Unveiling the Intricacies of Object-Oriented Programming in C++
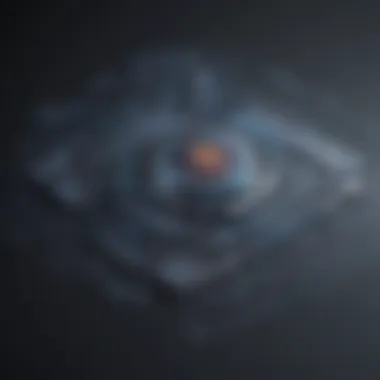
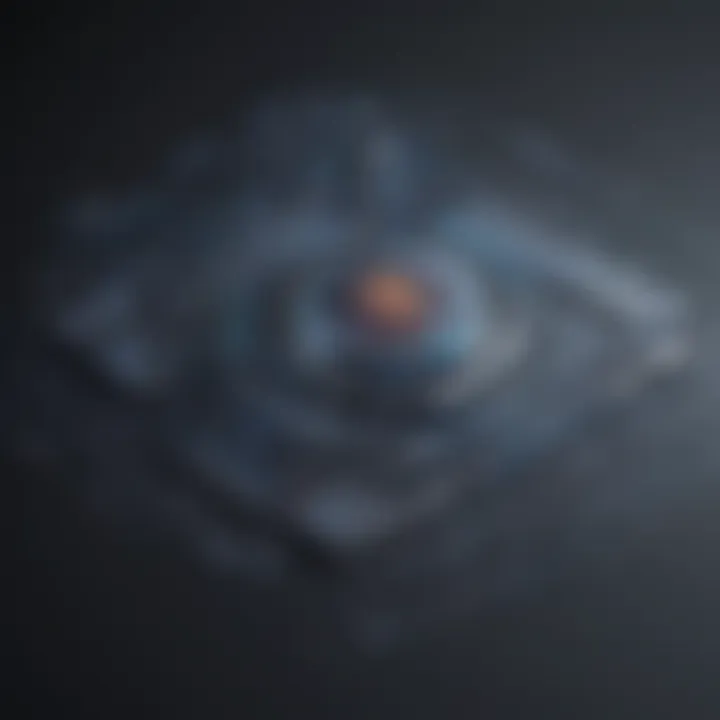
Introduction to Programming Language
Object-Oriented Programming in C++ is a complex yet powerful paradigm that enables developers to create robust and efficient software applications. Understanding the history and background of C++ is crucial in comprehending its significance in the realm of programming languages. C++ was developed by Bjarne Stroustrup in the 1980s as an extension of the C programming language, aiming to provide additional features for object-oriented programming. Its roots in C give C++ a high performance and efficiency, making it a preferred choice for developing system software, games, drivers, and complex applications. C++ is known for its flexibility and speed, allowing developers to have low-level access to memory and system resources.
Delving into the features and uses of C++ sheds light on the versatility of the language. C++ supports both procedural and object-oriented programming paradigms, offering developers the capabilities to write efficient and maintainable code. Its rich set of features includes classes, inheritance, polymorphism, and operator overloading, providing a structured approach to software development. With C++ being a statically typed language, developers can catch errors at compile time, ensuring code reliability and performance. The scope of C++ extends to a wide range of applications, including desktop software, video games, embedded systems, and high-performance applications.
The popularity and scope of C++ in the programming world are significant. C++ has stood the test of time and remains a prominent language in various domains. It is highly favored in industries that require high performance and low-level system programming. Due to its efficiency and speed, C++ is widely used in finance, gaming, telecommunications, and operating systems. Its vast community support and extensive libraries contribute to its enduring popularity among developers globally.
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) stands as a fundamental pillar in the realm of computer science, revolutionizing how software is designed and developed. In this article, we delve deep into the essence of OOP in the context of C++, shedding light on its core principles, functionalities, and applications. Understanding OOP not only nurtures structured code but also enhances code reusability and maintainability, crucial in modern software engineering practices.
