Unveiling Python Code Examples: From Novice to Intermediate Levels
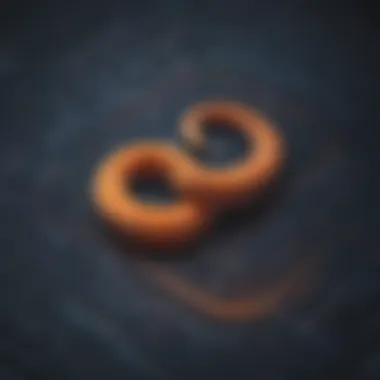
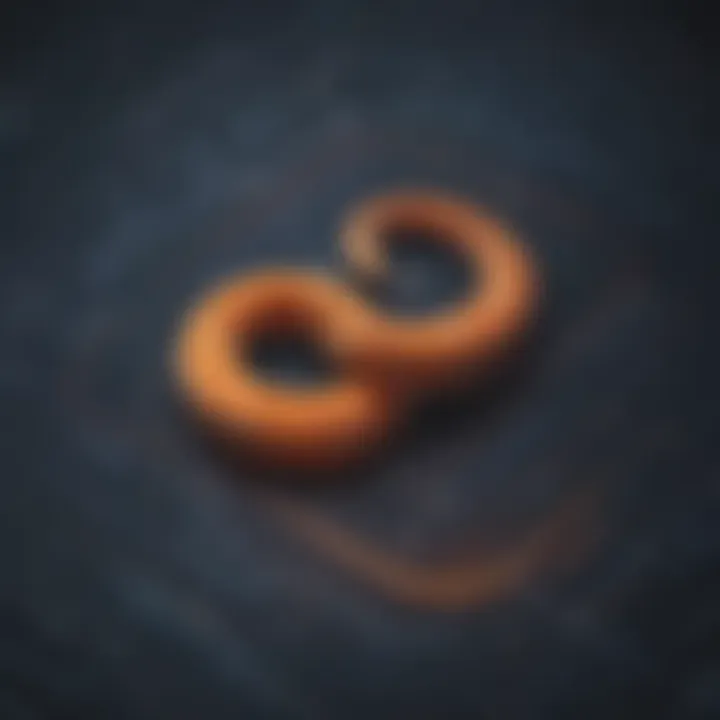
Introduction to Python Programming
Python is a high-level programming language that has gained widespread popularity among beginners and experienced programmers alike. Originally developed by Guido van Rossum in the late 1980s, Python was created with a focus on code readability and simplicity. Its versatility and ease of use make it an ideal choice for projects ranging from web development to data analysis.
History and Background
Python's development traces back to the Netherlands where van Rossum implemented this language to emphasize clear, logical code structure. Over the years, Python has undergone multiple updates and revisions, solidifying its position as a leading programming language.
Features and Uses
Known for its clean and concise syntax, Python boasts a rich library of modules and packages that support various applications. From scripting to web development, scientific computing to artificial intelligence, Python's flexibility makes it a go-to language for diverse projects.
Popularity and Scope
Python's rise in popularity can be attributed to its user-friendly nature and broad community support. With extensive documentation, online resources, and active user forums, Python continues to attract a vast audience of learners and developers seeking to harness its capabilities.
Introduction to Python Programming
Python programming serves as an essential gateway for individuals venturing into the world of coding. It lays a solid foundation for beginners and intermediate learners by offering a versatile and user-friendly platform for software development. Understanding Python basics unveils a plethora of opportunities to grasp fundamental concepts with ease and clarity. Highlighting the significance of Python variables, data types, syntax, and indentation rules becomes pivotal in mastering the art of programming. The structured format and readability of Python code make it a top choice for learners seeking to enhance their coding skills meticulously.
Understanding Python Basics
Python Variables and Data Types
Python's variables and data types play a significant role in defining the essence of programming logic. By empowering programmers with dynamic typing and a range of data structures, Python simplifies complex operations and enhances code efficiency. Understanding the dynamic nature of Python variables and the diverse data types available equips learners to manipulate data seamlessly. From integers to strings, lists to dictionaries, Python's data handling capabilities offer a robust foundation for aspiring developers. By incorporating Python variables and data types, beginners can unlock a world of possibilities in coding.
