Creating a Python Chatbot: A Complete Guide for Developers
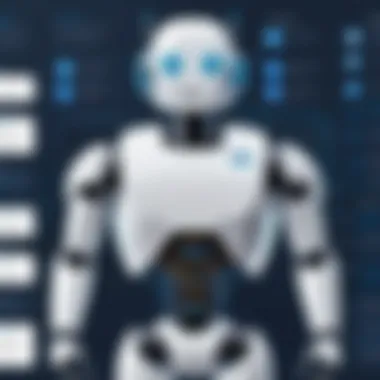
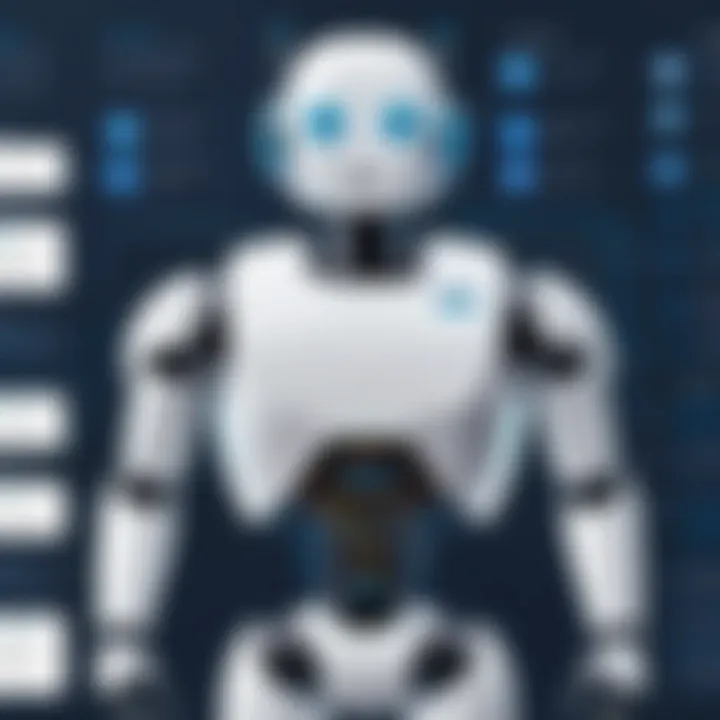
Intro
Building chatbots has become a hot topic, as businesses and developers explore ways to bring automated assistance into their operations. As those with a knack for programming delve into this field, it becomes essential to understand the landscape of the programming language being used—in this case, Python. This guide intends to demystify the chatbot creation process while shedding light on Python’s capabilities and features that make it a prime choice for both novices and seasoned developers.
The discussion will navigate through the foundational aspects of Python, explore its syntax and data structures, and dive into more advanced programming concepts that are crucial for building sophisticated chatbots. By understanding these sectors, readers will grasp not just the how, but also the why behind each step taken in this learning journey.
Prelims to Programming Language
History and Background
Python made its debut in the late 1980s, crafted by Guido van Rossum. Initially intended as a successor to the ABC programming language, its design philosophy prioritizes code readability and simplicity. Although it felt like a small fish in a big pond at first, Python's growth has been meteoric. From web development to data science, its applicability has broadened tremendously over the years.
Features and Uses
Python shines bright in several areas:
- Simplicity: Python code is straightforward, resembling plain English. This accessibility makes it a darling among beginners.
- Rich Libraries: Being equipped with a plethora of libraries like NLTK, TensorFlow, and Flask, Python adapts effortlessly to different programming needs.
- Versatility: Whether it’s creating web applications or performing data analysis, Python can be the Swiss Army knife for developers.
Popularity and Scope
Python’s popularity is evident across various platforms. It stands tall in forums, communities, and educational resources. The TIOBE Index often ranks Python as one of the top programming languages, showcasing its momentum and sustained relevance in an ever-changing tech landscape.
"In programming, as in life, simplicity is the ultimate sophistication."
This quote rings true for those stepping into the realm of chatbot development using Python, as clarity often leads to better understanding and implementation.
