Unveiling the Depths of Swift Code Examples for Coding Enthusiasts
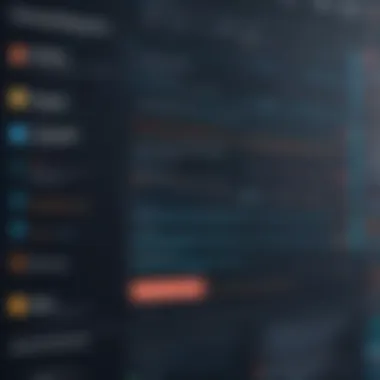
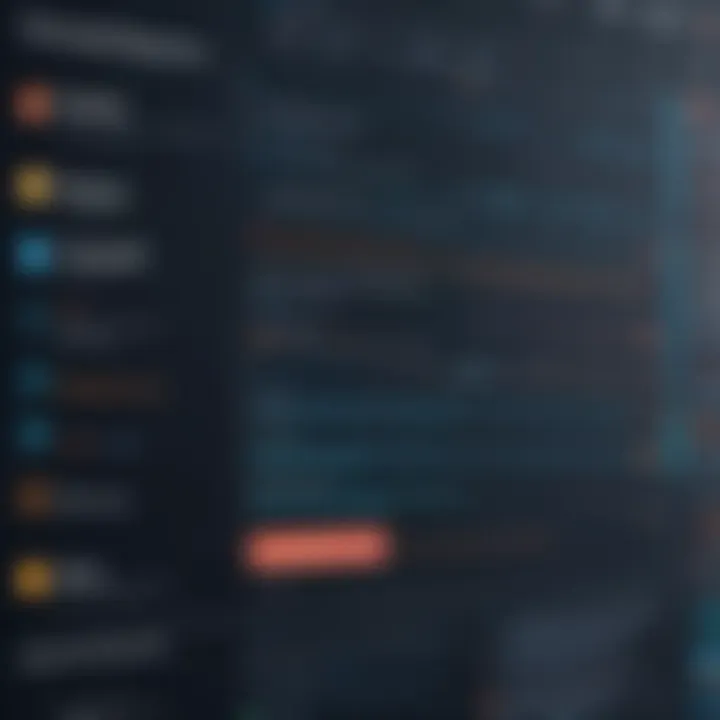
Introduction to Swift Programming Language
Swift is a modern, powerful programming language developed by Apple Inc. It was introduced in 2014 as a replacement for Objective-C and is designed to work seamlessly with Apple's frameworks. With a concise syntax and strong typing system, Swift is known for its safety features that help developers catch and fix errors early in the development process.
History and Background
The journey of Swift began in 2010 when Chris Lattner started working on a new language that would address the shortcomings of Objective-C. After years of development and refinement, Apple officially unveiled Swift to the world at WWDC 2014, marking a significant milestone in the realm of i OS and macOS development.
Features and Uses
One of the key features of Swift is its interoperability with Objective-C, allowing developers to leverage existing code and libraries within their Swift projects. Its speed and performance make it an ideal choice for building high-performance i OS, macOS, watchOS, and tvOS applications. Swift is also open-source, encouraging collaboration and innovation within the programming community.
Popularity and Scope
Since its release, Swift has witnessed a steady rise in popularity, becoming one of the fastest-growing programming languages. Its versatility extends beyond Apple's ecosystem, with the emergence of server-side Swift frameworks like Vapor and Kitura. This expansion has attracted a diverse range of developers, contributing to Swift's growing presence in the software development landscape.
Basic Syntax and Concepts
Understanding the fundamental aspects of Swift syntax is crucial for mastery in programming with this language. Let's delve into the basic building blocks that form the foundation of Swift development.
Variables and Data Types
In Swift, variables are declared using the 'var' keyword for mutable values and 'let' keyword for immutable values. The language supports various data types, including Integers, Doubles, Strings, Booleans, Arrays, and Dictionaries. Swift's type inference mechanism enables developers to declare variables without explicitly specifying their data types while ensuring type safety at compile time.
