Essential Algorithms to Master for Technical Interviews
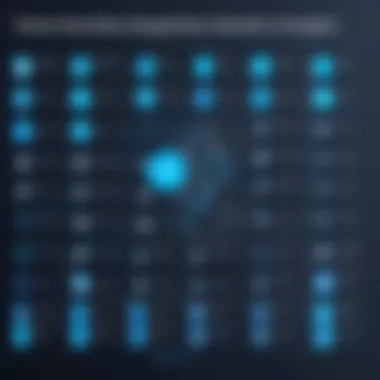
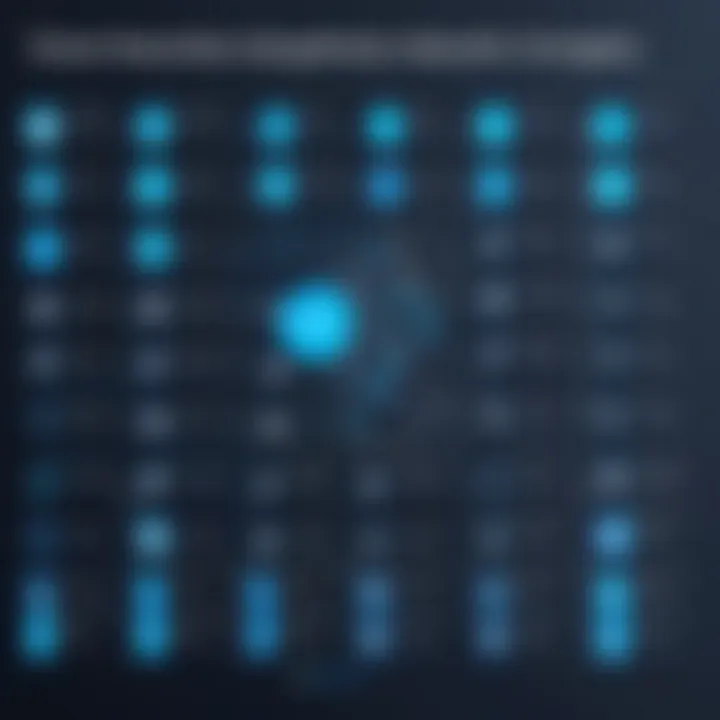
Intro
Algorithm interviews can often feel overwhelming to those preparing for technical job positions. Whether you're a seasoned dev or a newbie, understanding algorithms is crucial. So, let’s break it down in a way that’s digestible and relatable.
First off, let’s clarify what we mean by algorithms. At its core, an algorithm is just a step-by-step procedure for solving a problem. Think of it as a recipe in cooking; you need the right ingredients and instructions to end up with a delicious dish. Likewise, mastering algorithms is about knowing the right tools and how to apply them effectively.
Throughout this exploration, we will delve into some of the most prevalent algorithms that you might encounter during your technical interviews. We'll look at how these algorithms are structured and how they can be applied in real-world situations. In addition, we'll touch upon essential concepts that underpin their effectiveness. Today’s job market demands not just memorization of algorithms but an understanding of their functional role in programming.
Understanding this topic has far-reaching implications. Developers are often assessed on their problem-solving abilities, and algorithms form the backbone of many solutions they provide. So, get ready as we embark on this journey to unravel essential algorithms and equip yourself for the challenges ahead.
Key Points to Discuss
- What are Algorithms? An overview of algorithms and their definitions.
- Real-world Applications: How algorithms are used in various industries.
- Common Types: A look at the different types of algorithms that are popular in interviews.
- Problem-solving Techniques: Strategies to tackle algorithm-related questions effectively.
As we continue, you’ll find these concepts intertwined with practical examples designed to solidify your understanding. Let’s roll up our sleeves and get into the meat of this.
"Knowledge of algorithms is like having a compass in the vast ocean of programming. It directs you toward the right path, helping you to navigate challenges."
Stay tuned as we methodically dissect each component of this critical subject.
Understanding Algorithms
Understanding algorithms is crucial when diving into the world of software development and programming. Algorithms serve as the backbone of how data is processed and manipulated, impacting both performance and efficiency. They are not just theoretical concepts; they directly influence real-world applications like search engines, navigation software, and even social media recommendations. That is the power of algorithms—they make sense of chaos and help us derive meaningful conclusions from data.
Every programmer should grasp the significance of algorithms. When it comes to interviews, having a solid understanding of these concepts can set candidates apart from the crowd. Hiring managers are not just looking for technical skills; they want to see analytical thinking, problem-solving skills, and the ability to optimize code. Hence, this section will break down the fundamental aspects of algorithms, focusing on their definitions, their evaluation metrics, and practical implications.
