Mastering Data Structures for Technical Interviews
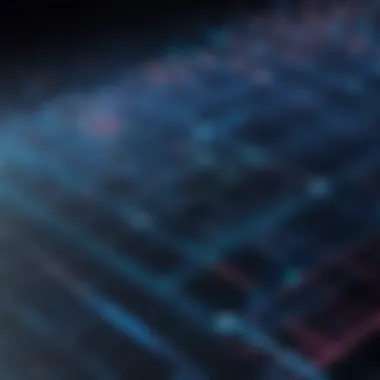
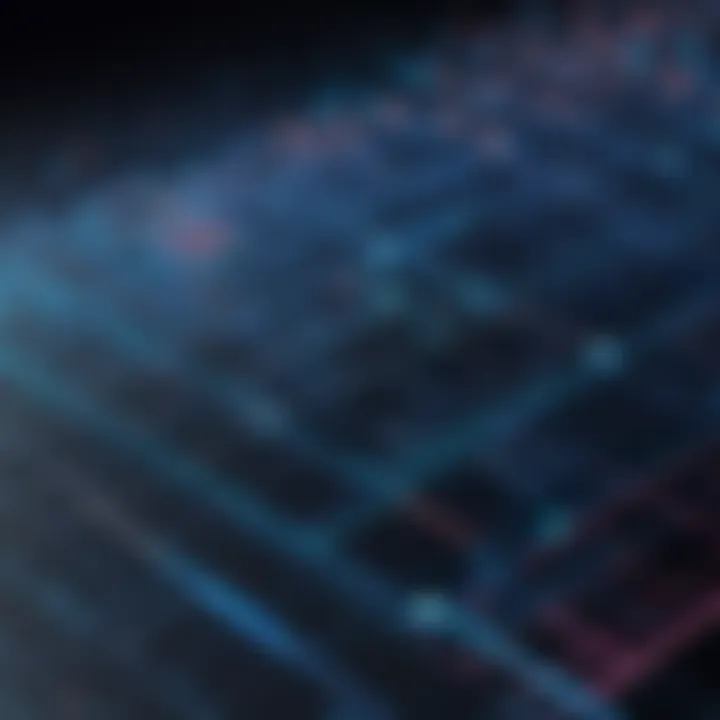
Intro
Technical interviews often pose challenges that are both practical and theoretical in nature. Among these challenges, a solid understanding of data structures stands out. Candidates must grasp these fundamental concepts deeply to navigate the complex problems they might face during interviews. Therefore, delving into data structures is essential for any aspiring developer or programmer.
Data structures are not just abstract concepts. They provide frameworks for organizing, managing, and storing data efficiently. Mastering them enables candidates to write better algorithms and understand the underlying principles that govern how data can be utilized. This article will explore different data structures, their applications in programming, and why they matter for interview preparation.
Understanding data structures can mean the difference between a successful and unsuccessful interview. Therefore, we will approach this subject methodically, ensuring that each section builds upon the last.
"Mastering data structures can significantly enhance problem-solving skills and is vital for technical interviews."
Each type of data structure has its own characteristics and use cases. By the end of this article, readers will not only know how to implement various data structures, but also when and why to use them. We will examine array, linked lists, stacks, queues, trees, and graphs, providing concrete examples and discussing their unique strengths and weaknesses.
Next, we will explore the importance of data structures in the software development life cycle and practical applications across various programming languages. A thorough understanding of these topics can offer a competitive edge in the job market and improve overall coding proficiency.
Preface to Data Structures in Interviews
Data structures form the backbone of computer science and programming. Understanding them is not just an academic exercise; it is essential in interview preparation for technical positions. Many interview processes emphasize knowing data structures because they are fundamental to writing efficient code. This section explores the significance of data structures in interviews.
Understanding the Role of Data Structures
Data structures organize and store data efficiently. The selection of an appropriate data structure can drastically affect the performance of an algorithm. In interviews, candidates often face problems where they need to choose the right data structure to solve specific tasks. For example, using a hash table for quick data retrieval contrasts with using a linked list for ordered element access. Knowing when and how to use these structures can demonstrate a candidate’s problem-solving abilities. Many technical challenges in interviews will test candidates' understanding of data structures.