Python Syntax and Indentation Rules
Python's distinctive syntax and indentation rules exemplify its emphasis on code readability and structure. With clear, concise syntax and significant whitespace, Python code stands out for its elegance and simplicity. The indentation rules enforced in Python foster a disciplined approach to coding, promoting organized and visually appealing scripts. Leveraging Python's syntax and indentation guidelines aids in avoiding common errors and streamlines the debugging process effectively. By adhering to these rules, programmers can enhance the clarity and coherence of their Python code effortlessly.
Python Built-in Function
Python's vast repertoire of built-in functions adds a layer of versatility and functionality to programming tasks. From mathematical calculations to string operations, Python's built-in functions simplify complex operations and reduce the need for extensive code. Exploring Python's built-in functions exposes learners to efficient problem-solving strategies and enhances code reusability. By harnessing the power of built-in functions like range(), len(), or print(), coders can optimize their workflow and achieve streamlined results. Integrating Python built-in functions into coding practices streamlines development processes and propels learners towards proficiency in programming.
Exploring Python Control Flow
If-else Statements in Python
The integration of if-else statements in Python amplifies the decision-making capabilities within code structures. By evaluating conditions and executing specific blocks of code based on true or false outcomes, if-else statements enhance program logic and functionality. Python's if-else statements offer a logical flow to operations, enabling programmers to address diverse scenarios effectively. Embracing if-else statements in Python fosters a structured approach to problem-solving and enhances the robustness of coding practices. By mastering the nuances of if-else statements, learners can navigate through complex coding challenges with precision and clarity.
Loops in Python: for and while
Python's versatile loop structures, including for and while loops, empower programmers to iterate through data sets and execute repetitive tasks efficiently. By utilizing for loops for finite iterations and while loops for conditional repetitions, Python enhances the efficiency of data processing and manipulation. Leveraging Python's loop mechanisms accelerates the coding process and facilitates dynamic data handling. The flexibility and scalability offered by Python's loop structures cater to a wide range of programming needs, from simple list traversals to intricate algorithm implementations. Incorporating loops in Python code exemplifies a strategic approach to effective problem-solving and algorithm design.
Exception Handling in Python
Exception handling in Python fortifies code resilience and safeguards against unforeseen errors during program execution. By implementing try-except blocks, programmers can anticipate and manage exceptions gracefully, ensuring the continuity of program flow. Python's robust exception handling mechanisms mitigate risks associated with unpredictable events, enhancing code reliability and user experience. Delving into Python's exception handling capabilities equips learners with the tools to address potential errors proactively and maintain system stability. Embracing exception handling in Python underscores a disciplined approach to coding and instills best practices for error management and prevention.
Working with Python Data Structures
Working with Python Data Structures is a crucial aspect of this article, as it lays the foundation for understanding how data is organized and manipulated in Python programming. Python data structures, such as lists, tuples, dictionaries, sets, and strings, play a pivotal role in storing and managing data efficiently. By comprehensively covering the intricacies of data structures, readers can gain the necessary skills to tackle complex programming tasks and enhance their problem-solving abilities.
Lists, Tuples, and Dictionaries
Manipulating Lists in Python
Manipulating Lists in Python involves a range of operations like adding, removing, and accessing elements within a list. This functionality is fundamental for tasks requiring dynamic data management and manipulation. The versatility of lists in Python makes them a popular choice for various programming scenarios, allowing for flexible storage and retrieval of data. Understanding how to manipulate lists effectively empowers programmers to efficiently work with collections of items in their code, enabling them to iterate over elements, sort lists, or perform list comprehensions with ease.
Understanding Tuple Immutability
Tuple immutability in Python refers to the fact that once a tuple is created, its elements cannot be altered or modified. This property ensures data integrity and stability, making tuples suitable for representing fixed collections of values. While immutability restricts direct changes to tuple elements, it provides benefits in terms of data security and stability. By discussing tuple immutability, readers can grasp the significance of choosing tuples for scenarios where data integrity is paramount.
Dictionary Operations in Python
Dictionaries in Python offer key-value pair storage and retrieval mechanisms, enabling efficient data mapping and access. Understanding various dictionary operations such as adding entries, updating values, and deleting key-value pairs is essential for effective data management in Python. The versatility and quick look-up capabilities of dictionaries make them invaluable for scenarios requiring fast data access and retrieval based on specific keys. Exploring dictionary operations equips readers with the skills needed to leverage this powerful data structure in their Python projects.