Operators and Expressions
Swift offers a wide range of operators for arithmetic, comparison, logical operations, and more. From basic mathematical operators like addition and subtraction to advanced operators like bitwise shifting, Swift provides developers with powerful tools to manipulate and evaluate values within their code. Expressions in Swift combine variables, literals, and operators to produce meaningful results.
Control Structures
Control structures such as 'if-else' statements, 'for' and 'while' loops, and 'switch' statements determine the flow of execution in a Swift program. These structures allow developers to make decisions, iterate over sequences, and handle different cases, enhancing the flexibility and logic of their code.
Advanced Topics
As you deepen your understanding of Swift programming, exploring advanced topics will equip you with the tools to build more complex and efficient applications. Let's embark on a journey into the intricacies of Swift's advanced concepts.
Functions and Methods
Functions in Swift enable encapsulation and reusability of code blocks, promoting modular programming practices. With support for parameters, return types, and function overloading, Swift functions offer a versatile mechanism for structuring code and promoting code reusability. Methods, on the other hand, are functions associated with a specific type or class, allowing for object-oriented programming paradigms.
Object-Oriented Programming
Swift embraces object-oriented programming principles, facilitating the creation of objects, classes, and inheritance hierarchies. By encapsulating data and behavior within objects, developers can model real-world entities and relationships in their applications. Polymorphism, inheritance, and encapsulation empower developers to write maintainable and extensible code in Swift.
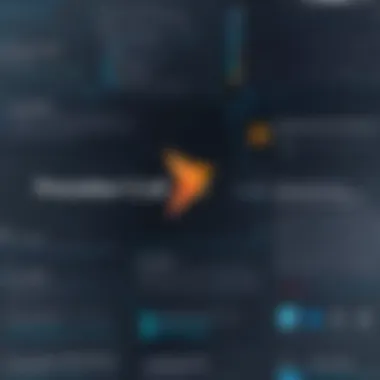
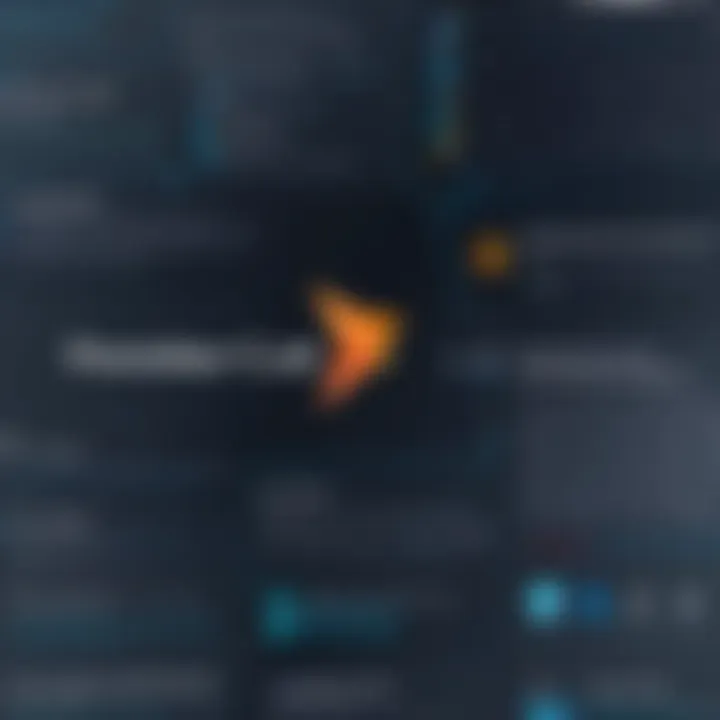
Exception Handling
Error handling in Swift is managed through the 'do-catch' mechanism, enabling developers to gracefully handle unexpected conditions and errors that may arise during program execution. By throwing and catching errors, Swift provides a robust framework for managing exceptional situations, ensuring the stability and reliability of applications.
Hands-On Examples
Practical application of Swift concepts through hands-on examples enriches your learning experience and reinforces your programming skills. Let's explore a curated selection of simple programs, intermediate projects, and code snippets to deepen your proficiency in Swift programming.
