Reading CSV Files in Android Apps: A Comprehensive Guide
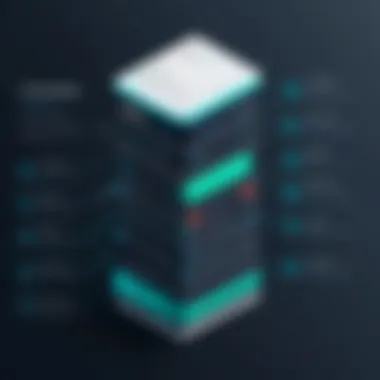
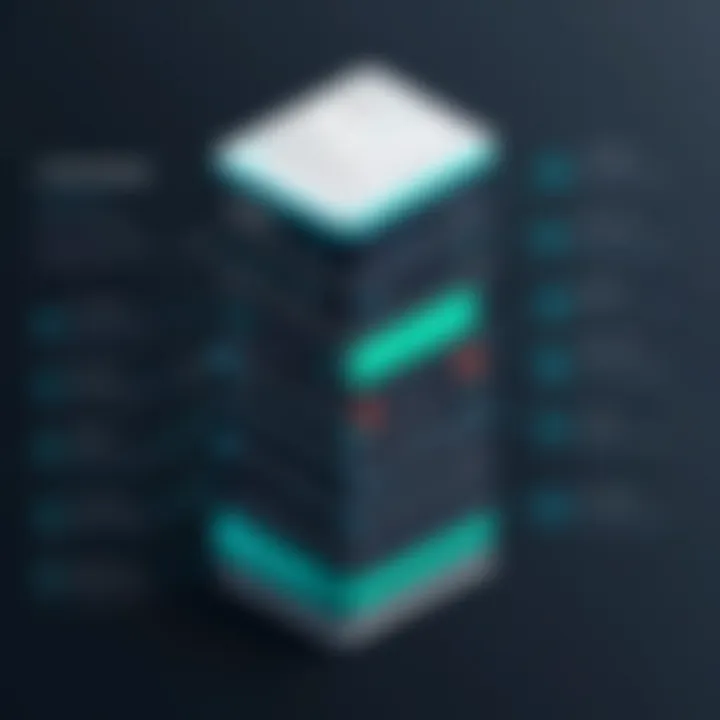
Intro
In the realm of Android development, managing data effectively is paramount. CSV files, or Comma Separated Values, have established themselves as a go-to format for data storage, particularly when it comes to exporting and importing significant datasets. This text will cover the intricate aspects of incorporating CSV file readers into Android applications, unraveling the libraries and methodologies that facilitate this process.
Understanding how to read CSV files not only enhances data management but also ensures that Android apps function seamlessly in handling various data inputs. In this article, we will explore the importance of CSV files, delve into the libraries available for CSV file handling in Android, and provide practical coding examples that illustrate best practices in real-world applications.
The Importance of CSV Files
CSV files are lightweight and easy to manipulate, making them ideal for transferring data between different platforms or applications. They serve as a bridge, enabling developers to work with structured data without getting bogged down by heavy frameworks. The simplicity of a CSV format means that it can be easily generated and interpreted by users and other programming languages alike.
"CSV files are the Swiss Army knife of data interchange, flexible and versatile, always ready to be employed in a pinch."
Overview of Libraries for CSV Reading
When it comes to reading CSV files in Android, several libraries are available that offer enhanced functionality. These libraries simplify the parsing of CSV files, eliminating the need for developers to code everything from scratch. Notably:
- Apache Commons CSV: A popular choice, it provides a robust API for reading and writing CSV files, enabling users to handle custom delimiters and complex datasets with ease.
- OpenCSV: This lightweight library offers flexibility and ease of use, allowing developers to take advantage of features like mapping CSV columns to Java objects.
- Super CSV: Particularly potent in handling complex CSV parsing, it is well-suited for applications requiring more sophisticated data handling capabilities.
These libraries do not just save time; they also help in maintaining code clarity and readability.
Best Practices for Implementing CSV Readers
When integrating CSV file readers in Android applications, adhering to best practices can significantly smooth out the development journey. Here are some guiding principles:
- Data Validation: Always verify the data being processed to avoid unexpected crashes or errors.