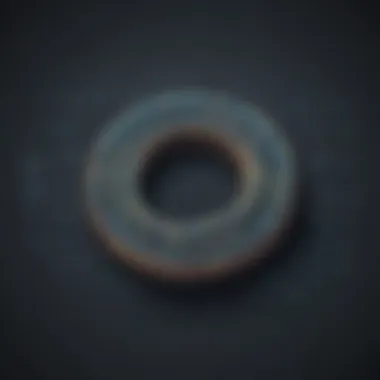
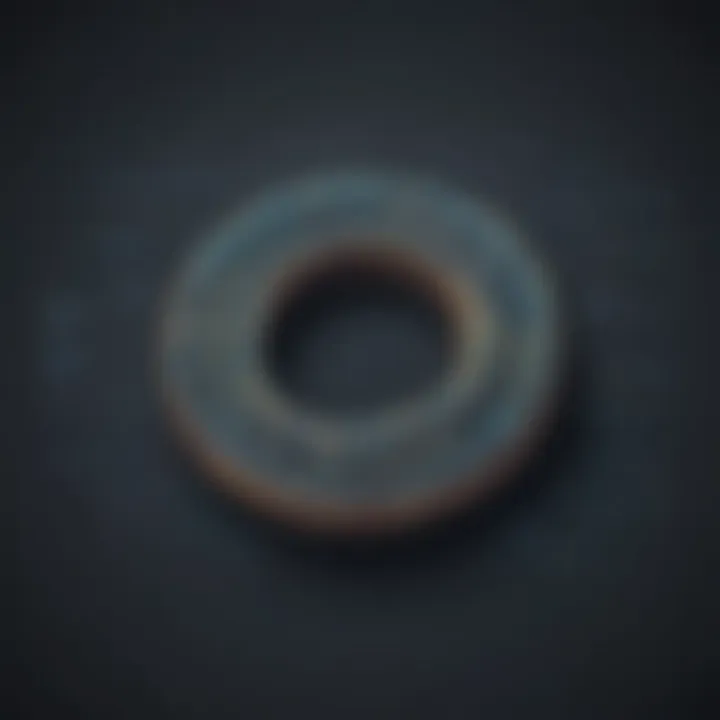
Sets and Strings in Python
Set Operations in Python
Set operations in Python entail common set operations like union, intersection, difference, and symmetric difference. Sets provide unique element storage and efficient membership testing, making them suitable for tasks that involve handling distinct values and set operations. The ability to perform set operations simplifies tasks such as deduplication, data comparison, and mathematical set calculations in Python code. By explaining set operations thoroughly, readers can harness the power of sets for managing unique collections of data elements.
String Manipulation Techniques
String manipulation techniques cover a wide array of operations such as concatenation, slicing, formatting, and searching within strings. Manipulating strings effectively is crucial for tasks involving text processing, data sanitization, and output formatting. Python offers robust string manipulation capabilities that facilitate tasks like pattern matching, character replacement, and encoding transformations. Understanding these techniques enables programmers to manipulate textual data efficiently and expressively in their Python scripts.
String Formatting in Python
String formatting in Python involves structuring output strings using placeholders or formatting directives to represent data dynamically. Proper string formatting enhances code readability, output precision, and enhances user interaction in text-based applications. Python provides multiple string formatting methods, including % formatting, str.format(), and f-strings, each offering unique benefits in terms of flexibility and readability. Exploring string formatting techniques equips readers with the skills to present data effectively and elegantly in their Python programs.
Functional Programming Concepts in Python
Functional Programming Concepts play a crucial role in the world of Python programming. By embracing functional programming, developers can write more concise, readable, and maintainable code. In this article, we delve into the significance of leveraging functional programming concepts in Python for beginners and intermediate learners. Understanding how to apply concepts like lambda functions, map-reduce, and functional paradigms can unlock new possibilities in Python programming. By emphasizing functional programming, readers can grasp a deeper understanding of Python's versatility and power.
Lambda Functions and Map-Reduce
Creating Lambda Functions in Python
Lambda functions in Python provide a concise way to create anonymous functions without the need to define a standard function using the def keyword. These compact functions are especially useful in situations where a small function is needed temporarily. Their succinct syntax and potential for use in situations like sorting or filtering make them a popular choice in Python programming. While lambda functions offer brevity and simplicity, their limitations lie in the complexity of operations they can handle, often restricted to single expressions.
