Data Types in Python: A Comprehensive Overview
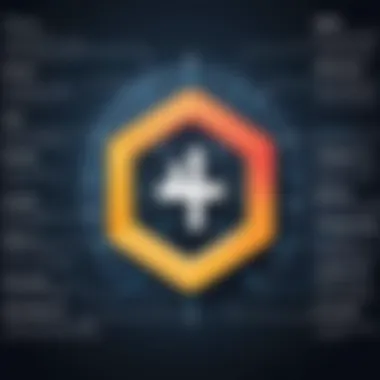
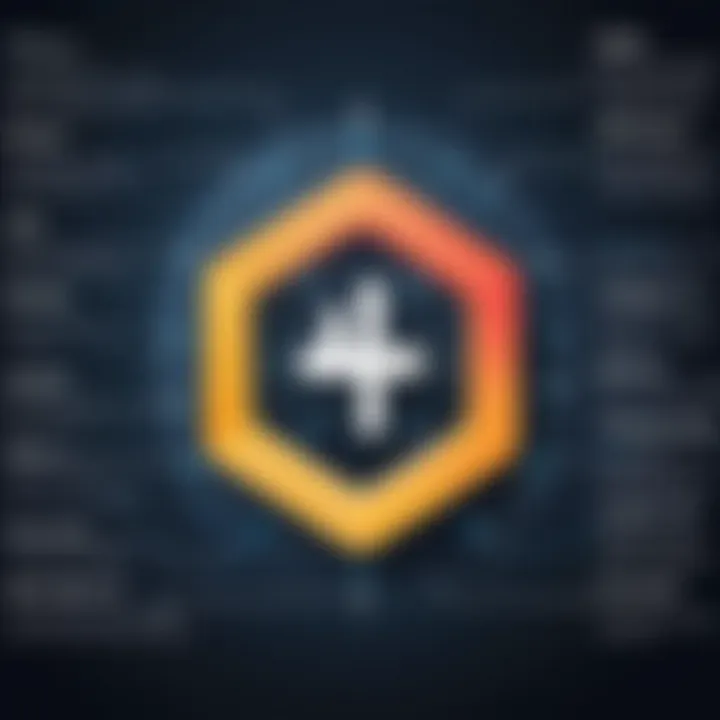
Intro
Programming languages serve as a foundational tool for building software applications. Among these languages, Python has risen in popularity. Understanding the data types in Python is essential for effective coding. Data types define the kind of data a variable can hold, affecting how information is processed.
History and Background
Python was created by Guido van Rossum and first released in 1991. Designed to be easy to read and write, Python focuses on code readability. Over the years, it has gained traction for various applications, from web development to data analysis. Its straightforward syntax appeals to programmers of all skill levels.
Features and Uses
Python offers several features. It provides dynamic typing, meaning that variable types do not need to be explicitly declared. This flexibility allows quick coding. The language is also interpreted, making it easier to test code snippets. Python is widely used for web development, scientific computing, data analysis, and machine learning. The extensive libraries available enhance its functionality, further fueling its popularity.
Popularity and Scope
According to recent surveys, Python consistently ranks among the top programming languages. Its community support contributes to its growth. Various resources, including documentation and forums, are available for learners. The language continues to evolve, with new features and improvements enhancing its performance and user experience.
With a solid understanding of Python's history and framework, one can appreciate the importance of data types in this powerful language.
Intro to Data Types
Understanding data types is crucial for any programming language, and Python is no exception. Each data type serves a specific purpose, defining how data can be stored, manipulated, and utilized within a program. This section analyzes the relevance and impact of data types in Python, focusing on their role in enhancing programming efficiency and accuracy.
Importance of Data Types in Programming
Data types provide the structure for data, influencing how programs process and handle information. In programming, each variable assigned must have an identified data type. This identification helps the interpreter manage memory and optimize performance.
- Efficiency: Data types help determine how much memory to allocate for each variable. For instance, integers require less memory compared to strings. Understanding these distinctions can significantly enhance program efficiency.
- Error Reduction: When data types are utilized correctly, the likelihood of errors reduces. Type-related errors can lead to runtime failures. Knowing the data types helps avoid such pitfalls.