- Thread Management: CSV reading can be resource-intensive, especially with large files. Offload operations to background threads to keep the UI thread responsive.
- Error Handling: Implement robust error handling strategies. Anticipate issues arising from malformed CSV files or unexpected data types.
In the sections that follow, we’ll dive into some hands-on coding examples and resources that will further illuminate the process of reading CSV files in Android.
Prolusion to CSV File Handling in Android
When diving into the realm of Android app development, the ability to manipulate data efficiently is essential. Among various data formats, CSV, or Comma Separated Values, emerges as a simple yet powerful tool. It allows developers to handle data in a widely recognized text format, making data integration and exchange straightforward. Understanding how to read and process CSV files effectively in Android is a critical skill that can enhance an app's functionality, making it more user-friendly and efficient.
CSV files boast a plain text format that can be easily generated and read by humans, enabling seamless interaction between different systems. For mobile developers, this means they can import and export data without the need for excessive resource consumption. In this context, CSV handling becomes an intrinsic part of building robust and flexible applications, ensuring data can flow freely between the app and external datasets.
Understanding CSV File Format
The CSV format is identifiable by its simplicity. Each line in a CSV file corresponds to a record, and each record consists of fields separated by commas. This structure might sound too basic, yet it permits extraordinary versatility. Here’s a glimpse into how this structure looks:
Programming languages, especially Java, can easily read these files without necessitating complex parsing logic. The straightforward nature of CSV allows for quick modifications, whether it's adding a new field or updating records. Its popularity is amplified by compatibility with numerous data analysis tools, databases, and software applications, providing an advantage for mobile developers wishing to scale up their data capabilities.
Moreover, while CSV files are easy to manage, understanding their quirks is crucial. Fields may contain commas which complicate the straightforward parsing process, requiring careful handling or encapsulation in quotes. Therefore, grasping these nuances can prevent common pitfalls and streamline the integration process in an Android environment.
Importance of CSV Files in Mobile Development
In the ever-growing field of mobile app development, CSV files perform various roles that contribute to enhanced user experiences. Whether it’s importing user data from external sources, enabling data export features, or facilitating analytics reporting, CSV handling is often right at the core of these functionalities. Here's why they matter:
- Interoperability: CSV is universally acknowledged, allowing easy transfer and recognition of data across different platforms.
- Simplicity: Using CSV files reduces the complexity when working with data, since it requires minimal setup and parsing logic.
- Performance: Compared to other file formats like JSON or XML, CSV files are lightweight, leading to faster read and write operations.
"CSV files are like a Swiss Army knife for mobile developers; they are incredibly versatile and applicable in numerous scenarios."
Given these benefits, incorporating CSV file handling into Android applications becomes not just a convenience, but a necessity. Whether you are managing user-generated content, storing app settings, or providing reports, mastering the nuances of CSV in your projects enhances the overall capability and appeal of your application.
Enabling your Android app to handle CSV files efficiently will not only improve functionality but also provide enhanced user experience. As we proceed further, we will delve into tools, libraries, and practices that will simplify CSV reading processes, equipping you with the right techniques to optimize the integration of CSV file readers into your Android applications.
Setting Up Your Android Environment for CSV Reading
Setting the stage for effective CSV file handling begins with the right environment. It is paramount for Android developers to establish a solid groundwork before diving into coding. This setup not only ensures compatibility and efficiency, but it also paves the way for a smoother development process. Without proper preparation, developers might find themselves in a tangled web of mismatched libraries, inconsistencies, and unforeseen errors. It's like trying to build a house on shaky foundations - a recipe for disaster.
Required Libraries and Dependencies
When it comes to reading CSV files on Android, the right libraries can make all the difference. Several libraries have been developed to simplify the reading and parsing of CSV files, enabling developers to focus more on functionality rather than nitty-gritty details. Here are a few libraries that every developer should consider:
- OpenCSV: Known for its simplicity, OpenCSV allows easy handling of CSV files and supports various features like custom delimiters.
- Apache Commons CSV: This library provides a robust API for handling CSV data, making it a popular choice among professionals.
- SimpleCSV: A lightweight option that focuses on simplicity without skimping on functionality.