Why Employers Value Data Structure Knowledge
Employers prioritize data structure knowledge because it directly influences the efficiency of software solutions. For instance, a deep understanding of trees or graphs can lead to optimizing algorithms for search and information retrieval. This knowledge shows that a candidate handles complex tasks effectively. Additionally, interviews testing for data structures often reveal a candidate's logical reasoning skills and ability to implement solutions. Therefore, showcasing this knowledge is essential for anyone looking to enter technical roles in software development.
"A strong grasp of data structures can set a candidate apart in a competitive job market."
The underlying principle behind these expectations is that software development relies heavily on effective data management. Possessing this knowledge helps candidates devise innovative solutions and contribute significantly to future projects. As candidates prepare for interviews, they must focus on honing their understanding of data structures. This focus aligns with industry needs and enhances their own problem-solving arsenal.
Fundamental Data Structures
Fundamental data structures form the backbone of efficient problem-solving in computer science. They enable developers to organize and store data in a manner that optimizes operations such as search, insertion, and deletion. Understanding these data structures can significantly enhance a candidate's ability to tackle technical interview questions and showcase their programming skills.
In interviews, a solid grasp of fundamental data structures may set a candidate apart. Many technical problems can be efficiently solved using these structures, making them indispensable tools. Furthermore, familiarity with these structures allows candidates to demonstrate their analytical thinking, which is a quality highly valued by employers.
Arrays: Basics and Applications
Arrays are one of the most basic data structures. They store elements in contiguous memory locations, allowing for efficient index-based access. This direct access is both a strength and a limitation; while retrieval is fast, inserting or deleting elements can require shifting others, which is time-consuming.
Arrays are used in various applications, from managing collections of data to implementing other data structures like heaps. For instance, they are integral to sorting algorithms. In scenarios where data size remains constant, arrays excel. However, their fixed size can be a drawback in systems requiring dynamic resizing.
Linked Lists: Types and Operations
Linked lists offer a flexible alternative to arrays, allowing for dynamic memory usage. Unlike arrays, linked lists do not require contiguous memory allocation. This aspect enables elements (nodes) to be efficiently inserted or deleted, making linked lists particularly useful in scenarios where data size fluctuates.
Single Linked Lists
Single linked lists consist of nodes containing data and a pointer to the next node. This structure's simplicity is a primary benefit, making it easy to implement and use. Operations like insertion at the beginning or end are straightforward. However, searching for an element can be slower than in arrays, due to the need for traversal from the head. Overall, single linked lists are popular for their simplicity and flexibility.
Doubly Linked Lists
Doubly linked lists enhance single linked lists by allowing traversal in both directions, forward and backward. This makes them suitable for scenarios requiring frequent inserts and deletes from both ends. While they offer a more versatile way to navigate the list, the trade-off is increased memory usage due to additional pointers. Their ability to enable two-way traversal can lead to more efficient algorithms in certain applications, despite requiring extra space.