Basic Syntax and Concepts
Variables and Data Types
Understanding how to manage data is the linchpin of programming. In Python, data is categorized mainly in ways: integers, floats, strings, and booleans. Variables serve as containers for these data types. For example, you might have:
This snippet demonstrates how to define variables in a clean manner.
Operators and Expressions
Operators in Python include arithmetic, comparison, and logical operators. They are the tools for manipulating data. For instance, if one were to assess whether a chatbot's reply count exceeds a certain threshold, they would use comparison operators, making use of expressions to drive decisions:
Control Structures
Control structures, such as loops and conditional statements, guide the flow of programs. These constructs allow the code to make decisions or repeat actions, forming the backbone of functional programming:
Advanced Topics
Functions and Methods
Functions allow for modular programming. They are like tools in a toolkit, used when needed. A simple function example is:
This function can be called whenever a greeting is needed, promoting reuse and clarity.
Object-Oriented Programming
For more complex systems, understanding object-oriented programming can be beneficial. It helps manage large codebases more effectively through encapsulation and by creating objects, which can represent real-world entities. For instance, a chatbot can be modeled as a class in Python:
Exception Handling
When developing, things don’t always go as planned. Exception handling comes into play to manage errors and exceptions gracefully. In Python, you might use:
Hands-On Examples
To solidify knowledge, practical examples are crucial. Crafting simple programs as a starting point builds confidence. Start small with a chatbot that responds to basic queries. As skills sharpen, intermediate projects can emerge, such as integrating with web frameworks like Flask for a full-fledged application.
Simple Programs
A straightforward echo chatbot could look like this:
This simple bot echoes back the user’s messages, demonstrating fundamental interaction.
Intermediate Projects
Consider enhancing your chatbot by integrating it with a natural language processing library. This provides richer interactions, as the bot learns to understand user inputs more deeply.
Code Snippets
As development progresses, it’s helpful to share snippets to foster learning:
Resources and Further Learning
To keep expanding your knowledge, various resources can help:
- Recommended Books: Automate the Boring Stuff with Python provides practical insights for beginners.
- Online Courses: Platforms like Coursera and Udemy offer structured learning paths.
- Community Forums: Engaging with others on Reddit can offer different perspectives as you troubleshoot and share your journey.
Exploring these will further solidify your knowledge and keep you plugged into the vibrant Python community.
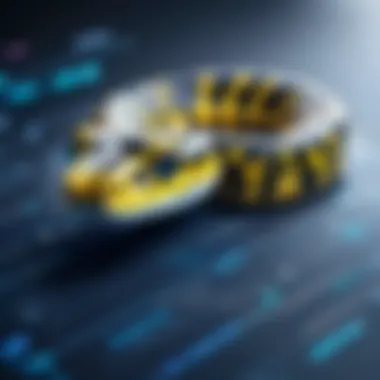
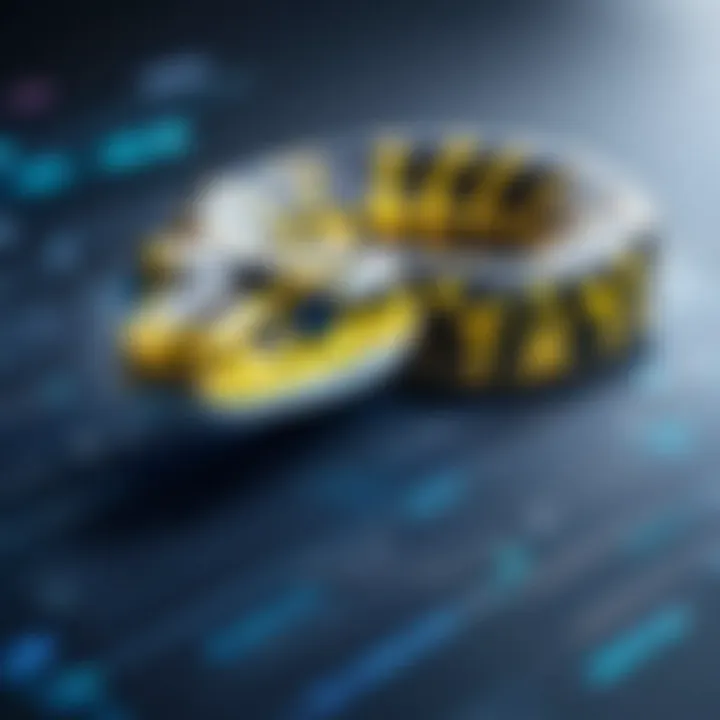
Prologue to Chatbots
In our digital age, chatbots have become a pivotal tool in facilitating communication across various platforms. Understanding chatbots is not just about knowing how they function; it's about grasping their role in modern interactions, both between humans and systems, and among individuals. This section will elucidate the core definition of chatbots and delve into their evolution, shedding light on why they hold such significance today.
