A Deep Dive into Java Variables and Their Uses
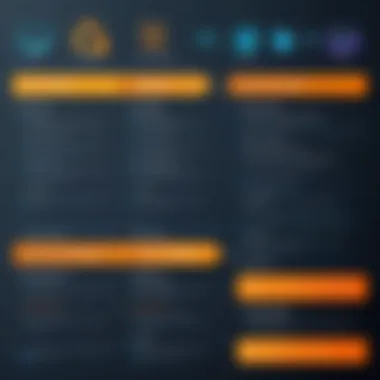
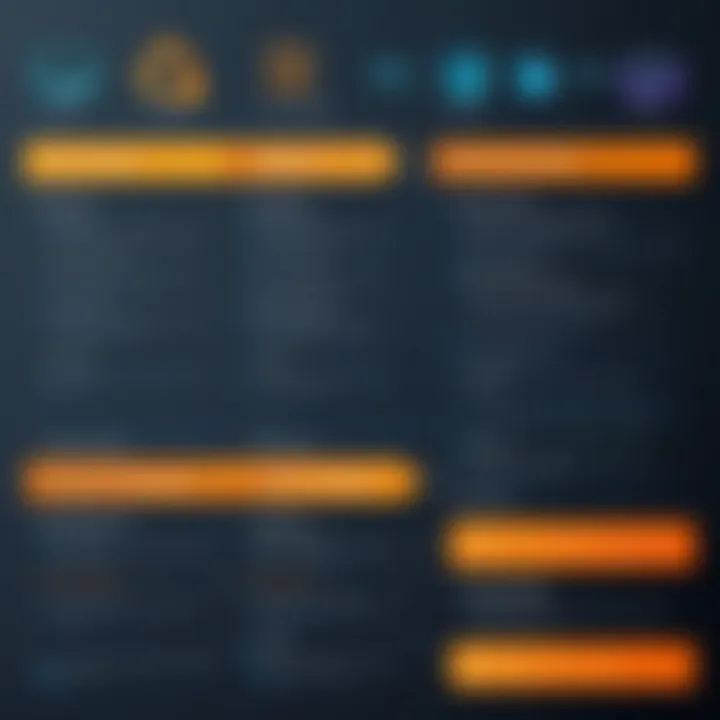
Intro
In the realm of programming languages, Java stands tall as a significant player. The foundation for understanding Java lies in its variables—the fundamental building blocks for storing data. As we navigate through this topic, we will uncover the intricacies of variables, including their types, scopes, and the best practices for their use in Java. This exploration aims to demystify the concept of variables, showcasing their importance in writing efficient and effective Java programs.
Preface to Programming Language
History and Background
Java was introduced in the mid-1990s by Sun Microsystems. Over the years, it has evolved from a simple programming language designed for small devices to a robust, versatile platform used across various applications—from mobile development to enterprise solutions. The drive behind Java's design was to create a language that was easy to use and adaptable, which has contributed to its lasting popularity.
Features and Uses
At its core, Java is an object-oriented language, which means it encourages developers to think in terms of objects rather than just code. This programming paradigm makes it easier to create complex applications that are modular and reusable. Notable features about Java include:
- Platform Independence: Java code can run on any machine that has the Java Virtual Machine (JVM), emphasizing its "write once, run anywhere" philosophy.
- Automatic Garbage Collection: Developers do not need to manually manage memory. Java automatically clears out unused objects, reducing memory leaks.
- Rich API: The Java Standard Library offers a comprehensive set of tools and libraries that facilitate everything from networking to graphics programming.
Popularity and Scope
Java remains highly regarded within the tech community. Its blend of reliability and scalability makes it the go-to choice for many organizations, especially those in finance, healthcare, and technology sectors. According to the TIOBE Index, Java constantly ranks among the top programming languages, evidencing its wide adoption and ongoing relevance.
Basic Syntax and Concepts
Variables and Data Types
At the heart of Java programming are variables. A variable can be understood as a container for storing data values which can be reused throughout your code. Java supports various data types, categorized into two broad categories: primitive types and reference types. Here's a quick overview:
- Primitive Types: Includes data types like , , , and . These types are predefined and have fixed sizes.
- Reference Types: Includes objects and arrays, which are created from classes.
Operators and Expressions
In Java, operators are essential for performing operations on variables. They can be classified into several types such as:
- Arithmetic Operators: Like , , , , and used for mathematical calculations.
- Comparison Operators: Such as , , ``, and which are crucial for making decisions in code.
- Logical Operators: These include , , and , enabling the construction of complex conditional statements.
Control Structures
Control structures direct the flow of execution in programs. Java offers several structures including if-else statements, switch, and loops like for, while, and do-while. They play a vital role in writing logic that can make decisions based on variable states.