Definition and Importance
An algorithm, in simple terms, is a step-by-step procedure for solving a problem or carrying out a task. Think of it as a recipe—follow it precisely, and you get the desired result. In programming, algorithms allow developers to write clear and efficient code, making it easier to maintain and understand.
Why is this important? Well, in tech interviews, candidates are often presented with problems that require a solid understanding of algorithms to solve. Demonstrating clarity in defining and applying algorithms can showcase a candidate's depth of knowledge, making them a desirable hire.
How Algorithms Are Evaluated
An algorithm isn’t just evaluated based on whether it works correctly; its efficiency is also of utmost importance. This evaluation focuses primarily on time and space complexity. Understanding these elements allows programmers to make informed decisions about which algorithm to use for a particular task.
Time Complexity
Time complexity measures how the execution time of an algorithm increases relative to the input size. It's expressed with big O notation, which provides an abstraction that simplifies this understanding.
For example, an algorithm with a time complexity of O(n) signifies that execution time grows linearly with the input size. Time complexity is crucial, especially in interviews, because it illustrates a candidate's ability to optimize their solutions effectively. If a solution has excessive time complexity, it might be unsuitable for large datasets.
In essence, having a grasp on time complexity can mean the difference between a functional program and a sluggish one.
Space Complexity
Space complexity focuses on the amount of memory an algorithm consumes in relation to the input size. Like time complexity, it's also denoted in big O notation. Knowing the space complexity is vital for writing efficient code, especially in environments with limited resources. If an algorithm requires excessive space to function, it might not be practical for implementation, particularly in resource-constrained devices.
An algorithm with low space complexity will typically be preferred, given that memory efficiency is often as crucial as execution time. This aspect is often overlooked but plays a vital role in creating robust applications.
Big O Notation
Big O notation is a mathematical concept used to describe the upper limit of an algorithm's performance concerning input size. It can provide clarity on performance trends without getting lost in specifics. For instance, an algorithm that runs in O(1) time indicates constant time, which is the pinnacle of efficiency, whereas an O(n^2) algorithm might become impractical for large datasets.
Understanding Big O notation is not just important; it’s essential. It allows programmers to communicate about performance in an efficient manner. In coding interviews, presenting solutions that leverage this notation can impress interviewers, demonstrating that the candidate not only knows how to write code but also understands its performance implications.
Sorting Algorithms
Sorting algorithms play a crucial role in computer science and programming interviews. They not only help to arrange data in a predictable order but also form the backbone of more complex algorithms and data structures. Mastering sorting algorithms equips candidates with the ability to tackle a wide range of programming problems, particularly in technical interviews where problem-solving skills are put to the test.
Efficiently sorting data can significantly enhance the speed and performance of applications, particularly when dealing with large datasets. Interviewers often seek candidates who understand various sorting methods because this knowledge reflects a deeper comprehension of fundamental algorithmic concepts. Moreover, sorting algorithms lay the groundwork for understanding how to optimize solutions and manage computational resources effectively.
Overview of Sorting Algorithms
Sorting algorithms are methods used to arrange elements in a particular order, often numerical or lexicographical. Various sorting algorithms come with diverse strategies and performance metrics, suitable for different contexts and data types. Understanding these methods can help simplify tasks and solve a multitude of problems, making them an essential study area for anyone preparing for a technical interview.
Commonly Used Sorting Algorithms
Bubble Sort
Bubble Sort is often the first algorithm taught in many programming courses, primarily due to its simplicity. The unique feature of Bubble Sort is its method of comparing adjacent elements and swapping them if they are in the wrong order. While this algorithm qualifies as one of the simplest sorting methods, a key characteristic is its time complexity of O(n^2) in both average and worst-case scenarios. This places it as a less efficient choice for larger datasets. However, its ease of implementation and conceptual clarity makes it a beneficial entry point for beginners learning about algorithms.
Advantages:
- Simple and easy to understand
- Minimal coding effort required
Disadvantages:
- Inefficient for large datasets
- Average and worst-case performance are poor
Merge Sort
Merge Sort stands out due to its divide-and-conquer strategy, where the dataset is recursively divided into smaller segments until each part contains a single element. It then merges these segments back together in a sorted manner. Merge Sort ensures a time complexity of O(n log n) in all cases, making it an excellent choice for larger datasets. This stability and efficiency is why Merge Sort is popular in many professional applications.
