Exploring OOP Principles in Java Programming
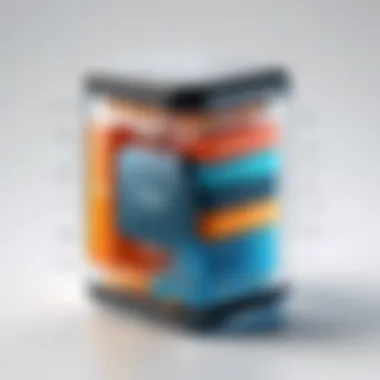
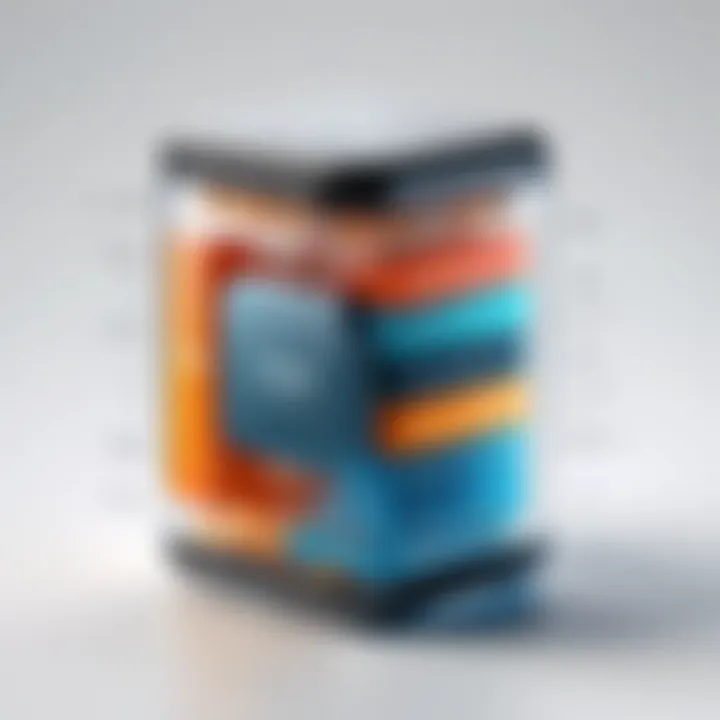
Intro
In the realm of software development, understanding the principles of Object-Oriented Programming (OOP) is akin to possessing a compass in uncharted territory. As a programming paradigm, OOP facilitates a structured way to design and manage software. Specifically in Java, these principles are woven into the fabric of the language, guiding how programmers approach data and functions. Before diving into these fundamental concepts of OOP, it’s crucial to grasp the broader picture of programming languages, their evolution, and their distinct characteristics.
Preamble to Programming Language
History and Background
Programming languages are like the distinct dialects of the computing world. They have evolved tremendously since the early days of computing. Consoles and basic machine code gave way to more sophisticated languages such as Fortran and COBOL. Java, introduced by Sun Microsystems in the mid-1990s, emerged as a game-changer, emphasizing portability and ease of use through the famous motto: "Write Once, Run Anywhere." This promise allowed developers to write code that could function across different platforms without modification, setting the stage for its widespread adoption.
Features and Uses
Java boasts a range of features that make it akin to a Swiss Army knife for developers:
- Platform Independence: Thanks to the Java Virtual Machine (JVM), Java applications can run on any device equipped with it.
- Rich Standard Library: A comprehensive collection of pre-built classes and methods facilitates faster development.
- Multithreading: This enables concurrent execution of threads, enhancing performance for complex applications.
- Strongly Typed: It requires explicit declaration of data types, adding a layer of error checking during compilation.
These attributes have made Java a preferred choice for diverse applications, from mobile software to enterprise-level solutions.
Popularity and Scope
Java's popularity hasn't waned over the years; it remains firmly in the developer's toolkit due to its versatility and widespread community support. As per recent statistics, Java ranks consistently among the top programming languages. Its usage spans web development with frameworks like Spring, mobile apps via Android, and desktop applications. Even in complex environments like cloud computing, Java's role is pivotal, showcasing its enduring relevance in the ever-evolving tech landscape.
"Understanding the foundation of Java, especially OOP principles, is essential for any programmer aiming for longevity in their career."
Basic Syntax and Concepts
Before delving into OOP, having a grip on Java’s basic syntax and concepts is essential. The language might look approachable, but the intricacies beneath the surface are what give it depth.
Variables and Data Types
At its very core, Java manipulates data with the help of variables, which are essentially named storage locations in memory. Knowing the appropriate data types—such as , , and —is crucial for efficient programming. For instance, when declaring an integer variable, it’s essential to denote it as follows:
Operators and Expressions
Operators in Java are the symbols that perform operations on variables. This includes arithmetic (like and ), logical (like ), and relational operators (like ). Good understanding of these operators is vital, especially when crafting expressions where results depend on complex calculations or logic flows.
Control Structures
Control structures, such as loops and conditional statements, direct the flow of execution in a program. The construct and loops like and allow for dynamic coding, responding effectively to user inputs and conditions.
