Essential C# Interview Questions and Answers Guide
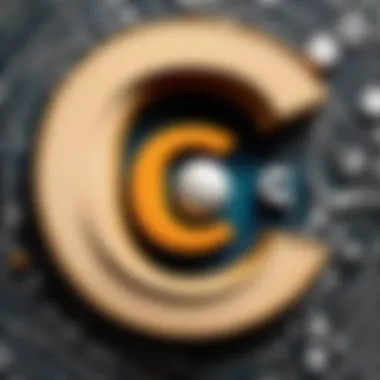
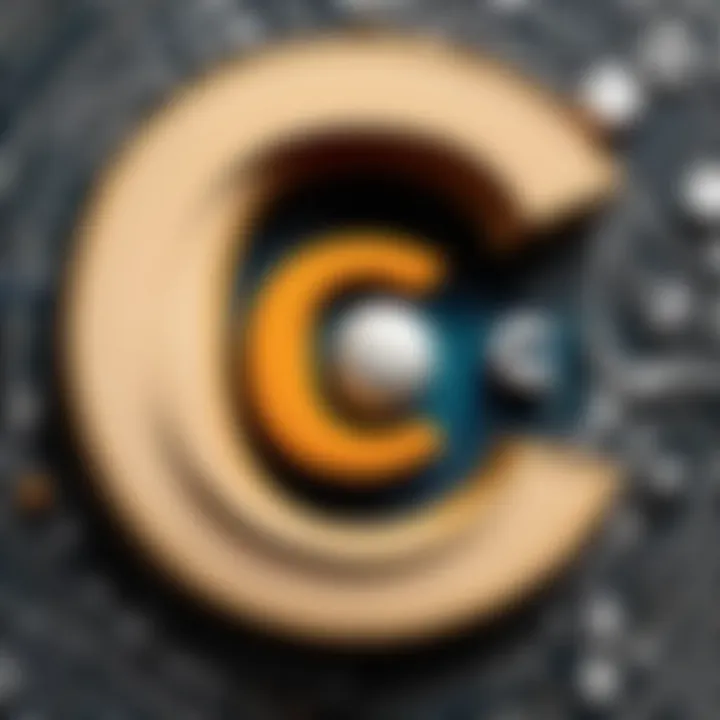
Intro
In the world of software development, mastering a programming language can feel like a daunting mountain to climb. One such language that stands out due to its versatility and robustness is C#. This article aims to guide aspiring developers through the labyrinth of C# interview questions and essential concepts, tailored for all learnersāfrom those just dipping their toes into coding to more seasoned programmers.
In preparing for a job interview centered around C#, itās crucial to have a solid grasp of both fundamental and advanced topics. C# is not only known for its user-friendly syntax but also its compatibility with different platforms, making it a strong candidate for any programmerās toolkit. This compendium of questions and answers provides a comprehensive overview that can help demystify the interviewing process.
"Preparation is the key to success."
With this thought in mind, understanding C# in its entirety can boost your confidence and position you favorably during interviews. We will traverse various sectionsācovering history and background, diving into basic syntax and concepts, exploring advanced topics, and even looking at hands-on examples. Ultimately, our goal is to equip you with the know-how and practical skills necessary to ace those interviews. Let's take the first step into the realm of C#.
Understanding
Basics
Grasping the fundamentals of C# serves as a cornerstone for mastering both the language itself and the myriad of applications it can tackle. Whether you are a budding programmer or someone looking to sharpen your existing skills, having a solid understanding of C basics lays the groundwork for more complex concepts and practical applications. This segment focuses on essential elements, benefits, and considerations surrounding this topic, providing a roadmap for learners eager to navigate C effectively.
What is #?
C# can be described as a modern, general-purpose programming language developed by Microsoft. It's primarily utilized for building diverse applications that run on the .NET framework, which introduced various powerful features. Broadly speaking, C is known for its simplicity and flexibility, making it a go-to option for developers aiming to create everything from desktop apps to web services and even mobile applications.
It's designed to be intuitive for individuals that may come from different programming backgrounds, accommodating a gentle learning curve while maintaining enough depth to engage seasoned developers. The languageās syntax is similar to other C-based languages like Java and C++, ensuring that those familiar with these languages can pick it up relatively easily. In practice, you might encounter C# being used in:
- Web and Cloud Applications: C# is behind many ASP.NET web applications and Azure cloud-based services.
- Game Development: The Unity Engine employs C# as its primary scripting language, allowing developers to create immersive gaming experiences.
- Desktop Applications: Windows Forms and WPF (Windows Presentation Foundation) leverage C# for rich enterprise applications.
The richness of C# is not just in its versatility but also how it enables developers to write clean, manageable, and robust code. Using features such as type safety, automatic garbage collection, and strong exception handling, C promotes best practices through its design.
