, which plays a crucial role in any program. The types of data include:
- int: For integer values.
- double: For floating-point numbers.
- String: For text data.
- boolean: For true/false values.
Understanding data types is essential when managing information from Google Maps API, such as coordinates or location details.
Operators and Expressions
Java supports various operators, including arithmetic and logical operators. These allow manipulation of data and helps process responses received from APIs. Expressing the handling of such data directly affects the quality of the application.
Control Structures
Control structures guide the flow of the program. Java uses constructs like if-else statements and loops to perform operations based on conditions, which are essential when processing data from Google Maps API. For instance, checking if a location exists before attempting to display it on the map can prevent unnecessary errors.
Advanced Topics
Functions and Methods
Java allows the creation of reusable blocks of code called methods. Understanding how to create and call these methods is crucial for organizing code efficiently. This is particularly useful when making repeated API calls.
Object-Oriented Programming
Object-oriented programming (OOP) is a significant concept in Java. It revolves around objects that can hold data and methods. Using OOP principles enables developers to create structured and maintainable code, which is advantageous when integrating with APIs.
Exception Handling
Error handling is crucial in any application. Java uses a robust mechanism for exception handling that helps manage runtime errors gracefully. This is critical while working with external APIs like Google Maps, as failure in network calls can disrupt the flow of the application.
Hands-On Examples
Simple Programs
To start using Google Maps API with Java, an initial setup is needed. Setting up an IDE like Eclipse can help streamline the process. Here is a simple setup example:
Intermediate Projects
Progressing to more complex scenarios, you can create applications that display maps with various markers. This involves using additional features of Google Maps API to manipulate data.
Code Snippets
Here is a basic example of fetching a place's details using the API:
Resources and Further Learning
Recommended Books and Tutorials
Books like "Effective Java" by Joshua Bloch offers insights into best practices in Java. Online platforms like Codecademy provide interactive Java tutorials that can be beneficial for beginners.
Online Courses and Platforms
Courses on platforms such as Udemy or Coursera can provide structured learning. Many courses focus on Java programming and API integrations specifically, equipping learners with practical skills.
Community Forums and Groups
Engagement with community forums like Reddit's Java subreddit can further your understanding. It is an excellent avenue for receiving feedback and solutions to programming challenges. Joining groups on Facebook dedicated to Java can also foster learning and networking.
Foreword to Google Maps API
Understanding the Google Maps API is crucial as it empowers developers to create dynamic and interactive maps for various applications. This section will shed light on essential elements of the Google Maps API, its benefits, and critical considerations when integrating it with Java applications.
Overview of Google Maps API
Google Maps API is a powerful tool that allows developers to embed maps into web and mobile applications. It offers a comprehensive suite of services, including geocoding, direction services, and street views. These features can elevate any geographic application, helping users visualize data in a more understandable format.
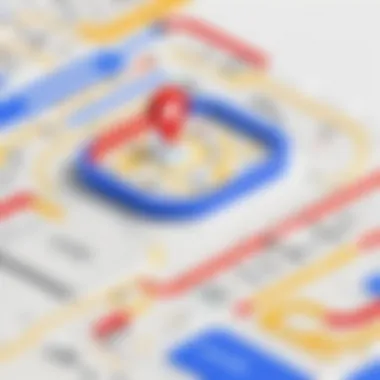
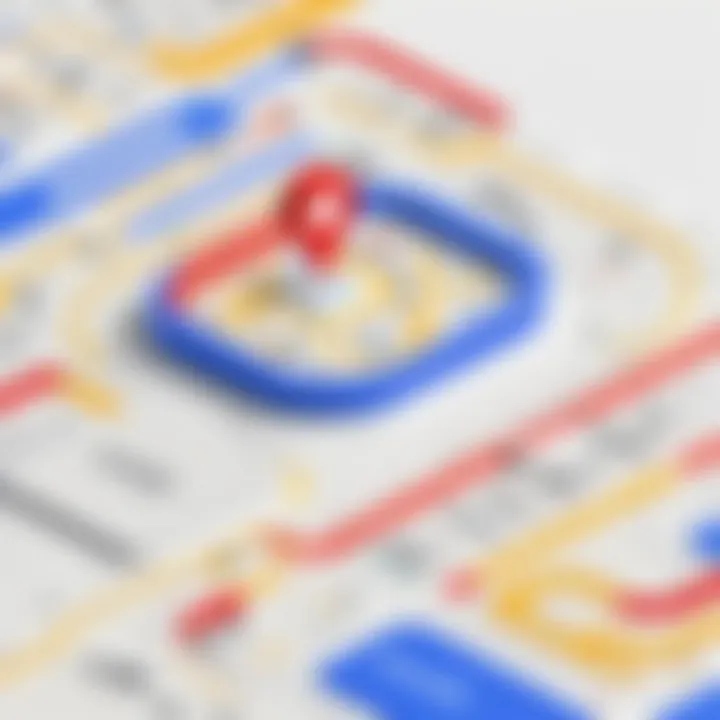
For instance, a real estate application can use Google Maps API to overlay available properties on a map. Moreover, developers can leverage the API to customize maps based on their unique requirements. They can alter UI elements, add layers, and manage markers conveniently. This level of flexibility can significantly enhance user experience.
