Mastering the Core Concepts of Java Programming for Beginners and Intermediates
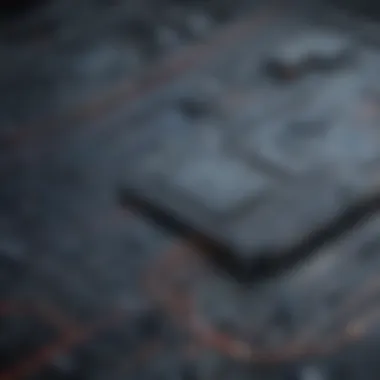
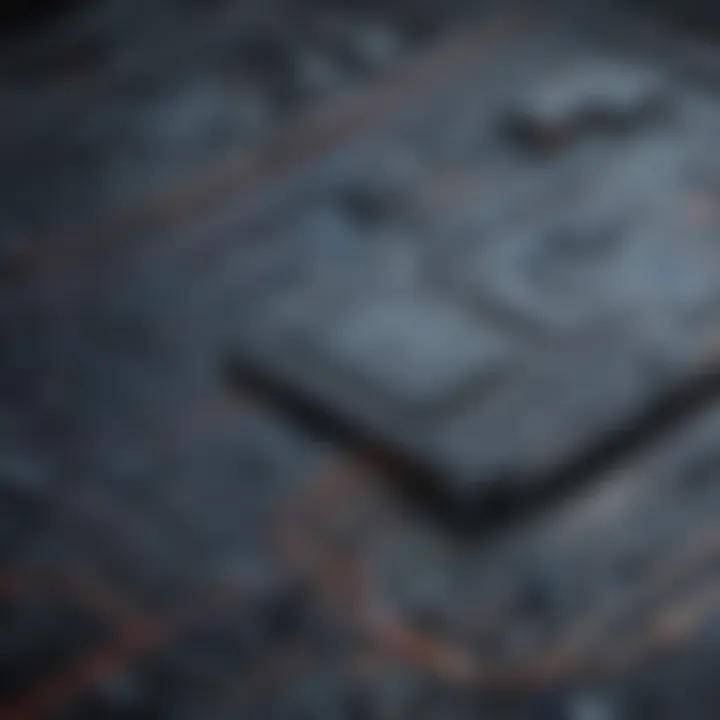
Introduction to Java Programming
Java is a versatile and robust programming language that has gained significant popularity and scope in the realm of software development. Understanding its history, background, features, and uses is crucial for anyone embarking on a programming journey.
History and Background
Originally developed by Sun Microsystems in 1995, Java was designed to be platform-independent, allowing code to run on any system with Java Virtual Machine (JVM) support. This feature revolutionized the world of software development, making Java a top choice for building diverse applications.
Features and Uses
Java is acclaimed for its simplicity, object-oriented structure, reliability, and excellent performance. It finds extensive applications in web development, mobile apps, enterprise systems, gaming, and more. Its versatility and scalability make it a desirable language in the tech industry.
Popularity and Scope
With a large community of developers worldwide, Java maintains its relevance in the evolving tech landscape. It serves as the foundation for numerous frameworks and libraries, contributing to its enduring popularity and wide range of applications.
Understanding Basic Syntax and Concepts
Diving into Java programming involves grasping key concepts such as variables, data types, operators, expressions, and control structures. Mastering these fundamental elements sets a strong foundation for writing efficient and meaningful code.
Variables and Data Types
In Java, variables are containers for storing data, each with a specific data type defining the kind of values it can hold. Understanding data types like integers, strings, and booleans is essential for manipulating information effectively within a program.
Operators and Expressions
Operators in Java enable various operations such as arithmetic, assignment, comparison, and logical evaluations. Learning how to use operators and construct expressions equips programmers with tools to perform complex calculations and decision-making processes.
Control Structures
Control structures like loops and conditional statements dictate the flow of a program. They allow for repetition, branching, and iteration, enabling programmers to create dynamic and responsive applications.
Exploring Advanced Topics
Delving deeper into Java unveils advanced topics like functions, methods, object-oriented programming (OOP), and exception handling. These topics elevate coding practices and empower developers to build sophisticated and resilient software.