Advanced Topics
Functions and Methods
Functions, or methods as deemed in Java, encapsulate code for specific tasks, promoting code reusability and organization. A well-structured approach to methods enhances code clarity. For instance:
Object-Oriented Programming
Central to our discussion, Object-Oriented Programming in Java rests on four basic principles: encapsulation, inheritance, polymorphism, and abstraction. Each of these pillars plays a crucial role in the design and functionality of Java applications.
Exception Handling
Error management is an often-overlooked aspect of programming. Java employs exception handling mechanisms to gracefully manage errors that arise during execution. This ensures that programs remain robust and user-friendly, even when unexpected situations occur.
Hands-On Examples
As they say, practice makes perfect. Engaging with simple programs provides a solid foundation. Begin with writing small Java applications that encapsulate OOP principles.
Simple Programs
Start with a basic program that models a 'Car' class, showcasing attributes and methods that illustrate encapsulation. For example:
Intermediate Projects
Progress to more complex constructs, such as creating an inheritance structure with a 'Vehicle' superclass. This allows for diverse classes like 'Car' and 'Truck' to inherit shared properties and methods, demonstrating the power of Java’s OOP design.
Code Snippets
Utilizing online resources, developers can often find snippets that illustrate best practices or unique solutions to common problems. These snippets can be incorporated to enhance project features and simplify complex code.
Resources and Further Learning
To further your understanding and mastery of Java and its OOP concepts, consider delving into the following resources:
- Recommended Books and Tutorials: Titles that are well-regarded in this field include "Effective Java" by Joshua Bloch and "Java: The Complete Reference" by Herbert Schildt.
- Online Courses and Platforms: Websites like Coursera and Udacity offer structured courses tailored to varying skill levels.
- Community Forums and Groups: Engaging in discussions on platforms such as Reddit and Stack Overflow can provide fresh perspectives and solutions to challenges you face in your coding journey.
Intro to Object-Oriented Programming
Object-Oriented Programming (OOP) is a fundamental paradigm in software development. Its significance stretches beyond mere syntax and code structure; it offers a framework that makes programming more intuitive and aligned with real-world concepts. The core concepts of OOP—encapsulation, inheritance, polymorphism, and abstraction—serve as building blocks that not only simplify complex programming tasks but also promote code reusability and maintainability.
Understanding OOP in Java is critical for any aspiring programmer. It's not just about learning to write code; it's about mastering a philosophy of thinking that influences how you approach problem-solving. The beauty of OOP lies in its ability to represent complex entities as objects, which mirror their real-life counterparts, making the logic more relatable.
In this article, we will delve into the essential components of OOP and their application in Java. By the end, readers will gain a thorough comprehension of how these principles enhance programming efficiency and robustness.
Defining Object-Oriented Programming
At its core, Object-Oriented Programming refers to a programming model that revolves around the concept of “objects.” These objects can represent anything from a tangible entity, like a car or a building, to more abstract concepts like a user or a transaction. Each object is characterized by its unique properties (attributes) and behaviors (methods).
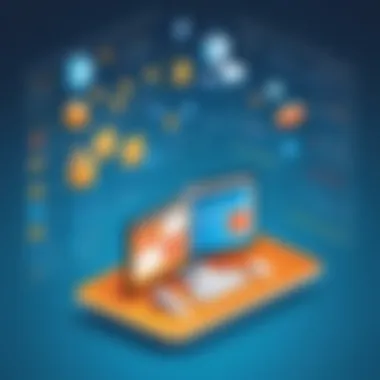
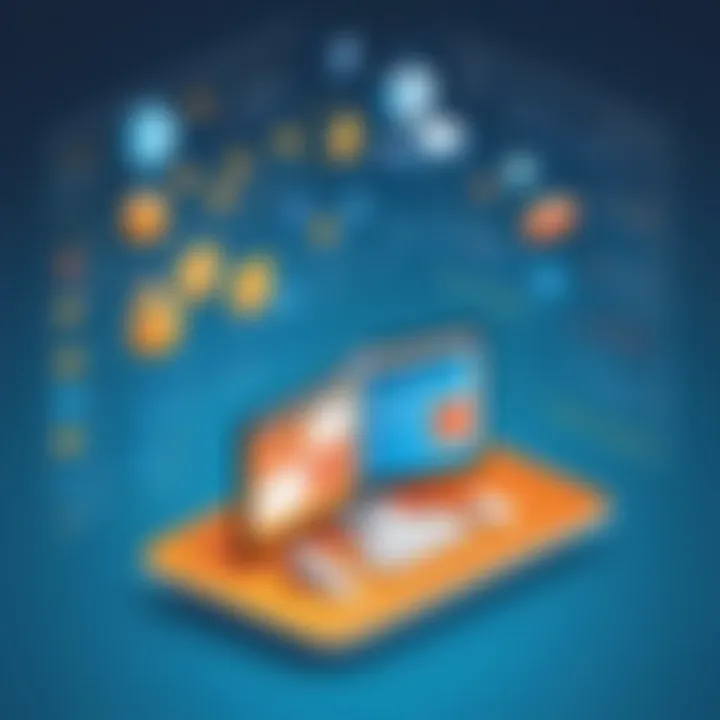
One of the defining features of OOP is the emphasis on organizing code in a way that mimics real-world interactions. This is often represented by the following key concepts:
- Classes and Objects: A class is essentially a blueprint for creating objects, defining their structure and behavior.
