Exploring Essential Data Structures for Programming
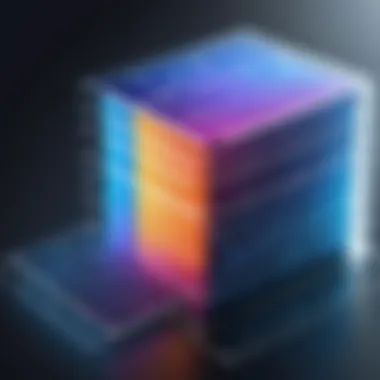
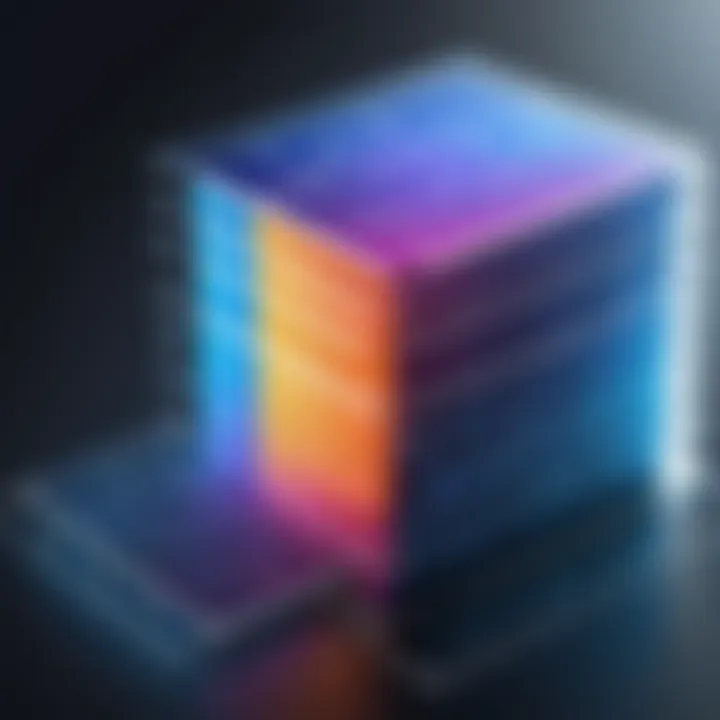
Intro
In the realm of programming and computer science, understanding data structures is not just a nice-to-have; it's a must. Data structures are the building blocks of efficient data manipulation and organization. Without a solid grasp of these concepts, coding can be a bit like trying to find your way in a fog without a map. Each type of data structure serves different purposes, has varied applications, and comes with its own set of strengths and limitations.
This guide aims to unravel the intricate tapestry of data structures, from basic arrays to more complex graphs and trees. By exploring each category in depth, you’ll not only get to know what they are but also understand when and why to use them. Let’s buckle up as we embark on this enlightening journey through the essentials of data structures in programming.
Understanding Data Structures
Data structures can be seen as specialized formats for organizing, processing, and storing data. The choice of a particular type impacts efficiency and ease of use in various programming scenarios.
Common Data Structures
It's crucial to know the core data structures widely used across programming languages:
- Arrays: Fixed-size sequences of elements, allowing for indexed access.
- Linked Lists: A sequence of elements where each element points to the next.
- Stacks: A last-in, first-out structure, perfect for scenarios like undo functionality in applications.
- Queues: First-in, first-out structures used in scenarios like ordering systems.
- Trees: Hierarchical structures useful for database indexing.
- Graphs: Structures used to model pairwise relationships, ideal for navigation systems and social networks.
Understanding the differences between these structures can save time and resources.
Key Considerations in Choosing a Data Structure
When selecting a data structure, consider factors like:
- Efficiency: How fast can you insert, delete, or access data?
- Memory Usage: How much memory is consumed?
- Usage Patterns: What operations will frequently be performed?
Practical Applications
Each data structure has its niche application, and knowing where to apply them can be a game-changer.
Arrays
Arrays are often used for storing data elements of the same type. In languages like Java, an array can be declared as follows:
This facilitates efficient indexing operations.
Linked Lists
Linked lists come into play when you need a dynamically resizable structure. For instance, to add an element, you might do:
This defines a node, allowing for flexible memory usage.
Trees and Graphs
Trees are utilized in applications requiring hierarchical sorting, like file organization. Graphs find their place in route planning and network design.
