Building Mobile Apps with React Native: A Comprehensive Guide
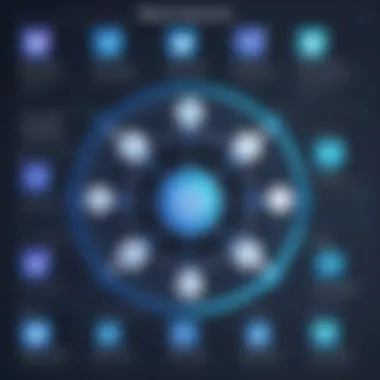
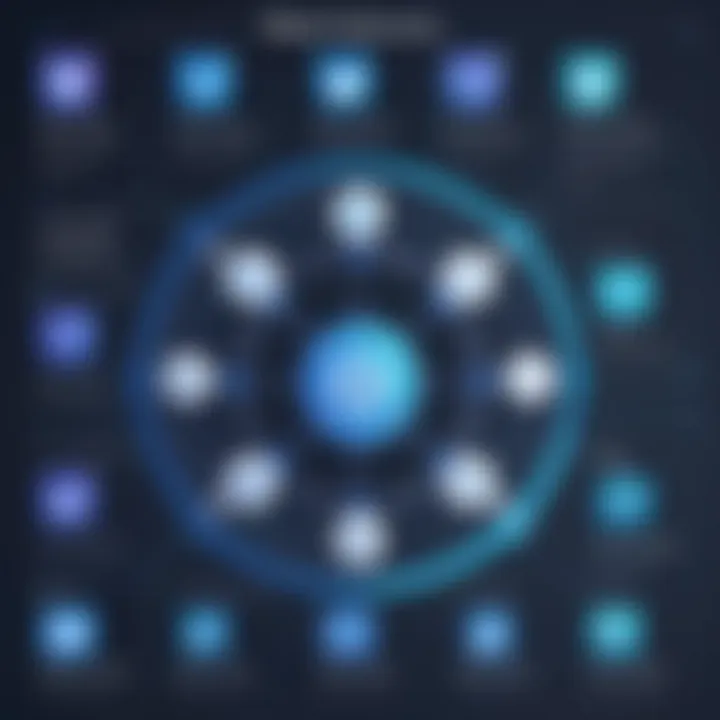
Intro
Creating mobile applications is not just a modern trend; itās an essential skill in todayās tech-savvy world. React Native has emerged as one of the leading frameworks for developing cross-platform applications efficiently. It allows developers to use JavaScript and React, leveraging their existing skills while reaching a broader audience by targeting both iOS and Android users simultaneously.
Prolusion to React Native
React Native, introduced by Facebook in 2015, was designed to allow developers to build truly native mobile apps using JavaScript and React. Instead of creating separate codebases for each platform, React Native enables the use of a single codebase, providing a cost-effective and time-saving approach.
History and Background
The inception of React Native stems from the need to unify mobile development. Before its release, developers often faced tough choices between native development and hybrid mobile frameworks. Facebookās own experiences with mobile speed and performance issues led to the decision to create React Native. The framework took inspiration from Reactās component-based architecture, a concept that has gained immense popularity in web development.
Features and Uses
React Native is renowned for its various features:
- Hot Reloading: This functionality allows developers to instantly see the results of the latest changes without recompiling the entire app.
- Native Components: React Native bridges the gap to native APIs, allowing for a smoother performance that mimics truly native apps.
- Cross-Platform Compatibility: Write once, run everywhereāthe mantra that resonates throughout this framework.
Due to these features, it garners attention from startups to big tech companies for various applications ranging from social media platforms to educational tools.
Popularity and Scope
The popularity of React Native in the developer community is evident, with many major companies like Instagram, Airbnb, and Uber Eats opting for it as their mobile development solution. It enjoys strong community support, which facilitates an expansive scope for learning and collaboration. As of late, React Native ranks as one of the top frameworks in developer surveys, demonstrating its reliability and functionality.
Basic Syntax and Concepts
Having an understanding of some basic concepts is essential for getting started with React Native. A firm grasp on these elements can lead to streamlined development processes and better app performance.
Variables and Data Types
In JavaScript, variables can be declared using , , or . Each of these declarations can behave differently:
- var: Function-scoped variable.
- let: Block-scoped variable, which is more modern and recommended.
- const: Also block-scoped, but for variables that wonāt change.
Popular data types in JavaScript include numbers, strings, booleans, objects, and arrays. Here is a quick example:
Operators and Expressions
JavaScript supports a range of operators including arithmetic, comparison, and logical operators, which are essential for performing tasks within your application.
Control Structures
Control structures like statements, loops, and statements help dictate how your code flows based on certain conditions. These are crucial when making decisions or iterating over data.
Advanced Topics
As one dives deeper, understanding advanced topics becomes essential for creating more complex functionalities within applications.
Functions and Methods
In JavaScript, functions can be declared in various ways, including function declarations and arrow functions. They are vital for code organization and reuse. For instance:
Object-Oriented Programming
React Native thrives on the principles of object-oriented programming. Objects can encapsulate data and behavior, promoting organization and modularity.
Exception Handling
Error handling is crucial for app stability and user experience. The blocks in JavaScript allow developers to manage exceptions gracefully, ensuring the application doesnāt crash unexpectedly.
Hands-On Examples
The best way to really grasp how React Native works is through hands-on examples.
Simple Programs
Creating a simple
Preamble to React Native
In the ever-evolving world of mobile app development, understanding the tools and frameworks at your disposal is crucial, and thatās where React Native steps in. It's not just another buzzword; itās a game-changer in how developers build applications for both iOS and Android platforms. React Native allows for the creation of natively-rendered mobile applications using JavaScript, which not only streamlines the development process but also enhances user experience significantly.