- Encapsulation: This principle involves bundling the data (attributes) and methods that operate on the data into a single unit or class. It restricts access to certain components, which is crucial for data protection.
- Inheritance: It allows new classes to take on properties and methods of existing classes, promoting code reuse.
- Polymorphism: This facilitates the use of a single interface to represent different underlying forms (data types), enhancing the flexibility of the code.
- Abstraction: It focuses on hiding the complex reality while exposing only the necessary parts. This is often implemented with abstract classes and interfaces.
This framework not only eases the writing of code but also its comprehension and maintenance, making it an indispensable tool in the software development landscape.
Historical Context of OOP
The roots of Object-Oriented Programming date back to the 1960s with the invention of Simula, widely credited as the first programming language to introduce objects. It was in the 1980s, with the arrival of languages like C++ and Smalltalk, that OOP began gaining traction in the programming community. These languages showcased the core principles of OOP and laid the groundwork for future programming languages.
Fast forward to the 1990s and 2000s, a shift towards OOP dominated the programming world with Java emerging as one of the most influential languages. It brought OOP into the mainstream by offering an impressive combination of objects and platform independence. Java's strong reliance on these principles through its architecture and libraries solidified OOP's importance in software development.
Today, OOP is widely practiced, with many modern programming languages embracing and expanding upon these original concepts. Its continued relevance in contemporary programming underscores how OOP has not only stood the test of time but also evolved to meet the needs of complex software solutions. Numerous industries, from finance to gaming, rely on the robust capacities provided by OOP principles to develop technology that is both functional and scalable.
The evolution of programming paradigms reflects broader changes in technology and user needs. Object-Oriented Programming stands as a testament to the necessity for adaptable software systems in a rapidly changing world.
Core Principles of OOP
Understanding the core principles of Object-Oriented Programming (OOP) is like finding the crux of a complex riddle; it unveils the foundational practices that programmers must exercise to create effective and sustainable software. Within this framework, encapsulation, inheritance, polymorphism, and abstraction serve as the four cardinal pillars. They are not merely academic concepts but rather practical tools that enhance modular design, improve code reusability, and facilitate easier maintenance which is crucial in modern software development.
Throughout this article, we will delve into each of these principles, dissecting their roles, significance, and impact on Java programming.
Explaining Encapsulation
Encapsulation is essentially a protective shield that bundles the data and the methods that operate on that data. This concept allows data to be hidden from the outside world, exposing only what is necessary through well-defined interfaces. Think of it as a capsule; what’s inside is carefully controlled and secured. In Java, this is typically implemented through the use of access modifiers like , , and .
As a result, encapsulation promotes safety and integrity of data by preventing unauthorized access and inadvertent modifications. Imagine a bank system where a customer’s information cannot be accessed freely; encapsulation plays a role similar to those security protocols in banking applications.
Through encapsulation, you can:
- Control the visibility of data
- Reduce the complexity of code
- Protect the integrity of the data
Understanding Inheritance
Inheritance allows a new class to inherit properties and methods from an existing class, promoting code reusability and hierarchy in your program. Think of it like a family tree where a child class inherits features from a parent class. This principle helps in establishing a clear relationship between classes, allowing developers to build upon already existing code without reinventing the wheel.
In Java, inheritance is implemented using the keyword. It’s beneficial because it promotes code sharing and reduces redundancy, leading to a more concise codebase. Additionally, it facilitates polymorphism, as you can treat objects of different classes in a uniform manner.
To illustrate, you might have a class that contains properties common to all vehicles, like speed and fuel capacity. Then you can create subclasses like and that inherit from .
Grasping Polymorphism
Polymorphism allows objects to be treated as instances of their parent class, particularly during method invocation. This means a single function can behave differently based on the object that is calling it. It's like having one tool that can adapt to different tasks depending on the context of its use.
Polymorphism can be categorized into two types: compile-time (or static) and run-time (or dynamic). The former is achieved through method overloading, while the latter is through method overriding. For instance, two classes may have a method named , but each class can implement this method differently depending on its specific needs.
Clarifying Abstraction
Abstraction focuses on hiding complex reality while exposing only the necessary parts. This concept allows programmers to work with high-level ideas without needing to know the intricate details. In Java, abstraction can be achieved using abstract classes and interfaces.
Consider a remote control for various appliances. You don’t need to know the inner workings of how each device operates; you just press its button to turn it on or off. Similarly, abstraction in programming allows users to interact with complex systems without getting bogged down in the specifics. This principle contributes significantly to reducing complexity and enhancing focus on the essential aspects of the software.