Advanced Topics
Functions and Methods
Functions, or methods as they are often referred to in Java, are blocks of code designed to perform specific tasks. They are reusable, making code cleaner and easier to maintain. You define a method in Java with its return type, name, visibility (like or ), and parameters.
Object-Oriented Programming
Java's object-oriented features allow developers to create applications through encapsulation, inheritance, and polymorphism. For instance, variables in Java can define the attributes of an object, with each instance maintaining its own state and behavior.
Exception Handling
In programming, errors are inevitable. Java offers a powerful mechanism to handle these errors gracefully using exceptions. Utilizing , , and blocks allows programmers to manage runtime errors without crashing the program.
Hands-On Examples
Simple Programs
For novice programmers, writing simple Java programs can be enlightening. A typical hello-world program might look like this:
Intermediate Projects
As skills develop, tackling intermediate projects—like creating a basic calculator—can solidify understanding. A calculator project introduces variables, methods, and user input effectively.
Code Snippets
Utilizing code snippets is another avenue for learning. For example, a snippet for swapping two variables can illustrate variable operations nicely:
Resources and Further Learning
Recommended Books and Tutorials
For a deeper dive into Java, consider:
- "Effective Java" by Joshua Bloch
- "Java: The Complete Reference" by Herbert Schildt
Online Courses and Platforms
Several online platforms offer robust courses in Java programming. Check out platforms like Coursera and Udemy for courses tailored to all skill levels.
Community Forums and Groups
Joining forums such as Reddit's r/Java or participating in Facebook groups can foster interaction and creation of a peer learning environment. Seeking guidance from experienced programmers can also boost your learning curve.
Remember, a well-structured approach to learning Java variables and their applications can greatly aid in becoming a proficient developer. The journey into Java programming is filled with opportunities for creativity and problem-solving.
Foreword to Variables in Java
In the realm of programming, variables are the bedrock upon which logic and functionality are built. They act as storage locations for data that programs can manipulate. Java, being a statically typed language, has a specific and precise mechanism in place for how variables are declared, initialized, and utilized. Understanding variables is not merely academic; it's essential for effective coding and achieving desired program outcomes.
Defining a Variable
At its core, a variable in Java serves as a symbolic representation of a value that can change during the execution of a program. In more straightforward terms, think of a variable as a labeled container that holds data. For instance, if you declare a variable , you’re essentially creating a box labeled "age" where you can store whole number values.
When defining a variable, you must specify its type, which dictates what kind of data it will hold. This type could be a primitive data type like , , , or a reference type like or an array. Here’s a simple example demonstrating how to define several types of variables:
Combining both type and name creates a clear and focused point of reference in your program, allowing for effective data handling and manipulation.
Importance of Variables in Programming
Without variables, programming would resemble a ship tossed at sea, lacking direction and stability. Variables allow programmers to abstract and manage data, making it far easier to write and maintain complex algorithms. Here’s why they are so crucial:
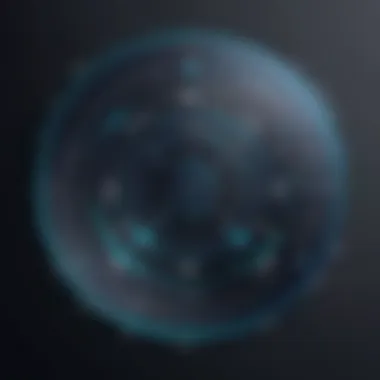
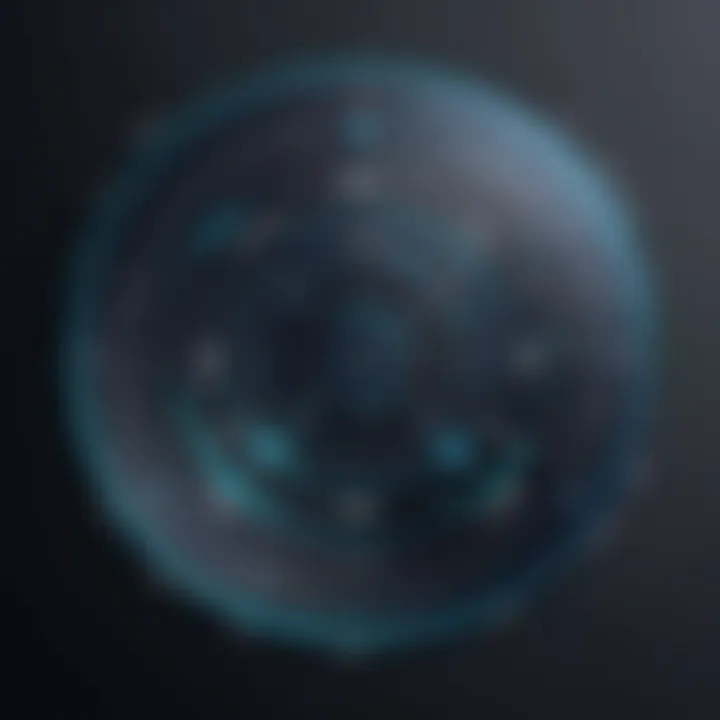
- Data Management: They enable programs to store, modify, and retrieve data efficiently.