Installation of these libraries can usually be done via Gradle. For example, to include OpenCSV, you would add the following line to your file:
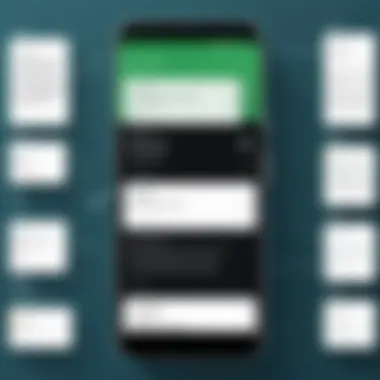
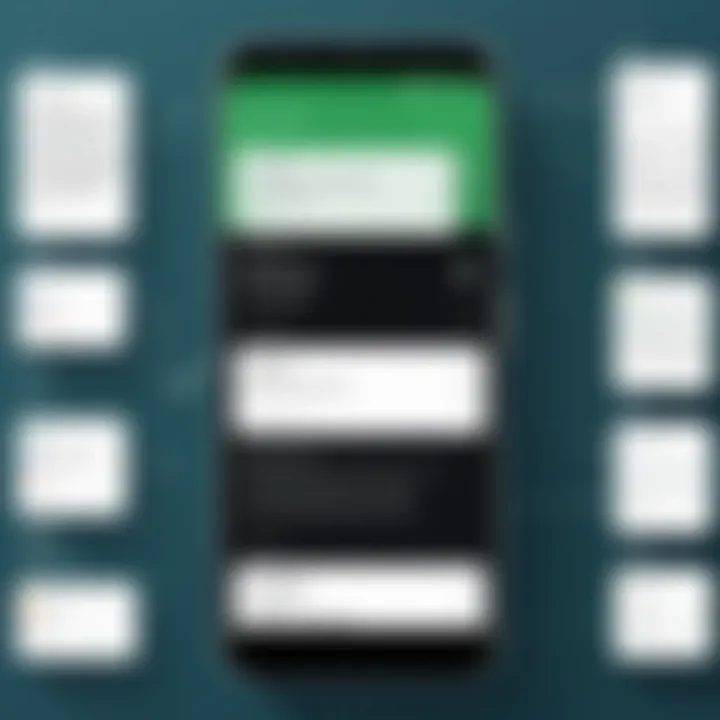
This simple act of including the right library can save hours of headaches later on!
Creating a New Android Project
With the necessary libraries in tow, the next step is to create a new Android project. This serves as the blank canvas where you’ll be painting the masterpiece of your CSV reader. Creating the project correctly lays the foundation for all subsequent development.
Follow these steps to start your new project:
- Open Android Studio: This is your primary tool for Android development.
- Start a New Project: Choose "New Project" and select a template that suits your needs.
- Configure Your Project: Name your project, choose a package name, and select a location. Make sure to choose the appropriate API level depending on your target audience.
- Add Dependencies: As mentioned earlier, don’t forget to add your chosen CSV library dependencies.
- Set Up Basic Structure: Create folders as necessary, including assets or external storage directories based on your CSV handling strategy.
By taking the time to properly set up your Android project, you create an organized space for coding and testing. Organization is key here; think of it as keeping a tidy toolbox while building something intricate. With that done, you're ready to move on to the exciting part of implementing your CSV reader!
Popular Libraries for CSV File Reading
When working with CSV files in Android applications, it’s crucial to leverage libraries that maximize both efficiency and usability. Libraries streamline the process, making complex tasks more manageable. They help developers focus on building features rather than reinventing the wheel. The right library can handle everything from basic parsing to more complicated scenarios with ease. These libraries not only save time but also ensure that your application performs well, particularly when processing large data sets.
OpenCSV: Features and Implementation
OpenCSV is often a go-to choice for Android developers due to its simplicity and flexibility. This library is designed to read and write CSV files seamlessly. One of its standout features is its ability to handle different delimiters and quote characters, which makes it adept at tackling a variety of CSV formats.
Here's a basic outline of how to use OpenCSV:
- Add Dependency: Incorporate the library into your project's file:
- Read CSV: Utilize the class to read from a CSV file. Here’s a basic example of how to do this:
- Process Data: Once you've read the data, you can directly manipulate the resulting List of String arrays, making it easy to integrate with the rest of your application.
Overall, OpenCSV’s straightforward API and comprehensive documentation make it a solid option for both beginners and seasoned developers.