Functions and Methods
Functions in Java encapsulate a set of actions that can be repeatedly executed within a program. Methods, on the other hand, are functions associated with objects in OOP, promoting code organization and reusability.
Object-Oriented Programming
Java is renowned for its support of OOP principles like inheritance, encapsulation, polymorphism, and abstraction. Leveraging these concepts enhances code modularity, flexibility, and scalability in software development.
Exception Handling
Exception handling in Java addresses error scenarios that may occur during program execution. By implementing robust error-handling mechanisms, developers can maintain code stability and gracefully manage unexpected situations.
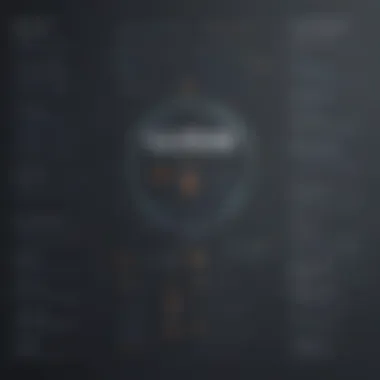
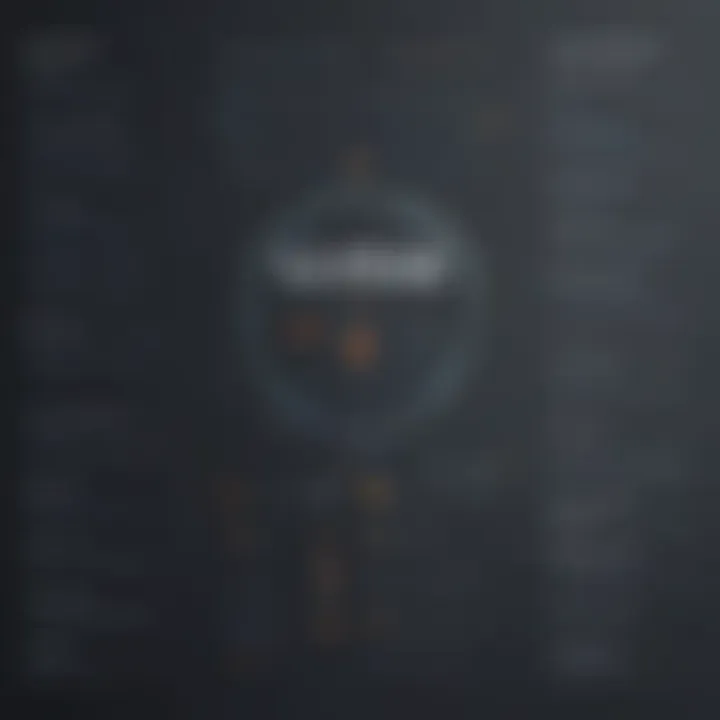
Practical Application with Hands-On Examples
Solidifying Java skills involves engaging in hands-on practice through simple programs, intermediate projects, and code snippets. Applying theoretical knowledge to practical scenarios fosters a deeper understanding and proficiency in Java programming.
Simple Programs
Creating basic programs allows learners to familiarize themselves with Java syntax and logic. Simple programs introduce foundational concepts like inputoutput, decision-making, and repetition, laying the groundwork for more complex projects.
Intermediate Projects
Undertaking intermediate projects involves combining various Java functionalities to solve real-world problems. These projects challenge developers to design efficient algorithms, implement algorithms, implement algorithms, implement data structures, data structures,etc ETC popular algorithms
Introduction to Java
Java, a renowned programming language, serves as the foundation for many software applications and platforms. In this comprehensive guide, we delve into the intricacies of Java to equip beginners and intermediate learners with essential skills. Understanding Java's syntax and core principles lays a solid groundwork for aspiring programmers.
History of Java
Origin and Evolution
The genesis and evolutionary path of Java symbolize innovation and adaptability in programming landscapes. Originating as a solution for consumer electronics, Java evolved to become a versatile language across diverse domains. Its portability and robustness distinguish Java as a preferred choice for developers, ensuring consistent performance across various platforms.
