Mastering the Essentials of C Programming Language
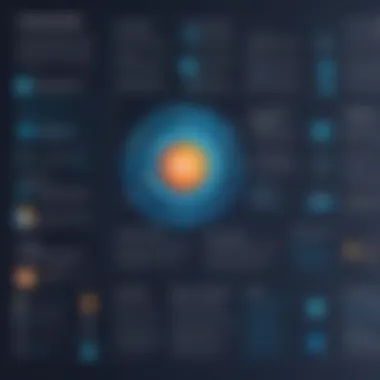
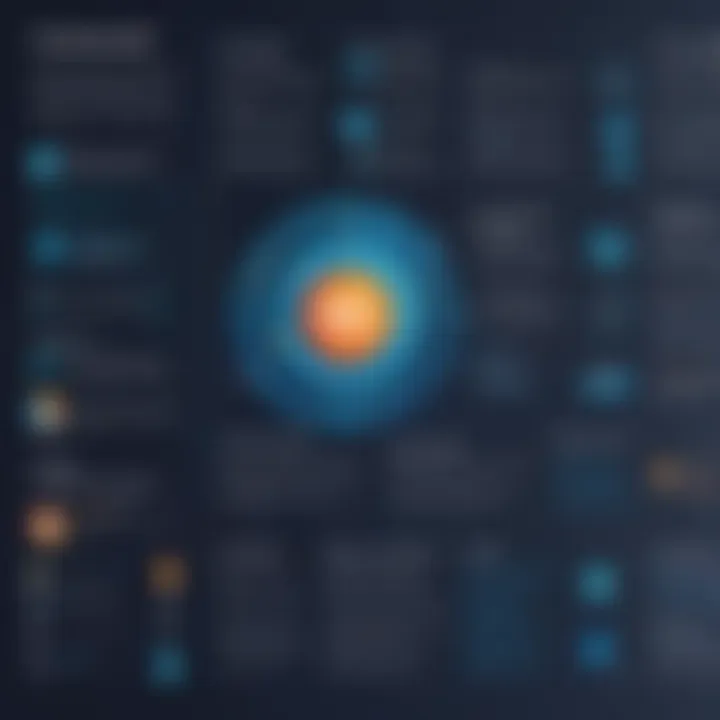
Intro
The essence of programming lies in understanding how to communicate with computers effectively. At its core, a programming language serves as a bridge between human intentions and machine processes. Among this vast landscape, C language holds a venerable position. Its influence is profound, acting as the cornerstone for many modern programming languages.
History and Background
C language emerged in the early 1970s, primarily developed by Dennis Ritchie at Bell Labs. It evolved from previous languages like B and BCPL, primarily designed for system programming and to write operating systems. Over the years, C has been synonymous with flexibility and performance. Its creation paved the way for the development of Unix, which further amplified its significance.
"C is quirky, flawed, and an enormous success." - Rob Pike
Features and Uses
C language provides a rich set of features that cater to both low-level and high-level programming needs. Here are some highlights:
- Efficiency: C is known for its ability to produce fast-running programs. The language allows direct manipulation of hardware, yielding superior performance.
- Portability: Programs written in C can be compiled and run on various platforms, making it highly versatile.
- Rich Library Support: A wealth of libraries is available for C, facilitating various functionalities ranging from simple I/O operations to complex mathematical calculations.
This is why C remains a popular choice for system software, embedded systems, and application development.
Popularity and Scope
Despite being over five decades old, C's popularity has not waned. Today, it is taught in computer science programs worldwide. An interesting aspect is the sheer number of languages influenced by C's syntax and structure, such as C++, C#, and Java.
In particular, developers appreciate its close-to-the-metal performance. Many applications, from operating systems like Linux to modern development environments, owe their existence to the capabilities offered by C. So, whether you are exploring embedded devices or complex algorithms, knowing C opens many doors.
Basic Syntax and Concepts
Understanding the basic syntax and concepts in C is essential to harness its power effectively. Just as knowing the alphabet is a prerequisite to reading, grasping C programming basics lays the groundwork for further exploration.
Variables and Data Types
In C, a variable acts as a symbolic name for a storage location, where data can be stored and manipulated. Each variable has a type, which dictates the kind of data it can hold. Here are some of the fundamental data types:
- int: Used for integer values.
- float: Represents floating-point numbers.
- char: Holds character data.
For instance, declaring an integer variable can be done as follows:
Operators and Expressions
Operators in C perform operations on variables and values. C supports various operators like arithmetic ( +, -, *, /), relational (==, !=, , >), and logical operators (&&, ||). Understanding how to construct expressions is vital, as they form the bedrock of computations in your programs.
Control Structures
Control structures dictate the flow of a program. C provides several control structures, such as:
- if-else statements: Allow decision-making based on conditions.
- for loops: Enable iteration over a block of code.
- while loops: Allow repeated execution based on a true condition.
These structures help dictate how a program behaves under specific conditions, making them essential in any programmer's toolkit.
Advanced Topics
After getting a grip on the basics, thereās a whole new world to explore in C.
Functions and Methods
Functions in C allow for code reusability and clarity. They help segment code into manageable sections, making it easier to understand and maintaining it. Each function can take parameters and return values, thus enabling modular programming.