Definition and Purpose
Chatbots can be succinctly defined as software applications designed to conduct conversations with users through auditory or text-based methods. They operate via rules or artificial intelligence, allowing them to engage users in a natural language manner.
Key purposes of chatbots include:
- Customer support: Addressing user queries and offering solutions around the clock, thus enhancing customer experience.
- Information retrieval: Providing quick access to data, be it for weather forecasts or product details, with minimal input from the user.
- Task automation: Streamlining routine processes, allowing users to perform functions like booking appointments without human intervention.
These functionalities underline the versatility of chatbots in various sectors — from e-commerce to healthcare — making them an invaluable asset in today’s fast-paced environment.
Evolution of Chatbots
The journey of chatbots has seen a remarkable transformation since their inception. Initially, programs like ELIZA in the 1960s were rudimentary, relying heavily on pattern matching to create the illusion of conversation. Although limited, ELIZA set the foundation for future advancements.
By the 1990s, with burgeoning advancements in technology, more sophisticated chatbots began to emerge, capable of performing specific tasks like booking flights through limited scripts. Fast forward to the 21st century, we've witnessed the advent of AI-driven frameworks, yielding chatbots that learn from past interactions.
This evolution has led to:
- Enhanced understanding of natural language: Thanks to algorithms that can effectively parse and understand user intent.
- Integration with advanced technologies: From machine learning to deep learning, which empowers chatbots to provide tailored responses.
- Increased accessibility: Chatbots are now present on various platforms—social media, websites, and apps—making them more reachable to users.
The continuous evolution of chatbots not only showcases technological advancements but also reflects changing user expectations. As we dive deeper into the world of chatbot development, recognizing their foundational aspects guides us in their creation, ensuring they meet the demands of a dynamic digital landscape.
Why Choose Python for Chatbot Development
The selection of a programming language for chatbot development can dictate the ease or complexity of the project. Python stands out as a top choice, and understanding its benefits is crucial for those diving into this field. Not only does Python boast a simple and clean syntax, which can be a breath of fresh air for beginners, but it also presents a myriad of advantages that makes it enticing for developers at any skill level.
Popularity and Community Support
One cannot understate the impact of community support when embarking on a software development journey. Python has an enormous and vibrant community. This means that as a developer, you're not climbing a mountain alone; you're surrounded by a bustling bazaar of knowledge and experience.
- Rich resources: From tutorials to forums, there's a treasure trove of information available. Websites like Reddit and Stack Overflow have dedicated sections for Python enthusiasts. Here, developers from all skill levels share tips, best practices, and solutions to common problems.
- Frequent updates and libraries: Because of its popularity, Python is updated frequently. New libraries and frameworks pop up regularly, giving developers the ability to tap into cutting-edge technology without starting from scratch. For instance, if you wish to create a simpler bot, libraries like ChatterBot or Rasa can streamline the process. Whether you're troubleshooting an issue or looking for a better way to accomplish a task, chances are someone else has run into the same problem and has crafted a solution which is freely available.
In this interconnected realm, support is fundamental. Whether it’s a new developer puzzled by a syntax error or a seasoned programmer wanting to optimize their chatbot's capabilities, the community stands ready. Support systems like these can often make or break a project; with Python, you have a proven safety net.
Rich Libraries and Frameworks
When it comes to crafting a chatbot, functionality is paramount. Having access to robust libraries and frameworks can cut down on tedious coding and allow developers to focus on what truly matters: the user experience.