Importance of Maps in Applications
Maps hold a vital role in many applications, providing users a contextual understanding of their surroundings. In today’s digital age, individuals expect intuitive geographical representations in apps. Companies that fail to incorporate mapping features risk losing competitive advantage.
Some of the key advantages of integrating maps in applications include:
- Enhanced User Experience: Maps provide visual cues that help users navigate complex datasets effectively.
- Location-Based Services: Businesses can utilize mapping features to deliver services tailored to user locations.
- Data Visualization: Maps can serve as a means to display geographic trends, making data interpretation simpler for end users.
Utilizing the Google Maps API permits developers to access a world-class mapping solution that enriches application functionalities and improves user engagement.
Getting Started with Java
Getting started with Java is a crucial step in the journey of integrating the Google Maps API into applications. Java serves as a robust, versatile programming language widely used in enterprise and web applications. Its object-oriented nature allows for better code organization and reuse, making it ideal for handling complex integrations like that of Google Maps. Understanding Java basics before diving into API integrations ensures that you have a solid foundation for creating effective applications.
Setting Up the Development Environment
Required Tools and Software
Having the right tools is essential when starting with Java development. The primary tool needed is an Integrated Development Environment (IDE), such as IntelliJ IDEA or Eclipse. These IDEs provide features like syntax highlighting, debugging support, and project management capabilities which can greatly enhance productivity.
One of the key characteristics of these IDEs is their user-friendly interface, which benefits both beginners and experienced developers. They support multiple programming languages, but they are specifically optimized for Java development, making them a popular choice for integrating APIs like Google Maps. A unique feature of IDEs such as IntelliJ is the built-in support for version control systems. This feature allows for collaborative projects and version tracking, which is advantageous when working on larger applications.
However, one drawback is that these environments might be resource-intensive, particularly on lower-end hardware, which could affect performance. Therefore, it is advisable to assess your machine's capability before selecting the right development tool.
Configuring Java Development Kit
The Java Development Kit (JDK) is another critical element for Java programming. This kit is necessary for compiling and running Java applications. A notable characteristic is that the JDK includes tools for debugging and monitoring Java applications. This makes it a beneficial choice for developers looking to integrate Google Maps API.
A unique feature of the JDK is its cross-platform capability. This means you can develop an application on one operating system and run it on another without modification. From this perspective, the JDK provides flexibility.
However, setting up a JDK may be complex for new users due to the installation and environment variable configuration. It is crucial to follow thorough documentation to avoid complications, especially when planning to work extensively with API integrations later.
Creating Your First Java Project
Project Structure
In Java, organizing your code into a coherent project structure is essential. A well-defined project structure can significantly simplify the process of integrating the Google Maps API. The typical structure includes directories for source code, resources, libraries, and configuration files.
The key characteristic of a good project structure is clarity. It allows developers to navigate through the codebase easily. This is beneficial, especially when understanding how different components interact with the Google Maps API. The unique feature of a structured approach is the ease in managing dependencies and libraries, which are crucial for API integrations.