When diving into this subject, itās key to understand the core elements of React Native. By utilizing one set of code for both platforms, developers minimize redundancy and accelerate the time to market. The importance of this becomes especially clear when you consider the fierce competition in the app landscape; speed, efficiency, and quality can often determine success. Moreover, since React Native employs components that adapt to both operating systems, it leads to a more cohesive product.
This article will unpack the multifaceted nature of React Native, providing you an insightful look into not just how it works, but why it has garnered such a following among developers. From setting up the development environment to navigating with built-in frameworks, each aspect of React Native is critical in creating cross-platform applications that are not only functional but also engaging.
What is React Native?
React Native is a framework developed by Facebook that enables developers to build mobile applications using just JavaScript and React. Simply put, it bridges the gap between web and mobile development by allowing developers to write code once and deploy it across both iOS and Android platforms. This is rather revolutionary as traditionally, creating apps for different platforms would necessitate distinct codebases, each tailored to the specific operating system.
React Native achieves its magic by compiling native components, making applications feel smooth and responsive just as if they were developed using traditional native languages like Swift or Java. This hybrid nature of React Native is what sets it apart, offering the best of both worlds: native performance using a web-like development experience.
Key Benefits of Using React Native
The advantages of using React Native are numerous and impactful, especially for developers who are looking to optimize their workflow. Here are some of the essential benefits:
- Cross-Platform Development: Write once, run anywhere. This efficiency in code reuse is a boon to development teams.
- Community Support: With a vast global community, resources ranging from tutorials to open-source libraries are readily available.
- Hot Reloading: This feature enables developers to see the results of their changes in real time without losing the state of the application, streamlining the development and testing phases.
- Performance: Applications built with React Native are nearly indistinguishable from those developed using traditional methods. The interactive elements are designed to perform seamlessly, ensuring the user experience is not compromised.
- Third-Party Plugin Compatibility: It allows for custom modules and third-party libraries to be integrated easily, providing flexibility in features and functionality.
These highlights make React Native a preferred choice for many developers worldwide, especially those aiming to deliver high-quality mobile apps swiftly.
Comparison with Other Frameworks
While React Native is noteworthy, understanding how it stacks up against other frameworks can help you make an informed choice for your next project. What sets it apart?
- Versus Flutter: Flutter, Googleās UI toolkit, also allows cross-platform app development but uses Dart. While Flutter offers deep customization, React Native's familiarity with JavaScript gives it an edge, making it easier for web developers to jump in.
- Versus Native Development: Native app development offers unparalleled performance but requires separate codebases for each platform, which can be time-consuming and costly. React Native mitigates these issues by providing a single codebase while still ensuring native-like performance.
- Versus Xamarin: Xamarin also allows for cross-platform functionality but relies extensively on the .NET framework. React Native, on the other hand, is more geared towards those already comfortable with JavaScript and focuses on a more straightforward setup.
In essence, the choice may depend on your specific project requirements, team expertise, or desired features. Each framework has unique strengths but React Native consistently shines for its ease of use and community backing.
"Choosing the right framework can make or break your app development journey; knowing your options is key!"
Setting Up the Development Environment
Setting up the development environment is the cornerstone of building any mobile application, particularly when delving into React Native. This process ensures that developers have all necessary tools and frameworks at their fingertips, making the coding experience smoother and more efficient. A well-configured environment saves time, reduces errors, and enhances productivity. Without an adequate setup, even the best ideas can fall flat due to technical hiccups or compatibility issues.
When you think about it, a developerās environment is akin to an artistās studio. Just as artists need quality tools, a comfortable space, and the right materials to create, developers require powerful editors, libraries, and compilers tailored to their project's needs. Let's dive into the tools essential for kicking off your React Native journey.
Installing Node.js and npm
Node.js serves as the backbone of the React Native ecosystem, and npm is the package manager that allows you to manage your application dependencies. Installing Node.js is a straightforward process, yet it's all about choosing the right version. The LTS (Long-Term Support) versions are recommended for consistency and stability when developing.
- Download: Head to the Node.js website and download the latest LTS version suitable for your operating system.
- Installation: Run the installer, opting for the default settings unless you have distinct preferences. This action will simultaneously install npm, which comes bundled with Node.js.
- Verification: After installation is complete, you can verify both installations. Open your terminal or command prompt and type:You'll see version numbers for both if the installations were successful.
By having Node.js and npm installed, you're setting a solid foundation for managing libraries and running scripts vital for React Native applications.