Object-Oriented Programming
Although C is primarily a procedural language, concepts of object-oriented programming can be simulated using structures and pointers. While pure OOP is more prominent in C++, the fundamental principles of encapsulation can still be applied.
Exception Handling
Handling errors is pivotal in coding. While C does not support exceptions in the traditional way like other languages, error handling is typically managed through return values and global variables, such as errno.
Hands-On Examples
What better way to solidify understanding than with practical examples? Here, you can engage with the language directly.
Simple Programs
A good starting point is creating a simple "Hello, World!" program. Itās a rite of passage for many programmers.
Intermediate Projects
From simple arithmetic calculators to text-based adventure games, there are plenty of opportunities to stretch your C muscles. Building a calculator in C can help understand control structures and functions more deeply.
Code Snippets
Exploring snippets of code can often ignite inspiration.
Resources and Further Learning
The journey of learning C can begin with several resources. Here are some valuable insights to guide you along:
Recommended Books and Tutorials
- The C Programming Language by Brian Kernighan and Dennis Ritchie
- C Programming: A Modern Approach by K. N. King
Online Courses and Platforms
Platforms like Coursera and Udemy offer in-depth courses that cater to all levels. These resources can guide hands-on practice and are great for visual learners.
Community Forums and Groups
Joining communities like reddit.com/r/C_Programming or finding groups on Facebook can provide support and shared knowledge.
Diving into C opens numerous possibilities, offering an exhilarating experience for students and aspiring developers. With rich history and application, learning C may well be one of the best decisions you make in your programming journey.
Prelims to Language
The C programming language holds a pivotal place in the world of software development. Its design emphasizes efficiency and flexibility, making it a go-to language for systems programming, application development, and much more. As learners journey through this language, understanding its core elements offers a foundation for grasping more complex programming paradigms.
Historical Context
To appreciate C, one must understand its roots. Born in the early 1970s, during a time when computers were becoming central to both scientific and commercial pursuits, C emerged from the laborious assembly language. Its creator, Dennis Ritchie, at Bell Labs, sought to create a language that facilitated system programming while being accessible and robust. Prior languages such as B and BCPL influenced C's syntax and structure, but C introduced a new level of abstraction that resonated beyond its contemporaries, allowing developers to write clearer and more maintainable code.
This languageās relevance was cemented with the development of Unix, the operating system that adopted C as its primary programming language. The simplicity and versatility of C allowed Unix to thrive, and as Unix spread, so did C's influence in programming. It's an interesting twist of fate that the systems programing language now serves as a stepping stone for many modern languages such as C++, Java, and Python.
Purpose and Applications
C is not just a relic of the past; its contemporary applications are vast and varied. With its low-level capabilities, C is often used for systems programming, embedded systems, and real-time applications. Here are some key areas where C excels:
- Operating Systems: C forms the backbone of many operating systems, providing control over hardware due to its efficiency and capacity for direct memory access.
- Embedded Systems: From medical devices to automotive controls, C's ability to run with minimal resources is essential for these systems.
- Game Development: High-performance game engines often utilize C or C++ for their speed and efficiency, ensuring smooth gameplay.
The language's standard libraries, rich syntax, and structured approach to coding facilitate various application domains. Whether developing compilers, interpreters, or intricate user interfaces, C consistently proves useful.
Overall, the significance of learning the C language cannot be overstated. It serves not only as a foundational tool for budding programmers but also as a powerful language for experienced developers who seek direct control over hardware and superior performance.
Language Syntax
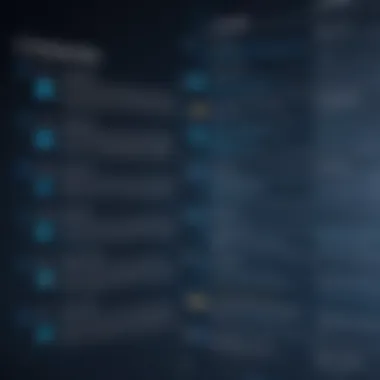
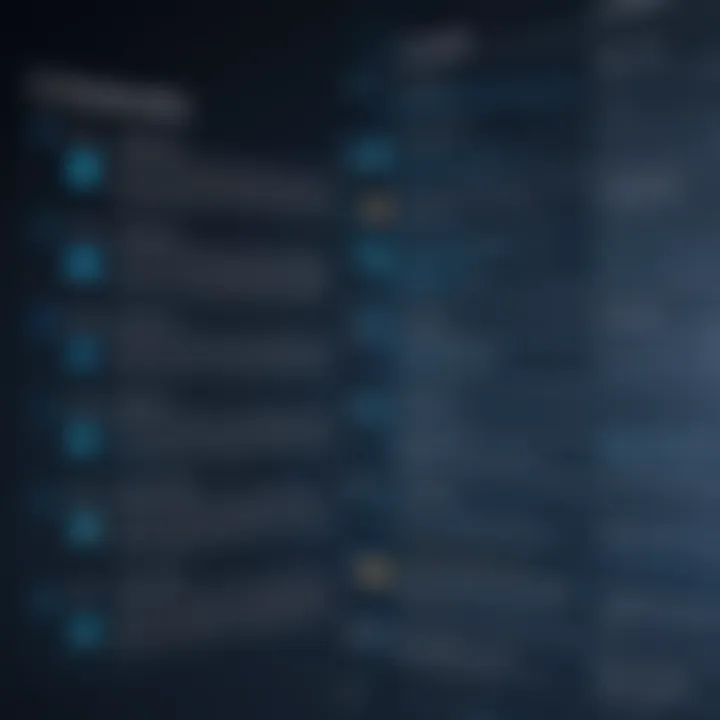
Understanding the syntax of the C language is vital for anyone looking to navigate the intricate maze of programming. Syntax provides the set of rules that dictate how code is written and structured. This framework is significant because even a small deviation, like a misplaced semicolon or an incorrectly closed bracket, can lead to errors that are often time-consuming to troubleshoot.