- Readability: Clear knowledge of data types can make code more readable and easier to understand. This readability is essential for collaboration in larger teams or projects.
Overview of Python's Data Type System
Python's data type system is dynamic and versatile. The language categorizes data types broadly into several categories, including numeric types, sequences, mappings, and sets. Each category offers unique functionalities that expand Python's capabilities.
- Primitive Data Types: This includes integers, floats, and booleans. They form the foundation for more complex types. Each primitive has its own set of operations and behaviors which will be explored further in subsequent sections.
- Collections: Lists, tuples, sets, and dictionaries are integral parts of Python’s data structure. They accommodate multiple items, allowing for complex data handling.
- Dynamic Typing: Python employs a dynamically typed system, meaning variables can change data types during execution. This flexibility is both a strength and a consideration when designing applications because it can lead to unexpected behaviors.
Understanding Python's data types goes beyond definitions and operations; it lays the groundwork for advanced programming concepts and techniques. This ongoing exploration of data types will guide learners through practical use cases and applications, enhancing their coding toolkit.
"Mastering data types is essential for every Python programmer, as it is the bedrock of logical data handling and manipulation."
As the article progresses, each data type will be dissected in detail, providing clarity and insight into their usage and significance.
Basic Data Types in Python
Basic data types in Python serve as the foundation of programming in this language. They are essential for understanding how to work with data, perform operations, and develop efficient algorithms. Familiarity with these data types aids beginners and seasoned developers alike in writing clearer and more effective code. Recognizing the attributes of these basic data types enables one to utilize Python in a broad array of applications, from simple scripts to complex applications.
Integers
Definition
Integers are whole numbers, both positive and negative, without decimal points. This fact underscores their role as a fundamental building block in coding. They can represent counting values or serve as indexes in data structures. A key characteristic is their capacity for mathematical operations. Integers are a popular choice due to their simplicity and immediacy in programming tasks that involve calculations.
Operations
The operations that can be performed on integers include addition, subtraction, multiplication, and division. Python supports these operations natively, making it easy to work with numerical data. An important aspect is the use of integer division, which only returns whole numbers. This uniqueness is beneficial in scenarios where decimal precision is unnecessary, though it may lead to confusion if not properly understood.
Use Cases
Integers find application in various situations like loops, counters, and index manipulation in data structures. They are particularly useful in algorithms that require discrete steps, such as iterating through a sequence. A notable advantage of using integers is their performance in terms of speed, as they do not require additional processing that comes with floating-point numbers.
Floats
Definition
Floats, or floating-point numbers, are numbers that contain decimal points. They are essential for representing real numbers in programming, especially when precision matters. A float's ability to hold fractional values makes it a popular choice in scientific calculations. However, they can introduce complexities due to floating-point arithmetic.
Precision Handling
Handling precision with floats is crucial because of potential inaccuracies. Floating-point arithmetic can lead to unexpected results due to rounding errors. This nuance requires programmers to be cautious, especially in financial applications where precision is vital. Python provides libraries, such as Decimal, to mitigate issues related to float precision, making it easier to manage these scenarios.