- Dynamic Behavior: Variables provide the flexibility to change values during execution, adapting the program to different scenarios or user inputs.
- Code Readability: Well-named variables can make code much more understandable. For example, a variable named is more descriptive than one called .
Variables are the lifeblood of any program—without them, your code would be nothing but a series of instructions without context.
Choosing appropriate variable names and understanding their scope within the program can significantly enhance code quality. Additionally, familiarizing oneself with the types of variables and their respective uses can lead to fewer errors and a smoother programming experience. So, grasping the concept of variables is not just advisable—it’s paramount.
Types of Variables
Understanding the different types of variables in Java is crucial for anyone diving into this programming language. In a nutshell, variables act as containers for data, and knowing their types helps in managing and manipulating that data effectively. Each type has its specific purpose and characteristics, which can affect performance, memory usage, and even how data is interacted with in an application. Let's break this topic down into two major categories: Primitive Data Types and Reference Data Types.
Primitive Data Types
int
The int type in Java is often the go-to choice for handling whole numbers. Its primary characteristic is that it uses a fixed size of 4 bytes, allowing it to store values from -2,147,483,648 to 2,147,483,647. This fixed size means it is efficient in terms of both memory and performance. The use of int is prevalent because it's easy to understand and fits well with most numeric calculations required in basic programming tasks.
One unique aspect of the int type is its ability to facilitate arithmetic operations without complex conversions, making it a popular pick among programmers looking for simplicity and reliability. However, it lacks the capability to handle larger numbers, which might require switching to data types like long.
char
Next is the char type, which is designed specifically for representing single characters. Each char takes up 2 bytes, as it is based on the Unicode character set, allowing it to store thousands of different characters, including those from multiple languages. This feature makes char especially useful for applications that need to accommodate internationalization or any multi-lingual functionality.
The key benefit of using char lies in its succinct representation of character data. However, it’s worth noting that using char to represent string data can be limiting, since it only holds one character at a time, which means working with strings will require either a collection of char values or transitioning to the String type.
float
The float data type is leveraged when dealing with decimal numbers. With a size of 4 bytes, it offers a compromise between precision and memory usage. It can store values with approximately 7 decimal digits of precision, which makes it suitable for applications where high precision is not critical, like certain graphics calculations or game development.
The unique feature of float is its ability to handle larger ranges of numbers than int, while being memory efficient. Nevertheless, caution is warranted as floats can introduce problems with precision during calculations, which can lead to less accurate outcomes in critical applications.
double
For those instances requiring more precision in decimal numbers, the double type steps in. It utilizes 8 bytes of memory and can represent values with about 15 decimal digits of precision. This makes it a preferred choice for complex scientific calculations, financial applications, and anywhere where rounding errors cannot be tolerated.
Overall, while double is more resource-intensive compared to float, its capabilities often justify the extra memory cost. Just keep in mind that like float, double is also subject to precision limitations, so it’s not immune to rounding issues.
boolean
Lastly, we arrive at the boolean type, which holds only two possible values: true and false. This simplicity makes it indispensable in programming for controlling flow and making logical decisions. For example, if you have a user authentication system, facing true for valid login credentials and false for invalid ones can easily govern user access.
The major advantage of using booleans is their ability to simplify conditions within the code, making it clearer and easier to read. However, the limitation lies in its functionality, being just a simple true or false, thus not suitable for quantitative data processing.
Reference Data Types
When shifting gears from primitive data types, we step into the realm of Reference Data Types, which can embody more complex structures. This category includes Strings, Arrays, and Objects, each having their own unique benefits and considerations in Java programming. Understanding these types allows programmers to handle and manipulate data in ways that are beyond the confines of mere primitive types.
Variable Declaration
Variable declaration is a crucial building block in Java programming. It's the step where a programmer introduces a variable, laying down the foundation for later use. Without proper declaration, the code becomes a tangled mess, potentially leading to confusion and errors. Essentially, this process dictates to the Java compiler what you intend to use in your program. It defines the variable's name and the type of data it will hold. Clear declarations can make or break the readability of code, allowing others (or even your future self) to decipher its intention without much hassle.
When it comes to variable declaration, understanding the syntax is key. This knowledge not only helps in avoiding common pitfalls but also enhances coding efficiency. Which brings us to the next point.
Syntax of Declaration
The syntax for declaring a variable in Java is straightforward. The basic format is:
Here’s a deeper breakdown:
- type>: This tells Java what kind of data your variable will hold, such as , , or .