One of the beauties of C syntax is its relative simplicity compared to other programming languages. It uses a clear and concise approach that is easy to understand, especially for beginners. Pair this simplicity with the power it wields in system programming and application development, and you can see why mastering C syntax is critical. Here are some key elements that underscore its importance:
- Foundation of Structure: The structure of a C program follows a predictable format, which allows the programmer to comprehend how code segments relate to one another. This is vital for collaboration and debugging efforts.
- Readability and Maintainability: Following the syntax rules leads to code that is easier to read and maintain. This can significantly enhance productivity in larger projects or teams where multiple programmers may work on the same codebase.
- Error Identification: A strong grasp of syntax can help programmers identify errors more swiftly. Knowing what constitutes valid syntax allows programmers to spot anomalies early.
Basic Structure of a Program
Every C program shares a common structure, which typically follows this pattern:
- Preprocessor Directives: This is the very first thing you see in a C program. Directives tell the compiler to include certain libraries, such as . They often set up functions necessary for input and output.
- Main Function: This function is the starting point of any C program. Itās where execution begins. The syntax looks like this:Here, signifies that the function will return an integer value, typically indicating the program's status upon completion.
- Variable Declarations: Before using variables, you need to declare them. This involves specifying their data types, such as for integers or for floating-point numbers.
- Statements: Finally, you include the executable statements of your program. These effectuate the logic that you want to implement.
By following this structure, programmers can ensure that their code is functional and logically sound from the get-go.
Comments and Documentation
Comments in C are essential for creating readable and maintainable code. They provide insights into the thought processes behind code decisions or complex logic, making it easier for others (or your future self) to understand your work.
C offers two types of comments:
- Single-line Comments: These are prefixed with . Anything following this on the same line will be ignored by the compiler. This is handy for short notes.
- Multi-line Comments: Enclosed within , this format can span several lines, ideal for detailed explanations or larger blocks of code you want to annotate.
To illustrate, consider the following snippet:
In terms of documentation, adhering to comments can make teamwork smoother. Different programmers can view intentions or logic without having to decipher the code from scratch. This practice directly elevates the quality of development projects, as it encourages a culture of clear communication.
"Good comments are like a signpost in the wilderness of code; they show you where you are and where you're going."
Ultimately, thoughtful syntax and adequate documentation lay the groundwork for effective programming in C, enabling coders to build both functionality and teamwork.
Data Types in
Understanding data types in C is vital. They determine how the program interprets and processes data, which can greatly influence performance and memory usage. Choosing the right data type ensures efficient storage and optimal manipulation of variables, allowing programmers to build robust applications. The importance of getting it right cannot be overstated, as improper use of data types can lead to unexpected behavior or, worse, program crashes.
Primitive Data Types
C has several primitive data types, which are basic building blocks for data manipulation. These include:
- int: Typically used for integers. Example:
- float: Suitable for floating-point numbers, it provides a way to handle decimals. Example:
- char: Represents single characters. Example:
- double: This type offers a higher precision than float, ideal for calculations where accuracy matters. Example:
The choice between these types hinges on the amount of memory they consume and the numerical range they cover. For instance, using for small integers saves memory compared to using , which occupies more space, but is necessary for accurate decimal calculations.
Derived Data Types
Derived data types extend the functionality of primitive types. They allow for more complex structures to be created. Common derived types in C include:
- Arrays: Collections of data elements, all of the same type. Example: can hold five integers.
- Structures (struct): Custom data types that group related variables of different types. Example:
- Unions: These are similar to structures but share the same memory location, allowing saving space when variables occupy different ranges.
- Pointers: They hold memory addresses, enabling direct memory access and manipulation. Example: .
Derived data types afford programmers a great deal of flexibility and power in managing data and building applications that can handle complex data relations.
Enumeration and Typedef
Enumerations, declared using the keyword, provide a way to define a variable that can hold a set of predefined constants. For example:
This makes the code clearer and easier to maintain, as using names instead of numbers helps avoid confusion.
Typedef allows programmers to create synonyms for existing types, enhancing readability. For instance:
Now can be used throughout the code, making it clear that this variable is of type without verbose declarations.
By using enumeration and typedef, better code clarity and governance can be achieved, which is indispensable in large-scale projects with many developers involved.
"Data types are the foundation upon which C programming builds its functionality and efficiency."
In summary, the proper use and understanding of data types in C is not only beneficial but also essential for developing efficient and error-free programs.
Control Structures
Control structures form the backbone of programming logic in C. They allow developers to dictate the flow of execution in programs based on specific conditions or repetitive tasks. Understanding these structures is crucial as they enable a program to make decisions, perform iterations, and effectively manage complex logic without muddling through a sea of code.
