Data Science Projects with Python: A Complete Guide
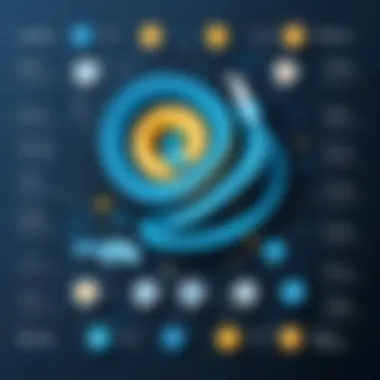
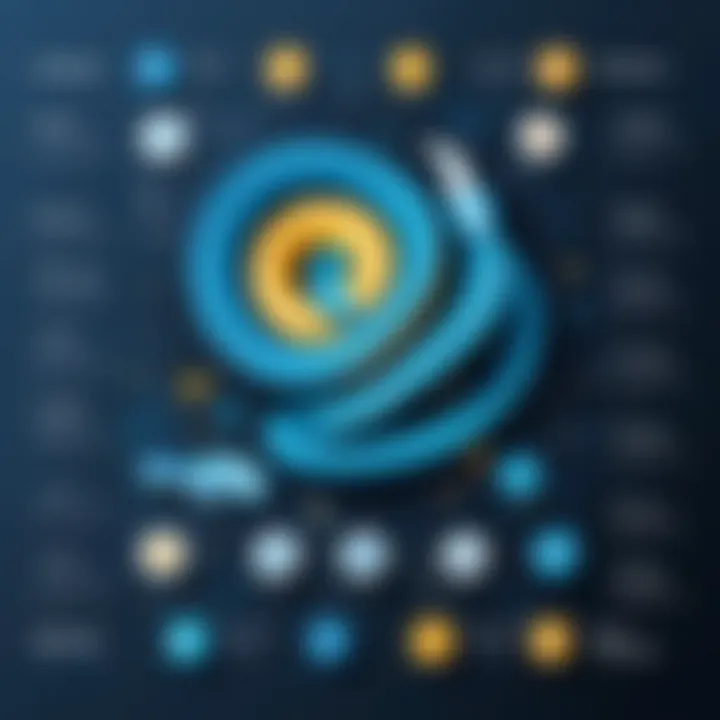
Prolusion to Programming Language
In the realm of data science, Python has carved out a significant place, making it the go-to programming language for many aspiring data scientists, analysts, and developers. Its simplicity and versatility are not mere coincidences; they stem from a robust history and a clear vision of what programming should be: accessible yet powerful.
History and Background
Python emerged in the late 1980s, designed by Guido van Rossum. The first version was released in 1991. From its inception, Python was created with readability in mind, emphasizing code that looks similar to plain English. This focus has made it a preferred choice for both beginners and experienced programmers. Over the years, Python has been adopted widely across diverse industries, ranging from web development to scientific computing.
Features and Uses
Python boasts a plethora of features that facilitate data manipulation and analysis:
- High-level Language: It abstracts complex coding details, allowing you to focus on problem-solving.
- Rich Libraries: Libraries like Pandas, NumPy, and Matplotlib provide powerful tools for data handling and visualization.
- Community Support: A strong, active community means solutions to problems are often just a search away.
- Cross-Platform: Python scripts run seamlessly across various operating systems, enhancing collaborative projects.
- Dynamic Typing: This feature encourages rapid development, making it easy to modify data types without extensive code rework.
Popularity and Scope
As of 2023, Python has surged in popularity, consistently ranking among the top programming languages. This rise can be attributed to its role in burgeoning fields like machine learning and artificial intelligence. According to data from Wikipedia), Pythonās community has expanded dramatically, leading to increased libraries and frameworks that make data science more approachable for beginners.
The scope of Python extends beyond data science; itās widely used in fields such as web development, game development, and automation, marking it as a multifaceted language that caters to a wide array of programming needs.
Basic Syntax and Concepts
Understanding the foundational elements of Python is vital for any data science project. Let's break down some core concepts:
Variables and Data Types
Variables in Python are straightforward. You can declare a variable simply by assigning it a value. For instance:
Python supports several data types:
- Integers: Whole numbers like 5, 100, or -27.
- Floats: Numbers with decimals, e.g., 3.14.
- Strings: Text enclosed in quotes, such as "Hello World!".
- Booleans: Represents True or False.
Operators and Expressions
Python provides a range of operators to perform calculations:
- Arithmetic Operators: +, -, *, /, %, etc.
- Comparison Operators: ==, !=, >, , etc.
- Logical Operators: and, or, not.
Control Structures
Control structures guide the flow of your program. Common structures include:
- If Statements: To execute code based on conditions.
- Loops: Such as For and While loops for repeating code blocks.
Advanced Topics
For those who have grasped the basics, exploring advanced topics can deepen your understanding and prowess in Python programming.