History and Evolution of
C# made its debut in the early 2000s, giving birth to what many consider a pivotal moment in software development. Developed by Anders Hejlsberg and his team at Microsoft, C was part of a larger initiative, the .NET framework, which aimed to standardize and simplify Windows programming. The language was officially launched alongside .NET in 2002, showcasing a fusion of strong object-oriented programming principles combined with functionalities inspired by other languages. This historical context holds significance in demonstrating how C has matured over the years.
As technology evolved, so did C#. Over the years, Microsoft introduced new versions, each bringing substantial improvements, features, and refinements:
- C# 2.0: Introduced generics, anonymous methods, and nullable types, making the language more flexible and powerful.
- C# 3.0: This version included features like Language Integrated Query (LINQ), allowing developers to write more expressive and readable code when handling data queries.
- C# 5.0: Introduced async and await keywords, simplifying asynchronous programming.
The language continues to evolve with time, including innovations like pattern matching, local functions, and enhanced performance. With the advent of cross-platform development through .NET Core and now .NET 5 and beyond, C# has found a place not only in Windows environments but also in Linux and macOS ecosystems.
As you can see, understanding both "What is C#?" and its "History and Evolution" offers a broader perspective that helps in developing skills and judiciously applying them across various projects.
"C# is a language that reflects the evolution of programming paradigms, seamlessly adapting to the needs of modern developers while maintaining its core principles."
In summary, the comprehension of C# basics is vital for substantiating your programming journey. It ās not just about learning another language; itās about integrating into a swiftly changing tech landscape, ready to tackle challenges that come your way.
Core
Concepts
When wading into the pool of programming languages, C# stands as a prime contender for developers wanting to create robust applications. Core C concepts encapsulate the foundational knowledge and technical prowess needed to write effective and maintainable code. Mastering these concepts equips candidates to not only answer interview questions convincingly but also to demonstrate practical skills in real-world scenarios. This section, therefore, holds immense importance for any aspirant looking to get a foothold in software development.
Syntax and Structure
The syntax of a programming language is akin to the grammar of a spoken language. A strong grasp of C# syntax and structure is essential for clear and efficient coding. For instance, basic constructs like loops, conditionals, and method definitions shape how developers express their logic. Understanding these elements can greatly enhance code readability and maintainability.
Key Components of
Syntax:
- Variables: These are essential for storing data. Just like our conversations rely on words, variables do the heavy lifting in coding, storing values for use in various operations.
- Data Structures: Arrays, lists, and dictionaries provide the framework for organizing data.
- Control Flow Structures: If-else statements and switch cases guide the programās execution path.
Careful attention to syntax can prevent common pitfalls that often snag novice programmers. For example, forgetting to close a parenthesis or misspelling a keyword can lead to frustrating compile errors. In preparation for interviews, itās wise to practice writing snippets and debugging them to internalize the structure.
"Understanding the syntax is the first step towards becoming a proficient C# developer."
Real World Example: Consider an if-statement that checks whether a student passes based on their score. Hereās a simple code snippet:
This example showcases how C# syntax allows developers to implement logic clearly.
Data Types and Variables
At the heart of any programming language lie its data types and variables. Data types dictate the kind of data that can be stored and manipulated. In C#, understanding various data types, such as , , , and , is paramount for effective programming.
Common
Data Types:
- int: Represents integers. Perfect for counting or indexing.
- string: Used for text. Itās everything from names to messages.
- bool: A binary type, it stores true or false values, guiding logic in applications.
- float: Ideal for decimal numbers, useful in calculations that require precision.
Variables are like labeled boxes where data is stored. A programmer must know how to use and manipulate these boxes to build functionality.
Tips for Handling Data Types:
- Always declare data types clearly to avoid confusion.
- Utilize type conversion when needed to ensure compatibility among different data types in calculations.