Understanding OOP Paradigm
The Evolution of Programming Paradigms
The Evolution of Programming Paradigms represents a pivotal shift in the way developers conceptualize and create software. It introduces a paradigm where modularization, reusability, and extensibility take center stage. This evolution emphasizes creating code that mirrors real-world entities, paving the way for clearer and more efficient software architecture. The benefits of this paradigm lie in its ability to streamline complex systems and foster collaboration among programmers.
Key Features of Object-Oriented Programming
Object-Oriented Programming offers a slew of key features that set it apart from traditional procedural programming. Encapsulation, inheritance, polymorphism, and abstraction serve as the cornerstone of OOP, imparting developers with powerful tools to model real-world scenarios effectively. These features aid in structuring code in a hierarchical and organized manner, facilitating easier maintenance and scalability. The adaptability and versatility of OOP make it a go-to choice for developing robust and flexible software solutions.
++ as an OOP Language
History of ++ and OOP
The historical narrative of C++ intertwines with the evolution of OOP, making it a symbiotic relationship that revolutionized software development. C++ was one of the pioneers in marrying OOP principles with procedural programming, offering developers a language that harmoniously blends efficiency with flexibility. Its robust features and versatile syntax have made it a popular choice for building complex systems and applications, standing the test of time as a cornerstone in the realm of OOP.
Benefits of Using ++ for OOP
C++'s prowess as an OOP language stems from its ability to seamlessly implement OOP concepts while retaining the speed and efficiency of low-level languages. The language's support for features like classes, polymorphism, and inheritance empowers developers to craft intricate software architectures with ease. Furthermore, C++'s performance optimization capabilities make it an ideal choice for resource-intensive applications, striking a balance between performance and functionality in the world of OOP.
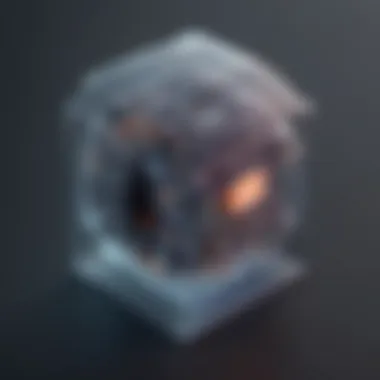
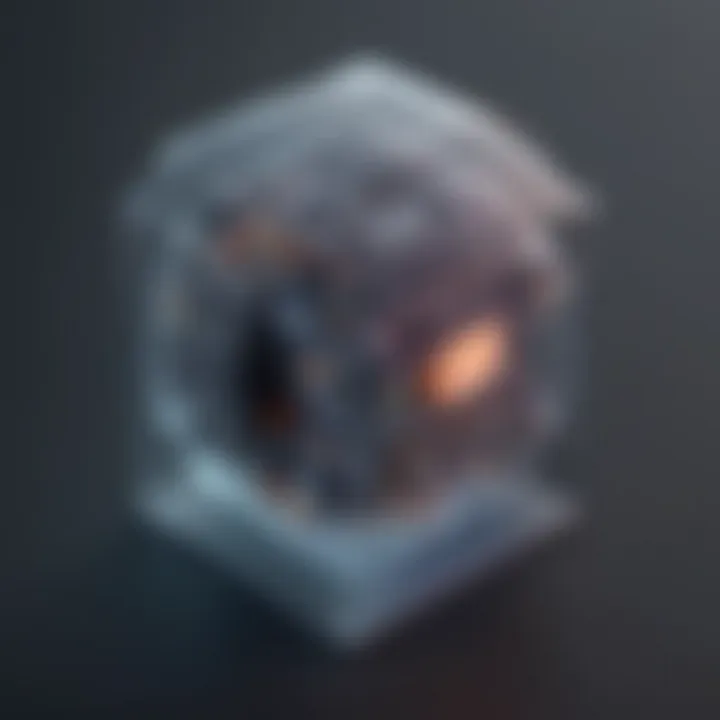
Core Concepts of OOP in ++
In exploring the intricacies of Object-Oriented Programming (OOP) in C++, the core concepts play a pivotal role in shaping the foundation of the language. Understanding these concepts is essential to grasp the fundamental principles that govern OOP in C++. Delving into classes, objects, encapsulation, inheritance, polymorphism, abstraction, and modularity provides a comprehensive overview of how C++ implements OOP paradigms. By unraveling these core concepts, programmers gain the necessary knowledge to design sophisticated and efficient software solutions in C++, leveraging the robust features offered by the language.