Functions and Methods
Functions are blocks of code designed to perform a specific task. They can be defined using the keyword. For example:
Object-Oriented Programming
Python supports object-oriented programming (OOP), which allows for data encapsulation and inheritance. Hereās a quick example:
Exception Handling
Errors can disrupt the flow of programs. Python provides a way to handle errors gracefully using try and except blocks:
Hands-On Examples
Now, let's apply some of what we've learned through practical coding examples emphasizing various skill levels.
Simple Programs
A simple calculator program could be a perfect starting point:
Intermediate Projects
Building a basic web scraper using Beautiful Soup can introduce you to web data collection, an essential skill in data science:
Code Snippets
To enhance your projects, consider incorporating snippets that automate repetitive tasks, like cleaning datasets:
Resources and Further Learning
For continuous improvement, here are some excellent resources:
- Recommended Books:
- Online Courses and Platforms:
- Community Forums and Groups:
- "Python for Data Analysis" by Wes McKinney
- "Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" by AurƩlien GƩron
- Coursera
- edX
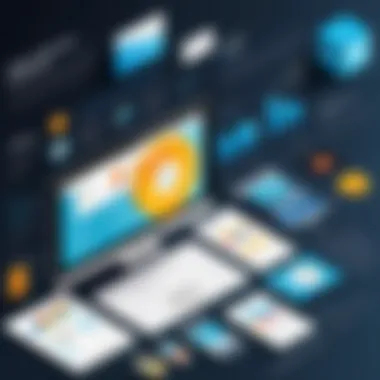
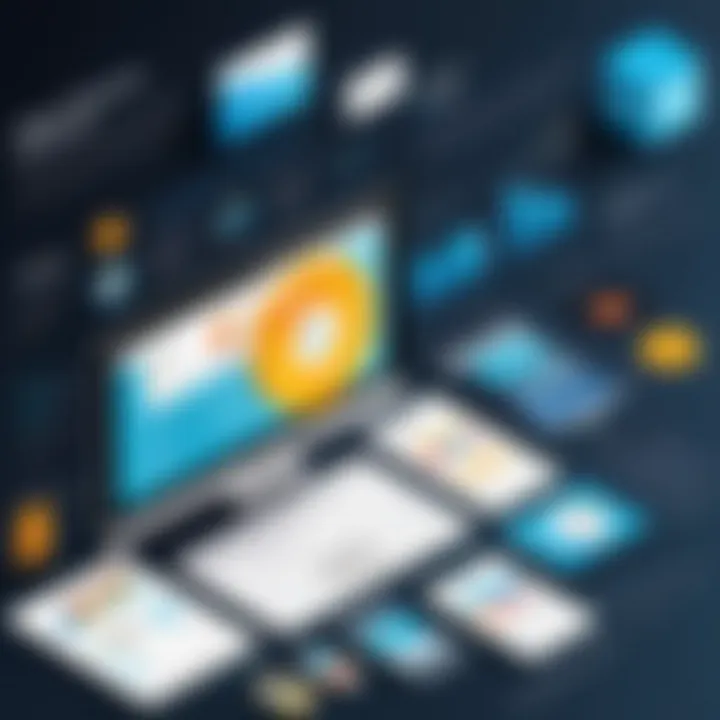
- Reddit has thriving discussions on projects and challenges.
- Facebook groups dedicated to Python programming are abundant with resources and support.
Learning data science is like piecing together a jigsaw puzzle; each project you undertake and every new concept you grasp dips your brush deeper into the art of programming.
Intro to Data Science and Python
In todayās fast-paced world, where data is crucial for decision-making, understanding data science is not just a luxury but a necessity. The marriage of data science and Python brings a powerful toolkit to the table, enabling individuals to analyze, interpret, and derive meaningful insights from data. This section serves as an introduction to the fundamental concepts surrounding data science and highlights Python's integral role in this domain.
Data science can be defined as a multi-disciplinary field that utilizes various techniques, algorithms, and systems to extract knowledge and insights from structured and unstructured data. Itās a blend of statistics, computer science, and domain-specific knowledge. As the volume of data grows exponentially, organizations increasingly rely on data scientists to turn raw data into actionable strategies.
Python, one of the most popular programming languages, has transformed the landscape of data science. Its simplicity and readability make it an ideal choice for both beginners and experienced programmers. With a rich ecosystem of libraries tailored for data manipulation, visualization, and machine learning, Python is equipped to tackle nearly any data challenge.
Defining Data Science
Understanding what data science is entails comprehending its foundational components. Data science serves as the backbone for making data-driven decisions across industries. It encompasses several vital processes, such as:
- Data Collection: Gathering data from various sources, both structured (such as databases) and unstructured (like text and images).
- Data Cleaning: Ensuring the data is accurate and usable by identifying and fixing errors. Missing or incorrect data can skew results.
- Data Exploration: Delving into the data to understand trends, patterns, and insights. This phase often employs descriptive analytics to summarize the present conditions of the data.
- Model Building: Constructing predictive models using statistical and machine learning algorithms. This step involves training the model and validating its accuracy.
- Communication: Presenting findings in a clear and understandable manner, often through visual aids like graphs and charts.
Each of these phases contributes holistically to the data analysis process, making data science essential for businesses aiming to harness insights from their vast amounts of data.