Advantages:
- Consistent performance regardless of data
- Relatively simple to implement recursively
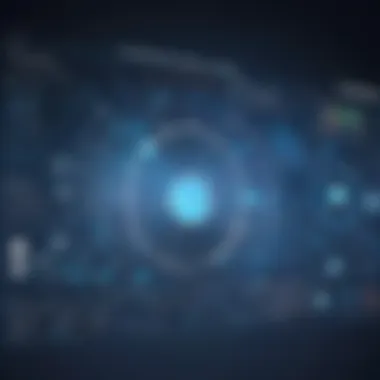
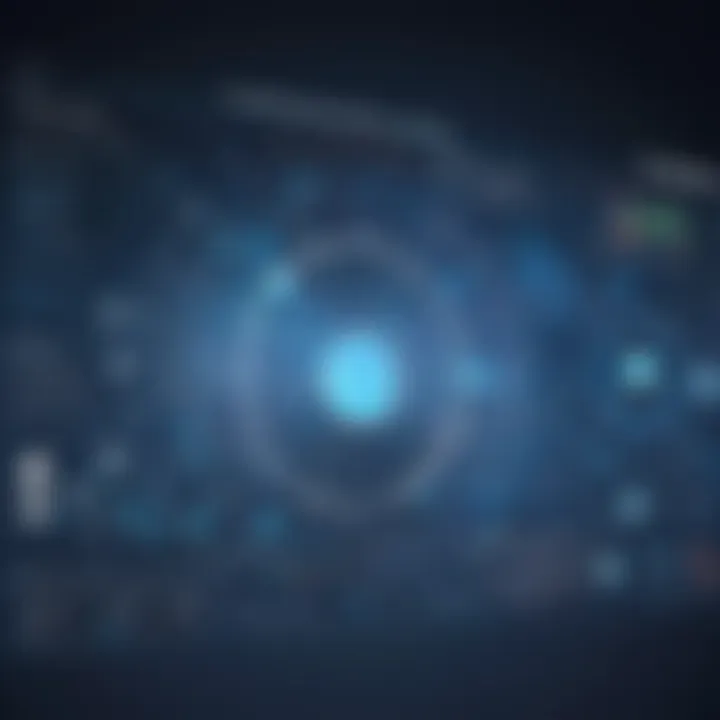
Disadvantages:
- Requires additional space, increasing memory usage
Quick Sort
Acknowledged for its speed, Quick Sort is a highly efficient sorting algorithm. It works on the concept of partitioning, where it selects a pivot, rearranges elements based on their relation to the pivot, and then recursively sorts the partitions. It generally achieves a time complexity of O(n log n) but can degrade to O(n^2) in certain scenarios, particularly with poor pivot choices. Because of its efficient average-case performance and low overhead, it’s favored in performance-focused applications.
Advantages:
- Fast average-case performance
- In-place sorting, requiring little extra memory
Disadvantages:
- Unstable sorting (equal elements may not retain original order)
- Worst-case performance can be poor
Insertion Sort
A great algorithm for small datasets or lists that are already partially sorted, Insertion Sort builds a final sorted array one item at a time. By comparing and inserting elements where necessary, it can achieve an average-case time complexity of O(n^2), though it runs in O(n) when the array is mostly sorted. It is simpler to implement and offers a practical solution in some real-world scenarios, making it a well-regarded option despite its inefficiency on larger lists.
Advantages:
- Excellent for small or partially sorted datasets
- Adaptive, as it can utilize pre-sorted elements
Disadvantages:
- Inefficient on larger lists
- Average and worst-case time complexity is poor
Selection Sort
Selection Sort is characterized by its straightforward strategy of repeatedly selecting the smallest (or largest) element from the unsorted portion of an array and moving it to the beginning. While its time complexity remains O(n^2) for all cases, the benefit lies in its space efficiency, as it does not rely on additional memory allocations. It is a good introductory algorithm that helps solidify foundational sorting techniques.
Advantages:
- Simple to understand and implement
- Requires little additional memory
Disadvantages:
- Poor efficiency on larger arrays
- Time complexity is steep
Comparison of Sorting Algorithms
When comparing sorting algorithms, it's vital to understanding performance nuances and best use cases.
Best Case Performance
The best-case performance of an algorithm refers to the scenario where the algorithm operates in the most optimal conditions. For instance, if a dataset is already sorted, algorithms like Insertion Sort can achieve a best-case complexity of O(n), whereas Bubble Sort also exhibits similar efficiency here. Understanding this can guide developers in choosing the right algorithm when they know specific data characteristics.
