Exploring How Computer Programs Function
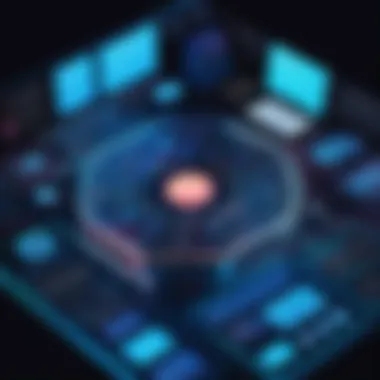
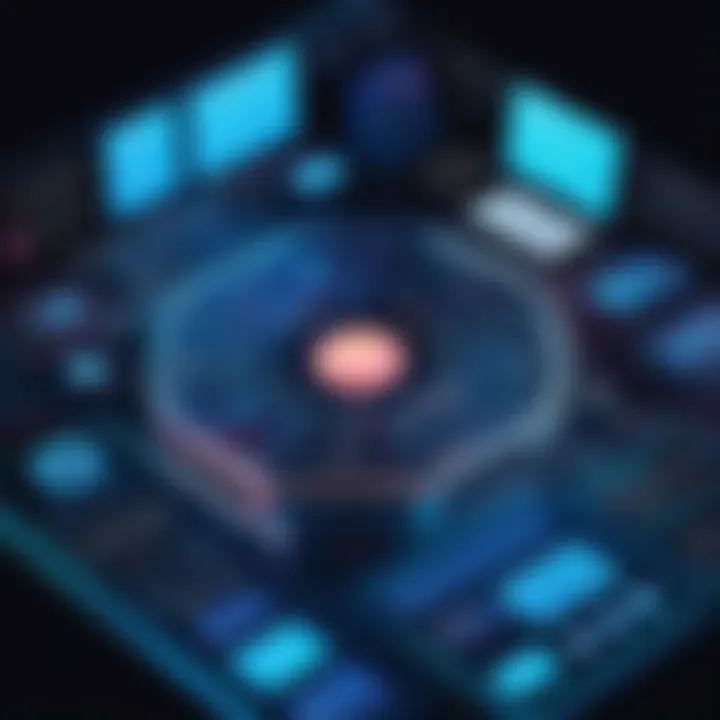
Prologue to Programming Language
Programming languages serve as the backbone of computer programs, functioning as a bridge between human logic and machine understanding. To truly grasp how computer applications operate, it's essential to delve into the languages that facilitate this communication.
History and Background
The evolution of programming languages spans several decades, tracing its roots back to the creation of assembly languages in the early 1950s. These languages, though starkly different from what we see today, were foundational in allowing programmers to write machine-level code in a somewhat comprehensible manner. Moving through the years, high-level languages such as Fortran and COBOL emerged, simplifying much of the coding burden. In the modern era, languages like Python, JavaScript, and Ruby have gained popularity, each bringing unique features that define their usability and performance in web development, data science, and software engineering.
Features and Uses
A programming language can be characterized by its syntax, semantics, and the paradigms it supports.
- Syntax is essentially the set of rules that defines the combinations of symbols that are considered to be correctly structured programs.
- Semantics refers to the meaning of those symbols and statements.
- Paradigms can be procedural, object-oriented, or functional, each offering distinct ways to approach problems and organize code.
Some languages are designed for specific tasks. For example, SQL is tailored for database management, while JavaScript excels in web application development. Understanding these features is crucial for aspiring programmers, as it helps them select the right language for their intended task.
Popularity and Scope
The choice of programming language often mirrors industry trends and technological advancements. The rise of data science and machine learning has catalyzed the growth of languages like R and Python. Meanwhile, the increasing demand for web applications has kept JavaScript at the forefront.
When choosing a language, consider not only its current popularity but also its growth trajectory. Community support, library availability, and industry relevance also significantly play a role in a programming language's effectiveness and longevity.
"As the digital world expands, understanding the languages that power it becomes increasingly vital for those looking to enter the tech workforce."
Overall, acquiring a solid foundation in programming languages opens doors to understanding complex computer programs and their functionalities, which are essential in today's technology-driven society.