Circular Linked Lists
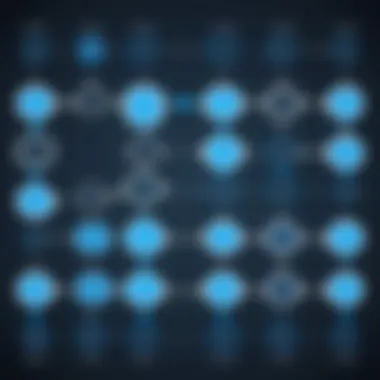
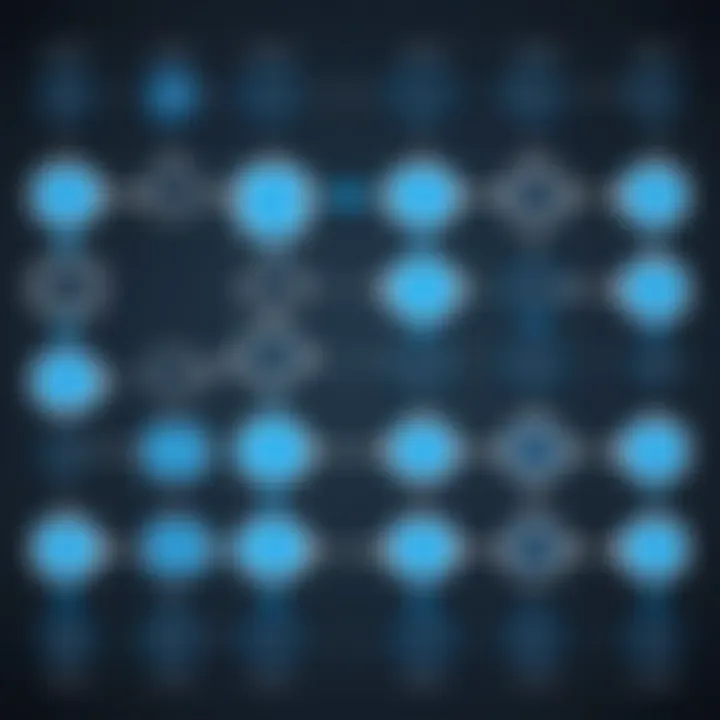
Circular linked lists connect the last node back to the first, creating a circular structure. This feature allows for continuous traversal without reaching an end. Circular linked lists are beneficial in applications like round-robin scheduling in operating systems. However, they can be complex to manage, particularly with regard to avoiding infinite loops. Their unique structure offers advantages in specific contexts, emphasizing the need for understanding different linked list types.
Stacks: Concepts and Use Cases
Stacks are collections of elements following the Last In First Out (LIFO) principle. This structure is vital in programming tasks like parsing expressions or managing function calls. Operations are usually defined as push (adding to the top) and pop (removing from the top). Stacks are often employed in scenarios needing the management of resources in a specific order, making them particularly helpful in algorithms.
Queues: Fundamental Principles
Queues operate on the First In First Out (FIFO) principle. They are crucial for managing tasks in a sequential manner. Queues find application in various fields such as scheduling processes in operating systems. Operations include enqueue (adding an element at the back) and dequeue (removing an element from the front).
Simple Queues
Simple queues are straightforward in implementation, using a straightforward structure to manage data. Their simplicity is a key characteristic, making them easy to understand and utilize. However, performance can degrade under high loads, leading to inefficiency due to the linear time complexity of operations in some implementations.
Priority Queues
Priority queues extend simple queues by assigning a priority to each element. The element with the highest priority is served before others. This feature is especially useful in scenarios like print job scheduling, where certain tasks require immediate attention. The complexity of implementing priority queues can vary, but their dynamic nature often outweighs the challenges.
Circular Queues
Circular queues optimize the use of array-based queues by treating the array as circular. This arrangement helps to achieve better memory utilization and avoid wastage due to the static nature of regular queues. While they require careful management to maintain the circular structure, their efficiency in resource allocation makes them a valuable choice in many applications.
Understanding fundamental data structures is essential for efficient coding and problem-solving in technical interviews.
These fundamental data structures provide the building blocks for various algorithms and applications, enabling candidates to demonstrate their proficiency during technical interviews.
Advanced Data Structures
Advanced data structures play a critical role in efficient data management and retrieval. Understanding these structures helps candidates navigate complex scenarios often encountered in technical interviews. Their incorporation allows for optimized algorithms, influencing both time and space complexity in problem-solving. Mastering advanced data structures is essential for developers who aim to solve intricate problems, ensuring they can handle various data challenges effectively.
Trees: Structure and Types
Binary Trees
Binary trees consist of nodes, with each parent node having two children at most. This structure is fundamental due to its simplicity and effectiveness in sorting and retrieving data. The unique aspect of binary trees is their hierarchical nature, allowing for efficient traversal methods such as in-order, pre-order, and post-order. Their balance affects performance, making balanced binary trees more beneficial for maintaining quick access and modification times.
Binary Search Trees
A binary search tree is a specific type of binary tree that maintains order. Each node has only two children, and the left subtree contains nodes with lesser values while the right subtree holds nodes with greater values. This structure allows for efficient searching, insertion, and deletion operations, typically achieved in O(log n) time. However, unbalanced trees can degrade to O(n) performance, leading to longer search times.