In summary, mastering these core principles forms the bedrock of effective programming practices in Java. They guide developers to produce cleaner, more maintainable, and reusable code—an essential skill in today’s fast-paced software development landscape.
Encapsulation in Java
Encapsulation is a fundamental pillar of Object-Oriented Programming, and its significance in Java cannot be overstated. By bundling the data (attributes) and methods (behaviors) that operate on the data into a single unit, or class, encapsulation enables a more structured approach to programming. This not only helps in organizing complex code but also enhances data security. Through encapsulation, you can control access to the inner workings of your classes. It raises a protective barrier around the data, allowing for controlled interaction between objects. In simpler terms, it keeps the bad stuff out while letting the good stuff in.
Defining Class and Object
In the world of Java, a class can be understood as a blueprint, while an object is an instance of that blueprint. When you define a class, you specify a set of properties and behaviors that all objects created from that class will share. For instance:
In this example, serves as the class, while and are the characteristics (attributes) and is a behavior (method). An object can then be created from this class like so:
Here, is an object that encapsulates the properties and methods defined in the class. By utilizing this encapsulation, changes made to data or behaviors within the class do not directly affect the object unless designed to do so, providing a layer of abstraction.
Access Modifiers and Their Role
Access modifiers are integral to encapsulation. They define the visibility of class members (attributes and methods). Java offers four primary access levels:
- Public: Members are accessible from any other class.
- Private: Members are only accessible within the defined class.
- Protected: Members are accessible within the same package and subclasses.
- Default (Package-Private): If no modifier is specified, members are only accessible within the same package.
Using access modifiers appropriately safeguards your data. For example, marking class members as private ensures they can't be accessed directly from other parts of your code, thus protecting the integrity of your object’s state. This is often used in conjunction with getters and setters.
Implementing Getters and Setters
Getters and setters are method types designed for controlled access to private class members. They allow for reading (getting) and modifying (setting) attributes safely.
For instance:
In this example, the class uses getter and setter methods to provide controlled access to the private fields and . This encapsulation principle facilitates additional functionality, like validation, without altering the object directly. Using getters and setters is not just a good practice; it's a cornerstone for establishing robust and maintainable Java applications.
"Encapsulation is about bundling the data with the methods that operate on that data. It’s a protective barrier that keeps the data safe from outside interference and misuse."
Inheritance Mechanisms
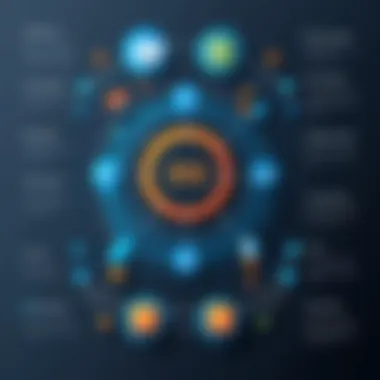
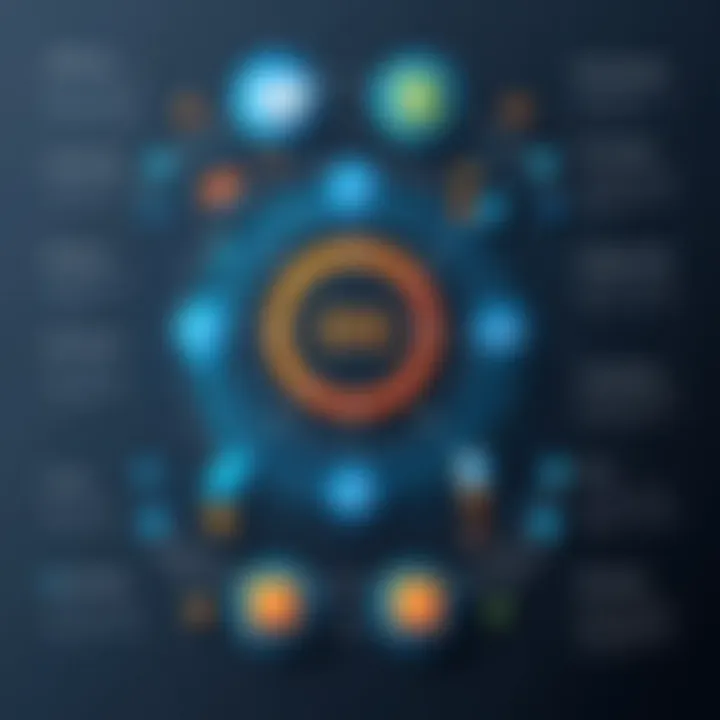
Inheritance plays a central role in the realm of Object-Oriented Programming (OOP), especially within the Java language. It allows programmers to create a new class based on an existing class, inheriting its properties and behaviors. This capability not only promotes code reusability but also helps in establishing a hierarchical relationship between different classes, which can simplify complex systems.
