Exploring the Swing Framework in Java: A Deep Dive
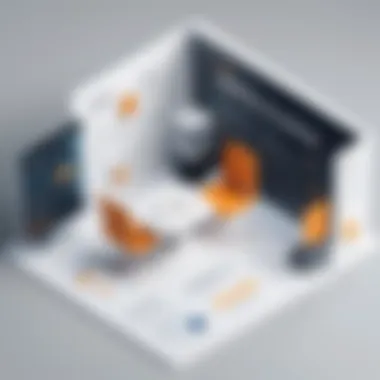
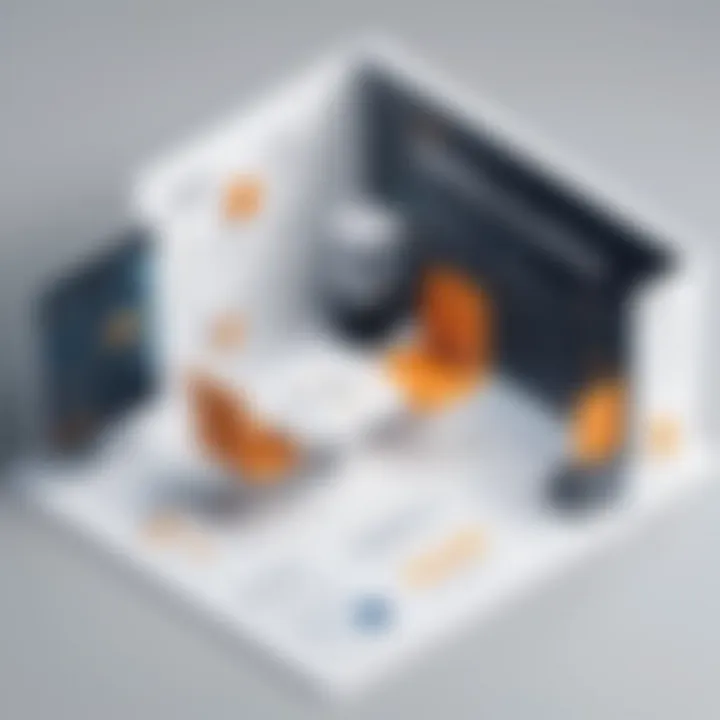
Intro
Swing in Java stands out as a robust framework for creating attractive graphical user interfaces (GUIs). It offers tremendous flexibility and a rich set of components, making it a favored choice among developers aiming to deliver engaging desktop applications. Swing isnāt just another framework; itās a toolkit that empowers programmers to craft high-quality interfaces with an intuitive feel.
To understand Swingās relevance, one must first appreciate the evolution of GUI programming in Java. Beginning with AWT (Abstract Window Toolkit), Java's original GUI toolkit, Swing emerged as a refined alternative. AWT relied heavily on the underlying native operating system, which resulted in significant limitations. Swing, however, is built entirely in Java, allowing it to be platform-independent. This not only boosts its appeal but ensures that applications behave consistently across different environments.
Swing offers a modern feel with a pluggable look and feel, meaning developers can change the appearance of their applications with minimal effort. This adaptability fosters creativity, enabling developers to build visually striking interfaces that can still perform efficiently. With its rich set of components, Swing caters to various needsāfrom simple buttons to advanced tables and trees, every visual component is covered.
As we navigate through this article, we will cover essential topics, focusing on Swingās architecture, components, and practical implementations. The aim is to equip both beginners and seasoned developers with the necessary tools to leverage Swing effectively in their Java applications.
"Swing offers a flexible framework for creating rich client applications, making it a vital part of the Java ecosystem."
The journey through Swing will encompass its history, core components, advanced capabilities, and practical examples to solidify your understanding. Let's dive deep into the specifics of what Swing has to offer.
Prelude to Swing
Swing is an essential component in the Java ecosystem, widely used for building rich graphical user interfaces (GUIs). Its significance lies not just in the ability to create visually appealing applications but also in its underlying architecture that promotes flexibility and ease of use. Understanding Swing is crucial for any Java developer aiming to create desktop applications that are both functional and user-friendly.
Overview of GUI Programming
Graphical User Interface (GUI) programming has transformed the way users interact with software. Unlike command-line interfaces that demand memorization of commands, GUIs offer an intuitive point-and-click way for users to navigate applications. This evolution has pushed developers to seek frameworks that streamline the GUI creation process. Swing, as one of these frameworks, excels in several areas:
- Component Richness: Swing comes packed with an assortment of pre-built widgets such as buttons, sliders, and text fields. These components save time and reduce the boilerplate code that developers have to write.
