Unraveling the Intricacies of Depth First Search Java Code - A Comprehensive Guide
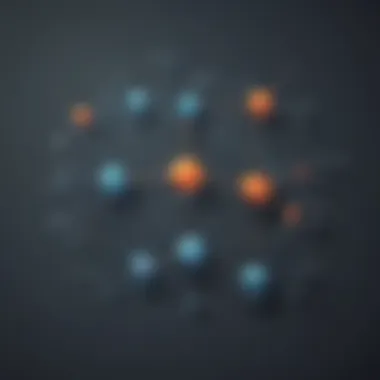
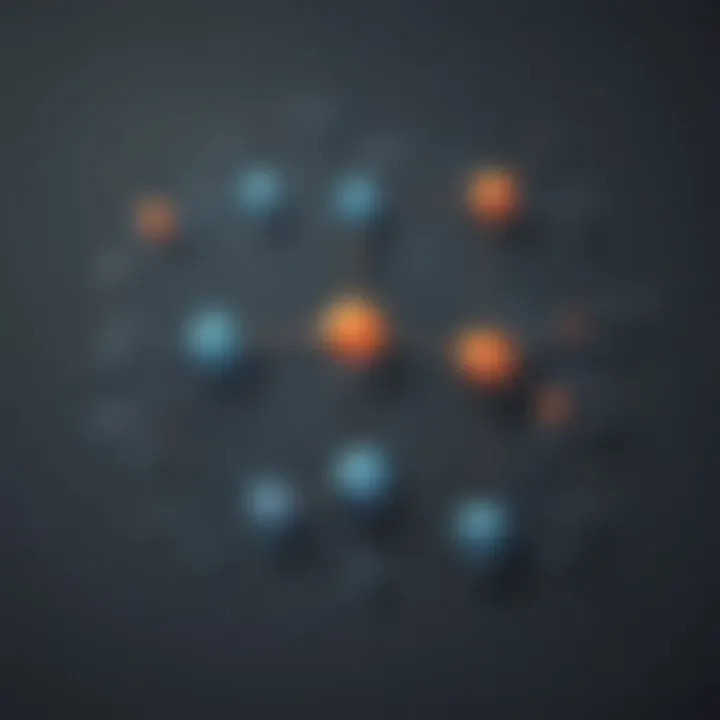
Introduction to Java Programming Language
Depth First Search (DFS) is a crucial algorithm in the field of computer science. To grasp the intricacies of DFS in the Java programming language, one must comprehend the fundamental concepts and syntax of Java. Understanding the history and background of Java is essential to appreciate its significance in modern programming. Java, known for its robust features and versatility, is widely utilized in various applications ranging from web development to mobile applications.
Basic Syntax and Concepts
When delving into Depth First Search in Java, familiarity with variables, data types, operators, expressions, and control structures is paramount. Variables serve as containers for storing data, while data types define the nature of this data. Operators and expressions are used to perform operations on variables, thereby manipulating data. Control structures like loops and conditional statements regulate the flow of a program, ensuring efficient execution.
Advanced Topics
As developers progress in their understanding of Java, exploring functions, methods, object-oriented programming (OOP), and exception handling is inevitable. Functions and methods encapsulate code for reusability and modularity, enhancing the efficiency of Java programs. OOP introduces concepts like classes and objects, enabling the construction of complex and scalable software systems. Exception handling safeguards programs from runtime errors, promoting stability and resilience.
Hands-On Examples
To solidify knowledge of Depth First Search in Java, engaging in hands-on examples is crucial. Beginning with simple programs to visualize basic concepts, enthusiasts can then graduate to intermediate projects that integrate multiple algorithms. Code snippets offer concise and practical solutions to implementing DFS in Java, empowering learners to experiment and refine their programming skills.
Resources and Further Learning
For individuals eager to deepen their understanding of DFS in Java, leveraging resources like recommended books and tutorials can provide invaluable insights. Online courses and platforms offer interactive learning environments, facilitating a dynamic educational experience. Engaging with community forums and groups allows enthusiasts to connect with like-minded individuals, fostering a collaborative and supportive learning ecosystem.
