Build Dynamic Web Applications with React JS
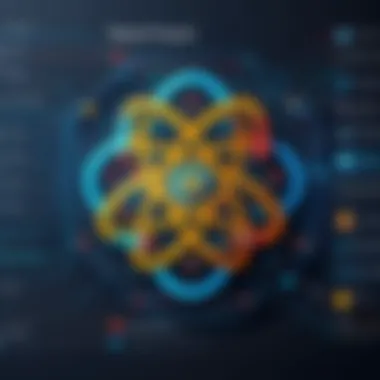
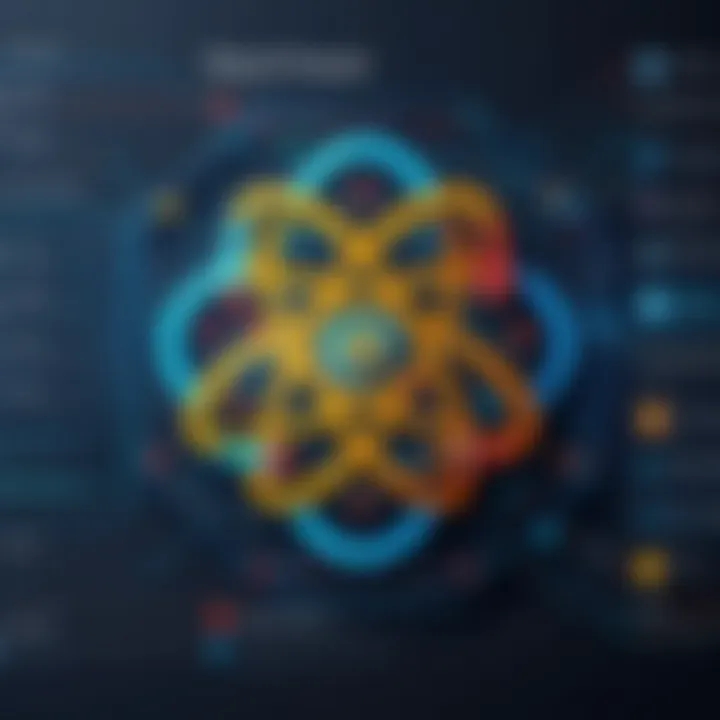
Intro
Creating web applications has become increasingly prominent in todayâs tech-driven world, and React JS stands out as a versatile library for building these dynamic interfaces. Whether you're a novice eager to dive into the coding world or an intermediate programmer looking to enhance your skills, understanding React is crucial. This guide aims to simplify the complex world of React, breaking it down into digestible chunks that both fledgling and seasoned developers can find valuable.
Why Learn React?
As the digital landscape continues to evolve, so does the demand for interactive, user-friendly applications. React JS, developed by Facebook, offers a robust solution. It enables developers to create single-page applications where users can interact without constant page reloads. This enhances user experience and boosts engagement.
What to Expect in This Guide
Throughout this article, we will explore the essential components and methodologies involved in creating React applications. From foundational concepts to advanced implementations, here's what weâll cover:
- Fundamental Concepts: Understanding how React works and its core principles.
- Development Tools: Essential tools for setting up your development environment.
- Hands-On Examples: Practical examples and projects that reinforce learning.
- Best Practices: Tips to enhance code quality and improve maintainability.
- Resources: A curated list of materials for continued learning beyond this guide.
By the end of this journey, youâll not only have a clearer understanding of React but also be equipped with the skills to embark on creating your own applications.
Prelude to React JS
The significance of React JS in web development can't be understated, especially for those who are new to programming. As a library for building user interfaces developed by Facebook, React offers a robust structure and flexibility that is critical in today's fast-paced tech environment. Understanding React is essential, not just for making interactive applications but also for grasping fundamental programming concepts that can apply across various platforms.
What is React JS?
React JS, at its core, is a JavaScript library designed for constructing user interfaces, primarily for single-page applications. Created by Facebook, its primary aim is to enable developers to build large web applications that can change data, without reloading the page. One of the main draws of React is its component-based architecture. This means developers can create encapsulated components that manage their own state, and then compose them to create complex UIs.
What sets React apart is its emphasis on declarative programming, meaning users can describe what their UI should look like for any given state, and React manages the updates as the state changes. This leads to more predictable and easier-to-debug code. If you're looking to create a dynamic, responsive web application, knowing what React is and how it functions lays a solid foundation.
Key Benefits of Using React
There are several reasons why React has become a staple in the toolbox of modern web developers. Let's delve into some of its key benefits:
- Component-Based Architecture: Each UI element is a component, which can be reused across the application. This promotes consistency and ease of updates.
- Virtual DOM: React creates a lightweight representation of the actual DOM, allowing for faster updates. This improvement in performance is not trivial when building complex applications.
- Unidirectional Data Flow: Data in React flows in one direction, which simplifies debugging and enhances the predictability of an application's behavior.
- Rich Ecosystem: The React ecosystem is filled with powerful tools like Redux for state management, providing solutions that can significantly enhance development speed and efficiency.
- Strong Community Support: With a massive community of contributors, resources, and libraries, finding help or improving your skills is easier than ever.
In summation, React provides the tools necessary to tackle both simpler projects and complex applications. Its flexible nature and supportive environment make it a prime choice for developers at all levels.