Ending
Understanding data structures is fundamental for writing efficient code. As we've outlined, each structure offers unique benefits and constraints. Mastering these will not only boost your programming proficiency but will also pave the way for effective problem-solving in real-world applications.
Particularly, learning how to leverage these structures can turn mundane coding tasks into streamlined, efficient operations. As we move into further sections, we will dig deeper into the details and applications of these data structures. Stay tuned!
Understanding Data Structures
The realm of programming is vast and intricate, filled with concepts that can make or break an application. One such cornerstone of computer science is the understanding of data structures. They serve as the building blocks for constructing programs, enabling efficient storage, retrieval, and manipulation of data. By grasping the intricacies of data structures, programmers not only enhance their coding prowess but also bolster the performance of their applications.
Defining Data Structures
At its core, a data structure is a specialized format for organizing and storing data in a computer. Just like a filing cabinet holds documents in a structured manner, data structures keep information organized so that it can be accessed and modified with ease. Essentially, there are two major categories: primitive and non-primitive.
- Primitive data structures include simple types like integers, booleans, and characters. They are the foundation, the raw materials from which more complex structures are formed.
- Non-primitive data structures can be further broken down into linear and non-linear structures. Linear structures, such as arrays and linked lists, arrange data in a sequential manner, while non-linear structures like trees and graphs organize data in a more complex hierarchy.
Understanding these definitions provides insight into how data operates within a computer system. You could think of it as a language; once you know the vocabulary and grammar, you can effectively communicate and build functional software.
Importance in Programming
The significance of data structures in programming cannot be overstated. They are pivotal for:
- Efficient Data Management: A well-chosen data structure allows for agile data manipulation, reducing the time taken for search, insert, or delete operations. For instance, if a programmer utilizes a hash table for lookups, they can retrieve information in constant time on average, whereas an array may require linear time.
- Optimal Performance: Performance often hinges on the underlying data structure. Using trees for hierarchical data can be significantly quicker than relying on arrays, especially when the data grows large.
- Scalability: As projects evolve and the amount of data increases, understanding data structures helps in scaling applications effectively. For example, when managing user data for a rapidly growing social media app, an efficient implementation of a graph could hold relationships among users better than a simple list.
- Resource Management: Different structures utilize memory differently. Knowing this can help programmers write leaner code, which can lead to cost savings in deployment, especially in cloud environments.
"Choosing the right data structure is like choosing the right tool for a job—each one has its strengths and weaknesses, and the wrong choice could turn a simple task into a nightmare."
In summary, a deep comprehension of data structures equips programmers with the ability to make informed decisions about how to manage and process data effectively. This knowledge is not simply academic; it's practical and crucial for anyone stepping into the coding world.
Types of Data Structures
Understanding the different types of data structures is crucial for anyone diving into programming. Each kind serves a specific purpose and comes with its own set of features, advantages, and drawbacks. As we break it down into primitive and non-primitive categories, it becomes clearer why knowing these distinctions is essential.
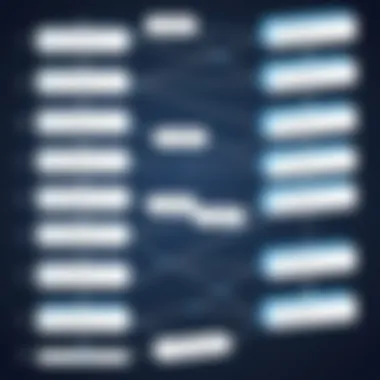
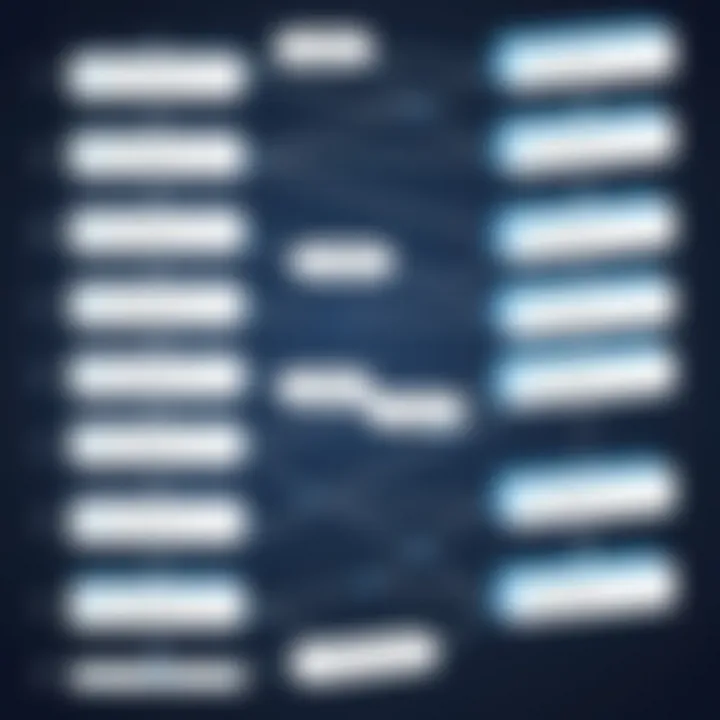
When you consider how you want to organize your data, the choice of structure can drastically affect efficiency and ease of implementation. For instance, using an inappropriate structure can lead to sluggish performance, bugs, and a tangled mess that nobody wants to deal with. Thus, a strong foundation in types of data structures allows you to choose the right tools for the job, leading to cleaner code and quicker applications.