However, if the project structure becomes too complex, it may lead to confusion and difficulty in maintaining the code over time. It is vital to strike a balance between organization and simplicity.
Adding Required Libraries
When working with APIs like Google Maps, adding the necessary libraries to your Java project is important. Libraries provide the pre-built functionalities that can save time and effort. They are often included via dependency management tools like Maven or Gradle.
The key characteristic of these libraries is that they encapsulate essential features required for interacting with the Google Maps API. This proves to be a beneficial choice, as it speeds up development. The unique feature is that they often come with extensive documentation, which assists developers in implementation and troubleshooting.
One possible disadvantage is that reliance on external libraries can create compatibility issues during updates. This underscores the importance of maintaining a careful eye on library version management, especially when the project evolves.
Utilizing an effective development environment and understanding project structure is instrumental for a successful integration with the Google Maps API.
Configuring Google Maps API
Configuring the Google Maps API is a pivotal step in the process of integrating this powerful tool with Java applications. This section will emphasize key actions that developers must take to get started effectively. Proper configuration allows developers to access various features and services within the API and ensures that applications run smoothly.
Obtaining API Key
An API key is essential when working with the Google Maps API. This key acts as a unique identifier that authenticates requests made to the API. Obtaining the API key involves creating a Google Cloud project and enabling the necessary API services. Without this key, the application will not function as intended, leading to access issues or failures in API calls.
To obtain an API key, follow these steps:
- Go to the Google Cloud Console.
- Create a new project.
- Navigate to the 'APIs & Services' dashboard.
- Enable the Google Maps API services you plan to use.
- Generate an API key under the 'Credentials' tab.
It is crucial to safeguard this key. Use proper access controls to limit who can use it. Failing to do so might expose the project to unauthorized access and potential misuse.
Enabling Google Maps API Services
Once the API key is obtained, developers need to enable the specific Google Maps services they wish to use. This step can significantly impact how the application will function, making it a fundamental part of the integration process.
Choosing the Right API Services
Selecting the right API services is critical for meeting the application's needs. Google Maps API offers various services, such as Directions API, Geocoding API, and Places API. Each of these services has unique capabilities that cater to different functionalities.
- Directions API: Useful for fetching accurate directions between locations.
- Geocoding API: Converts addresses into geographic coordinates.
- Places API: Provides detailed information about locations.
Choosing the most suitable API services aligns with development goals. Each service has its specific use cases, which can optimize user experience and interface design. By understanding these differences, developers can better implement functionality tailored to their applications.
Setting Quotas and Permissions
Setting quotas and permissions is an essential aspect of the Google Maps API configuration. It helps to manage the number of requests made to the API, preventing potential overuse. Google imposes limits on API calls to ensure fair usage among developers.
Establishing quotas is vital, as it protects projects from unexpected fees and performance issues. You can configure these settings in the Google Cloud Console, under the 'Quotas' section of the API services page.
Quotas also provide insights into usage patterns. This allows developers to make informed decisions regarding scaling application features. It is wise to adjust permissions to restrict access to only those who need it, enhancing overall security.
Remember: Proper configuration of the Google Maps API not only ensures smoother integration but also promotes efficient resource management and increased application stability.
By following the steps outlined here, you will be well on your way to effectively configuring Google Maps API for your Java applications. Proper preparation in this area sets a strong foundation for further development.