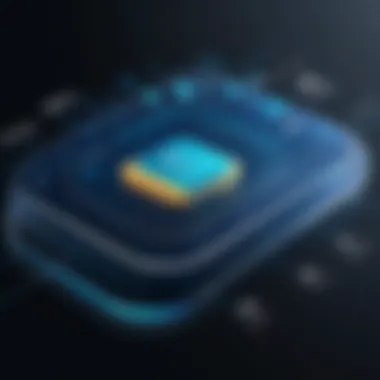
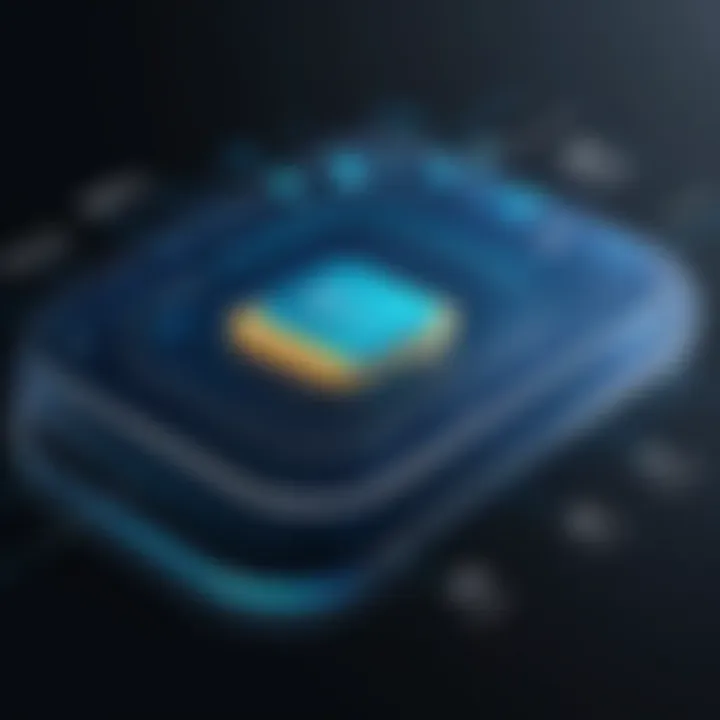
Applications
Floats come into play in simulations, financial calculations, and any domain requiring continuous data representation. They are beneficial in fields like engineering, where real-world measurements often involve fractions. However, their reliance on approximations is a disadvantage; programmers must recognize the potential pitfalls of using floats in critical calculations.
Booleans
True and False Values
Booleans represent binary values: True and False. This data type forms the core of logical operations and decision-making in programming, making it indispensable in control flow. The core characteristic of booleans is their simplicity, which translates complex scenarios into easily interpretable statements. However, their significance extends beyond mere representation, defining the language of logic within Python.
Logical Operations
Logical operations harness booleans to evaluate conditions. Operators such as AND, OR, and NOT enable intricate decision-making processes in applications. Their primary benefit lies in simplifying complex conditions into manageable expressions. Misunderstanding logical assessments can lead to errors that disrupt program flow, making careful attention to logic a necessity.
Conditional Statements
Conditional statements rely heavily on booleans to control the flow of execution. Statements like , , and allow Python programs to execute different actions based on boolean evaluations. This capability is invaluable for crafting responsive and adaptive applications. A significant feature is that it helps avoid redundant code, enhancing both readability and efficiency in programming.
Advanced Data Types
Advanced data types in Python play a crucial role in enhancing the functionality and flexibility of programming. Understanding how to use these data types effectively can greatly improve coding efficiency. This section explores strings, lists, tuples, dictionaries, and sets. Each data type offers unique characteristics that serve different purposes. Recognizing these idiosyncrasies will aid in making informed decisions in programming tasks.
Strings
Strings are sequences of characters. They are widely used for handling textual data. Working with strings can be essential for many applications, from simple user inputs to complex text processing. This section dives deeper into string manipulation, common methods, and formatting.
String Manipulation
String manipulation involves modifying, analyzing, or transforming string data. It includes tasks like searching, replacing, or changing the case of characters. A key characteristic of string manipulation is its versatility, allowing programmers to meet varied data handling needs. Its popularity stems from the intrinsic necessity of processing user input or data storage tasks. One unique feature of string manipulation is the slicing method, allowing to extract substrings easily. The main advantage is the simplicity of operations. However, one drawback is that strings are immutable in Python, meaning any change creates a new string rather than altering the existing one.
Common String Methods
Common string methods provide ready-made functions for a wide range of actions. These include , , , and many more. These methods improve code readability and save time. Their main characteristic is that they encapsulate frequently used functionalities. Thus, they are a popular choice among developers. Each method serves a specific purpose, such as removing whitespace or converting the case of characters. The advantage of using these methods is that they simplify string handling, but their limitation is that relying on methods can lead to less control over string manipulation when more custom behavior is needed.
String Formatting
String formatting refers to the process of crafting strings to include dynamic content. Tools such as f-strings and help achieve this task. A key characteristic of string formatting is allowing the integration of variables into strings seamlessly. This capability makes it a beneficial choice for creating user-friendly output. A unique feature is the ability to define precise formatting, such as specifying decimal places for numbers. While formatting enhances string presentation significantly, it can also introduce complexity in maintaining code clarity, especially for those unfamiliar with specific formatting syntax.