Conditional Statements
At the heart of C's control structures lie conditional statements. These statements allow a program to execute certain blocks of code based on whether predefined conditions evaluate to true or false. Essential to decision-making, conditional statements help developers construct programs that can respond to various inputs dynamically.
The most common types of conditional statements in C are , , and . For example, consider a scenario where we want to check if a number entered by the user is positive, negative, or zero. The code for this could look something like this:
In this snippet, the statement checks the condition of the number against zero. If the condition is met, the corresponding block of code runs. Using conditional statements efficiently can make the difference between a rigid program and a dynamic one that can handle different scenarios.
Looping Mechanisms
Looping mechanisms are another crucial aspect of control structures in C programming. They enable the execution of a block of code repeatedly until a specified condition evaluates to false. This is particularly helpful in scenarios where you need repetitive tasks, such as iterating through arrays.
C offers several types of loops: , , and . Each serves different needs but ultimately achieves the same goalārepetition.
For Loop: This is usually used when the number of iterations is known. Here's a simple usage example:
This loop runs five times, printing the iteration number each time.
While Loop: This loop continues until the condition specified is false.
Here, the loop continues to execute as long as remains less than 5.
Overall, understanding and leveraging these looping mechanisms can dramatically enhance the efficiency of a program, allowing for less code and improved runtime performance.
Switch Case Statements
Switch case statements are another effective control structure that simplifies decision-making by allowing a single variable (usually an integer) to be evaluated against multiple constants.
This can be particularly useful when you have several potential values to compare againstāsomething that can easily clutter your code when using statements. The syntax is straightforward:
The above code allows for clear and concise handling of user input, directing flow based on the chosen option, which can simplify both reading and maintaining the code.
In summary, control structures, including conditional statements, looping mechanisms, and switch case statements, equip C programmers with the tools to create complex, efficient, and effective programs. Understanding how to implement and leverage these constructs is essential for anyone looking to master C programming.
Functions in
Functions are a cornerstone of C programming, and understanding them is crucial for anyone looking to master the language. Functions allow for organized code, enabling us to break complicated problems into manageable parts. With functions, we can encapsulate functionality, enhance code reusability, and increase readability. This section will cover key aspects, benefits, and considerations about functions in C.
Defining Functions
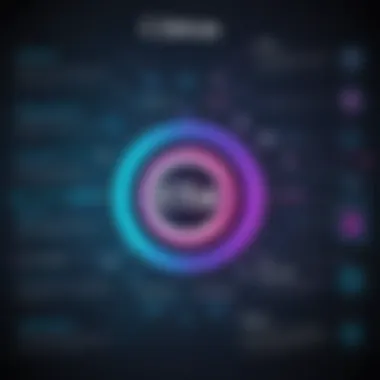
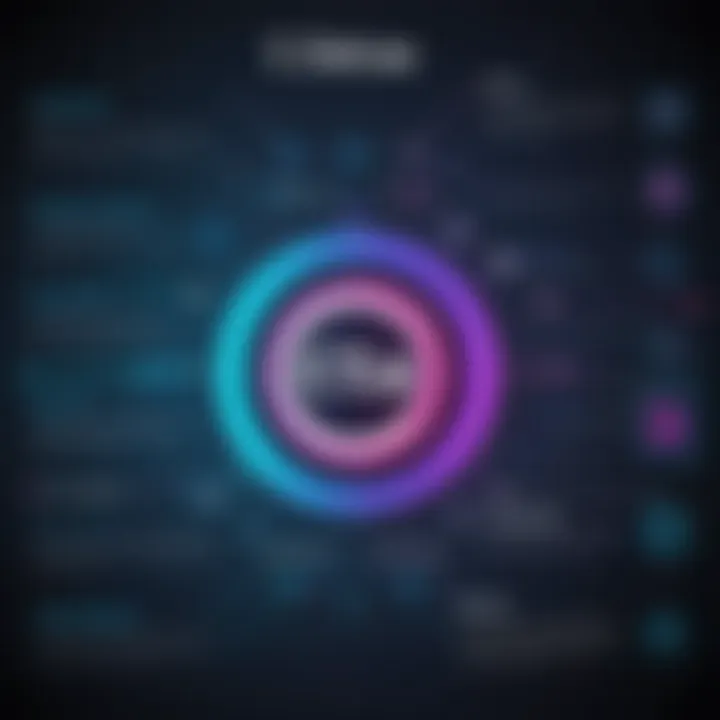
A function is a self-contained block of code designed to perform a specific task. The definition of a function in C includes a few essential components: the return type, function name, parameters (if any), and the function body itself. Hereās a simple structure:
For example, let's consider a function that adds two integers:
In the above snippet, is the return type, is the name, and and are parameters. When this function is called, it computes the sum of and and returns the result. Defining clear function names and maintaining a consistent structure facilitate easier understanding and maintenance.
Function Parameters and Return Types
Understanding parameters and return types is paramount in C functions.
- Parameters: These are variables that function can take to perform operations. They act as placeholders for the values (or arguments) passed to the function when it is called. Itās important to note that parameters can be of various types, including fundamental types like or , as well as user-defined ones.
- Return Types: This indicates the type of value the function will return after execution. A function can return multiple types (or none), which is defined at the time of its declaration. If a function doesnāt return a value, it uses the keyword.