Key Contributors
The luminaries behind Java's development play a pivotal role in shaping its success. Key contributors such as James Gosling and Patrick Naughton infused vision and expertise into Java's evolution. Their collective efforts propelled Java's growth, establishing it as a prominent player in the programming realm. Recognizing the contributions of these individuals underscores the collaborative spirit that underpins Java's legacy.
Java Development Environment
Setting Up JDK
Configuring the Java Development Kit (JDK) marks the initial step towards Java proficiency. Setting up JDK involves installing essential tools and libraries to facilitate Java programming. Understanding JDK's role in compiling and running Java programs is crucial for seamless development processes. Navigating through JDK setup ensures a conducive environment for coding and experimentation.
Choosing an IDE
Selecting an Integrated Development Environment (IDE) is a strategic decision for Java practitioners. IDEs like IntelliJ IDEA and Eclipse offer comprehensive tools for coding, debugging, and testing Java applications. Evaluating factors such as interface customization, plugin support, and debugging capabilities aids in choosing an IDE that aligns with one's workflow. Opting for the right IDE enhances productivity and streamlines the development workflow for Java projects.
Basic Concepts in Java
Understanding the Basic Concepts in Java is pivotal for anyone looking to grasp the foundation of this programming language. It serves as the building blocks for more advanced topics, laying the groundwork for a profound understanding of Java programming. Delving into Variables and Data Types provides insights into how information is stored and manipulated, while exploring Operators and Expressions enhances logical and computational skills. Control Flow Statements offer a structured approach to decision-making within a program. Mastering these Basic Concepts in Java is essential for programmers at all levels to enhance their proficiency and maximize the potential of their Java projects.
Variables and Data Types
Primitive vs. Reference Types
Primitive vs. Reference Types stand as a fundamental concept in Java, influencing how data is stored and accessed within a program. Primitive types encompass basic data entities, such as integers and characters, stored directly in memory. On the other hand, Reference Types point to objects in memory, facilitating complex data structures. The key characteristic of Primitive vs. Reference Types lies in their memory allocation behavior, with primitives storing data directly and references pointing to object instances. Understanding this delineation is crucial for efficient memory management and optimized program performance.
Variable Declaration
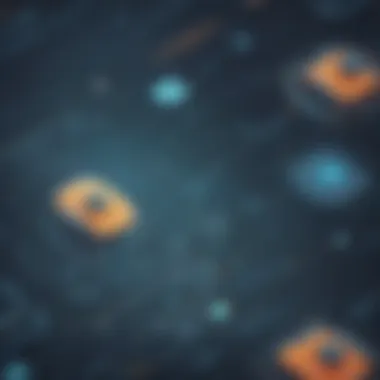
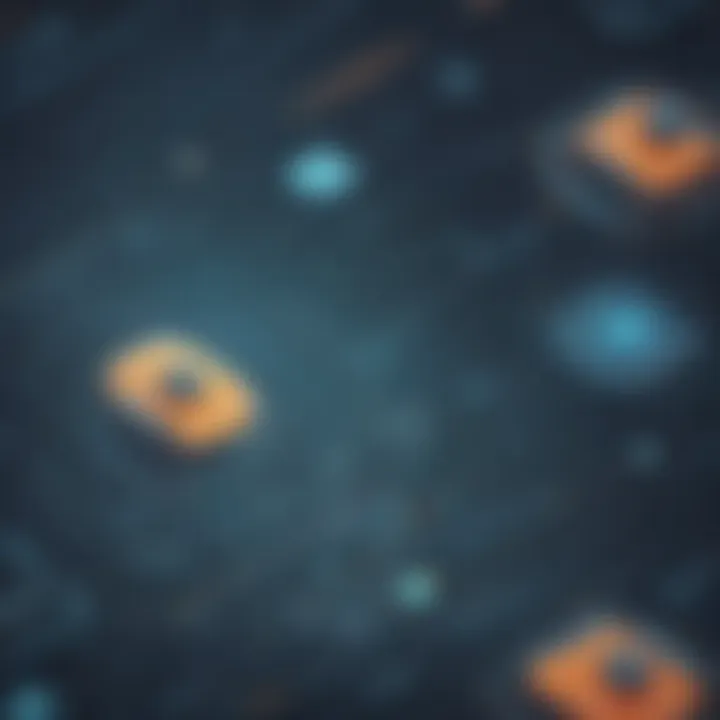
Variable Declaration equips programmers with the ability to define data entities within a Java program. By declaring variables, developers specify the type and name of the data being stored, allowing for better organization and utilization of information. The key characteristic of Variable Declaration is its role in memory allocation and type validation, ensuring data integrity during program execution. An advantage of Variable Declaration is its flexibility in accommodating various data types and enabling dynamic data manipulation. However, a potential disadvantage could arise from improper declaration leading to data inconsistencies and runtime errors within the program.