"Data is the new oil; itās valuable, but if unrefined it cannot really be used."
This saying encapsulates the essence of data scienceāthe necessity of refining data into a usable format.
Role of Python in Data Science
The role of Python in data science cannot be overstated. Its ability to easily integrate with other tools and technologies makes it a flexible ally in data-centric projects. Some of the significant advantages of Python include:
- Ease of Learning: Python's syntax is straightforward, allowing newcomers to quickly pick up the ropes, while still being powerful enough for seasoned professionals.
- Extensive Libraries: Python is home to libraries specifically designed for data science, such as NumPy for numerical data, Pandas for data manipulation, and Matplotlib for data visualization. Scikit-learn further elevates Python's capabilities by offering robust machine learning tools.
- Community Support: A thriving community of developers and data scientists ensures that help is readily available. From forums like Reddit to documentation on platforms such as Facebook, one can find resources and insights easily.
Key Libraries for Data Science Projects
In the realm of data science, especially with Python, libraries are the backbone supporting a myriad of tasks. These libraries provide pre-written code and robust functionality that significantly speed up the workflow. They allow practitioners to focus on analysis rather than reinventing the wheel. Each library offers unique capabilities, catering to a variety of data handling, analysis, and visualization needs. When embarking on a data science project, selecting the right libraries becomes crucial for efficiency and effectiveness.
NumPy: The Foundation of Data Manipulation
NumPy, short for Numerical Python, is fundamentally the first library you need for any data manipulation. It creates n-dimensional arrays, a powerful data structure that allows users to perform mathematical operations efficiently. The power of NumPy lies in its speed; operations on NumPy arrays are significantly faster than those on regular Python lists or arrays.
Take, for instance, the need to process large datasets. Using NumPy, you can run complex calculations in a fraction of the time compared to other methods. Some key features of NumPy include:
- N-dimensional support: Numbers are arranged in matrices, enabling multi-dimensional analyses.
- Mathematical functions: Provides a broad selection of functions for linear algebra, Fourier transforms, and random number generation.
- Interoperability: Easily integrates with other data science libraries like Pandas and Scikit-learn.
Pandas: Data Analysis Made Easy
Pandas makes data analysis straightforward and intuitive. It introduces two primary data structures: Series and DataFrame. Both are designed for handling structured data, with DataFrame resembling a table where each column can be of different types (e.g., integers, floats, strings).
Using Pandas is like having a Swiss Army knife for data operations. Hereās what it can do:
- Data Cleaning: Easily handles missing values and duplicates.
- Data Aggregation: With group by functionality, users can summarize data concisely.
- File I/O: Read and write data in various formats such as CSV, Excel, and JSON effortlessly.
This makes Pandas an essential tool for students and budding data analysts who want to streamline their workflows.
Matplotlib and Seaborn: Visualizing Data
When it comes to visualizing data, Matplotlib is the go-to library that provides a standard suite of tools for creating static, animated, and interactive visualizations. It allows for flexible, customizable plots that cater to any presentation requirement.
Seaborn builds on Matplotlib's groundwork, offering a higher-level interface for more attractive statistical graphics. It simplifies complex visualizations with fewer lines of code while also introducing additional plotting options and styles. Here are some features:
- Variety: From scatter plots to heatmaps, you can create a diverse range of visuals.
- Data Relationships: Visualize relationships between data variables with ease.
- Styling: Aesthetically pleasing visualizations that are publication-ready.
Simply put, for the presentation of findings, visualizations crafted using Matplotlib and Seaborn can speak volumes.
Scikit-learn: Machine Learning Capabilities
Scikit-learn is pivotal for anyone wanting to dive into machine learning. It encompasses a range of algorithms for classification, regression, and clustering, all standardized in simple APIs that ensure user-friendliness. The libraryās breadth makes it adaptable for different projects, no matter their complexity.
Some standout features of Scikit-learn include:
- Model Selection: Functions like cross-validation and grid search help in selecting the right algorithm.
- Feature Engineering: Tools for processing data to improve model performance.
- Documentation: Exceptionally detailed documentation and examples make it accessible to newcomers.
Overall, Scikit-learn is invaluable for data scientists who wish to implement machine learning models effectively.
For anyone venturing into data science projects using Python, gaining familiarity with these libraries makes learning feel less like climbing a steep hill and more like a gradual trek through a scenic route.
Essential Data Science Project Types
Understanding the various types of data science projects is crucial for anyone looking to carve their niche in this ever-evolving field. Each project type, whether predictive modeling or natural language processing, offers unique challenges and learning opportunities. Knowing these project types helps to align one's skills with industry needs and to identify areas for further study or professional development. They represent practical applications of theoretical knowledge, transforming abstract concepts into tangible outcomes.
Predictive Modeling Projects
Predictive modeling projects allow data scientists to make forecasts based on historical data. This form of modeling is incredibly valuable across industries. For instance, a retail business may use predictive modeling to forecast customer purchasing patterns during the holiday season.
