In-Depth Exploration of Java Classes: Understanding Object-Oriented Programming
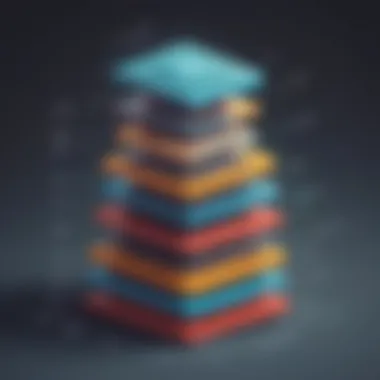
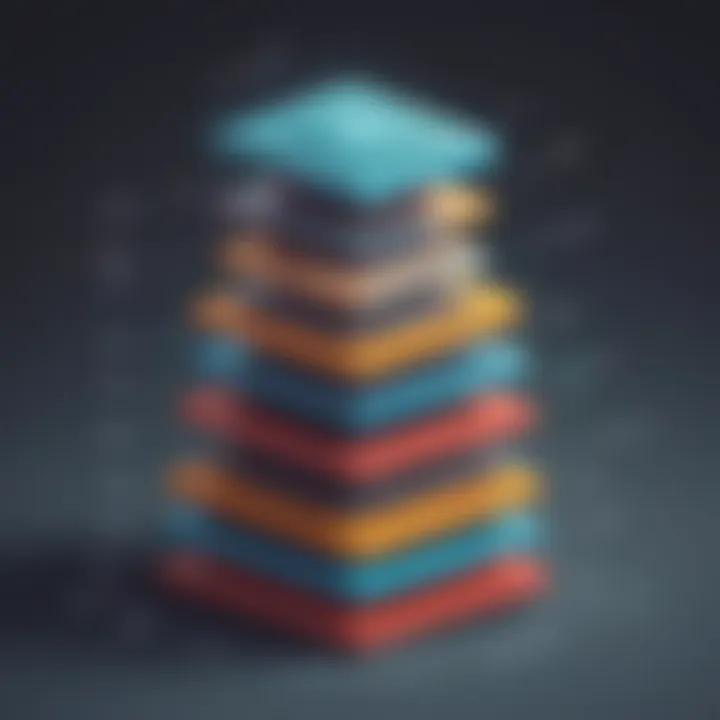
Resources and Further Learning
- Delving deeper into resources and further learning opportunities can expand your knowledge and expertise in Java programming, keeping you updated with the latest trends and techniques in the field. Recommended books and tutorials offer comprehensive insights into Java fundamentals, advanced topics, and best practices. By exploring renowned Java books such as 'Effective Java' by Joshua Bloch or online tutorials on platforms like Udemy and Coursera, you can enhance your learning experience and gain a deeper understanding of Java concepts and applications.
- Online courses and platforms provide interactive learning environments to sharpen your Java skills, engage with industry experts, and collaborate with fellow learners. Platforms like Codecademy, edX, and HackerRank offer Java courses ranging from beginner to advanced levels, catering to diverse learning needs and preferences. Community forums and groups establish a supportive network where Java enthusiasts can seek advice, share knowledge, and collaborate on projects, fostering a vibrant community of learners and practitioners passionate about mastering the art of Java programming.
Introduction to Classes in Java
In the realm of Java programming, understanding classes forms the cornerstone of object-oriented programming (OOP) paradigms. Classes serve as the blueprint for creating objects, encapsulating attributes and behaviors. Delving into the intricacies of classes in Java provides a foundational understanding essential for honing one's coding skills. Beginners and intermediate learners stand to benefit significantly from grasping the nuances of class structures, syntax, and functionality, paving the way for more robust and efficient code implementation.
Definition of Classes
Understanding the concept of classes
Unveiling the essence of classes in Java sheds light on how objects are instantiated and interact within a program. Fundamentally, classes encapsulate data (attributes) and methods (behaviors), fostering code reusability and organization. By grasping the concept of classes, developers gain mastery over creating modular and scalable code structures, integral to maintaining code integrity and fostering code maintainability.
Role of classes in Java programming
Classes in Java programming play a pivotal role in facilitating code organization, enhancing code readability, and promoting code reusability. The class acts as a blueprint from which objects are created, offering a clear hierarchy to navigate program functionalities. Understanding the role of classes in Java programming equips developers with the necessary tools to design robust and efficient software systems, essential for catering to complex programming requirements.
