Mastering C# Interviews: Key Insights and Tips
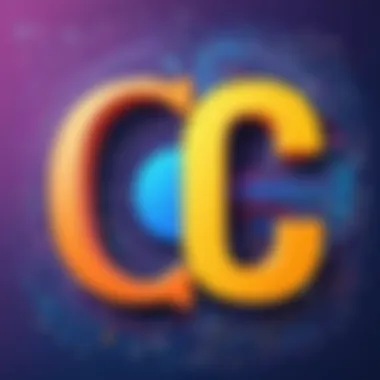
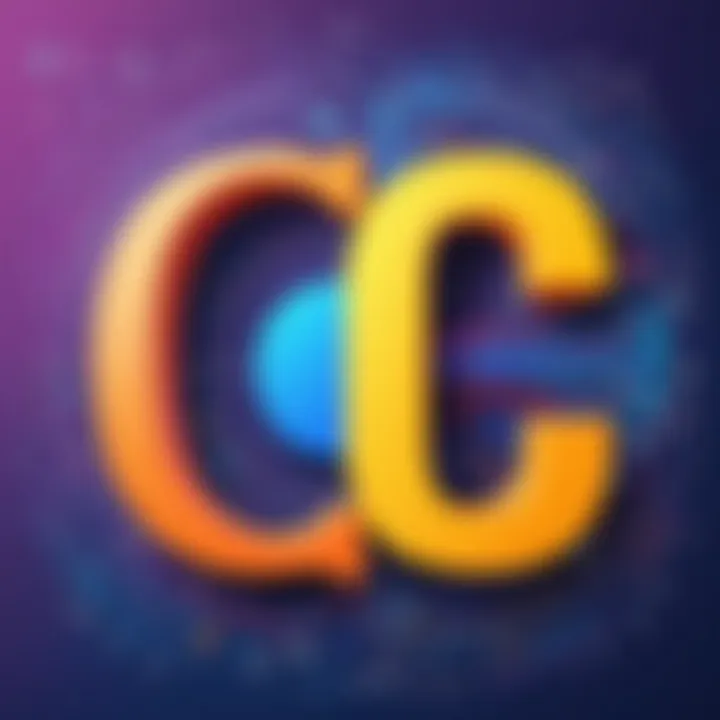
Prologue to Programming Language
C# has carved out a niche for itself in the realm of programming languages, particularly when it comes to developing robust applications and software. This language, released by Microsoft in the early 2000s, has evolved into a staple for many developers around the world. The roots of C are deeply intertwined with the .NET framework, emphasizing its powerful capabilities for building dynamic web applications, enterprise solutions, and even games. Its versatility speaks volumes; you can find C utilized in various sectors ranging from finance to entertainment.
History and Background
The inception of C# traces back to 2000 when Anders Heilsberg, a prominent engineer at Microsoft, introduced the language. Drawing inspiration from languages like C++ and Java, it aimed to provide a simpler syntactic structure while maintaining strong type-checking and object-oriented features. The language has continually evolved through different versions, each time introducing new features and improvements. With the introduction of .NET Core, C has truly become a cross-platform powerhouse, enabling developers to run applications on Windows, Linux, and macOS with relative ease.
Features and Uses
One of the most striking features of C# is its object-oriented capabilities, allowing developers to model real-world entities and foster code reusability. Additionally, C supports properties, events, and delegation, making it extremely versatile for handling various programming challenges. Here are a few key uses of C#:
- Web Development: Through ASP.NET, developers can build sophisticated web applications that are scalable and secure.
- Game Development: Unity, a leading game engine, utilizes C#, allowing developers to create immersive interactive experiences.
- Desktop Applications: C# is frequently used to develop Windows applications, giving them a modern look and feel.
- Cloud Services: With the growing focus on cloud computing, C# integrates seamlessly with Azure, Microsoft’s cloud platform, which significantly boosts its relevance.