Simple Programs
From 'Hello, World!' to basic arithmetic calculations, simple programs in Swift introduce foundational concepts such as inputoutput, variable manipulation, and control flow. By implementing these programs, you gain hands-on experience in translating logic into executable code, honing your problem-solving abilities.
Intermediate Projects
Intermediate projects in Swift challenge you to integrate multiple concepts and techniques into cohesive applications. Building projects like a task manager, weather app, or basic game fosters creativity and problem-solving skills while exposing you to best practices in software design and development. These projects serve as milestones in your journey towards becoming a proficient Swift programmer.
Code Snippets
Code snippets offer concise and focused examples of Swift code snippets that illustrate specific functionalities or programming patterns. Whether it's sorting an array, implementing a protocol, or handling asynchronous tasks, examining code snippets provides insight into effective coding practices and fosters a deeper understanding of Swift's features.
Resources and Further Learning
As you embark on your Swift programming journey, leveraging a variety of resources and platforms will enhance your learning experience and expand your knowledge base. Let's explore a range of recommended books, tutorials, online courses, and community forums that provide valuable insights and opportunities for further learning in Swift development.
Recommended Books and Tutorials
Discover a curated selection of books and online tutorials that cover a wide range of Swift topics, from language fundamentals to advanced application development. These resources offer in-depth explanations, code samples, and practical exercises to support your learning and growth as a Swift programmer.
Online Courses and Platforms
Explore online courses and learning platforms that cater to various skill levels and learning preferences in Swift programming. From beginner-friendly courses on Udemy and Coursera to specialized platforms like Ray Wenderlich and Pluralsight, you'll find a wealth of educational opportunities to deepen your expertise in Swift development.
Community Forums and Groups
Engage with the vibrant Swift programming community through online forums, social media groups, and local meetups. Platforms like Stack Overflow, Reddit's rswift, and Swift Forums provide avenues for asking questions, sharing insights, and connecting with fellow developers. Joining a supportive community enhances your learning journey and fosters collaborations in the dynamic world of Swift development.
Introduction to Swift Programming Language
In this article, we delve deep into the fundamental aspects of the Swift programming language. Swift is a powerful and versatile language developed by Apple, known for its modern approach to programming. Understanding the basics of Swift is essential for anyone looking to create robust and efficient i OS applications. By mastering Swift, programmers can harness a wide range of features and functionalities to bring their ideas to life in the digital landscape.
Understanding the Basics of Swift
Variables and Data Types
When it comes to Variables and Data Types in Swift, precision and flexibility are paramount. Variables allow programmers to store and manipulate data efficiently, while Data Types define the kind of data that can be stored. Swift provides a rich set of built-in data types, including Integers, Strings, and Booleans, offering programmers the tools they need to manage information effectively. Understanding Variables and Data Types is crucial for structuring code logically and maximizing performance.
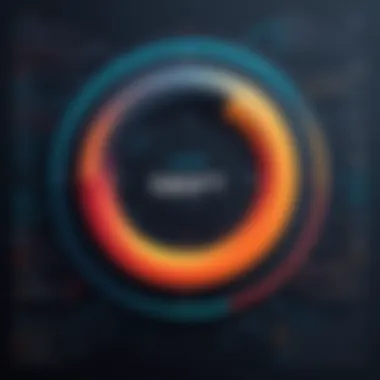
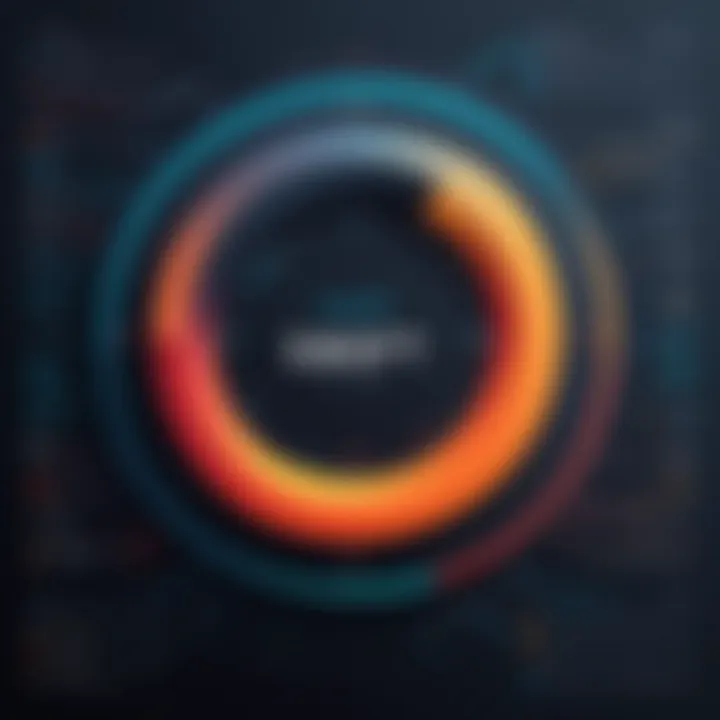
Constants and Operators