Understanding the Virtual DOM
The Virtual DOM is a pivotal concept in React that greatly enhances performance. Unlike the real DOM that directly updates the user interface, the Virtual DOM serves as a middleman. When any change occurs in a component's state, React first updates the Virtual DOM. Then, it compares the new Virtual DOM with the previous versionâa process known as 'diffing.'
This results in a very efficient update process. Only the components that have changed are updated in the real DOM, minimizing costly operations and resulting in a faster user experience. This method addresses one of the main performance issues in traditional web applications where changes to the DOM can lead to lag and inefficiencies. Understanding how the Virtual DOM works not only helps in optimizing applications but also serves as an enlightening lesson in efficient programming practices.
"React's Virtual DOM is like a clever assistant, working swiftly behind the scenes to keep your application spry and responsive."
In summary, grasping these core concepts about React provides you with a holistic understanding of what makes this library so powerful and essential for web development today.
Setting Up the Development Environment
Setting up a development environment is crucial in building robust React applications. It lays the groundwork for everything youâll do after. A well-configured space allows for smooth coding, effective testing, and streamlined deployment processes. Without proper tools and settings, you might feel like youâre trying to bake a cake without an ovenânot very effective. This section will cover the foundations of readying your environment for React development, ensuring that you're equipped with not just the right tools, but also the knowing of how to utilize them efficiently.
Prerequisites for React Development
Before jumping headfirst into building React applications, there are some prerequisites worth noting. First, having a solid grasp of HTML, CSS, and JavaScript is paramount. React relies heavily on JavaScript syntax, so familiarity with functions, arrays, and ES6 features will serve you well. Having a basic understanding of how browsers work and the Document Object Model (DOM) is also beneficial.
Donât overlook the importance of package managers and build tools. Git, for version control, and Node.js, for server-side JavaScript execution, are essential. Getting the hang of these tools prior to diving into React can save you a boatload of headaches down the road.
Installing Node.js and npm
Node.js is the backbone of your React development. It allows you to execute JavaScript server-side, and npm (Node Package Manager) is bundled alongside it, facilitating easy management of the packages you might need. Installing these is usually straightforward, but itâs important to download the right version from the official Node.js website.
Once installed, you can verify it by running the commands:
If everythingâs in shipshape, youâll see the respective version numbers displayed in your terminal. This is your first step toward getting things rolling with React.
Creating a New React Application
When it comes to creating a new React application, there are two commonly traveled paths: Using Create React App and Setting Up a Custom Configuration. Each has its pros and cons, and the choice largely depends on your project needs and personal preference.
Using Create React App
Create React App (CRA) is like the Swiss Army knife for budding React developers. It provides a simple setup allowing you to focus on writing code without getting mired in the configuration weeds. With CRA, you can create a new project with a single command:
This command initializes a new React project for you, complete with a development server and an optimized build setup. Itâs especially appealing because you can start working immediately, without delving into complex configurations.
One key characteristic of CRA is its built-in best practices. It's largely opinionated but also customizable to suit your needs. However, while itâs great for starting out, it may feel constraining for more advanced users who want fine-tuned control over the project.
Create React App offers a zero-configuration setup that, while easy, may limit flexibility as your app grows.
Setting Up a Custom Configuration
On the other hand, setting up a custom configuration can be a blessing for those who like to have everything just so. This approach involves manually setting up Webpack, Babel, and other tools. While this method requires more initial effort and know-how, it can provide a tailored environment that perfectly fits your project needs.
The key benefit of custom configuration is controlâyou call the shots. You can decide exactly how your project is structured, what tools you want to include, and how dependencies are managed. However, this can also lead to complexities that may slow you down, especially if things donât go as planned.
Being mindful of these aspects will help you make an informed choice about which setup to use for your React application, ensuring that youâre well-equipped to tackle your coding projects.
Core Concepts of React
In the realm of modern web development, understanding the core concepts of React is akin to possessing a compass in uncharted territory. These fundamentals guide developers to create efficient, scalable, and maintainable applications. Each cornerstone of React serves a distinct purpose and significantly impacts how applications behave and interact with users.
Components and Props
Components are the bedrock of any React application. They allow developers to break down complex UIs into smaller, reusable pieces. This not only enhances organization but also improves testability and maintainability. In simpler terms, components make your code cleaner and easier to follow.
Defining Functional Components
Functional components are among the simplest ways to create components in React. They are essentially just JavaScript functions that accept props as an argument and return React elements. These components are lightweight and often lead to cleaner and more concise code relative to their class counterparts.
One key characteristic of functional components is their stateless nature. They donât have their own state but can receive data through props, making them a good fit for presenting data and rendering UI. This simplicity is one of the reasons why functional components have taken center stage, especially with the introduction of React Hooks, which allow you to manage state and side effects within them.
However, a unique feature to consider is that they rely heavily upon external hooks for functionality that classes traditionally handled, such as lifecycle methods. This can sometimes confuse newcomers, especially when they come from a background with class-based components.
Understanding Class Components
Class components, on the other hand, provide a more traditional object-oriented approach to building React components. They offer a robust structure for managing local state and lifecycle events, making them suitable for components that require more advanced behavior.
A notable characteristic of class components is that they can hold their own state and come with built-in lifecycle methods, providing a more comprehensive way to handle component behavior. This is particularly useful for building more complex user interfaces that require a degree of interactivity and behavior management.