Popularity and Scope
Over the years, C# has gained a loyal following among developers and organizations alike. According to the TIOBE Index and Stack Overflow Developer Survey, C consistently ranks among the top ten most popular programming languages. This popularity can be attributed to a combination of robust community support, extensive libraries, and the backing of Microsoft, which ensures continuous development and improvement. As industries escalate their digital transformations, the demand for C developers is likely to rise further, underlining the importance of mastering its nuances for any aspiring programmer.
"C# is not just a language; it's a toolset that empowers developers to take their visions from concept to reality."
Now that we’ve set the stage with a foundational understanding of C#, we’ll venture into the core concepts and syntax that aspiring candidates will need to grasp thoroughly for their upcoming interviews.
Basic Syntax and Concepts
Understanding the basic syntax and foundational concepts is critical when preparing for a C# interview. Grasping how C structures code will give you the confidence to tackle either theoretical or practical questions during interviews.
Variables and Data Types
In C#, every piece of data you work with is categorized into a specific data type. Variables serve as containers for these data types. Key data types include:
- int: For integers ranging from -2,147,483,648 to 2,147,483,647.
- string: For text, enclosed in double quotes.
- bool: For values that are either true or false.
Here's a simple example of declaring variables in C#:
Operators and Expressions
C# comes with a rich set of operators that help manipulate data. These include arithmetic operators (+, -, *, /), logical operators (&&, ||, !), and relational operators (==, !=, >, ). Understanding how these operators work is essential for constructing logical expressions.
Control Structures
Control structures determine the flow of execution in a program. In C#, typical structures include:
- if statements: For conditional logic.
- for loops: To repeat actions until a specified condition is met.
- switch statements: To execute different parts of code based on the value of a variable.
Example of a simple if statement:
By mastering these foundational elements, candidates can significantly enhance their performance in interviews, prompting a smoother transition into advanced topics.
Understanding
and Its Relevance
Understanding the C# programming language is not just an academic exercise; it’s a necessity for those looking to carve a niche in today’s software development landscape. C opens the door to a myriad of career opportunities in various domains, particularly in web development, game development, and enterprise solutions. Its versatility and robust framework make it a staple in many organizations, their foundations built on the power of C#.
Moreover, as you prepare for a C# interview, grasping the fundamentals of C isn’t merely about memorizing syntax. It’s about understanding how this language fits into broader development practices and methodologies. Let's dive deeper into the nuances of C#.
Overview of
Language
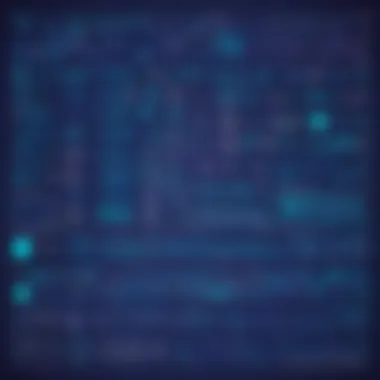
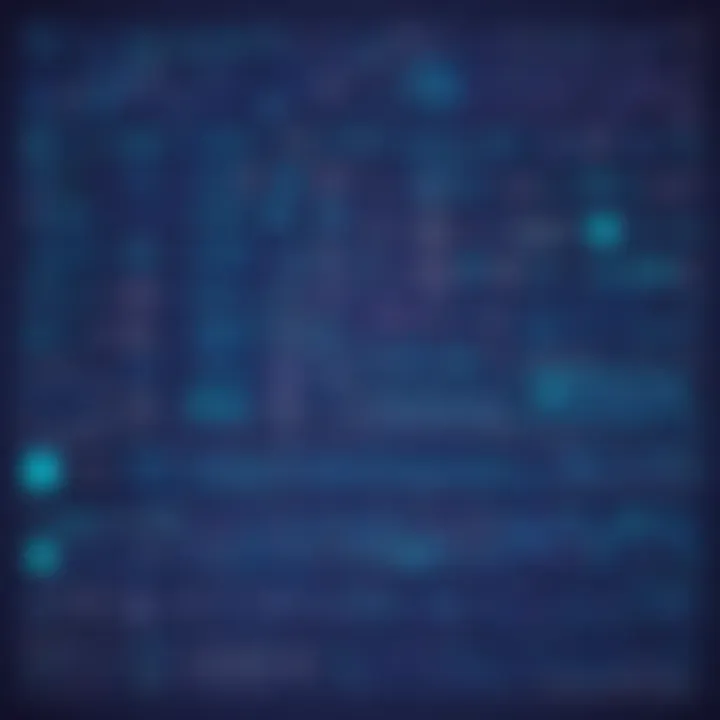
C# was developed by Microsoft in the early 2000s as part of its .NET initiative. At its core, C is an object-oriented language, emphasizing principles like encapsulation, inheritance, and polymorphism. This allows for strong software development patterns, leading to cleaner, maintainable code. Being part of the .NET ecosystem, it has seamless access to a wide array of libraries and tools that expedite the development process.