For example:
This shows a function that takes two floats and returns their product as a float.
Scope and Lifetime
Scope and lifetime are two critical concepts pertaining to variables in C functions.
- Scope refers to the visibility of variables within a program. C has several types of scope:
- Lifetime refers to the duration the variable exists in memory. Local variables are created when a function is called and destroyed once it exits, whereas global variables persist for the entire runtime of the program.
- Local Scope: Variables defined within a function are local to that function and cannot be accessed outside.
- Global Scope: Variables defined outside any function can be accessed throughout the entire code.
Understanding these concepts aids in managing resource allocation and avoiding potential errors.
With effective use of functions, programmers can greatly enhance the organization and efficiency of their code, resulting in cleaner and more maintainable software.
Pointers in
Pointers are a pivotal concept in the C programming language, acting as a gateway to understanding more complex programming paradigms. What sets pointers apart from other data types is their capability to store the memory address of variables, instead of holding actual values. This characteristic introduces flexibility and efficiency to your programs. For students and newcomers learning C, grasping pointers is essential as they are integral when it comes to dynamic memory allocation and the manipulation of data structures. So, letās dive deeper.
Understanding Pointers
At its core, a pointer is simply a variable that holds a memory address, usually the address of another variable. Think of it as a signpost on a road, pointing you to where a specific destination (or variable) can be found. To declare a pointer in C, you use the asterisk (*) symbol, making it clear that it is meant to hold an address rather than a value. For example:
In this declaration, is a pointer to an integer. The process of dereferencingāaccessing the value stored at the address pointed to by the pointerāis done with the same asterisk. This increases the flexibility of your code. Moreover, understanding pointers enhances your ability to optimize memory usage and performance in your applications. You can dynamically manage memory, avoiding the pitfalls of static allocation, which often leads to wasted resources.
"Pointers are like a double-edged sword. Master them, and you wield great power; neglect them, and you risk programming chaos." - Unknown
Pointer Arithmetic
Pointer arithmetic opens up a new level of manipulation with pointers. By performing arithmetic operations on pointers, you can traverse arrays and data structures with ease. For example, if you have an array of integers, the pointer can be incremented or decremented to point to various elements in the array:
In this snippet, we declare an array and assign the pointer to the beginning of the array. By incrementing , we effectively move to the next integer in the array. This demonstrates how pointers can be not just references to values, but also navigable paths through memory spaces. Each increment points to the next element based on its size, meaning you can traverse multi-dimensional arrays and structures with relative ease.
Memory Management
Memory management in C programming is a pivotal aspect that dictates how programs handle memory allocations, optimizations, and resource management both during compile-time and runtime. Understanding this topic is essential for both burgeoning programmers and seasoned developers alike, as it directly influences a programās performance, stability, and robustness.
Dynamic Memory Allocation
Dynamic memory allocation allows programmers to request memory allocation at runtime. This flexibility is crucial, particularly in scenarios where the total amount of memory needed cannot be predetermined during compile time. Think of it like living in a house with adjustable rooms; you expand or shrink your space based on your current needs.
In C, dynamic memory allocation is typically managed through functions provided in the standard library, such as , , , and .
- : Allocates a block of memory of the specified size. It returns a pointer to the beginning of the block. If the memory couldn't be allocated, it returns .
- : Allocates memory for an array of elements, initializing all bits to zero, which can be helpful in avoiding garbage values.
- : Changes the size of previously allocated memory and can help in adapting the memory footprint as needed.
- : Deallocates memory that was previously allocated, releasing it back to the system. Not freeing up memory can lead to leaks, akin to leaving the water running.
When utilizing dynamic memory, it's important to keep a keen eye on how you manage it, as failing to deallocate can lead to memory leaks that spiral into performance issues.
Common Memory Management Functions
Memory management functions are the backbone of dynamic memory handling in C, each designed for specific tasks and should be used judiciously. Hereās a closer look into these functions:
- : As mentioned, it allocates raw memory, but with no initialization. Each time you call , itās critical to check whether the return value is to ensure memory allocation was successful.
- : Perfect for initializing arrays. If you need an array of ints and want zeroed-out memory, does just that.
- : This function is particularly useful when the size of the memory block needs to be adjusted. It can also move the memory block to a new location if necessary, though it's crucial to capture the return of this function as it may change the original pointer.
- : Always remember to free up memory once you're done with it to avoid those pesky memory leaks. Itās like cleaning up your workspace after a project.
By understanding and utilizing these dynamic memory allocation functions, a programmer can write more efficient and reliable C programs.
Standard Libraries
In the realm of C programming, the concept of Standard Libraries holds a prominent place. These libraries are essentially pre-written collections of code that allow programmers to perform common tasks without having to write everything from scratch. This not only speeds up the development process but also ensures consistency and reliability in code. By leveraging these libraries, developers can tackle complex functions with relative ease, which is critical in an era where efficiency is key.
One of the significant benefits of using Standard Libraries is the abstraction they provide. Programmers can utilize a range of functionalities that are often taken for granted in modern development. From input and output handling to memory management and string manipulation, these libraries encapsulate intricate functionalities within simple function calls. Understanding their structure and capabilities becomes essential for anyone looking to write effective C code.