AVL Trees
AVL trees are a self-balancing type of binary search tree. The key characteristic is that the height difference between left and right subtrees remains no more than one. This balance helps maintain O(log n) time complexity for most operations. The unique feature of AVL trees lies in the automatic balancing process initiated after insertions or deletions, which can come with overhead due to rotation operations, but the efficiency gains in searching often justify this trade-off.
Red-Black Trees
Red-black trees are another self-balancing binary search tree variant. The defining characteristic is the use of colors (red and black) assigned to nodes to enforce balance rules. This structure guarantees that the longest path from root to leaf is no more than twice as long as the shortest path. Thus, it maintains O(log n) performance for insertion, deletion, and lookup, with smaller rigidity compared to AVL trees, resulting in potentially faster insertion and deletion operations.
Graphs: Representations and Algorithms
Directed vs. Undirected Graphs
Graphs are essential for representing relationships between entities. In directed graphs, edges have a direction, indicating a one-way relationship. In contrast, undirected graphs represent mutual relationships. Understanding the distinction aids in the right choice for modeling problems. Directed graphs are beneficial for scenarios like web page links, while undirected graphs excel in social network connections.
Graph Traversals
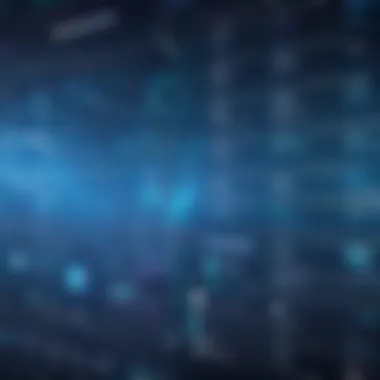
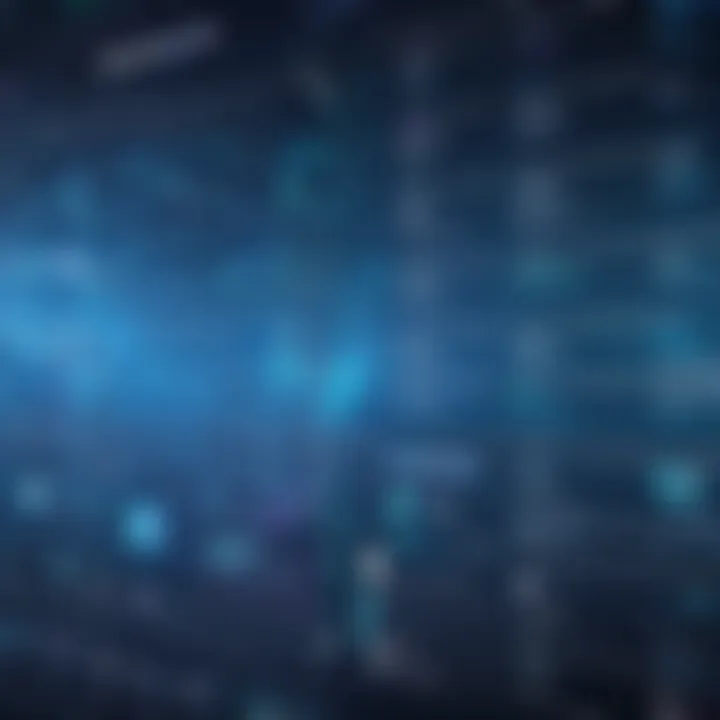
Graph traversal strategies like breadth-first search and depth-first search are fundamental algorithms used to explore nodes. Each method has unique benefits: breadth-first search is optimal for finding the shortest path in an unweighted graph, while depth-first search is useful for applications requiring comprehensive exploration. Knowing these traversal techniques is crucial in interview contexts where algorithm efficiency is evaluated.
Shortest Path Algorithms
Algorithms such as Dijkstra's and Bellman-Ford focus on finding the shortest path in graphs. Dijkstra's algorithm efficiently handles graphs with non-negative weights. In contrast, Bellman-Ford can manage negative weights and detects cycles. These algorithms are pivotal for applications ranging from networking to route planning, making them vital knowledge for technical interviews.