Creating a Class
Syntax for defining a class
The syntax for defining a class in Java follows a structured format, comprising class keywords, class names, and access specifiers. This syntax delineates the structure of a class, including variables, constructors, and methods, crucial for fostering clear code organization and defining object behavior. Understanding the syntax for defining a class is imperative for laying the foundation of object instantiation and manipulation within a Java program.
Naming conventions for classes
Follows a comprehensive naming convention for classes is pivotal to ensure code consistency, readability, and maintenance. By adhering to industry best practices regarding class naming, developers mitigate potential naming conflicts, enhance code comprehensibility, and streamline collaborative coding efforts. The importance of naming conventions for classes lies in establishing a standardized approach to class naming, contributing to code clarity and overall code quality.
Class Members
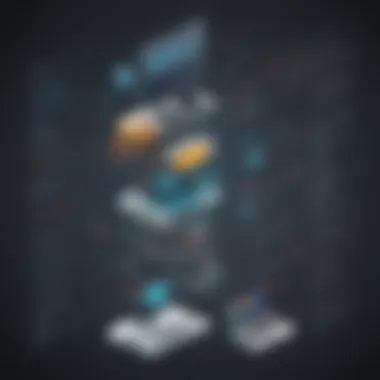
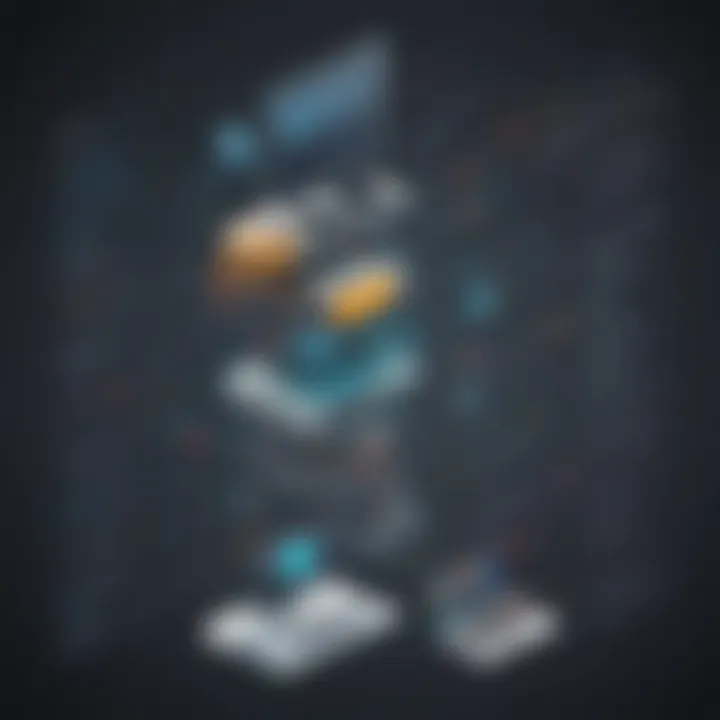
Fields or variables in a class
Fields or variables within a class store object state, encapsulating data pertinent to object instances. They serve as the building blocks for defining object attributes, helping instantiate objects with specific characteristics and properties. Understanding the role of fields or variables in a class empowers developers to model real-world entities effectively, ensuring precise data representation and manipulation within a Java program.
Methods within a class
Methods within a class encapsulate object behaviors, defining actions and operations that objects can perform. These functions enable developers to implement specific functionalities, fostering code modularity and reusability. Mastering the utilization of methods within a class equips developers with the ability to design intricate program logic, cater to diverse use cases, and enhance code efficiency, pivotal for developing robust and scalable software solutions.
Class Relationships
In the realm of Java programming, understanding Class Relationships is paramount. It forms the backbone of object-oriented programming, delineating how classes interact and relate to each other. Grasping Class Relationships aids in building scalable and maintainable code, fostering reusability and modularity within software development projects. By comprehending the hierarchy of classes, developers can streamline their code structure, enhance code organization, and facilitate efficient collaboration among team members. Moreover, delving into Class Relationships paves the way for implementing advanced concepts like inheritance, polymorphism, and encapsulation, all of which are pivotal in crafting robust and sophisticated Java applications.