Basic Syntax and Concepts
Understanding the basic syntax and fundamental concepts solidifies the groundwork for any language. This section explores variables, data types, operators, and control structures that nearly all programming languages share.
Variables and Data Types
At its core, a variable is a storage location paired with an associated name. This name represents a value.
Different programming languages support various data types, including:
- Integers for whole numbers
- Floats for decimal numbers
- Strings for sequences of characters
- Booleans for true or false values
Getting comfortable with data types helps you manipulate and use these values correctly in programs.
Operators and Expressions
Operators are symbols that perform operations on variables or values. Common types include:
- Arithmetic operators like +, -, *, and /
- Comparison operators such as ==, !=, , and >
- Logical operators such as && for 'and', || for 'or'
Understanding how to construct expressions using these operators is key to producing functional code.
Control Structures
Control structures dictate the flow of a program. Examples include:
- If statements for conditional logic
- For loops for iterating over a collection
- While loops for executing a block of code while a condition is true
These constructs allow you to implement complex behaviors in your programs.
Advanced Topics
Once you grasp the fundamentals, it’s time to dive into advanced topics that offer greater control and efficiency in your programming endeavors.
Functions and Methods
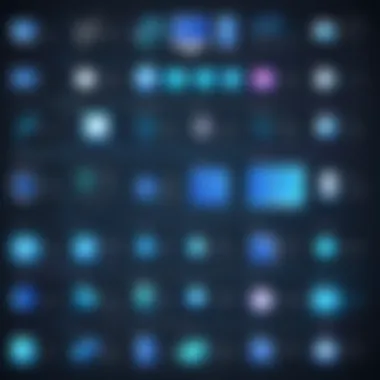
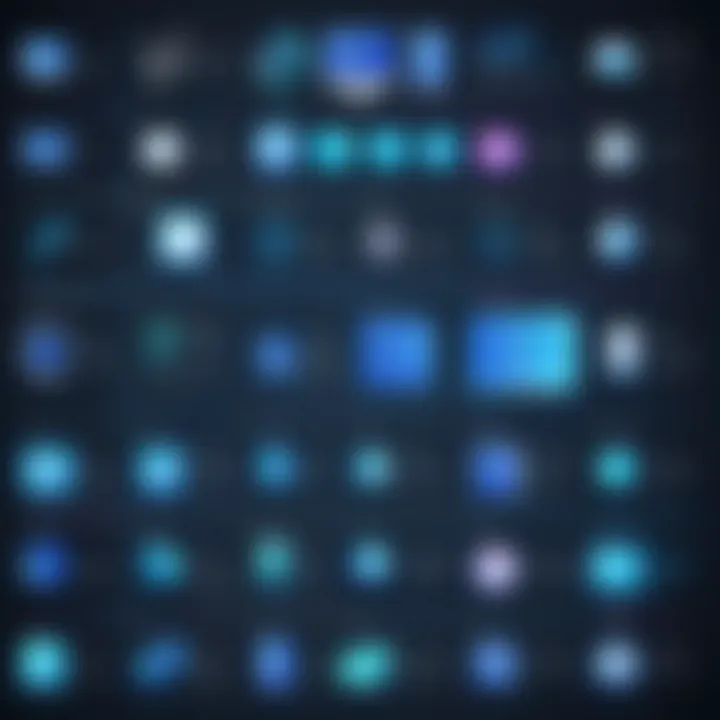
Functions are reusable blocks of code that perform a specific task. Understanding how to write and call functions not only makes programs easier to read but also helps in managing large codebases.
Methods, closely related to functions, are often associated with objects in object-oriented programming.
Object-Oriented Programming
If you've heard of classes and objects, you've touched on object-oriented programming (OOP). This programming paradigm models real-world entities, making it easier to relate to the code you write. Core principles include:
- Encapsulation: bundling of data with methods that operate on that data.
- Inheritance: allows one class to inherit the properties of another.
- Polymorphism: the ability to present the same interface for different underlying data types.
Exception Handling
Error management is pivotal in programming. Exception handling allows you to write code robust against failures. Using and blocks, programmers can anticipate potential errors and handle them gracefully, thus maintaining a smooth user experience.