The Standard Input/Output Library
When discussing the Standard Libraries in C, the Standard Input/Output Library stands out as one of the most important components. Commonly referred to as , this library is crucial for managing data input and output operations. Developers can access a slew of functions that handle everything from reading keyboard input to writing output to the console.
For instance, functions like and are cornerstones in C programming. Using , developers can format and display data, while provides a way to read formatted input from the user. This functionality is not just limited to console applications; it extends to file handling as well. Through pointers and functions such as , , , and , the library makes file manipulation straightforward.
Hereās a simple code snippet illustrating the use of and :
The importance of cannot be overstated; it not only plays a pivotal role in user interaction but also in data management for small and medium-sized applications. Itās like the Swiss army knife for conducting standard I/O tasks, making it indispensable.
String Handling Functions
Another critical aspect of Standard Libraries is the string handling functionalities provided by . In C, strings are not a distinct data type but rather arrays of characters terminated by a null character (). The library offers a plethora of functions designed to create, manipulate, and analyze these string arrays.
Some of the key functions include:
- : Computes the length of a string.
- : Copies one string to another, ensuring safe transfer of text.
- : Appends one string to the end of another.
- : Compares two strings lexicographically to determine their order.
By incorporating these functions, developers avoid manual operations on strings, which are not only cumbersome but also error-prone. For example, using instead of a manual copy loop results in cleaner code, reducing the risk of buffer overflows, a common pitfall in C.
Here is a brief example that showcases how to use with :
File Handling
File handling is a vital component in C programming that enables developers to interact with data stored outside the program itself. Whether itās to read user data, save settings or log activities, effective file handling ensures that your applications can manage data persistently. In this section, we will examine the fundamental aspects of file management, specifically focusing on how to open, close, read from, and write to files in C.
Opening and Closing Files
Before any file can be utilized in a program, it must first be opened. This process establishes a connection between the program and the file on the disk, allowing operations to be performed. In C, the function is used to open a file, specifying the path and the mode (read, write, append, etc.) in which the file is to be accessed.
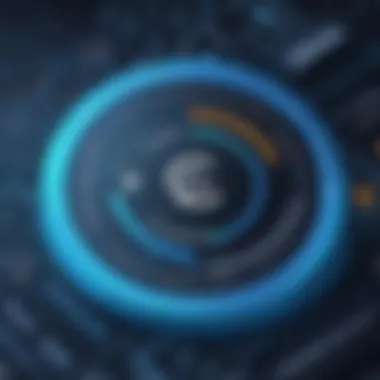
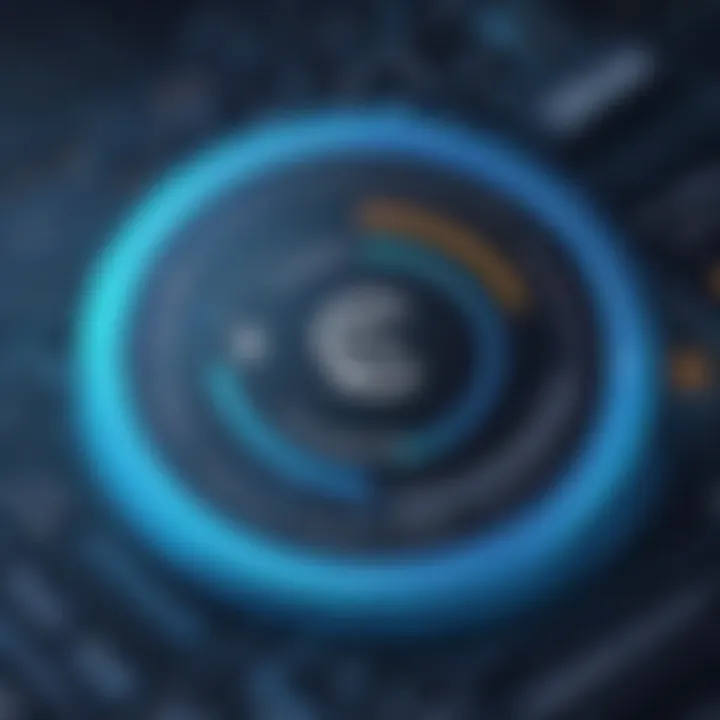
Here's a basic example:
In the example above, we attempt to open a file named "example.txt" in read mode. If the file cannot be opened, itās good practice to handle the error gracefully.
Once youāre done with the file, closing it properly is crucial. This is done using the function. Failing to close a file might lead to data loss or memory leaks. For instance:
Reading and Writing Files
After opening a file successfully, reading from or writing to it is the next step. C provides several functions for this task, giving you flexibility and control over how data is handled. Let's break down these operations into reading and writing.
Reading Data
To read data, you can use functions like for strings or to read formatted input. For example:
This approach reads a line from the file into the , allowing you to process the data as needed.
Writing Data
Writing data to a file involves functions like or . Hereās how you can write to a file:
In this example, "output.txt" is opened for writing, and a line is written into it. Remember that if the file does not exist, it will be created. However, if it does exist, this operation will overwrite the existing content.
Key Takeaway: Mastering file handling in C is essential to create interactive applications that need to manage input and output, making them more functional and user-friendly.
Considerations: When engaging in file operations, consider the modes you need, handle possible errors, and always ensure files are closed to maintain the integrity of data. Being meticulous with these aspects can save a lot of headaches later on.