Introduction to Depth First Search
Depth First Search (DFS) is a fundamental algorithm in the realm of computer science and programming. Understanding DFS is crucial for developers looking to traverse data structures efficiently. In this comprehensive guide, we will unravel the intricacies of DFS in the Java programming language. By delving into DFS, readers can enhance their problem-solving skills and gain a deeper understanding of graph algorithms.
Understanding the Concept of DFS
Definition of DFS
DFS, in essence, is a technique used for traversing or searching tree or graph data structures. This method starts at the root node and explores as far as possible along each branch before backtracking. The key characteristic of DFS lies in its depth-first nature, where it prioritizes exploring as far as possible before backtracking. This approach allows for a systematic exploration of the data structure, often leading to efficient solutions for a variety of problems in graph theory and beyond.
Key Characteristics
One of the primary features of DFS is its ability to visit as far as possible along a branch before backtracking. This characteristic makes DFS particularly useful for tasks such as exploring maze-like structures, identifying connected components in graphs, and solving puzzles that involve backtracking. While DFS is powerful in its ability to systematically explore graphs and trees, it can sometimes lead to infinite loops in graphs with cycles, an aspect that developers need to be mindful of during implementation.
Applications of DFS in Programming
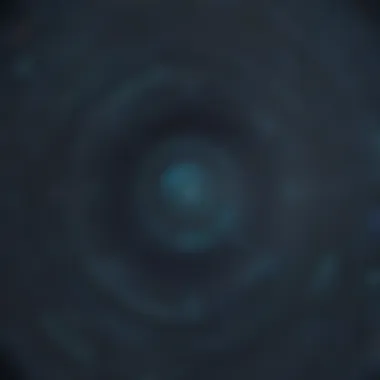
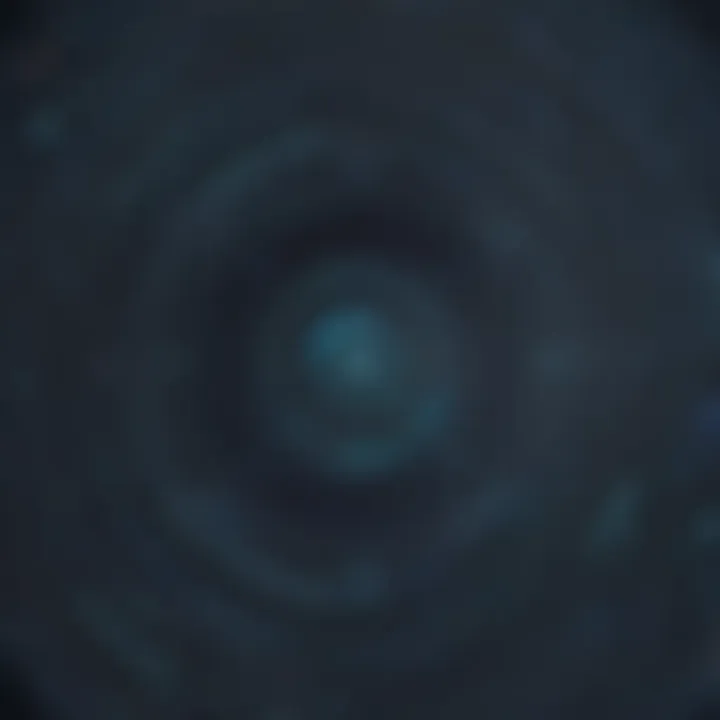
Graph Traversal
DFS plays a vital role in graph traversal by systematically visiting each node in a graph. This approach helps in exploring the connectivity of graph components and identifying paths between nodes efficiently. Graph traversal using DFS is beneficial for tasks like finding paths in mazes, solving puzzles, and mapping the structure of complex networks.
Cycle Detection
One of the key applications of DFS is cycle detection in graphs. By using DFS to traverse the graph and maintain a record of visited nodes, developers can detect cycles effectively. This capability is crucial in various scenarios, such as identifying deadlock situations in resource allocation systems, ensuring the acyclic nature of dependencies in project management, and detecting loops in fault tolerance mechanisms.