To build a predictive model, the data scientist typically engages in a series of steps: collecting relevant data, cleaning it, choosing the right algorithms for model training, and then validating that model. A popular method involves using machine learning algorithms such as linear regression or more advanced techniques like decision trees. The ultimate goal is not just to predict outcomes but rather to understand factors that influence those predictions.
"The only thing worse than training your employees and having them leave is not training them and having them stay."
Data Visualization Projects
Data visualization projects are essential for communicating insights derived from data in a clear and compelling way. Itās one thing to analyze data; itās another to tell a story with it. Good data visualization makes raw data accessible and understandable, often transforming complex datasets into engaging visuals, like graphs or infographics.
Tools like Matplotlib and Seaborn in Python are commonly employed for these tasks. Consider a project where a data scientist aims to illustrate trends in global temperatures. They might use various visualization techniques to show spikes in data, comparisons over time, or geographical distributions. In doing so, they open pathways for stakeholders to grasp trends and make informed decisions based on visual interpretations.
Natural Language Processing Projects
Natural Language Processing (NLP) projects focus on the interaction between computers and humans through natural language. NLP is a rapidly growing field with applications ranging from sentiment analysis to chatbots.
Imagine a sentiment analysis project analyzing Twitter data to assess public opinion on a recent political event. Using libraries such as NLTK or spaCy, a data scientist might tokenize the text, identify sentiment scores, and visualize the results. Such projects expose learners to linguistics, coding, and machine learning, making them a multifaceted avenue within data science.
Computer Vision Projects
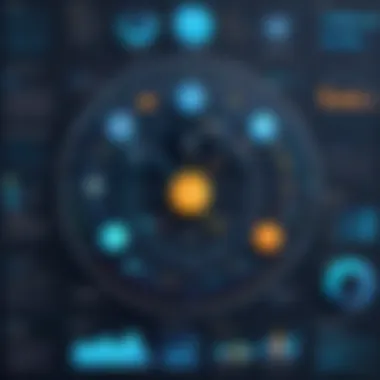
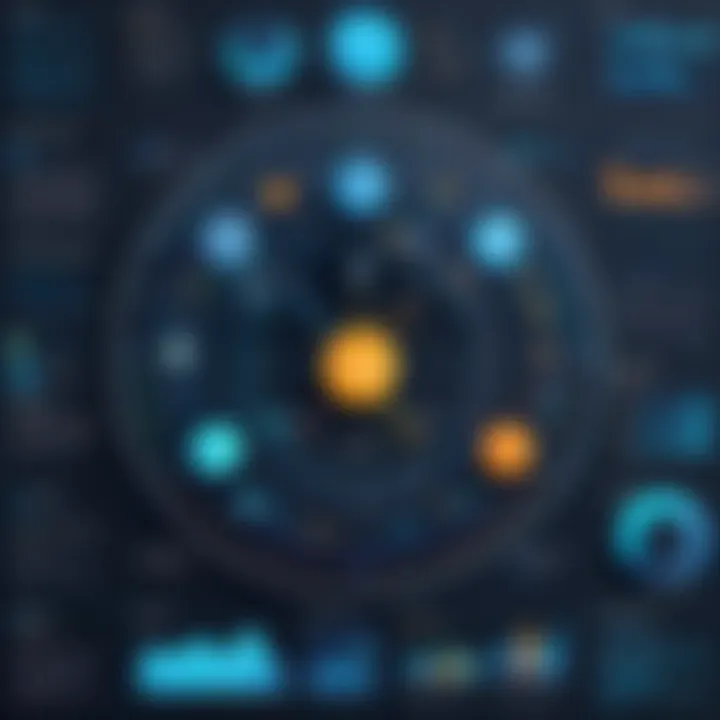
Computer vision projects enable machines to interpret and make decisions based on visual data. This area has gained massive traction with the rise of deep learning frameworks like TensorFlow and PyTorch. A common example might include an image classification project where a model distinguishes between different breeds of dog images.
In such a project, combining labeled datasets with convolutional neural networks can yield impressive results. Engaging in computer vision projects sharpens skills in image processing and model evaluation while offering a taste of how technology can impact real-world tasks like object detection in autonomous vehicles.
Through exploring these essential project types, aspiring data scientists can broaden their scope and focus on specific areas that interest them. Each type poses its unique challenges and requires a blend of analytical and technical skills, encouraging continuous learning and practical application.
Collecting Data for Your Project
Data collection forms the backbone of any successful data science project. It is through this process that raw data is transformed into valuable insights, making it a key player in driving the outcomes of your analysis. Getting quality data defines the trajectory of your work. Proper collection methods not only enhance the validity of your results but also ensure the effectiveness of the models that you will later implement. In this section, we will delve into various methods for collecting data, including web scraping, utilizing APIs, and tapping into public datasets.
Web Scraping Techniques
Web scraping is a valuable skill that assists data scientists in gathering information from websites. It involves extracting content from web pages, which can then be used for various analyses. Imagine needing to analyze the pricing patterns of a competitorāweb scraping can fetch the latest data from their online stores without manually gathering it.