Hash Tables: Efficiency and Collision Resolution
Hash tables create a fast lookup performance by using a hash function to compute an index in an array. It allows for O(1) average time complexity for search and insert operations. However, effective collision resolution is necessary as multiple entries may hash to the same index. Common methods include chaining and open addressing. Knowledge of hash tables enhances understanding of data organization and retrieval, essential for interview scenarios where performance is scrutinized.
Data Structure Performance Analysis
In the realm of programming, understanding data structure performance is essential. For candidates preparing for interviews, it is not enough to know the theoretical application of various data structures. Aspiring developers must be able to analyze their efficiency in terms of time and space. This section covers the critical concepts of Big O Notation, time complexity, and space complexity. Each of these elements provides insight into how data structures operate under different circumstances. Knowledge in these areas can enhance decision-making in selectin appropriate structures for specific tasks.
Big O Notation: A Brief Overview
Big O Notation is a mathematical concept used to describe the performance or complexity of an algorithm. It provides a high-level understanding of the scalability of algorithms as the input size increases. Key aspects to understand include:
- Efficiency Measurement: Big O helps to estimate the worst-case scenario of an algorithm’s growth rate.
- Comparison Tool: With Big O, one can compare different algorithms objectively. This comparison considers factors like execution time and memory usage relative to input size.
- Notation Simplification: It abstracts constants and lower order terms, focusing solely on the dominant factor that affects performance.
Common Big O notations include O(1), O(n), O(n^2), and O(log n), each representing different levels of algorithm efficiency.
Time Complexity Examples
Time complexity quantifies the amount of time an algorithm takes to complete relative to the size of its input. Understanding this concept is vital for selecting the right data structures in interviews. Key examples include:
- Constant Time - O(1): Accessing an array element by index is an O(1) operation because it takes a fixed amount of time regardless of the array size.
- Linear Time - O(n): Searching through an unsorted list requires checking each element sequentially, resulting in O(n) time complexity.
- Quadratic Time - O(n^2): A nested loop structure, such as bubble sort, exhibits O(n^2), taking time proportional to the square of the input size.
When preparing for interviews, it is prudent to practice coding algorithms of varying time complexities.
Space Complexity Considerations
Space complexity measures the amount of memory space required by an algorithm as the input size increases. This factor is crucial to understand especially when dealing with large datasets or limited memory. Key points to consider include:
- Memory Usage: Similar to time complexity, space complexity often categorizes algorithms in terms of O notation, such as O(1), O(n), or O(n^2).
- Shape of Data: Different data structures consume memory differently. For instance, a linked list may require more space than an array due to pointers.
- Trade-offs: When choosing data structures, consider both time and space complexity. A structure that uses less time might consume more space and vice versa.
Understanding both the time and space complexities of various data structures can significantly influence performance during implementation.
"The right choice of data structure is essential for achieving optimal performance in coding." - A fundamental principle in software development.
Common Interview Questions on Data Structures
Understanding common interview questions related to data structures is crucial for candidates preparing for technical interviews. These questions often reflect an employer's interest in a candidate's problem-solving abilities and coding proficiency. Interviewers use them to gauge how well candidates grasp essential concepts in programming and algorithm design, which are vital in the tech industry. Additionally, mastering these questions enables candidates to demonstrate their thought processes effectively and to articulate their reasoning behind chosen solutions.
The focus on data structures in interviews highlights their significance not just in academic settings but also in practical applications within the software development lifecycle. This section will explore various types of questions that candidates may encounter, giving insights into how to approach them and the thought processes involved.
Array-Based Questions
Array-based questions are common in interviews due to the fundamental role arrays play in programming. Candidates might be asked to manipulate arrays, search for elements, or optimize operations. Typical questions include tasks such as finding duplicates in an array or merging two sorted arrays.
Often, interviewers seek not just a working solution but an efficient one. For example, a question might involve finding the maximum product of two integers in an array. A candidate could implement a brute force solution with a time complexity of O(n^2) by using nested loops, but a more efficient approach would involve iterating through the array once, making the solution O(n).