Setting Up React Native
Once you've got Node.js and npm up and running, the next logical step is to install the React Native CLI. The CLI allows you to create and manage React Native projects effortlessly. The procedure is as easy as pie:
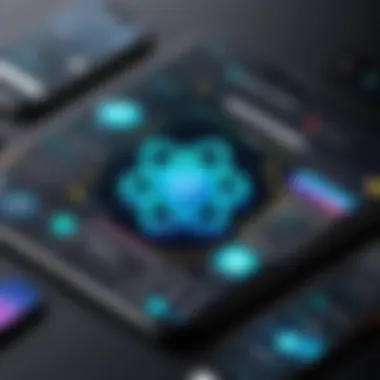
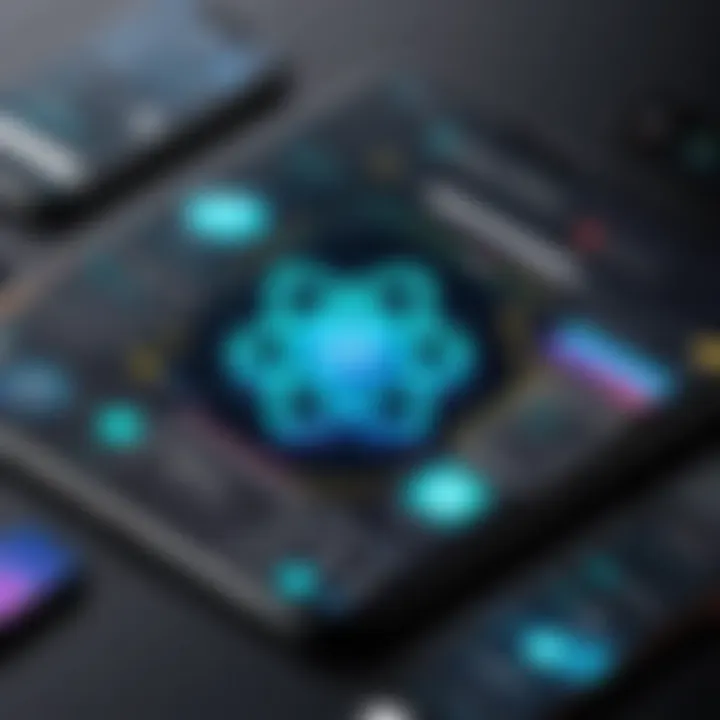
- Open Command Line: Start by opening your terminal (macOS/Linux) or command prompt (Windows).
- Run the Following Command: This command installs the CLI globally on your machine:
- Check Installation: You can ensure the correct installation of the CLI by checking the version:
Having the React Native CLI within reach streamlines project creation and management, empowering you to focus on coding rather than setup complications.
Configuring Android Studio and Xcode
For those aiming to build applications that run on both Android and iOS, configuring Android Studio and Xcode is non-negotiable. Each IDE provides the tools necessary to build and run React Native applications on their respective platforms.
- Android Studio:
- Xcode:
- Download and Install: Start by downloading Android Studio from the Android Developer site. Follow the installation instructions, which typically involve selecting a few options regarding SDKs and virtual devices.
- Setup the Android SDK: Open Android Studio and let it run its initial setup to install the necessary SDK packages. Make sure to set up an Android Emulator, which is vital for testing your app without needing a physical device.
- Download from App Store: If you're on a Mac, Xcode is available for free on the App Store. Install it as you would any other Mac application.
- Agree to Terms and Set Up: Upon the first launch, agree to the terms and let Xcode install necessary components. It will also prompt you to install some additional tools; do that to ensure you're ready to build iOS applications.
Having both IDEs configured correctly completes your development environment and ensures you can target both Android and iOS platforms without a hitch. While this setup may feel daunting initially, it pays off in spades for seamless development.
In summary, setting up your development environment defines the roadmap for a successful app. By carefully installing Node.js, setting up the React Native CLI, and configuring the necessary IDEs, you are nurturing a foundation for creativity and technological expression.
Understanding Core Concepts
In mobile app development using React Native, grasping the core concepts is essential. This understanding forms a bedrock for creating robust applications that can smoothly operate across platforms. These core teachings lay the groundwork for your journey, helping you navigate the intricate web of components, state management, and event handling.
Core concepts not only help beginners in breaking down complexities but also assist seasoned developers in optimizing their applications. Itās like knowing the ins and outs of your toolkitāwithout it, youāre likely to struggle when the time comes to assemble the pieces into a working app.
Components and Props
Components are the heart and soul of any React Native application. Think of them as the building blocks from which your app is crafted. Each component represents a part of your user interface, be it a button, a text input, or an entire screen. By mastering components, you gain the ability to craft reusable code, which can save you time and reduce bugs in the long run.
Props, or properties, are another critical aspect associated with components. They allow you to pass data and configurations down to your child components. This concept of passing data creates a flow that keeps dynamic content at the fingertips of developers. Itās almost like giving your components a specific job to complete based on the instructions you provided in the form of props.
For example, consider a simple button component designed to perform an action when clicked. By using props, you could customize its label or the function executed on press.
State and Lifecycle Methods
State serves as the memory of your component. It allows components to respond to user interactions or system events dynamically. For instance, think about an app where a user can toggle notifications on or off. Storing this preference in state ensures that the UI updates accordingly when changes occur.
Lifecycle methods, on the other hand, guide you through your componentās lifespanāfrom its birth in the application to its inevitable demise. These methods provide hooks at various points of its life, such as when the component mounts, updates, or unmounts. Knowing how to use lifecycle methods properly means you can optimize performance, like fetching data only when needed. Hereās a simple example:
Event Handling in React Native
When developing applications, users will inevitably interact with your app through taps, swipes, and input. Event handling is the mechanism that lets you respond to these user actions. In React Native, you can assign functions to events like , , or even By effectively handling these events, you ensure a responsive and engaging experience.
For instance, consider a text input that updates state every time the user types:
In this case, the event captures the userās input and automatically updates the state, reflecting changes in real-time.
An understanding of event handling is critical, as it not only shapes how your app feels but also maintains active communication between the user and your application.
"Mastering the fundamentals of React Native allows for greater flexibility and creativity in mobile app development."
Delving into these core concepts equips you with the tools and knowledge necessary to navigate the complexities of React Native efficiently. It sets a strong foundation for the more intricate aspects you'll face as you continue your development journey.
Building Your First Application
When venturing into the realm of mobile app development, the first project often carries significant weight. It serves as a rite of passage, a foundation where the theoretical meets the practical. This section focuses on the practical aspects of developing your first application with React Native, a journey that will cement your understanding of the framework while fostering your confidence as a developer.