There are several tools and libraries that facilitate web scraping in Python, such as Beautiful Soup and Scrapy. They allow you to parse HTML and XML documents, enabling extraction tasks to be completed smoothly. Itās crucial to stay aware of a websiteās terms of service, because not all sites permit scraping. Ignoring these guidelines can lead to unwanted consequences. The ethical aspect cannot be overstated. Whenever you harvest data, remember to act responsibly. Hereās a brief outline of the steps involved in web scraping:
- Identify the website: Determine where your data lies.
- Inspect the page: Use web browser tools to view the HTML structure.
- Write the scraper code: Use Beautiful Soup or Scrapy to extract the desired data.
- Store the data: Save it in a structured format like CSV or JSON for later analysis.
"Understanding how to scrape data effectively transforms the data science landscape for your projects. Not only can you uncover hidden patterns, but you can also enrich your datasets considerably."
Using APIs for Data Access
APIs, or Application Programming Interfaces, represent a treasure trove for data access. They enable you to pull information directly from services and platforms without needing manual data collection. This is particularly useful for live data and maintaining data accuracy. Popular platforms like Twitter and Google Maps offer APIs that allow developers to access their data seamlessly.
The process of using APIs usually entails:
- Authenticating your API key: Most APIs require you to register and obtain a key for security.
- Making requests: Utilize libraries like in Python to send requests to the API endpoint.
- Parsing the response: The data returned is often in JSON format, which you can then manipulate and analyze according to your needs.
APIs offer advantages such as real-time data access and higher reliability than scraping, as they follow the guidelines set by the service providing the data. Keeping up with API limitations is vital, as frequent calls may lead to exceeding usage limits.
Public Datasets: Where to Find Them
For those who might not want to scrape or utilize APIs, public datasets present a viable alternative. Numerous organizations and universities share datasets that are free for use. Websites such as Kaggle, UCI Machine Learning Repository, and government data portals provide easily accessible datasets on a vast range of topics.
When searching for public datasets, consider the following:
- Relevance: Ensure the data aligns with your project objectives.
- Quality: Assess the dataset for completeness, accuracy, and consistency.
- Licensing: Be aware of any restrictions regarding usage and distribution.
Some examples of popular public dataset sources include:
Finding the right dataset can save time and resources while providing a solid foundation for your data science projects. Engaging with these resources opens a world of possibilities for your exploration.
Data Cleaning and Preprocessing
In the realm of data science, one can't overlook the significance of data cleaning and preprocessing. Consider it the unsung hero of any data project. If the foundation isnāt solid, any analysis built on top will be shaky at best. Data in its raw form often resembles a messy room. It might have the key elements, but if you donāt get rid of the clutter, finding what you need can be quite the challenge.
Cleaning data tends to involve several steps which could drastically affect the outcomes of your analyses. From removing duplicate entries to handling ill-formatted input, the process isnāt just about tidiness; it's about enhancing the overall quality of your insights. When done right, these steps help to ensure that the data scientists can trust their models. Data cleaning might seem like a chore, but it lays the groundwork for robust data-driven decision-making, which is invaluable in any project.
Identifying and Handling Missing Values
One of the most common hurdles data scientists face is dealing with missing values. Itās almost like that one puzzle piece thatās always missing; it throws everything off balance. Missing data can arise for a variety of reasons: maybe the data wasnāt collected correctly, maybe an entry was skipped, or perhaps thereās just a discrepancy in the sources.
Ignoring missing values can lead to skewed results. So what can you do? Identifying where the gaps are is the first step. Here are a few common strategies for handling them:
- Imputation: This technique fills in the blanks. You might use the mean, median, or mode to replace missing values, depending on the data distribution.
- Deletion: If the holes are minimal, sometimes the best course of action is to remove those entries entirely. But tread carefully; when too much data is removed, it could skew the analysis.
- Flagging: Another approach is to create a flag column to indicate whether data is missing. This helps keep track of potential impacts on the model.
"The challenge isnāt just identifying missing values but deciding how to address them while minimizing bias."
Data Normalization Techniques
After the dust settles from dealing with missing values, the next step often involves data normalization. Generating consistent datasets enables better comparisons and analysis. Think of normalization as the process of bringing different scales down to a common denominator.
When handling large datasets, especially if they come from various sources, youāll likely encounter a mishmash of units and scales. It can be bewildering! Normalization is crucial for algorithms that compute distances or utilize gradient descent. Here are common techniques:
- Min-Max Scaling: This rescales the feature to a fixed range, usually between 0 and 1. The formula is:[ ]
- Z-Score Normalization: For those who want to account for standard deviations, Z-score normalization is an option that gives you a distribution centered around zero with a standard deviation of one.[ ]
- Log Transformation: This helps to address skewed data, making it more normally distributed. Itās especially useful in cases where you face exponential growth or heavy-tailed distributions.
Using normalization techniques can vastly improve model performance. It not only speeds up convergence during training but also enhances the interpretability of your dataset.