Hands-On Examples
Theory comes to life in practice. Engaging with hands-on examples can significantly enhance comprehension and retention.
Simple Programs
Building simple programs like a calculator or a to-do list can provide a solid understanding of syntax and structures in a language of your choice.
Intermediate Projects
Once familiar with the basics, consider stepping up your game with intermediate projects, like a personal budget calculator, which combine various programming concepts.
Code Snippets
Here’s a small code snippet in Python that demonstrates a basic function:
Learning from simple code examples helps solidify your understanding and prepares you for more complex challenges.
Resources and Further Learning
To continue your journey into programming, it's crucial to leverage available resources.
Recommended Books and Tutorials
Books such as Automate the Boring Stuff with Python and Eloquent JavaScript are excellent for beginners, providing practical examples that help cement knowledge.
Online Courses and Platforms
Platforms like Coursera and edX offer a myriad of courses from introductory to advanced programming topics, providing the flexibility to learn at your own pace.
Community Forums and Groups
Engaging with communities on platforms such as Reddit or Stack Overflow can provide support and guidance as you navigate your learning path. Discussions can reveal practical insights that are not always covered in formal resources.
With a strong understanding of programming languages, concepts, and community support, you’ll be well-prepared to embark on your programming journey.
Overview of Computer Programs
Understanding the intricacies of computer programs is like peeling an onion. Each layer reveals more about how these systems operate, and why they are crucial in today's tech-driven world. A solid grasp of computer programs lays the groundwork for anyone interested in programming, software development, or even troubleshooting household devices that rely on these programs. Additionally, knowing the ins and outs can ultimately aid in optimizing performance and enhancing user experience.
Computer programs are the lifeblood of our modern digital landscape. They perform an array of tasks, ranging from simple calculations on a spreadsheet to complex simulations used in scientific research. With technology advancing at breakneck speed, programs have rapidly become pivotal in automating processes that once required significant manpower. Thus, understanding their functionality is not only beneficial but essential for those looking to navigate the terrain of technology.
In this section, let’s delve into the definition of computer programs and categorize them, which will set the stage for deeper explorations into their underlying mechanics.
Definition of Computer Programs
At its core, a computer program is a sequence of instructions that from runtime to runtime guide the computer to perform specific tasks. Whether it's basic arithmetic or sophisticated data processing, programs can essentially communicate with hardware to mobilize activities that fulfill user requirements. A typical program is written in a programming language, which serves as an intermediary between human-directed instructions and machine-readable code.
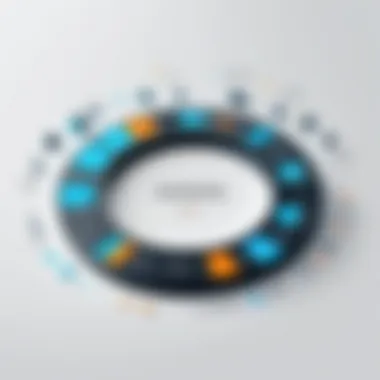
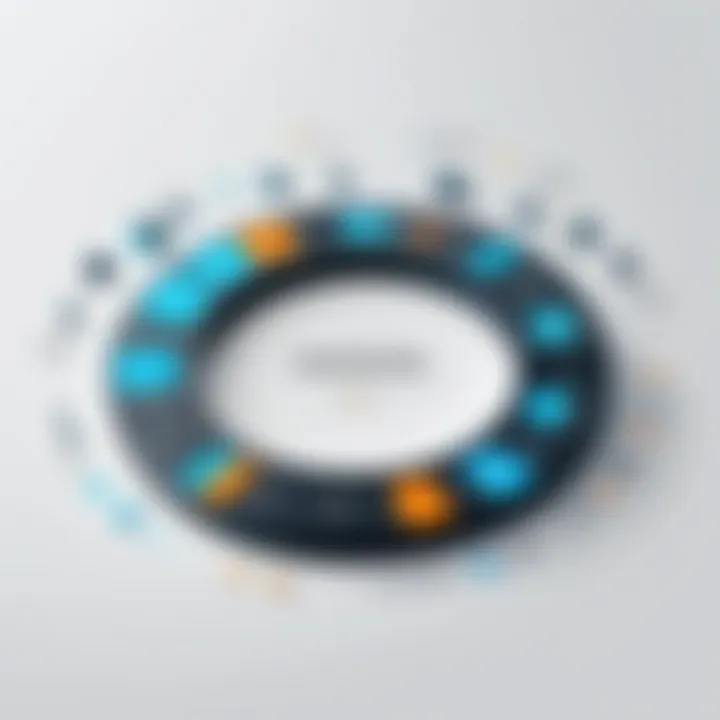
Types of Computer Programs
Understanding the different categories of computer programs is crucial as each type serves distinct purposes and functionalities that benefit users in specific domains. Here's a breakdown:
System Software
System software comprises the foundational programs that manage and operate computer hardware. This includes operating systems like Windows and Linux. They coordinate how hardware components interact, ensuring everything runs smoothly.