Operators and Expressions
Arithmetic Operators
Arithmetic Operators play a crucial role in performing mathematical calculations within Java programs. From addition to exponentiation, these operators facilitate numeric operations, enhancing the computational capabilities of a program. The key characteristic of Arithmetic Operators lies in their ability to manipulate numerical values efficiently and accurately, crucial for applications requiring precise calculations. While these operators offer computational precision, an inherent disadvantage may arise from potential data overflow or loss of precision in complex arithmetic operations, warranting careful usage and error handling.
Logical Operators
Logical Operators provide the framework for logical comparisons and decision-making in Java programming. With operators like AND, OR, and NOT, developers can establish conditional statements and control program flow based on logical evaluations. The key characteristic of Logical Operators is their role in enhancing program logic and enabling complex decision structures. Despite their benefits in streamlining program flow, an advantage of Logical Operators could turn into a disadvantage through intricate nesting of conditions, increasing code complexity and potentially reducing readability if not implemented judiciously.
Control Flow Statements
If-Else Statements
If-Else Statements offer a fundamental mechanism for implementing conditional logic in Java programs. By evaluating specified conditions, programmers can direct the program flow along different paths, enhancing interactivity and responsiveness. The key characteristic of If-Else Statements lies in their ability to enforce branching based on variable states, enabling dynamic program behavior. While this feature provides flexibility in decision-making, a potential drawback could arise from overly complex branching structures, leading to code redundancy and decreased maintainability.
Switch-Case Statements
Switch-Case Statements provide an alternative approach to multi-branching in Java programming, offering a concise way to evaluate a variable against multiple values. With a switch statement determining the control path based on the value of an expression, developers can streamline code readability and logic efficiency. The key characteristic of Switch-Case Statements is their suitability for scenarios with multiple branching options, simplifying decision-making processes within a program. However, care must be taken to avoid excessive nesting and ensure proper handling of cases to prevent logic errors and program inconsistencies.
Object-Oriented Programming in Java
Object-oriented programming in Java is crucial for understanding the principles that govern the structure and behavior of Java programs. By embracing the concept of objects and classes, developers can create modular, scalable, and reusable code, enhancing the efficiency and maintainability of their projects. Object-oriented programming not only fosters better organization of code but also promotes code reusability, inheritance, and polymorphism, making it integral to proficient Java development.
Classes and Objects
Defining Classes
Defining classes in Java allows programmers to encapsulate data and behavior into a single unit, facilitating code organization and modularity. A class defines the properties and methods that an object can exhibit, laying the foundation for object creation and manipulation. When designing classes, developers focus on attributes and behaviors that are intrinsic to a particular entity, leading to a more intuitive and structured program flow.
Creating Objects
Creating objects signifies the instantiation of a class, enabling the utilization of its defined attributes and methods. Objects serve as the tangible entities that interact within a Java program, allowing for data manipulation and task execution. Through object creation, developers can leverage class blueprints to spawn multiple instances, each capable of independent functioning and data manipulation, enhancing code reusability and efficiency.
Inheritance and Polymorphism
Class Inheritance
Class inheritance empowers developers to establish a hierarchical relationship between classes, enabling the propagation of attributes and methods from base classes to derived classes. This mechanism facilitates code reuse, minimizes redundancy, and promotes a structured approach to defining class hierarchies. By inheriting properties and behaviors, subclasses can extend and customize functionality, fostering adaptability and extensibility within the codebase.