With these tools and techniques under your belt, you can wield file handling to enhance the capabilities of your C programs, making them more dynamic and responsive to user needs.
Common Errors and Debugging
In the realm of C programming, understanding common errors and debugging techniques is akin to having a map in uncharted territory. Many novice programmers often stumble upon bugs and errors without fully grasping their origins. This section enlightens readers on the significance of recognizing and resolving coding issues that may arise during development. By honing the skill to identify these problems early, programmers can not only refine their code but also cultivate a more profound understanding of the intricacies of the C language.
Identifying Compilation Errors
Compilation errors are the initial hurdle a programmer encounters when writing C code. These arise during the compilation process, often due to syntax mistakes, unrecognized identifiers, or misplaced constructs. A keen eye for detail is essential here because even a minor error, like a missing semicolon, can halt the entire compilation.
Here are some common compilation errors:
- Missing semicolons at the end of statements
- Mismatched brackets or parentheses
- Undefined variables or functions
- Incorrectly typed data or inconsistent data types
The compiler typically provides feedback in the form of error messages, which may initially seem cryptic. Reading these messages carefully allows programmers to trace back to the source of the issue. For instance, an error message might indicate that a variable is undeclared. Itās important to check if the variable has been defined and if its scope encompasses the use in that part of the code.
Additionally, many modern Integrated Development Environments (IDEs) feature real-time syntax checking, which can highlight errors even before the compilation phase. Leveraging these tools can significantly reduce frustration, enabling a smoother coding experience.
Runtime Errors and Debugging Techniques
Once the code has successfully compiled, the next frontier emergesāruntime errors. These errors occur while the program is executing, often leading to unexpected behavior or crashes. Unlike compilation errors, runtime errors can be more challenging to track down because they might not surface until certain conditions are met during program execution.
Common types of runtime errors include:
- Segmentation faults: Accessing memory that isnāt allocated for the program.
- Infinite loops: Circling through a loop that never meets its exit condition.
- Division by zero: Attempting to divide a number by zero, which is mathematically undefined.
To debug runtime errors, here are several effective techniques:
- Print debugging: Inserting print statements at various points in the code to track variable values and flow of execution.
- Using a debugger: Tools like GDB (GNU Debugger) allow you to inspect the program's state at different execution points, which can reveal where things go awry.
- Code reviews: Having a fresh pair of eyes go through the code can help spot mistakes that the initial programmer might overlook.
"Debugging is like being the detective in a crime movie where you're also the murderer." Understanding this dynamic can foster patience and perseverance in tackling coding challenges that arise during C development.
Best Practices in Programming
When venturing into the vast world of C programming, establishing a solid foundation through best practices is crucial. Following these guidelines not only leads to cleaner code but also enhances maintainability, readability, and overall meaning of your programming efforts. Itās a bit like keeping a tidy desk; when everything is organized, finding the tools you need becomes much easier, and you can work without unnecessary distractions.
Effective Code Organization
Code organization is more than just aesthetic; it holds the key to efficient programming. Without a properly structured layout, even the simplest program can turn into a jumbled mess, making it difficult to troubleshoot and maintain. Here are some of the best practices for organizing your C code:
- Use Meaningful Names: Variable, function, and file names should describe their purpose, which helps others (and your future self) understand your intent. Instead of naming a variable , consider or similar.
- Consistent Indentation: Stick to a single style of indentation, whether it's tabs, spaces, or a mix, but be consistent throughout your code. This helps create visual hierarchy, making the code more readable.
- Modular Programming: Break down your programs into smaller, manageable functions or modules. Each function should do one thing well. This practice not only minimize complexity, but it also promotes reusability of code.
- Comment Generously: While it might seem tedious, providing comments elucidating the functionality of your code can save time in the long-run. Itās beneficial for anyone who may work with your code later.
"The best way to predict the future is to invent it."
ā Alan Kay
Applying these principles will assist in navigating your projects with clarity and thoughtfulness. Remember, organized code can save hours of confusion.
Code Documentation Standards
Alongside effective organization, code documentation is an essential aspect of programming that often gets swept under the rug. Well-documented code acts as a guide, making it easier for anyone to step in and understand whatās going on, or troubleshoot issues that arise. Hereās what you should keep in mind:
- Maintain Clear Comments: Comments should explain why a piece of code exists, what it does, and how it fits into the bigger picture. Avoid stating the obvious, as it can lead to clutter.
- Use Documentation Tools: Tools such as Doxygen help generate documentation automatically from comments in code. This can greatly reduce the burden of keeping documentation synchronized with code changes.
- Regular Updates: As you modify and improve your code, ensure that the documentation reflects these changes. Outdated docs can create confusion and lead to errors.
- Structured Format: If youāre using comments, adhere to a standard formatting structure within the codebase. This could be adhering to specific conventions for function descriptions, parameter explanations, or return type annotations.
In a nutshell, both effective code organization and meticulous documentation play pivotal roles in best practices for C programming. By embedding these habits into your coding routine, youāre setting yourself up for success as a well-rounded programmer.
Language in Modern Development
The C programming language has remained relevant over the decades due to its efficiency and robust nature. In todayās tech-savvy world, understanding C is invaluable. This section will explore why C holds such an important position in modern software development, compare it with prevalent programming languages, and discuss current trends in its usage.