Primitive Data Structures
Primitive data structures are the simplest forms of data handling that you can find in any programming language. These are the building blocks that form the basis for more complex structures. Examples include integers, floats, characters, and booleans. While they may seem basic, these structures are fundamental for any operation, as they help in storing simple values directly within variables.
A key characteristic of primitive data structures is their fixed size in memory, which means they are lightweight and easy to work with. This becomes especially significant when you consider performance and memory usage in, for instance, embedded systems or applications where every byte counts. By utilizing primitive types, programmers can keep their applications efficient and responsive.
In practice, you would typically use these structures for mathematical operations, logical comparisons, or when a program needs to store simple values. For example, a boolean type might be used to control flow in your code, determining which path to follow based on a true/false condition.
Non-Primitive Data Structures
Unlike primitive structures, non-primitive data structures allow you to handle complex data relationships and hierarchies. These can be categorized further into linear and non-linear structures. They expand your toolkit for organizing, retrieving, and manipulating data in more sophisticated ways than what primatives can manage alone.
Linear Structures
Let’s start with linear structures. This category includes arrays and linked lists, where elements are organized in a sequential order. One of the defining features of linear structures is simplicity in access, as they allow easy traversal of elements.
For instance, an array provides quick access to its elements via an index, which makes it a favorite for operations that require frequent data retrieval. However, while they offer this speed, their fixed size can sometimes be a hurdle. You can't change the size of an array once it's declared, which can lead to wasted space if you allocate too much or inadequate storage if you've underestimated your needs.
The major advantage here is the ease of iteration. With a double-ended queue (deque), accessing both ends becomes straightforward, making operations like adding or removing items efficient, which is a crucial feature in many algorithms.
Non-Linear Structures
In contrast, non-linear structures like trees and graphs don't follow a sequential pattern. Instead, they offer a way to model relationships between various entities. Their uniqueness lies in how they can represent data that branches out in different directions, making them exceptionally suited for various applications.
One key characteristic of non-linear structures is their hierarchical organization. A binary tree, for example, organizes data in a parent-child relationship, making searches faster and allowing for better data dynamics in specific contexts. While trees facilitate speedy data searching, they can become complex with unbalanced structures which can slow down operations like lookups and insertions.
Graphs, on the other hand, are incredibly versatile and are used in everything from social networks to map applications. They’re built of nodes and edges, and their capacity for complexity is unmatched. However, they also require more memory and can be troublesome to implement due to their intricate relationships.
Ending
Each type of data structure, be it linear or non-linear, primitive or non-primitive, holds unique qualities that can be leveraged in programming to achieve various tasks efficiently. Understanding these structures allows developers to build algorithms that not only perform well but also solve real-world problems systematically.
Knowing when to implement each type is where the true art of programming lies.
Linear Data Structures
Linear data structures play a crucial role in the world of programming and data management. Understanding their characteristics, applications, and benefits lays the groundwork for becoming proficient in coding. These structures are designed to store data in a sequential manner, where elements are arranged in a linear order. This implies that each item in the structure is connected to its preceding and succeeding element, which helps in maintaining organization.
When dealing with linear data structures, programmers can expect some specific benefits, such as ease of implementation and straightforward memory management. However, they also come with their considerations, such as potential limitations in efficiency for certain operations compared to more complex structures.