Lists
Lists are ordered collections of items. They can hold diverse data types, making them incredibly flexible. Understanding how to create and operate on lists will facilitate various coding tasks. This section addresses creating lists, performing operations, and utilizing comprehensions.
Creating Lists
Creating lists involves defining an ordered collection of items. A significant aspect of list creation is its syntactic simplicity. Using square brackets is a straightforward and beneficial choice for beginners and experienced coders alike. Adding items to lists is done using the append method, allowing dynamic growth. Lists can also be created from different data types, which gives them a unique feature of versatility. However, this flexibility can sometimes lead to unexpected behaviors when mixing incompatible data types, requiring caution during implementation.
List Operations
List operations encompass a variety of functional tasks, such as adding, removing, and iterating over list items. One main characteristic is the powerful indexing system, allowing access to individual elements. Operations such as and are common choices that add to a programmer’s arsenal. A unique feature is the ability to sort lists easily, which enhances data management. Though list operations are relatively easy to grasp, their performance may degrade when the list grows substantially, demanding attention to efficiency.
List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. This aspect allows for powerful data manipulation in fewer lines of code, contributing to cleaner programming style. The key characteristic of list comprehensions is their syntactic brevity, making them a popular tool among Python developers. They enable filtering and transforming data in one go. A unique feature is their ability to improve speed compared to traditional methods. However, their use can also lead to less readable code for complex operations, which is a consideration developers should account for.
Tuples
Tuples are similar to lists but are immutable, meaning once created, their structure cannot change. This ensures consistency and can enhance the safety of data. Their unique characteristics provide specific use cases.
Definition
A tuple is defined using parentheses, offering an ordered collection of items. The immutability of tuples makes them a popular choice for functions that require data integrity. This characteristic adds reliability. One advantage of tuples is their light memory footprint compared to lists, significantly improving performance in scenarios involving vast amounts of data. Yet, the inability to modify tuples can be limiting.
Immutability
Immutability is central to understanding tuples. This concept reinforces the security of data by preventing accidental changes. A crucial aspect is that functions requiring consistent data can benefit significantly from using tuples. This restricts unintended alterations and improves reliability. However, this unique feature can also be a disadvantage if dynamic updates are necessary, prompting the need for considering when to use tuples versus lists.
Uses of Tuples
Tuples find utility in various programming scenarios. They are often used to return multiple values from functions or as keys in dictionaries due to their hashable nature. The capacity to group related pieces of data without modification stands out as a key characteristic. Their reliability makes them a beneficial choice for configuration settings. Nonetheless, limited flexibility can be a drawback if frequent adjustments to the data set are anticipated.
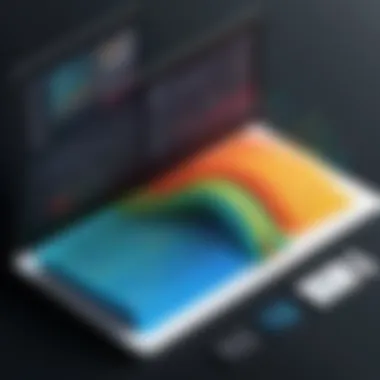
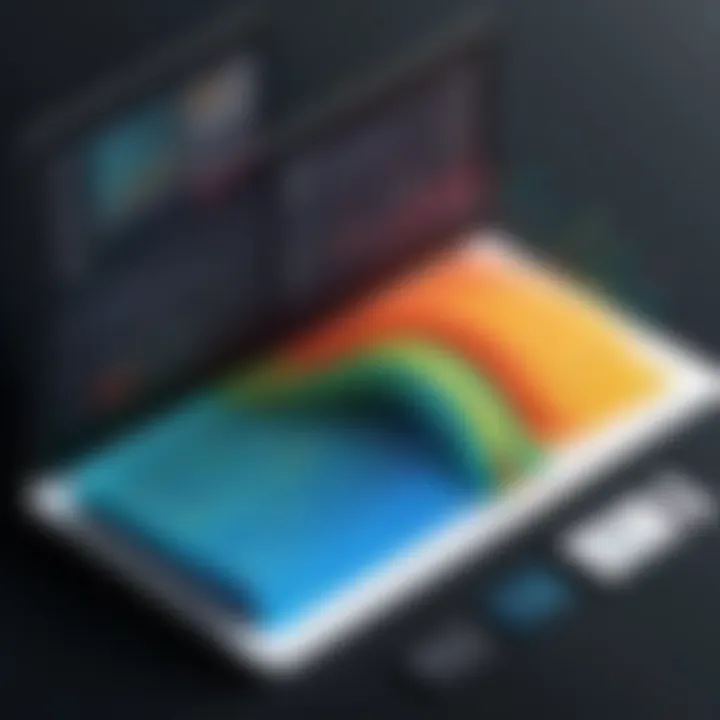
Dictionaries
Dictionaries serve as key-value pairs, allowing for fast data retrieval. This structure offers an effective means of associating data with unique identifiers.