- Diverse options: Python offers an abundance of libraries designed specifically for natural language processing and machine learning. For example, if you're looking to perform tokenization, stemming, and lemmatization, the NLTK library can be your best friend. If you require more advanced capabilities, spaCy provides powerful tools tailored for commercial applications.
- Framework efficiency: Frameworks like Flask provide simplified routes for building web applications, including chatbots. They enable developers to set up servers and manage user requests with minimal fuss. This means you can spend less time on boilerplate code and more time fleshing out the unique aspects of your bot.
Just think about it in practical terms: having a solid framework and useful libraries is like having a toolbox with all the right instruments. Instead of crafting each tool from raw materials, you can pick the right tool for the job, simplifying the entire development process.
In summary, choosing Python for chatbot development is not just a matter of preference; it opens doors to a world of resources and support. As you embark on your coding journey, remember: you're stepping onto a path paved with experience, tools, and a community ready to assist. The programming landscape is vast, but with Python, you’ll find it's much easier to navigate.
Necessary Tools and Frameworks
To build a chatbot successfully, choosing the right tools and frameworks is absolutely crucial. These elements form the backbone of your bot, guiding its development and ensuring it meets the intended functional requirements. The right framework can smooth out the bumps in the development process and make your experience far more enjoyable—and effective. It’s like picking the right ingredients before you start cooking; you wouldn’t want to add salt instead of sugar, would you? In this section, we’ll dive into the key frameworks available for Python, along with considerations on how to set up your environment to get started.
Choosing the Right Framework
Navigating through the myriad of frameworks can feel like finding a needle in a haystack—complicated and daunting. The aim here is to simplify that choice. Each framework has its strengths and weaknesses, and the best choice often depends on your specific use case. Let’s take a closer look at three prominent frameworks.
ChatterBot
ChatterBot stands out as a popular choice among beginners, mainly due to its simplicity. It's designed to respond to user input in a conversational way, making it an excellent starting point if you’re new to chatbot development.
One key characteristic of ChatterBot is its ability to learn from interactions. This means it gets smarter as it converses, which can be invaluable for tasks that require adaptation over time.
However, it’s not without its disadvantages. ChatterBot can sometimes produce responses that are a bit off the mark, especially if it hasn’t been trained well. This can lead to some awkward interactions, so it’s essential to keep that in mind as you develop your chatbot. Its learning feature is both a blessing and a curse—while it becomes more adept, it also needs a substantial amount of data to do so effectively.
Rasa
Moving onto Rasa, this framework is a more robust option geared towards complex environments where dialogue management is key. Rasa leverages machine learning for natural language understanding, providing more control for developers.
The standout trait of Rasa is its contextual understanding across conversations; it doesn’t just react—it can remember past interactions, which is invaluable for creating a human-like dialogue. This makes it a preferred choice for businesses needing chatbots that handle intricate queries.
That said, Rasa requires a bit more of a learning curve to configure and may feel overwhelming for a novice programmer. The trade-off is significant; it’s suited for projects that require heavy lifting and deep customization.
Flask
Flask, while not a chatbot-specific framework, can help you create an entire web application to host your chatbot. It’s lightweight and flexible, making it a good fit if you want a tailor-made solution.
Flask's key feature is its simplicity and minimalism. This means you only include the essential features you need and avoid unnecessary bloat. For developers already familiar with web frameworks, Flask offers a relatively easy path toward integrating chatbot capabilities into existing web applications.
On the flip side, going the Flask route means you’ll have to manage more aspects of the application yourself, such as database integration and user authentication, which adds complexity to the project. If you’re not cautious, those details can easily pile up and become overwhelming.
Python Environment Setup
Before diving headfirst into chatbot coding, setting up your Python environment is a prerequisite. Having the right environment eases your development journey and keeps efforts organized.
To set up your Python environment:
- Install Python: Make sure you have the latest version of Python installed. Package managers like Anaconda can simplify this process.
- Create a Virtual Environment: Use libraries like or to isolate your project’s dependencies. This way, different projects won’t interfere with one another’s libraries.