Constants and Operators play a significant role in Swift programming by enabling developers to create immutable values and perform mathematical operations with ease. Constants ensure that a value remains constant throughout the program, enhancing predictability and code clarity. Operators, such as +, -, *, and , empower programmers to manipulate variables and constants efficiently, streamlining the development process. Incorporating Constants and Operators in Swift code enhances readability and simplifies complex calculations.
Control Flow Statements
Control Flow Statements in Swift dictate the flow of execution within a program, allowing developers to make decisions based on specified conditions. By using Control Flow Statements like if, else, and switch, programmers can create logical structures that guide the behavior of their applications. These statements provide a dynamic way to control program flow, enabling the implementation of different paths based on various scenarios. Mastering Control Flow Statements enhances a developer's ability to create adaptive and responsive code structures.
Practical Swift Code Examples
In this section of the article, we delve deep into the significance of practical Swift code examples and how they provide invaluable insights for both novice and intermediate programmers. The essence of practical Swift examples lies in their ability to offer hands-on learning experiences, enabling programmers to grasp core concepts effectively. By exploring real-world scenarios through code snippets and applications, readers can bridge the gap between theoretical knowledge and practical implementation. Understanding the nuances of practical Swift examples is crucial for enhancing one's problem-solving skills and honing coding proficiency. Providing a solid foundation in Swift programming, these examples serve as building blocks for creating innovative solutions and optimizing code efficiency.
Working with Arrays and Dictionaries
Creating and Manipulating Arrays
When it comes to Creating and Manipulating Arrays in Swift, the focus is on manipulating collections of data efficiently. Arrays play a vital role in storing and organizing elements systematically, offering quick access and modification capabilities. By mastering the art of creating and manipulating arrays, programmers can streamline data management processes and enhance algorithmic efficiency. The key characteristic of Creating and Manipulating Arrays lies in its versatility, allowing for dynamic resizing and easy traversal of elements. This flexibility makes it a popular choice for handling datasets and iterating over vast amounts of information within Swift programs.
Implementing Key-Value Pairs in Dictionaries
Implementing Key-Value Pairs in Dictionaries adds a layer of complexity to data organization in Swift. Dictionaries provide a structured approach to storing data, relying on unique keys for efficient retrieval and manipulation. The key characteristic of using dictionaries is their key-value mapping, facilitating quick data access based on specific identifiers. While implementing key-value pairs offers a structured data storage solution, it may require additional memory allocation compared to arrays, impacting performance in certain scenarios. Understanding the advantages and disadvantages of dictionaries is essential for optimizing data handling processes within Swift applications.
Using Array and Dictionary Methods
Leveraging the built-in methods for Arrays and Dictionaries in Swift is essential for efficient data processing. These methods offer a range of functionalities, including sorting, filtering, and mapping elements, enhancing programmer productivity and code readability. By utilizing array and dictionary methods, programmers can streamline common data operations, such as searching for elements or modifying key-value pairs. The unique feature of using these methods lies in their abstraction of complex algorithms, providing a simple and intuitive interface for interacting with data structures. However, excessive reliance on built-in methods may lead to potential performance bottlenecks, requiring careful consideration of computational overhead in larger-scale applications.
