Mastering the Essentials of C++ Programming
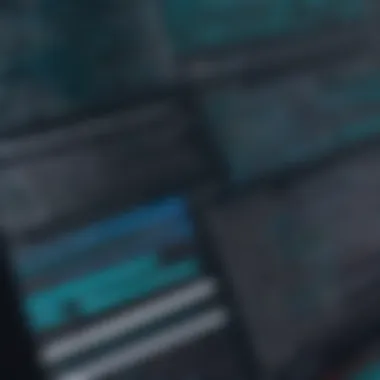
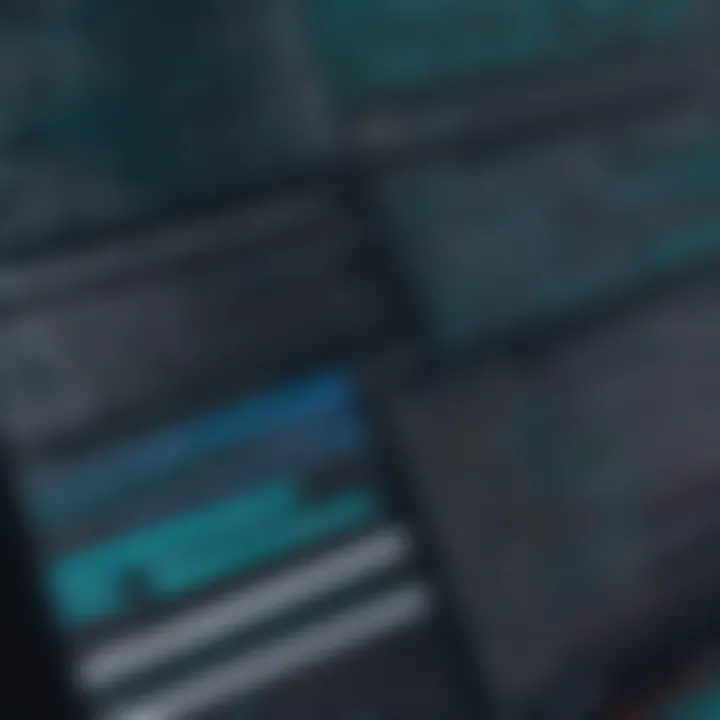
Preamble to Programming Language
As technology continues to evolve at breakneck speed, programming languages remain a cornerstone for creating software applications that power our digital world. C++ is among the languages that have stood the test of time, making it essential for anyone stepping into the realm of programming. Understanding its fundamentals ushers newcomers into a vibrant community where they can develop a variety of software solutions.
History and Background
C++ emerged in the early 1980s, conceptualized by Bjarne Stroustrup at Bell Labs. The intention was to add object-oriented features to the C programming language, providing a more flexible and powerful means for developers to tackle complex problems. The combination of procedural and object-oriented programming paradigms empowered developers to write efficient, high-performance code. Over the decades, C++ has seen several revisions, with the most recent major update being C++20, which introduced numerous enhancements that facilitate more straightforward programming patterns and improved software design.
Features and Uses
C++ boasts a rich set of features that cater to various programming needs. It allows for:
- Object-Oriented Programming (OOP): This paradigm streamlines the development process through encapsulation, inheritance, and polymorphism.
- Low-Level Memory Manipulation: Programmers have granular control over memory, which can lead to optimized performance.
- Standard Library: The C++ Standard Library offers built-in functions and classes that simplify everyday programming tasks.
These features make C++ an excellent choice for applications ranging from system software and game development to real-time simulations and even applications requiring high-performance computations.
"C++ is the best architect to build versatile and powerful software solutions, making it an invaluable tool in a developer's toolkit."
Popularity and Scope
The popularity of C++ has waned and waned over the years, but it still holds a significant position in the industry. It is among the top choices for performance-critical applications, earning its stripes in sectors like game development, finance, and in industries requiring real-time data processing. In academic settings, C++ is often the backbone for computer science curricula, cementing its role in shaping aspiring programmers. The scope of C++ seems to expand constantly with the advent of new technologies and methodologies, ensuring that today's learners can harness its power effectively in the future.
Basic Syntax and Concepts
Having gained initial insights into C++ and its significance, it's imperative to dive into the core syntax and concepts crucial for effective programming. Understanding how C++ operates is fundamental to writing any code efficiently.
Variables and Data Types
At the heart of any programming language are variables. In C++, a variable acts as a placeholder for data. Each variable has a specific type that determines what kind of data it can hold. Common data types in C++ include:
- int: Represents integer values.
- float: Used for floating-point numbers, i.e., decimals.
- char: Represents single characters.
- string: Used to represent sequences of characters.
Example of variable declaration:
Operators and Expressions
Operators in C++ allow programmers to perform specific operations on variables. They can be classified into various types, including:
- Arithmetic Operators: Perform mathematical operations like addition and subtraction.
- Relational Operators: Compare values, yielding boolean results.
- Logical Operators: Combine or invert boolean values.
Expressions result from the combination of variables and operators, yielding another value that can be assigned to a variable or evaluated further.
Control Structures
Control structures direct the flow of program execution. In C++, the primary control structures include:
- if statements: Used for conditional branching.
- loops: Such as , , and , enabling repetitive execution of code blocks.