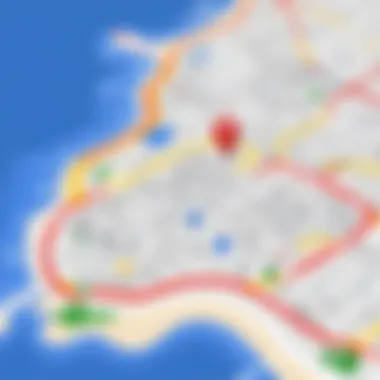
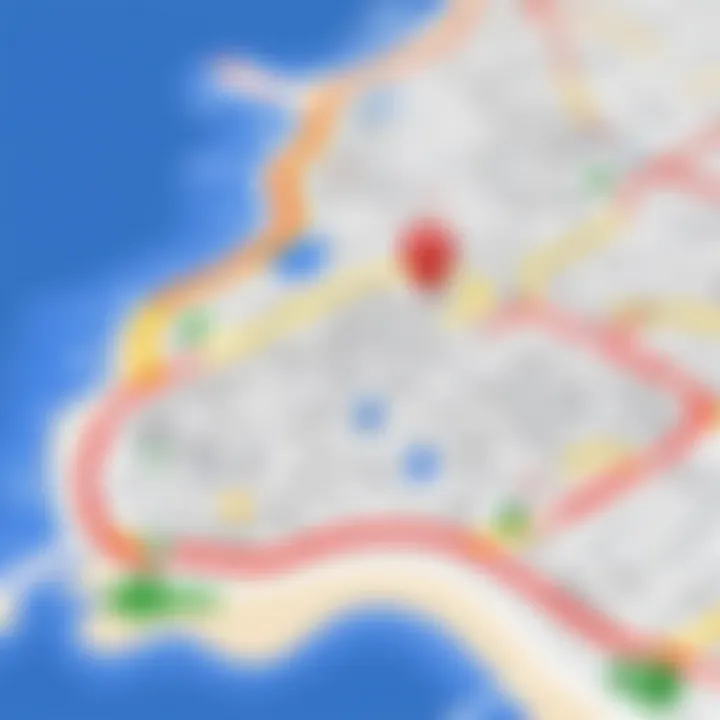
Integrating Google Maps with Java
Integrating Google Maps with Java expands the capabilities of your applications, bringing location-based services into the forefront of user experience. The Google Maps API enables developers to incorporate various mapping functionalities, enhancing data visualization and overall usability. Key benefits include the ability to display interactive maps, customize information layers, and harness real-time location data. The unique integration of these elements forms a seamless experience for the user, which is essential in today’s data-centric applications. By diving into this section, developers will learn about connecting to the Google Maps platform and how to display maps effectively in Java applications.
Making the First API Call
Connecting to the API
Connecting to the Google Maps API is crucial for any developer aiming to leverage mapping functionalities. This initial step establishes a direct communication link between the application and the API server, facilitating data retrieval. The integration process involves creating connection parameters and handling authentication securely. The simplicity of using HTTP requests to connect allows developers to effortlessly incorporate maps without deep knowledge of networking. This straightforward approach characterizes the API’s user-friendly nature, making it a popular choice among programmers.
When you perform an API connection, you benefit from returning data in a structured format, typically JSON. This format is lightweight and easy to parse, which can enhance performance. However, developers should pay attention to rate limits and response time to ensure efficient data processing. Overall, connecting to the API builds the foundation for using mapping services effectively.
Fetching Map Data
Fetching map data is a pivotal action after establishing a connection to the Google Maps API. This process allows applications to receive necessary geographical information, such as markers, routes, and other dynamic elements. By sending specific requests, such as retrieving location data based on coordinates, developers can customize the user experience.
A key characteristic of fetching data lies in its flexibility. Developers can tailor requests to acquire various formats of data, adapting the application to the unique needs of the users. The ability to handle different response formats is advantageous when building applications with diverse functionalities. On the downside, improper fetching techniques may lead to increased response times or erroneous data. Thus, a nuanced understanding of the API’s data structures is vital for optimizing the process.
Displaying Maps in Java Applications
Creating Map View
Creating a map view is the next step after fetching the necessary data from the Google Maps API. This process involves rendering the map within the Java user interface, allowing users to visualize their location context. The map view can be highly customized, with options for different map types such as roadmap, satellite, or hybrid. This flexibility facilitates specific use cases, catering to the application's purpose.
Moreover, integrating a map view directly supports user interactivity, making it a significant advantage for developers. Instead of simply viewing static data, users can Zoom in or out and explore various layers. This interactivity enriches the overall functionality. However, implementing complex layering or customized markers could impact performance if not managed properly, making efficient coding practices essential.