- Platform Independence: One of the hallmarks of Swing is its ability to run on any platform that supports Java. This means a Swing application can look and behave the same, whether on Windows, macOS, or Linux, thanks to the underlying Java Virtual Machine.
- Customizability: Swing allows for a high degree of customization. Developers can not only change the appearance of the components, but can also create entirely new components tailored to specific needs.
In this day and age, where user experience reigns supreme, having a solid grasp of GUI programming through frameworks like Swing is invaluable.
History and Evolution of Swing
Swing's journey began in the mid-1990s as a response to the limitations of the Abstract Window Toolkit (AWT). While AWT provided the foundational building blocks for GUIs in Java, it relied on native platform resources, leading to inconsistencies across different operating systems. This was problematic, especially when developers needed to ensure similar behavior and design across varied environments.
Swing emerged as a lightweight alternative to AWT, built entirely in Java. Its design was heavily influenced by the Model-View-Controller (MVC) architecture, promoting better separation of concerns. Hereās a brief timeline highlighting key milestones in Swingās evolution:
- 1996: Introduction of Swing as part of Java Foundation Classes, aimed at enhancing AWT.
- 1998: Swing became the standard for building user-friendly GUIs with the release of JDK 1.2, significantly improving the capabilities of Java in desktop applications.
- 2004: With Java 5, various enhancements were introduced, including support for pluggable look and feel, making it even more versatile.
- 2011: The release of Java 7 included critical updates and performance improvements to Swing, reflecting the ongoing commitment to refine the framework.
The evolution of Swing signifies the broader narrative of Java's adaptability in keeping pace with user interface demands. As the landscape of software development continues to shift, Swing remains relevant and serves as a backbone for many Java applications, highlighting its enduring importance in GUI programming.
Architecture of Swing
Understanding the architecture of Swing is akin to peeking under the hood of a finely tuned engine. It's not just about knowing how things fit togetherāit's about grasping how these parts work synergistically to create a smooth, responsive graphical user interface (GUI). At its core, Swing's architecture is built upon the Model-View-Controller (MVC) paradigm, which stands out for its ability to cleanly separate the logic of an application from its user interface. This level of separation is essential as it enhances maintainability and scalability, particularly in larger applications.
Model-View-Controller Paradigm
The MVC paradigm is pivotal in Swingās architecture. In this framework, the Model represents the data and business logic, the View handles the graphics and user interface, while the Controller is responsible for the communication between the Model and the View. This separation allows developers to modify one part of the application without adversely affecting others.
For example, if there's a need to enhance the GUI without messing with the underlying data processing logic, MVC allows for that ease of modification. One could even swap out the View entirely for a new design while keeping the Model intact. Such flexibility is invaluable in long-term projects where requirements can evolve.
Swing Components and Containers
Swing comprises a variety of components and containers that help developers build complex UIs efficiently. At the top level, developers often create a JFrame, which serves as the primary window for the application. The JFrame is, if you will, the proverbial stage where all the action takes place.
JFrame
The JFrame acts as the main application window in a Swing application. One of its standout features is that it's customizable and can hold other components like buttons, labels, and panels. This flexibility to integrate various components makes JFrame a popular choice among developers.
Unique to JFrame is its ability to manage events efficiently. When a user interacts with the application, the JFrame captures these events and directs them appropriately within the application. This feature is especially beneficial in programming environments where responsiveness is critical.
JPanel
JPanel serves as a flexible container for organizing components inside a JFrame. It's like a blank canvas, allowing developers to paint their GUI design. The key characteristic of JPanel is its lightweight natureāitās less resource-intensive than other containers while providing the same organizational benefits.
Another unique feature of JPanel is its capacity to be easily customized through layout managers. This makes it easier to arrange components in a visually appealing manner. However, unlike JFrame, it cannot stand alone. You need to add it to a container like JFrame to function effectively.
JComponent
JComponent is the foundational class for all Swing components. Whether you're working with buttons or complex custom components, JComponent provides a solid base. The significant advantage of JComponent is its rich set of features such as painting capabilities, event handling, and tooltipsāall of which enhance user interaction.
One unique feature of JComponent is its double-buffering technique, which helps eliminate flicker during updatesāa common issue in GUIs. Although JComponent is versatile and powerful, it may not be the best choice as a standalone entity, as it lacks windowing capabilities like JFrame or JPanel.