An example of declaring variables in C# might look like this:
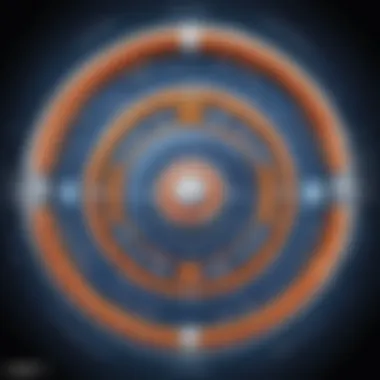
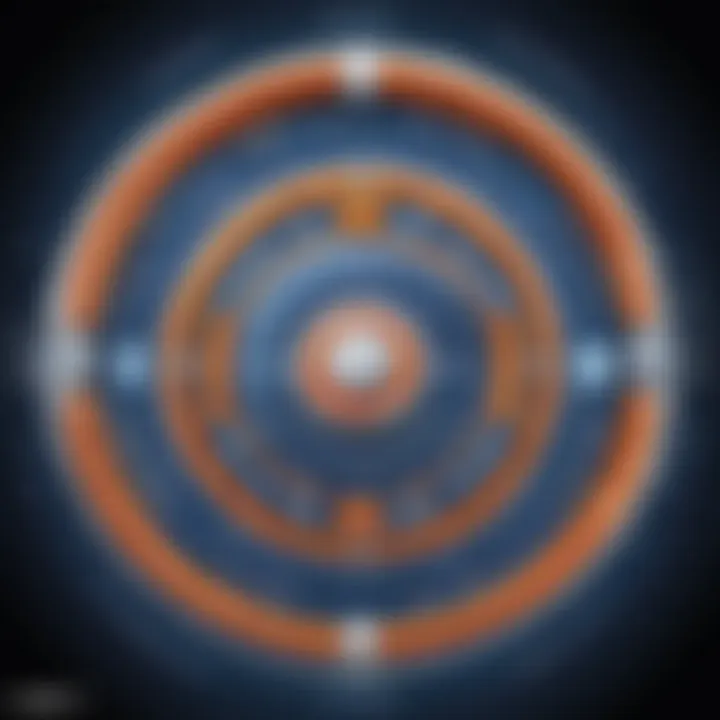
Understanding how to declare and use variables with appropriate data types not only simplifies coding but also enhances the performance of applications.
Object-Oriented Programming in
In the sphere of software development, Object-Oriented Programming (OOP) is often regarded as a cornerstone for crafting robust, scalable applications. Its significance in C# cannot be overstated; it molds the way programmers conceptualize structures and relationships within their code. Through OOP principles, developers can model real-world entities, making it easier to organize complex code and enhance reusability. This, in turn, not only streamlines the development process but also simplifies maintenance, thus reducing long-term costs.
When we dive into the realm of C#, we encounter four fundamental pillars of OOP: encapsulation, inheritance, polymorphism, and abstraction. Each of these components serves a unique purpose and together they lay the groundwork for effective program design.
- Encapsulation helps in bundling data and methods that operate on that data within a single class. By restricting access to certain parts of the object, it protects the integrity of the data.
- Inheritance allows a new class to inherit properties and behavior from an existing class. This mechanism fosters code reuse and establishes a natural hierarchy.
- Polymorphism enables methods to do different things based on the object it is acting upon, adding flexibility to the code.
- Abstraction simplifies complex reality by modeling classes based on essential properties and behaviors while hiding unnecessary details.
By leveraging these principles, C# developers can create applications that are not just functional, but also maintainable and scalable. Letās delve deeper into each of these concepts to understand their practical applications within C#.
Classes and Objects
In C#, classes form the blueprint for creating objects. They define properties and methods that represent real-world entities. An object is an instance of a class, encapsulating both state (data) and behavior (methods).
For instance, consider a class called . This class can have properties like , , and . It might also include methods such as and . To create an instance of , you can do the following:
This simple blueprint demonstrates how classes serve as a mold for creating objects with unique states. By implementing classes, programmers gain the flexibility to manage complex systems efficiently, laying the foundation for larger applications.
Inheritance and Polymorphism
Inheritance extends the virtue of classes by allowing a new class to acquire the properties and methods of an existing one. This helps reduce code duplication and fosters reuse.
Take for example a superclass called . We can derive subclasses such as , , and , which inherit the common properties of (like and ) while having their own unique attributes.
Polymorphism complements this by allowing methods to work in different ways based on the object invoking them. A method in the class can be overridden in the class to tailor its functionality. This ensures that the method called corresponds to the object type:
Here, when is called on a object, it will execute the overridden method specific to while keeping the common vehicle behavior intact. This capability to interact with objects in a uniform way while enabling diverse implementations is a hallmark of OOP in C#.
Encapsulation and Abstraction
Encapsulation not only organizes code but also serves as a safeguard for object data. By using access modifiers, developers can control the visibility of certain member variables and methods. For example, marking properties as prevents outside entities from modifying the state of the object directly. Instead, public methods can be used to facilitate controlled access and modification.
In parallel, abstraction focuses on hiding the complex realities while exposing only the necessary parts of an object. This is achieved in C# through abstract classes and interfaces. An abstract class can define a blueprint for derived classes without providing complete implementations. This ensures a sensible architecture where complex systems are effectively distilled into manageable components.
By employing both encapsulation and abstraction, programmers can create a clean interface for their code, which not only enhances readability but also minimizes bugs.
"The simpler you say it, the more eloquent it is."
interviews, understanding these principles will be invaluable in articulating the benefits of OOP and demonstrating your depth of knowledge in the language.