Key Characteristic: One of the defining traits of system software is its ability to manage resources. It acts as a bridge between users, application software, and the hardware, facilitating effective communication.
Unique Feature: Another unique aspect is stability and security. System software often includes features that safeguard hardware from misuse, making them a backbone of any computer environment.
Advantages include enhanced performance, while a significant disadvantage often noted is that upgrading or changing existing system software can lead to compatibility issues with existing applications.
Application Software
Whereas system software serves the hardware, application software caters directly to users. This type includes programs designed to help users perform tasks, such as Microsoft Word for document editing or Adobe Photoshop for graphic design.
Key Characteristic: Application software is characterized by its user-centric design. These programs are built to fulfill specific needs, making them highly beneficial in an array of contexts from business to education.
Unique Feature: They often come with various customizations that enhance user experience, allowing tailored workflows. Advantages here include ease of use, but a downside might be that application software can become outdated quickly, necessitating regular updates.
Utility Software
Utility software focuses on maintenance and optimization tasks rather than performing general computational tasks. Examples include antivirus programs and disk management tools.
Key Characteristic: The hallmark of utility software is its support functionalities. These programs often help to enhance system performance, security, and efficiency.
Unique Feature: Many utilities offer automated functions that handle routine tasks, thus freeing users to focus on their core activities. However, while they provide essential services, an over-reliance on utilities can lead to bloated systems and sluggish performance too.
In summary, the array of computer programs—spanning system, application, and utility types—forms a complex ecosystem that ensures efficient operation and tailored user experiences. By mapping this landscape, students and budding programmers can better grasp which tools serve their needs, whether they aim to develop software, provide tech support, or simply aspire to better understand the digital world they live in.
The Role of Algorithms
Algorithms are the bedrock of computer programs, orchestrating the logic and flow of processes within software. They represent systematic, step-by-step procedures designed to perform calculations, data processing, and automated reasoning tasks. In a nutshell, algorithms turn abstract concepts into concrete tasks that machines can execute. This section aims to illuminate the significance of algorithms in programming by outlining their definitions and importance.
Defining Algorithms
In the simplest terms, an algorithm is a defined set of rules or instructions that outlines how to achieve a particular outcome. Consider a recipe. Just as a recipe details the steps needed to bake a cake, an algorithm delineates the steps necessary to complete a task in a computer program. It includes inputs, a sequence of operations, and outputs. Here’s a basic example:
- Input: Gather ingredients (data).
- Process: Mix ingredients (operations).
- Output: Bake the cake (resulting data).
Algorithms can vary widely in complexity. From sorting numbers to performing complex calculations in machine learning, they operate in various domains, always vying for efficiency.
"An algorithm must be unambiguous and have a clear stopping point. Without these features, it can become a tangled mess, akin to being lost in a maze."
Importance of Algorithms in Programming
Algorithms hold immense value in the programming landscape for several reasons:
- Problem-Solving Framework: They provide a structured approach to solve problems efficiently, breaking down intricate tasks into manageable steps.
- Performance Optimization: Efficient algorithms can significantly reduce the time and resources needed, which is crucial in today's fast-paced digital world. Think about sorting algorithms. For large datasets, quicksort usually outperforms bubble sort by a large margin, saving valuable processing time.