Advanced Swift Concepts and Best Practice
In the realm of mastering the Swift programming language, delving into Advanced Swift Concepts and Best Practices stands as a pivotal step for enthusiasts seeking to elevate their skills to the next level. This section serves as a cornerstone in understanding the intricacies and optimizing the efficiency of Swift code. By honing in on sophisticated concepts and industry best practices, learners can enhance their code quality, performance, and overall development proficiency. Emphasizing the significance of Advanced Swift Concepts and Best Practices not only equips programmers with the knowledge to write robust and error-free code but also provides them with the tools to tackle complex programming challenges with agility and finesse.
Concurrency and Multithreading in Swift
Understanding Grand Central Dispatch
Embarking on the journey of Concurrency and Multithreading in Swift, one encounters the fundamental aspect of Understanding Grand Central Dispatch, a powerful mechanism that orchestrates parallel operations seamlessly. This pivotal feature plays a key role in optimizing resource utilization and enhancing the responsiveness of Swift applications. Its adept handling of task distribution and multithreading synchronization make it a popular choice for developers aiming to streamline operations and achieve optimal performance. Diving into Understanding Grand Central Dispatch uncovers its unique capability to manage concurrent tasks efficiently, revolutionizing the way programmers approach parallel computation for heightened productivity and responsiveness.
Asynchronous Operations with Dispatch
Queue #####
Transitioning to the realm of Asynchronous Operations with Dispatch Queue unveils a mechanism designed to facilitate non-blocking task execution, enabling developers to enhance application responsiveness and user experience. By leveraging DispatchQueue for asynchronous task management, programmers can decouple time-consuming operations from the main thread, preventing UI freezes and ensuring smooth application performance. The key characteristic of DispatchQueue lies in its ability to prioritize tasks, allocate system resources effectively, and execute operations concurrently, thereby maximizing the utilization of available computing resources. Harnessing the unique features of Asynchronous Operations with DispatchQueue empowers developers to create responsive and dynamic applications that resonate with modern user expectations, elevating the overall user experience.
Multithreading Techniques for Performance Optimization
Exploring Multithreading Techniques for Performance Optimization sheds light on strategies aimed at maximizing computational efficiency and resource utilization within Swift applications. By adopting multithreading methodologies, developers can parallelize tasks, distribute workloads across multiple threads, and exploit the full potential of modern multicore processors for accelerated application performance. The key characteristic of Multithreading Techniques for Performance Optimization lies in its ability to optimize task scheduling, minimize resource contention, and leverage the full processing power of hardware components. Unveiling the unique features of Multithreading Techniques for Performance Optimization equips programmers with the tools to design high-performing applications that excel in responsiveness and efficiency, setting a new standard for code optimization and execution speed.
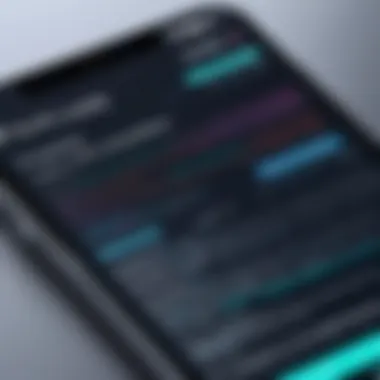
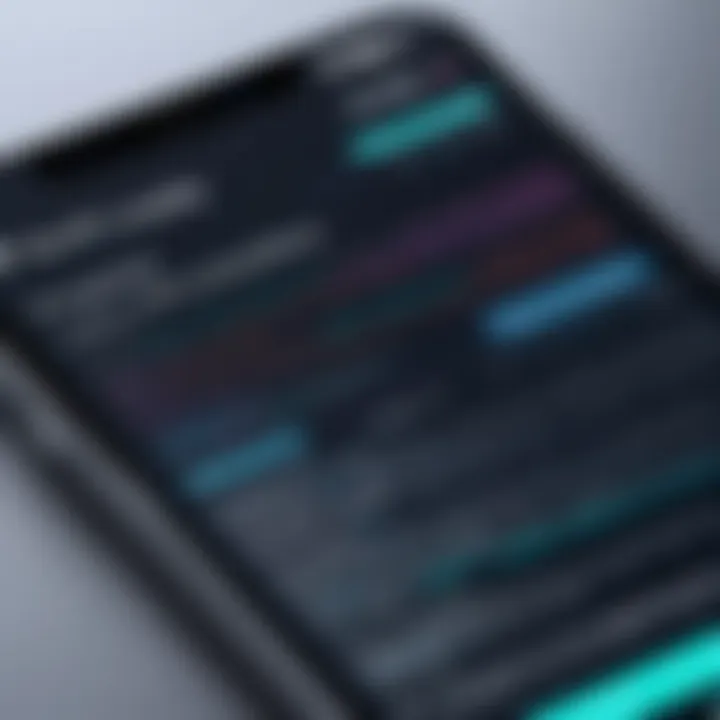
Exploration of Swift Libraries and Frameworks
