Unveiling the Core Elements of Object-Oriented Programming Concepts
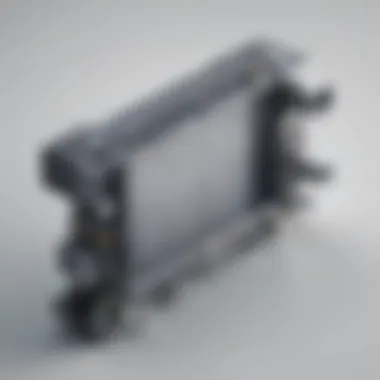
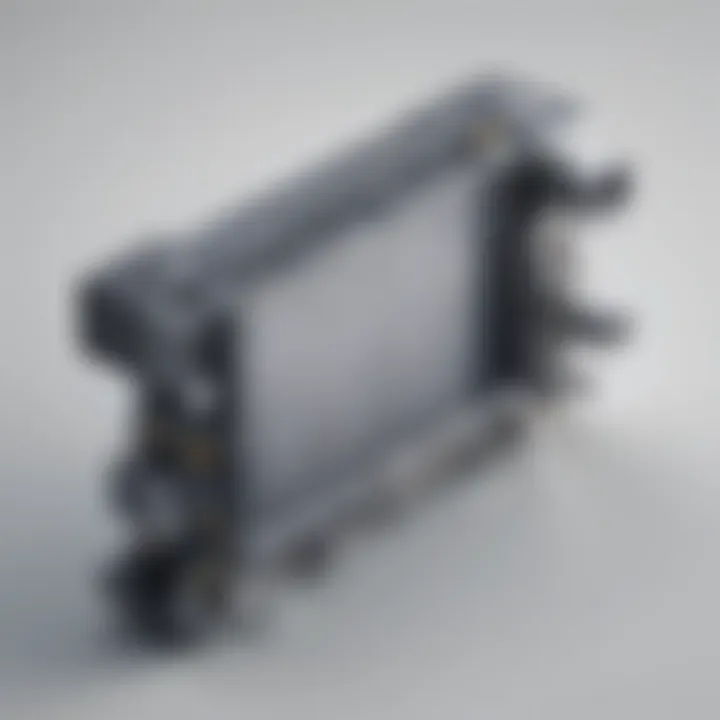
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of 'objects,' which can contain both data and code. These objects interact with one another, creating a structured approach to building applications. OOP is a fundamental aspect of modern programming languages and is widely used in software development across various industries. Understanding OOP principles is essential for mastering programming concepts.
Basic Concepts of OOP
Classes and Objects
In OOP, a class is a blueprint for creating objects with similar attributes and behaviors. Objects are instances of classes that encapsulate data and functions. By defining classes and creating objects, developers can model real-world entities in their programs, facilitating code organization and reusability.
Inheritance and Polymorphism
Inheritance allows a class to inherit attributes and methods from another class, promoting code reuse and establishing hierarchical relationships. Polymorphism enables objects to be treated as instances of their parent class, enhancing flexibility and modularity in code implementation.
Encapsulation and Abstraction
Encapsulation involves bundling data and methods within a class, ensuring data protection and controlled access. Abstraction focuses on hiding complex implementation details from the external world, allowing developers to work with high-level interfaces and reducing code complexity.
Advanced OOP Concepts
Functions and Methods
Functions are standalone blocks of code that perform specific tasks, while methods are functions associated with objects in OOP. By encapsulating functionality within objects, developers can achieve better code organization and maintainability.
Exception Handling
Exception handling is crucial in OOP to manage and respond to runtime errors effectively. By implementing error-handling mechanisms, developers can prevent application crashes and improve overall system robustness and reliability.
Practical Application of OOP
Building Simple Programs
Start by creating basic programs that demonstrate the use of classes, objects, and inheritance. Develop small applications to practice applying OOP principles and gain hands-on experience in program structuring.
Intermediate Projects
Advance to more complex projects that require extensive use of OOP concepts such as polymorphism and encapsulation. Design sophisticated applications that showcase the power and versatility of OOP in solving real-world problems.
Utilizing Code Snippets
Explore and analyze code snippets that illustrate OOP concepts in different programming languages. By studying existing code examples, learners can deepen their understanding of OOP paradigms and enhance their coding proficiency.