Classes and Objects
Defining Classes in ++
When it comes to defining classes in C++, precision and clarity are of utmost importance. A class serves as a blueprint for creating objects, encapsulating data attributes, and defining member functions that operate on the encapsulated data. By structuring code within class definitions, programmers can achieve code reusability, maintainability, and structured organization. The power of defining classes lies in its ability to model real-world entities, enabling developers to represent complex systems in a concise and efficient manner within C++ programs.
Creating and Manipulating Objects
Creating and manipulating objects in C++ involves instantiating class templates to create concrete instances that abide by the class structure. Through object instantiation, programmers can access the member functions and data members defined within the class, allowing for data manipulation and interaction within the program. Effective manipulation of objects is crucial for implementing the logic of the software application, managing data flow, and ensuring the proper functioning of the program's functionalities. The ability to create and manipulate objects efficiently is a key aspect of object-oriented design in C++, offering a high level of flexibility and scalability to developers.
Encapsulation and Data Hiding
The Principle of Encapsulation
Encapsulation lies at the heart of OOP in C++, emphasizing the bundling of data fields and methods within a class to restrict external access and ensure data integrity. By encapsulating data within class boundaries, C++ promotes information hiding, preventing unauthorized access and manipulation of class members from outside the class scope. The principle of encapsulation enhances code security, promotes modular design, and reduces dependencies, leading to more robust and maintainable software solutions. Implementing encapsulation practices in C++ paves the way for building secure and well-structured codebases that adhere to OOP principles.
Access Specifiers in ++
In C++, access specifiers such as public, private, and protected play a vital role in determining the visibility and accessibility of class members. By specifying access levels for class attributes and methods, programmers can control the interaction between objects, encapsulation levels, and inheritance hierarchies. The use of access specifiers enhances code encapsulation, fosters information hiding, and establishes clear boundaries for data manipulation and function invocation. Understanding and applying access specifiers in C++ is essential for maintaining code integrity, enforcing data protection, and facilitating modular design practices within OOP projects.
Inheritance and Polymorphism
Types of Inheritance in ++
Inheritance, a key concept in OOP, allows classes to derive properties and behaviors from existing classes, fostering code reuse and hierarchical relationships. In C++, various types of inheritance, including single, multiple, hierarchical, and hybrid, offer flexibility in designing class hierarchies to model complex relationships and behaviors. By leveraging inheritance, programmers can establish class hierarchies that promote code organization, facilitate extensibility, and streamline software maintenance efforts. Understanding the nuances of inheritance in C++ enables developers to create efficient and scalable class structures that imbibe the essence of OOP principles.
Polymorphic Behavior in OOP
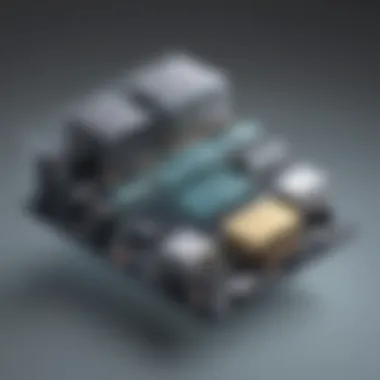
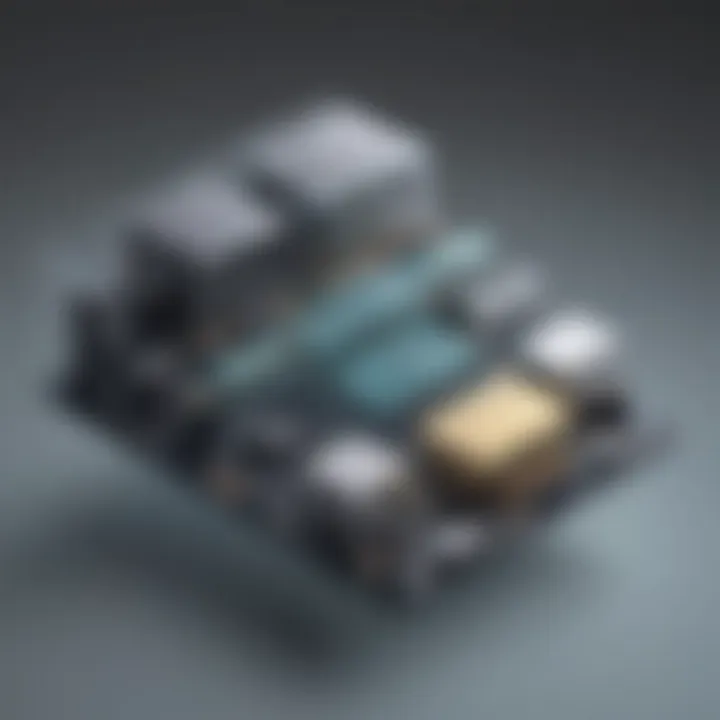
Polymorphism in C++ empowers objects to exhibit multiple forms based on their underlying class types, enabling dynamic runtime behavior and flexibility in method invocation. By implementing polymorphic behavior through virtual functions and function overriding, programmers can design adaptable and extensible software solutions that cater to varying requirements. Polymorphism fosters code modularity, enhances code readability, and promotes uniform interfaces, facilitating enhanced code reusability and scalability within OOP projects. Embracing polymorphic behavior in C++ unlocks new dimensions of flexibility and modularity in software design, enriching the development process and enhancing code maintainability.