One of C#'s standout features is its type safety. This ensures that type errors are caught at compile time rather than at runtime, reducing bugs that may creep into production. Coupled with its garbage collection capabilities, C# simplifies memory management for developers, allowing them to focus on creating functional and efficient applications.
More than just a programming language, C# incorporates support for asynchronous programming, enabling developers to build more responsive applications. This has proven invaluable in today’s fast-paced environment where user experience is paramount. To put it simply, knowing C well can be the difference between a good developer and a great one.
in the Modern Development Landscape
The modern development landscape is bustling with myriad programming tools and frameworks, yet C# stands its ground as a robust choice for many developers. Today, C is not confined to traditional application development; its relevance extends into web development with ASP.NET, game development using Unity, and even mobile app development through Xamarin. This flexibility allows developers to pivot across disciplines without the need to start from ground zero in a new language.
C# boasts a rich community and an extensive support system, measurable through forums and resources such as Reddit and Stack Overflow. The language receives continuous updates, integrating the latest programming trends, making it a forward-looking choice. With the push towards cloud computing and microservices, C keeps pace with innovations like Azure, enabling easy deployment and scalability of applications.
In today’s job market, proficiency in C# is often seen as a strong asset, captivating the eye of hiring managers. Whether you're aiming for a position at a tech giant or a startup, the ability to navigate C confidently can place you a step ahead of the competition.
"Learning C# is not just about understanding the language; it's about embracing the extensive ecosystem that it brings along."
Key Concepts to Master
Understanding the essential concepts of C# is vital for any candidate aiming to stand out in interviews. This section digs into the foundational elements necessary for mastering C#, which ultimately shapes your approach to programming and problem-solving. A clear grasp of these concepts not only prepares you for technical questions but also helps in demonstrating your proficiency in real-time coding scenarios. By the end of this section, you will appreciate their importance and how they integrate into the broader C ecosystem.
Object-Oriented Programming Principles
Object-oriented programming, or OOP, serves as the backbone of C#. Understanding its principles is much more than a good-to-have; it's a must. The four main principles – encapsulation, polymorphism, inheritance, and abstraction – provide a framework for writing clean, maintainable, and efficient code. Let's break down each principle to see how they relate to C# and why they are beneficial choices for any programmer.
Encapsulation
Encapsulation involves bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class. The key characteristic of encapsulation is that it restricts direct access to some of the object's components, which helps to protect the integrity of the data. For instance, consider a banking application: account balances should be stored privately, only allowing access through methods like deposit or withdraw. This makes it a popular choice in the context of C# development, as it enforces a cleaner design.
One unique feature of encapsulation is getter and setter methods, allowing controlled access to class properties. The main advantage is safeguarding sensitive data from unintended modifications. However, a downside could be reduced direct access to data, potentially leading to more complex code when the encapsulation is overly strict. In this article, we endorse encapsulation not just for security but also as it leads to a greater code organization.