Embedding Maps in Swing Applications
Embedding maps in Swing applications allows for a native Java feel while leveraging the powerful mapping features of Google Maps. Swing offers a robust framework for building graphical interfaces, and integrating maps into this environment enhances the overall usability of applications. The primary advantage of using Swing for map embedding is its compatibility with Java’s core libraries, ensuring cross-platform functionality regardless of the operating system.
However, embedding maps can introduce challenges when managing events or ensuring responsiveness in dynamic applications. Developers should consider testing across different environments to maintain consistency. The powerful support available for Swing applications, combined with the rich features of Google Maps, can lead to highly effective applications.
"The integration of mapping capabilities into Java applications allows developers to create powerful solutions that meet user demands. Understanding how to connect, fetch, and display map data is indispensable."
Advanced Features of Google Maps API
The advanced features of the Google Maps API are essential for creating dynamic and responsive mapping applications. These features enhance the user experience by offering various tools and functionalities that go beyond simple map display. They facilitate a deeper interaction between users and the geographical information presented, allowing developers to tailor applications to specific needs. In this section, we will cover two key areas: Marker and Layer Management, and Geocoding and Reverse Geocoding.
Marker and Layer Management
The management of markers and layers is an integral aspect of using the Google Maps API effectively. Markers represent specific geographic points on the map, while layers help in organizing multiple data sets for better visualization.
Adding Markers to Maps
Adding markers to maps serves as one of the most straightforward ways to provide users with visual cues about important locations. Each marker can represent different entities, such as locations of interest, user-generated points, or businesses. The primary characteristic of markers is their customizability, as developers can change the icon, color, and even animations depending on user interaction. This flexibility makes them a valuable choice in applications focusing on user engagement.
A unique feature of markers is the ability to attach event listeners that respond to user interactions, such as clicks or hovers. This aspect enables dynamic content to be displayed, such as information windows or pop-ups with details about the marker. This leads to an enriched user experience and often makes the application more engaging. One possible disadvantage is the need to manage multiple markers efficiently, especially in densely packed areas, which can clutter the map and confuse users.
Working with Different Layers
Working with different map layers allows developers to overlay additional information or visual effects on top of the base map. Layers can include traffic information, public transit details, or even custom data sets relevant to specific use cases. The key characteristic of this feature is its ability to provide contextual information that aids in decision-making. For instance, users can view real-time traffic and adjust their routes accordingly.
The unique aspect of layers is their capability to be toggled on and off. This functionality grants users control over their viewing experience, allowing them to focus on what matters most to them. However, excessive use of layers may lead to performance issues, especially on devices with limited resources, causing delays in rendering the map.
Geocoding and Reverse Geocoding
Geocoding and reverse geocoding form the backbone of location intelligence in mapping applications. These features allow developers to convert addresses into coordinates and vice versa, forming a critical link between user input and mapping accuracy.
Understanding Geocoding
Understanding geocoding is vital for applications that need to convert user-entered addresses into usable latitude and longitude coordinates. This functionality not only simplifies data processing but also enriches the user experience by translating natural language into actionable data. The benefit of geocoding is clear: it allows for the precise placement of points on the map based on a simple address search.
A unique feature of geocoding is the ability to batch process multiple addresses, which can be particularly useful for applications that handle large datasets, such as real estate or delivery services. However, this feature may require rate limiting, as excessive requests to the API can incur additional charges or could lead to temporary access restrictions based on Google’s policies.
Implementing Reverse Geocoding
Implementing reverse geocoding is equally important, especially when users wish to retrieve human-readable addresses from geographic coordinates. This process enhances usability by allowing the system to respond contextually to user locations. It ensures that services are more relevant and personalized based on where a user is situated.
A significant benefit of reverse geocoding is its ability to integrate with location-based services, thereby unlocking new potentials for applications focused on navigation or local services. However, the accuracy of the results can vary based on the density of the data in a particular geographic area. Sparse or poorly indexed locations might yield suboptimal address results, which is something developers should anticipate and mitigate in their applications.