In Java, understanding inheritance is crucial for writing efficient, maintainable code. When you master inheritance, it paves the way for improved organization of code and makes it easier to manage large systems. A well-structured class hierarchy can lead to better readability and a more logical flow of code.
Single vs. Multiple Inheritance
Single inheritance refers to a scenario where a class can inherit from only one superclass. This is the default approach in Java. Think of it as a family tree where each child has one set of parents. Despite its simplicity, single inheritance can sometimes limit the versatility of class structures, especially when trying to incorporate diverse behaviors from multiple sources.
On the flip side, multiple inheritance allows a class to inherit properties and methods from multiple classes. While it enhances flexibility, it also introduces complications, such as the diamond problem, where ambiguity arises if two superclasses have methods with the same name. Although Java doesn’t support multiple inheritance directly through classes to avoid such complexities, it allows the use of interfaces to achieve a similar goal, thereby providing a workaround that embraces the advantages of multiple inheritance without its risks.
"Inheritance is the cornerstone of OOP, providing a foundation for creating rich class hierarchies that streamline coding efforts."
The Concept of Base and Derived Classes
In the context of inheritance, base classes—also known as parent classes—serve as the foundational class, while derived classes inherit attributes from this base class. Imagine the base class as a blueprint for a structure; every derived class builds upon that blueprint to create more specialized versions of itself.
For instance, consider a base class called . This class could have properties like , , and methods such as or . Derived classes like or can inherit these characteristics while adding their own unique features, such as for and for . This not only fosters a clean codebase but also helps in implementing common functionalities across different classes easily.
Method Overriding and Its Importance
Method overriding is a powerful feature of Java inheritance that allows a derived class to provide a specific implementation of a method that is already defined in its base class. This means that the derived class can change the behavior of a method by redefining it, while still retaining the same method signature.
This practice is key to achieving runtime polymorphism, which ultimately promotes flexibility and robustness in your programs. By allowing subclasses to modify inherited behaviors, you can tailor their operations according to specific needs without altering the base class. For example, if the class has a method , the class could override it to return a bark, while the class could override it to return a meow.
Polymorphism Explained
Polymorphism, a cornerstone of Object-Oriented Programming (OOP), is like a chameleon in the world of programming. It adapts to various contexts without losing its inherent form. Understanding this principle is vital for programmers using Java, as it not only enhances code flexibility but also promotes reusable code. By allowing the same method to perform different tasks based on the object’s state, polymorphism simplifies complex systems, making them easier to manage and extend.
Compile-Time vs. Run-Time Polymorphism
Polymorphism can be broadly categorized into two types: compile-time and run-time.
- Compile-Time Polymorphism: Also known as static polymorphism, this occurs during the compile stage of the program’s lifecycle. In Java, method overloading exemplifies this type. When a method has multiple definitions with different parameter types or counts, the compiler determines which method to invoke based on the arguments provided. This decision happens before the program runs, making it efficient. For example,In the above snippet, two methods exist — one for integers and one for doubles. The appropriate method is selected during compilation based on the input type.
- Run-Time Polymorphism: This type is realized during the execution of the program with method overriding. When a subclass alters a method from its superclass, the call to that method resolves to the most specific implementation at runtime, through dynamic method dispatch. This flexibility enables Java to exhibit behaviors that can adapt dynamically according to the object's class at runtime. A practical example involves:Here, the method is invoked on objects of type , but the actual method executed depends on the object type created ( or ). Thus, polymorphism permits different subclasses to provide specific implementations of the same method.
Polymorphic Behavior in Methods
The behavior of polymorphism enlivens Java methods. With polymorphism, methods can be designed to operate on objects of various classes without having explicit knowledge of their types. It brings several advantages:
- Code Reusability: One implementation can cater to various subclass types, avoiding redundancy in code.
- Flexibility and Maintainability: As systems evolve, developers can add new subclasses without modifying the existing code, thereby enhancing maintainability.
- Interchangeability: Objects can be exchanged, provided they share a common superclass, thus supporting a plug-and-play approach with plugins and extensions.
In addition, polymorphism invites complexities, especially concerning developer expectations and behaviors assigned to different classes. When using polymorphism extensively, careful documentation and architectural planning are crucial.
"Polymorphism allows parallelism of behaviors and interactions, hence creating a more flexible and open programming environment."
The insight of polymorphism is not just a mere academic abstraction; it’s a practical tool that molds better software.
In a nutshell, mastering polymorphism in Java paves the way for elegant coding solutions and heightens the ability to manage large-scale systems effortlessly. By understanding the unique traits of compile-time and run-time polymorphism, developers can harness this concept to create more adaptive and maintainable applications.
Understanding Abstraction in Java
Abstraction is a core principle of Object-Oriented Programming (OOP) that helps simplify complex systems by highlighting essential features while hiding irrelevant details. This is particularly important in Java, where managing complexity is a key concern for developers. By focusing only on the necessary aspects, abstraction enables programmers to work more efficiently and create cleaner code. The scope of abstraction in Java ranges from the design of applications to the implementation of features, making it a fundamental aspect worth understanding thoroughly.