Inheritance
Concept of inheritance in Java
The Concept of inheritance in Java embodies a fundamental principle of object-oriented programming, allowing classes to inherit attributes and behaviors from parent classes. This mechanism promotes code reusability, enabling developers to create a hierarchical structure of classes where child classes can access and extend functionalities defined in their superclass. Inheritance facilitates the establishment of an 'is-a' relationship between classes, enhancing code modularity and reducing redundancy. By leveraging inheritance, developers can enhance code readability, promote efficient maintenance, and implement common functionalities at higher abstraction levels.
Extending classes and superclasses
Extending classes and superclasses in Java exemplifies the extension of functionality from a superclass to a subclass. This mechanism enables developers to add specialized features to existing classes without altering their core structure, fostering code scalability and flexibility. By extending classes and superclasses, developers can create a robust class hierarchy, promoting code reusability and minimizing code replication. This approach empowers developers to enhance code modularity, facilitate future enhancements, and establish a clear and organized class structure, supporting the evolution of complex Java applications.
Polymorphism
Understanding polymorphic behavior
The essence of polymorphic behavior lies in the ability of objects to exhibit different forms based on their context. In Java, polymorphism allows objects of different classes to be treated uniformly through method overriding and method overloading. This feature enhances code flexibility, facilitating the implementation of diverse behaviors without altering existing code structures. Understanding polymorphic behavior opens avenues for creating adaptable and extensible code, promoting code reusability, and simplifying code maintenance. By embracing polymorphism, developers can design versatile and dynamic applications, laying the foundation for robust and efficient Java programming practices.
Method overriding and method overloading
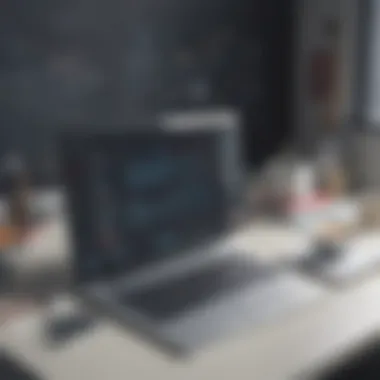
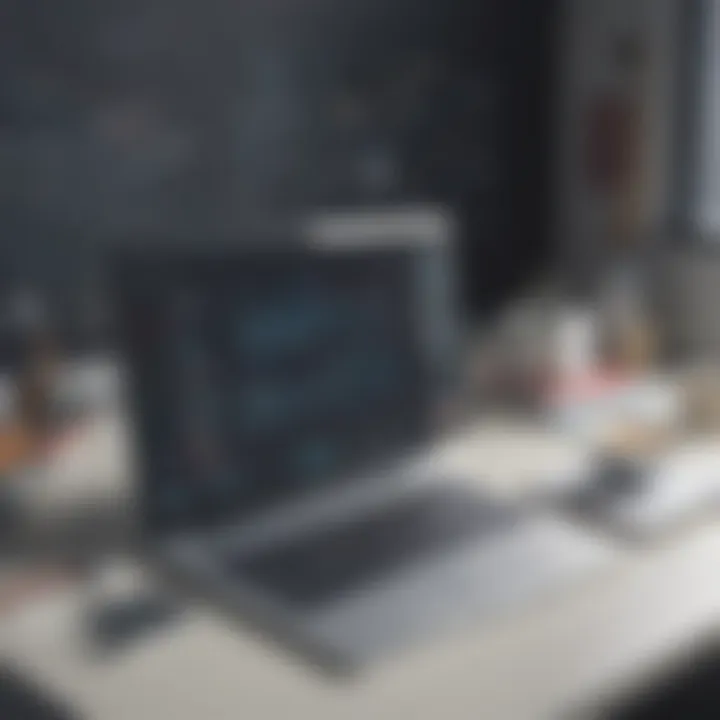
Method overriding and method overloading in Java encapsulate the concepts of dynamic polymorphism, enabling developers to redefine superclass methods in subclasses and define multiple methods with the same name but different parameters, respectively. These features enhance code modularity, facilitate code customization, and foster extensibility within Java applications. By implementing method overriding and method overloading, developers can tailor functionality to specific requirements, promote code adaptability, and enhance code readability. This approach empowers developers to design expressive and scalable code, catering to varying application needs and evolving programming paradigms.