Advantages:
- Highlights the efficiency gaps between algorithms
- Useful in determining which algorithm to apply under optimal conditions
Disadvantages:
- Real-world data rarely meets best-case conditions
Average Case Performance
Average case performance allows for a more realistic assessment of algorithm efficiency. Here, complex factors come into play as it uses statistical analysis of performance across various inputs. Algorithms such as Quick Sort generally show a superior average-case time complexity of O(n log n), whereas others may lag, emphasizing the engineering decision required based on expected data characteristics.
Advantages:
- Reflects true efficiency much better than best-case
- Helps predict performance on random datasets
Disadvantages:
- Requires deeper understanding and analysis
- Can vary based on input distribution
Worst Case Performance
Worst-case performance examines scenarios where the algorithm performs the poorest. This often results when an algorithm faces extreme conditions - like sorted in reverse - effectively leading to maximum time complexities. For example, Quick Sort at its worst can reach O(n^2), emphasizing how pivot choice affects performance.
Advantages:
- Crucial for understanding algorithm limits
- Guides on error handling and edge cases
Disadvantages:
- May not reflect actual usage
- Can cause unnecessary concern over performance issues if not managed properly
Overall, sorting algorithms are foundational to understanding programming. Knowing when and how to use these diverse methods can deeply influence performance and resource allocation, crucial knowledge in interviews.
Searching Algorithms
Searching algorithms play a critical role in the realm of computer science and software development. These algorithms empower us to locate data within a vast sea of information swiftly and efficiently. In technical interviews, understanding search algorithms not only reflects one’s ability to solve problems but also highlights a candidate's knowledge of how to manipulate data structures effectively. Interviewers frequently pose questions about these algorithms because they directly connect to real-world applications, especially in data retrieval, retrieval speed, and overall performance. By mastering these algorithms, candidates can exemplify their readiness for software engineering roles.
Preface to Search Algorithms
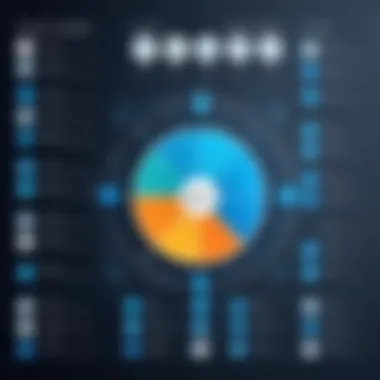
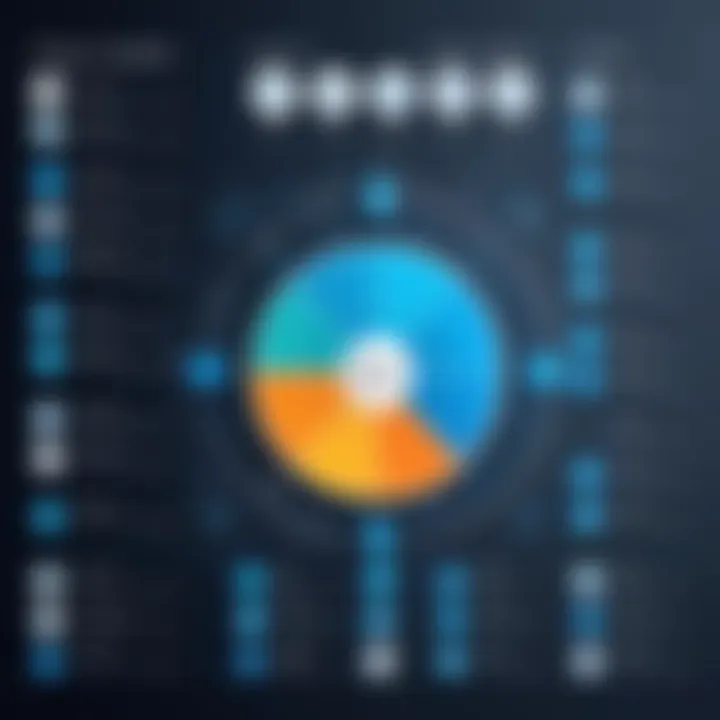
At its core, a search algorithm is a method for locating specific data within a data structure, like an array or list. As straightforward as it may seem, the choice of search algorithm can significantly impact performance. Whether you're sifting through a short list of names or navigating a complex graph, understanding the nuances of each searching strategy is pivotal. Algorithms exist to cater to various scenarios, ensuring not just functionality, but optimal performance.
Types of Search Algorithms
Linear Search
Linear search is the most basic of search algorithms. This method involves scanning each element in a data set until the desired item is found or the end of the list is reached. A key characteristic of linear search is its simplicity—no preprocessing of data is required. As such, it’s a popular choice for small data sets or unsorted lists where quick implementation is preferred over efficiency.