Method Overriding
Method overriding allows subclasses to provide a specific implementation for inherited methods, tailoring functionality to suit individual class requirements. This flexibility enables developers to customize behavior while maintaining a consistent interface across related classes. By overriding methods, programmers can implement polymorphism, where objects of different classes can be treated uniformly based on their shared superclasses, enhancing code flexibility and adaptability.
Encapsulation and Abstraction
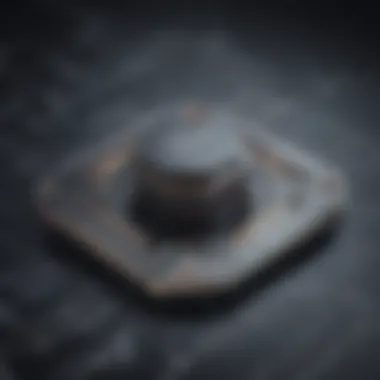
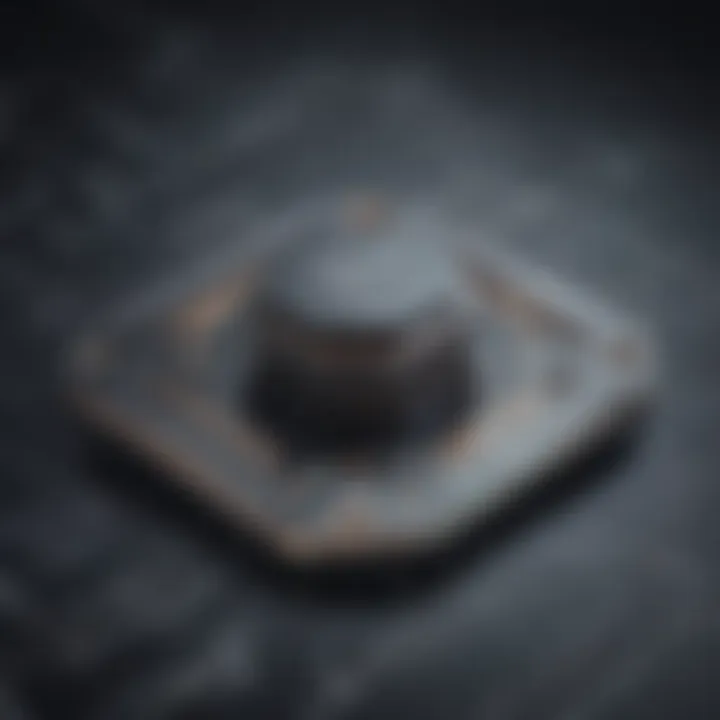
Access Modifiers
Access modifiers regulate the visibility and accessibility of classes, methods, and data within a Java program, promoting encapsulation and information hiding. These modifiers determine the level of exposure that class members have to external entities, enhancing code security and maintainability. By defining access levels, developers can control data integrity and prevent unauthorized access, reinforcing the robustness and integrity of their applications.
Abstract Classes
Abstract classes offer a blueprint for creating other classes, providing common methods and ensuring a cohesive structure among related classes. These classes cannot be instantiated on their own and may contain abstract methods that concrete subclasses must implement. By defining abstract classes, developers can establish a template for future class implementations, enforcing consistency and facilitating code reusability and extensibility.
Exception Handling
Exception handling in Java is a crucial concept that plays a vital role in developing robust and reliable software applications. By effectively managing exceptions, programmers can anticipate and address potential errors or unexpected behaviors during runtime. This proactive approach not only enhances the overall stability of the application but also improves its maintainability and user experience. Exception handling empowers developers to gracefully handle runtime errors, preventing abrupt termination of the program and enabling smoother execution.
Try-Catch Blocks
Handling Exceptions:
Handling exceptions within try-catch blocks allows developers to isolate and manage specific types of errors that may occur during program execution. By enclosing potentially error-prone code within a try block and specifying appropriate catch blocks to handle different exceptions, programmers can enforce customized error-handling logic. This granular control not only enables precise identification and resolution of issues but also enhances code readability and fault tolerance. Handling exceptions strategically within try-catch blocks is a best practice that reinforces the reliability and resilience of Java applications.