Here’s a simple example:
Advanced Topics
With a grasp of the basics, programmers can advance to more sophisticated concepts within C++. A deeper understanding of these advanced topics can drastically improve coding skills and software architecture.
Functions and Methods
Functions are reusable pieces of code that perform specific tasks. They take inputs—also called parameters—and can return an output. For instance:
Methods are similar to functions but are tied to objects in an OOP context, allowing for structured functionality based on data encapsulation.
Object-Oriented Programming
OOP is at the heart of C++'s design philosophy. Understanding the key concepts of classes and objects, inheritance, and polymorphism is essential. For example, a class can represent a blueprint for creating objects, and derived classes can inherit properties and methods from base classes, promoting code reusability.
Exception Handling
Robust applications must anticipate errors and handle them gracefully. C++ offers exception handling through , , and keywords. This allows programs to manage unexpected events without crashing.
Hands-On Examples
Theory is well and good, but practical application is where programming comes to life. Here are some examples:
Simple Programs
Start with small exercises, such as creating a program that asks for the user’s name and age, then displays a congratulatory message. This helps cement the basic concepts while providing immediate feedback.
Intermediate Projects
Consider working on a simple game or a calculator application to challenge your skills and constructively apply various elements of C++.
Code Snippets
Integrating code snippets from projects can foster understanding and serve as references for future endeavors. Utilize platforms like GitHub to host and share your work with others.
Resources and Further Learning
To build your C++ skills and understanding further, an array of resources exist. Here are some recommendations:
Recommended Books and Tutorials
- "C++ Primer" by Stanley B. Lippman: A great resource for beginners to get up to speed with the language.
- "Effective C++" by Scott Meyers: Offers insights into best practices and common pitfalls in C++ programming.
Online Courses and Plataforms
- Coursera: Offers several courses on C++ programming that accommodate different skill levels.
- edX: Features professional courses on advanced C++ applications.
Community Forums and Groups
- Join discussions on platforms like Reddit or Facebook where fellow programming enthusiasts congregate to share knowledge, challenges, and solutions.
Prelims to ++
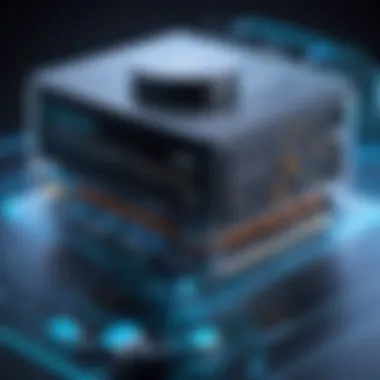
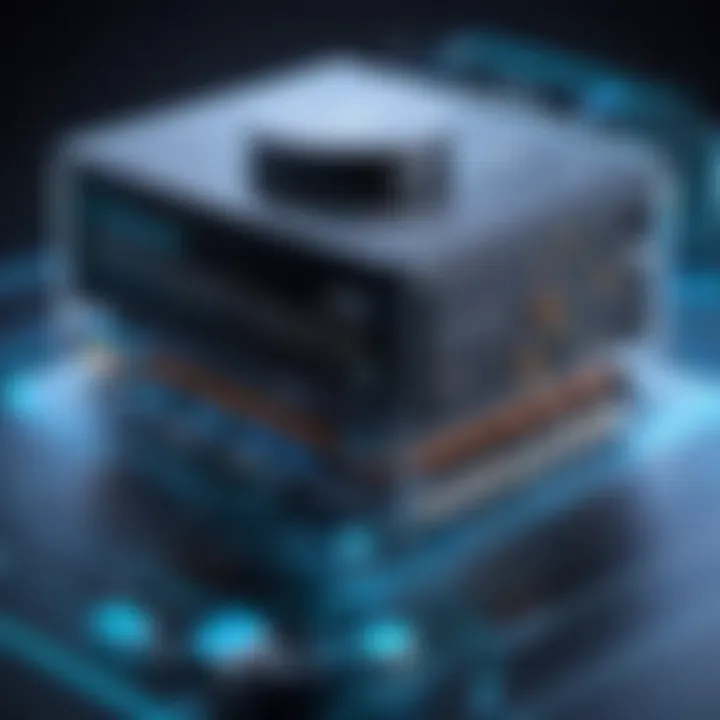
C++ is not just another programming language; it stands as a fundamental pillar of the coding world. Its significance spans decades and still resonates in the realms of software engineering, game development, and performance-critical systems. Whether you're a fresh-faced student eager to delve into the world of programming or a seasoned coder brushing up on core concepts, understanding C++ is indispensable. This section aims to elucidate C++'s pivotal role, both in the past and in contemporary applications, while paving the way for further exploration into its features and functionalities.
Brief History of ++
C++ has a storied history, tracing its roots back to the early 1980s. It emerged from the mind of Bjarne Stroustrup at Bell Labs, who sought to augment the C programming language by adding object-oriented programming features. Initially called "C with Classes," it underwent various refinements before becoming known as C++. The name itself hints at its evolution—"C++" signifies an increment in functionality, akin to the increment operator (++) in the language itself.