Abstraction and Modularity
Abstract Classes and Methods
Abstraction in C++ allows developers to define abstract classes and methods that declare interfaces without specifying implementation details. Abstract classes serve as templates for concrete subclasses, providing a structural framework for defining common behavior and characteristics. By embracing abstraction, programmers can design modular and extensible systems that adhere to OOP principles, promoting code reuse and scalability. Abstract classes and methods enable developers to establish clear abstractions, promote code decoupling, and enhance code maintainability, laying the foundation for building modular and adaptive software solutions in C++.
Building Modular Programs in ++
Modularity in C++ revolves around structuring programs into coherent modules or units, encapsulating related functionality and data to promote code organization and reusability. By breaking down complex systems into manageable modules, programmers can enhance code comprehension, maintainability, and extensibility. Building modular programs in C++ involves employing techniques such as header files, namespaces, and separation of concerns to create cohesive and scalable software architectures. Modular design fosters code partitioning, promotes code encapsulation, and facilitates collaborative development, enabling teams to work on distinct modules independently while ensuring overall program coherence and efficiency.
Advanced Topics in OOP with ++
When delving into the intricacies of C++ programming, exploring advanced topics in Object-Oriented Programming becomes essential. This section serves as a gateway to in-depth discussions surrounding complex concepts that elevate one's understanding of OOP principles within the C++ framework. Emphasizing key components like templates, generic programming, exception handling, and the Standard Template Library (STL) is crucial for those aiming to master the nuances of C++. By comprehensively examining these advanced topics, individuals can enhance their programming expertise and approach problem-solving in a structured manner.
Templates and Generic Programming
Creating Template Classes
In the realm of C++ programming, the creation of template classes stands out as a pivotal aspect of generic programming. Template classes enable programmers to define generic types that can adapt to various data structures without compromising code integrity. This flexibility not only streamlines the development process but also enhances code reusability and maintainability. By delving into creating template classes, programmers can harness the power of abstraction to construct robust and adaptable solutions. However, it is crucial to acknowledge that improper usage of templates may lead to code bloat and compilation issues, making a judicious approach vital when integrating template classes into C++ projects.
Utilizing Generic Algorithms
Exploring the realm of generic algorithms introduces programmers to a versatile approach to handling data processing and manipulation. By leveraging generic algorithms, developers can write code that operates on different data types without the need for redundant implementations. This methodology not only optimizes code efficiency but also fosters code consistency and readability. The unique feature of generic algorithms lies in their ability to promote code abstraction and code reuse, fundamental aspects of modern programming practices. However, understanding the intricacies of generic algorithms is paramount to harnessing their full potential and avoiding pitfalls such as reduced code clarity or performance inefficiencies.