However, one disadvantage is that class components can be more verbose and harder to read compared to functional components, with a steeper learning curve for new developers. Transitioning from classes to hooks can also be a mental hurdle for those already steeped in class-based structures.
State Management in React
State management is crucial for any interactive application, as it dictates how components react to user inputs, data changes, and more. Properly managing state ensures your application can perform optimally and provide a fluid user experience.
Understanding State
In React, state represents the parts of an app that can change over time. It is an object that determines a component's behavior and how it renders. Managing state effectively is key to building dynamic applications.
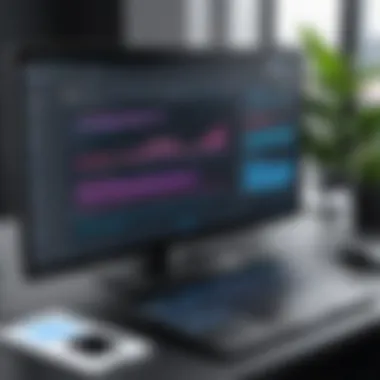
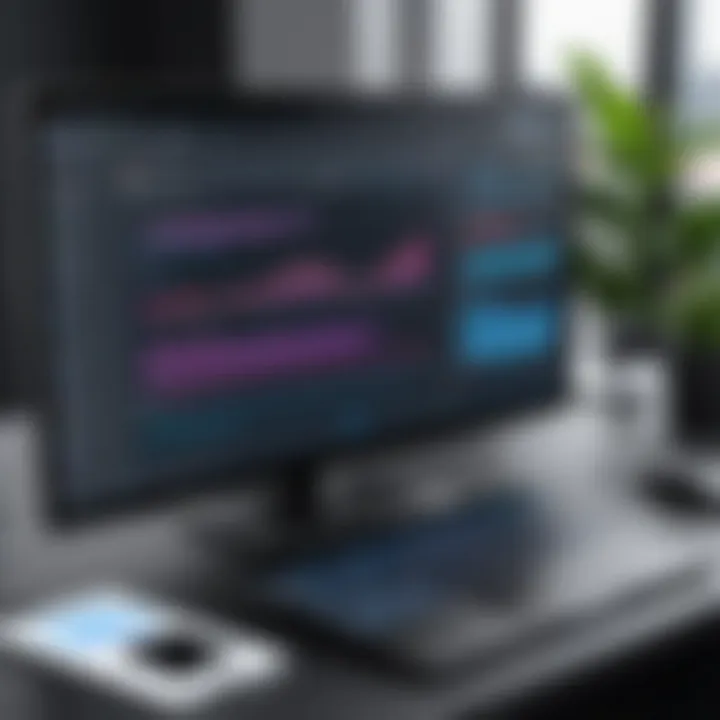
A significant characteristic of state in React is its encapsulation within components. Each component maintains its own state, which can interact with other states through props. This separation promotes modularity, meaning that changes in one component's state don't directly affect others without explicitly passing data.
A unique aspect of state is that it initiates the re-rendering of components when it updates. This can be a double-edged sword: while it makes sure the UI aligns with the data, developers must be mindful of optimizing re-renders to avoid performance hiccups.
Updating State with setState
To update state, React provides the method, which is crucial for enabling interactivity within components. This method takes an object or a function as an argument, allowing developers to incrementally change the state rather than replace it entirely.
The main characteristic of is its asynchronous nature. Challenges can arise due to this delay in state updates, which may lead to unexpected results when relying on the current state for calculations. Understanding this asynchronous behavior of state updates is vital for smooth app functionality.
One unique aspect of this method is its batched updates, where multiple state updates can be grouped together to avoid excessive rendering, which is beneficial for performance. However, neglecting this behavior can lead to confusing bugs if not properly managed.
Lifecycle Methods
Lifecycle methods are the hooks that give developers insight and control over a componentâs life cycle, from mounting to unmounting. These methods are essential for handling tasks such as data loading, timer operations, and cleanup actions.
Component Mounting
When a component mounts, it signifies the initial phase of its life cycle. Developers can leverage this moment to fetch data or set up initial states.
A key feature of mounting is the method, allowing code to run right after the component is inserted into the DOM. This makes it a prime spot for fetching data, as it ensures the component is ready to display the information.
However, this phase can lead to performance issues if data fetching isnât optimized, particularly if large datasets are involved.
Component Updating
Component updating occurs whenever a componentâs state or props change. This phase is critical as it affects how the UI reacts to user actions or external changes in data.
One of the essential methods triggered during this phase is . This method allows you to act after changes to the component, such as fetching new data as a response. Understanding when and how to utilize this method is vital for developing responsive applications.
Overusing updates, however, can lead to performance bottlenecks, especially when updates cause more updates.
Component Unmounting
The unmounting phase may not get as much focus, but it plays an equally important role in application efficiency. When a component unmounts, it's your chance to perform cleanups such as cancelling API calls or invalidating timers.
The method is the designated spot for such actions. This method is crucial for ensuring that your app doesnât leak memory by preventing operations from continuing on removed components.
Neglecting this cleanup can lead to persistent data processes that slow down or crash your application over time.