- variableName>: This is the label you give to the variable, which you'll use to refer to the data it contains.
For instance:
This makes it clear to anyone reading the code what each variable represents. Fine-tuning these details can help immeasurably in larger projects where clarity and efficiency are essential.
Examples of Variable Declaration
Understanding the syntax alone is not enough; seeing it in action is equally important. Here are a few examples:
- Integer Variable Declaration:Here, is declared as an integer variable, suitable for holding values like , , or .
- String Variable Declaration:This declaration works for text such as "Computer Science" or "Java Programming".
- Character Variable Declaration:The character can represent letters like , , or .
It’s critical to remember that Java is a strongly typed language. This means that the type must be declared at the outset, ensuring that your variable behaves as expected in your code.
"When you declare a variable in Java, you are laying the groundwork for data manipulation—get it right early on."
By grasping variable declaration and getting it correct the first time, you provide a solid base for your code, which can significantly ease future development tasks.
Variable Initialization
Variable initialization is a crucial step in the programming lifecycle in Java. It refers to assigning a value to a variable at the time of declaration or afterwards. This process solidifies the intention behind the variable's existence to not only store data but to represent a certain state or value throughout the execution of your program. Understanding and correctly utilizing variable initialization can significantly enhance code readability, maintainability, and even efficiency.
Types of Initialization
There are primarily two approaches to initializing variables in Java: Static Initialization and Dynamic Initialization. Each approach has its nuances and practical applications. Let's delve deeper into these types.
Static Initialization
Static initialization is when a variable is assigned a value at the time of its declaration. For instance:
This line of code demonstrates static initialization where the integer variable is set to 10 immediately. The main characteristic of static initialization is its predictability. When a variable is statically initialized, the assigned value is fixed and easily recognizable, making it a popular choice among programmers for a couple of reasons:
- Clarity: It offers immediate comprehension about the intent and state of the variable.
- Performance: Since the value is predefined and known at compile time, it eliminates any need for further computations or allocations at runtime.
However, static initialization may limit flexibility. If you need to change the initial value based on certain conditions, it falls short. That’s where dynamic initialization comes into play.
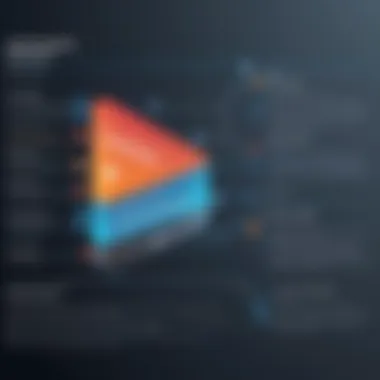
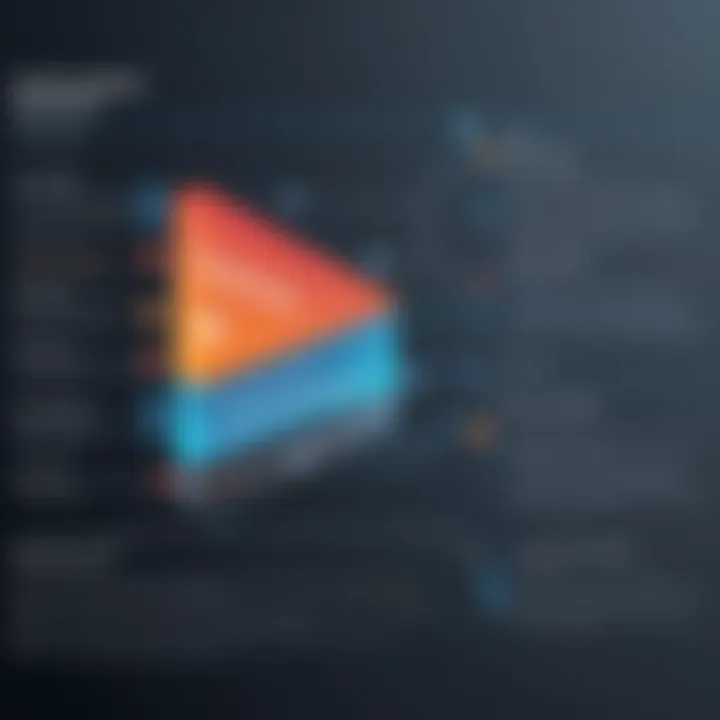
Dynamic Initialization
Dynamic initialization involves assigning a value to a variable at runtime. This can occur based on user input, calculations, or other runtime data. Consider this example:
In this case, does not get assigned until the user provides input, showcasing one of dynamic initialization’s key traits: flexibility. This approach caters to the need for adaptable programming, enabling developers to respond to various runtime scenarios. Here are some aspects of dynamic initialization:
- Versatility: You can set variables based on conditions or calculations, making your program responsive to varying situations.