Apache Commons CSV: Overview and Use Cases
Apache Commons CSV is another powerful tool worth noting. This library is part of the Apache Commons project, which is known for high-quality open-source software. With its dynamic capabilities, Commons CSV makes handling CSV files feel less like a chore.
Key features include:
- Multi-format Support: It supports various CSV formats, including custom configurations for delimiters and quoting.
- Streaming Support: Ideal for large files, its streaming approach minimizes memory footprint and aids performance.
- Extensive Error Handling: This library provides robust mechanisms for dealing with improper formatting, ensuring that your application can gracefully manage unexpected input.
An example usage would look similar to this:
The rich feature set makes Apache Commons CSV an excellent choice for projects where reliability and flexibility are paramount.
Other Notable Libraries
While OpenCSV and Apache Commons CSV are popular choices, various other libraries can serve specific needs:
- SuperCsv: Offers advanced capabilities such as custom formatting and cell processors, catering to complex data structures.
- FlatCSV: Focused on ease of use, it simplifies CSV management with an easy-to-understand API.
- uniVocity-parsers: A high-performance library for reading various types of delimited files. It stands out for its focus on scalability and speed, especially with large datasets.
Choosing the right library ultimately depends on the specific requirements of your Android application and the complexities you anticipate.
The right library can improve not only the development speed but app performance, ensuring your application runs smoothly.
Implementing a CSV File Reader in Android
In the realm of mobile development, implementing a CSV file reader is not merely a technical requirement but also an opportunity to enhance the user experience within an app. CSV, or Comma-Separated Values, serves as a bridge, allowing developers to read and write structured data seamlessly. When done correctly, this functionality can transform an application, enabling it to manage data effectively, whether it's from an asset, external file, or other data sources.
Reading CSV from Assets Folder
Reading a CSV file from the assets folder is often the first step many developers take. This method is particularly useful for loading pre-defined datasets that come packaged with the app. To access these files, you need to understand the structure of the assets folder in your Android project. This folder is specifically designed for storing raw resource files, making it a go-to location for CSV files.
To read a CSV file from the assets folder, you typically use the class. Here's a straightforward approach that elucidates this process:
By employing this code, developers can easily access and parse the contents of a CSV file bundled in the app. The placement of these files in the assets folder ensures that they are easily retrievable during runtime while keeping the main application code clean and succinct.
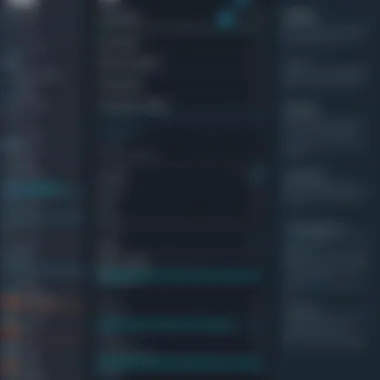
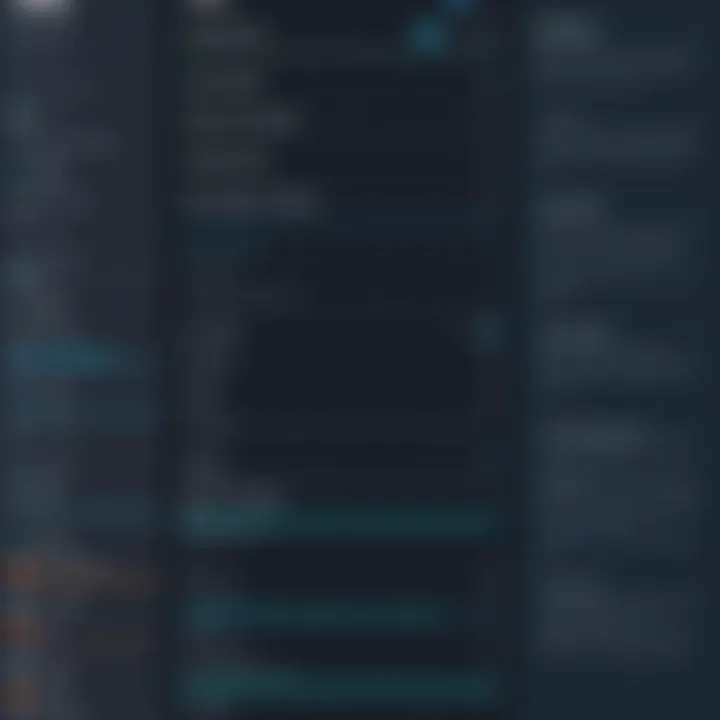
It’s important to note that when reading files from assets, you should handle potential exceptions. If the file cannot be found or is unreadable, it’s prudent to provide a graceful fallback. This ensures a smoother experience for end-users. After all, bogging down a user with errors is the exact opposite of what an app seeks to achieve.