Handling Events and Forms
When it comes to building interactive applications, handling events and forms is right at the heart of user experience. Itâs how you make your application responsive to user actions, be it a mouse click or a keyboard input. In the world of React, getting a grasp on these concepts can make or break an application's overall functionality. Each interaction has to feel seamless, so a solid understanding of handling events and forms can be a game-changer.
Event Handling in React
Reactâs approach to event handling diverges from traditional JavaScript. In plain JavaScript, one might attach listeners directly to DOM elements. React, however, uses a synthetic event system, allowing a consistent and normalized way to handle events.
This means that when you write code in React, you're dealing with events in a more predictable manner. Youâll often use JSX to bind events using camelCase naming conventions. For instance:
Whatâs important to note is that React events are effectively wrappers around native events. They are passed directly to React components, which can then be accessed through functions. This helps in maintaining the declarative nature of React, allowing you to focus on what the UI should look like at any given moment based on the state of your application.
Creating Controlled Components
Controlled components are the backbone of form handling in React. In these components, form data is handled by the component's state rather than the DOM. This means that every input field's value is tied directly to React state.
For example, consider a simple text input:
This level of control allows for dynamic validation and complex interactions, as the state can be modified based on the input received. Moreover, by using pure functions to dictate how state is transformed, your components become easier to test and maintain.
Validating Form Inputs
Ensuring that your forms are collecting valid and meaningful data is crucial. With uncontrolled components, validation can be more of a challenge. Controlled components allow for real-time validation to result in a smoother user experience.
Here are a few strategies for validating form inputs:
- Client-side validation: Use built-in HTML validation attributes, or leverage libraries like Formik or Yup to enforce schema-based validation. This saves server calls and improves responsiveness.
- Custom validation logic: Implement custom validation functions that check user input as they type. For instance, a password input might need to check for a minimum length.
- User feedback: Always inform users of validation errors immediately. This can be done through visual cues (like changing border colors) or through error message displays.
"A good user experience involves not just collecting data, but ensuring the data collected is accurate and complete."
By employing effective validation strategies, you position your application to collect quality data while also enhancing the overall user journey. This takes user engagement to the next level, ensuring that every interaction serves its purpose and the user remains informed throughout.
In summary, handling events and forms is undoubtedly a critical aspect of creating React applications; mastering it can significantly elevate how users interact with your application, making it an essential skill for developers.
Routing in React Applications
When creating a web application with React, routing often becomes a backbone feature. As applications grow in size and complexity, the need for seamless navigation cannot be overstated. The concept of routing allows developers to render different components based on the URL the user is navigating to, making it easy to build single-page applications (SPAs) that respond swiftly to user interactions.
Incorporating a router is vital for various reasons. First and foremost, it enhances user experience by enabling quick transitions between views without rerendering the entire application. This results in a more fluid experience reminiscent of desktop applications. Moreover, routing helps to maintain application scalability. With well-structured routes, expanding an application becomes simpler since each section can be modularized effectively.
However, amidst the benefits, proper consideration must be given to routing strategies. Improper setup can lead to bad routing practices that can confuse users or compromise the applicationâs performance. Hence, understanding routing is not just an option; itâs essential for anyone looking to develop robust React applications.
"In the world of web development, routes form the very fabric that ties different components together, enabling a harmonious user experience."
Preface to React Router
React Router is the go-to library for handling routing in React applications. Its design allows components to render conditionally based on the path specified in the URL. This provides significant flexibility, especially for building applications where users expect dynamic changes in views based on their interactions.
One key aspect of React Router is its declarative approach. This means that developers define their routes using a clear syntax, making the router easy to use and configure. The core component of React Router revolves around the and components, which provide a structure in which different components can be rendered for specified paths.
Creating Routes for Components
Setting up routes for various components in your React application is a straightforward yet crucial aspect of routing. With React Router in place, developers can define specific routes for different application views. Hereâs a basic example illustrating how to create routes:
In this snippet, the component is integral, as it ensures that only the first that matches the URL is rendered, preventing multiple components from being displayed simultaneously.
Linking and Navigation
Links in a React application are managed through the component, which allows navigation between different routes effortlessly. This standard method avoids the page refresh that occurs in traditional web applications. Hereâs how you can implement a simple navigation bar using :
In creating intuitive link structures, developers should aim for clarity. Descriptive link texts help users understand where they are heading, thus improving overall usability.
In summary, mastering routing in React applications forms a critical skill set. With tools like React Router, developers can enhance navigational fluidity while ensuring that user experiences are sharp and coherent.
State Management Libraries
State management is a crucial aspect of building robust React applications. As applications grow in complexity, managing the state effectively becomes vital. State management libraries provide tools that simplify and organize state handling, ensuring that components reflect current data and user interactions seamlessly. The right state management solution is not just about simplifying your workload; it can greatly enhance performance and maintainability.
Intro to Redux
Redux is arguably the most popular state management library in the React ecosystem. Its design revolves around a central store that holds the entire application state, with components subscribing to changes in that state. This unidirectional data flow means that you have predictability in how data and UI update, reducing potential bugs.