In this section, we will delve into two primary forms of linear data structures: arrays and linked lists. Each has its own distinctive features and use cases that can differ significantly, thus influencing the choice of which to employ in a given context.
Arrays
Definition and Characteristics
An array is a collection of elements, all of the same type, stored in contiguous memory locations. This layout allows for efficient access and manipulation of data since you can directly address any element using its index. One of the standout characteristics of arrays is their ability to provide immediate access to their elements, making operations like retrieval and updating particularly swift. Because of their straightforward nature, arrays are a popular choice in programming.
However, arrays have their limitations. For instance, they have a fixed size which means once they are created, it’s not easy to adjust their capacity without creating a new array and copying the data. This could lead to inefficient memory usage in scenarios where data size fluctuates frequently.
Usage and Applications
Arrays find their footing in a wide range of applications. They are often used in algorithms that require quick data access, such as searching and sorting algorithms. They can hold collections of data like numbers, strings, or any other objects that need to be processed in order.
For example, if a developer is working on implementing a sorting algorithm like Quick Sort or Merge Sort, arrays become invaluable tools, as sorting operations rely heavily on index-based access and manipulation. Despite their limitations, the performance benefits that arrays provide in specific applications make them indispensable in programming.
Linked Lists
Types of Linked Lists
Linked lists are another form of linear data structure, distinguished by their dynamic size and ease of element insertion and deletion. The basic principle is that every element, often referred to as a node, contains the data and a reference (or link) to the next node in the sequence. There are several types of linked lists, including singly linked lists, doubly linked lists, and circular linked lists.
- Singly Linked List: Contains nodes that point to the next node only, making traversal straightforward but somewhat limiting in backward navigation.
- Doubly Linked List: Comes equipped with pointers to both previous and next nodes, offering the flexibility of bidirectional traversal.
- Circular Linked List: In this structure, the last node points back to the first node, forming a circle that can be particularly useful for implementing certain types of queues or buffers.
Each type has its own unique features that can greatly influence their use cases, often depending on the requirements of the task at hand.
Benefits and Drawbacks
When discussing the benefits of linked lists, one must consider their flexible sizing. Since they allow dynamic memory allocation, they don’t suffer from the rigidity that arrays do with fixed capacities. Moreover, their structure allows for efficient insertions and deletions, which is a strong advantage when developing applications that require frequent updates.
On the flip side, linked lists come with certain drawbacks that may impact performance negatively. For instance, because elements are not stored in contiguous memory locations, accessing elements takes longer as it may require traversing multiple nodes. This can lead to efficiency concerns in situations requiring random access.
Non-Linear Data Structures
Non-linear data structures are an essential part of the larger landscape of data organization, particularly when we deal with complex relationships among data elements. Unlike linear data structures, where data elements are arranged sequentially, non-linear structures allow for a more intricate portrayal of interconnections. This feature leads to greater efficiency in various applications, especially in fields like computer science, data analysis, and artificial intelligence.
Understanding the unique characteristics of non-linear data structures can offer several significant benefits:
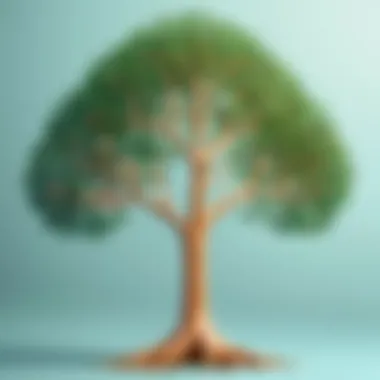
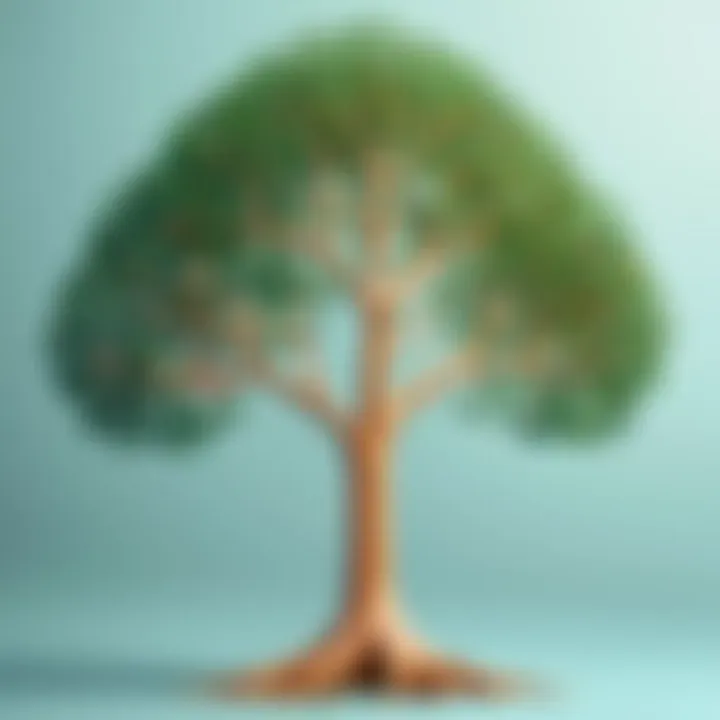
- Hierarchical Representation: Structures like trees facilitate a parent-child relationship, making them ideal for representing hierarchical data such as organizational charts or file systems.