In summary, data cleaning and preprocessing is instrumental in ensuring that your project stands on stable ground. It lays the foundation for subsequent phases of analysis, modeling, and ultimately, decision-making.
Executing the Data Science Project
In the realm of data science, executing your project can often be the make-or-break phase. This is where the theoretical concepts learned earlier take shape into tangible outcomes. It involves a systematic approach to ensure that the model not only meets the expectations but also solves the problem at hand. An efficient project execution provides clarity, maintains timelines, and significantly increases the chances of success.
More often than not, the practical application of data science concepts often reveals insights that theoretical knowledge alone can't capture. Engaging in this phase forces one to critically think about data, methodologies, and results, pushing the boundaries of one's understanding. The subsequent actions during execution directly impact how the model behaves and how reliable the insights generated will ultimately be.
Developing the Model
At the heart of any data science project is the development of the model. This process typically requires selecting the right algorithms based on the problem type, data characteristics, and expected outcomes. Implementing models revolves around not just picking algorithms at random, but instead,
- Evaluating the complexities of the datasets
- Considering how different algorithms work under varying circumstances
- Understanding hyperparameters that need adjustment
For example, if you're working on predictive modeling, you might consider linear regression, decision trees, or perhaps neural networks. Each will come with its benefits and caveats. Therefore, itās not merely about applying algorithms; itās about choosing them wisely.
Key Steps in Model Development:
- Data Partitioning: Splitting your dataset into training, validation, and test sets ensures your model learns from one part of the data while being evaluated on another.
- Feature Engineering: Crafting and refining input features can greatly enhance model performance. This stage is akin to polishing a rough diamond.
- Model Training: This involves running the selected algorithms on the training dataset. Monitor the outcome and adjust hyperparameters to optimize the model.
Testing and Validating the Model
Testing and validation are essential components that ensure your model is robust and trustworthy. Once your model's training is done, itās time for some serious examination. Think of it like going for a checkup after a workout; you want to ensure that everything is functioning well.
- Metrics Evaluation: Choosing the right metrics for assessment is pivotal. You wouldn't measure the success of a horse by how quickly it flies. Common metrics include accuracy, precision, recall, and F1 score, all of which serve as yardsticks for performance evaluation.
- Cross-Validation: This technique ensures that the model's performance is consistent across various subsets of data. Itās as if youāre running multiple tests to confirm the results aren't just a fluke.
- Overfitting and Underfitting Checks: These are classic issues in model performance. An overfit model may describe the training data well but fail miserably on new data, while an underfit model may have missed the curves altogether. Carefully tuning it to find a balance is essential.
A well-tested model can mean the difference between insights that illuminate and those that mislead.
Through a systematic approach to both developing and testing the model, the groundwork is laid for reliable conclusions. Each phase builds upon the previous one, culminating in a data science project that stands tall amidst empirical scrutiny.
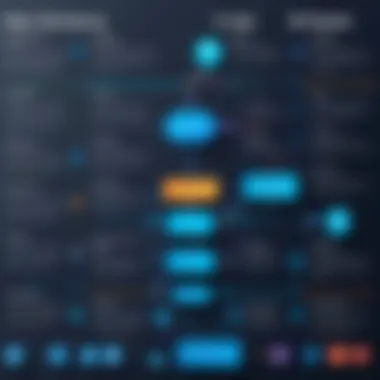
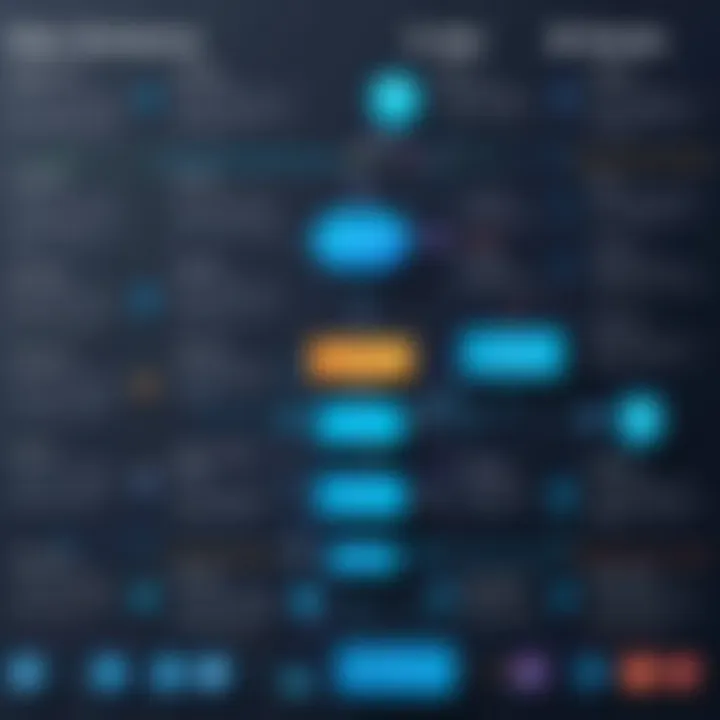
Interpreting Results and Drawing The Ends
Interpreting results and drawing conclusions is a pivotal step in any data science project. Itās where the initial labor of gathering, cleaning, and modeling data aligns with actionable insights. This stage transforms raw numbers and graphs into narratives that can guide decision-making. Itās like piecing together a puzzle; until every piece fits, the big picture remains unclear. Moreover, making sense of data allows us to validate our hypotheses or even challenge our initial assumptions.