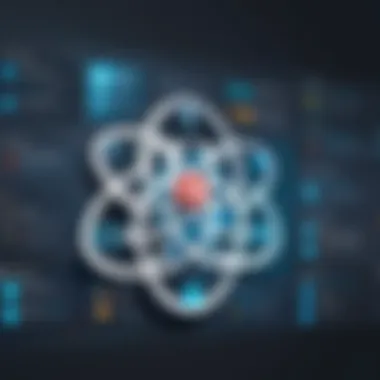
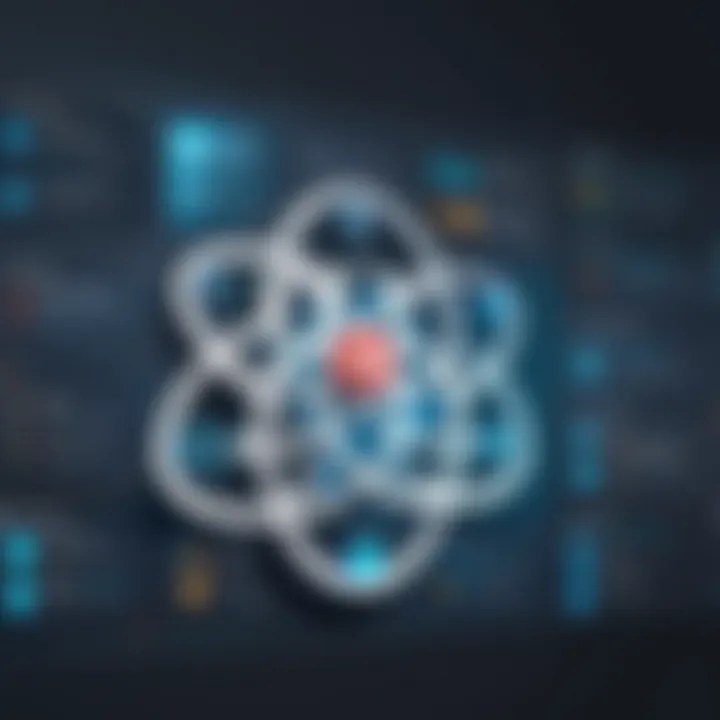
One of its key traits is that it promotes immutability, meaning that instead of modifying the existing state, new state objects are created. This ensures a clear record of state changes, which can be important for debugging and tracking user actions. Moreover, Redux has a strong community and ecosystem, offering middleware like Redux Thunk and Redux Saga, which can be used to handle asynchronous operations efficiently.
Setting Up Redux in a React App
To get Redux rolling in a React application, the setup process is relatively straightforward. Here is a high-level view of the steps:
- Install Redux and React-Redux: You can easily add them to your project via npm:
- Create the Store: In your main application file, you will create the Redux store.
- Provide the Store: Use the component to pass the store to your React application.
- Create Reducers and Actions: Define how the state updates in response to actionsâthis is where the business logic lies.
Through these steps, your React app can leverage the full power of Redux to manage its state effectively, making your components cleaner and more focused on presentation.
Alternative State Management Solutions
While Redux shines brightly, it's not the sole option available for managing state in React applications. There are other libraries worth considering, each offering unique advantages.
Context API
The Context API is built into React, allowing state to be shared directly between components without the need for intermediate components. Itâs particularly useful for passing data deep down the component tree, avoiding prop drilling. One of its significant characteristics is simplicity. You can define a context and provide it via a provider component which allows your components to consume it as needed.
- Benefits:
- Unique Feature: Being built into React means thereâs less overhead in terms of additional packages, making it a quick option for smaller applications or features.
- Considerations:
- Reduces the complexity of your code when managing simple state.
- Fewer dependencies compared to others like Redux.
- As the app scales, managing multiple contexts may lead to performance issues or complicated state management scenarios when compared to Redux.
MobX
MobX is another alternative that offers a different approach to state management. It utilizes observable states and eliminates the boilerplate code required for managing state updates. The key characteristic of MobX is its reactivity; any changes in the state trigger updates in the components that depend on that state automatically. This can lead to a more intuitive development experience.
- Benefits:
- Unique Feature: The use of decorators and powerful observable patterns makes it easy to express state relationships and reactivity.
- Considerations:
- Less code is needed, as it can keep your components clean and more focused on their specific role.
- MobX can feel like it hides a lot of complexity, which may be a disadvantage for those who prefer a more predictable flow of data, like what Redux offers.
Testing React Applications
Testing an application is like putting the product through its paces before it hits the shelf. It's about making sure that everything works as it should and that the users have a smooth experience while using your application. Now, in the realm of React JS, testing isnât just a luxury; it's a necessity. When creating a React application, the dynamic nature of components means that we're constantly changing code, refactoring, and adding features. That's where testing becomes crucial. It allows developers to ensure that existing features work as intended, even as the application evolves.
A solid testing framework can save countless hours in manual testing. Automated tests enable developers to quickly catch bugs, reducing the time and effort spent troubleshooting issues later. Moreover, with a well-tested application, you can confidently roll out updates and features, knowing that you have safeguards in place to catch potential regressions.
Importance of Testing in Development
Incorporating testing into development practices leads to numerous benefits:
- Bug Detection: It's much easier to find and fix bugs during development than after deployment. Testing helps identify issues early.
- Refactoring Confidence: With tests in place, developers can refactor code and ensure that existing functionality remains intact.
- Improved Code Quality: Writing tests often leads developers to think more carefully about their code's design, encouraging better practices.