In summary, leveraging the advanced features of the Google Maps API enables developers to elevate the functionality of their applications significantly. Attention to detail in implementing markers, layers, geocoding, and reverse geocoding can lead to a more productive and engaging user experience.
Handling User Interactions
Handling user interactions is a crucial aspect of integrating Google Maps API with Java. User interactions determine how effectively users can engage with map elements, such as markers, layers, and controls. This section will explore various methods to enhance user experience while using maps in Java applications. By addressing click events and customizing user interface elements, we can create a more responsive and intuitive application.
Responding to Click Events
Click events serve as the primary means of user engagement with the map. Whenever a user clicks on a map or any element within it, the application should respond appropriately. This aspect is vital for providing real-time feedback to users, which can lead to a more enjoyable experience.
To handle click events, developers must first set up listeners within the Java application. These listeners should respond to various events, including clicks on markers or map areas. The significance of responding to these events lies in presenting critical information or functionalities, like showing the exact location of a marker or launching additional features when users click on specific areas.
Here is a simple example of how to add a click listener to a marker:
This code snippet indicates how to track the user's interaction with a map marker and perform specific actions based on that interaction.
Customizing User Interface Elements
Customizing user interface elements enhances the usability of maps within Java applications. Focused attention on UI components, such as info windows and search functionalities, can significantly improve user experience. Tailoring these elements allows developers to provide relevant information without overwhelming users.
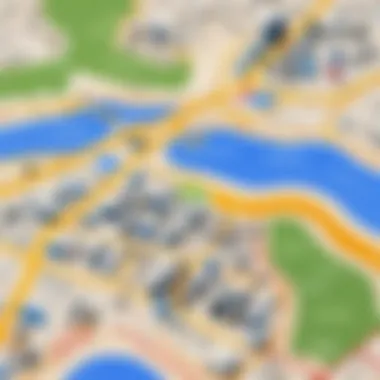
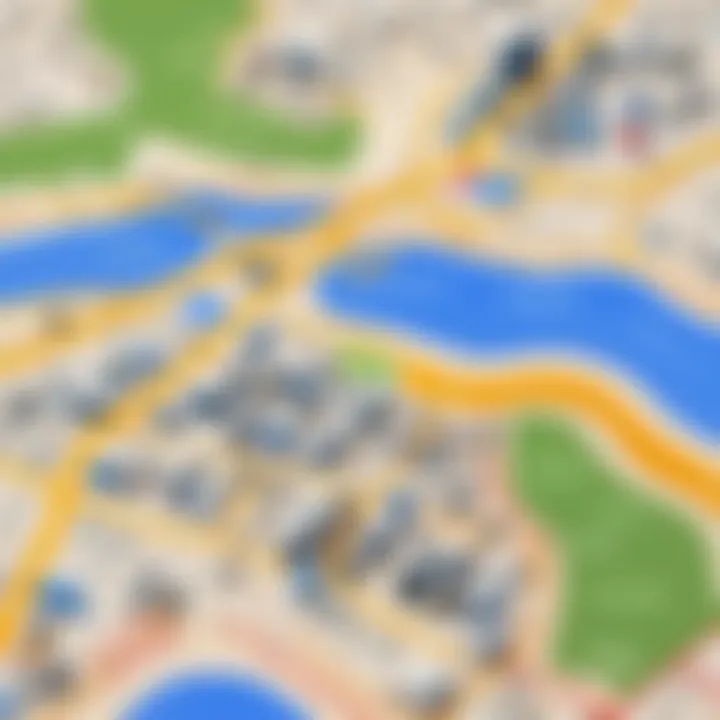
Custom Info Windows
Custom info windows are a vital component of user interface customization. They provide a way to display supplementary information when users interact with map markers. This feature contributes to the overall goal of delivering contextually relevant data, ensuring users have access to the details they need at a glance.
The key characteristic of custom info windows is their flexibility. They can be designed to match the application's aesthetic and provide comprehensive data presentation. Their popularity stems from the ability to show rich content, such as images, text, and interactive elements, all in a compact form.