Visualizing Results Effectively
Effectively visualizing results is about presenting your findings in a way that is coherent and insightful. Think of a well-crafted graph as storytelling for your data. Instead of drowning in a sea of numbers, a thoughtfully designed visualization can highlight key trends and patterns, much like a lighthouse guiding the way through the fog.
When constructing visualizations, itās essential to consider your audience. Not everyone is data-literate, so opting for simplicity can be beneficial. Here are some tips for making your visualizations as effective as possible:
- Choose the Right Type of Chart: Bar charts for comparisons, line graphs for trends over time, and scatter plots for relationships between variables. Each has its place in your toolbox.
- Color Matters: Use colors thoughtfully to emphasize important points or differentiate data sets, but avoid overwhelming the audience with too many hues.
- Label Clearly: Donāt leave your audience guessing. Labels and legends should be straightforward and informative.
Hereās an example using Matplotlib in Python:
This simple bar chart can quickly convey the differences between categories without delving into complex explanations. The aim here is clarity - the visual speaks louder than the data alone.
Statistical Significance and Reporting
Statistical significance is the backbone for validating your results. It answers the question of whether the findings are likely due to chance or reflect true effects. In other words, it provides a measure of confidence in the conclusions drawn from your data analysis.
When you report your findings, it's imperative to include your p-values and confidence intervals to substantiate your claims. Here are some common practices you should consider:
- P-Values: A p-value below 0.05 is commonly accepted as statistically significant. It indicates that the observed results would be highly unlikely under the null hypothesis.
- Confidence Intervals: Reporting a confidence interval gives your audience a range of plausible values for the parameter youāre estimating, providing context around your point estimate.
- Be Transparent: Always disclose your methods and any potential biases or limitations in your analysis. This honesty enhances your credibility.
āIn data science, transparency is not just a best practice; it's a necessity.ā
By adhering to these guidelines, you foster trust in your findings and invite others to engage with your work. Ultimately, strong conclusions stem from rigorous analysis, ethical considerations, and clear communication, shaping how data informs decisions in various contexts.
Challenges in Data Science Projects
When embarking on data science projects, a multitude of challenges can arise, potentially throwing a wrench into even the best-laid plans. Understanding these complications, however, is crucial for anyone looking to navigate this intricate field. Identifying potential roadblocks before they appear allows for strategic action, ensuring a smoother project journey. Here, weāll examine the significance of addressing challenges in data science projects, focusing on common pitfalls and ethical considerations that can impact the effectiveness of your work.
Common Pitfalls and How to Avoid Them
Like sailing in uncharted waters, data science projects can often lead to unexpected challenges. One prevalent pitfall is working with poor-quality data. Bad data can stem from misformatted inputs or incomplete entries, skewing results and leading to incorrect conclusions. Before starting any analysis, you should assess your data rigorously. Always consider employing data validation steps early in your data collection to mitigate this.
Another frequent trap is trying to tackle too big a problem at once. Data science is as much about focus as it is about analysis. Itās easy for a beginner to feel overwhelmed and lag behind. Instead, break down your project into smaller, manageable chunks. Set clear, achievable milestones that guide you and help maintain momentum along the way.
Moreover, overfitting is a term that many encounter in the modeling phase. This occurs when a model learns the noise in the training data rather than the actual signal, making it perform poorly on new, unseen data. To counteract this, one should prioritize using validation sets and proper metrics for evaluating model performance. Regularly assess your model and adjust parameters as necessary.
To summarize some vital tips to avoid common pitfalls:
- Thoroughly assess data quality before analysis
- Break the project into manageable tasks to prevent overwhelm
- Do not ignore model validation to avoid overfitting issues
"An ounce of prevention is worth a pound of cure." - Benjamin Franklin
Ethical Considerations in Data Science
Engaging in data science isn't just about numbers; it's a realm where ethical considerations reign supreme. The power of data can significantly influence decision-making processes, but it also comes with heavy responsibilities. Misusing data or neglecting ethical implications can tarnish your reputation and undermine the integrity of your entire project.
A major ethical challenge arises when it comes to data privacy. With the increasing amount of personal information being collected across various platforms, data scientists should prioritize user privacy above all. This includes not only ensuring compliance with laws like the General Data Protection Regulation (GDPR) but also being proactive in gaining consent for using personal data.
Bias is another ethical concern that deserves attention. Data can reflect biases inherent in society, and if not carefully managed, your models could inadvertently perpetuate these biases, leading to unfair outcomes. Therefore, itās important to actively seek diverse datasets and continually analyze model results for any biases that may emerge.
Here are some vital considerations when dealing with ethical dilemmas in data science:
- Always prioritize user privacy and comply with legal regulations.