Creating a New Project
The very first step in building your application is establishing a new project. Leveraging React Native CLI makes this task straightforward. Once your React Native development environment is set up, you can kick off a new project in a snap. Use the command:
This command scaffolds a new React Native application with the name "MyFirstApp". It initializes the project directory with essential files and folders, covering both Android and iOS platforms. The organization is neat, making it easier to locate components, assets, and configuration files.
But itās not merely about creating a project. Itās about understanding the structure and arrangement of these files so you can manipulate them with confidence. The folder structure includes the file, which is the entry point of your app, and the file that registers the application. These files are your springboard into the expansive capabilities of React Native.
Implementing Basic Components
Once the project is set up, itās time to roll up your sleeves and dive into implementing basic components. React Native allows you to craft an interface with an array of built-in components like View, Text, Button, and Image.
For instance, to create a simple greeting app, you could replace the contents of your file with:
This snippet demonstrates how to arrange components and implement simple styling. By mastering these fundamental blocks, you prepare to explore more complex user interactions within your application.
Styling Your Application
Aesthetics play a vital role in engaging users. React Native provides StyleSheet, a way to centralize styling in a coherent and manageable manner. Leveraging inline styles or the StyleSheet API helps in maintaining a clean codebase while enhancing the app's look and feel.
In the previous snippet, we used to define styles. Creating a consistent and visually appealing user experience means being deliberate in your design decisions. Consider the following points:
- Color Schemes: Choose colors that align with your brand or inspire a particular emotion. Avoid using colors that clash or confuse.
- Typography: The font size and weight can greatly influence readability. Strive for a balance that draws users in without overwhelming them.
- Layouts: React Nativeās Flexbox layout system aids in crafting responsive designs. Mastering Flexbox is important as it aids in providing that fluid experience across various device sizes.
"A well-styled app not only looks good but also communicates effectively with its users. Remember, first impressions matter!"
As you progress, consider delving into tools like Styled Components or libraries like NativeBase for more advanced styling capabilities. They can save time while providing sleek designs.
Navigating with React Navigation
When it comes to mobile app development, user experience is king. One aspect that often plays a significant role in determining this experience is navigation. Effective navigation can mean the difference between a happily engaged user and one who is frustrated and quickly closes your app. React Navigation has emerged as one of the leading libraries for managing navigation in React Native applications, and for good reason. Its versatility and ease of use allow developers to build complex navigation patterns without breaking a sweat.
Understanding the ins and outs of React Navigation is crucial, as it not only influences the usability of your application but also impacts performance. Unlike web applications, mobile interfaces are constrained by screen size and touch gestures, making thoughtful navigation design paramount. React Navigation allows for a fluid user journey through stack and tab navigation, ensuring users can find what they need with minimal fuss.
Installation and Setup
Setting up React Navigation is a walk in the park for anyone who has dipped their toes in React Native. The process kicks off by installing the library through npm or yarn, which are package managers that make adding libraries a breeze. To begin, you would run:
But wait, thereās more. Depending on your navigation needs, you might find yourself adding specific libraries for stack navigation, bottom tab navigation, or drawer navigation. Each of those features offers unique navigation elements intended to enhance user experience.
For example, you might choose to install the stack navigator like so:
Once you have the libraries ready, the next key step is integrating them into your application. Youāll need to wrap your app in a NavigationContainer, which acts as a parent for all navigators. Inside this, you can create your stack or tab navigators, defining screens along the way. Hereās a simple example:
Stack Navigation vs Tab Navigation
In the realm of mobile navigation, two of the heavyweights are stack navigation and tab navigation. Understanding these two can help you make a solid decision on which suits your application's needs best.
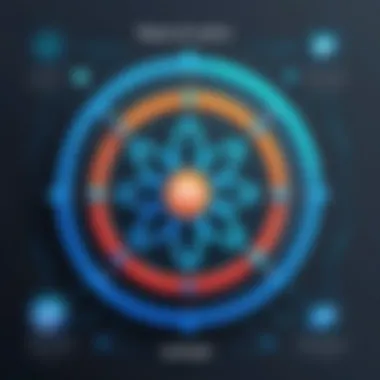
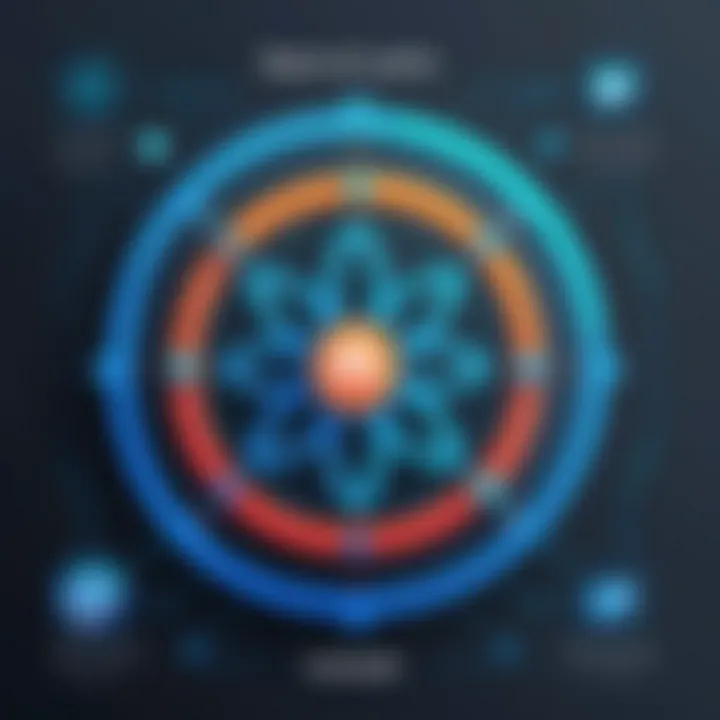
- Stack Navigation: Think of this like a stack of plates. Each screen sits on top of the other, giving you a clear idea of where youāve been and allowing you to navigate back effortlessly. Itās fantastic for tasks that require drilling down into more detailed views. For instance, in an e-commerce app, users might start at the home screen, tap to view a product, and then go even deeper into product details, layering each new screen on top.