- Adaptability: Non-linear approaches, such as graphs, allow for varied connections, which are suited for applications like social networks or network routing.
These data structures demand a thorough understanding because they play a critical role in optimizing performance for specific computing problems.
Trees
Binary Trees
Binary trees are a type of tree structure where each node has at most two children. This characteristic makes them a fundamental structure for various applications, including expression evaluation and data searching. One key aspect of binary trees is their efficiency in performing operations, such as insertion, deletion, and traversal.
The balanced variety of binary trees, like AVL or Red-Black trees, helps maintain performance even as datasets grow large. Their structure permits faster search times compared to other complex structures, keeping operations around O(log n) under optimal conditions.
However, binary trees are not without their pitfalls. If not managed properly, they can become unbalanced, leading to performance degradation. Regular maintenance and rebalancing are crucial to leveraging the strengths of binary trees properly.
Traversals and Operations
Traversal methods, such as in-order, pre-order, and post-order traversals, are fundamental in processing and accessing elements in binary trees. Each traversal has its own characteristics and use cases. In-order traversal, for example, is particularly advantageous when the goal is to retrieve data in a sorted order, as it visits the left subtree, then the node itself, followed by the right subtree.
One unique feature of these traversal algorithms is their ability to operate recursively or iteratively. This flexibility allows programmers to choose an approach that best suits their application, whether it be constrained by stack space or requiring greater performance.
While traversals offer numerous advantages, they can also introduce complexity in implementation. Mismanaging recursive calls could lead to stack overflow, particularly in large trees, so proper understanding and planning is essential.
Graphs
Directed vs. Undirected Graphs
Graphs are another towering figure among non-linear data structures. A core aspect of graphs is the distinction between directed and undirected types. In directed graphs, edges have a specific direction, meaning that connections are one-way. This can be particularly useful in applications that require a sense of flow, such as web pages linking to one another or task scheduling.
On the other hand, undirected graphs allow for bi-directional relationships, often seen in social networks where connections can be mutual. Each type has its own use, making it crucial to analyze the specific needs of the problem at hand when choosing between them.
The adaptability of graphs is undeniably one of their strongest advantages. However, they also come with their own set of challenges, such as increased complexity in algorithms designed to traverse them.
Graph Algorithms
Graph algorithms, such as Dijkstra's for shortest paths or Depth First Search for traversal, are pivotal in numerous applications ranging from networking to optimization problems. The core advantage of graph algorithms lies in their ability to extract insights from complex relationships by systematically exploring connections and traversing edges.
One unique feature of these algorithms is their capacity to handle cycles and ensure completeness in exploring nodes. While powerful, they also run the risk of requiring substantial computational resources, particularly in densely connected graphs. Understanding the trade-offs involved, such as time complexity vs. space usage, can greatly enhance the effectiveness of algorithms utilized in real-world applications.
"Understanding the nuances of non-linear data structures unleashes your capacity to solve complex problems efficiently."
In summary, non-linear data structures, particularly trees and graphs, serve as robust tools for handling complex data relationships. Their layered approaches allow better performance and responsiveness to a plethora of programming needs. Yet, they bring along considerations that must be navigated with care to reap their full potential.