Topological Sorting
DFS also finds valuable application in topological sorting, a fundamental concept in directed acyclic graphs (DAGs). By applying DFS to a DAG, developers can order the nodes such that for every directed edge from node A to node B, A precedes B in the ordering. Topological sorting using DFS is essential in tasks like scheduling jobs, resolving dependencies in software builds, and organizing tasks based on precedence relationships.
Implementing DFS in Java
Implementing DFS in Java is a crucial part of this comprehensive guide, as it dives into the practical application of DFS algorithms in Java programming. By understanding and mastering the implementation of DFS, readers can enhance their problem-solving skills and graph traversal techniques. The discussion will encompass the various methods and approaches to implementing DFS in Java, highlighting the significance of efficient coding practices and algorithm design.
DFS Algorithm Overview
Stack Implementation
Delving into Stack Implementation within DFS algorithms is essential to grasp the underlying mechanics of depth-first search. The utilization of a stack data structure plays a vital role in tracking and revisiting nodes during traversal. The inherent Last-In-First-Out (LIFO) property of a stack aligns perfectly with the depth-first search strategy, allowing for systematic exploration of graph elements. While efficient for iterative processes, stack implementation may exhibit limitations in memory usage due to potential stack overflow.
Recursive Approach
The Recursive Approach in DFS algorithm design represents a fundamental technique that simplifies traversal logic through recursive function calls. By recursively exploring adjacent nodes, the algorithm efficiently navigates through graph structures, minimizing code complexity and enhancing readability. However, recursive techniques may encounter issues with deep recursion levels, leading to stack overflows and performance bottlenecks. Despite these challenges, the recursive approach remains a preferred method for implementing DFS due to its elegant and concise implementation.
Pseudocode
Incorporating Pseudocode into DFS algorithm development offers a pseudo-representation of the code logic, aiding in algorithm visualization and implementation planning. Pseudocode serves as an intermediary step between algorithm design and actual coding, outlining the sequential steps and decision points involved in a DFS traversal. By providing a high-level overview of the algorithm structure, pseudocode facilitates collaboration among programmers and serves as a blueprint for translating concepts into executable code.
Coding DFS in Java
Creating Graph Class
Creating a Graph Class forms the foundation for implementing DFS in Java, allowing for the representation of graph structures and operations within a program. The Graph Class encapsulates nodes, edges, and related functions, providing a modular and organized approach to graph management. By defining the essential properties and methods within the Graph Class, programmers can streamline DFS implementation and ensure scalability for larger graph datasets.
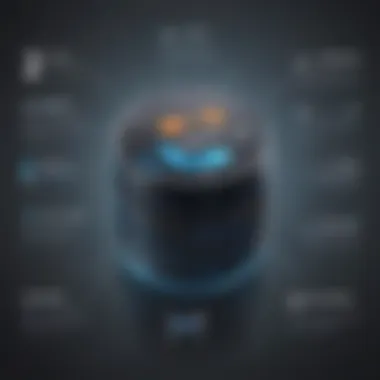
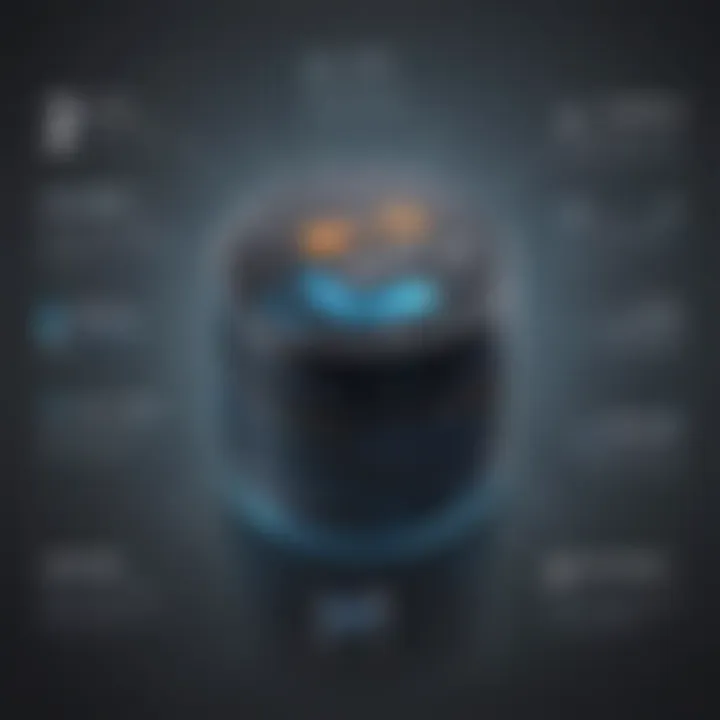
DFS Function
The DFS Function serves as the core component of DFS implementation in Java, responsible for executing the depth-first search traversal within a graph. This function embodies the recursive or iterative logic required to traverse graph nodes systematically and discover connected components. Through efficient implementation of the DFS function, programmers can achieve optimal performance in graph exploration and facilitate problem-solving in various applications.