Throwing Exceptions:
Throwing exceptions in Java is a mechanism through which programmers can explicitly signal and propagate errors to higher levels of the program. By using the throw keyword, developers can trigger specific exceptions based on defined conditions or validation checks, allowing for seamless error propagation and centralized handling. Throwing exceptions facilitates clear communication of exceptional scenarios and promotes structured error management within Java applications. Despite the added complexity of exception propagation, throwing exceptions contributes to a more robust and predictable software behavior.
Checked vs. Unchecked Exceptions
Differences and Use Cases:
Differentiating between checked and unchecked exceptions is pivotal in Java exception handling. Checked exceptions, which must be either caught or declared in the method signature, enforce compile-time error checking, ensuring explicit exception handling by developers. In contrast, unchecked exceptions do not require explicit handling, providing flexibility but also necessitating cautious error monitoring during runtime. Understanding the distinctions between checked and unchecked exceptions guides developers in selecting appropriate exception types based on the context and criticality of potential errors, optimizing code clarity and reliability.
Best Practices:
Adhering to best practices in exception handling fosters code consistency and reliability in Java development. Embracing practices such as logging exceptions, providing informative error messages, and utilizing exception hierarchies enhances error traceability and expedited issue resolution. By establishing consistent exception handling patterns and error reporting mechanisms, developers can streamline debugging processes and fortify the resilience of their Java applications. Incorporating best practices into exception handling routines elevates the overall quality and maintainability of the codebase, fostering a robust programming environment.
Java Collections Framework
In the vast realm of Java programming, the Java Collections Framework stands out as a pivotal area that warrants meticulous attention. This segment is crucial in handling and manipulating groups of objects, offering a structured approach to data organization. By delving into the Java Collections Framework, readers will grasp the intricacies of managing data structures efficiently and effectively. Understanding this framework is paramount for developers seeking to optimize their code and enhance overall performance.
Lists and Sets
ArrayList
When it comes to the Java Collections Framework, the ArrayList plays a significant role in facilitating the storage and retrieval of data elements. As a dynamic array, the ArrayList boasts the capability to resize itself dynamically, providing flexibility in managing collections of varying sizes. Its key characteristic lies in its ability to store elements sequentially and access them through index-based retrieval, showcasing a convenient and efficient data structure. The ArrayList's popularity stems from its versatility and ease of use, making it a preferred choice for numerous programming tasks. Despite its advantages, developers must also consider potential drawbacks such as slower performance with frequent insertions and deletions.
HashSet
Within the realm of Sets in the Java Collections Framework, the HashSet holds prominence for its unique characteristics and functionalities. Serving as an implementation of a Set interface, HashSet offers high-speed performance in storing and retrieving elements. Its key characteristic lies in its ability to maintain a collection of unique elements, eliminating duplicates effortlessly. This feature makes HashSet an ideal choice for scenarios where uniqueness of elements is crucial. Developers value HashSet for its efficiency in checking for element existence and ensuring data integrity. However, users should be mindful of HashSet's unordered nature, which may impact specific use cases.
Maps and Queues
HashMap
In the landscape of Java Collections, HashMap emerges as a fundamental component that revolutionizes data management through key-value pairs. This data structure enables rapid retrieval of data based on unique keys, enhancing efficiency in organizing and accessing information. HashMap's key characteristic involves its hashing function, which enables quick indexing and retrieval of values, making it a popular option for various programming tasks. Developers appreciate HashMap for its speed and versatility in storing and retrieving key-value pairs swiftly. While HashMap excels in performance, individuals should be cautious about handling collisions that may impact its efficiency in specific contexts.
PriorityQueue
Introducing PriorityQueue, a critical element in the Queue segment of the Java Collections Framework renowned for its prioritized data retrieval mechanism. PriorityQueue distinguishes itself by allowing elements to be stored based on a defined priority order, ensuring that the highest priority element is readily accessible. Its key characteristic lies in maintaining the highest priority element at the forefront, enabling swift retrieval operations. PriorityQueue proves advantageous in scenarios requiring the processing of elements based on specific criteria. Nonetheless, developers must consider potential disadvantages like increased complexity in maintaining the priority order, which may influence its usability in certain scenarios.