- Tab Navigation: If stack navigation is like a pile of plates, tab navigation is akin to the various sections of a multi-layered sandwich. At any one time, users can access multiple tabs representing different sections of your app without needing to traverse back and forth. This is great for applications where users need to access different content categories regularly, like a social media app with a feed, notifications, and profile sections all available at a glance.
In practical terms, combining both navigation types can create a powerful experience. For instance, tab navigation can be used as a main stack, and each of those tabs could lead to different stacks, enabling a rich navigational structure.
"The right navigation setup not only simplifies user interactions but makes them intuitive and enjoyable."
By investing time in understanding these navigational paradigms and implementing them properly, you pave the way for a mobile application that feels just right for users, allowing them to get where they want to go with ease.
State Management in React Native
When it comes to building mobile applications with React Native, state management is a crucial aspect that developers cannot afford to overlook. With the essence of React being about rendering dynamic UIs based on application state, understanding how to effectively manage that state is key to ensuring applications are both responsive and maintainable. This section dives into family of state management tools available to React Native developers, specifically focusing on Redux and the Context API.
Maintaining the right state at the right time can dramatically improve user experience. Imagine a social media app; when a user makes a post, that action needs to trigger changes across multiple parts of the app - the post feed, notifications, and possibly even the userās profile. Each of these components relies on shared state. This scenario demonstrates the need for a solid state management approach.
Foreword to Redux
Redux stands out as one of the most popular state management libraries in the React ecosystem. At its core, it's built on a few key principles:
- Single Source of Truth: The entire state of your application is stored in a single object, or store. This makes it easier to track changes and debug.
- State is Read-Only: To modify the state, you dispatch actions. This approach prevents direct state changes, leading to better predictability and consistency.
- Changes are Made with Pure Functions: Specifically, reducers, which are pure functions that take the previous state and an action to return a new state.
For developers looking to implement Redux, the initial setup may feel a bit cumbersome. However, its vast community and ecosystem of libraries like make integration a manageable task. The learning curve is steep, but once understood, Redux provides a predictable way to handle state in complex applications. Hereās a very simple example of a Redux reducer:
In this case, the function updates the state based on the received action. This clarity can work wonders for larger teams working on expansive applications, providing a structured way to manage changes and enhancements.
Using Context API for State Management
An alternative to Redux is the Context API, which is included with React itself. It offers a way to pass data through the component tree without having to pass props manually at every level. The Context API is often seen as simpler and lighter compared to Redux, making it suitable for smaller applications or when functionality does not demand a full-fledged solution like Redux.
The Context API facilitates global state management, and it's excellent for cases where multiple components need access to shared state. To use the Context API effectively, create a context, provide the context at a high level in the application, and consume it where necessary. Here's a brief overview of its implementation:
- Create a Context:
- Provide the Context:
- Consume the Context:
By using the Context API, developers gain a straightforward and efficient way to manage state across components, especially in less complex applications. However, it may become challenging to manage as an app scales, indicating that the choice of state management should depend on the app's requirements and size.
Ultimately, the selection between Redux and the Context API can significantly impact your development workflow. Understanding their unique strengths and weaknesses is fundamental in making an informed decision that aligns with your project needs.
Performance Optimization Techniques
Optimizing performance in mobile applications is not just a good practice; itās often a necessity. Users today expect their apps to be fast and responsive. With React Native, developers often encounter unique challenges because of the intricate balance between JavaScript operations and native performance. Therefore, understanding performance optimization techniques can significantly impact user experience, rendering, and overall application success.
Avoiding Unnecessary Re-renders
One key strategy for boosting performance in React Native applications is to avoid unnecessary re-renders. When components re-render too often, it can bog down the user interface. For example, imagine a chat application where each message triggers a full re-render of the chat window, resulting in sluggish performance. Developers can mitigate this by strategically using the lifecycle method, which determines whether a component should re-render.
Here are several tips on how to avoid re-renders:
- Use PureComponent or React.memo: These methods help ensure components only update when their props or state change.
- Move state-up: By lifting state up to parent components, you reduce the number of components that need to re-render.
- Key prop: Assigning a unique key prop to elements in a list helps React identify which items have changed, are added, or are removed.
By implementing these strategies effectively, the application can achieve smoother interactions and a more fluid user experience.
Using memoization
Memoization is another powerful method for enhancing performance, especially in contexts where computationally expensive functions are involved. In simple terms, memoization stores the results of function calls and returns the cached result when the same inputs occur again. This saves time and resources, a real boon for mobile devices with limited computational power.
In React Native, we can leverage hooks like and to effectively implement memoization:
- useMemo: This hook is useful for expensive calculations. It recalculates the value only when dependencies change, ensuring efficiency.
- useCallback: It returns a memoized version of the callback function, which is particularly helpful in child components that rely on reference equality for performance optimizations.
Hereās a quick example of using in a functional component:
In this example, gets called only when change, reducing unnecessary calculations.
Efficient performance optimization is like a well-oiled machine. Every part must move in harmony to achieve smooth operation.
Integrating with APIs
In the realm of mobile app development, integrating with Application Programming Interfaces (APIs) has become a fundamental pillar. This process essentially allows your app to communicate with external services, fetching and sending data as needed. Integrating APIs not only enhances the functionality of your application but also enriches the user experience by providing real-time data, authentication services, and various functionalities that might be too complex or resource-consuming to implement from scratch.