Resources for Further Learning
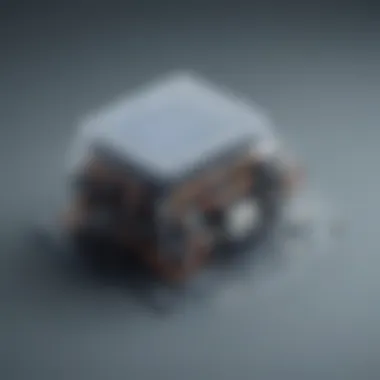
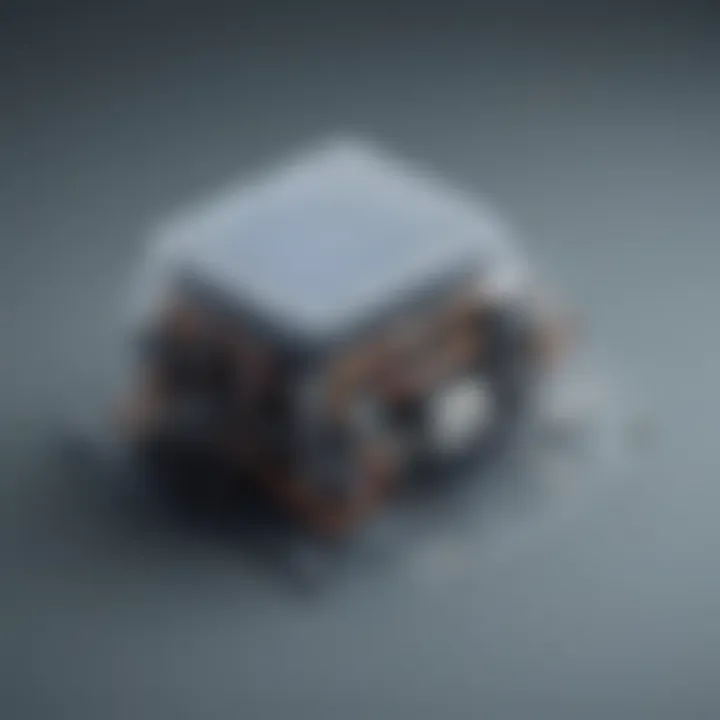
Recommended Books and Tutorials
Discover a curated list of books and online tutorials that delve into OOP principles and best practices. Enhance your knowledge and skills with comprehensive resources that provide in-depth explanations and practical exercises.
Online Courses and Platforms
Enroll in online courses and platforms offering specialized OOP training programs. Access interactive learning materials, virtual labs, and expert-led sessions to further elevate your OOP proficiency and programming capabilities.
Community Forums and Groups
Engage with like-minded individuals in programming forums and communities to share insights, seek assistance, and participate in discussions on OOP topics. Collaborate with peers, receive feedback on your projects, and stay updated on the latest trends in the ever-evolving field of OOP.
Introduction to OOP
Object-Oriented Programming (OOP) stands as a cornerstone in modern software development, revolutionizing the way programmers approach problem-solving and code structuring. As we embark on this journey of exploring OOP concepts, it is vital to grasp the fundamental principles that underpin the creation of robust and scalable applications. Understanding OOP affords developers the ability to organize code logically, promote code reusability, and enhance the overall maintainability of software systems. By harnessing the power of classes, objects, inheritance, polymorphism, encapsulation, and abstraction, one can unleash the full potential of OOP in transforming intricate problems into elegant solutions that stand the test of time.
Understanding Classes and Objects
Definition of Classes
At the core of OOP lie classes, blueprints that define the structure and behavior of objects within a system. A class encapsulates data attributes and methods, laying the groundwork for object creation and interaction. The elegance of classes lies in their ability to instantiate multiple objects sharing similar characteristics, fostering code modularity and efficiency. By defining classes, developers can achieve a high degree of code organization, paving the way for scalable and maintainable software solutions. However, one must tread cautiously, as overly complex class definitions can lead to code bloat and decreased readability.
Creating Objects
Creating objects from classes is the essence of OOP, instilling life into the abstract entities defined by classes. Objects represent tangible instances of classes, each holding its state and behavior. Through object instantiation, developers can model real-world entities in software, enabling dynamic data manipulation and system versatility. The creation of objects fosters code reusability, allowing developers to instantiate objects across various contexts, thus promoting efficient and concise code implementation. However, excessive object instantiation can lead to memory overhead and decreased performance, underscoring the need for a balanced approach in object creation.