In this section, we will delve into the crucial topic of Exploration of Swift Libraries and Frameworks. Understanding Swift libraries and frameworks is essential for any Swift developer looking to enhance their projects with ready-made solutions. By utilizing existing libraries and frameworks, developers can significantly expedite the development process and tap into the vast resources available within the Swift community. Exploring Swift libraries and frameworks provides programmers with access to pre-built functionalities, modules, and tools that can streamline development, improve code quality, and enhance overall productivity. Additionally, incorporating Swift libraries and frameworks in projects allows developers to leverage the expertise of other professionals in the field, leading to more robust and efficient solutions.
Utilizing Alamofire for Networking Operations
Making API Requests with Alamofire
When delving into the realm of networking operations in Swift, leveraging Alamofire for making API requests stands out as a prominent choice. Alamofire simplifies the process of sending network requests and handling responses, offering a clean and intuitive interface for developers. One of the key characteristics of making API requests with Alamofire is its asynchronous nature, allowing developers to perform network operations without blocking the main thread. This asynchronous behavior enhances the performance and responsiveness of the application, ensuring a seamless user experience. The unique feature of Alamofire lies in its built-in response serialization, making it effortless to convert network data into Swift objects with minimal code. However, developers should be mindful of the potential overhead introduced by Alamofire, especially in scenarios requiring lightweight networking solutions.
Handling Network Responses and Errors
Efficiently managing network responses and errors is paramount in networking operations, and Alamofire excels in this aspect. Handling network responses and errors with Alamofire involves robust error handling mechanisms, enabling developers to gracefully manage various scenarios, such as timeouts, server errors, or connectivity issues. The key characteristic of this process is the flexibility it provides in customizing error handling logic to suit specific project requirements. Alamofire's succinct error messages and comprehensive error codes simplify debugging and troubleshooting, enhancing the overall development workflow. Despite its numerous advantages, developers should consider the learning curve associated with mastering Alamofire's error handling mechanisms.
Integration of Alamofire in i
OS Applications
Integrating Alamofire into i OS applications facilitates seamless network operations and enhances the app's connectivity features. The key characteristic of incorporating Alamofire lies in its versatility and compatibility with different iOS versions, ensuring consistent networking performance across a wide range of devices. By integrating Alamofire, developers can centralize network requests, reducing code duplication and promoting code maintenance. The unique feature of Alamofire integration is its support for advanced network functionalities, such as request chaining, authentication handling, and response validation, offering developers a comprehensive toolkit for building robust networking components. However, developers should exercise caution when using Alamofire's advanced features to avoid unnecessary complexity and overhead in their iOS applications.
Conclusion: Mastering Swift Programming with Real-World Examples
This section serves as a pivotal point in the article as it encapsulates the essence of applying practical Swift knowledge in real-life scenarios. By mastering Swift programming through tangible examples, readers can solidify their understanding of the language's intricacies and functionalities. The real-world applications discussed here bridge the gap between theoretical knowledge and practical proficiency, preparing enthusiasts to tackle programming challenges with confidence and precision. Understanding how to implement Swift concepts in actual projects is crucial for honing programming skills and enhancing problem-solving capabilities. By delving into real-world examples, readers can explore the versatility of Swift and unlock its full potential, equipping them with the tools necessary to excel in diverse programming projects.