Advanced
Features
The realm of C# is vast, and as a language evolves, it brings forth features that elevate the way we write, understand, and execute our code. When preparing for C interviews, grasping advanced concepts not only showcases oneās technical prowess but also a solid understanding of real-world applications. Advanced features often reflect efficiency and modern programming practices. This section aims to delve into three significant components: Delegates and Events, LINQ Queries, and Async and Await. Each of these constructs can dramatically alter the fabric of C coding practices, leading to cleaner, more maintainable, and performance-oriented applications.
Delegates and Events
At its core, a delegate in C# is a type that references methods with a particular parameter list and return type. Think of it as a pointer to a method. Delegates provide a way to encapsulate a method in a way that allows the method to be called later, even if you don't know what method itāll be at runtime. This concept is crucial in event-driven programming. The beauty of delegates lies in their ability to promote loose coupling in code, allowing changes to be made with minimal impact on other components.
Events build on delegates. When you think of an event, picture it as a notification system. For instance, if an object does something interesting, it can raise an event to notify other objects that care about it. To put it into perspective:
"Events in programming are like dinner bells; they ring when it's time for others to pay attention to what's happening in the kitchen."
When you implement an event, you're generally allowing other classes to subscribe to it, creating a more responsive system. This responsiveness is hugely beneficial for user interfaces where actions (like clicks) should trigger immediate responses.
Example code highlights this usage:
Understanding delegates and events is essential in interviews as it reflects the candidate's ability to write decoupled and event-driven code, which is often desired in dynamic applications.
LINQ Queries
Language Integrated Query (LINQ) is an impressive feature that significantly simplifies data manipulation in C#. It provides a seamless way to query data from various sources like arrays, collections, databases, or even XML. Using LINQ, you can write queries in your C# code that are analogous to SQL statements, greatly enhancing readability and usability.
Imagine trying to find a needle in a haystack, where the haystack represents your large dataset. With LINQ, you effectively have a strong magnet that helps you pull out that needle, all while crafting elegant and succinct queries. The syntax is intuitive, and it embraces functional programming paradigms, making your code cleaner and more expressive. Some common operations include:
- Select: to project data.
- Where: to filter data.
- OrderBy/OrderByDescending: to sort data.
Example:
This simplicity allows developers to perform complex data queries with a fraction of the code. In the context of an interview, being adept in LINQ demonstrates a candidate's ability to handle data efficiently and effectively, which is critical in many programming environments.
Async and Await
As systems become more intricate, so too does the need for non-blocking operations. The async and await keywords in C# are game-changers in this landscape, allowing developers to write asynchronous code that is easy to read and maintain. When a method is marked as async, it's telling the compiler that it can be paused during long-running operations, letting other code execute meanwhile.
Imagine you're cooking dinner while also waiting for the washing machine to finish. Instead of staring at the machine doing nothing, you continue with your meal prep until the washing machine signals itās done. This analogy is fitting when discussing async operations. Itās about multitasking intelligently.
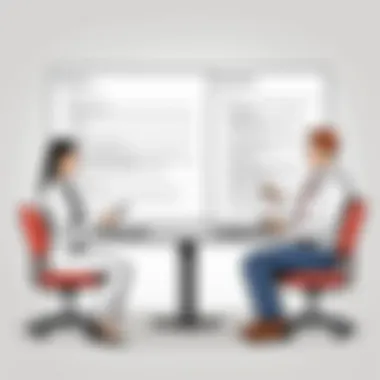
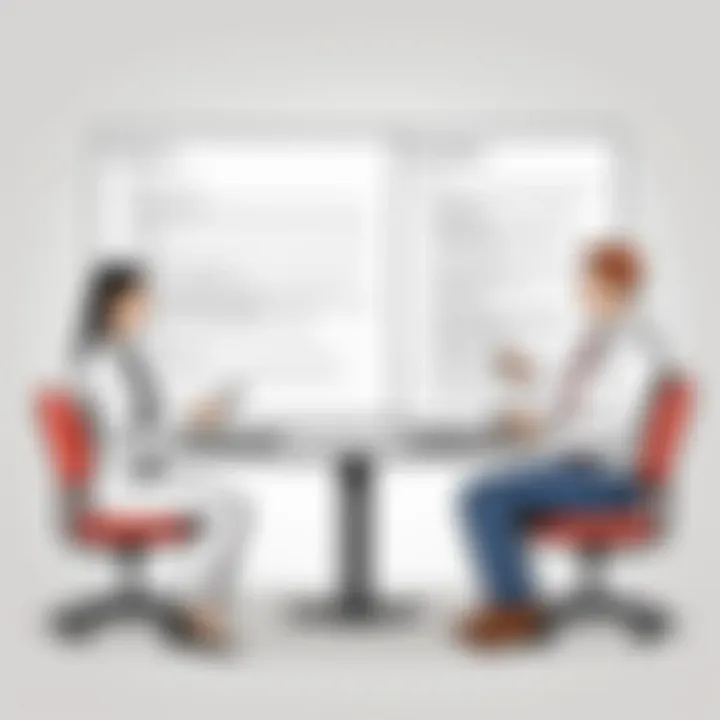
In practical terms, using async and await lets your applications remain responsive, which is paramount for user experience, particularly in UI applications. An interview might include questions on handling exceptions in async methods or best practices in structuring async code. Example code demonstrating async and await might look like this:
The rise of asynchronous programming is a testament to the evolving nature of application demands, making it essential knowledge for anyone entering the C# programming field.