Class Members
Class members encompass attributes and methods encapsulated within a class, delineating the data and behavior associated with objects instantiated from the class. Member variables store object state, while member functions dictate the actions an object can perform. By meticulously designing class members, developers can ensure data integrity, promote code coherence, and streamline object interactions. Careful consideration must be given to access levels of class members to strike a balance between data encapsulation and code extensibility. Class members form the building blocks of object-oriented design, embodying the core functionality and structure of OOP paradigms.
Advanced OOP Concepts
Object-Oriented Programming (OOP) is a fundamental paradigm in software development, and delving into Advanced OOP Concepts is crucial for programmers aiming to elevate their skills. This section explores the intricacies beyond basic OOP principles, offering insights into complex concepts that enhance code structure and efficiency. By understanding Advanced OOP Concepts, developers can streamline their programs, improve scalability, and maintain code maintainability effectively.
Composition and Aggregation
Relationships between Objects
Relationships between Objects play a pivotal role in software design, defining how objects interact and collaborate within a system. This aspect emphasizes the dynamic connections and dependencies between objects, elucidating the flow of data and functionality. By establishing clear relationships, developers can create modular and flexible systems that facilitate code reusability and enhance overall system robustness.
Code Reusability
Code Reusability is a key principle that emphasizes the optimization of code through the creation of reusable components. By encapsulating common functionalities into reusable modules, developers can reduce redundancy, promote consistency, and enhance code maintainability. This practice not only accelerates development speed but also improves the scalability and flexibility of the software system.
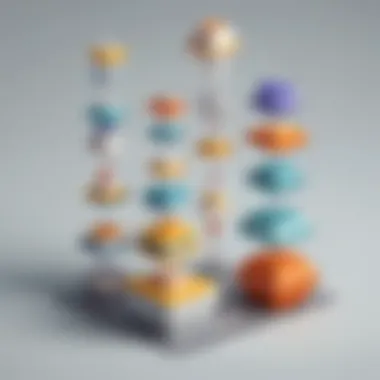
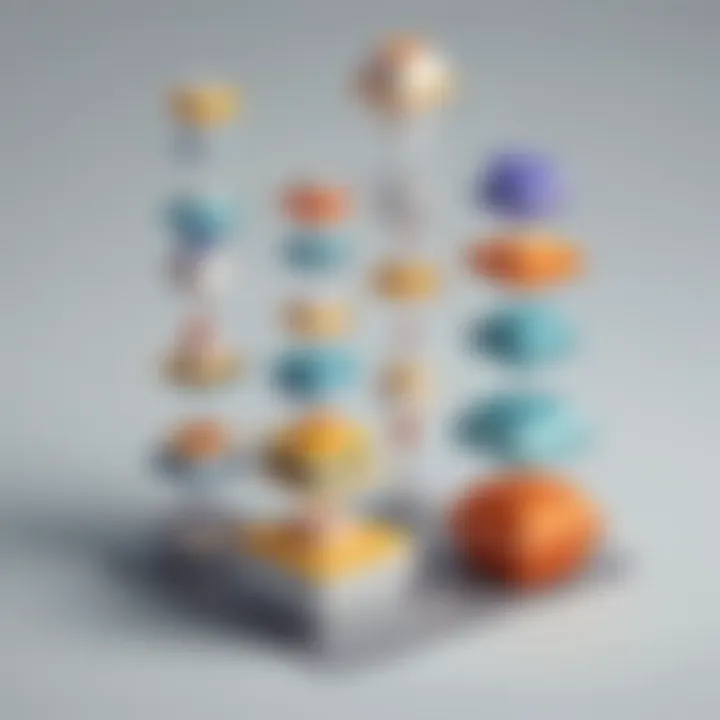
Containment vs. Inheritance
The distinction between Containment and Inheritance lies in their approaches to structuring relationships within object-oriented systems. Containment involves the integration of one object within another to provide functionality, promoting code modularization and flexibility. In contrast, Inheritance allows objects to inherit attributes and behaviors from parent classes, enabling code reusability and facilitating hierarchical structure. Each method presents unique advantages and considerations based on the system requirements and design goals, impacting system extensibility and maintenance.
Static vs. Dynamic Binding
Compile-Time vs. Runtime Binding
Compile-Time vs. Runtime Binding defines how programming languages bind method calls to method definitions. Compile-Time Binding associates method calls at compile time, enabling early error detection but limiting flexibility. In contrast, Runtime Binding binds method calls during program execution, providing dynamic flexibility but potentially introducing runtime errors. Understanding the trade-offs between these binding mechanisms is crucial for efficient program design and performance optimization.