Applying Map and Reduce Functions
The map and reduce functions in Python complement lambda functions by enabling operations on iterable data structures with ease. Map applies a specific function to each element in an iterable, while reduce performs a cumulative operation on a sequence. These functions enhance the capabilities of lambda functions by streamlining data manipulation tasks. While map and reduce offer efficiency, they may not always be the most readable choice, particularly in scenarios requiring more complex logic. Understanding when to utilize these functions is essential for optimizing Python code effectively.
Functional Paradigms in Python
Functional paradigms in Python encompass broader programming principles that focus on treating computation as the evaluation of mathematical functions and avoiding changing-state and mutable data. By adopting functional paradigms, developers can achieve a higher level of abstraction and separation of concerns in their code. While functional programming promotes reusability and reduces side effects in programs, it may introduce challenges for programmers accustomed to imperative styles. Embracing functional paradigms encourages a shift in mindset towards a more declarative and concise coding approach.
List Comprehensions in Python
Understanding List Comprehensions
List comprehensions offer a compact syntax for creating lists in Python based on existing sequences. By expressing a list comprehension in a single line, developers can achieve concise and readable code. The ability to apply conditions and loops within list comprehensions enhances their flexibility for generating lists efficiently. Despite their advantages in simplifying code, list comprehensions may become unreadable when overly complex, affecting code maintainability.
Advanced Techniques with List Comprehensions
Exploring advanced techniques with list comprehensions allows developers to leverage additional features such as nested comprehensions and multiple iterators. These techniques enable more sophisticated list generation strategies, improving code expressiveness and efficiency. However, complexity can arise when incorporating intricate logic within list comprehensions, potentially reducing code clarity and readability.
Benefits of List Comprehensions
The benefits of list comprehensions lie in their ability to offer a more expressive and concise alternative to traditional loops and conditional constructs. By condensing iterative operations into a single line of code, list comprehensions streamline the process of list creation and manipulation. This approach leads to cleaner and more efficient code, enhancing code readability and maintainability. While the advantages of list comprehensions are significant, developers should exercise caution to prevent excessive nesting and maintain code clarity.
Object-Oriented Programming in Python
Object-oriented programming is a crucial aspect in Python, providing a structured approach to software development. By focusing on objects and classes, developers can efficiently manage complex projects, enhance code reusability, and promote modular code design. Object-oriented programming encourages encapsulation, inheritance, and polymorphism, fostering code flexibility and scalability. Understanding OOP principles in Python is essential for building robust and maintainable applications, making it a fundamental concept for both beginners and intermediate learners.
Classes and Objects in Python
Defining Classes and Creating Objects
Defining classes in Python allows programmers to create blueprints for objects, enabling them to define attributes and methods. By encapsulating data and functionality within classes, developers can achieve code organization and abstraction. Creating objects from these classes enables the instantiation of specific instances with unique attributes. This practice enhances code efficiency, readability, and reusability, laying a strong foundation for a structured programming approach. Despite the overhead of class declaration, the benefits of clear structure and logical grouping outweigh the initial setup complexity.
Inheritance and Polymorphism in Python
Inheritance in Python allows classes to inherit attributes and methods from parent classes, promoting code reusability and hierarchical relationships. Polymorphism enables objects to take on multiple forms, allowing flexibility in method implementation based on object type. These concepts facilitate code extensibility, reducing redundancy and promoting efficient code design. While inheritance simplifies code maintenance and enhances modularity, excessive levels of inheritance can result in complex class hierarchies, leading to potential maintenance challenges.
Encapsulation in Python
Encapsulation in Python involves bundling data and methods within a class, restricting direct access from external sources. This practice promotes data security, prevents unintended modifications, and enhances code maintainability. Encapsulation fosters information hiding, allowing developers to shield internal implementations from external interference. While encapsulation improves code organization and security, excessive encapsulation can lead to rigid design and hinder code extensibility.