- Regularly assess for bias in your data and models to ensure fair outcomes.
- Engage in transparent communication about data usage and findings.
Presenting Your Work
Presenting your work is not just a formality in the world of data science projects; itās a vital piece of the puzzle. Itās the bridge that connects your technical expertise with your audienceās understanding. Ultimately, the way you communicate your findings can determine the impact and applicability of your work. This section dives into why presenting your work matters, the key elements you should deliver, and what considerations to keep in mind.
Creating Clear Documentation
When it comes to data science, clear documentation is your best friend. Think of documentation as the map that guides others through the intricacies of your project. Properly documented projects can foster collaboration, making it easier for colleagues to understand your logic, replicate your results, or build upon your work.
Benefits of Clear Documentation:
- Enhances Clarity: Clear explanations of your methods and findings help avoid confusion.
- Facilitates Transparency: Good documentation allows others to see the steps taken, which can lead to better scrutiny and trust in your results.
- Aids Learning: If someone is new to your project or field, thorough documentation can act as a learning aid, guiding them through concepts step by step.
- Encourages Collaboration: When others can easily understand your work, they are more inclined to contribute.
To create effective documentation, consider the following elements:
- Overview and Objectives: Start with a brief description of the project and what you aimed to accomplish.
- Methodology: Clearly detail the methods used and any libraries or frameworks leveraged.
- Results: Present your findings in a structured format. Graphs, tables, and visuals are particularly helpful here.
- Conclusion and Future Work: Wrap it up with key takeaways and any potential next steps or future projects that could expand on your work.
Best Practices for Presentations
When the time comes to present your findings, the way you deliver information is just as crucial as the content itself. A well-structured presentation can engage your audience, making even complex data resonate. Here are some best practices to adopt:
- Know Your Audience: Tailor your presentation to fit your audience's background. Avoid jargon if presenting to a non-technical crowd.
- Structure Matters: Start with an outline of what youāll discuss. This helps set expectations for your audience.
- Use Visuals Wisely: Whether itās charts, graphs, or images, visuals can make data more digestible. Stick to key points to avoid overwhelming your audience.
- Engage with Questions: Ask questions throughout or encourage audience members to ask theirs. This fosters dialogue and makes your presentation interactive.
- Practice, Practice, Practice: Rehearse your presentation multiple times to ensure smooth delivery. Familiarity with your material can reduce nerves significantly.
Future Trends in Data Science
Understanding the future trends in data science is paramount for anyone engaged in the field. As technology evolves, the landscape of data science continuously transforms, presenting both opportunities and challenges. These trends not only influence how data science projects are approached but also inform which skills and tools will be relevant in the coming years. With organizations increasingly relying on data for decision-making, keeping abreast of these developments ensures that practitioners remain competitive and innovative.
Emerging Technologies and Their Impact
The rise of artificial intelligence (AI) and machine learning (ML) is transforming data science in profound ways. These technologies enhance the ability to make accurate predictions and automate processes. For instance, AI enables predictive analytics, which businesses use for customer behavior forecasting. A significant portion of data professionals now focus on integrating ML algorithms into their projects, thereby enhancing efficiency.
Moreover, cloud computing has revolutionized how data is stored and accessed. Instead of relying on traditional databases, many data scientists leverage platforms like Amazon Web Services or Google Cloud Platform. This shift not only allows for better scalability but also provides access to virtually unlimited computational resources, making it cheaper and faster to conduct data analysis.
As data privacy concerns heighten, emerging tools focused on privacy-preserving data analysis are gaining traction. Techniques such as federated learning allow multiple parties to collaborate on machine learning without sharing their data, maintaining confidentiality. This development is essential for aligning data practices with ethical standards, enabling organizations to harness insights without compromising user privacy.
"The future belongs to those who understand data, but also respect the privacy and ethics behind data usage." - Anonymous
The Evolving Role of Data Scientists
The role of data scientists is shifting, adapting to the increasing complexity of data and technology. No longer just analysts, data scientists are becoming conduits between raw data and actionable insights. They increasingly need to possess interdisciplinary skill sets, blending knowledge in domain-specific areas with technical prowess in data manipulation and analysis.
One key trend is the integration of data storytelling into data science projects. Communicating findings in an understandable and engaging manner is as crucial as the analysis itself. With stakeholders increasingly requiring clarity and actionable insights, data scientists must create narratives around their data to facilitate informed decision-making.
Moreover, the emphasis on collaboration and teamwork is rising. Data scientists are now often part of cross-functional teams working with business analysts, engineers, and product managers. This integrated approach ensures that data-driven insights are aligned with business goals, enhancing overall project effectiveness.
New methodologies such as Agile and DevOps are also influencing the work of data scientists. Emphasizing iterative development and continuous feedback helps adapt projects swiftly, responding dynamically to changing data landscapes or business needs.
In summary, keeping an eye on these emerging trends is not merely advantageous but necessary. The future of data science holds exciting possibilities, and for those prepared to adapt and learn, it can be immensely rewarding.