Method Overloading vs. Method Overriding
Method Overloading and Method Overriding are essential concepts in OOP that enable developers to redefine methods in child classes. Method Overloading involves creating multiple methods with the same name but different parameters, enhancing code readability and promoting efficient parameter handling. Conversely, Method Overriding allows child classes to provide a specific implementation for inherited methods, facilitating polymorphic behavior and customization. By leveraging these mechanisms, developers can design versatile and extensible systems that cater to diverse requirements.
Implementation Differences
Implementation Differences encompass the variations in how programming languages and platforms execute code, impacting program behavior and performance. These differences influence factors such as memory management, performance optimization, and error handling, necessitating strategic decisions during system design. By discerning the implementation disparities, developers can fine-tune their code for efficiency, robustness, and cross-platform compatibility.
Object-Oriented Analysis and Design
Modeling Concepts
Modeling Concepts form the cornerstone of software development, enabling developers to visualize system structures, relationships, and behaviors. By utilizing modeling techniques such as class diagrams and entity-relationship diagrams, developers can create a blueprint of the system architecture, fostering clarity and coherence in design. Modeling Concepts facilitate effective communication among team members, aiding in collaborative design and consistent implementation.
Unified Modeling Language (UML)
Unified Modeling Language (UML) is a standardized notation system that enhances software design and communication. UML diagrams, such as class diagrams, sequence diagrams, and activity diagrams, provide a visual representation of system components and interactions. By adhering to UML standards, developers can streamline the design process, mitigate errors, and improve stakeholder understanding of the system architecture.
Design Patterns
Design Patterns are reusable solutions to common software design problems, encapsulating best practices and design principles. By applying established patterns such as Singleton, Factory, and Observer, developers can enhance code maintainability, scalability, and extensibility. Design Patterns promote flexibility in system design, enabling developers to address complex design challenges systematically and efficiently.
Real-World Applications of OOP
Object-Oriented Programming (OOP) transcends theoretical concepts to manifest in real-world applications, revolutionizing the realm of software development. In modern technological landscapes, the application of OOP principles is ubiquitous, enhancing the efficiency and scalability of software systems. The fundamental considerations of Real-World Applications of OOP serve as pillars in constructing robust and adaptable software solutions, catering to diverse industry needs and evolving user demands. The convergence of Modularity and Reusability, Maintainability and Scalability, and Object-Oriented Programming Languages fortify the foundation of Real-World Applications of OOP, fostering innovation and excellence.
Software Development Practices
Modularity and Reusability
Modularity and Reusability epitomize the essence of streamlined code development and maintenance in software engineering. By compartmentalizing functionalities into discrete modules, Modularity promotes cohesive design structures, facilitating code reusability across multiple components or projects. This approach not only expedites development timelines but also enhances code maintainability by isolating changes within specific modules without affecting the entire codebase. The intrinsic advantage of Modularity lies in its ability to enhance code organization and structural integrity, promoting efficient collaboration among developers while mitigating the risks of code duplication or convoluted dependencies.