Class Methods and Attributes
Working with Class Methods
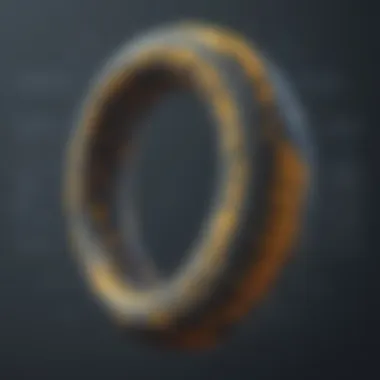
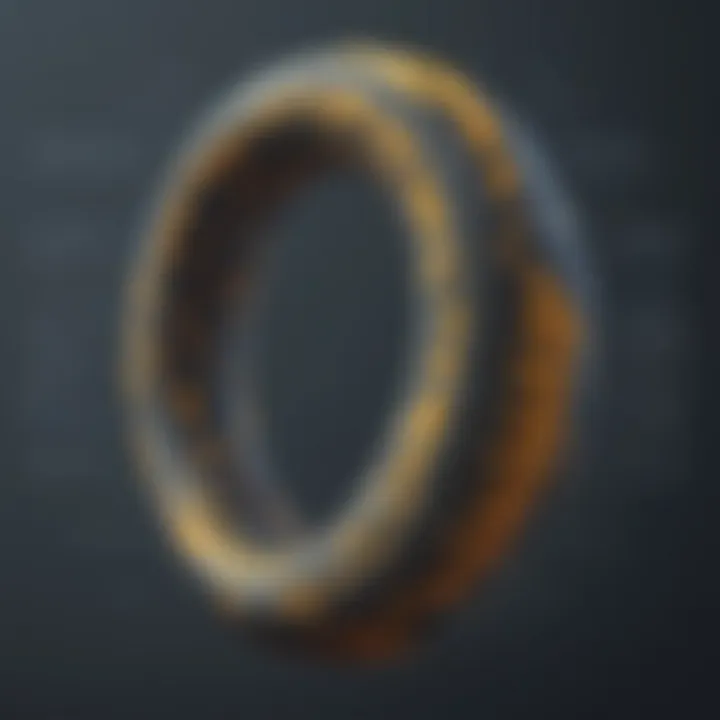
Class methods in Python are functions that operate on the class itself rather than instances. These methods are accessed using the class name and can modify class variables and access class attributes. Working with class methods simplifies code logic, promotes code encapsulation, and enhances class-level operations. By defining class methods, developers can implement actions that impact the class as a whole, facilitating centralized functionality and promoting code efficiency.
Understanding Class Attributes
Class attributes in Python are variables that are shared by all instances of a class. These attributes define common properties applicable to all class instances, providing consistent behavior across objects. By utilizing class attributes, developers can maintain data integrity, promote code consistency, and reduce redundancy. Understanding class attributes is crucial for achieving uniformity in class behavior, ensuring systematic data representation and efficient memory allocation.
Class Inheritance and Method Overriding
Class inheritance in Python allows subclasses to inherit attributes and methods from parent classes, promoting code reuse and extension. Method overriding enables subclasses to provide a specific implementation for inherited methods, tailoring functionality to meet subclass requirements. By leveraging class inheritance and method overriding, developers can build hierarchical relationships, enhance code flexibility, and promote modular design. While inheritance simplifies code organization and minimizes redundancy, excessive method overriding can lead to code intricacy and potential confusion among class interactions.
Utilizing Modules and Packages in Python
Utilizing modules and packages in Python is a crucial aspect covered in this article. When it comes to Python programming, modules and packages are indispensable tools that help in organizing code efficiently and promoting reusability. By utilizing modules, developers can break down their code into manageable chunks, making it easier to maintain and scale their projects. Packages, on the other hand, enable the structuring of related modules together, streamlining the development process.
Importing and Using Modules
Importing Standard Library Modules
Importing standard library modules is vital for accessing a plethora of pre-built functionalities in Python without the need to reinvent the wheel. These modules contain a wide range of tools and utilities that cater to diverse programming requirements. By integrating standard library modules, developers can significantly reduce development time and enhance code quality.
Exploring Third-Party Packages
Exploring third-party packages introduces developers to a vast ecosystem of additional functionalities beyond the standard library. These packages, developed by the Python community, offer specialized tools for specific tasks, widening the scope of possibilities for Python projects. While third-party packages extend the capabilities of Python, developers need to exercise caution regarding compatibility and dependencies to ensure seamless integration.