Overall, mastering these advanced features of C# not only bolsters oneās technical understanding but also equips a programmer with tools to tackle modern programming challenges head-on.
Common
Interview Questions
Common C# interview questions serve as a backbone for assessing a candidateās grasp on the language of C#. These inquiries are likely to come up in various scenarios including coding interviews, technical screenings, or even final interviews with hiring managers. They help to gauge foundational knowledge alongside practical skills in using C#.
Why focus on common questions?
Understanding the most frequently asked questions can illuminate areas where candidates often find difficulties. It can also shed light on the concepts that employers prioritize. For instance, familiarity with syntax and core principles of object-oriented programming are common focal points.
Moreover, common questions often highlight industry standards and best practices, thus serving not just as a preparation tool, but also ensuring that aspirants are aligned with the expectations set forth by potential employers. Grasping these questions increases both confidence and competence during interviews.
Questions about
Syntax
C# syntax questions are pivotal during interviews because they gauge a candidateās familiarity with the structure and conventions of the language. Syntax is the framework upon which programming logic rests.
- What is the significance of a semicolon in C#?
A semicolon acts as a statement terminator. Omitting it can lead to compilation errors, which underscores the importance of precise adherence to syntax rules. - How do you define a variable in C#?
Understanding how to declare variables is fundamental. The declaration has the general form , which signifies type safety inherent in C#. - What are the different types of loops in C#?
Familiarity with loops such as , , and is beneficial since it reflects on a candidateās capacity to manage repetitive tasks without redundancies.
The effectiveness of syntax-related questions lies in their ability to demonstrate a candidateās attention to detail and ability to follow structured guidelines.
Object-Oriented Programming Questions
Object-oriented programming is a cornerstone of C#. Candidates can expect questions that explore fundamental concepts such as classes, objects, inheritance, and polymorphism. These topics often come packed with nuanced scenarios that require both explanation and practical understanding.
- What is a class?
A class is a blueprint from which individual objects are created. An interviewee should describe how classes encapsulate data and behavior, leveraging encapsulation to protect object integrity. - How is inheritance used in C#?
Inheritance allows one class to inherit properties and methods from another. Candidates might be asked to explain the benefits, such as code reusability and enhanced organization. - What is polymorphism?
Polymorphism allows methods to do different things based on the object that itās acting upon. Clarifying this can be a pivotal moment in an interview, as it shows depth of understanding in designing flexible systems.
These inquiries not only test knowledge but spur discussion on how candidates can implement these concepts in real-world scenarios.
Concurrency and Multithreading
C# is equipped with powerful systems for concurrent programming, which is why the subject comes up in interviews. Blocks that address multithreading aim to assess the candidate's grasp on executing multiple threads concurrently and the challenges that come with it.
- What is multithreading?
In simple terms, it allows multiple threads to run simultaneously for better resource utilization. Understanding thread management and synchronization mechanisms is crucial here. - How do you handle thread synchronization?
Candidates should discuss various approaches, such as using locks or semaphores to avoid race conditions. Defining terms clearly displays a commitment to thread safety practices in applications. - What are common problems with concurrency?
Identifying issues like deadlocks, race conditions, and thread starvation will reflect a candidateās capacity to foresee and mitigate risks associated with concurrent programming.
In sum, gaining a firm grasp on concurrency and multithreading encompasses foundational elements that form the basis of efficient and responsive applications in C#.
Throughout these sections, attention to fundamental principles can significantly enhance a candidate's performance in any C# interview. As with any subject of study, mastering these questions is less about rote memorization and more about building a solid conceptual framework to tackle practical challenges.
Hands-on Coding Challenges
Hands-on coding challenges serve as a practical cornerstone in the journey of mastering C#. They bridge the gap between theoretical understanding and practical application, making them indispensable for aspiring programmers. In the realm of C# interviews, these challenges are often focal points as they not only test coding proficiency but also evaluate problem-solving skills and the ability to think algorithmically.