However, this simplicity comes with its downsides. The average time complexity of a linear search is O(n), which means performance can plummet with larger lists.
Binary Search
Binary search is a more efficient algorithm, applicable to sorted lists. The process begins by examining the middle element of the array. If the target value is less than the middle element, the search continues in the left subarray; if more, it shifts to the right. This divide-and-conquer strategy significantly reduces the number of comparisons necessary to locate an item.
With a time complexity of O(log n), binary search is a great choice when you have access to sorted data, balancing speed with lower resource consumption. Yet, the necessity of a sorted list can make its initial implementation a touch tricky depending on one’s data scenario.
Depth-First Search
Depth-First Search, often abbreviated as DFS, is a graph-based searching technique. It ventures as far down a branch of the graph as possible before backtracking, which is akin to exploring an intricate maze. A hallmark of DFS is its space efficiency, as it requires less memory than some alternative approaches.
The algorithm is extensively used in various applications, including web crawling and artificial intelligence. However, it can become inefficient with very deep or dense graphs since it doesn’t guarantee the shortest path. Therefore, while DFS is powerful in many contexts, candidates should also be aware of its limitations and when it might not be the best tool for the job.
Breadth-First Search
Breadth-First Search, or BFS, traverses a graph level by level. Starting from the root node, it explores all neighbors before diving deeper. This particular method shines in scenarios requiring the shortest path in an unweighted graph, making it a go-to choice in numerous applications like networking and route finding.
One of the trade-offs of BFS is its demand for more memory—particularly when dealing with wider graphs. The breadth-first approach ensures thorough exploration, but at the cost of additional resources.
Application Scenarios for Search Algorithms
Searching algorithms find their niche across various industries and technologies. Here are some scenarios where these algorithms come into play:
- Database Queries: Efficient searching directly impacts the speed of data retrieval in databases.
- Recommendation Systems: Algorithms help find user preferences in large datasets, powering personalized experiences.
- Networking: Graph search algorithms facilitate routing and pathfinding in complex networks.
- Game Development: Search algorithms assist in AI decision-making for character movements or pathfinding in gaming environments.
Dynamic Programming
Dynamic programming has emerged as a fundamental concept in computer science, especially pertinent during technical interviews. It's not just another item on the checklist of algorithms; it represents a technique that can significantly simplify complex problems. This approach breaks problems down into smaller, manageable subproblems and solves each just once, storing the results for future reference. This efficiency is why dynamic programming is a prominent feature in interview settings, where candidates are often assessed on their problem-solving acumen under time constraints.
The core strength of dynamic programming lies in its ability to optimize recursive solutions. It transforms exponential time calculations into polynomial time, allowing for quicker and more effective problem resolution. Furthermore, understanding dynamic programming's intricacies can showcase candidates’ analytical skills, critical thinking, and comprehensive understanding of algorithms—all essential qualities for prospective software developers.
Defining Dynamic Programming
Dynamic programming (DP) can be defined as a method for solving complex problems by breaking them down into simpler subproblems in a recursive manner. It is characterized by two main properties: overlapping subproblems and optimal substructure. These elements allow problems to be solved efficiently, avoiding redundant calculations by storing the results of already-solved subproblems.
Key Principles of Dynamic Programming
Overlapping Subproblems
At the heart of dynamic programming is the concept of overlapping subproblems. This means that the same subproblems are solved multiple times in the process of solving larger problems. Consider the example of calculating Fibonacci numbers—each number is derived from the sum of the two preceding ones. Using a naive recursive approach leads to many recalculations of the same Fibonacci numbers, making it extremely inefficient.
The key characteristic of overlapping subproblems is that it enables you to store solutions for these subproblems. By caching these results, or using techniques like memoization, overall computation time can be drastically reduced. This is what makes overlapping subproblems such a beneficial choice for tackling a wide range of algorithmic challenges in interviews. Having this stored information allows for quick lookups, saving precious time and resources.
Optimal Substructure
Optimal substructure is another crucial principle in dynamic programming. A problem exhibits optimal substructure if an optimal solution can be constructed efficiently from optimal solutions of its subproblems. For instance, in the case of the shortest path problem, if you find the shortest path to a node, you can leverage that to find the path to subsequent nodes, creating an efficient route with minimal overall cost.
This characteristic makes optimal substructure a vital component of dynamic programming. It provides candidates with a framework to think systematically about problems. By breaking down the problem into smaller parts and ensuring each part is optimally addressed, the final solution is guaranteed to be optimal as well. This aspect can showcase a programmer's depth of thought and methodical approach during interviews, reflecting their capability of handling complex real-world problems.