Language vs Other Programming Languages
When we stack C against popular languages like Python, Java, or JavaScript, several distinct characteristics emerge. C is often hailed for its performance that is pretty darn hard to beat. Programs written in C can be compiled to machine code which means they run extremely fast, crucial when executing time-sensitive operations.
- Control Over System Resources: Unlike higher-level languages that abstract away hardware details, C gives developers the reins to manage memory allocation and other low-level functionalities. This specific trait makes C the go-to language for system programming, embedded systems, and operating system development.
- Portability: C's principles are anchored in standardized coding practices. Software written in C can easily be moved from one platform to another with minimal changes. This portability is a treasure in a world where multi-platform support is often a requirement.
- Foundation for Other Languages: Many modern programming languages, like C++, Java, and Python, have C at their roots. Learning C equips you with a solid foundation upon which other languages can be understood. Itās like learning the building blocks of a kitchen before becoming a master chef in gourmet cuisine.
Of course, higher-level languages have their perks too ā they often allow for rapid development and easier debugging. But the trade-offs for sheer speed and control can sway a developerās choice back to C when the project demands it.
Trends in Language Usage
Current trends indicate that C's popularity is not just lingering but evolving. Despite the rise of higher-level languages, C plays a critical role in numerous tech sectors:
- Embedded Systems: It's everywhere, from automotive software to industrial machine automation. C's efficiency makes it perfect for running on hardware with limited resources.
- Game Development: Many renowned game engines, such as Unreal Engine, utilize C++āa language derived from C. Thus, a firm grasp of C helps in understanding game performance optimizations, graphics rendering, and low-level memory operations.
- Cybersecurity: With the growing emphasis on cybersecurity, C is utilized in building security tools and analyzing vulnerabilities. Understanding C enables program analysis at a low level where many exploits and weaknesses can be identified.
- Open Source Projects: An influx of open-source projects continues to thrive in C, attracting developers looking to contribute to large collaborative projects. This participation cultivates a rich community built around learning and sharing.
In summary, while other programming languages offer their own unique benefits, Cās intelligence lies in its blend of speed, efficiency, and adaptability in various domains. Understanding the nuanced landscape of C in modern development is essential for aspiring programmers.
"C is quirky, flawed, and an enormous success." ā Dennis Ritchie
By embracing C, developers not only enhance their programming arsenal but also embrace a legacy of computing that continues to shape our technological world.
The End
In wrapping up our exploration of the C programming language, itās pivotal to underscore why this conclusion section matters. As weāve navigated through the various facets of Cāhistorical backgrounds, syntax, data types, and best practicesāthe importance lies not just in understanding these elements individually but in recognizing how they collectively contribute to the language's robust foundation.
The ability to grasp the core principles and concepts allows programmers, especially students embarking on their coding journey, to build important skills that frame their understanding of programming as a whole. Here are the principal benefits:
- C as a Building Block: C forms the basis for numerous modern languages, so knowing it provides insight into languages like C++, Java, and even Python.
- Efficiency and Performance: Understanding C mechanics and its memory management can greatly enhance a programmer's ability to write efficient, high-performance applications.
- Problem-Solving Skills: Engaging with C instills critical thinking and algorithmic problem-solving capabilities, which are transferable to other programming tasks.
"Programming is like poetry; it requires precision, creativity, and a firm grasp of structure."
This conclusion does not merely sum up information but rather serves as a stepping stone into the larger world of coding. While the journey with C may be concluding here, it opens doors to countless possibilities in computing.
Summary of Key Takeaways
As programmers forge ahead, several key takeaways from this guide can help to anchor their understanding of C:
- Historical Perspective: Familiarize yourself with the evolution of C, as understanding its past can enrich your approach to using it today.
- Syntax Fundamentals: Mastering Cās syntax is crucial; itās the skeleton upon which all programming builds.
- Data Types and Control Structures: Knowing the different data types and their control structures provides the necessary toolkit for effective programming.
- Pointer Mastery: Gaining a comprehensive understanding of pointers is invaluable, as they are central to many C functionalities.
- Dynamic Memory Management: Learning how to manage memory dynamically empowers programmers to create efficient and resource-aware applications.
- Debugging and Error Handling: Familiarity with common errors and debugging strategies can save considerable time in development.
Future Learning Paths
The pathway forward from mastering C opens various avenues for further learning:
- Advanced C Programming: Delve into deeper topics, such as advanced data structures, algorithms, and systems programming.
- C++ Development: Transitioning from C to C++ can broaden one's programming abilities with more complex concepts such as object-oriented programming.
- Embedded Systems: C is extensively used in embedded systems, making it beneficial to explore electronics and firmware development.
- Software Development Life Cycle: Incorporating concepts of SDLC to understand how C fits into larger project frameworks enhances overall expertise.
- Contributions to Open-source Projects: Engaging with open-source communities can provide real-world coding experience and build collaborative skills.
- Comparative Language Studies: Exploring languages like Rust, Go, or Python will deepen oneās understanding and appreciation of how C interfaces with other languages.
The journey of programming doesnāt end here; learning is a continuous process. Equip yourself with the knowledge of C as a stepping stone towards newer horizons in the ever-evolving field of technology. Whether you're tackling algorithmic challenges, designing software architectures, or venturing into the realms of data science, the fundamentals you've absorbed will serve as a solid foundation for future endeavors.