In practical terms, abstraction allows developers to create models that can represent real-world systems without getting bogged down in unnecessary details. For instance, when designing a mobile application, one can abstractly represent the functionality of the phone's camera without needing to detail the myriad of electronic components that make it work. This not only streamlines the development process but also enhances maintainability, as changes can be made to underlying systems without affecting the overall architecture.
Abstract Classes vs. Interfaces
Abstract classes and interfaces are two key constructs in Java that serve to implement abstraction. Both serve as a blueprint for other classes but have distinct characteristics and use cases.
- Abstract Classes: These classes can contain both abstract methods (which do not have bodies) and concrete methods (which do). They allow you to create methods that can be shared across multiple subclasses while still defining certain methods that must be implemented by inheriting classes. An example of an abstract class might be a class that includes methods like and , while leaving the implementation details to subclasses like and .
- Interfaces: These are pure abstract types that declare methods but provide no implementation. Any class that implements an interface must provide the bodies for the methods it declares. For example, an interface might specify methods such as and , which any class like or must implement. Interfaces allow for greater flexibility since a single class can implement multiple interfaces, promoting a more modular approach to programming.
"The way to develop is to create. Abstraction simplifies the process by allowing focus on the necessary without losing sight of the larger system."
Real-World Applications of Abstraction
Abstraction finds its place in various real-world applications, underlining its importance in Java programming. Here are several practical scenarios:
- Software Design: In large projects, using abstraction allows teams to define high-level interfaces that various components adhere to, ensuring consistency across implementations.
- API Development: When creating APIs, developers use abstraction to provide users with an interface that may hide the complexity of underlying logic, thereby delivering a more user-friendly experience.
- Simulations: In systems like flight simulators or virtual environments, abstraction allows developers to represent complex operations—like flight dynamics—without delving into the nitty-gritty details. Users interact with a simplified model that captures essential behaviors without overwhelming them.
Ultimately, understanding abstraction equips programmers with the tools to design better systems that are easier to understand and maintain. As developers seek to create more robust and flexible Java applications, an in-depth grasp of abstraction principles will serve as a valuable asset.
Practical Applications of OOP in Java
Understanding the practical applications of Object-Oriented Programming (OOP) in Java is crucial for grasping how programming concepts translate into real-world use cases. OOP allows for a modular and organized way to develop software. By utilizing core principles like encapsulation, inheritance, polymorphism, and abstraction, developers can create applications that are easier to manage, test, and scale.
Benefits of OOP in Java
The significance of applying OOP principles in Java can be distilled into several key benefits:
- Modularity: OOP breaks down a program into smaller, manageable pieces. Each class can be developed independently, making collaboration easier among team members.
- Reusability: Through inheritance, common logic can be reused across multiple classes. This helps reduce redundancy and saves time during development.
- Maintainability: Changes made in one part of the code can be isolated, minimizing the risk of adversely affecting other sections. This certainly reduces the cost and complexity of maintaining software solutions.
- Scalability: As projects grow, OOP facilitates the addition of new features without destabilizing existing functionalities. With well-defined interfaces and abstractions, new components can be integrated seamlessly.
Considerations for Implementing OOP
While the advantages of OOP are substantial, developers should remain mindful of several considerations:
- Over-Engineering: It can be tempting to over-design a system with complex relationships and abstractions, leading to unnecessary complications.
- Learning Curve: For beginners, grasping OOP concepts can be challenging at first. Practical experience, along with the theoretical understanding, forms the best approach.
- Performance: Although OOP provides various benefits, there may be slight performance overhead due to the abstraction layers. Being aware of these trade-offs is important for optimization.
Culmination
In sum, the practical applications of OOP in Java aren't just theoretical; they offer real advantages that can enhance software development. From simplified maintenance to improved scalability, embracing OOP practices can significantly contribute to the efficacy and longevity of Java applications.