In its early days, C++ was recognized for its prowess with system software and application development. Over time, as technology advanced, so did C++. The language embraced numerous standards, with C++98 and C++11 introducing significant enhancements, followed by C++14, C++17, and most recently, C++20. Each iteration expanded the language's capabilities, offering improved performance, enhanced functionality, and greater ease of use. The nostalgic echoes of its lineage still ring true, as many concepts rooted in C++ have flourished in languages that came after, like C# and Java.
Why Choose ++?
Opting for C++ comes with a bundle of benefits that any aspiring coder would appreciate. Here are a few compelling reasons to consider:
- Performance: Highly efficient when it comes to execution time and resource usage, C++ allows programmers to write code that runs incredibly fast, making it suitable for high-performance applications like games and real-time systems.
- Rich Libraries: The Standard Template Library (STL), which is a powerful and versatile collection of templates, enables rapid development through reusable components.
- Object-oriented: With its focus on classes and objects, C++ promotes a clear organizational structure, enhancing code readability and maintainability.
- Cross-platform: One of its strong suits is the ability to run on various platforms, making it a favorite for software that demands high portability.
- Community and Resources: C++ has a vast community of developers, offering copious resources, tutorials, and forums. Whether you hit a wall or unlock a new concept, help is often just a few clicks away.
In summary, the benefits of learning C++ are plentiful, making it a worthy addition to any programmer's toolkit. Its blend of flexibility, power, and efficiency ensures that it won't fall out of favor any time soon. Take the plunge into C++, and you may find it one of the most rewarding programming languages you'll ever master.
Setting Up Your Environment
Programming in C++ begins long before you start writing code. A crucial first step is setting up your programming environment. This phase can be likened to preparing a canvas for an artist. Without the right tools, even the most talented individuals struggle to create their masterpieces. Let's dive into what’s involved in getting your environment ready and why it matters.
The primary goal of setting up your environment is to create a space that optimizes your productivity and makes it easier to spot and fix errors. When everything runs smoothly, you can focus on learning and developing your skills without the hassle of technical hiccups. For newcomers, this setup may seem daunting. But once you grasp the key components, it becomes second nature.
Choosing an Integrated Development Environment (IDE)
Selecting an Integrated Development Environment (IDE) is like picking a pair of shoes for a marathon. It needs to fit well and support you throughout your journey. IDEs offer features like code suggestions, debugging tools, and built-in compilation support that can significantly enhance your learning experience. Common choices include Microsoft Visual Studio, Code::Blocks, and CLion, each with unique strengths.
- Microsoft Visual Studio: This is a powerful option, especially for Windows users. It comes packed with features and is great for those intending to work on larger projects or applications that require heavy lifting.
- Code::Blocks: A more lightweight alternative, this IDE is user-friendly and also cross-platform. It’s a favorite among learners thanks to its simplicity.
- CLion: Designed for C and C++ development, CLion offers smart coding assistance. The downside? It requires a subscription for full features.
When choosing an IDE, consider factors such as:
- Your operating system
- The types of projects you wish to work on
- Available features that suit your programming style
You might want to try a few different options before settling on one that feels right. After all, it's about what works best for you.
Installing ++ Compilers
Once you've settled on an IDE, it’s time to install a C++ compiler. Think of the compiler as the translator between your code and the machine. Compilers convert the high-level C++ code you write into machine code that your computer can execute.
Popular compilers include GCC (GNU Compiler Collection) and Clang. Here’s a quick look at what they bring to the table:
- GCC: Extremely robust and widely used, it supports many programming languages besides C++. Not just for experienced programmers, it’s also free and open-source, making it accessible for individuals at every skill level.
- Clang: Known for its speed and efficiency, Clang has gained popularity for its user-friendly error messages, which can make debugging easier.
To install these compilers, follow the steps outlined for your operating system:
- Windows: You can install MinGW, which comes with GCC.
- Linux: Often comes pre-installed. You can check by typing in the terminal. If it’s not there, simply use your package manager.
- MacOS: Install Xcode, which contains Clang.
"A good compiler not only compiles your code, but also helps you learn from your mistakes!"
With the compiler installed, verify it’s working by compiling a simple program. Just type a short snippet of C++ code, compile it, and check if everything runs smoothly. If it does, consider it a green light to start delving into the realms of C++.
Setting up your environment may require some time and patience, but the fruits of your labor will be a solid foundation for your C++ programming journey.
Understanding Basic ++ Syntax
C++ serves as a robust tool in the programming ecosystem, exploited for its versatile syntax that is not only powerful but also approachable for budding and seasoned developers alike. Grasping the fundamental syntax of C++ sets a solid groundwork, vital for successfully navigating advanced topics in software development. Proficient understanding of syntax encompasses recognizing how to effectively communicate with the compiler, enabling you to translate human logic into machine language seamlessly.
Syntax is akin to a language's grammar; without it, the meaning of sentences is lost. In C++, syntax dictates how code is written to perform specific functions or tasks. Therefore, mastering this aspect of C++ allows programmers to articulate their intentions clearly, reducing errors and frustrations in coding. Here, we will delve into several critical elements: Variables and Data Types, Operators, and Control Structures. Each of these components plays a significant role in shaping the functionality and logic of a C++ program.