Applying Practical Swift Knowledge in Projects
Developing i
OS Apps with Swift: Developing i OS apps with Swift is a fundamental aspect that empowers programmers to create dynamic and interactive applications tailored for Apple devices. The key characteristic of utilizing Swift for iOS app development lies in its seamless integration with Apple's ecosystem, offering access to exclusive features and optimizing performance. The unique feature of Swift in developing iOS apps is its robustness and versatility, allowing programmers to craft engaging user experiences with ease. While Swift streamlines app development processes and enhances productivity, its main advantage lies in its ability to leverage native iOS functionalities efficiently. However, one may face challenges in terms of cross-platform compatibility and learning curve due to its specific syntax and conventions.
Enhancing Performance and User Experience:
Enhancing app performance and user experience is a critical factor in the success of any software project. By focusing on optimizing code efficiency and user interaction, programmers can ensure that their apps run smoothly and deliver a seamless experience to users. The key characteristic of enhancing performance and user experience lies in identifying and addressing bottlenecks that may affect app speed and responsiveness. The unique feature of this aspect is its impact on user retention and overall satisfaction, as fast and user-friendly apps tend to garner positive feedback and reviews. While improving performance and user experience enhances the app's market appeal and user engagement, it may require a balance between feature-rich interfaces and optimized functionality.
Contributing to Open-Source Swift Projects:
Contributing to open-source Swift projects offers programmers the opportunity to collaborate with peers, gain valuable experience, and contribute to the larger programming community. The key characteristic of engaging in open-source projects is the exposure to diverse coding styles, practices, and project scopes, allowing individuals to expand their skill set and knowledge base. The unique feature of participating in open-source Swift projects is the potential for creating impactful solutions that benefit a broader audience and foster innovation within the programming sphere. While contributing to open-source projects promotes transparency, collaboration, and collective learning, challenges may arise in terms of project coordination, code consistency, and community dynamics.
Continuous Learning and Skill Development
Exploring Advanced Topics in Swift:
Delving into advanced topics in Swift enables programmers to push the boundaries of their knowledge and expertise, unraveling complex concepts and unlocking new possibilities within the language. The key characteristic of exploring advanced topics is the depth of understanding it offers, allowing individuals to grasp intricate concepts such as generics, protocol-oriented programming, and advanced data structures. The unique feature of exploring advanced topics lies in its ability to cultivate problem-solving skills, critical thinking, and creativity in tackling programming challenges. While venturing into advanced territories can expand one's programming horizons and enhance coding proficiency, it may require a higher level of focus, dedication, and perseverance to master the nuances of advanced Swift concepts.
Participating in Coding Challenges and Competitions:
Engaging in coding challenges and competitions provides programmers with a platform to test their skills, benchmark their abilities against peers, and showcase their problem-solving acumen in a competitive environment. The key characteristic of participating in coding challenges is the opportunity to finesse programming techniques, explore diverse algorithmic solutions, and refine coding efficiency under time constraints. The unique feature of this aspect is its capacity to breed a culture of continuous improvement, resilience, and camaraderie among participants striving for excellence. While participating in coding challenges fosters a sense of achievement, community support, and skill validation, it may introduce pressure, time constraints, and focus challenges that require strategic planning and adaptive learning.
Networking with Swift Community for Growth:
Networking within the Swift community fosters connections, collaborations, and knowledge-sharing opportunities that propel individuals towards personal and professional growth. The key characteristic of networking within the Swift community is the synergy of diverse perspectives, experiences, and expertise that enrich the learning journey and broaden one's understanding of Swift development practices. The unique feature of networking with the Swift community lies in the support, mentorship, and career opportunities it offers, nurturing a supportive ecosystem for continuous learning and skill enhancement. While engaging with the Swift community cultivates a sense of belonging, mentorship, and exposure to industry trends, it may require active participation, communication skills, and relationship-building efforts to maximize networking benefits.