Reading CSV from External Storage
When you need flexibility in handling larger datasets or dynamic data that changes frequently, reading CSV files from external storage becomes paramount. External storage allows users to upload or modify data files, making your app a powerful tool for processes like data analysis or logging user-generated data.
To facilitate this, you'll usually request permission to access external storage, particularly important in today’s data privacy landscape. Once you’ve secured those permissions, reading from external storage can follow a similar pattern:
This code snippet demonstrates how easily you can switch gears from accessing static files in assets to working with more customizable files on external storage.
One vital point to keep in mind is the impact on user experience. Accessing files from external storage might carry latency; thus, consider implementing practices such as asynchronous file reading or background services.
Ultimately, the ability to read CSV files from both the assets folder and external storage rounds out a comprehensive approach to data management in Android applications. Each method offers unique advantages, and the choices made will largely depend on the specific requirements of the app, making CSV file readers a foundational component of effective Android development.
Error Handling and Data Validation
Error handling and data validation are critical components in the development of robust Android applications, especially when dealing with CSV file inputs. As developers work with data, ensuring the integrity and correctness of that data becomes paramount. Mismanagement can lead to unexpected behavior within an app or worse, crashes, affecting user experience significantly.
Handling errors gracefully ensures that the application can continue functioning or at least inform the user of what went wrong. For instance, if a CSV file is malformed - whether due to extra commas, wrong delimiters or any other aberration - it could throw an exception that halts the app’s operation. Implementing proper error handling strategies helps developers ascertain where the issue lies and what possible steps can be taken to mitigate it.
Furthermore, validating CSV data before processing is equally significant. Not only does this create a reliable foundation for the application's functionalities, but it also prevents data-related issues down the line, such as integrating invalid records into the database or generating incorrect outputs for users.
Common Errors While Reading CSV Files
When reading CSV files, several common errors can crop up that developers must be aware of:
- Malformed CSV Files: The structure of a CSV file might not conform to the expected format, causing parsing issues. Missing headers or improper delimiters can lead to misaligned data.
- Encoding Problems: Some files may contain special characters requiring a specific text encoding, which, if not correctly specified during reading, can result in garbled text.
- Missing Data: Empty cells in rows can create discrepancies in how data is handled, especially if the application expects a specific number of columns.
- File Not Found: Often, developers might mistakenly reference the wrong file path, leading to the inability of the application to access the desired data.
Visualizing these challenges can remind developers to approach CSV handling with caution. Speaking of which, a quick byte of wisdom is to always log errors effectively so that you can trace back what went wrong - that’s a life-saver when debugging.
Validating CSV Data
Data validation goes hand in hand with error handling; it’s more about ensuring the data meets certain standards before operations are carried out. Here are key points to consider for effective validation:
- Schema Validation: Define a clear schema for what a valid CSV should look like. This includes the expected number of columns, data types (such as integer, string), and valid formats (like dates). It can prevent unwanted or malicious data from being processed.
- Type Checking: Ensure that the data matches the expected types. For example, if a column is supposed to contain numerical data, verify that all entries can be parsed into numbers. Failing this can throw runtime exceptions that mar user experience.
- Value Range Checking: For certain columns, such as ages or scores, thorough checks on the allowed ranges can help catch illogical inputs early.
- Duplicate Entry Checks: Sometimes you might not want duplicates in your dataset. Implement validation checks that confirm data uniqueness when necessary.
- Special Characters: Be wary of unexpected characters that don't align with the expected values. Implement logic that highlights or excludes these problematic entries.
Ultimately, the exhaustive validation of CSV data fosters a healthier, more reliable application environment. The rigorous combination of error handling and data validation empowers developers to build applications that stand tall against common pitfalls, ensuring that users enjoy a seamless experience while interacting with their software.