Encapsulation
Data hiding and access modifiers
Data hiding and access modifiers encapsulate private data within classes, restricting direct access and manipulation from external entities. This practice enhances data security, promotes information hiding, and prevents unauthorized modifications, fostering code integrity and stability. By incorporating data hiding and access modifiers, developers can enforce data encapsulation, mitigate potential security vulnerabilities, and enhance code maintainability. This approach ensures data confidentiality, safeguarding critical information within Java classes and fortifying the robustness of software applications.
Encapsulation for data security
Encapsulation for data security fortifies Java applications by encapsulating data within classes and providing controlled access through defined interfaces. This practice enhances information security, facilitates controlled data sharing, and safeguards sensitive information from unauthorized access. By embracing encapsulation for data security, developers can fortify data protection measures, enhance data confidentiality, and mitigate risks associated with data exposure. This approach reinforces the resilience and reliability of Java applications, instilling trust and confidence among users while upholding data privacy and security standards.
Advanced Concepts in Java Classes
In the realm of Java programming, delving into advanced concepts is paramount for any aspiring coder. Understanding abstract classes and interfaces is a crucial step towards mastering the intricacies of object-oriented programming. Abstract classes provide a blueprint for other classes to inherit and implement, fostering code reusability and structure. On the other hand, interfaces act as contracts, defining a set of methods that must be implemented by classes that utilize them. Embracing these concepts elevates the flexibility and scalability of Java code, enabling developers to build robust systems with ease.
Defining abstract classes
When defining abstract classes, programmers designate them as templates that cannot be instantiated on their own. These classes may contain abstract methods, which are meant to be implemented by subclasses inheriting from them. The beauty of abstract classes lies in their ability to enforce a structure across related classes while allowing flexibility in method implementations. By utilizing abstract classes, developers can streamline their code architecture and promote a clear hierarchy among classes.
Implementing interfaces in Java
Implementing interfaces in Java is a powerful mechanism for achieving multiple inheritances and fostering code consistency. Interfaces define a contract that classes can subscribe to, ensuring that specific methods are implemented. By incorporating interfaces, developers can design code that is highly adaptable and modular, facilitating easier maintenance and extensibility. Leveraging interfaces alongside classes enhances the overall organization and coherence of Java programs, promoting good coding practices and enhancing overall code quality.
Static Members in Classes
Static variables and methods play a crucial role in Java programming, offering a shared memory space that is accessible across all instances of a class. Understanding static elements is vital for optimizing memory usage and achieving efficient code functionality. Static variables retain their values across instances, making them ideal for constants or variables shared among all objects. Likewise, static methods do not operate on a specific instance but contribute to the class as a whole, offering utility functions and behaviors that can be accessed without instantiation.
Understanding static variables and methods
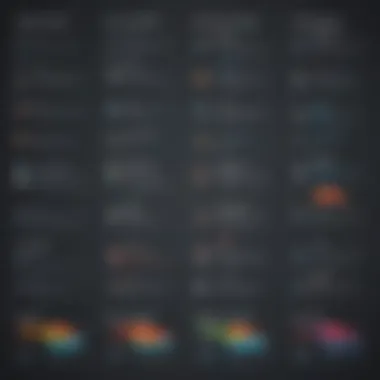
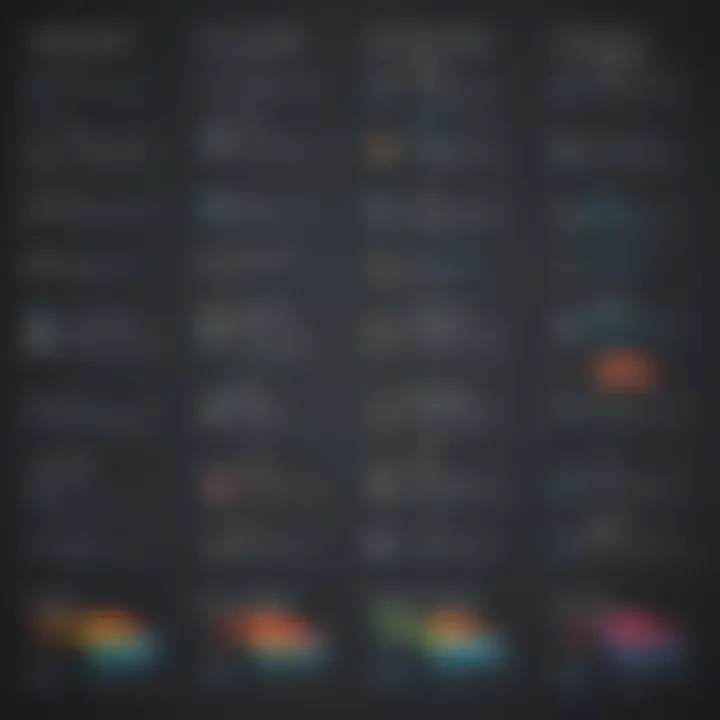
Static variables and methods are declared using the \
Best Practices for Class Design
In the realm of Java programming, adhering to best practices for class design is paramount for creating efficient and maintainable code. It involves meticulously crafting the structure and functionality of classes to optimize their performance and ensure scalability. By following established standards and guidelines, developers can enhance code quality, readability, and reusability, laying a solid foundation for robust software development. Embracing best practices for class design fosters modularity, encapsulation, and abstraction, facilitating easier troubleshooting and modifications in the future.