Famous Problems Using Dynamic Programming
Fibonacci Sequence
The Fibonacci sequence is one of the simplest yet most illustrative examples of dynamic programming. It simply states that each number is the sum of the two preceding ones. This is a classic case of overlapping subproblems, as many Fibonacci numbers are recalculated multiple times in a naive recursive solution. By applying dynamic programming, you can compute the sequence much more efficiently by storing previously computed numbers—and thus solving the problem with a time complexity of O(n) instead of O(2^n).
This makes it a valuable example in interviews, allowing candidates to demonstrate their grasp of recursion, optimization, and the practical application of dynamic programming techniques.
Knapsack Problem
The Knapsack problem illustrates how dynamic programming can be applied to solve optimal resource allocation issues. In this hypothetical scenario, you need to maximize the value you can carry in a knapsack without exceeding its weight limit. By breaking down the problem into smaller pieces, where you evaluate the most valuable combinations of items, dynamic programming allows for an efficient solution that evaluates all possibilities yet stores results to avoid redundant calculations.
The Knapsack problem remains a popular choice in interviews since it encapsulates resource management, optimization, and the real-world challenges many developers face in cost-benefit analysis scenarios.
Longest Common Subsequence
The Longest Common Subsequence (LCS) problem explores how to find the longest subsequence common to two sequences. This problem is significant as it highlights the utility of dynamic programming in a field such as bioinformatics, where it is often used to analyze DNA sequencing.
By employing dynamic programming, one can break down the LCS into smaller problems of finding LCSs of prefixes and then combining these results. This method reveals not only the optimal solutions more efficiently but also sheds light on the relationship between the two sequences, providing depth to the analysis.
Graph Algorithms
Graph algorithms play a significant role in understanding complex relationships in data structures and systems. In the realm of interviews, grasping these algorithms can set candidates apart, showcasing their analytical skills and problem-solving prowess. Graphs, which consist of nodes and edges, are instrumental in representing various real-world scenarios such as social networks, navigation systems, and project scheduling. By mastering these algorithms, candidates will not only prepare themselves for technical evaluations but also gain insights into how problems can be tackled efficiently through intelligent design and strategy.
Understanding Graphs
Graphs are versatile tools that model relationships between paired entities. Each graph comprises vertices (nodes) and edges (connections between nodes). Understanding graphs begins with distinguishing between different types: directed graphs have edges with a specified direction, while undirected graphs feature edges that do not. Moreover, graphs can be weighted, where each edge carries a value representing cost or distance, or unweighted, where all edges are considered equal.
In technical interviews, comprehending the fundamental building blocks of graphs is crucial. For instance, a candidate should easily identify how data flows across a network or how to assess which connection offers the most efficient path. This knowledge is foundational and leads into deeper studies of algorithm applications.
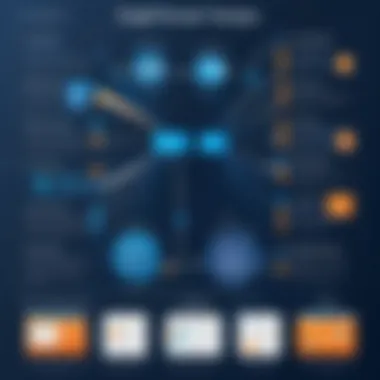
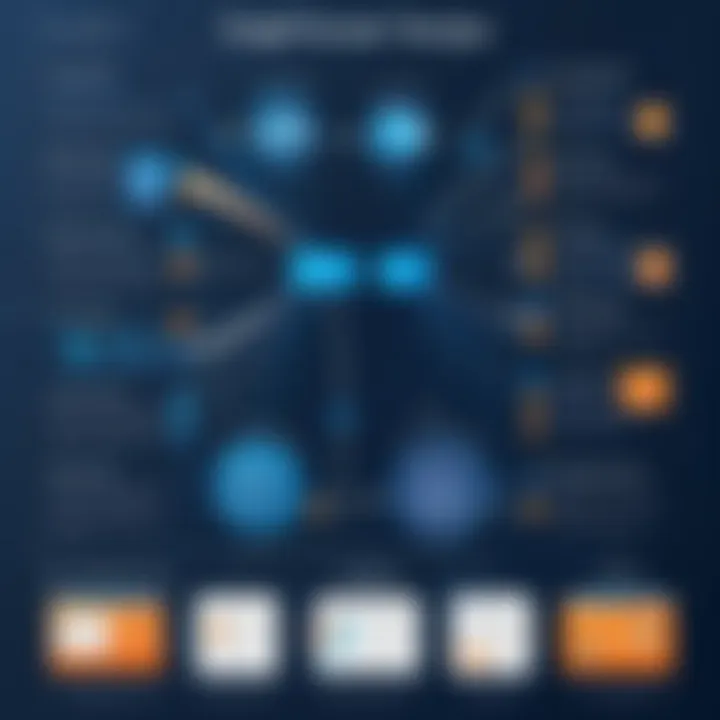
Key Graph Algorithms
Several algorithms stand out in graph theory, often appearing in interview questions. These algorithms help solve various problems, from finding shortest paths to calculating minimum spanning trees.