Key-Value Pairs
Key-value pairs are fundamental to dictionaries. Each key corresponds to a value, providing a direct way to access data. A key characteristic is the ability to use immutable data types as keys, ensuring data integrity. This method simplifies complex data organization significantly. One unique feature is constant-time complexity for lookups, a major advantage in performance. However, if not managed properly, dictionary entries can lead to unintentional overwriting of values, which can create confusion.
Dictionary Operations
Dictionary operations focus on tasks such as adding, modifying, and removing entries. One prominent aspect is the clear syntax, which enhances the intuitiveness of dictionary management. The ability to check for existing keys using is a popular choice among developers. The efficiency of these operations is notable, especially when dealing with large datasets. One unique feature of dictionaries is the method , which enables simultaneous iteration over keys and values. However, dictionaries can consume more memory than simpler data structures, so balancing use is essential.
Practical Applications
Practically, dictionaries serve in various applications, from simple configurations to complex data models in applications. The ability of dictionaries to organize data logically lends itself to easy manipulation. Their most significant characteristic is versatility across programming tasks. One unique property is their capability to nest, allowing for complex data representations. Although dictionaries provide advantages in manageability, they can lead to higher memory overhead compared to simpler types, requiring mindful use.
Sets
Sets are collections of unique items. They provide a means to manage unique elements efficiently. Sets eliminate duplicates and allow for mathematical set operations.
Properties of Sets
The properties of sets include uniqueness and unordered collections. Each item must be unique, which simplifies data integrity management significantly. A primary characteristic is that operations can be performed on entire collections rather than on individual items, promoting efficiency. Additionally, sets are mutable, allowing for dynamic updates. This flexibility makes using sets beneficial for removing duplicates. However, since sets are unordered, accessing elements by index is not possible, which might be a limitation in certain use cases.
Set Operations
Set operations include union, intersection, and difference. These capabilities make it easy to perform mathematical computations on datasets. A key characteristic is their focus on performance and efficiency, especially when working with large amounts of data requiring these operations. The unique feature of set operations is the simplicity of syntax that aids clarity and comprehension. Nonetheless, certain operations can lead to unexpected results if duplicates are present in the source data, necessitating diligence.
Set Use Cases
Sets serve various applications, including membership testing and removing duplicates from a list. They are particularly advantageous in scenarios needing quick membership checking, as they provide faster performance than lists. Their key characteristic is the inherent uniqueness that eliminates redundancy. The unique feature of sets is the ability to operate with large data efficiently. However, if order matters, using sets may not be ideal, presenting a limitation in certain scenarios.
Type Casting and Conversion
In Python programming, understanding type casting and conversion is essential. It allows developers to alter the data type of variables to meet the needs of their applications. This concept is not only important for data manipulation but also plays a critical role in ensuring that the code works as expected without errors.
Type casting can be broadly divided into two categories: implicit and explicit. Implicit casting is typically automatic, while explicit casting requires the programmer to specify the conversion. Knowing when and how to apply both types assists in writing cleaner, more efficient code. It also helps avoid potential data loss and errors during operation.
Implicit Type Casting
Implicit type casting, often referred to as coercion, happens automatically. Python handles conversion between compatible data types seamlessly without user intervention. For example, when you add an integer and a float, Python converts the integer into a float before performing the operation. This automatic conversion might seem trivial, but it illustrates one of Python's strengths in reducing the need for manual type conversion.