Polymorphism
Polymorphism enables objects to be treated as instances of their parent class rather than their actual class. This principle is particularly breathing life into interfaces and virtual methods within C#. A key characteristic of polymorphism is its ability to call the same method on different objects, adapting its behavior accordingly. This makes it a beneficial approach in constructing flexible code.
Imagine an interface for a payment processor: you can implement different payment methods like credit card, PayPal, or bank transfer, each having its own functionality while still adhering to a common interface. The advantage? It lends itself to easier scalability and code maintenance. A disadvantage could arise in terms of performance overhead, as method resolution at runtime can be less efficient than using traditional method calls. In this article, we advocate for polymorphism as a means to write extensible applications.
Inheritance
Inheritance allows a new class to inherit properties and behaviors from an existing class. The core characteristic here is the 'is-a' relationship, meaning subclasses can extend or override functionalities of their parent class. This principle is crucial for code reusability, a prominent benefit when you deal with multiple similar entities.
For instance, if you have a base class called , derived classes such as and can inherit common properties and methods. This significantly reduces code duplication, making maintenance easier. However, a potential downside is the risk of creating a fragile class hierarchy; changes to parent classes can have cascading effects on subclasses. Hence, in this narrative, we emphasize the power of inheritance while also advising caution regarding complex class relationships.
Abstraction
Abstraction is about focusing on the essential qualities of an object while hiding any unnecessary details. This means presenting users with only the relevant information. The key characteristic of abstraction in C# is the use of abstract classes and interfaces which define contracts that derived classes must adhere to.
Think of a remote control for a television: you only interact with buttons like "Power" and "Volume", without needing to know the inner workings of the device. The advantage here is that it simplifies interactions with complex systems and promotes a more user-friendly experience. However, too much abstraction can lead to interfaces that are so general, they become cumbersome to implement. For this article, we see abstraction as essential to building systems that are not only simpler to use but also scalable.
Common
Data Types
Data types form the foundation upon which programming constructs are built. In C#, understanding these types is necessary not only for writing effective code but also for troubleshooting potential issues. Both value types and reference types play their roles here. Value types, like or , store the data directly, while reference types, like objects or arrays, store references to the actual data. Knowing the difference matters when managing memory efficiently and when dealing with performance.
Error Handling and Exceptions
In any software development context, robust error handling is indispensable. C# provides a structured way to handle exceptions using , , and blocks. Understanding how to gracefully manage errors not only protects your application from crashing but also improves user experience. Also notable is the creation of custom exceptions tailored for specific scenarios which can help streamline debugging processes. A solid grasp of exception handling is, therefore, not just recommended; it’s fundamental.
Interview Preparation Techniques
Preparing for a C# interview goes beyond just brushing up on your resume. It requires a strategic approach where you focus on key techniques that can set you apart from other candidates. Mastering these interview preparation techniques can significantly improve your chances of impressing potential employers and landing the job you desire.