Variables and Data Types
At the heart of programming, variables act as containers for storing data that can be referenced and manipulated throughout a program. In C++, every variable must have a specific data type that dictates what kind of values it can hold. Options range from integers, floating-point numbers, characters, to more complex data types like arrays and structures. For instance, an can store whole numbers like 5 or -3, while a can address decimals like 3.14.
Variables in C++ are defined by declaring their type, followed by a chosen name. This simple yet powerful mechanism allows programmers to create dynamic programs that respond and adapt to various inputs. Here’s an example declaration:
Understanding the significance of different data types is crucial because it directly impacts memory usage and performance. Using the wrong data type can result in inaccurate calculations or even program crashes. Moreover, C++ provides the flexibility to create user-defined data types, enhancing how you manage complex data.
Operators in ++
Operators are essential building blocks in the manipulation of variables and data. C++ provides a rich set of operators that allow for various operations on data, such as arithmetic operations (addition, subtraction, etc.), relational checks (greater than, less than), and logical conjunctions (AND, OR).
For instance, arithmetic operators are fundamental for performing calculations. They connect two or more values and produce a new value based on a particular operation. Here’s a typical example using an arithmetic operator:
Understanding how to use operators effectively expands a programmer's capacity to handle and manipulate data, making it crucial for building functional programs.
Control Structures
Control structures govern the flow of execution in a program, steering it based on certain conditions or iterations. C++ primarily utilizes conditional statements and loops, both of which are pillars of program logic.
Conditional Statements
Conditional statements, or if-else statements, empower programmers to make decisions in their code. You can execute certain blocks of code depending on whether a condition evaluates to true or false. This aspect is pivotal because it allows for creating dynamic behaviors in programs. For example:
The key characteristic of conditional statements lies in their flexibility. They enable branching logic, essential for accommodating various scenarios that arise during program execution. Moreover, you can create nested conditions, although caution is advisable to maintain clarity in your code.
Remember: Keeping conditional logic simple increases readability and reduces bugs!
Loops
Loops facilitate repetition in programming—allowing a block of code to be executed multiple times until a specified condition is met. This not only streamlines code but also reduces redundancy, contributing to cleaner and more efficient scripts. C++ supports several looping constructs, such as , , and . The loop is particularly adept at automating repetitive tasks that require a predetermined number of iterations. Here’s an example:
Loops in C++ are favored for their efficiency and clarity, promoting a smooth flow of operations. However, caution must be exercised to avoid infinite loops that can hang or crash a program.
Together, these foundational elements of C++—variables, data types, operators, and control structures—form a precise and expressive syntax that prepares you to tackle more intricate programming challenges ahead.
++ Functions
In C++, functions are more than mere snippets of code; they are the building blocks of efficient programming. They not only encapsulate functionality but also enhance the clarity and reusability of your code. When you lay out the framework of a C++ program, think of functions as neatly organized drawers in a filing cabinet. Each function does its job without needing to suck up the main workspace with details, helping keep your program organized.
A well-defined function can improve maintenance, make testing easier, and foster collaborative work. So how do you define and leverage functions effectively in C++? Let's break it down.
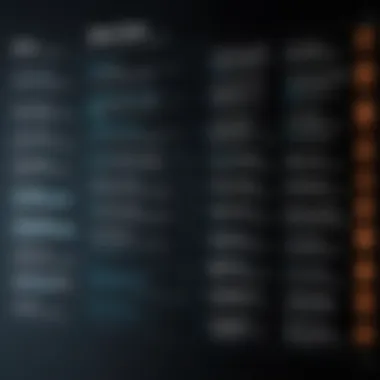
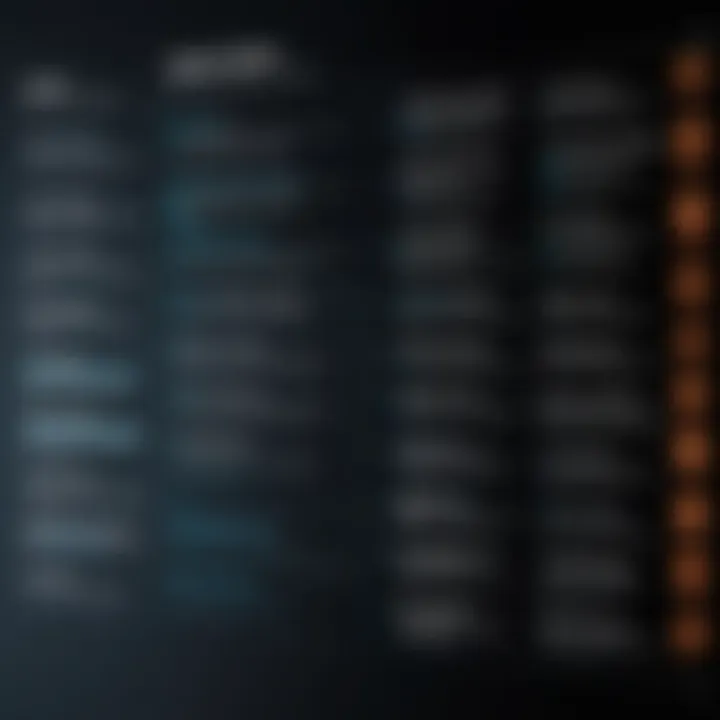
Defining Functions
Defining a function in C++ is quite straightforward. In essence, you need to declare the return type, function name, and parameters. It’s like crafting a recipe: you list what you need, what you’ll create, and the steps to blend them all together. Here’s an example:
This simple function named takes two integer inputs, adds them, and returns the result. The return type——indicates that this function will give back an integer value. It’s critically important to define parameters that accurately reflect what your function needs to perform its task. You can also define functions without parameters, just like a recipe that doesn’t require any ingredients.