Performance Considerations for CSV File Reading
In any Android application, reading files efficiently can make or break user experience. With CSV files being a common format for data interchange, understanding performance considerations is vital. This section delves into strategies that help improve CSV file reading processes to create smooth, responsive applications. By optimizing these operations, you not only enhance performance but also ensure that resources are used wisely, leading to better memory management and app stability.
Optimizing File Reading Operations
When it comes to reading CSV files, there are several optimizations that can be implemented. The aim here is to ensure minimal overhead during file reading.
- Use Buffered Reading: Instead of reading data line-by-line, which can be taxing, utilize buffered readers. BufferedInputStream can drastically reduce the number of I/O operations by reading larger chunks of data at once. This speeds up file access significantly.
- Asynchronous Operations: Blocking the main thread can cause a poor user experience by making the app unresponsive. Utilize asynchronous tasks, such as AsyncTask or Kotlin coroutines, for reading CSV files. This way, your app remains responsive while background operations proceed seamlessly.
- Limit Data Processing: Often, a CSV file contains more data than you need for immediate use. Implement filtering mechanisms to read only necessary rows or columns. By avoiding the processing of superfluous data, you can reduce memory consumption and processing time.
- Batch Processing: If dealing with extensive datasets, try to process records in batches. This approach minimizes the overhead like memory allocation and helps keep the application fluid.
- Direct File Access via Path: If the file is stored as internal or external storage, ensure that you access it directly by its path instead of working through layers of file management APIs. This can save time and system resources.
Memory Management Strategies
Managing memory efficiently is crucial for any Android application, particularly when dealing with large CSV files that can put a strain on the system.
- Utilize Streams Instead of Arrays: In Java, using streams allows you to process data without loading entire datasets into memory at once. This can prevent memory overflow even with large files. For instance, using can keep memory usage in check.
- Implement Weak References: If your application requires keeping data in memory, consider using WeakReference for certain data structures. This allows the garbage collector to reclaim memory if it is running low, improving overall stability.
- Clean Up Resources: Always close file readers or streams when they are no longer needed. Failing to do so might lead to memory leaks and other issues. Using try-with-resources statements can help automate resource management.
- Monitor Memory Usage: Tools provided by Android, like Android Profiler, can help developers track memory allocation and investigate leaks. Monitoring your app’s memory usage during CSV file reading will help you pinpoint potential bottlenecks and optimize accordingly.
Effective performance optimization is like fine-tuning an instrument; every adjustment counts toward that beautiful harmony.
By fine-tuning your approach to reading CSV files, you ensure that the user experience is not just good, but great. Addressing file reading performance and memory management strategies will strengthen your application's foundation, offering both you and your users a seamless experience.
Advanced CSV Handling Techniques
In the realm of Android development, simply reading and writing CSV files may not cover every use case. Therefore, understanding advanced CSV handling techniques can be a game changer. These techniques allow developers to manipulate complex data structures, ensuring that your app remains efficient and user-friendly. Let’s delve into the nitty-gritty of two specific advanced techniques: parsing complex CSV structures and integrating CSV data with other formats.
Parsing Complex CSV Structures
Parsing complex CSV structures can feel like deciphering a puzzle. In reality, many CSV files encountered in real-world applications contain intricacies like nested quotes, irregular delimiters, or multiline fields. Instead of just treating each line as a separate entity, adopting a robust parser ensures that you accurately capture the intended data.