Reviewing the Fundamentals
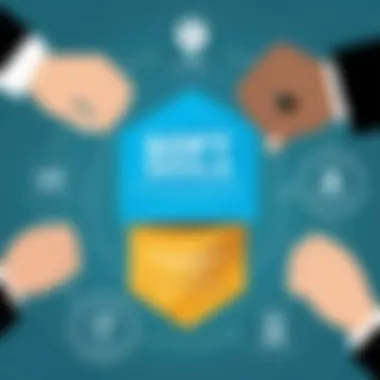
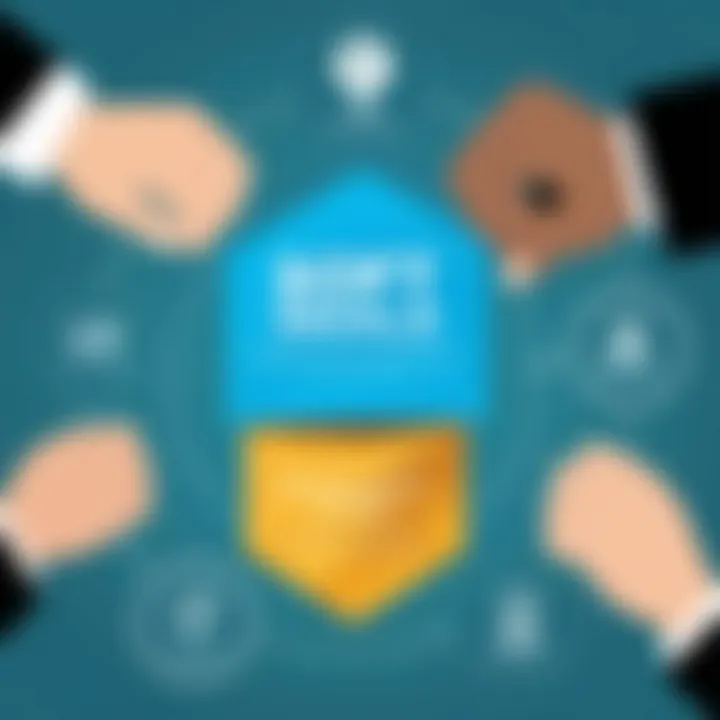
Fundamentals lay the groundwork for any successful interview. A thorough understanding of C# concepts, syntax, and programming paradigms is essential. Start with a clear revision of core C principles like variables, data types, and control structures. Don't just skim through them; delve deep. Setting up small projects or practice tasks is a good way to solidify this knowledge.
Moreover, understanding how C# integrates with frameworks like .NET can be a game changer. Knowing the ins and outs, such as managing credentials or deploying an application, helps paint a complete picture. As the saying goes, knowledge is power, and a strong foundation can make you the go-to candidate.
Practice Coding Challenges
Engaging with coding challenges is not only productive but also vital for sharpening your technical skills. These challenges are often representative of what you'll face in an actual coding interview, allowing you to think critically and on your feet.
Resources for Coding Practice
There are a plethora of resources available for coding practice, which can be particularly beneficial for honing your problem-solving skills. Websites like LeetCode, HackerRank, and CodeSignal offer a wide array of coding challenges tailored for different skill levels. The unique feature of these platforms is their interactive environment, allowing you to code and test your solutions in real-time. These sites help you track progress and provide a ranking system that can motivate you to push further.
The downside? The sheer volume of problems can feel overwhelming. It can also be tempting to rush through them in search of quick answers. Taking your time to analyze each problem and understand various solutions is paramount to mastering them.
Common Algorithm Problems
Understanding common algorithm problems is crucial because they can often be staples in interviews. Problems involving arrays, strings, trees, and graphs are frequently asked. Familiarizing yourself with algorithms like binary search, depth-first search or sorting techniques gives you a practical toolbox to draw from.
These problems compel you to think logically and apply various C# features effectively. The takeaway is not just to solve these problems but to also articulate your thought process. This is a key trait that employers often look for—the ability to communicate your reasoning.
Mock Interviews for Real-World Experience
Finally, mock interviews can provide invaluable real-world experience. Practicing with friends, mentors, or through platforms like Pramp not only increases your comfort level but also uncovers areas needing improvement. Feedback from these mock sessions can be incredibly beneficial, highlighting your weaknesses without the pressure of an actual interview. Consider using feedback to structure your study sessions; this gives you a focused approach, turning every mock session into a valuable learning experience.
Commonly Asked
Interview Questions
When gearing up for a C# interview, understanding the commonly asked questions can be a game changer. These questions not only help you gauge your own understanding of the language but also prepare you to respond effectively under pressure. Furthermore, many of these questions provide insight into what interviewers are most concerned about, allowing you to tailor your study and practice sessions accordingly.