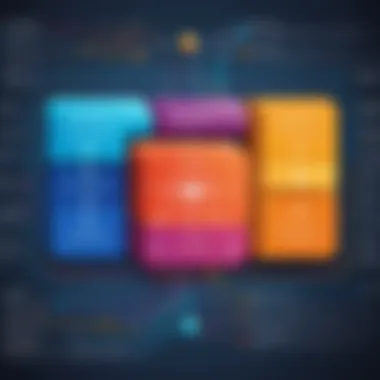
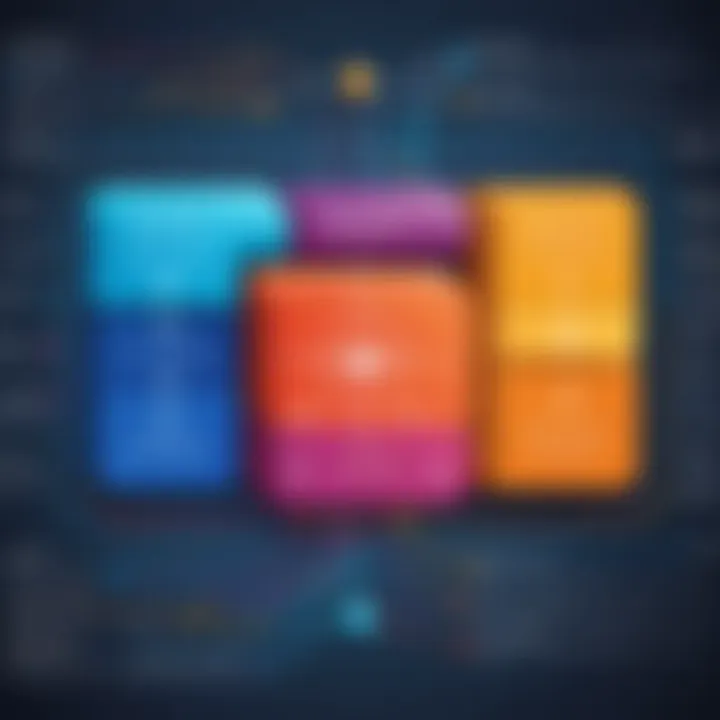
"With Object-Oriented Programming, you invest in the structure and design of your code, setting the stage for future growth and adaptation."
Case Study: Building a Simple Java Application
In this section, we’ll delve into a practical example of building a simple Java application, showcasing how OOP principles can guide the development process.
Understanding the Project
Imagine we want to create a straightforward student management system. The idea is to track student details, enrollments, and course registrations in a clean, organized manner.
Step-by-Step Implementation
- Define Classes and Objects:
We can create a class that encapsulates properties such as student ID, name, and email. Objects of this class will represent individual students. - Utilize Inheritance:
Next, we can derive classes from for specialized types, like and . This illustrates how inheritance allows us to enhance existing functionality smoothly. - Implement Polymorphism:
To demonstrate polymorphism, we can create a method in our base class and override it in derived classes, showcasing varied representations based on the student type. - Create a Main Class:
Finally, a main class can instantiate these objects and use their methods to interact with the student information, solidifying our understanding of how these principles work together in a cohesive application.
In summary, this simple application illustrates how OOP principles such as encapsulation, inheritance, and polymorphism culminate in a structured approach to programming.
Design Patterns and Their Relevance
Design patterns form a vital part of software development, especially in the realm of OOP. They provide standardized solutions to common issues in software design, enhancing adaptability and efficiency.
Importance of Design Patterns
The relevance of design patterns in Java can be summarized as follows:
- Proven Solutions: They offer tried and tested solutions to frequent problems faced during software development, reducing guesswork.
- Improved Communication: Understanding design patterns allows developers to communicate more effectively using a shared vocabulary.
- Flexibility and Reusability: Many design patterns facilitate reusability and modifiable code, leading to less redundancy and more maintainable applications.
Popular Design Patterns in Java
- Singleton Pattern: Ensures a class has only one instance and provides a global access point to it.
- Observer Pattern: Defines a one-to-many dependency between objects where changes in one object trigger updates in others.
- Factory Pattern: Allows for the creation of objects without specifying the exact class, promoting loose coupling.
Awareness and understanding of these design patterns can lead to more robust, easier to manage applications that adhere to sound OOP principles. Embracing design patterns not only aids in building advanced Java applications but also prepares developers for a future where code needs to be flexible and ever-evolving.
Challenges in Applying OOP Principles
Object-Oriented Programming (OOP) offers a robust framework for organizing complex software systems, yet applying these principles can be tricky. Understanding the challenges that come with OOP principles is paramount for both new learners and seasoned developers. It’s not enough to grasp concepts like encapsulation and inheritance; one must also navigate the potential pitfalls and intricacies that can arise during implementation.
Mastering OOP is akin to navigating a ship through a stormy sea—one wrong turn could lead to a shipwreck. Here, we dissect two of the most significant challenges: common pitfalls for beginners and the complexities inherent to larger systems.
Common Pitfalls for Beginners
OOP has a steep learning curve, which can be daunting for newcomers. Several issues often trip up these aspiring coders, leading to frustration and confusion. Here are some typical missteps to avoid:
- Over-using Inheritance: Beginners often lean too heavily on inheritance instead of composition, creating rigid hierarchies that complicate code maintenance. Remember, inheritance should be used judiciously.
- Misunderstanding Encapsulation: A lack of clarity about what needs to be encapsulated can lead to exposing too much of an object’s internal state, negating the advantages of the principle.
- Ignoring Abstraction: Failing to recognize the importance of abstraction can result in bloated classes with too many responsibilities. Striking a balance between detail and simplicity is crucial.
- Inconsistent Naming Conventions: Establishing clear, uniform naming conventions is significant for readability. Failing to do this can lead to confusion down the line.
To overcome these issues, beginners should embrace the iterative approach—build simple prototypes, refactor regularly, and don’t hesitate to ask for help or feedback.
"It’s easier to build a bridge than to rebuild a collapsed one."