Maintainability and Scalability
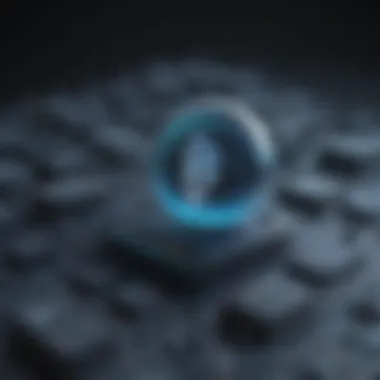
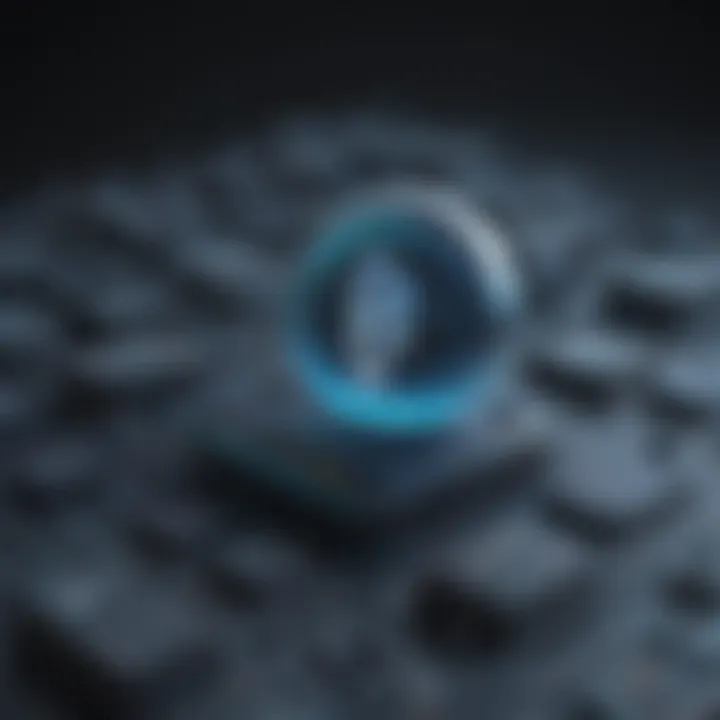
Maintainability and Scalability are pivotal facets in the software development lifecycle, delineating the ease of managing and expanding software systems over time. The hallmark of Maintainability lies in its capacity to simplify code upkeep and troubleshooting, enabling developers to rectify bugs or introduce enhancements expediently. Scalability, on the other hand, embodies the scalability of software systems to accommodate increasing workload demands or user interactions seamlessly. By adhering to principles of Maintainability and Scalability, developers can craft resilient and adaptable software solutions that thrive amidst dynamic operational environments, ensuring sustained performance and user satisfaction.
Object-Oriented Programming Languages
Object-Oriented Programming Languages serve as the conduits through which OOP principles are materialized into functional software applications. These languages encapsulate OOP paradigms encompassing classes, objects, inheritance, and polymorphism, empowering developers to architect sophisticated software systems with intricate hierarchies and interactions. The key characteristic of Object-Oriented Programming Languages lies in their intuitive syntax and expressive features, fostering code readability and maintainability. Despite the advantages of enhanced structuring and code organization, Object-Oriented Programming Languages may pose challenges in performance optimization and memory management, necessitating adept programming practices to mitigate potential inefficiencies.
Creating Custom Libraries and Frameworks
Encapsulation of Functionality
Encapsulation of Functionality embodies the encapsulation of data and behavior within objects, encapsulating implementation details from external entities. This modular approach streamlines code development by abstracting complex operational components into reusable modules, promoting code extensibility and adaptability. The key advantage of Encapsulation of Functionality lies in its ability to enforce data confidentiality and access control, safeguarding critical information from unauthorized manipulations or exposures.
Interoperability with Other Systems
Interoperability with Other Systems underscores the compatibility and integration of software components across disparate systems or platforms, fostering seamless interaction and data exchange. This interoperable functionality enables developers to orchestrate cohesive software ecosystems that harmonize diverse technologies, enhancing operational efficiencies and user experiences. The unique feature of Interoperability with Other Systems lies in its protocol-independent communication channels, facilitating seamless integration without necessitating substantial modifications or compatibility workarounds.
Extensibility and Flexibility
Extensibility and Flexibility delineate the adaptability and expansiveness of software frameworks and libraries, accommodating evolving requirements and technological advancements. The extensible nature of custom libraries and frameworks empowers developers to augment existing functionalities or integrate new features without disrupting core functionalities. This flexibility fosters innovation and scalability, enabling software systems to evolve organically in response to changing market dynamics and user preferences, ensuring continued relevance and competitiveness.
Object-Oriented Database Management Systems
Database Modeling
Database Modeling embodies the strategic design and structuring of data repositories within OOP frameworks, facilitating data organization and retrieval efficiency. By modeling data entities, relationships, and constraints, developers can architect robust database schemas that align with application requirements and scalability objectives. The key characteristic of Database Modeling lies in its capacity to optimize data storage and retrieval processes, enhancing query performance and data integrity within database systems.
Query Optimization
Query Optimization stands as a cornerstone in enhancing database performance by streamlining query execution and resource utilization. Through query optimization techniques such as indexing, query restructuring, and cache utilization, developers can expedite data retrieval processes and minimize computational overhead. The intrinsic advantage of Query Optimization lies in its ability to enhance database responsiveness and system efficiency, mitigating latency issues and improving user experiences through expedited data access.