The essence of mastering these questions lies in their ability to bridge the gap between theoretical knowledge and practical application. By familiarizing yourself with the types of inquiries you might face, you can develop a more strategic approach to your preparation.
Technical Questions to Expect
Technical questions often form the crux of interviews for C# roles. Expect inquiries that delve into fundamental concepts and advanced features of the language. These questions usually assess your problem-solving skills and your understanding of the language's architecture. Here are some examples and themes you may encounter:
- Data Types and Variables: "What are the differences between value and reference types?"
- Object-Oriented Programming: "Can you explain polymorphism and its use cases in C#?"
- Delegates and Events: "How do delegates differ from interfaces? Can you provide an example of when you would use an event?"
- LINQ Queries: "What is LINQ, and how does it simplify data manipulation in C#?"
- Garbage Collection: "Can you describe how the garbage collector works in .NET?"
Each of these questions not only examines your technical skills but also your ability to apply knowledge effectively. Having clear, concise, and articulate answers prepared can greatly increase your confidence during the interview.
Behavioral Questions: Preparing for Soft Skills
Behavioral questions represent another important facet of the interview process. Interviewers often use these questions to gauge your interpersonal skills and how you interact with team members. They are designed to assess your cultural fit within the company, your problem-solving abilities in real-world situations, and how you cope under pressure.
Here are some typical behavioral questions you might face:
- Team Collaboration: "Can you describe a time when you had to work closely with others on a project? What role did you play?"
- Conflict Resolution: "Tell us about a challenge you faced with a colleague. How did you handle it?"
- Adaptability: "How do you manage deadlines when you have multiple tasks on your plate?"
- Learning from Mistakes: "Describe a project that did not go as planned. What did you learn from that experience?"
Approaching behavioral questions with a structured method such as the STAR technique (Situation, Task, Action, Result) can help articulate your experiences more effectively.
Additional Skills to Highlight
When it comes to C# interviews, technical knowledge is just the tip of the iceberg. Highlighting additional skills can make the difference between securing a position or being passed over for another candidate. In this regard, two specific areas stand out—software development lifecycles and version control systems. Both aspects not only demonstrate a well-rounded candidate but also showcase key competencies that are increasingly demanded in modern software development environments.
Understanding Software Development Lifecycles
Knowledge of software development lifecycles (SDLC) is pivotal for any aspiring developer. It refers to the various stages that a software project goes through, from initial planning through to deployment and maintenance. The steps in the SDLC can vary between methodologies, but common stages include:
- Requirement Analysis: Understanding what the users want and need from the software.
- Design: Planning the architecture of the software.
- Implementation: Writing the actual code, which in the case of this article, would likely be in C#.
- Testing: Ensuring the application is free of bugs and fulfills all requirements.
- Deployment: Releasing the software to users.
- Maintenance: Ongoing support and updates for the software.
Knowing SDLCs can give you an edge in interviews for a couple of reasons. First, it shows you understand how your contributions fit into the larger picture of software development. It also highlights your ability to work collaboratively with other team members across different stages of the process.