Function Overloading
Function overloading is a feature in C++ that allows you to define multiple functions with the same name but different parameters. Picture a toolbox where the same tool can serve various purposes; the versatility is a game-changer. For example:
In this scenario, both functions are named , but one works with integers, while the other works with doubles. The compiler differentiates between them based on the arguments passed. This means no more clumsy names that become unwieldy as your program grows. Function overloading is a neat way to keep your code clean and understandable.
Recursion in ++
Recursion is a nifty concept in C++ where a function calls itself, directly or indirectly. It's like a mirror reflecting itself in another mirror, producing an infinite view. Recursion is particularly useful for solving problems that can be broken down into smaller, more manageable parts. For instance:
This function calculates the factorial of a number. Here, it calls itself with a decremented value until it hits the base case of . One needs to be cautious with recursion; if not implemented correctly, it can lead to stack overflow. The essence of recursion lies in understanding the problem at hand and designing a base case that will eventually halt the endless loop.
Key Insight: Functions in C++ not only modularize code but also foster better organization and readability, making your programming journey smoother.
Understanding functions is fundamental in programming; they are indispensable in building complex applications. Each function adds another layer of sophistication while allowing for simpler troubleshooting, overall enhancing the development experience. So when diving into C++, embrace the power of functions, keep them well structured, and let them be the compass that navigates through your code.
Object-Oriented Programming Concepts
Object-Oriented Programming (OOP) stands as a cornerstone of modern programming, particularly within C++. This approach, unlike others, structures software design to resemble real-world systems, casting classes and objects as the primary elements. The advantages of utilizing OOP in C++ include enhanced modularity, easier maintenance, and reusable code—key features that cater to both novice and seasoned programmers alike. With these benefits, it becomes clearer why OOP is indispensable in crafting high-level applications effectively.
Understanding Classes and Objects
Classes and objects represent the foundation of OOP in C++. A class can be thought of as a blueprint that outlines the properties (attributes) and behaviors (methods) of a certain kind of object. For instance, consider a class. This class might have attributes such as , , and , while the behaviors might include methods like or . Objects, on the other hand, are instances of classes. When you create a new object, you’re using that blueprint to make a specific vehicle, say a red 2020 Honda Civic.
Here's a simple example to illustrate this concept:
Using classes and objects allows programmers to encapsulate data and functionalities, streamlining their code significantly. This encapsulation promotes data hiding and minimizes the risk of accidental exposure of internal states, making the system more secure and understandable.
Inheritance in ++
Inheritance is a mechanism that facilitates the new class (subclass or derived class) to inherit attributes and methods from an existing class (base class or parent class). This is akin to how one might inherit traits within a family, leveraging the established foundation while being able to extend or modify it. It fosters code reuse and logical structure.
For example, if you have a base class with some common attributes and methods, you can create specific classes like and derived from it that inherit those properties while adding their unique features. Here's an example:
In this setup, both and inherit the speed and acceleration methods from , thus eliminating code redundancy while promoting a clear organizational structure.
Polymorphism Explained
Polymorphism, perhaps a bit of a tongue twister, simply means "many shapes." In C++, it allows objects of different classes to be treated as objects of a common superclass. It plays a crucial role in OOP as it promotes flexibility and the implementation of abstract concepts. This means different classes can be designed with the same interface, but with specific implementations for their methods.
There are two types of polymorphism in C++: compile-time (or static) polymorphism, achieved through function overloading, and run-time (or dynamic) polymorphism, primarily through virtual functions.
An everyday example is a method in a class hierarchy involving different shapes. Each shape, like and , implements its way to draw, but from a common interface:
With polymorphism, you can have an array of pointers and invoke on any object, and it will call the appropriate method based on the actual object type, whether it’s a or .
Polymorphism is pivotal in crafting scalable and adaptable C++ applications, enabling fine control over behaviors without cumbersome code changes.
Memory Management in ++
Effective memory management is a cornerstone of writing efficient programs in C++. In C++, developers have a vast range of control over system resources, which can lead to high performance but also comes with its own set of challenges. This section aims to discuss the methods used for managing memory in C++, focusing on dynamic memory allocation and issues related to memory leaks.
Dynamic Memory Allocation
Dynamic memory allocation enables the program to request memory during its runtime, allowing for the creation of data structures that can grow or shrink as the program executes. Unlike static memory allocation, where the size must be known at compile-time, dynamic allocation gives developers the flexibility to use only the needed amount of memory. This is particularly useful in situations where the data size is not predetermined.
For example, consider a situation where a program needs to manage an array of user inputs. If the array size is fixed at compile time, you might end up wasting memory or running out of space. Using the keyword, a developer can allocate memory only when required. This memory remains in the heap until it is de-allocated using the keyword.
Here's an example in C++:
However, dynamic memory comes with its own risks, especially related to improper management of allocated resources.