Hashing and Hash Tables
Hashing and hash tables play a pivotal role in optimizing data retrieval and storage, which makes them essential to any savvy programmer’s toolkit. Unlike traditional data structures that often involve linear searches and complex data management, hashing offers a tantalizingly efficient alternative. The essence of hashing lies in its unique ability to quickly map data from large sets into manageable indexes. This characteristic accelerates data access and retrieval, ultimately enhancing performance and application responsiveness—features that are critical in today’s data-driven landscape.
Concept of Hashing
At its core, hashing is the process of converting an input (or 'key') into a fixed-size string of bytes via a mathematical function known as a hash function. The output, often termed a 'hash code,' ideally reflects the input in a way that its original form is obscured.
This ability to condense information can significantly reduce the time needed for data operations. For example:
- Direct Access: When searching for a record in a hash table, the key is hashed, creating a unique index. The data associated with that key can be accessed directly without traversing through a list or array.
- Collision Resolution: While hashing minimizes the likelihood of duplicates, collisions (where two keys hash to the same index) can still occur. Techniques such as separate chaining or open addressing can effectively manage these scenarios, ensuring data integrity and accessibility.
Imagine needing to store user profiles where keys represent usernames. Instead of sorting through a long list of profiles, a hash table allows you to jump straight to the corresponding index with lightning speed.
Applications of Hash Tables
The practical applications of hash tables are plentiful and span across numerous domains, making them a vital component of many algorithms and data management systems.
- Database Indexing: Hash tables enhance the performance of database lookups. Instead of searching for an entry through every row, an indexed hash table can pinpoint exact matches swiftly.
- Caching Data: Hashing is instrumental in caching frequently accessed data in web applications. By storing pre-computed results associated with keys, it saves time on repetitive calculations.
- Symbol Tables in Compilers: During code compilation, symbol tables use hash tables to keep track of variables and their attributes, ensuring efficient resolutions at runtime.
- Cryptography: Hash functions serve as a backbone in cryptographic algorithms, ensuring the integrity of data through unique hash outputs that can detect alterations.
- Load Balancing Algorithms: Utilizing hashing techniques can distribute network traffic across multiple servers, resulting in improved efficiency and reliability.
Using hashing techniques can lead to significant performance improvements, especially when implemented for large datasets.
In practical programming scenarios, choosing to implement a hash table often results in seemingly instantaneous data access, making complex data management more manageable. This quality solidifies hashing as a powerful, indispensable asset in the world of data structures.
Comparing Data Structures
In the realm of programming, selecting the right data structure can often feel like searching for a needle in a haystack. Understanding how to compare data structures is vital, as it helps programmers make informed decisions tailored to the specific needs of their applications. Each structure comes with its own set of strengths and weaknesses, which is why this topic is essential. When you take a deep dive into comparing data structures, you're not just looking at what they are but also how they perform under different circumstances.
Complexity Analysis
A primary focus in comparing various data structures should be complexity analysis, which dives into both time and space complexities. These metrics give programmers insights into how well a data structure will perform regarding efficiency and resource usage.
Time Complexity
Time complexity is one of the cornerstones in the realm of data structures. It outlines how the runtime of an algorithm grows relative to the size of the input data set. When considering algorithms, understanding time complexity is critical because it sheds light on potential bottlenecks your application might face as it scales. Commonly expressed with Big O notation, time complexity helps identify how an algorithm will perform in the worst-case scenario, average scenario, and sometimes even the best-case scenario.
Moreover, an intriguing aspect of time complexity is how it sharply contrasts the expected performance with a more nuanced interpretation of real-world application. For instance, an O(n) algorithm can be efficient for smaller data sets, but as the data grows to millions of elements, it may become inefficient. Thus, one unique feature of time complexity is its ability to allow programmers to differentiate between various algorithms, helping them choose wisely based on the use case.