Familiarity with Version Control Systems
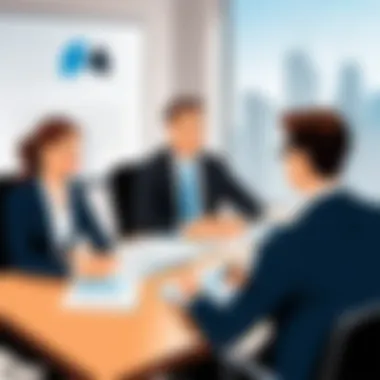
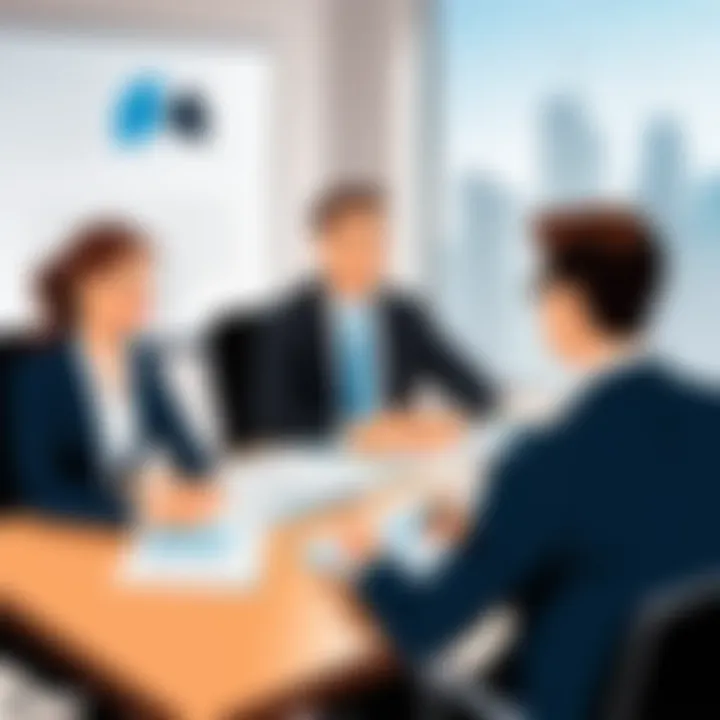
Another skill that potential employers value is familiarity with version control systems, with Git being the most prominent example. Version control helps track changes in project files, allowing multiple developers to collaborate efficiently. Here’s why it’s important:
- Collaboration: You can work on projects simultaneously without stepping on each other's toes.
- History Tracking: Version control maintains a history of changes, which can be invaluable when debugging or understanding previous decisions.
- Branching and Merging: You can try out new features in isolated branches and merge them once they are stable.
Understanding Git commands and workflows is often a prerequisite in many job postings. You might be asked about your experience with push, pull, commit, or branch. Proficiency in these areas shows that you can integrate into a development team quickly, minimizing onboarding time and potential errors in collaborative projects.
"Having a good grasp of software lifecycles and version control systems can make you not just a candidate, but a valuable asset to any team."
In summary, while mastering C# is undoubtedly crucial, supplementing your technical expertise with knowledge of software development lifecycles and version control can significantly enhance your appeal during the interview process. These additional skills better position you within a competitive job market, signaling to potential employers that you are both knowledgeable and adaptable, ready to tackle the challenges that come with software development.
Post-Interview Strategies
After stepping out of a C# interview, candidates often find themselves in a whirlwind of thoughts. What went right? What went wrong? Is it too late to add that one point I flubbed? This phase, often overlooked, is crucial for self-improvement and future interview success. Effective post-interview strategies not only provide clarity but also act as a bridge to ongoing personal and professional development.
Reflecting on Interview Performance
Taking the time to honestly reflect on one’s performance can be invaluable. This isn't just a casual stroll through memory lane; it's about dissecting every part of the interview. Candidates should ask themselves:
- What questions stumped me?
- Which topics did I handle well?
- Were my responses aligned with what the interviewer sought?
Creating a written account can help solidify these reflections. One could maintain a small journal dedicated to interviews, detailing the questions asked and the answers provided. This way, the candidate can identify patterns in their responses, which may reveal strengths to leverage and weaknesses to work on.
Understanding where one faltered will inform preparation for the next opportunity, allowing candidates to turn mishaps into motivation. It's a bit like going back and watching game footage as an athlete — you learn from both the successes and the blindsides that happened on the field.
Following Up with Interviewers
Sending a follow-up message after an interview isn't just about being polite; it's a strategic move. A well-composed thank-you email goes a long way. It shows professionalism and reaffirms interest in the position.
In this email, candidates should:
- Express gratitude for the opportunity to interview.