When using React Native, understanding how to work with APIs can significantly elevate your appās capabilities. It facilitates features such as social integration, location services, and payment processing, which can be vital depending on the appās objective.
Some specific benefits of integrating APIs in React Native applications include:
- Modularity: APIs allow for a modular approach to app development. You can use numerous services and functionalities without having to build them from the ground up.
- Efficiency: This can save you a heap of time and effort. Instead of reinventing the wheel, you can plug into existing solutions tailored to specific problems.
- Flexibility and Scalability: As your app grows, you can easily swap out or add new APIs without massive rewrites, ensuring that your application can adapt over time.
- Access to Rich Data Sources: Integrating with APIs like Google Maps or social network services can bring in valuable data that enhances the application through external content.
However, while integrating APIs is powerful, there are considerations to keep in mind, such as rate limiting, data usage, and security concerns. Ensuring robust error handling and user experience during API calls is essential.
"An API can be an app's best friend, but only if it's handled wisely."
Making API Calls with Fetch
In React Native, one of the straightforward ways to make API calls is by using the built-in function. This native JavaScript function provides a clean way to interact with network resources without the need for additional libraries. The fetch API returns a promise, meaning it operates asynchronously, which is ideal for mobile applications where performance is critical.
Here's a simple example of how you might use the fetch API:
This snippet demonstrates a basic GET request, but you can also perform POST, PUT, and DELETE requests. The key point here is to handle both success and error scenarios, ensuring robust application performance.
Handling Asynchronous Data
Working with APIs often means dealing with asynchronous data. In the mobile app ecosystem, how you manage this data can make or break the user experience. When fetching data, itās crucial to effectively handle the loading states, success responses, and errors to keep the user informed.
React Native provides several ways to manage asynchronous data:
- State Management: Using Reactās built-in state management with the and hooks enables you to manage loading states and data effectively. You can dynamically update the UI to reflect loading or error states.
- Promise Handling: As described earlier, utilizing promises ensures that you're handling data correctly. Make sure to chain your and methods properly to handle success and failure cases.
- Libraries for More Complexity: If things get complicated, libraries like Axios can streamline the process, offering higher-level configurations like interceptors, which may be necessary for larger applications.
Understanding how to handle asynchronous data in React Native is critical. It ensures your app remains responsive even in the face of network delays, ultimately leading to a smoother user experience.
Using Third-Party Libraries
In the realm of mobile app development, utilizing third-party libraries can significantly enhance productivity and streamline the coding process. These libraries provide pre-built solutions for common challenges, which means developers donāt have to reinvent the wheel. Instead, they can focus on crafting unique features that set their applications apart. This section discusses why integrating third-party libraries should be a core part of your development strategy, particularly when working with React Native.
Popular Libraries and Their Uses
When diving into React Native, you'll encounter a plethora of libraries, each with specific functions that can greatly aid in development. Here are a few that stand out:
- Axios: A promise-based HTTP client for making requests. It simplifies the process of sending asynchronous requests to APIs and is more versatile than the built-in fetch API.
- React Navigation: This library is essential for navigating your mobile app easily. It offers different navigation patterns, like stack and tab navigation, to enhance user experience and fluidity.
- Redux: While state management is crucial, Redux provides a predictable state container. This can help manage the applicationās state across various components efficiently.
- Native Base: This library aims to help developers create a consistent user interface. With pre-styled components designed for mobile applications, it saves time and ensures a uniform experience on both iOS and Android.
- React Native Paper: If your application requires material design components, this library is a treasure trove. It provides beautifully crafted UI components that follow Material Design guidelines.
Integrating these libraries can make your development process significantly smoother. They often come with rich documentation and active communities, which can be a lifesaver when you run into issues.
Installing and Linking Libraries
Getting started with third-party libraries in React Native is often as easy as pie, but there are some considerations to keep in mind. Hereās how you can do it effectively:
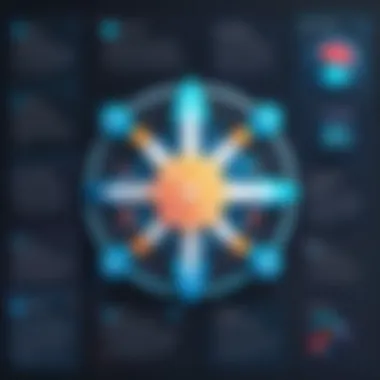
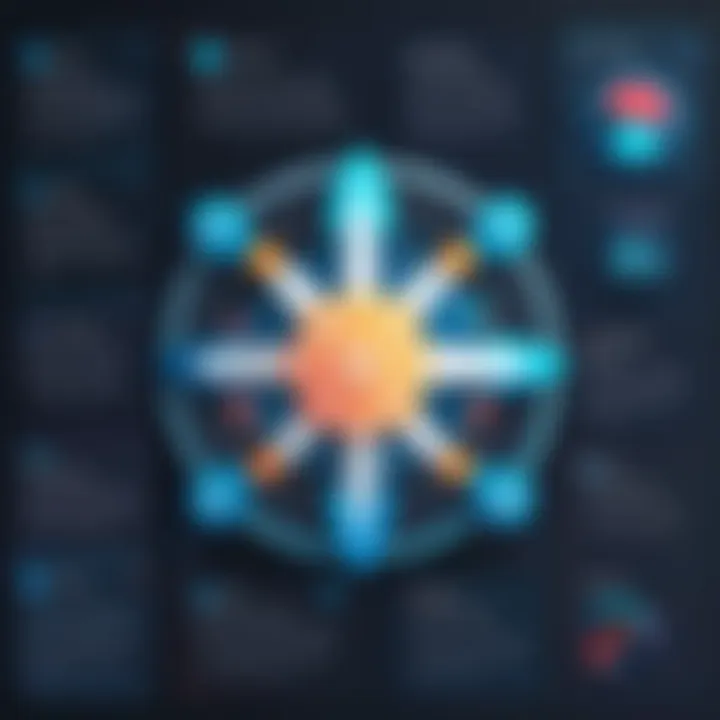
- Installation: Use npm or yarn to install libraries. For instance, to install Axios, you might run:or
- Linking: For some libraries, especially those that involve native code, linking may be necessary. React Native provides a command for this, but newer versions mostly support auto-linking. If needed, you can link manually using:For example:
- Configuration: After installation, some libraries might require extra steps in configuration. This could involve modifying files like or updating native code in iOS and Android folders. Always refer to the libraryās documentation for precise steps.