- Complexity: However, with this flexibility comes increased complexity in debugging and ensuring that the variables are correctly set before use.
Best Practices for Initialization
To ensure you harness the full power of variable initialization, keep these best practices in mind:
- Use Static Initialization where a constant value is required. This keeps the code simple and enhances performance.
- Opt for Dynamic Initialization when your variables require values based on user input or other calculations. This adds adaptability to your code.
- Avoid Uninitialized Variables: Always strive to initialize your variables, even if to a default value, to prevent unexpected behaviors in your code.
- Keep Readability: Choose meaningful variable names that reflect their purpose, regardless of whether you employ static or dynamic initialization.
"Initialization isn’t just a step; it’s a foundational concept that shapes how your program understands data across its lifecycle."
Following these practices can lead to cleaner, more efficient code. Embracing both static and dynamic initialization strategies allows for a more comprehensive understanding of how variables function within Java.
Variable Scope
Understanding variable scope is essential for anyone diving into Java programming. It defines the accessibility of a variable and dictates where the variable can be utilized within the code. Essentially, scope can be viewed as the boundaries set around variables, determining their lifespan and visibility in different contexts. This knowledge can enhance code readability, troubleshoot errors, and maintain cleaner code practices.
Local Variables
Local variables are defined within a method, constructor, or block of code, and can only be accessed from that specific point, ceasing to exist once the flow of execution exits that block. These variables are not visible outside their defined scope, hence, management of local variables promotes good programming habits. When you declare a local variable, it demands initialization before it can be utilized. Otherwise, your program will throw a compile-time error.
Consider this example:
In the above scenario, the variable can’t be accessed beyond the method. This decision on its life span helps to avoid unintentional conflicts between variables among various methods. Utilizing local variables responsibly can improve not just flow, but also memory management since these variables are only allocated on the stack when needed.
Instance Variables
Instance variables, unlike local variables, are defined within a class but outside any method or block. They represent an object's properties, storing values unique to each instance of the class. If you instantiate the same class multiple times, each object will have its own copy of these variables.
An attractive aspect of instance variables is their visibility. They can be accessed by all the methods in the corresponding class, as well as by subclasses (depending on their access modifiers). For instance, a scenario might look like this:
In this instance, can be set upon creating a object and accessed anywhere within the class methods. Notably, unlike local variables, instance variables persist as long as the object exists, contributing to the statefulness of class instances.
Static Variables
Static variables are a notion worth delving into when discussing variable scope in Java. These variables belong to the class rather than instances of it and are defined using the keyword. This means there is only one copy of the variable, regardless of how many objects are created from that class. Such a feature is particularly useful for attributes that should be shared across instances.
Furthermore, static variables are initialized only once, which can be advantageous for resource allocation. Take a look at the example below:
In this case, every time a new is created, that static variable increases. When you call , it reveals an accumulated count of how many instances have been created. The significance of scope here is profound, as it allows maintaining a global state tied to the class itself rather than to individual objects.
Understanding the distinctions in variable scope enables developers to write cleaner and more organized code, avoiding conflicts and reducing memory usage better.
In summary, grasping variable scope is not just about knowing where variables can be accessed; it is about making informed decisions that elevate the quality and efficiency of your Java coding. Local, instance, and static variables each have their unique roles and scopes, and mastering these can lead to superb programming outcomes.
Variable Naming Conventions
Naming conventions in Java carry substantial weight. The way programmers name variables impacts code readability, maintainability, and overall functionality. When you think about it, names are the first communication between your code and whoever picks it up later—whether it's your future self or a colleague who has to debug. Getting these names right can save time, reduce the likelihood of bugs, and enhance collaboration. Variables should be descriptive enough that anyone can guess their purpose without having to pry into the implementation.
Rules for Naming Variables
When it comes to naming variables in Java, adhering to established rules is critical. These rules ensure that names are not only valid but also meaningful. Here’s a rundown of essential rules:
- Start with a letter: Variable names must begin with a letter (a-z or A-Z), an underscore (_), or a dollar sign ($). Starting with a numeral is a no-go.
- Use camelCase for multi-word names: This involves capitalizing the first letter of each subsequent word. For example, is preferable to .
- Length matters: While there's no strict character limit in Java, keeping variable names concise yet descriptive aids comprehension. Aim for clarity rather than brevity.
- Avoid reserved keywords: Names like , , or are reserved for Java's own use. Using them as variable names will result in confusion and potential errors.
These rules might seem simple, but straying from them can lead to chaos. If the name doesn’t follow the standard, you might find yourself wrestling with the compiler.
Common Practices in Naming
Beyond mere rules, several common practices can enhance variable naming even further. Following these tendencies can help you stand out in the world of programming:
- Be descriptive: Instead of , opt for something like which communicates the variable's purpose clearly.