Candidates should be prepared to discuss their time and space complexity analysis alongside their code. Understanding the trade-offs between different approaches is key, as is having the ability to articulate these distinctions in a clear manner.
Linked List Problems
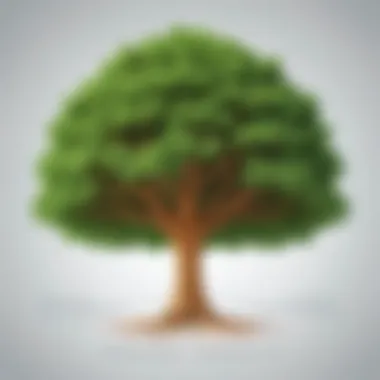
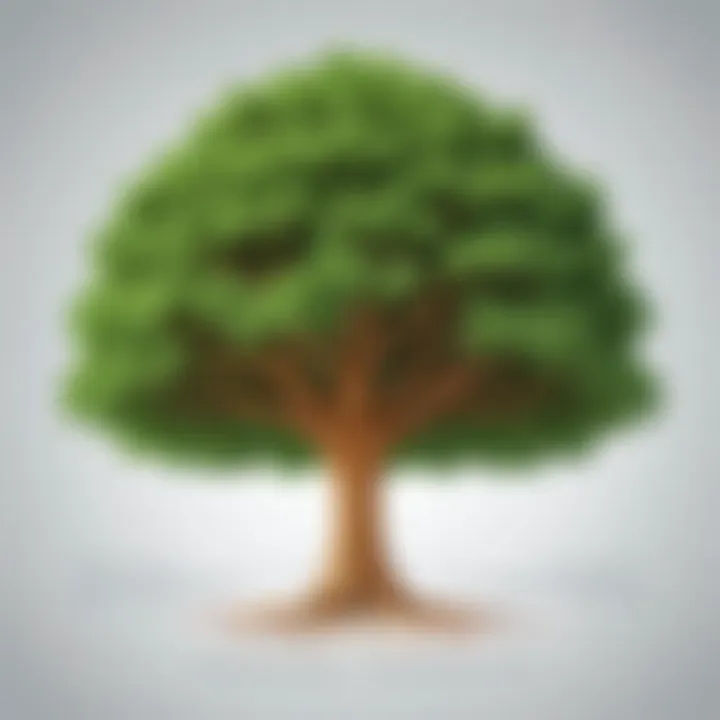
Linked list problems are frequently presented in interviews, challenging candidates to demonstrate their grasp of dynamic data structures. Common questions focus on operations such as reversing a linked list or detecting cycles within it. Candidates might be asked to implement algorithms that manipulate the linked list structure effectively.
For instance, a question like "How would you detect a cycle in a linked list?" requires a clear understanding of pointers and the concept of slow and fast traversal techniques. Here, using Floyd’s Cycle-Finding Algorithm allows for detection with O(n) time complexity and O(1) space usage. In interviews, detailing the thought process behind the chosen approach can set candidates apart from others.
Stack and Queue Challenges
Stacks and queues are essential data structures with unique properties that lend themselves to specific problems. Interview questions might involve implementing stack and queue operations, evaluating expressions using stacks, or using queues to manage process scheduling.
A classic challenge might involve evaluating a postfix (Reverse Polish Notation) expression. Candidates must demonstrate their ability to utilize a stack by pushing elements and popping them as needed to compute the final result. Emphasizing the depth of understanding in how stacks operate can enhance the effectiveness of their interviews.
Similarly, questions about queues often revolve around real-world scenarios, such as handling requests in web servers or managing print jobs. Understanding the differences between simple queues, priority queues, and circular queues can provide valuable context in these discussions.
Tree and Graph Scenarios
Tree and graph scenarios present a different level of complexity, often employing recursion and traversals. Interviewees might face questions regarding depth-first search and breadth-first search algorithms on binary trees or general graphs. The capability to traverse structures and count nodes or find paths within trees illustrates a solid comprehension of hierarchical data.
Consider a question like: "How would you determine if a binary tree is height-balanced?" Candidates can reference algorithms that utilize recursion, showcasing both problem-solving skills and familiarity with tree properties. Another common type of question might relate to graph traversal methods, such as finding the shortest path between nodes, which emphasizes practical algorithms like Dijkstra’s or A*.