- Documentation: Tests can serve as a form of documentation. They explain how components should behave, making it easier for new team members to understand the codebase.
Thus, it's evident that testing is not just a checkbox to tick off; it's an integral part of the development lifecycle.
Testing Tools and Frameworks
Choosing the right tools and frameworks for testing is equally as important as the act of testing itself. In the React ecosystem, two popular choices are Jest and React Testing Library. Each has its own strengths and weaknesses.
Jest
Jest is a testing framework developed by Facebook, tailored specifically for JavaScript. Its primary offering is simplicity, making it ideal for both newcomers and seasoned developers. One significant aspect of Jest is its built-in assertion library and its support for mocking functions and modules. This can drastically minimize the boilerplate code developers have to write, allowing them to focus on what matters.
Key Characteristics:
- Snapshot Testing: Jest provides powerful snapshot testing capabilities. This means you can compare the rendered output of a component against a saved snapshot to check for changes.
- Great Performance: It runs tests in parallel, so even larger test suites run quickly.
Advantages:
- Very easy to set up and get running.
- Offers a range of built-in functionalities that streamline the testing process.
Disadvantages:
- While it's powerful, it might be an overkill for simpler projects, leading to unnecessary complexity.
React Testing Library
React Testing Library focuses on the way users interact with the application rather than the implementation details. It promotes writing tests in a way that reflects how the application will be used.
Key Characteristics:
- User-Centric Approach: It encourages testing of components by simulating user behavior, leading to more relevant tests.
- Lightweight: The library is remarkably lightweight and integrates seamlessly with Jest.
Advantages:
- Produces tests that are less susceptible to breaking due to implementation changes, enhancing maintenance.
Disadvantages:
- Developers may need to have a solid grasp of user interactions to effectively use the library.
Writing Effective Test Cases
Creating effective test cases is paramount for ensuring the reliability of your application. The tests should be clear, purposeful, and cover various scenarios, particularly edge cases. Here are some tips for writing effective test cases:
- Be Specific: Clearly define what each test is supposed to achieve. Avoid ambiguous descriptions that leave room for misinterpretations.
- Keep it Simple: Each test should ideally cover just one aspect of the functionality. This makes it easier to identify what went wrong when tests fail.
- Include Edge Cases: Don't just test the happy path; consider scenarios that might break your application.
- Regularly Update Tests: As your application evolves, update your tests accordingly to ensure they remain relevant and useful.
In summary, testing React applications is not just a routine task; itâs an essential practice that can lead to significant quality improvements and developer confidence. The right tools and well-crafted test cases can position your project for success.
Optimizing React Applications
Optimizing a React application is paramount for enhancing user experience and ensuring that web applications run smoothly. When applications lag or are sluggish, users might bounce away faster than a rabbit on the run. This underscores the importance of paying attention to performance right from the initiation of the development process. A well-optimized application can significantly improve loading times, responsiveness, and overall efficiency, making it vital for developers to understand the best practices surrounding this topic.
Performance Best Practices
Ensuring high performance in any React application requires a strategic approach. Here are some key practices that developers should keep in mind:
- Keep Components Small and Focused: When crafting components, aim for small, reusable ones. Not only does this make maintenance easier, but it also helps in enhancing performance due to better rendering efficiency.
- Use Functional Components: Familiarize yourself with functional components and React hooks, as they are generally more performant than class components, especially in modern development.
- Avoid Anonymous Functions in Render: Declaring functions directly in the render method leads to the creation of a new function on every render cycle, which can slow the app down. Instead, define functions outside the render method.
- Optimize Image Sizes: The size of images can slow down an application drastically. Therefore, utilize modern formats like WebP, and consider tools to compress images without losing quality.
By keeping these strategies in mind, developers can minimize render times and ensure that applications are not only effective but also efficient.
Code Splitting and Lazy Loading
A well-timed approach to enhancing performance is to employ code splitting and lazy loading. These techniques limit the amount of code that a user needs to download initially, presenting only what is necessary for the current view. This is crucial because users tend to expect web applications to load instantaneouslly.
- Code Splitting: Code splitting can be achieved by using tools such as Webpack, which allows for breaking the code into smaller chunks. This means that instead of loading the entire application at once, only the necessary parts are loaded on demand. The benefits include faster load times and reduced initial payloads.
- Lazy Loading: Implementing lazy loading can lead to significant improvements in performance. This technique loads components only when they become visible on the screen. For instance, a photo gallery might load images only when the user scrolls to that section, saving resources. You can utilize dynamic statements in React for this.