Traversal Examples
Exploring Traversal Examples offers practical insights into the application of DFS algorithms in Java programming. By showcasing real-world scenarios and graph structures, these examples elucidate the step-by-step traversal process and highlight key decision points during DFS execution. Through analyzing traversal examples, readers can deepen their understanding of DFS principles and gain proficiency in integrating DFS algorithms into diverse programming tasks.
Optimizing DFS Performance
In this article, we delve into the crucial concept of optimizing Depth First Search (DFS) performance in Java. Optimizing DFS performance plays a vital role in enhancing algorithm efficiency and overall code functionality. By implementing specific techniques and strategies, programmers can significantly improve the speed and resource utilization of their DFS algorithms. This section will explore key elements such as memoization and caching results, shedding light on their benefits and considerations within the context of optimizing DFS performance.
Depth First Search Techniques
Memoization
Memoization is a fundamental aspect of optimizing DFS performance, contributing to the reduction of redundant computations and enhancing overall algorithm speed. The key characteristic of memoization is its ability to store and reuse previously computed results, thereby eliminating the need to recalculate the same values multiple times. In the context of this article, memoization serves as a valuable tool for improving the efficiency of DFS algorithms by storing intermediate results and avoiding unnecessary repetitive operations. Its unique feature lies in its capacity to increase algorithm speed by recalling previously solved subproblems, ultimately leading to a more optimized DFS implementation. While memoization offers advantages such as improved runtime performance and reduced computational complexity, it may also come with the challenge of increased memory consumption depending on the size of cached data.
Caching Results
Another essential aspect of optimizing DFS performance is the practice of caching results, which involves storing computed values for future reference. Caching results plays a pivotal role in accelerating algorithm execution by storing and accessing precomputed solutions to subproblems. The key characteristic of caching results lies in its ability to reduce the repetitive calculation of values, thus enhancing the overall efficiency of DFS algorithms. In the context of this article, caching results emerge as a popular choice for improving DFS performance due to its capacity to minimize redundant operations and expedite algorithm execution. The unique feature of caching results is its potential to streamline algorithm workflows by maintaining a record of computed values, thereby optimizing the traversal process. While caching results offer advantages such as faster execution and improved scalability, developers must carefully manage the stored data to prevent excessive memory usage and performance degradation.
Complexity Analysis
Time Complexity
Time complexity analysis is essential in evaluating the efficiency of DFS algorithms and assessing their computational performance. The key characteristic of time complexity lies in measuring the amount of time required for an algorithm to run as a function of the input size. In the context of this article, time complexity serves as a valuable metric for understanding the runtime behavior of DFS implementations and predicting their scalability. The unique feature of time complexity is its ability to provide insights into algorithm optimization opportunities and guide developers in improving overall performance. While low time complexity signifies faster algorithm execution and efficient resource utilization, high time complexity may result in slower runtime and increased computational overhead.