- Reusability: Once an algorithm is created, it can be reused for various applications, akin to a tool in a toolbox that can serve different purposes depending on the task at hand.
- Scalability: Well-designed algorithms can handle increased input sizes. For instance, as a website grows, having efficient algorithms ensures it doesn't crumble under load.
Consequently, understanding algorithms is not merely an academic exercise. It serves as a vital skill for anyone delving into programming, shaping their ability to create effective and robust computer programs.
Programming Languages and Their Function
Programming languages serve as the backbone of computer programs, acting as the bridge between human comprehension and machine execution. Their significance lies not only in their capacity to perform tasks, but in how they enable developers to express complex ideas succinctly for the machine to interpret. Each language comes with its set of rules and syntax, akin to how different spoken languages have grammatical structures.
When breaking down the essence of programming languages, one finds the benefits extend beyond mere communication with the hardware. They provide a framework for developers to innovate, build applications, and solve problems. Choosing the right programming language can often determine the efficiency of the software being developed and the ease with which developers can maintain and expand it.
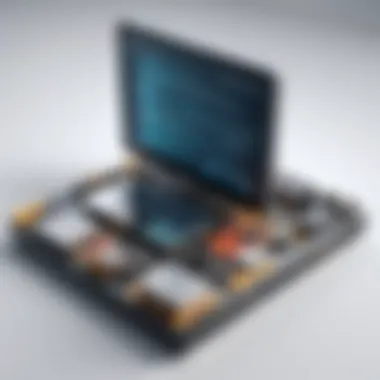
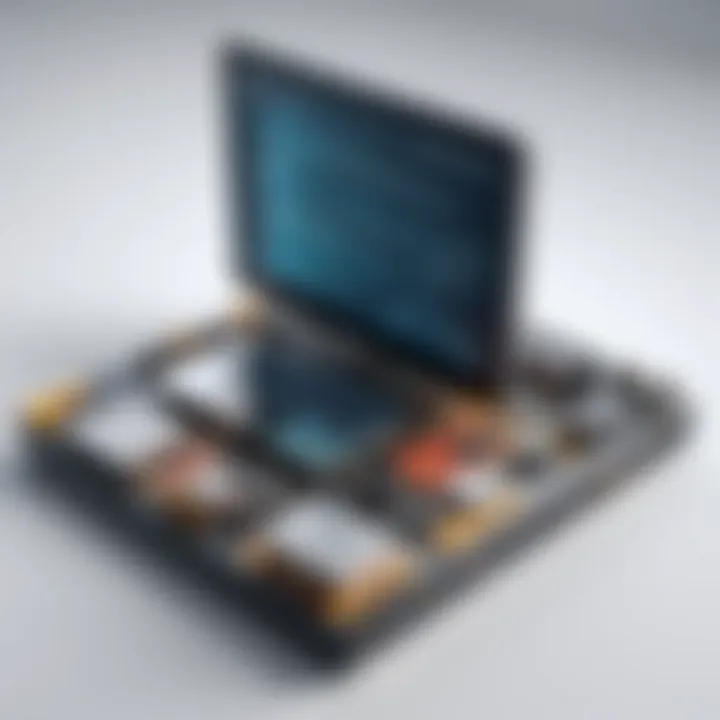
As we explore the different facets of programming languages, we should appreciate their role in shaping the software ecosystem and fostering collaboration among programmers.
What is a Programming Language?
A programming language is a formal system that consists of a set of instructions for communicating with a computer. These languages allow programmers to dictate the behavior of a program through terms a machine can process. It’s much like having a conversation in a foreign language where the syntax and vocabulary must be followed meticulously to convey the correct meaning.
In essence, programming languages can be thought of as tools that simplify the process of giving instructions to computers. Recognizing various programming constructs is fundamental for beginners embarking on their coding journey. With the right programming language, the complex becomes understandable, enabling the development of everything from simple scripts to intricate applications.
High-Level vs. Low-Level Languages
Understanding the distinction between high-level and low-level languages is critical in navigating the programming landscape. High-level languages, like Python and Java, are designed to be easy for humans to read and write. They abstract away much of the underlying complexity, allowing programmers to focus on problem-solving rather than intricate hardware details.