Event Handling Mechanism
Event handling is a crucial aspect of creating dynamic and interactive user interfaces. Swing employs a model where developers need to interface components with event listeners and adapters to cater for user interactions smoothly.
Listeners and Adapters
Listeners are methods in your code that provide feedback based on user actions. For instance, clicking a button can trigger an ActionListener. These listeners are not only a vital part of making the application interactive but are also kept separate from the GUI code, adhering to the MVC principles. The use of adapters, in turn, allows you to implement only the methods you need, alleviating the need to implement an entire interface. This selective implementation enhances code clarity and efficiency.
Delegation Event Model
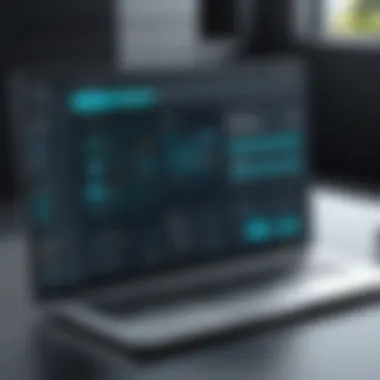
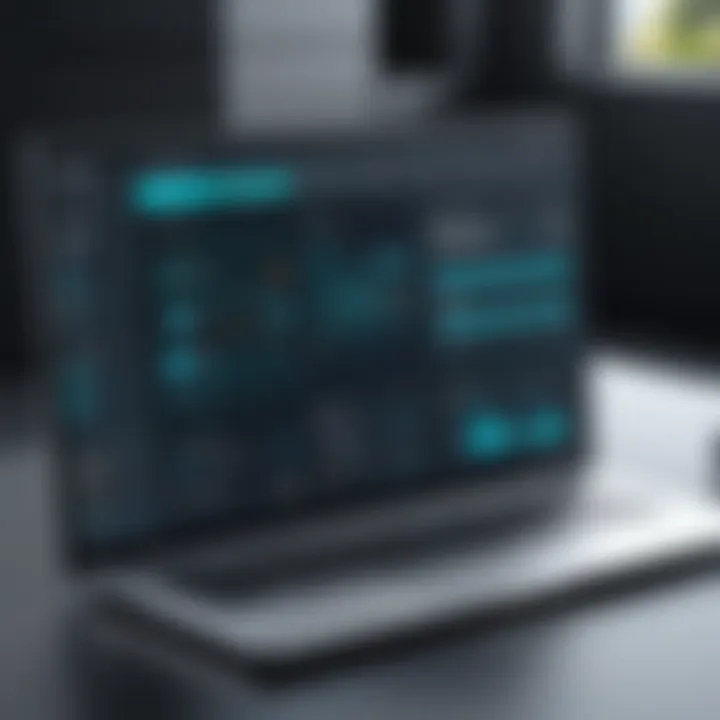
The delegation event model is another cornerstone in Swingās event handling. Unlike traditional models that use callbacks, this approach delegates the event-handling tasks to specialized event listeners. This separation of concerns allows each component to manage its events efficiently while reducing the complexity of managing numerous event handlers.
By using this model, you can create a responsive application that can effectively manage multiple events without overwhelming the system.
Using MVC with Swing offers developers a clear path to effectively building robust applications while also maintaining a high level of user interface responsiveness.
Key Components of Swing
Understanding the key components of Swing is essential for anyone interested in mastering Javaās graphical user interface capabilities. Swing provides a rich set of controls and features that maximize user experience while maintaining flexibility in design. When diving into GUI programming with Swing, it's essential to familiarize yourself with both basic and advanced components, as they form the backbone of any application you create. Each component plays a significant role in building user interfaces that are not just functional, but also engaging and accessible.
Basic Swing Controls
Buttons
Buttons are one of the cornerstones of interactive applications. They allow users to perform actions with a simple click, making them a vital part of usability. A key characteristic of buttons in Swing is their ability to handle events like clicks and hovers, which is essential for creating responsive applications.
The unique feature of buttons is their customizable look and feel, thanks to the pluggable look and feel architecture in Swing. This enables developers to modify button appearances without changing the underlying logic. A potential downside is that if not designed carefully, buttons can become visually overwhelming, particularly if numerous are used in a single interface.
Labels
Labels serve a more subtle yet equally important role in Swing applications. They provide context and descriptions for other components without requiring direct user interaction. This helps in guiding users through applications, thereby enhancing understanding and navigation.