Memory Leaks and Management Strategies
Memory leaks occur when the program allocates dynamic memory but fails to release it back to the system. Over time, this can consume a significant amount of memory and slow down or even crash a program. Not only does this impact the performance, but it can also cause unexpected behaviors that are hard to debug.
Best Practices to Avoid Memory Leaks:
- Always deallocate memory: Make it a practice to pair every with a . If you allocate an array, make sure to use .
- Use smart pointers: Modern C++ offers smart pointers, like and , which help automate memory management. These pointers take care of deallocation when they go out of scope, effectively reducing the risk of memory leaks.
- Regularly test for leaks: Tools such as Valgrind can be used to detect memory leaks in C++ applications. Employing these tools during development can catch leaks before they become problems in production.
"The best defense against memory leaks is a good offense. Proactively managing your resources can save you countless headaches."
In summary, understanding memory management in C++ is not just about allocation; it involves actively managing the lifecycle of resources. By mastering dynamic memory allocation and adopting effective strategies to prevent leaks, a developer can ensure that their applications run smoothly and efficiently.
Handling Errors and Exceptions
Error handling and exception management in C++ is an essential piece of the programming puzzle. It’s about more than just preventing crashes; it’s about enabling your programs to respond to unexpected situations gracefully. In the ever-evolving landscape of software development, robust error handling can be the difference between a functional application and a complete disaster.
By implementing effective error handling strategies, you can ensure that your program behaves predictably, even when things go south. This not only improves the user experience but also simplifies the debugging process. Imagine a scenario where an application crashes because it failed to read a file. Without proper error handling, pinpointing the issue could feel like finding a needle in a haystack. However, with a systematic approach, you can catch these issues before they escalate into larger problems.
With the fundamentals in place, let’s move on to specific techniques you can use.
Using Try and Catch
In C++, one of the primary mechanisms for handling errors is the and block. This structure allows you to write cleaner code, isolating regular operations from those that may throw exceptions.
Here’s how it generally works: You place the code that might throw an exception within a block. If an exception occurs, control is passed to the block that follows.
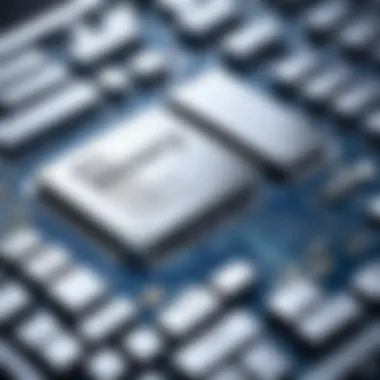
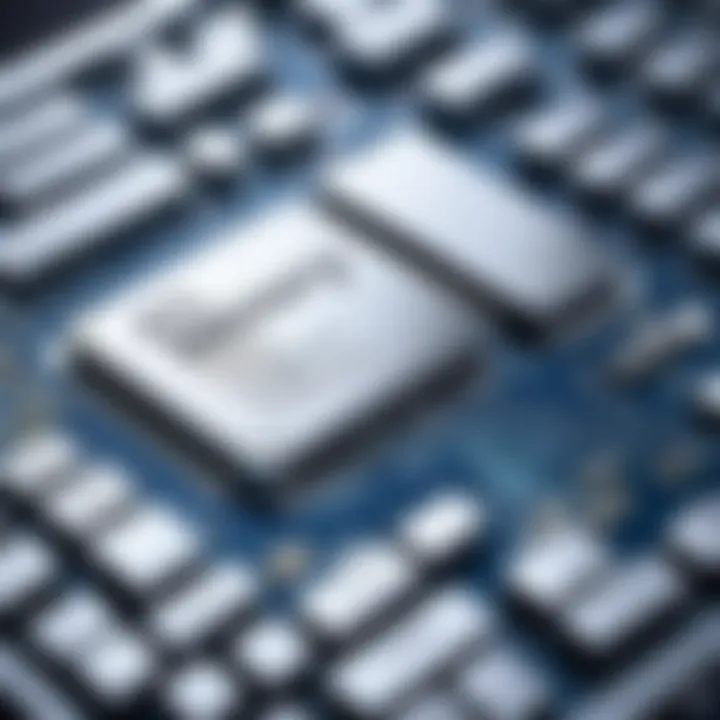
Why use this approach? It keeps your error handling separate and makes it easier to read your code. Here’s an example that illustrates this:
In this code, if an exception is thrown, it’s caught by the block, and an error message is displayed instead of the program crashing. This effectively allows you to address issues without compromising the overall functionality.
Best Practices in Error Handling
When it comes to error handling, there are some strategies worth considering to make your code cleaner and more maintainable:
- Be Specific with Exceptions: Catch exceptions by type instead of catching everything with a generic exception. This helps maintain clarity.
- Avoid Silent Failures: Ignoring exceptions can lead to harder-to-track bugs later. Always log or handle exceptions appropriately.
- Resource Management: Remember to handle resources correctly, perhaps through resource management techniques. Using RAII (Resource Acquisition Is Initialization) can prevent memory leaks even if exceptions occur.
- Fail Fast: If your program is in a faulty state, it’s often best to abort execution rather than attempt to recover from the error. This can prevent further issues down the line.
Effective error handling isn’t just a luxury. It’s a lifeline for your software, allowing you to manage, recover from, and diagnose unexpected issues right when they arise.