By engaging in coding challenges, candidates develop a deeper comprehension of various data structures, algorithms, and C# syntax. Furthermore, these exercises reinforce learning by compelling individuals to apply concepts actively rather than passively absorbing information. Some notable benefits include:
- Skill Enhancement: Tackling diverse problems sharpens the problem-solving toolkit, honing critical thinking and analytical skills.
- Confidence Building: Successfully solving coding challenges fosters a sense of accomplishment, preparing candidates for the pressure of live coding during interviews.
- Exposure to Real-World Problems: Many coding challenges mirror scenarios encountered in actual development environments, enabling students to gain insight into industry standards and expectations.
Yet, it's important to approach these challenges with consideration. Candidates should focus on understanding the problems thoroughly before diving into coding. Misunderstanding a problem could lead to misguided solutions that waste valuable time. Moreover, revisiting and analyzing coding solutions post-challenge can aid in identifying mistakes and reinforcing learning.
Basic Problem-Solving
Basic problem-solving challenges typically involve fundamental algorithms and simple data structures. They lay the groundwork for developing effective coding styles and debugging techniques.
For instance, a common exercise involves writing a method to reverse a string. This not only tests basic string manipulation skills but also encourages candidates to think about efficiency.
Incorporating basic challenges into a study regimen fosters incremental learning. Candidates are encouraged to review different strategies, such as iterative vs. recursive approaches, and contemplate time and space complexities. These reflections further solidify foundational knowledge.
Intermediate Coding Problems
As candidates elevate their proficiency, they encounter intermediate problems that demand a more sophisticated understanding of C#. Such challenges might involve algorithms like sorting or searching, or even implementing various data structures like linked lists or binary trees.
For example, a frequently encountered coding challenge is to determine if a given binary tree is balanced. This task requires knowledge of tree traversal techniques and a firm grasp of recursion.
Hereās a conceptual approach:
- Define a method that computes the height of the binary tree.
- Check the height difference of the left and right subtrees to see if itās no more than one.
- Recursively apply this check to all nodes of the tree.
By consistently engaging with these intermediate-level challenges, candidates not only refine their coding skills but also build the strategies needed to approach complex problems efficiently.
From understanding coding challenges to effectively solve them, the journey through hands-on problems in C# not only prepares candidates for interviews but also equips them with invaluable programming skills for their future careers.
Tips for Preparing for
Interviews
Preparing for a C# interview isnāt just about knowing your syntax or being able to explain concepts like polymorphism or encapsulation. Itās about being ready to tackle the unexpected during the interview process. In todayās tech industry, where programming skills are a hot commodity, acing that interview can be the make-or-break point in landing your desired job.
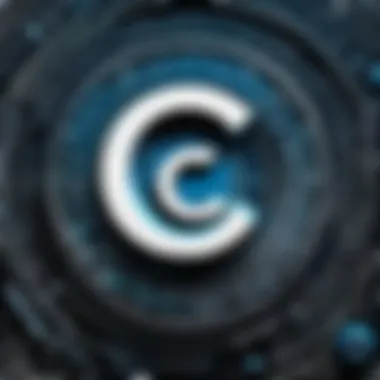
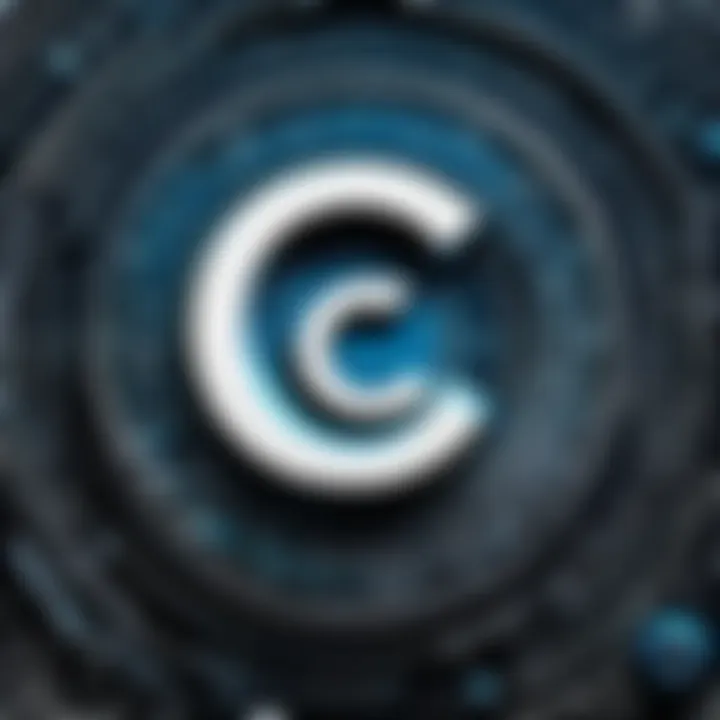
Understanding the Job Requirements
Job descriptions can feel like a jigsaw puzzle, with key skills scattered about. Itās crucial to decode these requirements. First, focus on understanding not just the technical skills listed but also the context in which these skills will be applied. For instance, if a job asks for experience with ASP.NET, dive deeper into what parts of ASP.NET youād use day-to-day. Consider the following when reviewing job descriptions:
- Technical Skills: Look for specific programming languages, libraries, or frameworks mentioned. C# is pivotal, but see if thereās a heavy emphasis on others.