A standout aspect of labels is their simplicity; they can display text, images, or even both, depending on what needs to be conveyed. Labels are often a beneficial choice as they require minimal resources to render. However, if labels become cluttered or overly complex, they may detract from the applicationās usability, potentially confusing users instead of assisting them.
Text Fields
Text fields are the gateways for user input within a graphical interface. They allow users to enter dataāa core function in any interactive program. The key characteristic that makes text fields popular is their flexibility, allowing for single-line or multi-line entries, depending on the design requirements.
One unique feature of text fields is the ability to include placeholder text; this is a helpful prompt for users to understand what kind of input is expected. However, a downside is that if the field's purpose is unclear, users might struggle to provide the correct input, leaving room for frustration.
Advanced Swing Components
Tables
Tables in Swing offer a robust way to display data in a structured formatāideal for applications that require displaying and manipulating large sets of information. Their key characteristic is the ability to present data rows and columns efficiently, supporting complex data operations like sorting and filtering.
Tables become a beneficial choice when data visualization is paramount. Unique features, such as cell rendering and model-based data management, provide developers fine control over the appearance and behavior of their data sets. One disadvantage lies in their complexity; improper implementation may lead to performance issues, especially with very large data sets.
Trees
Tree structures in Swing represent hierarchical data effectively. Users can visualize relationships between items, making trees a fantastic way to organize related data. The key characteristic of trees is their ability to expand or collapse sub-items, allowing users to navigate large amounts of information intuitively.
An advantage of using trees is their clear representation of parent-child relationships in data, which can be vital for applications that require a detailed exploration of data sets. However, managing the complexities of tree structures, especially with heavy nesting, can pose challenges and lead to a steep learning curve for developers.
Menus
Menus in Swing are pivotal for giving users navigation options without overwhelming them with choices on the main interface. The key characteristic that sets menus apart is their ability to group commands and functionalities, neatly organizing them into categories.
Menus provide a professional touch and make applications user-friendly. One unique feature is the context menu that opens with right-clicks, offering additional options without taking space on the screen. On the downside, a poorly designed menu can be frustrating if users find it hard to locate the functions they need, leading to inefficiency and lowering the overall user experience.
Creating a Simple Swing Application
Creating a simple Swing application is an essential step for anyone looking to master GUI programming in Java. This process lays the groundwork for understanding how graphical user interfaces (GUIs) function and interact with the user. In this section, we will explore the benefits of developing a straightforward application, as it provides clarity on the Swing frameworkās capabilities while allowing programmers to familiarize themselves with its core components.
By constructing a basic application, developers can gain insights into the layout management, event handling, and the design patterns unique to Swing. Moreover, it acts as a catalyst for creativity, encouraging new ideas for projects of increasing complexity.
Setting Up Your Development Environment
Before diving into code, setting up your development environment is crucial. It ensures you have the proper tools at your disposal for building Swing applications. Typically, a Java Development Kit (JDK) needs to be installed on your machine. Follow these steps to create a conducive environment:
- Install JDK: Make sure you download the latest version if you haven't already. You can find it at Oracle's official site.
- Choose an IDE: Integrated Development Environments (IDEs) like IntelliJ IDEA, NetBeans, or Eclipse are popular among developers. They provide a lot of functionality to ease the process of writing code.
- Create a New Project: Open your chosen IDE, and create a new Java project. This step typically involves specifying your project name and location.
- Add Libraries: Although Swing is included in the JDK, check that you have the necessary libraries added to your project settings.
Once your environment is ready, you can start building applications swiftly and efficiently.
Writing Your First Swing Program
Writing your first Swing program is an exciting journey into the world of GUI applications. In this part, we will cover essential aspects like basic UI creation and adding controls and listeners to enhance interactivity.
Basic UI Creation
Creating a basic user interface is the first step in your Swing journey. This fundamental aspect helps lay a solid foundation for UI design and user experience. By utilizing the JFrame and JPanel classes, developers can set the stage for their applications. A JFrame serves as the main window of the application, while JPanel can act as a container where various components reside.
A key characteristic of basic UI creation is simplicity. By focusing on the essential elements first, developers can build upon this foundation gradually. This method not only streamlines the learning curve but also enables one to understand how different components interact within the UI.
Here is a unique feature of basic UI creation:
- Modularity: You can create different panels for specific tasks and switch between them, making it easy to manage complex interfaces.
Although the advantages of starting simple are apparent, the potential downside is that it can be a bit limiting if not utilized creatively. Nonetheless, it's a vital stepping stone on your way to creating more intricate Swing applications.