Consistency and Security
Consistency and Security underpin the integrity and confidentiality of data stored within Object-Oriented Database Management Systems. By enforcing consistency constraints and access controls, developers can safeguard data integrity and prevent anomalous transactions or data corruptions. The paramount consideration of Consistency and Security lies in their role in fortifying data privacy and system reliability, ensuring regulatory compliance and user trust. Despite the advantages of stringent security protocols and data consistency mechanisms, these stringent measures may introduce performance overhead or operational complexities, necessitating a judicious balance between security measures and system performance optimizations.
Challenges and Best Practices
Understanding and implementing the best practices in the domain of Object-Oriented Programming (OOP) is of paramount significance when aiming for code efficiency and maintainability. By delving into the challenges and best practices associated with OOP, developers can ensure the robustness and scalability of their software solutions. Effective utilization of OOP principles demands a keen awareness of common pitfalls that may impede productivity and hinder the overall quality of code. Embracing best practices not only mitigates these obstacles but also fosters a structured approach to programming, enhancing code readability and reusability.
Common Pitfalls in OOP
- Over-Engineering: Over-Engineering, a common pitfall in software development, involves the unnecessary complexity of design or implementation beyond the project requirements. While this might initially seem an attractive choice, it often leads to bloated codebases, decreased maintainability, and diminished performance. Avoiding Over-Engineering necessitates striking a balance between functionality and simplicity to ensure optimal solutions that align with the project scope.
- Violation of SOLID Principles: Violation of SOLID principles signifies a departure from fundamental principles governing OOP design. This deviation can result in code fragility, increased coupling between components, and reduced adaptability to changes. Adherence to SOLID principles - emphasizing the fundamental tenets of software design such as Single Responsibility, OpenClosed, Liskov Substitution, Interface Segregation, and Dependency Inversion principles - is instrumental in fostering scalable, maintainable, and extensible codebases.
- Class Explosion: Class Explosion refers to the proliferation of classes within a system, leading to intricately interconnected class hierarchies and bloated code structures. This phenomenon can complicate code maintenance, impair system understanding, and hinder future scalability. Implementing strategies like composition over inheritance and modular design can mitigate the risks associated with Class Explosion, streamlining code management and enhancing system flexibility.
Guidelines for Effective OOP
- Single Responsibility Principle (SRP): The Single Responsibility Principle advocates for a class having a single responsibility, promoting high cohesion and modular design. By adhering to SRP, developers enhance code maintainability, readability, and testability. This principle helps in isolating changes, reducing the risk of unintended side effects, and facilitating code evolution without cascading modifications to unrelated functionalities.
- OpenClosed Principle (OCP): The OpenClosed Principle underscores the importance of code entities being open for extension but closed for modification. This principle spurs developers to design flexible and adaptive code structures by introducing new functionality through extension rather than altering existing code. By embracing OCP, software systems become more resilient to change, enabling seamless integration of new features without compromising existing functionalities.
- Liskov Substitution Principle (LSP): The Liskov Substitution Principle advocates for subtypes being substitutable for their base types, fostering polymorphic behavior and enhancing code reusability. Adhering to LSP ensures compatibility and interoperability between supertypes and subtypes, facilitating the interchangeability of objects within a hierarchy. By upholding LSP, developers streamline code maintenance and evolution, refining system scalability and adaptability.
Testing OOP Code
- Unit Testing Strategies: Unit Testing forms a cornerstone in verifying the functional correctness of individual units of code. By adopting Unit Testing Strategies, developers ascertain the reliability and robustness of code components, mitigating defects and enhancing code quality. Unit Testing aids in detecting deviations from expected behavior, facilitating pinpointed issue identification and expedited debugging processes.
- Mocking and Dependency Injection: Mocking and Dependency Injection techniques play a pivotal role in isolating dependencies and facilitating unit testing of interdependent components. Through Mocking, developers simulate external dependencies to ensure controlled testing environments and validate component interactions. Dependency Injection, on the other hand, enhances code flexibility and testability by decoupling dependencies, promoting modular and extensible design practices.
- Code Coverage Analysis: Code Coverage Analysis offers insights into the adequacy of testing scenarios, highlighting areas of code untested by existing test cases. By evaluating code coverage metrics, developers gauge the effectiveness of test suites in exercising all code paths, thereby identifying potential gaps in test coverage. Employing Code Coverage Analysis aids in enhancing test suite comprehensiveness and adequacy, bolstering code quality and reliability.