Creating and Importing Custom Modules
Creating and importing custom modules provides developers with the flexibility to tailor code according to project-specific needs. By encapsulating related functionalities within custom modules, developers can promote code modularity and maintainability. Custom modules allow for a structured approach to code organization, fostering collaboration among team members and simplifying debugging processes.
Managing Packages with Pip
Installing Packages with Pip
Installing packages with Pip is an essential step in leveraging external libraries and tools within Python projects. Pip, the package installer for Python, automates the process of downloading and installing packages from the Python Package Index (PyPI). This facilitates the integration of third-party functionalities seamlessly, enhancing the efficiency and effectiveness of Python development.
Updating and Removing Packages
Updating and removing packages with Pip ensure that Python projects stay up-to-date with the latest features and security patches. Managing package versions is crucial for maintaining project stability and mitigating vulnerabilities. By updating packages regularly and removing deprecated dependencies, developers can optimize project performance and enhance security.
Virtual Environments with Venv
Virtual environments with Venv offer developers isolated environments for Python projects, enabling them to manage dependencies and package versions seamlessly. By creating virtual environments, developers can avoid conflicts between project requirements and system-wide packages. Venv enhances project portability and reproducibility, ensuring consistency across different development environments.
Testing and Debugging Python Code
Testing and debugging Python code are critical aspects in this article as they ensure program reliability and efficiency. Thorough testing helps in identifying and fixing errors, minimizing potential issues in the code. Debugging, on the other hand, involves locating and rectifying errors that may arise during program execution. By focusing on testing and debugging, learners can grasp the importance of quality assurance and error handling in Python development, leading to more robust and stable programs.
Writing Test Cases in Python
Unit Testing with PyTest
Unit testing with PyTest is a key component in the testing process. PyTest offers a simple and scalable framework for writing test cases. Its ability to easily integrate with other testing tools and libraries makes it a popular choice among developers. The unique feature of PyTest lies in its concise syntax, making it efficient for testing various scenarios within the codebase. While it enhances code quality and reliability, the challenge with PyTest lies in setting up fixtures and understanding parameterization.
Test-Driven Development (TDD)
Test-Driven Development (TDD) emphasizes writing tests before actual code implementation. TDD promotes a systematic approach to programming and encourages breaking down tasks into smaller, manageable units. The key characteristic of TDD is its iterative nature, allowing developers to continuously test and refine their code. By following TDD practices, programmers can improve code quality, maintainability, and overall development speed. However, some developers may find the strict TDD workflow restrictive, especially when dealing with complex features.
Debugging Techniques in Python
Debugging techniques in Python are instrumental in identifying and resolving errors in code. Python provides robust tools like pdb for interactive debugging, allowing developers to step through code and evaluate variables at each stage. The main advantage of Python debugging is its simplicity and flexibility in handling complex issues. However, debugging can be time-consuming, especially when dealing with intricate logic or large codebases. It is essential to master debugging techniques to streamline the development process and ensure code accuracy.
Handling Exceptions and Errors
Handling exceptions and errors is essential in maintaining code integrity and preventing unexpected program crashes. By addressing common errors and tracebacks, developers can anticipate and manage potential issues before they impact the application. The key characteristic of handling errors lies in preemptive problem-solving and effective error message communication. While try-except blocks offer a structured approach to managing exceptions, developers should exercise caution to avoid masking errors unintentionally.