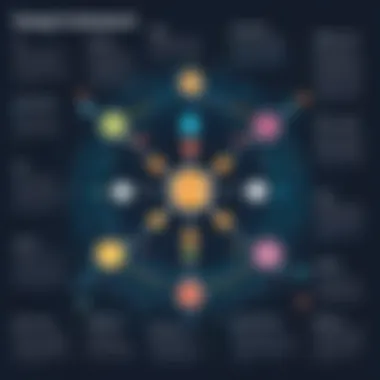
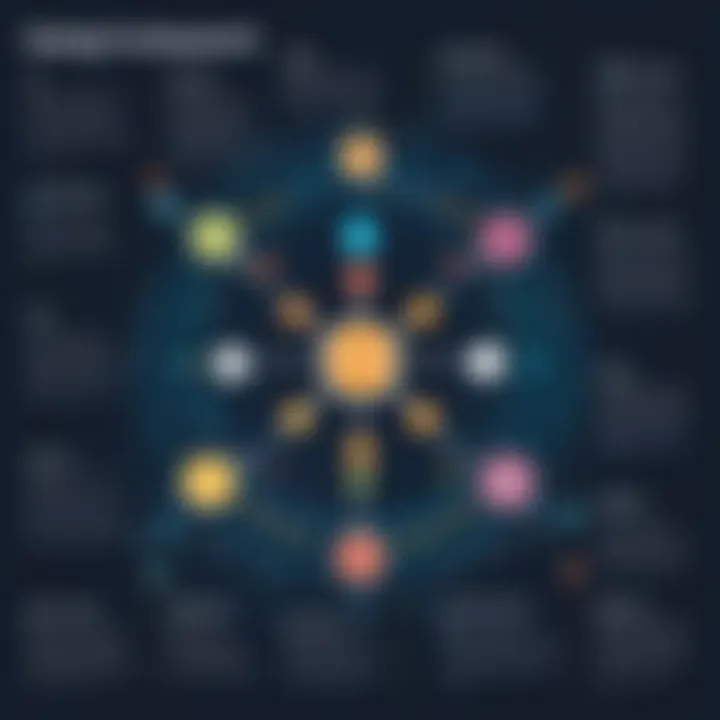
Adding Controls and Listeners
Once the basic UI is in place, the next logical step is to add controls and listeners. This aspect is necessary for making your application interactive. Swing provides various controls like buttons, text fields, and combo boxes that allow users to perform actions and input data.
A key characteristic of adding controls is enhancing user interaction. For instance, adding a JButton allows users to trigger specific actions, while JTextField enables data input. This interactivity transforms a static interface into a dynamic experience.
The unique feature of adding controls and listeners lies in the event-driven programming paradigm it introduces. When a user interacts with a component, events are fired, and listeners respond to these events accordingly. This behavior leads to a responsive and engaging application.
Tip: When implementing listeners, itās common to use anonymous inner classes or lambda expressions for conciseness and clarity.
However, the drawback can be the initial complexity, especially for beginners not familiar with event handling. Nonetheless, mastering these concepts is fundamental for creating advanced applications.
By focusing on these elements, you can effectively start building simple yet functional Swing applications. This hands-on experience will strengthen your understanding and prepare you for the complexities that lie ahead in your Swing programming journey.
Swing vs. AWT and JavaFX
When it comes to creating graphical user interfaces (GUIs) in Java, understanding the distinctions between Swing, AWT, and JavaFX is crucial. Each has its unique strengths and weaknesses, shaping how developers approach their projects. This section unpacks these GUI frameworks, examining their performance, customizability, and ease of use. By delving into these aspects, developers can make informed choices that align with their project requirements.
Comparative Analysis
Performance
The performance of a GUI framework dictates how responsive and fluid an application feels to end users. In general, Swing tends to showcase better performance compared to AWT, which relies on the system's native GUI components. Swing, being lightweight and written entirely in Java, allows for more consistent rendering across platforms. This means developers can deliver applications that look and feel the same, regardless of the operating system. Every time a UI is rendered using AWT, it pulls native components from the host OS, which can lead to inconsistencies and performance hiccups.
One unique aspect of Swing is its double-buffering mechanism, which minimizes flickering during updatesāa vital feature for applications demanding smooth graphics. However, when it comes to resource-heavy applications, JavaFX offers a performance edge through hardware acceleration, particularly in environments demanding rich graphics.