Consider a situation where your CSV data format includes quotations around fields that could contain line breaks. For instance:
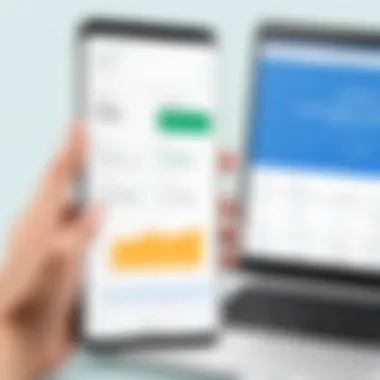
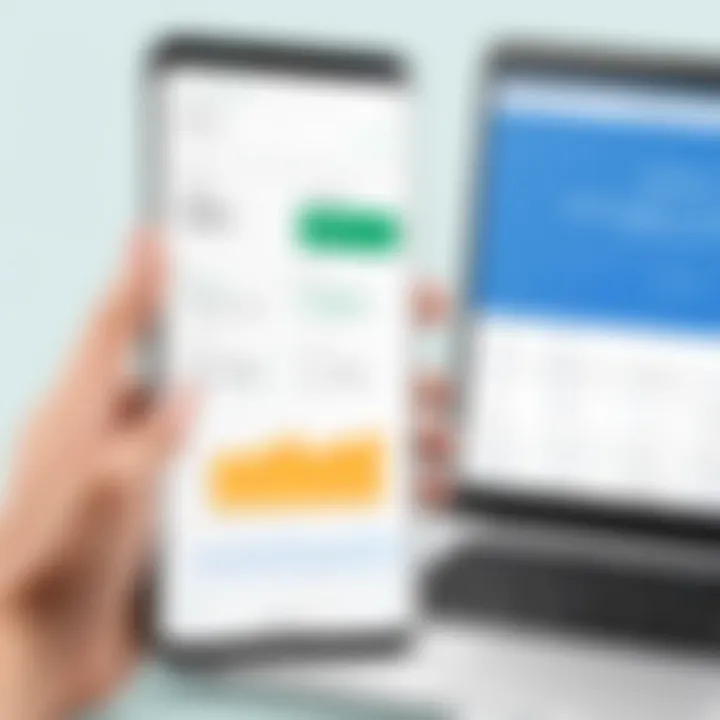
"Name, Age, Occupation
"John Doe", 30, "Software Developer, Part-time"
"Jane Smith", 25, "Data Analyst"
In this context, a naïve CSV reader could trip over the line breaks as if they denote a new record, thus leading to corrupted data output. Handling such complexities requires a parser that can intelligently recognize which characters belong to text fields and which denote structure. Tools like OpenCSV provide built-in mechanisms to address these scenarios, allowing you to specify delimiters and quote characters.
Employing such techniques not only enhances reliability but also elevates user trust in your application, as you present data accurately and clearly without unintended hiccups. Here are key considerations when parsing complex CSV files:
- Identify your delimiter: Always clarify what separates your fields. The default may be a comma, but you may encounter semicolons or tabs used for this purpose.
- Account for nested quotes: Recognize that CSV can contain string literals that include delimiters and need to be handled accordingly.
- Test rigorously: Before rolling out your app, test against diverse CSV inputs to ensure that it can handle edge cases effectively.
Integrating CSV Data with Other Formats
CSV files are versatile, but they aren’t the only data players in town. Often, applications require merging or converting CSV data with other formats like JSON or XML. This process can facilitate better data interchange, giving your app the ability to handle diverse datasets adequately.
When you integrate CSV data with other formats, consider the following key points:
- Data Structure: Understand that CSV is flat, while other formats can be hierarchical. You may want to transform CSV records into nested JSON objects.
- Library Support: Utilize libraries that support multiple formats. For instance, libraries like Jackson for JSON work seamlessly with CSV as well, providing methods to convert between formats effortlessly.
- Performance: Watch out for potential performance impacts. The larger the datasets and the more complex the transformations, the more crucial it is to optimize processing time.
Here's a simple illustration of using Jackson for integrating CSV with JSON:
This code snippet demonstrates the synergy between CSV and JSON, allowing you to manipulate and present data in formats that meet user expectations.
In summary, advanced CSV handling techniques encompass not only handling complexity within CSV files but also integrating them with other formats to give your application a more robust data management capacity. Understanding these aspects is indispensable for developers seeking to build sophisticated, cross-functional Android applications.
Testing and Debugging CSV Readers
When it comes to integrating CSV readers into Android applications, testing and debugging are absolutely crucial. Think of it like building a sturdy bridge; it has to be solid enough to hold the weight of data without crumbling under pressure. A well-tested CSV reader not only enhances the user experience but also ensures the integrity and reliability of the data being processed.
Unit Testing Approaches
Unit testing is all about breaking down your CSV reader into its smallest parts and testing each piece thoroughly. In this context, each function or method in your CSV reading logic should be treated as an independent unit, validated for expected outputs against varying inputs.
Here’s how you can go about implementing unit tests for your CSV reader:
- Use Testing Frameworks: Frameworks like JUnit or Mockito can be employed to create robust tests. JUnit works well for running your test cases, while Mockito can help to mock the behavior of external dependencies, such as file I/O.