Common Errors and Tracebacks
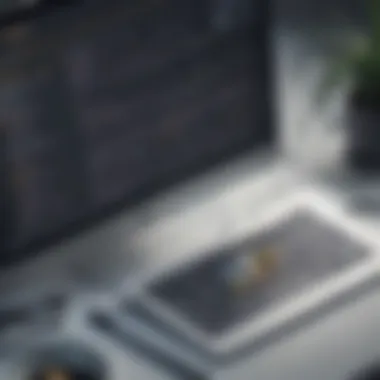
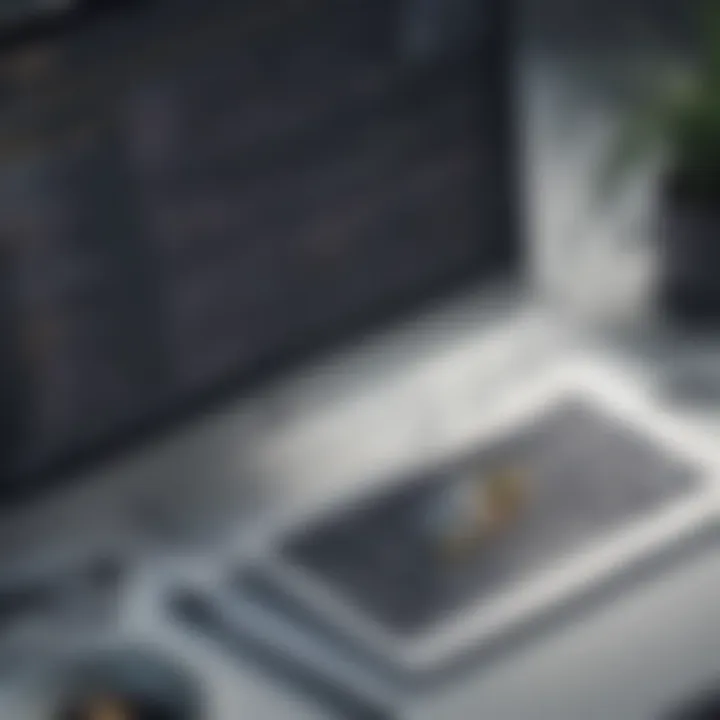
Common errors and tracebacks are prevalent in Python programming, often caused by syntax errors, improper variable usage, or incorrect function calls. Understanding common errors helps in quick troubleshooting and resolution, minimizing downtime during development. Highlighting common errors and tracebacks enables programmers to tackle issues proactively and enhance code robustness.
Try-Except Blocks in Python
Try-except blocks provide a mechanism to handle exceptions gracefully, preventing abrupt program termination. By encapsulating risky code within try blocks and defining appropriate exception handling in except blocks, developers can ensure code continuity and user experience. The key advantage of try-except blocks is their ability to maintain program flow even in the presence of errors. However, excessive use of try-except blocks can obscure code logic and complicate error diagnosis.
Logging and Error Handling
Logging and error handling play a crucial role in monitoring application behavior and capturing critical data for analysis. Effective logging practices facilitate error tracking, performance evaluation, and system debugging. The unique feature of logging lies in its configurability, allowing developers to define logging levels, outputs, and formats according to specific requirements. While proficient error handling enhances user experience and system reliability, improper logging implementation may lead to log file clutter and performance overhead.
Optimizing Performance in Python
Optimizing performance in Python is crucial for enhancing the efficiency of code execution, especially when dealing with computationally intensive tasks. It involves fine-tuning various aspects of code to minimize resource consumption and maximize processing speed. In this article, a detailed examination of optimizing performance in Python will be provided to equip beginners and intermediate learners with the necessary knowledge to write optimized code. By understanding the importance of performance optimization, readers can elevate their coding skills and create more efficient Python programs.
Profiling and Benchmarking Tools
Using cProfile for Performance Analysis
When it comes to analyzing the performance of Python code, cProfile stands out as a prominent tool. By utilizing cProfile, developers can identify bottlenecks and inefficiencies in their code, allowing for targeted optimizations. The distinctive feature of cProfile lies in its ability to provide detailed insights into the execution time of different functions and methods, aiding in the identification of areas that require improvement. Incorporating cProfile into the development workflow can significantly enhance code efficiency and streamline performance optimization efforts in Python.
Measuring Code Efficiency
Measuring code efficiency is a critical aspect of assessing the performance of Python programs. By evaluating factors such as algorithm complexity and resource utilization, developers can quantify the efficiency of their code. The key characteristic of measuring code efficiency is its objective nature, providing concrete metrics to gauge performance levels. Understanding the nuances of code efficiency measurement empowers programmers to make informed decisions when optimizing their Python code, leading to enhanced performance and streamlined execution.
Benchmarking Python Code
Benchmarking Python code serves as a means to compare the performance of different implementations or algorithms. By running benchmarks, developers can assess the speed and resource usage of their code under various conditions, facilitating data-driven optimization strategies. The primary advantage of benchmarking Python code is its ability to provide empirical evidence of performance improvements, enabling developers to make informed choices when optimizing their programs. Leveraging benchmarking techniques equips programmers with the insights needed to fine-tune their code and achieve optimal performance levels.