Customizability
Customizability is another battleground where these frameworks differ. Swing shines bright in this arena; it allows for extensive customization of components. Developers can change every aspect of a componentās appearance, from colors to shapes, without being tethered to the native look and feel. This flexibility is particularly beneficial for applications requiring a distinct brand identity or a non-standard user interface design.
On the flip side, AWT is limited by the look and feel of the host operating system. If a developer aims for a unique design, AWT can become a cumbersome bottleneck. However, while Swing provides vast customization options, it requires a deeper understanding of the framework to implement effectively. JavaFX also offers customization, notably through CSS styling, which presents a user-friendly way to manipulate component aesthetics without diving into a multitude of Java classes. This makes it appealing for those who wish to keep their code neat while achieving a high degree of visual flair.
Ease of Use
The ease of use of a framework can significantly influence a developer's efficiency. Swing, with its flexible model, has a steeper learning curve. New developers might find themselves grappling with the intricacies of its component hierarchy and event handling model. Yet, once mastered, Swing can empower developers to create intricate and responsive UIs.
AWT, being the older framework, is simpler for basic applications but fails to provide many advanced features, which can limit developers as their projects grow. JavaFX, however, strikes an appealing balance. It blends a comprehensive set of features with a more intuitive design approach. Developers new to GUI programming may find the declarative approach in JavaFX, alongside its implementation of FXML and the ability to mix Java code with XML layout, both refreshing and productive.
When to Use Swing
Choosing the right framework often depends on the projectās specific needs. Swing should be your go-to choice if you require a highly customizable application's appearance and can allocate time for learning its complexity. It suits applications needing consistent cross-platform performance with a heavy emphasis on user interface customization.
On the other hand, if you aspire to create highly interactive applications that utilize rich media and graphics, leaning on JavaFX might be worth considering. Nonetheless, for students and budding developers, Swing remains an excellent educational tool for grasping the fundamentals of GUI programming in Java.
Best Practices for Swing Development
When diving into Swing development, implementing best practices is essential to create efficient, maintainable, and user-friendly applications. These practices not only make the development process smoother but also enhance the quality of the final product. Adhering to best practices ensures that your application performs well, offers a good user experience, and remains easy to update and extend over time.
Design Patterns in Swing
Singleton
The Singleton pattern is instrumental in managing a single instance of a class throughout the application. In Swing, this pattern is often used for managing resources that should only have one instance, such as configuration settings or application-wide resources.
A key characteristic of the Singleton is its ability to restrict instantiation. This is particularly beneficial when multiple parts of the application need to access the same resource without creating unnecessary copies. The unique feature of the Singleton pattern is its controlled access, ensuring synchronized access to its instance, making it safe for use in a multi-threaded contextācrucial for Swing applications where multiple threads might interact with the UI.
Advantages:
- Saves memory since only one instance exists.
- Simplifies development by providing a single point of access to resources.
Disadvantages:
- Can introduce global state, which might complicate testing.
- If not managed properly, could lead to tight coupling between components, making future changes challenging.
Observer
The Observer pattern is another cornerstone in Swing development. This model allows an object, known as the subject, to automatically notify its observers of any changes in state, thus facilitating a responsive GUI. This pattern is beneficial in scenarios where multiple components need to react to changes in a single data model, such as user inputs or application updates.
A defining characteristic of the Observer pattern is its decoupling of objects, meaning that the subject doesn't need to know specifics about its observers, just that they implement a certain interface. This allows flexibility and the ability to add or remove observers without modifying the subject, promoting cleaner code.
Advantages:
- Promotes loose coupling between components, enhancing flexibility.
- Facilitates real-time updates to UI elements in response to data changes.
Disadvantages:
- Managing dependencies can become complex as the number of observers increases.
- Without careful management, it may lead to memory leaks if observers are not properly removed when no longer needed.