Example of Dynamic Import
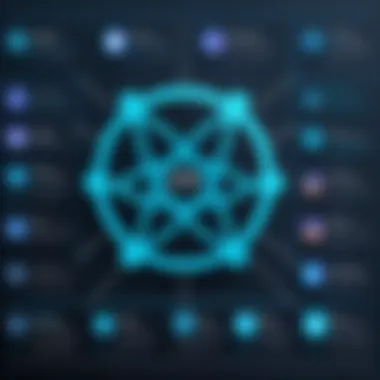
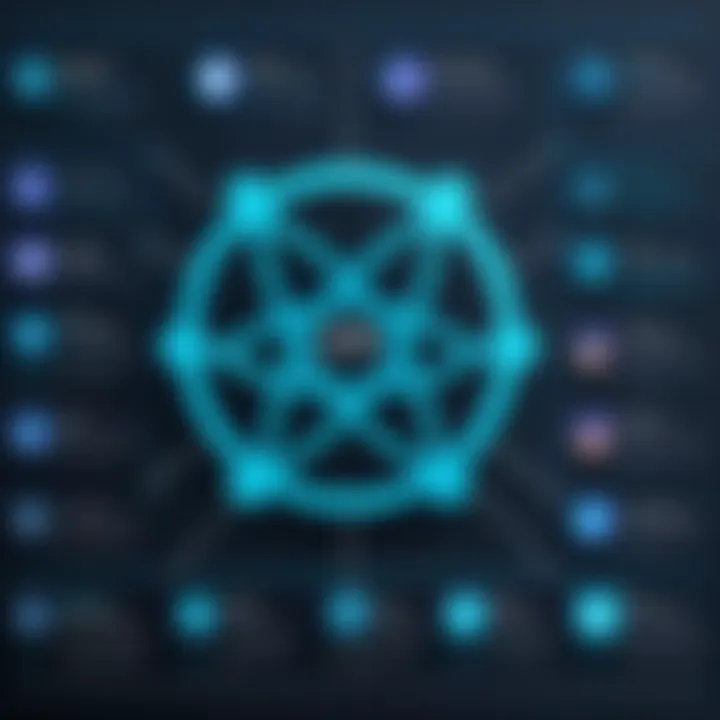
By applying these practices, developers can enhance user experience, especially in applications that house extensive data.
Using React.memo and useMemo
React offers powerful features like and to further fine-tune performance.
- React.memo: This higher-order component can help in memoizing functional components, allowing React to skip rendering those components when their props havenât changed. This can lead to performance boosts for complex components that render often.
- useMemo: This hook helps in storing the results of expensive calculations and recomputes them only when necessary, preventing unnecessary re-renders. Itâs particularly useful when working with large datasets or complex computations that donât change frequently.
An important takeaway here is to apply these tools judiciously. Overuse can lead to unintended consequences, such as increased complexity.
"The greatest performance boost comes from doing less work in the first place."
In a nutshell, optimizing a React application involves a mix of strategy, foresight, and the application of specific techniques that contribute to a smooth user experience. From adhering to performance best practices to leveraging Reactâs built-in optimizations, there's a myriad of approaches to achieve your goal of a responsive application. By following these guidelines, developers can effectively optimize not just the performance but the satisfaction of users interacting with their applications.
Deploying React Applications
Deploying a React application is like setting the stage for a show; it's the final step of your hard work and creativity where all your coding expertise shines. This topic matters a lot in this guide because getting your app live is important for showcasing your skills and enabling real users to interact with your creation. Without proper deployment, even the most brilliant piece of code remains locked away on your computer.
When you deploy, you open the doors to the world. Think about it: after hours of coding, networking components, handling state, and debugging, the last piece is to ensure the app runs smoothly in a production environment. The deployment process not only helps in presenting your work, but it also gives you a sense of accomplishment. Beyond that, it teaches the basics of operational needs like server management, response times, and efficiencyâall essential in todayâs coding landscape.
Preparing for Deployment
Before you step into the deployment arena, it's wise to prepare your app. Just like packing a suitcase for a long trip, proper prep will save you a headache later on. Hereâs what to consider:
- Optimize Your Build: Use the production build with to minimize file size and improve load times.
- Clean Up the Code: Eliminate any unused components, and check your dependencies. Itâs best not to leave any loose ends before launching your app.
- Test Thoroughly: Go through all functionalities to make sure everything works just like a well-oiled machine. No one likes bugs when the curtain goes up!
After you have crossed these checkpoints, you will be more confident to take the final leap into deployment.
Deploying to Netlify and Vercel
Netlify and Vercel are two of the most popular choices for deploying a React app. They are user-friendly and connect smoothly to your Git repositories, making them a breeze to work with.
Steps to Deploy on Netlify:
- Sign up for a Netlify account and link it with your GitHub or GitLab repository.
- Choose your repository where your React app is stored.
- When prompted, select the build settings. You typically want to set the build command to and the publish directory to .
- Finally, click on Deploy Site.
Netlify takes care of everything mostly automatically, letting you focus more on coding and less on configuration.
Steps to Deploy on Vercel:
- Create an account on Vercel and follow the same linking process to your Git repository.
- Once linked, Vercel will automatically detect that you're using React and pre-fill the necessary settings for you.
- Hit Deploy, and voilĂ ! Your app is live.
Both options come with automatic deployments which means every time you push code to your repository, they'll redeploy your site automatically. How convenient is that?
"Deployment is not just a technical act; it's the bridge connecting developers to users."
Using Docker for Deployment
Docker provides another powerful way to deploy your React applications. If youâve ever wrestled with environment setups, you know how tricky it can get to ensure consistency across different machines. Docker takes care of this by encapsulating everything needed to run your app in containers, making deployment more predictable.
Steps to Deploy Using Docker:
- Install Docker: Make sure youâve got Docker set on your machine.
- Create a Dockerfile: This file tells Docker how to set up your environment and run your app. A sample Dockerfile for a React app might look like this:
- Build the Image: Run in your terminal.
- Run the Container: Use to start your app.