Dijkstra’s Algorithm
Dijkstra’s Algorithm is a fundamental approach for finding the shortest path in a weighted graph. This algorithm operates under the principle of relaxation—updating the shortest known distance to each vertex by considering its neighboring vertices. One key characteristic of Dijkstra’s Algorithm is that it guarantees the shortest path to the vertex with the smallest known distance at each step.
This algorithm is particularly favored in situations where there are no negative weight edges, making it reliable and effective for various applications, like GPS navigation systems. Although it has a relatively high time complexity in cases of dense graphs, its efficiency greatly increases with implementations like priority queues, making it a common choice among programmers. Understanding its implementation details can aid candidates in interviews, guiding them through potential complexity analysis questions and edge cases.
Kruskal’s Algorithm
Kruskal’s Algorithm focuses on finding a minimum spanning tree for a weighted, undirected graph. Its process involves sorting all edges in ascending order according to weight and adding them one by one while avoiding cycles. A standout feature of this algorithm is its ease of use with disjoint-set data structures, making it efficient for large datasets.
Kruskal's tends to be invaluable when there is an emphasis on edge weights. It’s notably advantageous in network design applications, such as reducing costs in telecommunications. However, its reliance on sorting can result in higher time complexity with significant data, a factor candidates should weigh in their evaluations.
Prim’s Algorithm
Prim's Algorithm, similar to Kruskal’s, is another method for determining a minimum spanning tree. However, it unfurls its efficiency by starting from a single node and expanding outward, continually selecting the smallest edge connecting a vertex in the tree to a vertex outside of it. The key characteristic here is its greedy nature—it always makes a choice that seems the best at that moment.
This algorithm is particularly beneficial for dense graphs, ensuring practical performance due to a focus on immediate optimization. It’s often a preferred choice in transportation and networking applications, allowing interview candidates to showcase a broader range of problem-solving skills. While Prim’s offers substantial advantages, it can lead to inefficiencies in sparse graphs where a different algorithm might perform better.
Practical Applications of Graph Algorithms
Graph algorithms aren't just academic exercises; they have practical significance across multiple domains. Here are a few applications:
- Social Network Analysis: Connecting users and analyzing their interactions.
- Route Finding: Navigation systems use algorithms to determine the quickest paths.
- Network Connectivity: Assessing the robustness of networks by finding paths or clusters within.
- Project Management: Using graphs to represent tasks and dependencies enhances project scheduling.
Ultimately, understanding graph algorithms equips candidates with analytical strategies that can apply to a multitude of real-life scenarios. By grasping the nuances of these algorithms, students and emerging programmers are better prepared to tackle a variety of challenges in the tech industry.
Practical Tips for Interview Preparation
Preparing for technical interviews, especially those revolving around algorithms, can often feel like a high-stakes game. The importance of being well-prepared cannot be overstated; it forms the backbone of any successful interview strategy. This section will shine a light on various practical tips that can equip candidates to navigate the choppy waters of tech interviews.
Engaging with algorithms is not merely a procedure; it’s about developing a mindset geared for problem-solving. Having a set of practical tips at your disposal can lead to tremendous confidence on the big day. These tips include flexibly structuring your study plan, focusing on quality over quantity in practice, and strategically examining your weak points to foster growth.
Resources for Learning Algorithms
Leaning into various resources can make all the difference. This can range from textbooks, online courses, to interactive learning platforms. The growing proliferation of online content has made it possible for anyone with an internet connection to access top-quality educational material. Engaging with diverse kinds of resources helps to accommodate different learning styles. Some may prefer visual aids, while others may find coding exercises more enlightening. The crux is, don’t lock yourself into just one type of learning.
Practice Problems and Platforms
LeetCode
LeetCode has gained traction for its abundant coding problems that span various difficulty levels. This platform serves as an excellent opportunity to practice and refine algorithm skills, catering especially well to interview prep. A key characteristic of LeetCode is its vast repository of problems, which not only covers classic questions seen in interviews but also new ones that can present a competitive edge.