Adopting these practices can transform your program from a fragile entity into a robust application capable of handling a variety of errors and exceptions. So, focus on mastering these concepts, as they’ll serve you well in any programming venture.
Standard Template Library (STL)
The Standard Template Library, commonly known as STL, plays a significant role in the C++ programming language. It provides ready-to-use classes and functions that enable programmers to leverage powerful data structures, algorithms, and functions without the need to create them from scratch. This can save a lot of time and effort while ensuring efficient performance of C++ applications.
STL is crucial for those venturing into more complex programming tasks. As you delve into C++, you'll find that mastering STL can greatly enhance your programming efficiency and capability. With STL, C++ programmers can handle collections of data easily and rapidly.
Prolusion to Containers
One of the cornerstones of STL is its containers. Containers are essentially objects that store data. STL provides a variety of container types designed for specific needs. Some of the widely used containers are:
- Vector: This is a dynamic array that can grow as needed. It's like a regular array but with the added ability to change size. When you want to append elements easily, vectors are your go-to structure.
- List: Unlike vectors, lists allow for efficient insertion and deletion of elements at any position. They don't guarantee memory locality as vectors do, but they shine when you frequently modify the size of the container.
- Map: This is a collection of key-value pairs. It allows for fast retrieval based on keys, making it ideal for associative arrays. If you need to search for data quickly and pair it with a key, maps can be a huge help.
- Set: Sets are unordered collections of unique elements. They are best when you want to store distinct items and check for membership without worrying about duplicates.
The flexibility offered by these containers enables you to choose the most appropriate type depending on the problem at hand.
Here’s a simple example using a vector:
This code initializes a vector of integers, adds a few elements, and prints them. You can see how quickly you can manage a list of data with STL!
Algorithms Offered by STL
Alongside the rich variety of containers, STL also comes packed with a toolbox of algorithms. These algorithms can be utilized with the containers to manipulate data, making tasks such as searching, sorting, and transforming datasets remarkably straightforward. Here are key algorithms provided by STL:
- Sorting: With algorithms like , you can easily sort your containers. This saves you from having to implement your own sorting algorithm, which can be tricky.
- Searching: Algorithms like help you quickly locate elements in your containers. Instead of looping through items manually, this function does the heavy lifting for you.
- Transformations: Functions such as let you apply transformations to your data easily, allowing for concise coding.
- Accumulation: You can sum up your elements through . This is handy when you want quick results without a lot of boilerplate code.
STL’s combination of containers and algorithms fosters a powerful programming environment. By understanding how to leverage these efficiently, you will elevate your C++ programming capabilities considerably.
"Using STL in C++ is like having a robust toolkit at your disposal: the more you explore it, the easier it is to tackle complex problems with simplicity."
Incorporating STL into your programming practice will not only speed up development but also enhance the overall quality of your code. Remember, when you write—write with clarity and intent, and the STL will guide you along the way.
Practical Applications of ++
C++ is not just another programming language; it’s a powerhouse with real-world applications that span various domains. Understanding how C++ is applied practically is essential for both aspiring and seasoned programmers. Recognizing these applications instills an appreciation for the versatility of the language. You could say it’s where the theoretical meets the practical, turning lines of code into tangible outcomes.
++ in Game Development
Game development stands as one of the most vibrant realms for C++. It’s not just about creating visuals or engaging narratives; it’s about performance and control. C++ provides the speed and efficiency necessary for complex simulations and real-time graphics. It’s a favored choice for many major game engines, like Unreal Engine. This powerful engine allows developers to create breathtaking 3D worlds while leveraging the strengths of C++.
"In the world of game dev, C++ is like a Swiss Army knife—multifunctional and indispensable."
C++ enables developers to manipulate hardware directly, making it easier to optimize performance. This means frame rates are high, graphics are sharp, and user experiences are smooth. Since many games involve heavy graphics and physics calculations, the efficiency of C++ makes it the go-to choice.
Consider the intricacies involved in crafting a character’s movements, calculating collision detection, or managing complex AI behavior. Each of these elements demands fluid execution. Using C++, developers have the ability to create intricate algorithms that ensure the game runs without a hitch. Furthermore, the object-oriented nature of C++ allows for modular design of game features, fostering reusability and easier maintenance.
++ in System/Operating Software
When it comes to system or operating software, C++ holds a significant position as well. Operating systems (OS) like Microsoft Windows are largely written in C++. The language’s ability to provide a mix of high-level features with low-level memory manipulation makes it ideal for this domain.
Operating systems manage computer hardware and software resources; thus, they require a language that operates effectively on multiple levels of abstraction. C++ gives developers precise control over system resources, contributing to stability and efficiency. This aspect is vital, especially in environments that demand robust performance under stress, like servers or industrial automation systems.
Additionally, software applications, utilities, and drivers are also commonly built in C++. These applications often require high-performance processing and must interact closely with the hardware, which is C++’s forte. Therefore, understanding C++’s role in system development is crucial for grasping how software interfaces with the underlying hardware, laying the foundation for future advancements in technology.
By exploring these practical applications, programmers can identify suitable contexts for employing C++. This not only enhances one's coding skills but also equips them to tackle real-world challenges. Ultimately, this bridges the gap between theory and actuality, showcasing C++ as a vital tool in the programmer’s toolkit.