- Stick to a convention: Choose either camelCase or snake_case and stick with it throughout your code. Consistency reduces mental overhead.
- Group related variables: When working with similar variables, try to use prefixes that indicate they are part of a family. For instance, , , and all share a clear connection.
- Use nouns: Variables often represent entities. Using nouns like or helps encapsulate the intended meaning clearly.
As you navigate the maze of coding, remember that a well-named variable dramatically contributes to the clarity of your codebase. It’s not just about getting the code to work; it’s about crafting a narrative that others can follow.
"The right name is a thing of beauty and can make dangerous code safe, while a poor one can hide the most benign logic."
Memory Management and Variables
Memory management is a cornerstone of programming, especially in languages like Java where understanding how variables operate can significantly impact the performance of an application. Managing memory efficiently allows programmers to optimize the use of resources, ensuring that applications run smoothly while limiting leaks or crashes. In the context of variables, memory management involves understanding how different types of variables consume memory and how they can be allocated effectively.
When programmers create variables, they often overlook how much memory these variables take up, particularly in larger applications. The more awareness you have about memory allocation and garbage collection, the better you'll be equipped to write efficient code. Here we’ll explore two crucial aspects of memory management in Java: memory allocation for variables and the garbage collection mechanism.
Memory Allocation for Variables
When you declare a variable in Java, the Java Virtual Machine (JVM) allocates a certain amount of memory for it based on its data type. For example, an typically takes up 4 bytes of memory, while a requires 8 bytes. Each data type has specific memory requirements that can impact the overall memory footprint of an application.
Here's a brief overview of memory allocation for some common types of variables:
- Primitive Types:
- Reference Types:
- : 4 bytes
- : 2 bytes
- : 4 bytes
- : 8 bytes
- : 1 byte (though implementation details may vary)
- Strings: Requires additional memory for storing character data, along with overhead for object management.
- Arrays: Memory allocated dynamically based on the length of the array.
- Objects: The actual memory usage will vary based on the fields defined in the class.
The JVM utilizes a specific area of memory called the heap for dynamic memory allocation when you create objects at runtime. Understanding how Java allocates memory for different variable types aids in writing code that minimizes unnecessary memory consumption and maximizes efficiency.
Garbage Collection Mechanism
Garbage collection in Java refers to the process of automatically reclaiming memory by deleting objects that are no longer in use. This is crucial in managing memory, as it prevents memory leaks—situations where allocated memory is not released back to the system, leading to inefficient memory consumption and potentially crashing an application.
The garbage collector operates in the background, periodically scanning for object references that are no longer accessible. When such objects are identified, the garbage collector deallocates their memory. It's important to note that while Java handles garbage collection automatically, this doesn’t mean developers shouldn’t care about memory management.
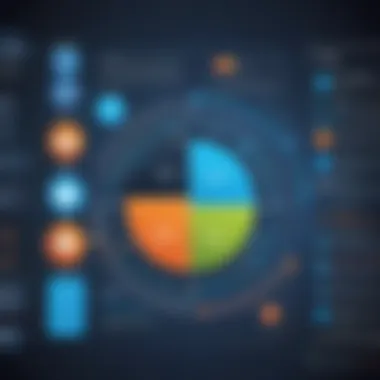
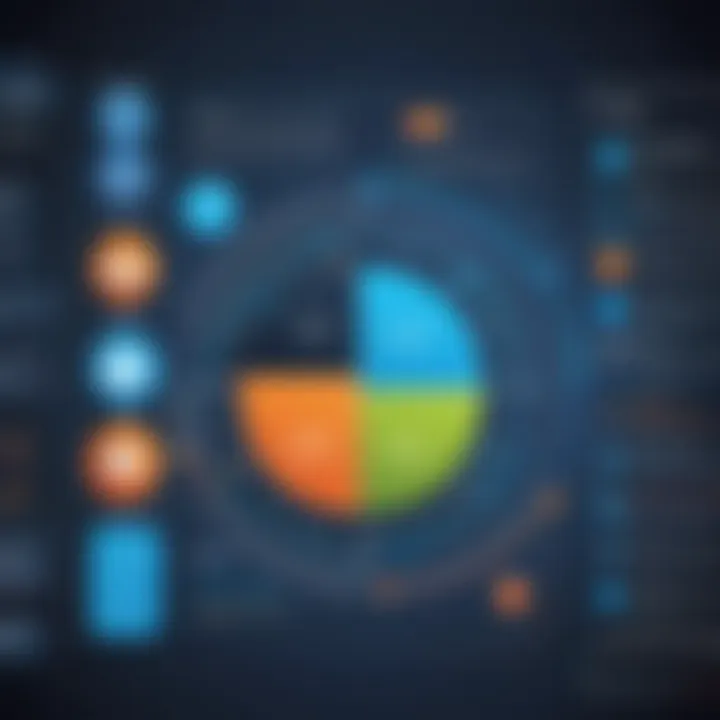
Some key points about garbage collection include:
- Generational Approach: Objects in Java are usually categorized by age—newly created objects are placed in the Young Generation, while older objects are moved to the Old Generation after surviving some garbage collection cycles. This helps in optimizing the collection process, as most objects are short-lived.