- Soft Skills: Often overlooked, these are just as vital. Communication or teamwork may be highlighted.
- Nature of Projects: Check if the role involves developing web applications, desktop software, or mobile apps, as this can shape which C# features you should focus on.
By aligning your preparation with the job requirements, you can showcase a tailored approach during the interview, making you a more attractive candidate.
Practicing Coding Skills
Nothing beats practice when it comes to mastering coding, and C# is no exception. Familiarity with coding can transform anxiety into confidence when facing those technical questions. Here are some effective ways to sharpen your skills:
- Online Coding Platforms: Websites like LeetCode or HackerRank provide an extensive array of problems. Try to tackle C# specific challenges regularly.
- Pair Programming: Team up with a friend or colleague. This exercise not only improves coding skills but also aids in sharpening your communication skills while coding.
- Projects: Build real-world projects that excite you. Whether itās a personal blog platform or a simple game, applying your skills in practical scenarios helps reinforce your understanding.
- Mock Interviews: Conducting mock interviews can be invaluable. You might feel weird at first, but the feedback will be golden. Platforms like Pramp or Interviewing.io can simulate real interview contexts.
- Review and Reflect: After solving problems, spend a few moments reflecting on your approach. What couldāve gone better? Did you fully understand the problem before jumping in? These insights are crucial for growth.
Never underestimate the power of practice. To quote an old saying, "Practice makes perfect." It might sound clichĆ©d, but thereās truth in it.
Utilizing Online Resources
In today's tech-savvy world, preparing for a C# interview extends far beyond just studying textbooks. Utilizing online resources plays a crucial role in shaping a well-rounded applicant. The combination of accessibility and variety found online can significantly boost one's grasp of essential C concepts. Here, we delve into some notable elements, benefits, and considerations about making the most of these resources.
Tutorials and Practice Platforms
Peer-reviewed platforms such as Microsoft Learn and Codecademy offer interactive lessons that break down complex C# topics into digestible segments. These platforms often incorporate real-world applications that bring abstract concepts into focus. For instance, while learning about delegates in C#, a tutorial may prompt a user to create a delegate-based event handler, reinforcing learning through practical experience.
Benefits of leveraging these tutorials include:
- Immediate Feedback: Users receive instant assessments as they complete lessons, allowing for quick identification of areas needing improvement.
- Structured Learning Paths: Many tutorials provide a step-by-step roadmap, making it easier to track progress and cover all necessary topics systematically.
- Variety of Learning Formats: From written content, videos, to interactive exercises, different formats cater to various learning preferences.
It's essential, however, to choose platforms that offer credible and up-to-date information. Resources that seem outdated can lead to misunderstanding core concepts.
Coding Communities and Forums
Connecting with others who share your interest in C# can be invaluable. Websites like Reddit or dedicated forums provide a space to exchange ideas, ask questions, and even find mentors. It's often said that learning is better in groups, and coding communities embody this spirit. When faced with a tricky C syntax example or a peculiar bug, posting in these communities can yield diverse perspectives and solutions.
- Networking Opportunities: Engaging in discussions can lead to valuable networking opportunities in the tech field.
- Expert Insights: Many seasoned professionals frequent these forums, ready to share their knowledge. Itās a goldmine for insider tips that textbooks might miss.
- Collaboration: Finding someone with complementary skills can lead to collaborative coding projects that enhance practical knowledge.
In summary, making effective use of online resources can greatly enrich your preparation journey for a C# interview. By integrating tutorials, practice platforms, and community interactions, you build a comprehensive understanding of the language that prepares you for success in interviews and beyond.
Expectation Management During Interviews
Managing expectations during an interview is crucial for both candidates and interviewers alike. For someone preparing for a C# interview, understanding this aspect can make the difference between a panic-stricken experience and a more successful, confident performance. The ability to align what one anticipates with what truly transpires can lead to a better self-presentation and insight into the company's goals.
Candidates often walk into interviews with a slew of ideas about what the process might entail ā from the technical depth of questions to the overall ambiance of the interview. However, these preconceptions can be misleading. Many newcomers to the field might think that the interview is merely a test of their technical skills, but it's also about how well they fit within the company's culture and their ability to communicate effectively. Hence, it's vital to cultivate realistic expectations.