Future Trends in ++ Programming
As the world of technology continues to evolve, so does programming, especially with a versatile language like C++. Understanding the future trends in C++ programming is vital not just for staying relevant but also for leveraging emerging capabilities that C++ has to offer. This section sheds light on the evolution of C++ standards and how new technologies are shaping the C++ landscape, providing readers with a roadmap to navigate their future in software development.
Evolution of ++ Standards
Over the years, C++ has seen its fair share of updates, each bringing new features and enhancements aimed at improving performance and usability. The journey from C++98 to C++20 showcases the language's adaptability and commitment to modern programming requirements. It's worth noting that the introduction of features such as auto keyword, lambda expressions, and smart pointers not only changes how developers write code but also how they think about coding in a more efficient way.
C++ standards evolve through a collaborative effort involving the industry, academics, and the community. The following points highlight key aspects that mark the evolution of C++ standards:
- C++11: Introduced concepts like range-based for loops, nullptr, and concurrency features, making parallel programming more accessible.
- C++14: Refined features from C++11 and added some new ones like generic lambdas, enhancing code reusability.
- C++17: Introduced parallel algorithms in the Standard Library and optional types, paving the way for better performance in multi-threaded applications.
- C++20: Brought in concepts, modules, and co-routines, which are set to revolutionize how large-scale software systems are built by simplifying and organizing codebases.
It's important for programmers to adapt to these updates, as they not only improve performance but also help in writing cleaner and more maintainable code. Making a habit of keeping up with the latest standards ensures proficiency in the latest programming techniques.
"Staying updated with C++ standards isn't just a good practice; it's essential for any serious programmer's toolkit."
Emerging Technologies and ++
With the proliferation of new technologies such as artificial intelligence, machine learning, and the Internet of Things, C++ remains a significant player. As applications demand ever-greater efficiency and capability, C++ continues to find its newfound roles alongside these technologies.
- Game Development: Many modern game engines like Unreal Engine still utilize C++ for its performance advantages. The trend toward more immersive and complex gaming environments means that a robust language like C++ is essential.
- Data Science: While languages like Python often dominate in the data science field, C++ has gained traction for scenarios demanding high-performance computations. Libraries like TensorFlow make use of C++ for backend operations, optimizing speed and efficiency.
- Artificial Intelligence: C++ aids in performance-intensive AI applications. Its low-level capabilities allow for system-level memory management, making it an excellent choice for developing algorithms that require heavy computations.
- Internet of Things (IoT): C++ is also being widely adopted in IoT applications due to its ability to work closely with hardware. The lightweight nature of C++ makes it ideal for running on devices with limited processing power.
Closure
Drawing the curtain on this exploration of programming in C++, it's vital to underscore what we’ve tackled along the way. This section is not just the finale; it’s a pivotal juncture, reinforcing the core knowledge accumulated throughout the article. Without a doubt, grasping C++ is like holding a multi-tool – versatile and indispensable in the ever-evolving world of software development. Understanding the fundamentals of any programming language establishes a strong foundation from which one can delve into more advanced topics seamlessly.
In the journey through these pages, we took a thorough look at the language’s unique syntax, control structures, and powerful object-oriented features. The examination of how memory management works plus how to handle errors equips readers to fortify their coding practices.
Recap of Key Concepts
"Programming isn't just about writing code; it's a way to express logical solutions to complex problems."
It’s essential to reflect on the highlights:
- Basic Syntax: We demystified the elements like variables, data types, and operators. These serve as the building blocks for all programming tasks.
- Control Structures: Conditional statements and loops give the ability to direct the flow of execution, an element crucial in crafting efficient algorithms.
- Functions: Understanding how to define, overload, and use recursion in functions opens doors to modular and clean code.
- Object-Oriented Programming: Classes, inheritance, and polymorphism encourage the reuse of code, making development faster and management easier.
- Memory Management: Discussing dynamic memory allocation and prevention of memory leaks guides best practices, crucial for optimizing application performance.
- Error Handling: Techniques using try-catch structures to manage exceptions ensure robustness in programs.
- STL and Practical Applications: Knowing how to leverage the Standard Template Library provides tools that speed up development, alongside the diverse realms where C++ excels, like game development and system software.
Next Steps in Learning ++
Now that you have a solid grasp of fundamental concepts, it’s time to put that knowledge into action. Here are some prudent steps to further your C++ proficiency:
- Hands-On Coding: Choose simple projects that resonate with your interests, whether it's a game, a utility, or a small application. Building something from scratch will cement your understanding.
- Explore Advanced Topics: Dive into multithreading, networking, or graphical programming as your confidence grows.
- Contribute to Open Source: Engaging in open source projects on platforms like GitHub can provide real-world experience and community support.
- Continuing Education: Consider enrolling in online courses or webinars dedicated to advanced C++ techniques and practices.
- Join Communities: Engage with fellow learners on platforms such as Reddit or specialized forums. Sharing experiences can unveil insights that you might overlook in isolation.
In a nutshell, the knowledge acquired here serves as a launching pad into the vast possibilities that C++ programming offers. Keep that learning momentum going, and don't hesitate to reach out for further resources or support as you dive deeper into the C++ landscape.