By leveraging third-party libraries, you gain access to a wealth of resources that can make development less cumbersome and more enjoyable. Remember, donāt overdo it; choose libraries that fit well with your project to avoid unnecessary complexity.
"Using external libraries can turn a cumbersome effort into a smooth glide through development. Choose wisely."
In summary, integrating the right set of third-party libraries while developing mobile applications in React Native can not only save time but also enhance the capabilities of your app. With the right tools at your disposal, your development experience can be both efficient and fulfilling.
Testing and Debugging
Testing and debugging are absolutely essential components in the realm of mobile application development. They not only help in identifying defects but also ensure that your app runs smoothly across different devices. The importance of these processes can't be overstated. When you consider the millions of users who might download your app, the stakes for releasing a bug-free product skyrocket.
In the context of React Native, effective testing and debugging are crucial because the framework interacts with native platform components. You want to ensure that the user experience remains seamless, regardless of the device in question. There are several key aspects to emphasize here:
- Enhanced User Satisfaction: Thorough testing minimizes the chances of encountering bugs that can frustrate users.
- Cost Efficiency: Identifying issues during the development stage, rather than post-release, saves both time and money.
- Higher App Ratings: Apps that function well on first install tend to receive better reviews, improving visibility in app stores.
By integrating regular testing and debugging into your development workflow, you'll not only improve the quality of your application but also streamline the overall development process.
Unit Testing with Jest
When it comes to unit testing in React Native, Jest is often hailed as the go-to framework. It provides a robust environment to validate the functionality of individual units of code. But why choose Jest specifically?
- Easy Setup: Right out of the box, Jest is configured to work seamlessly with React Native projects.
- Snapshot Testing: This feature allows developers to capture a snapshot of rendered components, making it easy to track changes over time.
- Fast Execution: Jest's parallel test execution means that testing time is kept minimal, enabling efficient development cycles.
Hereās a simple example to illustrate how to set up a test using Jest. Let's say youāve got a component named :
In this snippet, we import the necessary libraries and then check whether our component renders the expected text. If it doesnāt, the test will fail, alerting you to a potential issue in your code.
Using React Native Debugger
Once your app is running, debugging becomes essential to address issues that testing might not catch upfront. React Native Debugger is a highly versatile tool for diagnosing problems. It combines several features into one handy package, making your life a bit easier when it comes to tracking down bugs.
Some key features of the React Native Debugger include:
- Performance Monitoring: It provides real-time insights into how your app performs, helping you pinpoint lagging components.
- Inspect Network Requests: You can watch API calls live, which is invaluable when youāre trying to trace issues related to API integration.
- State Inspection: This feature allows you to inspect the current state of your app, giving you insight into how state changes affect your UI.
To launch the React Native Debugger, you typically run the following command in your terminal:
From there, you can start inspecting your app in real-time.
In summary, both testing and debugging are vital practices that ensure your mobile applications not only function as intended but also offer a delightful experience for users. Utilizing tools like Jest and the React Native Debugger enriches your development process, paving the way for a robust, user-friendly application.
Publishing Your Application
Publishing your mobile application is the final step of the development journey. It encompasses the nuances of transforming your finished product into a downloadable package that users can access. This process is not merely a technical hurdle; it's an essential phase that can significantly impact your app's success and user engagement. Once youāve gone through the meticulous processes of designing, coding, and testing your app, getting it out there in the marketplace is crucial for harnessing user feedback, engaging audiences, and potentially monetizing your efforts.
Preparing for App Store Submission
When it comes to publishing on platforms like the Apple App Store, there are specific steps you need to follow to ensure a smooth submission. First off, itās essential to thoroughly test your app. This isnāt the time to cut corners. Users' first impressions are vital, and your app should run without issues across various devices. A good practice is to simulate different environments that mimic real-world usage.
Once youāve ironed out the kinks, you must create an Apple Developer account. This allows you access to tools and resources needed for app distribution. If you haven't already, youāll have to set up your appās metadata, including its name, icon, and description. Keep in mind that Apple scrutinizes every submission based on their guidelines, so make sure your app is in compliance to avoid rejections. Here are several key elements to consider:
- App Name and Icon: Choose a catchy name that resonates with your target audience and design an intuitive icon that stands out.
- Screenshots and Preview Videos: Make sure they are high quality. Show off your appās features and functionality.
- Privacy Policy: This is legally required; describe how your app collects, uses, and shares user data.
- Versioning: Submit a version that highlights the best features of your app.
Having organized all of this, you can use Xcode to upload your application to the App Store. After this initial upload, a review process begins, which can take anywhere from a few days to a couple of weeks, depending on various factors.
"Remember, every delay in the submission process can impact your market entry and subsequent user acquisition."