Navigating Complexity in Large Systems
As projects grow in size and complexity, managing OOP principles can become an intricate endeavor. Large systems introduce layers of dependencies and interactions that can make understanding and maintaining the code a Herculean task.
The following factors often complicate OOP in larger applications:
- Coupling and Cohesion: High coupling can create a web of interdependencies between classes, making maintenance and testing a nightmare. Each change could ripple through the system, introducing bugs in seemingly unrelated parts.
- Managing State: Keeping track of an object’s state in complicated systems can lead to bugs if not handled correctly. Problems can arise from unintended side-effects when sharing state across different classes.
- Performance Overhead: Sometimes, principles like polymorphism and abstraction introduce performance penalties, especially if they lead to excessive object creation or method calls.
- Integration with Legacy Code: Many large systems have legacy components that may not adhere to OOP principles, leading to conflicts and clunky interactions.
Tackling these challenges requires a solid understanding of design patterns and architectural principles. While it may seem daunting, focusing on modular design, clear interfaces, and rigorous testing can alleviate some of the gotchas of OOP in larger systems.
Ultimately, the key to overcoming both beginner pitfalls and complexities in larger systems lies in continuous learning and adaptation. With experience and deliberate practice, one can turn obstacles into stepping stones for creating efficient and elegant code.
Future Directions of OOP in Java
The landscape of programming is constantly evolving. As Java remains a prominent player in the software development arena, understanding the future directions of Object-Oriented Programming (OOP) in Java becomes essential. This article segment addresses the fresh trends, potential innovations, and underlying concepts that will shape the upcoming generations of OOP, revealing not just the patterns but also the benefits of these emerging paradigms.
Current Trends and Innovations
Currently, several trends in the realm of OOP in Java are noteworthy. One of the significant movements is the shift towards functional programming, which began to gain momentum with the introduction of Java 8. Utilizing lambdas and streams, developers can write cleaner and more concise code that complements OOP principles. This fusion allows for more flexible and efficient coding practices.
In addition, the introduction of module systems has revolutionized how Java applications are built. Modularization promotes better organization of code, making it easier to manage large applications. As developers aim for higher reusability and maintainability, embracing modular design simplifies dependency management and enhances collaboration among teams.
Another stirring trend is the rise of domain-driven design (DDD). This methodology places emphasis on complex business requirements and real-world problems. Within Java, this fosters a deeper integration of OOP principles by closely aligning the code with the business domain concepts, enhancing the overall coherence of the software system.
Understanding these trends helps developers to stay relevant and effectively address modern challenges in software development.
The Role of OOP in Modern Software Development
As we look ahead, the role of OOP in software development continues to be crucial. Not only does it facilitate a natural way of thinking about software, but it also encourages a mindset that promotes reusability, scalability, and adaptability. This aspect is increasingly vital in today’s fast-paced development cycles where Agile and DevOps practices are the norm.
Furthermore, with the rise of cloud computing and microservices architecture, the design patterns derived from OOP provide a robust framework for building scalable applications. For instance, implementing polymorphic behavior across microservices can streamline service interactions while encapsulating the complexities involved in service management.
Additionally, the growing interface between artificial intelligence and OOP is presenting new opportunities. As AI tools become an integral part of software development, integrating OOP principles can help in building more robust and intelligible AI systems.
To summarize, keeping tabs on the future directions of OOP in Java isn’t merely an academic exercise. It’s a strategic necessity for anyone serious about making contributions in the programming field. Staying updated with trends such as functional programming, modularization, and domain-driven design enriches developers’ toolkit and enhances their ability to construct more maintainable and effective software.
Epilogue
The concluding segment of this article serves as a crucial wrap-up, offering an opportunity to distill the essence of Object-Oriented Programming (OOP) principles as they are applied in Java. The importance of this section is not just to summarize but to reflect on how understanding these concepts can significantly elevate one's programming acumen.
Summarizing Key Takeaways
In our exploration of OOP within the Java realm, we've encountered several pivotal ideas that interlace to create a robust structure for software design. Here are the primary takeaways:
- Core Principles: Encapsulation, inheritance, polymorphism, and abstraction form the bedrock of OOP. Each principle has its distinct role, contributing to making code more maintainable and comprehensible.
- Practical Implementation: Applying these concepts in real-world scenarios equips developers with strategies to build applications that are both efficient and scalable. Understanding how to translate these principles into Java code is paramount.
- Overcoming Challenges: Acknowledging common pitfalls can pave the way for a smoother journey in adopting these concepts. By reflecting on potential hurdles such as navigating complexity in large systems, programmers can arm themselves better.
- Future Prospects: Recognizing current trends and innovations within OOP not only keeps developers in the loop but also helps them stay ahead of the curve in an ever-evolving tech landscape.
To sum up, mastering OOP principles in Java is not just about the technical know-how. It's about cultivating a mindset that values effective software design. With these principles under your belt, you stand a better chance of creating software that is not only functional but also adaptable to future demands. As you venture into your own Java projects, let the insights gained from this article guide your path, ensuring that the code you craft is built on solid OOP foundations.