Class Composition
Building classes through composition
Building classes through composition is a fundamental concept in Java that emphasizes constructing complex objects by combining simpler ones. This approach promotes code reusability, flexibility, and segregation of concerns, enabling developers to create more modular and scalable systems. By composing classes, developers can achieve a higher level of abstraction, reducing dependencies and promoting a clearer separation of responsibilities within the codebase. While this design pattern enhances maintainability and extensibility, it may introduce added complexity in managing relationships between classes, requiring careful structuring and documentation to ensure clarity and coherence.
Relationship between classes
The relationship between classes defines how different classes interact and collaborate within a software system. Establishing clear relationships is crucial for defining the flow of data and behavior between objects, facilitating a cohesive and well-structured application architecture. By defining relationships such as associations, aggregations, or dependencies, developers can model real-world scenarios effectively, enhancing the readability and maintainability of the codebase. While well-defined relationships promote code clarity and modularization, improper design choices can lead to tight coupling and potential cascading changes across the system, underscoring the importance of thoughtful class relationships in software design.
Single Responsibility Principle
Designing classes with a single purpose
The Single Responsibility Principle (SRP) advocates for designing classes that have a singular, well-defined purpose or responsibility within a program. This principle aims to enhance code readability, maintenance, and testability by restricting each class to a distinct functionality or behavior. By adhering to SRP, developers can create more concise, focused, and coherent classes that are easier to understand and troubleshoot. Designing classes with a single responsibility simplifies code management, promotes code reuse, and reduces the risk of introducing bugs or unintended side effects during development.
Benefits of adhering to SRP
Adhering to the Single Responsibility Principle offers numerous benefits, including improved code organization, increased reusability, and enhanced flexibility in software design. By structuring classes based on specific responsibilities, developers can isolate changes, promote code scalability, and streamline collaboration among team members. Additionally, adhering to SRP facilitates unit testing, code refactoring, and overall code maintainability, leading to more stable and robust software solutions. Embracing SRP encourages a modular and cohesive approach to class design, fostering code quality and long-term sustainability in Java projects.
Effective Class Naming
Guidelines for naming classes
Naming classes effectively is a critical aspect of class design, influencing code clarity, consistency, and understandability. Choosing descriptive and meaningful names for classes communicates their purpose and functionality, aiding developers in comprehending the role of each component within the software system. Following naming conventions such as using camelCase or PascalCase, avoiding abbreviations, and selecting precise terminology can enhance code readability and maintainability. Guidelines for naming classes should prioritize clarity over brevity, ensuring that class names convey their intended use accurately and concisely.
Naming conventions for readability
Utilizing consistent naming conventions for readability promotes uniformity and coherence across a codebase, fostering collaboration and comprehension among developers. Establishing naming conventions for variables, methods, and classes streamlines the development process, reduces cognitive overhead, and enhances code consistency. By adhering to conventions such as prefixing interfaces with 'I' or suffixing classes with 'Impl,' developers can create a standardized naming scheme that facilitates code navigation and reduces ambiguity. Naming conventions for readability serve as a cornerstone of effective class design, promoting clarity, maintainability, and scalability in Java projects.