Exception Handling
Error Handling Strategies in ++
Within the landscape of C++ programming, the implementation of robust error handling strategies plays a vital role in ensuring code reliability and integrity. Error handling strategies in C++ encompass a spectrum of techniques aimed at gracefully managing exceptional situations that may arise during program execution. By adopting effective error handling mechanisms, programmers can fortify their code against unforeseen errors, enhancing the overall robustness of their applications. The essence of error handling lies in preemptively identifying potential failure points and establishing clear protocols to address such scenarios. However, it is imperative to strike a balance between comprehensive error handling and code simplicity to maintain code readability and prevent over-complication.
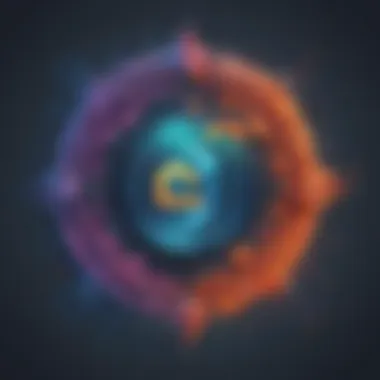
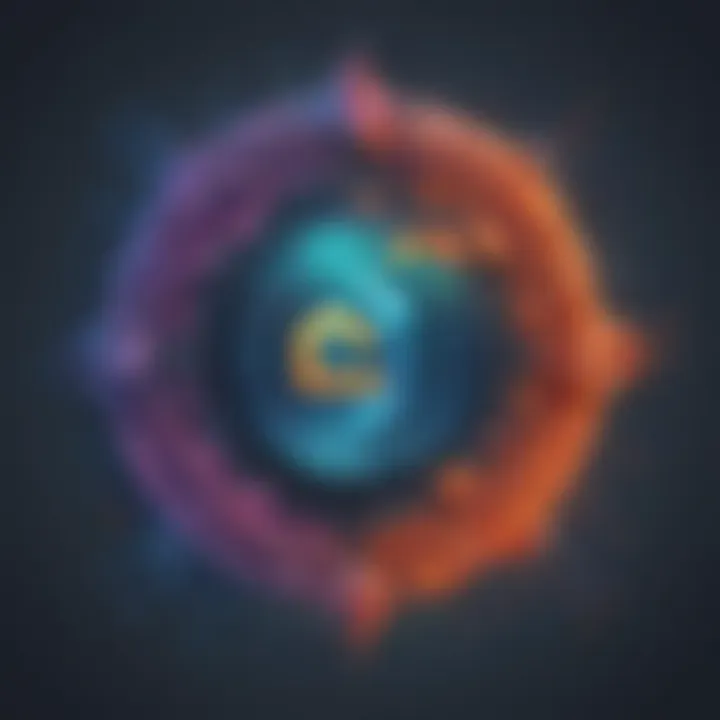
Try-Catch Blocks and Throwing Exceptions
The utilization of try-catch blocks and throwing exceptions stands as a fundamental practice in mitigating runtime errors and maintaining program stability. Try-catch blocks serve as a protective barrier, enabling developers to intercept exceptions and execute alternative paths in the event of errors. By strategically incorporating try-catch blocks, programmers can gracefully handle exceptions and prevent abrupt program termination, fostering a user-friendly experience. Throwing exceptions allows developers to signal exceptional conditions within their code, facilitating error propagation and resolution. However, excessive reliance on try-catch blocks may introduce overhead that impacts program performance, underscoring the importance of prudent exception handling practices.
STL and Containers
Standard Template Library Overview
The Standard Template Library (STL) in C++ offers a comprehensive collection of reusable container classes and algorithms, serving as a cornerstone of generic programming in C++. The STL provides programmers with a rich set of data structures and functions that streamline common programming tasks and promote code standardization. By familiarizing oneself with the STL, developers can expedite the development process and enhance code maintainability through the adoption of tested and optimized implementations. The key feature of the STL lies in its ability to abstract complex data structures into simple, reusable components, revolutionizing the way programmers approach data management and manipulation. Nevertheless, understanding the nuances of the STL's intricate components is essential to leverage its full potential and avoid unintended consequences.