In contrast, low-level languages, such as Assembly, interact more directly with the computer's hardware. They offer less abstraction and demand a particular technical aptitude, representing more closely how a computer processes information. While low-level programming can yield highly optimized code, it is often more time-consuming and less intuitive compared to higher-level languages.
Understanding these differences can significantly influence a programmer's choice of language based on the project's specific needs.
Popular Programming Languages Today
Java
Java is revered for its portability across different computing platforms, thanks to its Write Once, Run Anywhere capability. This means that code written in Java can run on any device that supports the Java Virtual Machine (JVM). This pivotal feature makes Java remarkably beneficial for cross-platform development, aligning well with the needs of modern applications. With strong community support and a wealth of libraries, Java consistently ranks among the most popular programming languages.
The unique feature of Java is its object-oriented structure, which fosters code reusability and modularity. However, while it is a robust choice, Java can be resource-intensive, which may pose challenges in environments with limited resources.
C language is often labeled the "mother" of modern programming languages due to its influential role in shaping later languages. Its syntax is straightforward, making it a fantastic choice for learners to grasp fundamental programming concepts. One of its key characteristics is its ability to manipulate hardware directly, providing a strong underpinning for developing operating systems and system software.
The unique aspect of C is its efficiency and performance, enabling developers to create high-performance applications. Yet, its power comes with a caveat—increased complexity in memory management that can lead to vulnerabilities if not handled correctly.
++
C++ extends the capabilities of C, integrating object-oriented programming features that promote code organization and reuse. This language is a preferred choice in sectors requiring high efficiency, such as game development and performance-critical applications. One key characteristic of C++ is its support for both procedural and object-oriented paradigms, granting flexibility to developers.
However, the added complexity of C++ can lead to longer development times and steeper learning curves when compared to simpler languages.
Python
Python has gained a significant foothold in programming communities, primarily due to its simplicity and readability. It allows developers to express concepts in fewer lines of code compared to many other languages, facilitating a faster development process. The fundamental advantage of Python is its extensive standard library, along with the richness of third-party libraries available for tasks ranging from web development to data analysis.
The unique attribute of Python is its dynamic typing and ease of use, making it a natural choice for beginners as well as seasoned professionals. However, its interpreted nature may cause performance issues in scenarios where execution speed is critical.
The Process of Writing Code
Writing code is akin to crafting a unique narrative that communicates with both human readers and machines. This process isn't just about reflecting ideas in a programming language's syntax; it encompasses a systematic approach that ensures clear instruction and efficiency. The importance of this phase cannot be overstated as it lays the groundwork for what transforms concepts into functional applications.
Understanding Syntax
Syntax refers to the set of rules that defines how code must be written in a particular programming language. Think of it as the grammar of programming; just as a sentence needs a subject, verb, and object to make sense, a code must adhere to its language's syntax rules.
- Clarity: A well-structured syntax can significantly reduce errors, making the code easier to read and maintain. Misplaced brackets or incorrect keywords can lead to frustrating bugs that are time-consuming to resolve.
- Language Specifics: Each programming language has its own syntax. For instance, Python uses indentation to define blocks of code instead of braces, which are common in languages like C or Java. Learning the nuances of syntax across different languages enhances one's adaptability as a programmer.
- Consistencey: Utilizing established syntax makes it easier for others to read and understand your code. This is particularly crucial when collaborating in teams where clear communication is vital.
Understanding the nuances of syntax not only boosts accuracy in programming but fosters an environment where ideas can flow freely into functional code. With a firm grasp, a student or new programmer will find themselves building up from simple concepts to complex applications with more confidence.
Best Practices in Coding
Taking the time to establish good coding practices can save vast amounts of time and frustration down the road. This seems like common sense, but it is often overlooked by newcomers eager to see their code in action.
- Comment Your Code: Just like authors add notes or footnotes in their books, programmers should document their thought processes. Clear comments can guide others through complex logic and identify the purpose of specific code blocks. Consider something like this:
This function calculates the factorial of a number
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1)