Space Complexity
Space complexity evaluation is crucial for analyzing the memory utilization of DFS algorithms and determining their storage requirements. The key characteristic of space complexity revolves around estimating the amount of memory needed by an algorithm to solve a problem instance based on the input size. In the context of this article, space complexity plays a significant role in optimizing DFS performance by considering the memory consumption of different algorithmic approaches. The unique feature of space complexity lies in its ability to highlight the trade-off between memory usage and algorithm efficiency, guiding developers in designing more space-efficient DFS solutions. While low space complexity indicates minimal memory usage and better storage optimization, high space complexity may lead to increased memory footprint and potential performance constraints.
Advanced Concepts in DFS
In this section of the article, we delve into the advanced concepts in DFS, which play a crucial role in enhancing our understanding of the topic. By exploring these advanced concepts, readers will gain deeper insights into the intricacies of the DFS algorithm in Java. Understanding these advanced concepts is essential for programmers looking to optimize their code and improve performance. By dissecting these complexities, we aim to equip readers with the knowledge and skills necessary to master DFS implementation in Java.
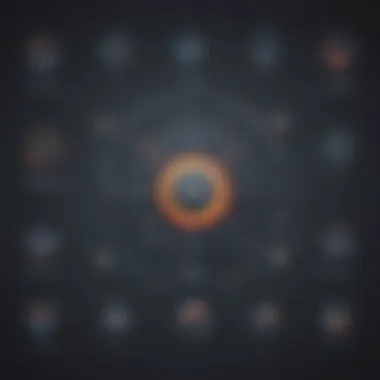
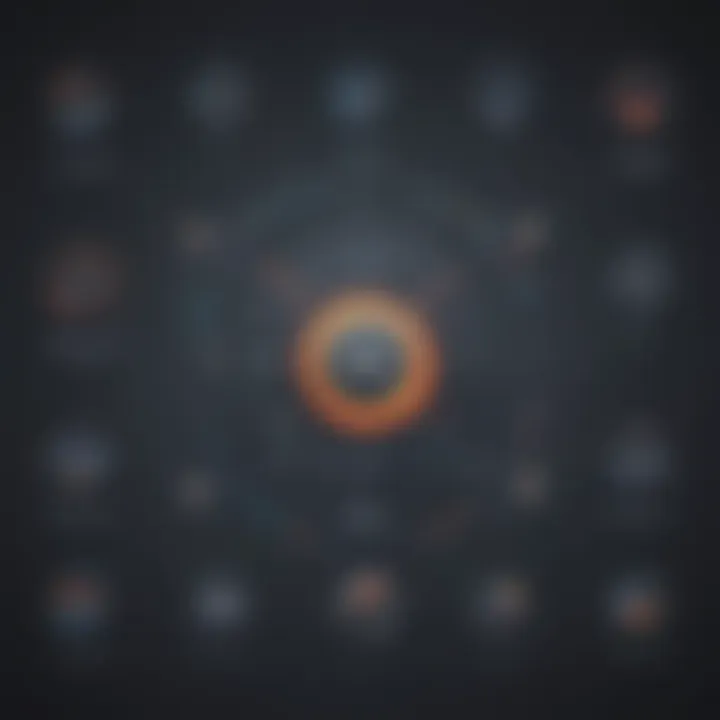
Backtracking and DFS
Integration Methods
Delving into the integration methods within the realm of DFS sheds light on a vital aspect of optimizing algorithmic performance. These methods provide a structured approach to incorporating backtracking into DFS, allowing for efficient problem-solving and algorithmic traversal. The key characteristic of integration methods lies in their ability to streamline the process of backtracking, thereby facilitating a more organized and systematic approach to problem-solving. This article underscores the significance of integration methods in enhancing the overall efficiency and effectiveness of DFS in Java, emphasizing their role in improving code readability and optimizing algorithm performance.
Examples
Examining specific examples within the context of DFS offers invaluable insights into real-world applications of the algorithm. By dissecting these examples, readers can visualize how DFS operates in various scenarios and understand its practical implications. Highlighting key characteristics of examples allows for a comprehensive analysis of their impact on the overall DFS implementation in Java. This section underscores the importance of examples in solidifying conceptual understanding and reinforcing theoretical knowledge, making them an indispensable tool for programmers seeking to master DFS algorithms.