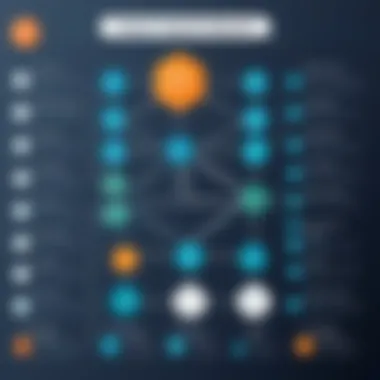
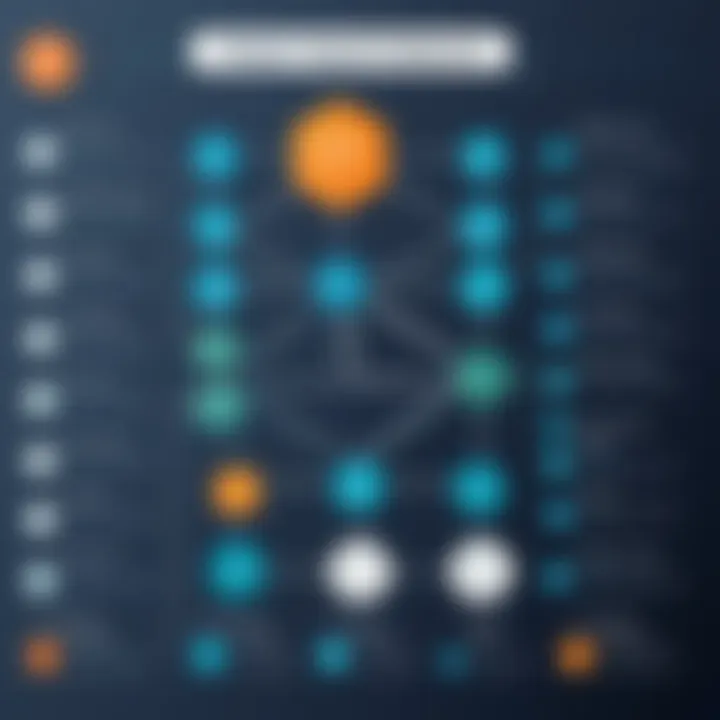
Performance Optimization Techniques
Optimizing performance is crucial in Swing applications. A slow UI can frustrate users and lead them to abandon your application. Here are some practical techniques for improving the performance of your Swing application:
- Minimize Redrawing: Reducing the amount of repainting can significantly enhance responsiveness. Batch updates to the UI instead of refreshing it for every minor change.
- Use Double Buffering: This technique helps to eliminate flickering by drawing the content off-screen before displaying it on the screen.
- Profile and Monitor Performance: Utilize profiling tools to identify bottlenecks in your application and address them efficiently.
Remember to test performance under real-world conditions. What seems efficient during development can turn sluggish under user load.
By embracing these best practices and design patterns, developers can create robust, maintainable, and user-friendly applications using Swing. With careful attention to detail, your Swing applications can stand the test of time.
Common Challenges in Swing Development
When it comes to building user interfaces in Java, Swing offers a robust framework. However, working with it isnāt a walk in the park. Understanding the challenges faced during Swing development is crucial for both novice and seasoned programmers. This section delves into some common issues and provides insights into solutions, ensuring that you can navigate the landscape of Swing more proficiently.
Threading Issues and SwingWorker
One of the most persistent challenges when developing with Swing is dealing with threading issues. Swing is not thread-safe, meaning updates to the GUI from non-Event Dispatch Thread (EDT) threads can lead to unpredictable behavior. Take, for instance, running a long data-fetch operation directly within a button listener. This could potentially freeze the UI, leaving users staring at a non-responsive application.
To tackle this problem efficiently, developers can utilize the class. This class allows you to perform background operations on a separate thread, while safely updating the Swing components on the EDT.
Hereās a basic example:
In this code:
- The method handles the lengthy task.
- The method facilitates the safe updating of the UI components with feedback from the background worker.
UI Responsiveness
Another notable challenge is UI responsiveness. Users today expect applications to be sleek and snappy. If operations take too long, users might get impatient and even abandon the application.
A responsive UI isn't just about aesthetics; it significantly impacts user experience. Intuitive design involves feedback for user actionsālike displaying a loading spinner or changing a button's text to āProcessingā¦ā while data is being handled.
To maintain responsiveness, consider implementing the following strategies:
- Avoid executing heavy computations on the EDT. Use background threads instead, as described with .
- Provide feedback for long-running operations, so users know the application is working.
- Test your application under conditions of maximum load to understand how it performs under stress. This helps in fine-tuning performance issues.
"A sluggish interface can be worse than no interface at all. Never underestimate the value of user's patience."
Understanding these challenges is crucial for creating effective, functional Swing applications that meet user expectations. Addressing threading concerns and ensuring responsiveness not only enhances the user experience but also solidifies your reputation as a skilled Java developer.
Future of Swing
As technology continues to evolve, the future of Swing remains a hot topic among developers. It's important to critically assess the role Swing plays today and how it might adapt to fit into modern software development. This discussion centers on the specific elements that contribute to Swing's relevance, its potential benefits in upcoming projects, and considerations that developers should keep in mind when working with this framework.