One unique feature of custom info windows is their responsiveness to interaction. Users can often click buttons or links within these windows, facilitating further actions without navigating away from the map. The major advantage of using custom info windows is the enhanced engagement they foster, allowing users to dive deeper into the content related to a specific geographic area. However, developers must be careful not to clutter them, as excessive information may lead to confusion.
Integrating Search Functionality
Integrating search functionality is another crucial aspect of enhancing user interaction. This feature allows users to quickly find locations and addresses within the map interface. The convenience of having a search bar right within the map interface is a benefit to most applications, as it saves time and effort for users trying to locate specific points of interest.
The key characteristic of integrating search functionality is its focus on user convenience and accessibility. Users often prefer a quick search option rather than manually navigating the map. Besides, the ability to auto-suggest locations makes the process even smoother, improving the user's experience overall.
A unique feature of search functionality is its ability to utilize the existing database of geographic information effectively. While it enhances user engagement, it can also lead to performance issues if not implemented correctly. For instance, excessive API calls during the search can result in delays or exceed usage quotas, which can diminish the user experience. Thus, careful implementation alongside caching strategies becomes essential to optimize this feature.
Error Handling and Debugging
Error handling and debugging are critical components when integrating Google Maps API with Java. As developers, we often encounter unexpected issues that can hinder the user experience and disrupt functionality. Effective error handling ensures that your application can gracefully manage errors, allowing the application to remain stable even in the face of challenges. Debugging techniques, on the other hand, are essential for identifying and resolving these errors promptly. Working with APIs can introduce various complexities, making robust error management indispensable. In this section, we will explore common error scenarios and effective debugging techniques to empower developers in managing their applications more efficiently.
Common Error Scenarios
When working with the Google Maps API, various error scenarios can arise. Identifying these common issues can save developers time and effort in troubleshooting. Here are some typical error scenarios:
- Invalid API Key: An incorrect or expired API key can result in authentication failures. It is crucial to ensure that the provided key is valid and properly enabled for the required services.
- Quota Exceeded: Each Google Maps service has quotas that limit the number of requests made within a specific period. Exceeding these limits can temporarily restrict access.
- Network Issues: Connectivity problems can interfere with requests made to the API, leading to timeouts or dropped connections.
- Malformed Requests: Sending requests that do not conform to API specifications can result in errors. This may include issues with URL formatting or missing required parameters.
- Location Not Found: When attempting to access location-specific data, the API may return errors if the requested location is not found.
Awareness of these scenarios is the first step in effective error management.
Debugging Techniques
Debugging is a systematic approach to identifying and rectifying issues in your application. Here are two significant debugging techniques to employ when working with Google Maps API and Java:
Using Logging Frameworks
Utilizing logging frameworks is an essential practice in debugging Java applications. By logging relevant information during the execution of the program, developers can track the application's behavior and identify points of failure.
Key Characteristics: Logging frameworks, such as Log4j or SLF4J, provide a structured way to record messages. These messages can vary in severity from informational to critical, allowing developers to focus on the most pressing issues.
Benefits: Using logging frameworks simplifies the debugging process. They support different logging levels, which helps in filtering logs based on priority. Furthermore, they offer configuration options that allow developers to direct logs to various outputs, such as files or consoles.
Unique Feature: The unique feature of most logging frameworks is their ability to manage log output effectively. This capability aids developers in reviewing historical data when errors arise. However, one downside is that poorly configured logs can generate excessive data, making it harder to find the relevant information.
Network Requests Debugging
Network requests debugging is another crucial technique in diagnosing issues related to API integration. Understanding how requests are sent and responses are received can uncover underlying issues in the application.
Key Characteristics: This process involves monitoring the traffic between your application and the Google Maps API. Tools such as Postman or browser developer tools can help analyze these requests for any discrepancies.
Benefits: Network request debugging allows developers to confirm that API calls are correctly formed and responses are accurate. This transparency aids in pinpointing any problems in the communication flow, thus revealing connection issues or incorrect data being sent.
Unique Feature: An important feature of network debugging tools is their ability to visualize request and response data. This visualization aids in understanding the complete transaction cycle. However, a potential drawback is that it requires a good understanding of networking concepts, which might be intimidating for beginners.