Multi-dimensional Array Traversal
Strategies
Exploring strategies for multi-dimensional array traversal provides a comprehensive approach to optimizing DFS performance. By understanding these strategies, programmers can efficiently navigate through complex arrays, enabling seamless traversal and efficient data processing. The key characteristic of these strategies lies in their ability to enhance algorithmic efficiency and reduce computational complexity, making them a valuable asset in DFS implementation. This article emphasizes the importance of leveraging these strategies to optimize multi-dimensional array traversal in Java, ensuring streamlined and effective algorithm execution.
Impact on Performance
Analyzing the impact of multi-dimensional array traversal on performance is essential for gauging the effectiveness of DFS algorithms. Understanding how traversal strategies affect overall performance helps programmers make informed decisions about optimizing their code. The key characteristic of this analysis lies in its ability to highlight areas for improvement and optimization, resulting in enhanced algorithm efficiency. By comprehensively examining the impact of traversal strategies on performance, this article equips readers with the knowledge needed to enhance DFS implementation in Java and improve overall algorithmic performance.
Challenges and Best Practices
In this section of the comprehensive guide on Exploring Depth First Search Java Code, the focus shifts towards understanding the vital aspects of challenges and best practices associated with DFS implementation. Delving deep into this topic is crucial as it sheds light on the potential obstacles that programmers may encounter while navigating the complexities of DFS algorithms. By discussing the notable challenges and recommended best practices, readers can glean valuable insights into optimizing their coding strategies for efficient DFS implementation. Emphasizing the significance of identifying common pitfalls and incorporating industry-standard guidelines underscores the practical applicability of the theoretical knowledge presented in earlier sections.
Common Pitfalls to Avoid
Issues with Recursion
In the realm of DFS implementation, a prevalent challenge that programmers face is the issue of recursion. This recursive technique, while fundamental to the essence of DFS algorithms, can sometimes lead to difficulties in managing memory allocation and computational resources. Understanding the nuances of recursion and its impact on runtime efficiency is crucial for effective DFS coding. Despite its intrinsic value in simplifying complex problems, unchecked recursion levels can result in stack overflow errors and hinder program performance. By comprehensively analyzing the implications of recursive structures within DFS algorithms, programmers can strategize and optimize their code for streamlined execution.
Inefficient Data Structures
Another critical aspect to consider in DFS implementation is the choice of data structures. Opting for inefficient data structures can significantly impact the runtime and memory utilization of DFS algorithms. The selection of appropriate data structures, such as graphs or stacks, plays a pivotal role in enhancing the overall efficiency of DFS traversal. Inefficient data structures can lead to redundant computation and suboptimal performance, underscoring the importance of employing optimized data structures for DFS coding. By evaluating the suitability of different data structures and their alignment with the specific requirements of DFS algorithms, programmers can mitigate potential bottlenecks and elevate the robustness of their code.
Recommended Guidelines
Code Readability
Ensuring code readability is paramount when delving into DFS Java code implementation. The clarity and coherence of coding logic significantly influence the comprehensibility and maintainability of the DFS algorithm. By adhering to standardized coding conventions and adopting structured coding practices, programmers can enhance the readability of their codebase. Improved code readability not only facilitates collaboration and debugging processes but also enhances the scalability of the DFS implementation. Employing descriptive variable names, concise comments, and logical indentation patterns fosters a codebase that is intuitive and user-friendly, promoting efficient comprehension and long-term sustainability.
Optimization Strategies
Optimizing DFS performance through strategic coding strategies is essential for maximizing algorithm efficiency. By scrutinizing the computational complexities of DFS algorithms, programmers can identify optimization opportunities and fine-tune their coding approaches accordingly. Implementing algorithmic enhancements, such as memoization and result caching, can significantly reduce redundant computations and expedite algorithm execution. Strategic optimization strategies tailored to the specific requirements of DFS traversal can yield substantial performance gains and elevate the overall effectiveness of the algorithm. By integrating optimization techniques into the coding workflow, programmers can achieve optimal algorithmic performance and harness the full potential of DFS implementation.