- Create Test Cases: Develop a range of test cases that cover typical scenarios, including:
- Assertions: Check outcomes with assertions to ensure the expected results match. For instance, if a CSV is supposed to yield five rows of data, assert that the output received is indeed five rows. If it's not, you need to investigate further.
- Test Coverage: Aim for a high test coverage percentage, aiming to include at least 85% coverage across your code. This means testing all paths of execution, ensuring that your methods can handle all the use cases.
- Successfully reading a well-formed CSV
- Handling of malformed CSV files
- Boundary cases, such as empty files or extreme data sizes
By rigorously testing your CSV reader functions, you'll be better equipped to detect issues early, making the final application more reliable.
Common Debugging Techniques
Even with pre-emptive testing, issues can still arise during runtime. Debugging is where the rubber meets the road. In the realm of debugging CSV readers, there are several techniques that can be utilized to identify and rectify issues fast:
- Log Statements: One of the simplest but most effective debugging techniques is to pepper your code with log statements. These can help you track the flow of data and quickly pinpoint where things might be going wrong. Using libraries like Log4j can immensely assist in this.
- Breakpoints: Utilizing breakpoints through your IDE allows you to pause execution at critical points, examining variables and the state of your application. Tools like Android Studio provide an intuitive interface for setting breakpoints and stepping through code line by line.
- Error Handling: Good error handling is key to effective debugging. Instead of your application crashing unceremoniously, graceful error handling allows you to catch exceptions and log them in a structured manner, providing insights into the problem. You might want to integrate error logging using libraries like Sentry or Firebase Crashlytics for detailed insights.
- Test with Various Inputs: Sometimes your CSV files come from unexpected sources or have peculiarities. Tests should not only be about expected inputs but also about extreme cases. Feeding your reader unusual inputs can help elucidate how it behaves during anomalies.
- Peer Reviews: Sometimes, just a fresh pair of eyes can identify problems that you've been overlooking. Conducting peer code reviews can lead to improved code quality and can surface hidden bugs.
"Debugging is like being the detective in a crime movie where you are also the murderer."
By combining thorough unit tests with efficient debugging practices, you can create a robust mechanism for reading CSV files in your Android application. The beauty lies in creating a system that not just works, but works reliably under varied conditions.
End and Future Outlook
In wrapping up our exploration of CSV file readers within Android applications, it’s essential to reflect on the significant takeaways from this study. CSV files have established themselves as a fundamental format in data storage and transport, but understanding how to handle them efficiently in an Android environment can substantially enhance app functionality.
Having reviewed the popular libraries, implementation strategies, and debugging methodologies, developers are well-equipped to incorporate CSV reading capabilities into their projects. But it doesn’t stop there; the landscape of data management is in constant flux.
CSV files might be simple, but simplicity is often the backbone of efficiency in data processing.
It’s crucial to stay updated with advances in both the Android platform and the libraries available. Future iterations of these libraries might introduce enhanced features enabling easier manipulation or improved handling of large datasets. As mobile applications continue to evolve in complexity and capability, the ways in which they interact with external data formats like CSV also need to adapt.
Recap of Key Concepts
To summarize the core ideas discussed:
- CSV Files: A widely used format that allows for simple text-based data storage.
- Library Options: Tools like OpenCSV and Apache Commons CSV offer varying features suited to different development needs.
- Implementation Techniques: Strategies for reading CSVs from both internal assets and external storage.
- Error Handling: Understanding potential pitfalls and establishing robust validation processes.
- Performance and Optimization: Guidelines for ensuring seamless operations and effective memory management.
This digest underscores the backbone of practical knowledge developers need when embarking on data management endeavors in Android development.
The Future of Data Management in Android
Looking ahead, one can only speculate about the directions that data management will take within the Android ecosystem. The importance of CSV in mobile development will persist, but further integrations of more sophisticated data handling techniques will come into play.
- Integration with APIs: As applications become even more interconnected, linking CSV data with real-time databases or cloud services could become commonplace.
- Big Data Challenges: Addressing the needs of big data will prompt advancements in library optimizations, facilitating better handling of extensive datasets.
- User Experience Priority: Developers will continue to prioritize user experience, ensuring that data-rendering processes remain seamless and efficient for end-users.