Here is a simple demonstration of implicit type casting:
In this case, the integer becomes a float without needing any explicit instruction from the programmer. This feature promotes efficiency in coding and minimizes errors related to type mismatches. However, developers should still be aware of when this happens to maintain the precision of their calculations.
Explicit Type Casting
Explicit type casting requires intentional direction from the programmer to convert one data type to another. This method is commonly used when data needs to be transformed consciously to fulfill specific requirements. In Python, functions such as , , and are employed for this purpose.
Here’s an example of explicit type casting:
In this example, the float is transformed into the integer . It is important to recognize that during this conversion, the decimal portion is discarded, which might lead to data loss if not handled correctly.
Thus, explicit casting allows control over how data is converted, but it also necessitates care. The developer must be aware of the consequences of the conversion to avoid unintentional data loss or bugs.
Understanding Mutable and Immutable Types
Understanding the concepts of mutable and immutable types is crucial for programmers using Python. These terms refer to the ability to change the content of data structures at runtime. Recognizing the differences between mutable and immutable types can lead to better management of memory and performance, preventing unintended side effects when manipulating objects.
Definition of Mutable and Immutable
Mutable types are those data types whose value can be changed after they are created. This means that when you modify a mutable object, it can retain its original reference, allowing for more efficient memory use. Common examples include lists and dictionaries. Conversely, immutable types are data types that cannot be changed once they are created. Any modification results in the creation of a new object. Strings and tuples fall into this category. The distinction influences not only performance but also how data is handled in Python.
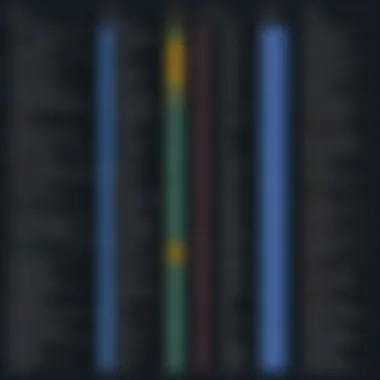
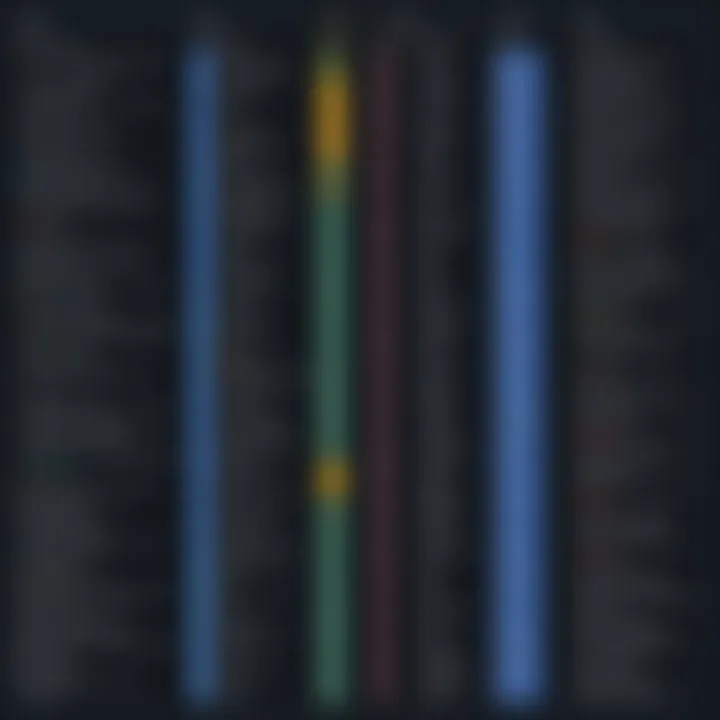
Examples in Python
In Python, the distinction between mutable and immutable types manifests clearly.
Mutable Types
- Lists: Lists can be modified by adding, removing, or changing elements.
my_list.append(4)# list becomes [1, 2, 3, 4]
Immutable Types
- Strings: Once a string is created, it cannot be modified. Any change requires creating a new string.
my_string = my_string.upper()# creates a new string "HELLO"
By grasping these concepts, programmers can utilize Python's data types more effectively, optimizing both the development process and application performance. Understanding mutable and immutable types assists in making informed decisions about data handling, thereby enhancing overall coding efficiency.