Familiarity with these questions not only prepares candidates for the interview but also bolsters their overall confidence in handling real-world programming challenges.
Tips for Mastering Data Structures
Mastering data structures is vital for anyone preparing for technical interviews in programming and software development. Understanding these concepts thoroughly not only helps in solving coding challenges but also deepens the knowledge required to make effective decisions in software design. The emphasis on practical skills, coupled with theoretical knowledge, can significantly impact a candidate’s performance.
Practical Coding Exercises
Engaging in practical coding exercises is one of the best ways to solidify understanding of data structures. It allows candidates to apply their theoretical knowledge in real scenarios. Consider the following points when incorporating coding exercises into your study routine:
- Hands-on Practice: Simply reading about data structures is not enough. Implementing them through coding reinforces memory and understanding. Websites like LeetCode and HackerRank offer numerous problems tailored specifically for practicing different data structures.
- Diverse Scenarios: Tackle a wide range of problems that require different data structures. This diversity aids in recognizing when to use which structures effectively. Start with easier problems and gradually move to complex ones.
- Time Yourself: Simulating interview conditions by timing your exercises creates familiarity with the pressure of actual interviews. This practice can help improve speed and accuracy.
Utilizing Online Resources
The internet is full of resources that can enhance your understanding of data structures. Optimization of learning can be achieved through various online platforms:
- Tutorials and Courses: Websites like Coursera and edX offer specialized courses focused on data structures and algorithms. These can be structured and provide a well-rounded education.
- Video Lectures: YouTube hosts many educational channels that break down complex topics into digestible pieces. Watching these can help visualize how data structures work in action.
- Interactive Platforms: Use interactive coding platforms such as Codecademy, which lets you practice directly in the browser while receiving immediate feedback on your solutions.
Peer Discussions and Study Groups
Learning in isolation can often lead to gaps in understanding. Hence, involving peers in your study process can be beneficial:
- Create Study Groups: Collaborating with peers allows for shared knowledge and different perspectives on problem-solving. Each member can tackle a specific data structure and present their findings back to the group.
- Engage in Discussions: Participate in forums such as Reddit or Stack Overflow. Discussing problems and solutions helps solidify concepts and gain insights that may not be immediately clear from textbooks or online tutorials.
- Mock Interviews: Organizing mock interview sessions with peers can help simulate real interview experiences. This builds confidence and helps identify areas that need improvement.
Regular practice, combined with discussion and feedback from peers, builds a solid foundation in data structures.
Mastering data structures is not a one-time effort; it requires continuous learning and adaptation. As you prepare for interviews, remember these tips. They will guide your journey towards becoming proficient and confident in your knowledge of data structures.
Ending
In the realm of technical interviews, a solid grasp of data structures becomes crucial for candidates aiming to impress potential employers. Understanding the fundamentals of data structures not only reflects a candidate's readiness for coding challenges but also demonstrates the depth of their analytical skills.
Reinforcing the Importance of Data Structures
Data structures serve as the backbone of efficient software development. They allow candidates to organize and manage data effectively, which is essential when addressing complex problems during interviews. When a candidate is proficient with arrays, linked lists, trees, and other structures, it becomes easier to write optimized algorithms for specific tasks. This knowledge can set aparts a candidate in a competitive job market. Employers are thus inclined to prefer those who showcase a clear understanding of how to utilize each data structure to solve problems systematically.
"Mastering data structures is not merely an academic exercise; it is the key to unlocking sophisticated problem-solving capabilities."
Final Thoughts on Interview Preparation
Preparation for interviews requires more than just standard practice questions. A deep understanding of data structures enhances a programmer's ability to think critically and approach problems logically. It allows for better performance under pressure and equips candidates with the tools they need to adapt their solutions in real time.
Candidates should actively engage with coding challenges and create a routine around practice. Online platforms provide a multitude of resources that can help reinforce this knowledge. Furthermore, peer discussions can lead to deeper insights and understanding. Ultimately, the preparation process should not be daunting; instead, it should be viewed as an opportunity to immerse oneself in the fascinating world of programming.