Effective error handling and debugging are essential for a smooth integration of Google Maps API, ensuring a robust user experience and application stability.
Best Practices for Using Google Maps API
Using the Google Maps API effectively can significantly enhance the user experience of an application. Following best practices not only optimizes performance but also ensures compliance with Google's usage policies. This focus on effective integration reveals potential benefits like improved application responsiveness and user satisfaction. This section outlines essential strategies for leveraging the API while minimizing latency and maximizing efficiency.
Optimizing Performance
Performance directly influences how users interact with an application. Therefore, optimizing performance through practical methodologies can lead to a smoother experience. Two specific strategies that stand out in this regard are reducing API calls and implementing caching strategies.
Reducing API Calls
Reducing API calls is an essential aspect to enhance performance. Making fewer requests to the Google Maps API can lead to quicker response times and less strain on bandwidth. This practice is beneficial because it minimizes the chances of hitting usage limits and reduces latency. For instance, a single API call could be designed to fetch multiple points of interest instead of making individual requests for each point. This approach not only optimizes data retrieval but also decreases the overall costs associated with the API usage.
Another characteristic of reducing API calls is leveraging batch processing whenever possible. This method involves grouping multiple requests into one call, which can significantly enhance performance and user satisfaction. However, it is important to monitor the volume of data being requested, as excessively large responses could also slow down processing.
Caching Strategies
Implementing caching strategies serves as a pivotal element for performance enhancement. Caching involves storing frequently accessed data locally to reduce the need for repetitive API calls. This approach can significantly boost application response times by loading data from local storage rather than making a new request each time.
One key characteristic of caching is its ability to improve application responsiveness. By storing previously loaded maps or data, users can experience faster load times during their interactions. It is essential to manage cache effectively to ensure that outdated data does not lead to a poor user experience. For example, keeping a balance between a reasonable cache size and its expiration policy is crucial. This way, the data stays relevant without overwhelming local resources.
Ensuring Compliance with Google Policies
Compliance with Google’s policies ensures that developers maintain ethical practices and avoid penalties related to improper usage. Adhering to data usage limits and privacy requirements is essential. Developers should familiarize themselves with the Terms of Service, which outline acceptable usage scenarios and performance expectations. Additionally, designated API quotas should be monitored consistently to prevent violations that may lead to service disruptions.
The importance of this compliance cannot be understated. It builds trust with users and promotes sustainable app development practices. Following Google’s guidelines also aids in implementing features that are both reliable and scalable.
Ending
In this article, the conclusion serves as an important summary of the key learnings surrounding the integration of Google Maps API with Java. This section reinforces the value derived from effectively utilizing the API in applications, highlighting how it can enhance user experiences by providing dynamic mapping features. The importance of mastering integration techniques and understanding the API's advanced capabilities cannot be overstated.
Recap of Key Points
In summary, key points discussed in this article include:
- API Setup: Understanding the steps required to obtain an API key and configure necessary settings to commence integrating Google Maps into your Java applications.
- Core Concepts: Exploring fundamental features such as map displays, markers, and layers, which are pivotal for creating functional mapping solutions.
- Advanced Functionalities: Examining techniques for geocoding, reverse geocoding, and handling user interactions, which can significantly enrich application capabilities.
- Error Handling: Being prepared for common errors and debugging methodologies strengthens application reliability.
- Best Practices: Strategies for optimizing performance and ensuring compliance with Google’s policies help maintain application integrity and user satisfaction.
Future Trends in Mapping Technologies
As map technologies continue to evolve, several trends promise to significantly impact the future of applications using Google Maps API. Potential developments include:
- Increased AI Integration: Tools that leverage artificial intelligence for more accurate geocoding, predictive routing, and enhanced user recommendations.
- Real-Time Data Usage: The emergence of real-time data sources providing live traffic updates and route adjustments, enhancing the user's journey.
- Augmented Reality: The advent of AR features integrated into map services to present a more immersive user experience, allowing for interactive navigation.
Staying informed on these advancements will be essential for developers. Continuing to adapt and integrate new features as they arise will keep applications relevant and optimized.