Swing in Modern Development
Swing, although established, still holds its ground in creating rich desktop applications. There are several reasons for this:
- Rich User Interfaces: Swing provides a plethora of components allowing developers to craft engaging user interfaces easily. This richness can be particularly advantageous when developing applications that require complex interactions.
- Cross-platform Capabilities: One thing that stands out about Swing is its capability to run seamlessly on multiple operating systems. An application built with Swing can function on Windows, macOS, and Linux, making it a desirable choice for reaching a broad audience without extensive modifications.
- Integration with Java Ecosystem: Swing benefits from being part of Java's extensive ecosystem. It can interact well with other Java libraries and tools, allowing developers to leverage established technologies to enhance functionality.
However, developers must also be aware of the pitfalls. For instance, the predominance of web-based applications today might overshadow traditional desktop applications. The need for a more flexible, browser-based or mobile approach may urge some developers to pivot towards frameworks tailored for those environments, like JavaFX or web technologies. Balancing these considerations is crucial as one decides whether to use Swing in their future projects.
"In todayās digital landscape, āuse the right tool for the jobā is more than just a saying; itās a guiding principle when it comes to selecting frameworks like Swing."
Integration with Other Frameworks
Swingās future could also flourish through strategic integration with other frameworks, providing enriched functionalities that cater to diverse development needs. Here are some insights about how Swing can interoperate:
- JavaFX: Integrating Swing with JavaFX allows developers to harness the strengths of both frameworks. For example, a Swing application can host JavaFX components, enabling applications to adopt modern UI elements while maintaining legacy components.
- Spring Framework: Utilizing Spring with Swing can bolster an applicationās backend. Applying the dependency injection and aspect-oriented programming principles of Spring can enhance a Swing applicationās architecture, making it scalable and maintainable.
- RESTful Services: By connecting Swing applications to RESTful APIs, developers can extend functionality without reinventing the wheel. For instance, developers can pull in dynamic data from web services, giving life to applications that were once static.
Closure
In the world of Java development, Swing remains a relevant and valuable tool for creating desktop applications. This article delineates the intricate elements of Swing, and it emphasizes why a thorough understanding is essential for development today. Firstly, knowing how to leverage Swing allows developers to create visually appealing interfaces that can enhance user experiences significantly.
The benefits of Swing extend beyond aesthetics. Its flexibility in terms of architectureāpermitting developers to encapsulate their designs using the Model-View-Controller patternāenables better code organization and maintainability. Moreover, the decoupling of components through this architecture fosters smoother collaboration among development teams, which is crucial for larger projects.
Additionally, as programming paradigms shift, understanding Swing's integration with modern frameworks can ensure a seamless transition and maintenance. Swing often interplays with other tools, allowing older applications to remain alive in newer ecosystems. This adaptability is a cornerstone for those who wish to keep their legacy applications relevant.
Recap of Key Points
To offer you a quick overview:
- Swing Architecture: Understanding the MVC pattern and how it structures applications is pivotal for managing complexity.
- Component Variety: Swing's rich library of components, from buttons to advanced tables, equips developers with the tools necessary for robust application building.
- Event Handling: The event-driven nature of Swing, facilitated through listeners and adapters, enhances application interactivity.
- Performance Considerations: Strategies to optimize performance, especially concerning UI responsiveness, are crucial for creating user-friendly interfaces.
- Future Relevance: The adaptability of Swing to modern frameworks ensures its continued significance in Java programming.
Final Thoughts on Swing Programming
The journey through Swing is not merely about coding; it's about crafting experiences that resonate with users while keeping in mind the underpinnings of good design and performance. Swing's capability of building dependable, effective, and visually appealing applications is something every aspiring programmer should embrace.
As technology progresses, Swing will continue to play a role, albeit one interwoven with modern tools. It's essential for developers to remain curious and adaptable. Tapping into resources, whether community forums like Reddit or educational sites like Wikipedia, can prove invaluable for continuous learning. Each new project becomes an opportunity to hone skills and explore novel solutions.
"The best software engineers are those who remain lifelong students of their craft."
Swing, with its depth and versatility, offers an avenue for students and new developers to grasp fundamental principles in a user-friendly environment. Itās indeed a gateway to mastering GUI development. Embracing the lessons and experiences from the Swing framework can pave the way for future endeavors in programming.