Optimizing Code Execution
Optimizing code execution involves refining the structure and logic of Python programs to boost performance output. By addressing bottlenecks and improving code efficiency, developers can enhance the speed and responsiveness of their applications. In this section, we will delve into key aspects of optimizing code execution to equip readers with practical strategies for enhancing program performance.
Identifying Bottlenecks in Python
Identifying bottlenecks in Python code is essential for pinpointing areas that impede execution speed. By closely examining the execution flow and resource allocation within a program, developers can isolate performance bottlenecks and prioritize optimization efforts. The key characteristic of identifying bottlenecks lies in its focus on enhancing critical sections of code to achieve significant performance gains. Effectively identifying and addressing bottlenecks empowers developers to streamline program execution and maximize efficiency.
Improving Code Efficiency
Improving code efficiency entails refining algorithms, data structures, and coding practices to reduce resource consumption and enhance performance. By optimizing loops, simplifying algorithms, and reducing redundant operations, developers can improve the overall efficiency of their code. The unique feature of improving code efficiency is its versatility, allowing for targeted optimizations tailored to specific performance issues. Implementing code efficiency improvements leads to faster execution times and more responsive applications, elevating the overall user experience.
Utilizing Numba for Speedups
Numba offers a compelling solution for accelerating Python code through just-in-time compilation. By leveraging Numba, developers can optimize critical sections of code by transforming Python functions into highly optimized machine code. The key characteristic of Numba lies in its ability to boost performance without sacrificing code readability or simplicity. Utilizing Numba for speedups provides developers with a powerful tool to enhance the efficiency of their code, making it an invaluable asset for performance optimization in Python.
Conclusion
Summary of Key Python Concepts
Recap of Python Basics and Control Flow
The Recap of Python Basics and Control Flow segment serves as a foundational review of fundamental programming concepts. It revisits essential topics such as variables, data types, syntax, and control structures, reaffirming their significance in building a strong programming foundation. By refreshing key concepts like if-else statements and loops, this recap not only solidifies understanding but also reinforces good coding practices. Its concise nature aids in quick revision, making it a valuable reference point for beginners and intermediate learners seeking to strengthen their Python knowledge.
Highlighting Essential Data Structures
Shedding light on essential data structures, this section focuses on the significance of lists, tuples, dictionaries, sets, and strings in Python programming. Understanding the manipulation and operations related to these data structures is crucial for effective information handling and retrieval. By exploring the unique characteristics and functionalities of each structure, learners gain insights into their practical applications and optimal usage scenarios. The emphasis on data structures underscores their role in organizing and managing data efficiently, enhancing the overall quality of Python code.
Emphasizing Object-Oriented Principles
Emphasizing Object-Oriented Principles delves into the core concepts of classes, objects, inheritance, and encapsulation in Python. By elucidating how object-oriented programming enhances code reusability and modularity, this section underscores the importance of structured design and development. Recognizing the benefits of class inheritance and method overriding allows programmers to create well-organized and scalable codebases. Understanding object-oriented principles equips learners with the tools to architect robust solutions and abstract complex systems effectively.
Future Learning Pathways
Advanced Python Topics to Explore
Delving into advanced Python topics, this segment expands on complex concepts such as decorators, generators, and metaclasses. Exploring these higher-level concepts challenges learners to deepen their understanding and expand their programming repertoire. By venturing into intricacies like metaprogramming and asynchronous programming, individuals can unlock new dimensions of Python's capabilities. Diving into advanced topics not only broadens knowledge but also fosters creative problem-solving skills essential for tackling advanced software development challenges.
Project Ideas for Application Development
By presenting diverse project ideas for application development, this section inspires learners to apply their Python skills to real-world scenarios. From web development projects to data analysis applications, the suggested project ideas cater to a range of interests and skill levels. Implementing these projects not only reinforces theoretical knowledge but also cultivates practical experience crucial for professional growth. Emphasizing project work encourages learners to explore their creativity and innovation, empowering them to turn abstract concepts into tangible solutions.
Continuing Education in Python
Continuing Education in Python outlines various avenues for ongoing professional development and learning. From advanced courses and certifications to community engagement and open-source contributions, this section underscores the importance of continuous learning in the ever-evolving field of programming. By staying abreast of industry trends and participating in collaborative learning environments, individuals can expand their skill sets and stay competitive in the dynamic job market. Prioritizing continuing education propels learners towards mastery and expertise in Python programming, setting a solid foundation for future success.