Using Containers for Data Storage
Containers serve as fundamental building blocks within the realm of C++ programming, facilitating efficient data storage and retrieval operations. By employing a variety of containers such as vectors, lists, maps, and queues, programmers can tailor their data structures to suit specific application requirements with precision. The versatility of containers lies in their ability to encapsulate data, provide efficient access mechanisms, and maintain data integrity throughout the program's execution. Leveraging containers for data storage not only optimizes memory utilization but also enhances code modularity and scalability. However, selecting the appropriate container type based on the operational characteristics and performance considerations of the application is paramount to achieving optimal program efficiency and resource management.
Best Practices and Optimization Techniques
In the realm of object-oriented programming in C++, delving into best practices and optimization techniques becomes paramount for programmers seeking efficient and robust code structures. Emphasizing coding standards, efficient algorithms, and streamlined approaches, these practices aim to enhance code quality, maintainability, and performance. By adhering to best practices, developers ensure consistency across projects, mitigate errors, and foster collaboration among team members. Optimization techniques, on the other hand, focus on refining code for improved execution speed and resource utilization. Strategies such as algorithmic optimizations, memory management, and profiling contribute to enhancing the overall efficiency of C++ programs.
Design Patterns in ++
Common Design Patterns
Within the landscape of object-oriented programming, common design patterns serve as fundamental templates for solving recurring design problems effectively. These patterns encapsulate successful design strategies, offering proven solutions for structuring code and promoting code reuse. The Singleton pattern, for instance, restricts a class to a single instance, ensuring global access to that instance. Its simplicity and applicability make it a popular choice in various software development scenarios. On the other hand, the Observer pattern facilitates a one-to-many dependency between objects, allowing changes in one object to notify and update multiple dependent objects efficiently. Understanding common design patterns equips developers with versatile tools for developing scalable, maintainable, and extensible software solutions.
Applying Design Patterns to OOP Projects
Applying design patterns to object-oriented programming projects empowers developers to leverage proven solutions for specific design challenges. By integrating design patterns such as Factory Method, Strategy, or Decorator into OOP projects, developers enhance code structure, flexibility, and scalability. The Factory Method pattern, for example, defines an interface for creating objects while allowing subclasses to alter the type of objects that will be created, promoting a loosely coupled design. By contrast, the Strategy pattern encapsulates interchangeable algorithms within a family of objects, enabling clients to choose algorithms dynamically. Applying design patterns to OOP projects fosters code reusability, maintainability, and adaptability, elevating the overall quality and efficiency of software development.
Performance Optimization
Efficient Memory Management
Efficient memory management stands as a critical aspect of performance optimization in C++, significantly impacting program speed and resource utilization. By utilizing memory efficiently through strategies like smart pointers, memory pools, and RAII (Resource Acquisition Is Initialization), developers can mitigate memory leaks, reduce overhead, and enhance the responsiveness of their applications. Smart pointers, such as unique_ptr and shared_ptr, automate memory deallocation, thereby minimizing the risk of memory leaks and simplifying resource management. Memory pools optimize memory allocation by pre-allocating a fixed amount of memory, reducing overhead associated with frequent allocations and deallocations. RAII, a programming idiom in C++, ties the lifespan of resources to the lifespan of objects, ensuring timely resource deallocation and promoting deterministic memory management.
Minimizing Runtime Overhead
In the pursuit of optimized performance, minimizing runtime overhead becomes a core objective for developers aiming to enhance the efficiency and responsiveness of their C++ programs. Runtime overhead refers to the additional computational burden imposed by certain operations or structures, potentially impacting program speed and resource consumption. Strategies such as optimizing loops, reducing redundant function calls, and employing efficient data structures help alleviate runtime overhead and improve program performance. By optimizing loops through efficient iterator usage, loop unrolling, or loop restructuring, developers can enhance the execution speed of iterative operations. Similarly, minimizing redundant function calls and utilizing data structures like maps, sets, or arrays tailored to specific tasks can reduce unnecessary computational costs and streamline program execution, ultimately optimizing the overall performance of C++ applications.