- Tuning Option: The garbage collector can be tuned for specific behaviors, allowing programmers to enhance performance depending on the needs of their application. Different algorithms, like G1 or CMS, come with optimizations suitable for various scenarios.
In summary, grasping how memory management intertwines with variables in Java is essential for maintaining robust and performant applications. Both memory allocation and garbage collection are key components that every Java developer must understand to utilize variables effectively, optimize performance, and avoid common pitfalls associated with inefficient memory use.
Variables in Object-Oriented Programming
Variables play a crucial role in object-oriented programming (OOP) as they serve as the backbone for managing state and behavior within objects. In Java, the treatment of variables through OOP principles enables programs to be more modular, readable, and maintainable. Understanding how variables interact with classes and objects provides insights into data encapsulation, inheritance, and abstraction—core tenets of OOP that influence software design.
Encapsulation and Variables
Encapsulation refers to the bundling of data (variables) and methods (functions) that operate on that data into a single unit, or class. It restricts access to certain components, which is a protective barrier that prevents outside interference and misuse of the methods and variables in the object.
The significance of encapsulation in relation to variables can’t be overstated. Here are key elements to consider:
- Data Hiding: Through encapsulation, you can make variables private. This means they cannot be accessed directly from outside the class. This is vital for maintaining the integrity of the variable’s data.
- Getters and Setters: To access or modify the variables, classes use getter and setter methods. For instance, if you have a variable , you might have a method to get its value and another to update it. This allows you to place conditions on how variables are modified.
The approach above reinforces the notion that variables are not merely placeholders for data; they also incorporate rules governing how that data is manipulated. This encapsulation leads to cleaner code, where modifications and maintenance are simplified since one can alter the implementation without the need to change how the rest of the program interacts with it.
Static vs Non-Static Variables
The distinction between static and non-static variables is fundamental in understanding memory management in Java. Both types of variables serve unique purposes, and knowing when to use each is essential for effective programming.
- Static Variables: Also known as class variables, they are shared among all instances of a class. A static variable is initialized only once, at the start of the program. This means any change made by one instance affects all others. This is particularly useful for maintaining a common state across all instances.
- Non-Static Variables: Also known as instance variables, these are tied to individual instances of a class. Every time you create a new object, a fresh copy of the non-static variable is created. Therefore, changes in one object do not impact the state of another.
Here’s a simple example:
In this example, is a static variable shared by all object instances, while remains unique to each instance. That difference holds weight when designing your classes; using static variables wisely can lead to memory savings and streamlined code, while non-static variables enhance the individuality of each object’s state.
Managing variables in object-oriented contexts enriches Java programming, ensuring data is treated with respect and clarity. As more developers embrace these principles, understanding the interplay between encapsulation, static, and non-static variables becomes even more pertinent.
Common Errors with Variables
When embarking on the journey of programming in Java, understanding the common errors associated with variables can be the difference between a smooth sailing experience and a stormy endeavor. This section sheds light on those pitfalls, arming learners with the insight to navigate around them effectively. Addressing these errors not only improves coding skills but also boosts confidence when tackling more complex topics later on.
Uninitialized Variables
One of the most frequent errors programmers encounter is the use of uninitialized variables. In Java, when a variable is declared but not initialized, attempting to use that variable can lead to a . This is because Java enforces rules to ensure that variables hold some definite value before they are accessed.
For instance, consider a situation where a programmer declares an variable to hold a numerical value but forgets to assign it one:
In the example above, the compilation will fail because has not been initialized. This error is akin to trying to start a car without key; it simply doesn’t work. To avoid this, programmers should ensure that all variables are initialized before use, either at the time of declaration or right before their first use. Initializing variables is a good habit that helps maintain code clarity and reduces errors down the line.
Type Mismatch Errors
Another headache that can pop up when dealing with variables is type mismatch errors. These arise when a variable is assigned a value that does not match its declared data type. Such mistakes can lead to unexpected behavior and bugs, often requiring time-consuming debugging to remedy.
Here’s a straightforward example:
In this case, trying to assign a string to an integer variable is like fitting a square peg into a round hole; it simply won't fit. Java is a statically typed language, meaning types are checked at compile-time. If there's a mismatch between the variable's type and the assigned value, the compiler will raise an error, prompting the programmer to fix the inconsistency.