Common Misconceptions
One prevalent misconception is that C# interviews solely focus on coding challenges. While technical skills are significant, interviewers often evaluate soft skills, such as communication and problem-solving abilities. They want candidates who can express their thought processes clearly and work through problems collaboratively.
- Misconception 1: "It's all about asking questions in a vacuum."
- Misconception 2: "I have to get every question right."
- Many candidates assume that interview questions will not relate to real-world scenarios. In reality, interviewers frequently frame their queries in the context of practical applications, wanting to see how candidates can utilize their knowledge in practical scenarios.
- Perfection is not the goal. Most interviewers appreciate candidates who can admit when they are unsure and demonstrate a willingness to learn. Showing thoughtfulness in addressing problems often holds more weight than arriving at the correct answer immediately.
By confronting these misconceptions, candidates can approach interviews with a clearer understanding, allowing for a more relaxed demeanor, which can positively affect their performance.
Understanding Interview Dynamics
Understanding the dynamics of an interview process is another key element for effective expectation management. Interviews are a two-way street, where both the candidate and the interviewer assess each other for suitability.
Several aspects play into this dynamic:
- The Role of the Interviewer: The interviewer is not there to solely judge; they also want to find the right candidate for their team. This shifts the atmosphere from an interrogation to a conversation. Candidates should view interviews as opportunities to present themselves, but also to gauge whether the company aligns with their values and aspirations.
- The Importance of Questions: A successful interview often includes back-and-forth questioning. Candidates should prepare thoughtful questions that not only convey interest but can also show their knowledge about the companyās projects and objectives. This can create a more engaging dialogue, enhancing the overall experience for both parties.
- Body Language Signals: Non-verbal communication plays a significant role. A firm handshake, eye contact, and an open posture can express confidence. Candidates should be mindful of their body language and interpret the interviewer's non-verbal cues as well. This awareness can help adjust responses as necessary, ensuring a more favorable interaction.
"An interview isnāt just about answering questions; itās about creating a connection that invites further discussion."
In sum, comprehending and managing expectations when entering C# interviews can remove a layer of anxiety and increase confidence. This allows candidates to approach the interview situation not just as an evaluator but as a participant in a dialogue that could lead to their future professional home.
End and Future Learning Paths
The Conclusion and Future Learning Paths section serves as a crucial wrap-up to the extensive journey through the multifaceted world of C#. Itās not merely a summation; it encapsulates the essence of what has been discussed while offering pathways for further exploration and growth in this programming discipline.
Understanding the key takeaways allows learners to solidify their grasp on fundamental concepts, and this is essential, particularly in the fast-paced world of technology. Whether youāre a budding developer or someone sharpening their skills, recognizing what youāve learned is vital for retention and application in real-world scenarios.
Summarizing Key Takeaways
As the article winds down, itās beneficial to reflect on the pivotal points.
- C# Basics: Mastery of the foundational aspects of C is indispensable. This covers syntax, data types, and core principles that form the bedrock of numerous applications.
- Object-Oriented Programming: A clear understanding of OOP concepts, including classes, inheritance, and polymorphism, is essential. This knowledge aids in creating efficient, scalable applications.
- Advanced Features: Grasping advanced functionalities, like LINQ and async programming, opens doors to writing more robust and flow-efficient code. This aspect can significantly improve your coding toolkit.
- Interview Preparation: Articulating concepts during interviews isnāt just about knowing them; it's also about demonstrating your thought process and problem-solving abilities.
These points are not just abstract notes; they serve as concrete blocks upon which future learning can build. Keep aiming to refine these skills further, as they can make a remarkable difference.
Continuous Learning in Programming
Programming is never a static endeavor. Itās a continual journey where new languages emerge, frameworks evolve, and best practices shift. Hence, engaging in Continuous Learning in Programming becomes indispensable. Here are some strategies:
- Online Resources: Platforms like tutorialspoint, Codecademy and Stack Overflow offer a wealth of tutorials and discussions.
- Community Engagement: Consider joining C# coding communities on platforms such as Reddit or Facebook groups. Engaging in discussions can spur new ideas and insights.
- Project Development: Build personal projects. Diving into hands-on coding challenges can reinforce what you have learned and help identify areas needing improvement.
- Stay Updated: Following industry news can keep you informed about the latest updates in C#. Websites like Microsoftās documentation are valuable resources.
Investing time in continued education in programming will not just augment your skills but also enrich your overall understanding of the field. Remember, staying adaptable and curious is the name of the game in the ever-changing tech landscape.
"In any field, continuous learning is the key to mastery and innovation."
Embarking on this path doesnāt just prepare you for the next interview; it shapes you into a proficient developer capable of navigating complex challenges and contributing meaningfully to any project.