One unique feature is the "discuss" section, where users share solutions and strategies, creating a supportive community. However, it can feel overwhelming for beginners due to its extensive problem bank. Prioritizing the right problems is essential, rather than jumping into the deep end without proper guidance.
HackerRank
HackerRank is praised for its easy-to-navigate interface and the wide array of coding challenges that focus not only on algorithms but on data structures and even technical skills relevant for interviews. Its gamified approach makes practicing engaging, as many users enjoy the sense of competition it fosters. One notable advantage here is its real-time coding environment, granting quick feedback on attempts. Yet, some may find the platform’s focus on speed can sometimes overshadow the depth of understanding.
CodeSignal
CodeSignal has made a name for itself by providing a unique assessment tool that centers on coding skills and potential. This platform is distinctly focused on helping users improve their coding abilities through varied challenges, applying algorithms in practical scenarios. The standout feature is its "Arcade" mode, where learners can tackle problems in a game-like environment. However, some argue it's less beneficial for those looking for direct interview coaching as compared to more traditional platforms.
Mock Interviews and Coding Challenges
Engaging in mock interviews can be a game-changer. They allow aspiring candidates to simulate a real interview environment, providing not only a chance to practice problem-solving but also to enhance communication skills. Participating in coding challenges further aids in building muscle memory for algorithms, enabling individuals to think swiftly and critically when under pressure.
A robust preparation strategy involves combining resource utilization, practice platforms, and mock interview experiences. As you stitch together your preparation plan, remember: the goal isn’t just to solve problems but to embody the thinking process that allows for effective, creative solutions.
"In the world of algorithms, it’s not just the destination that matters; it’s the path you take to get there."
Ultimately, whether you are navigating sorting algorithms or searching techniques, being well-prepared is the key to cracking even the toughest technical interviews.
End
In the world of software development, mastering algorithms can feel like negotiating a labyrinth. Yet, understanding these algorithms is not merely an academic exercise; it is pivotal for anyone looking to make their mark in technology-focused roles. The conclusion of this article ties together the essential threads woven throughout the discussion, notably emphasizing the importance of algorithms in technical interviews.
Algorithms are the backbone of problem-solving in programming. Their significance in interviews stems from their ability to demonstrate a candidate's logical thinking and analytical prowess. Employers often seek individuals who can not just memorize code but can also employ algorithms to create efficient, effective solutions to real-world problems.
Highlights from this article also signify that algorithms extend beyond the confines of an interview room; they hold significant weight in software design and application development. Understanding how to manipulate data structures and algorithms can lead to creating software that performs better and scales more efficiently. Here are some key benefits of understanding these concepts:
- Enhanced Problem-Solving Skills: Exposure to different algorithms enables better strategizing in problem resolution.
- Increased Job Opportunities: Candidates with a solid grasp of algorithms are often viewed as more competent.
- Real-World Application: Skills gained through learning algorithms can lead to improved performance in work projects.
Reflecting on such elements, it becomes clear that diving deep into the nuances of popular algorithms can pay dividends not just in interviews but throughout one's career.
Summary of Key Points
In this exploration, several core topics have been discussed, with each section providing valuable insights:
- The importance of algorithms in technical interviews, highlighting their role in evaluating candidates.
- An overview of various sorting and searching algorithms, crucial for efficient data organization and retrieval.
- A comprehensive look into dynamic programming and its key principles, which offer strategic ways to tackle complex problems.
- An engagement with graph algorithms that are fundamental for operations involving networks.
- Practical tips and resources aimed to enhance preparation for coding interviews, elevating one’s readiness and confidence.
This knowledge builds a strong foundation for future learning and success in the tech industry.
Encouragement for Continuous Learning
The journey of mastering algorithms is ongoing. The tech landscape is ever-evolving, and what may be deemed effective today could be eclipsed by more efficient methods tomorrow. Therefore, it is essential to cultivate a habit of continuous learning. Here are some recommended strategies to keep the momentum going:
- Regular Practice: Engage with platforms such as LeetCode and HackerRank to solve problems regularly. The more you practice, the more intuitive algorithms become.
- Stay Updated: Follow relevant discussions and advancements on forums like Reddit or Facebook groups that focus on programming and algorithms.
- Collaborate and Share: Learning from peers can deepen understanding. Participate in coding meetups or workshops.
By adopting these approaches, individuals can sharpen their algorithmic thinking and problem-solving abilities, ensuring they are prepared not just for interviews but also for real-world applications in their careers.