Data Type Limitations and Considerations
Understanding the limitations and considerations of data types is crucial in programming with Python. It goes beyond mere recognition of different types; it allows developers to make informed choices that can significantly affect performance and memory usage. In an environment where efficiency matters, especially in data-intensive applications, being aware of constraints helps programmers optimize their code.
Performance Implications
Performance is a key factor in software development. The choice of data type can influence the speed of operations and overall execution time. For instance, operations performed on integers are typically faster than on larger floating-point numbers. This is due to how Python handles memory and arithmetic processing.
When using certain data structures, the choice can also impact speed.
- Lists, while very flexible, may suffer from slower performance when it comes to searching and sorting compared to tuples, which are more memory efficient and faster in iteration due to their immutability.
- On the other hand, dictionaries can provide fast data retrieval thanks to their hash table implementation, but they can be slower in scenarios where memory consumption is high.
Thus, it is essential for developers to consider the specific use case and choose the most appropriate data type. Misjudgments can lead to measurable performance dips, especially in larger datasets.
Memory Management
Memory management is another significant aspect to consider. Different data types have varying memory footprints, and understanding these can prevent wastage of resources. For example, an integer occupies less space than a float, which means that in large datasets, utilizing integers over floats could lead to substantial memory savings.
Python manages memory through a process known as garbage collection, but that does not negate the importance of being conscious of what types are being used.
- Immutable types, like strings and tuples, can help in memory-efficient programming. When a new value is assigned, Python creates a new object instead of modifying the existing one.
- Conversely, mutable types, such as lists and dictionaries, can lead to increased memory usage over time, especially if unnecessary copies are created during manipulations.
"A good programmer is always aware of the application's memory consumption and seeks to optimize it, especially when working with large data."
Being proactive about memory management by choosing the right data type can significantly enhance efficiency and performance in applications. Therefore, thoughtful consideration of these limitations helps ensure that Python programming is both effective and efficient.
Closure
The conclusion serves as a pivotal element in any article, consolidating the insights discussed throughout the text. It allows for a clear summarization of the core subjects, reinforcing what has been learned about Python's data types. This section also holds the potential to underscore the implications of mastering these data types, especially for students and individuals exploring programming.
A strong grasp of data types enhances not only the coding efficiency but also the ability to manage data effectively. The distinctions between various types such as integers, floats, strings, lists, and dictionaries manifest in their respective usage, performance, and flexibility.
Understanding data types is fundamental in programming. It lays the groundwork for efficient algorithm design and optimization.
In Python, the myriad of data types makes it essential to choose the right one for the task at hand. Choosing wisely can facilitate better memory management and improved performance in applications, showcasing the vital significance of this knowledge.
Moreover, as technology evolves, so do the demands for more sophisticated programming skills. Understanding the limitations and potential issues tied to data types is crucial for adapting and enhancing one’s coding capabilities in diverse environments.
Recap of Key Points
- Diverse Data Types: Python features various built-in data types which include integers, floats, strings, lists, tuples, dictionaries, and sets. Each has unique attributes and suitable applications.
- Casting and Conversion: Implicit and explicit type casting illustrates how Python handles data types dynamically, allowing for flexibility while coding.
- Mutable vs Immutable: A critical distinction in Python is the characteristic of mutability. Understanding which data types can be changed and which cannot is core to efficient programming.
- Limitations: Knowledge of performance implications and memory management ensures that developers make informed decisions about which data types to use based on their requirements.
Encouragement for Further Learning
As coding continues to be an essential skill in various fields, a deeper exploration of data types can enhance your programming narratives. This article has highlighted fundamental concepts, but the journey does not stop here. There is always more to discover.
- Explore Libraries: Libraries like NumPy and Pandas are built on advanced data types and concepts that push Python's capabilities further.
- Build Projects: Putting knowledge into practice through projects can solidify understanding. Simple applications such as a basic calculator or a todo list can help apply data types more deeply.
- Join Programming Communities: Engaging with other learners and experienced programmers on platforms like Reddit or dedicated forums can provide new perspectives and real-world insights.
Continually seek resources, tutorials, and courses to further your learning. By doing so, you not only learn how to use Python more effectively but also position yourself better in an ever-evolving field.