Docker might sound complex at first, but once you get comfortable with it, youâll appreciate the control and efficiency it adds. Plus, it makes your team collaboration a whole lot easier.
Building a Sample React Application
Creating a sample React application is a cornerstone of understanding how React operates in practical scenarios. This section holds significant importance because it bridges the gap between theoretical knowledge and real-world application. Often, the leap from learning core concepts to building an actual app can feel like diving into deep waters without a life vest. By working through a hands-on example, readers can solidify their grasp of React's features while identifying how various components interact.
Defining Application Requirements
Before starting any project, it is crucial to lay down a solid foundation. This is akin to drafting a blueprint before construction. In the context of building a React application, defining requirements involves several key elements:
- Understanding the Audience: Who will use the application? Tailoring design and functionality to user needs enhances engagement.
- Setting Functional Goals: Outline what the application should do. Will it manage tasks? Display user data? Establishing these goals guides development.
- Identifying Technical Specifications: Consider which technologies will be involved. React is often paired with tools like Redux for state management or Axios for API calls.
- Performance Expectations: Establish what is deemed acceptable in terms of load times and responsiveness.
With these requirements in place, developers can move into the realm of implementation with clarity and purpose.
Implementing Core Features
With a well-defined roadmap, it's time to dive into coding. This phase focuses on translating theoretical concepts into functional components. Some core features to implement could include:
- User Authentication: Allowing users to log in securely can involve integrating libraries like Firebase Authentication or building a custom solution using JWT.
- Displaying Data: Utilizing the React component model, developers can create dynamic interfaces that pull data, perhaps displaying user profiles or lists of items from an API.
- Interactive Elements: Incorporate forms and buttons that respond to user actions. Here, state management plays a pivotal role; components must reflect changes as users interact.
\ Example Code Snippet:
\
Building out features layer by layer illustrates how various pieces connect and work together in a cohesive manner. Each feature then becomes part of a larger narrative that adds depth to the application.
Finalizing and Testing the Application
Once the core features are implemented, itâs time to ensure everything runs smoothly. This stage can reveal bugs or inconsistencies that were not immediately obvious. Finalizing the application also prepares it for release. Key considerations include:
- Testing: Implement unit tests and integration tests to validate functionality. Tools like Jest and React Testing Library can prove invaluable here.
- Refining User Experience: Gather feedback from potential users or peers, making iterative improvements based on their insights.
- Optimization: Review performance metrics to ensure the application meets expectations. This might include code splitting or tree shaking to reduce bundle size.
- Deployment Preparation: Make sure the app is ready for the outside world. This involves checking configuration settings, API connectivity, and cross-browser functionality.
"It's not just about building code; it's about creating an experience that resonates with users."
Future Trends in React Development
As the landscape of web development continues to evolve, keeping an eye on the horizon is imperative for developers who want to stay ahead of the curve. Future trends in React are not just speculative; they represent the direction in which the framework and its ecosystem are headed. With advancements in technology and shifting user expectations, understanding these trends can significantly impact the way applications are built. The following discussion highlights the specific elements, benefits, and considerations surrounding these trends, showcasing their relevance to both new and seasoned developers.
React Server Components
React Server Components are turning heads in the development community. This innovative feature aims to improve performance by allowing developers to render components on the server instead of relying solely on the client-side. It merges the advantages of server-side rendering (SSR) with the React paradigm, ensuring faster load times and better SEO performance.
- Benefits:
- Reduced time to interactive, leading to happier users.
- The server can manage heavier data operations, thus offloading the client.
- Seamless integration with existing React components.
"The introduction of Server Components is a game changer, altering how we approach the rendering logic â less weight on the client means more efficient applications."
While it sounds enticing, developers should consider the complexities introduced with server-client data management and how to effectively structure applications to leverage this feature properly.
The Role of TypeScript in React
The emergence of TypeScript has unlocked new potential within Reactâs ecosystem. Many developers now ponder whether going for TypeScript over plain JavaScript is worth the transition. The ability to add static typing to JavaScript can reduce runtime errors and enhance overall code quality.
- Key advantages:Adopting TypeScript can indeed be a step up for teams wanting to embrace strong typing and structure, especially in large applications where multiple developers contribute.
- Improved maintainability through clear type definitions.
- Enhanced code editor support, offering autocompletion and refactoring tools.
- Early detection of bugs during development rather than after deployment.
Anticipated Changes in the Ecosystem
As dynamic as React itself, the ecosystem surrounding it is continuously shifting. Several anticipated changes are underway, and understanding them is crucial for developers striving to keep their skills and applications relevant.
- Updates to Third-Party Libraries: Popular libraries such as Redux and React Router are likely to evolve, aligning more closely with React's core features and simplifying state management.
- Adoption of React Query: This emerging library is making waves as it streamlines data-fetching in React applications, offering developers a more efficient way to handle server state.
- Functional and Declarative Approaches: As functional programming gains traction, embracing hooks and context API will likely become the norm, steering developers away from traditional, class-based components.
These changes hint at a movement toward a more cohesive, efficient, and developer-friendly environment that aligns well with the fast pace of technological advancements.
In summary, recognizing and adapting to these future trends in React is not merely a preference for developers but a necessity. The integration of Server Components, TypeScriptâs robust features, and the evolving ecosystem will shape the future of React applications, presenting opportunities and challenges alike.