To steer clear of these errors, it's pivotal to double-check variable assignments and ensure that the right type is being used both for declaration and initialization. Common types, such as , , , and , each have specific values they can hold, and being mindful of these can save a programmer a lot of unnecessary grief.
Remember: Pay attention to variable types and ensure they match when assigning values. This practice not only prevents compilation errors but also helps to maintain readable and robust code.
By recognizing and addressing these common variable errors, programmers can avoid many headaches, fostering a more harmonious coding experience. Taking the time to learn these lessons early on can pay off significantly as one dives deeper into the complexities of Java.
Advanced Concepts in Variables
Understanding advanced concepts in Java variables is vital for any developer who seeks to push their skills beyond the basics. Such knowledge can lead to more efficient and cleaner code, helping you avoid pitfalls that might otherwise bog down development. Here, we will explore some key themes, benefits, and considerations regarding variable shadowing, constants, and new features like the keyword in modern Java.
Variable Shadowing
When discussing Java variables, variable shadowing can catch many developers off guard. This phenomenon occurs when a variable declared within a certain scope has the same name as a variable in an outer scope. Essentially, the inner variable 'shadows' the outer one, leading to potential confusion. For instance:
In this example, while inside the method, the used will refer to the inner variable. Variable shadowing can be a double-edged sword. On one hand, it allows for more localized control of variable values. On the other hand, it can create bugs that are hard to trace and errors that may take some time to surface.
Important Note: Always be conscious of variable names and scopes. Choosing distinct names can save a lot of headaches down the line.
Constants and Final Variables
Constants, or final variables in Java, represent a crucial part of variable management. When we declare a variable as , it signifies that the variable's value cannot be changed after its initialization. This feature is widely used in situations where a constant value is needed, for example:
Using final variables improves code readability and maintainability. Identifying which values are constants helps both you and others working on the project to understand the constraints and intentions of your code. Beyond just naming conventions, this practice prevents accidental changes to important values, ensuring stability across the program.
VarKeyword in Modern Java
Introduced in Java 10, the keyword allows developers to declare local variables without explicitly specifying their type. This can make the code cleaner and easier to read, although it also requires developers to pay extra attention to the context in which the variable is used. For example:
In the above line, is automatically inferred to be an . While using can enhance your coding efficiency, it's important to use it judiciously. Clarity should always take precedence; if the type is not obvious, it might be better to explicitly define it.
Epilogue
As we draw to a close on our exploration of variables in Java, it's crucial to grasp just how significant a well-rounded understanding of this topic is. The realms of programming are vast and layered, but variables stand as the very backbone of any effective code. They allow developers to store, manipulate, and retrieve data, making them central to the logic and flow of any program. In essence, mastering variables equips learners with essential tools to tackle more complicated programming paradigms in Java and beyond.
Recap of Key Points
To summarize our discussion, here are the salient points we've traversed:
- Definition and Types: We defined what variables are and classified them into primitive and reference data types. Each type has its unique properties that dictate how data is stored and manipulated.
- Variable Declaration and Initialization: We examined the syntax for declaring variables, as well as the vital process of initialization, emphasizing the nuances of static and dynamic methods.
- Scope and Naming Conventions: The understanding of scope—where a variable can be accessed within the program—is essential. Name your variables wisely; appropriate conventions make your code readable and maintainable.
- Memory Management: Variables require memory allocation, and we highlighted the importance of garbage collection, which helps manage memory usage effectively.
- Integration in Object-Oriented Programming: We touched on the significant role of variables in encapsulation and how they set a solid foundation for creating complex systems.
- Common Errors: Understanding potential pitfalls, like uninitialized variables and type mismatch errors, strengthens programming skills.
- Advanced Concepts: Lastly, we explored concepts like variable shadowing and the keyword in modern Java, offering a glimpse into more nuanced programming techniques.
These elements lay the groundwork for any aspiring programmer navigating the Java waters, providing invaluable insights into efficient coding practices.
Future Directions in Variable Handling
Looking ahead, the handling of variables in Java will likely evolve as technology advances. Here are a few key considerations and potential trends:
- Increased Focus on Memory Efficiency: Applications demand more from memory management. Developers will need to hone their skills in optimizing variable usage, minimizing footprint while maintaining performance.
- Static and Dynamic Typing Trends: The rise of languages that support dynamic typing may influence Java's approach to variable declaration. Balancing type safety and flexibility will be an intriguing challenge for future iterations of the language.
- Higher-level Abstractions: As frameworks grow, there's a push towards abstracting variable management away from programmers. Understanding how variables work under the hood will remain vital to troubleshoot and optimize.
- Integration with Emerging Technologies: Variables will play an essential role in Artificial Intelligence and Machine Learning applications that leverage Java. Thus, a robust grasp on how to work with variables will be paramount.