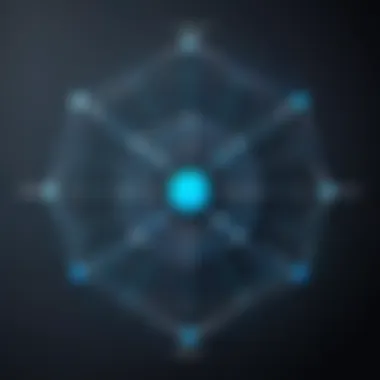
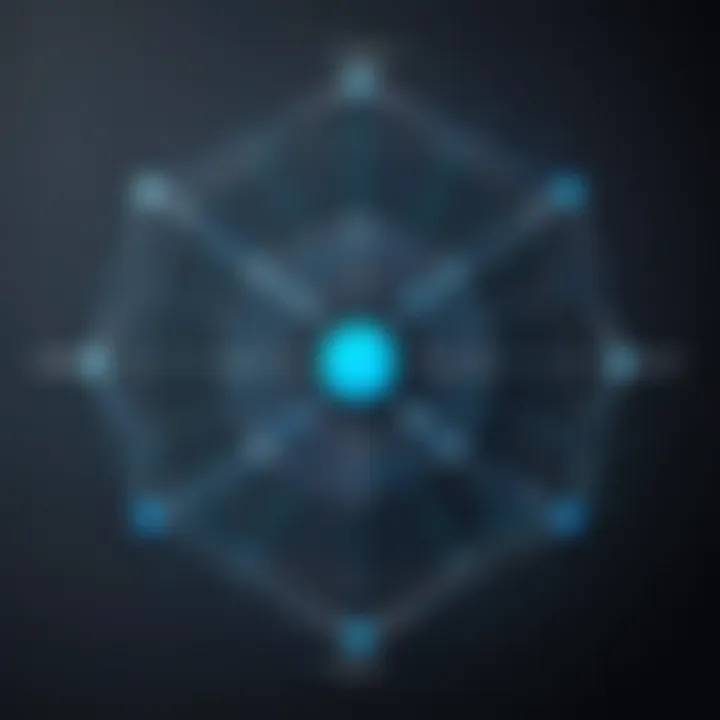
Space Complexity
On the flip side, space complexity examines the amount of memory space an algorithm requires as a function of input size. It's another critical aspect that cannot be ignored, especially since efficient algorithms often need to balance both time and space resources.
The key characteristic of space complexity is that it accounts not just for the space required for the input data but also for the auxiliary space needed for processing. An algorithm using a lot of temporary data storage may have a space complexity of O(n), indicating it scales directly with the input size. This can hinder performance in environments where memory resources are limited, like embedded systems.
A unique advantage of understanding space complexity is the ability to optimize data processing by minimizing additional memory usage. However, excessive space optimization can cause sacrifices in speed, showcasing the perennial trade-off between speed and space.
Choosing the Right Structure
Now, after grasping the fundamentals of complexity, the next step is to choose the right data structure based on the requirements of your application. This is where things can get a bit intricate. Every project has different needs—some might require fast access times, while others might prioritize memory efficiency.
But how do you determine what fits your needs? Here are some points to consider:
- Access Time: If you need quick lookups, you might lean towards hash tables or arrays.
- Insertion/Deletion Requirements: If your application involves a ton of insertions or deletions, a linked list might suit you better than an array.
- Memory Constraints: Keep an eye on how much memory you can afford to allocate. Some data structures might be memory hogs and slow down your application.
In the end, comparing data structures equips you with a valuable perspective that empowers you to approach coding more effectively. Each data structure is akin to a tool in a toolbox; knowing when and how to use them optimally can make all the difference in your programming journey.
Practical Applications
Understanding how data structures function is not just an academic exercise; it is crucial for honing problem-solving skills and improving programming efficiency. Practical applications give context to the theories we've covered. They provide insights into how abstract concepts translate into tangible solutions for real-world problems. When you grasp the practical aspect of data structures, you empower yourself to make informed decisions on which structures to wield depending on the challenge at hand.
Data Structures in Real-World Applications
Data structures play a pivotal role across various domains, impacting everything from everyday applications to high-stakes systems. For example:
- Databases: When a user queries databases, the underlying structure often employs trees or hash tables to retrieve data swiftly. This architecture ensures that information is fetched in an efficient manner, reducing wait times significantly.
- Navigation Systems: Pathfinding algorithms, which are essential for GPS applications, utilize graphs extensively. Each intersection can represent a node, and the routes between them form edges, aiding in navigating from one point to another effectively.
- Social Media Platforms: In platforms like Facebook, the connections between users can be visualized using graph structures. Each user is a node, and relationships are edges, facilitating friend suggestions and recommendations that enhance user engagement.
Understanding these practical applications allows budding programmers to relate theoretical knowledge directly to industry needs, ensuring their skills are relevant.
Coding Examples in Different Languages
To truly appreciate the utility of data structures, solid coding examples in popular programming languages are vital. Each language brings its strengths and quirks, allowing developers to leverage data structures in unique ways.