- Mention a specific moment from the discussion to personalize the note. This could be a shared interest or a topic that was particularly engaging during the interview.
- Reiterate excitement for the role and the company. This keeps the candidate's name fresh in the interviewer's mind.
A timely and thoughtful follow-up can differentiate candidates from others who may have interviewed for the same position.
The timing is key; sending this email within 24 hours of the interview is ideal. It shows eagerness and respect for the interviewer's time. Additionally, if candidates haven't heard back from employers within a week or two, a gentle nudge may be appropriate. This further expresses continued interest and provides opportunities to re-engage in conversation, potentially keeping the door open for future discussions.
In essence, the post-interview stage operates as a critical component of the enduring job search journey. By reflecting on performance and maintaining communication with interviewers, candidates can refine their skills, enhance their visibility, and position themselves favorably for future opportunities. Engaging actively in these strategies can transform the often nerve-racking experience of interviewing into a springboard for professional growth.
Resources for Ongoing Learning
In the ever-evolving world of technology, keeping abreast with the latest advancements and skills is more than just beneficial—it's essential. Having a strong grasp of C# and its ecosystem requires continual learning, and that's where the concept of ongoing resources comes into play. This segment discusses key areas that are vital for candidates, offering routes to stay informed and ahead of the curve.
Continuous learning equips you with practical knowledge that can be directly applied in real-world scenarios. It also sharpens your problem-solving skills, making you a more appealing candidate in a competitive job market. With the tech industry’s rapid growth, the skills you have today might become outdated tomorrow. Therefore, sourcing ongoing learning opportunities ensures that you remain relevant and capable of tackling fresh challenges as they arise.
Online Courses and Certifications
Online courses and certifications provide structured and flexible learning options for aspiring C# professionals. Platforms like Coursera, Udemy, and Pluralsight offer a variety of C courses tailored to different skill levels. These courses often include video lectures, coding exercises, and peer discussions, which can enhance your understanding of complex concepts.
One major advantage of these courses is the opportunity they give you to learn at your own pace. For instance, if you find a specific topic particularly challenging, you can take your time going over the related materials without the pressure of a timeline.
Some course highlights to consider include:
- Real-World Projects: Engaging in projects simulates real-life programming scenarios. This practical exposure reinforces theoretical concepts.
- Certification: Earning certificates can significantly bolster your resume. Companies recognize certifications as proof of a candidate’s commitment and proficiency in a specific skill set.
- Networking Opportunities: Forums and community groups associated with these courses can enable you to connect with fellow learners and professionals in the field. Through platforms like LinkedIn, you can also showcase your completed projects and certificates.
While choosing online courses, always look for reviews and course content that aligns closely with what you need to learn.
Books and Documentation
Books and official documentation are also invaluable resources for learning C#. They allow for deeper exploration into topics that courses might only skim over. Texts such as "C# in Depth" by Jon Skeet or "Pro C#" by Andrew Troelsen offer thorough insights and advanced concepts that challenge the reader’s understanding.
Moreover, Microsoft’s official documentation is an underutilized gem filled with detailed information on syntax, best practices, and libraries. This is especially pertinent for coding languages like C# that are subject to change.
Key benefits of books and documentation include:
- In-Depth Knowledge: Books provide comprehensive coverage on subjects, often including nuances that online courses may overlook.
- Reference Material: Both books and documentation serve as handy references. You can go back to specific sections when you need clarification on complex topics or when you’re coding and need to understand how to implement a certain feature.
Increasing your knowledge base should be both rigorous and enjoyable. Make a habit to read regularly, bookmark useful documentation, and perhaps take notes. You can even create a learning plan that includes a mixture of both online and traditional forms of study to maintain a balanced approach to mastering the language.
"The beautiful thing about learning is that no one can take it away from you."
Ongoing education is vital for professional growth. By utilizing the resources available to you, you increase the chances of not only getting the job but excelling at it. As the saying goes, with great knowledge comes great opportunity.