Publishing on Google Play
Publishing on Google Play tends to be a bit more straightforward than Apple, but it still requires significant attention to detail. The first step to take is creating a Google Play Developer account. Again, this opens doors to necessary tools for managing your app post-launch. You can skip some of the stringent compliance checks, but itās best to have your app rigorously tested.
Once your Developer account is ready, you need to
- Prepare App Details: Just like with iOS, prepare your app's title, description, and screenshots. Make sure the description is rich with relevant keywords to boost discoverability.
- Sign Your App: To protect your app's integrity, it's important to sign it before releasing it to the public.
- Select Distribution Preferences: Here, you can define whether your app is free or paid and set distribution specifics based on geographic metrics.
Once you've uploaded your APK file along with the required information, you set it for review. Generally, Googleās review process tends to be faster than Appleās. If all goes well, your app will be available for download shortly.
In both ecosystems, it's imperative to monitor your app's performance once it's live. Gathering user feedback and analyzing app store metrics can help you refine the user experience and guide future updates.
Common Challenges and Solutions
Dealing with Performance Issues
Performance hangs like a dark cloud over many React Native projects, often trailing developers throughout the development process. When an app stutters or lags, users may jump ship faster than you can say "download failure." Therefore, addressing performance hiccups head-on is vital not just for user satisfaction but also for the long-term success of your application.
One commonly mentioned problem relates to slow rendering times that can frustrate users. React Native relies on a virtual DOM to optimize updates, but that doesn't always lead to smooth sailing. To enhance performance, consider the following approaches:
- Use Native Modules: If some features are a bit heavy in terms of processing, you may want to offload part of your work to native modules written in Java or Swift. This can help you skip over performance bottlenecks when necessary.
- Optimization with PureComponent: Leverage or to avoid unnecessary re-renders when props or state have not changed. This can significantly reduce the rendering cycles, especially in complex UIs like lists.
- Monitor with Performance Monitor: Tools like the React Native Performance Monitor can give you a quick overview of what's dragging your app down, helping pinpoint problematic areas more precisely.
These strategies may not fix every issue that pops up, but they can certainly lighten your load when it comes to performance concerns.
Debugging Common Errors
When building an app, you can bet your last dime that errors are lurking around each corner. Debugging is part and parcel of the development process, but it can generally be a real thorn in the side.
Common errors often range from syntax errors in your JavaScript code to more specific issues related to libraries, dependencies, or even the native code bridge between JavaScript and the underlying platform.
To tackle these issues in a systematic way, consider the following tactics:
- Leverage the React Native Debugger: This tool combines several debugging features in one place, letting you inspect network requests, view the state of your Redux store, and more. Itās a good practice to use it regularly to catch problems before they snowball out of control.
- Error-boundary Components: Implement error boundaries as a way to catch and log errors effectively. They can help prevent the entire application from crashing because of an unexpected issue.
- Community Resources: Platforms like Reddit and Stack Overflow have active communities of developers who often share similar experiences. Donāt hesitate to tap into that resource if you're stuck.
Maintaining a finger on the pulse of debugging can be tedious, but steering through the errors will empower you to build applications that not only function but also shine in terms of performance and usability.
"Every problem is a gift ā without problems we would not grow." ā Tony Robbins
Being mindful of common challenges and their solutions can pave the way for a smoother development experience with React Native. š ļø Developers can learn from each hurdle and continue honing their craft as they strive to create high-performing, user-friendly applications.
Ending
The conclusion of this exploration into React Native serves as a pivotal point for anyone poised to step into mobile app development with this framework. Itās the summary of a journey that began with understanding the basics and culminated into recognizing the broader implications of using React Native in the tech landscape. In this section, weāll underscore the importance of reflection and future considerations, as these are key in grasping both the essence of what has been learned and what lies ahead.
Reflecting on the React Native Journey
As we look back on the path taken through our journey with React Native, it becomes clear that the framework's versatility holds significant value for aspiring developers. React Native has changed the game for building cross-platform applications. It allows developers to write code once and deploy it on both iOS and Android, cutting down on time and resources. This efficiency is worth its weight in goldāwhether you're a solo developer or part of a larger team.
Moreover, through understanding concepts like components, state management, and navigation, we have built a solid foundation. Reflecting on these elements underlines their importance in creating intuitive user experiences. For instance, the concept of reusability in components doesn't just simplify coding; it encourages a more organized structure which makes it easier to maintain as applications grow in complexity. We've also pinpointed ways to enhance performance and troubleshoot issues, which adds to the arsenal of skills that developers can wield effectively.
Itās not merely about the code or the tools used, but about the mindsetāa mindset geared towards continuous learning and adaptation. Embracing feedback from testing and debugging not only elevates the quality of applications but also cultivates a cycle of improvement that benefits not just the project at hand but one's career as a whole. Knowledge is indeed a powerful currency in our field.
Future of Mobile Development with React Native
Peering into the future of mobile development, React Native stands as a beacon of innovation. The constant evolution of the framework reflects the dynamic nature of technology itself. As mobile devices become ever more integral to everyday life, the demand for robust, cross-platform applications will only grow.
Looking forward, advancements in frameworks and libraries will likely continue to blur the lines between web and mobile development. React Native, with its strong backing from Facebook and an active community, seems poised to adapt to these trends seamlessly.
Considerations such as augmented reality, machine learning, and enhanced user interface designs appear to be on the horizon. This evolution opens doors to entirely new applications and experiences, potentially redefining user interaction as we know it.
Additionally, as cloud services and APIs become commonplace, the synergy between mobile applications and cloud architecture will be crucial. React Nativeās compatibility with various third-party libraries can facilitate the integration of tools that support these emerging technologies.