Java Implementation
Java is often lauded for its versatility and is prevalent in enterprise-level applications. A key characteristic of Java is its robust standard library, which includes implementations for a variety of data structures such as ArrayLists and HashMaps. This makes it convenient for programmers to access pre-defined data structures without reinventing the wheel.
One unique advantage of Java is its automatic memory management through garbage collection. This means programmers can focus more on problem-solving rather than manual memory allocation. However, one downside is that garbage collection can introduce unpredictability in performance during runtime.
Here's a quick snippet demonstrating a simple HashMap usage in Java:
This code captures the essence of how straightforward it is to implement and use data structures in Java. The ability to effortlessly store and retrieve data makes Java's data structures a popular choice among developers.
++ Visualization
C++ stands out for its performance and control over system resources. A key trait of C++ is its support for pointer manipulation, which offers developers fine-grained control over memory allocation. This is particularly beneficial when implementing data structures where memory management is crucial.
However, working with pointers can be a double-edged sword. While it provides flexibility, it also raises the complexity significantly, potentially leading to memory leaks and more bugs if not handled carefully. It's this command over system resources that makes C++ a favorable choice for systems programming and applications where performance is critical.
Consider a basic implementation of a linked list in C++:
This code illustrates how to create and traverse a linked list, highlighting C++'s preference for direct memory management. The balance of benefits and drawbacks between these programming languages underscores the importance of understanding data structures fully and how they can vary in implementation.
End
The conclusion of this guide serves as a crucial encapsulation of the intricacies surrounding data structures. Understanding the fundamental concepts highlighted throughout the article empowers programmers, from novices to seasoned developers, to make informed decisions while designing and implementing algorithms. When one carefully considers the strengths and drawbacks of the various data structures discussed, not only do they optimize their code, but they also enhance the overall performance and efficiency of applications.
Here are some essential points to keep in mind:
- Relevance: Selecting the right data structure directly impacts the efficiency of your program. It’s not merely about choosing the most advanced option; sometimes, a simple array or linked list can outperform complex structures when used correctly.
- Adaptability: As programming languages evolve and applications demand more robust capabilities, the ability to adapt one’s understanding of data structures becomes paramount. Familiarity with adaptable structures like trees and graphs can set one apart in the rapidly changing tech landscape.
- Real-World Applications: The practical applications provided earlier in this guide demonstrate the direct correlation between theory and practice. A solid grasp of data structures equips developers to tackle challenges in real-world projects, ensuring they can create solutions that are both effective and scalable.
Ultimately, a programmer's toolkit is not complete without a deep understanding of data structures. This knowledge will serve as a backbone of problem-solving skills and creative programming endeavors.
Summary of Key Points
- Data structures are foundational in programming, serving purposes that range from storage efficiency to ease of use in data manipulation.
- Types of data structures include primitive and non-primitive varieties, with each having unique traits and use cases.
- Complexity analysis when comparing structures is vital for understanding time and space efficiency, guiding developers in their choices.
- Practical implementations in programming languages such as Java and C++ illustrate the real-world implications and importance of these structures.
In summary, having a comprehensive understanding of data structures enables programmers to review and select the most applicable structures tailored to their specific use cases in varied scenarios.
Future Directions in Data Structures
The field of data structures is constantly evolving, much like the tech landscape itself. Here are some possible avenues to explore:
- Enhanced Data Structures: As data sets grow exponentially, traditional data structures are undergoing enhancements. Structures like advanced hash tables or self-balancing trees will gain more focus as the demand for efficiency increases.
- Integration with AI and Machine Learning: The fusion of data structures with machine learning algorithms is opening new doors. For example, understanding graph structures can significantly enhance neural network design, where relationships and network pathways become pivotal.
- Distributed Data Structures: As more applications run in distributed environments, structures designed for distributed architecture are a hot topic. This includes consensus protocols and structures that optimize data retrieval across network nodes.
- Emerging Programming Languages: With the rise of new languages, there will also be innovative ways to handle data in these contexts, pushing the boundaries of what's known about traditional data structures.
In keeping an eye on these future trends, programmers can anticipate changes in best practices and equip themselves with the skills necessary to leverage new advancements effectively.
"Understanding data structures is akin to having a roadmap in the wilderness of programming; it guides every decision and optimizes every step."
By considering these points, one can appreciate the ongoing journey of learning in the realm of data structures. Each step taken towards mastering this area transforms a programmer's approach to tackling challenges in coding.