- Install Necessary Libraries: Once your environment is established, install frameworks like ChatterBot, Rasa, or Flask depending on your choice using pip.
- Test the Setup: A quick test run will confirm if everything’s working correctly before you jump into chatbot development.
By ensuring the right tools and environment are in place, you’ll be setting yourself up for success, allowing you to focus more on crafting your chatbot instead of wrestling with setups and configurations.
Understanding Natural Language Processing
Natural Language Processing (NLP) forms the backbone of any effective chatbot. It allows the chatbot to interpret, understand, and generate human language, which is crucial for engaging in real conversations. In the context of building a chatbot with Python, mastering NLP lies at the heart of creating meaningful interactions and improving user experiences.
Prologue to NLP
NLP is the part of artificial intelligence that teaches machines to understand and respond to language that humans speak. The significance of this technology in chatbot development cannot be overstated. For instance, without NLP, a chatbot would merely follow rigid scripts, unable to adapt to the varied ways users might phrase their queries. By employing NLP, the chatbot can also achieve better accuracy in grasping the intent behind the user's words, leading to more relevant responses. Over the past few years, advancements in NLP have made it much easier for developers to integrate sophisticated language processing capabilities into their chatbots. This results in a more natural dialogue flow, mimicking human conversation.
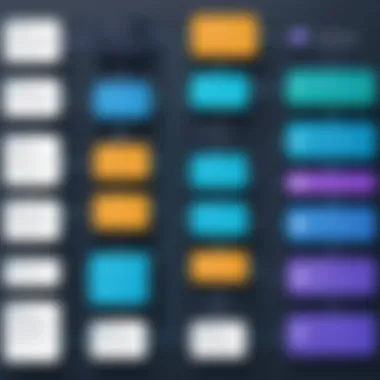
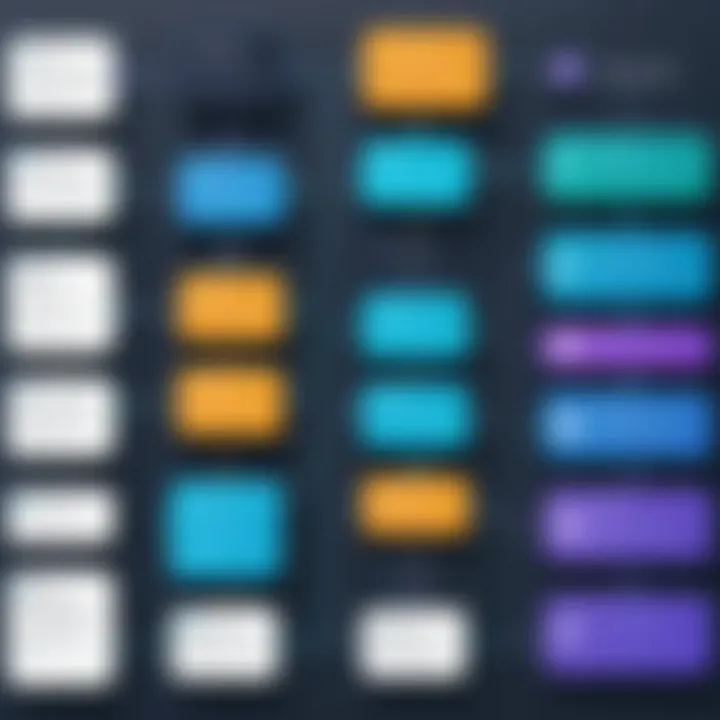
Libraries for NLP in Python
When developing a chatbot in Python, leveraging the right libraries can make a world of difference. Here, we will discuss two prominent libraries: NLTK and spaCy. Choosing the right one can affect the ease of implementing language processing capabilities into your chatbot.
NLTK
The Natural Language Toolkit, commonly known as NLTK, is a leading library for NLP tasks in Python. One of its strongest characteristics is versatility. NLTK supports a wide suite of text processing libraries for classification, tokenization, stemming, tagging, parsing, and more. A major advantage of NLTK is the plethora of tutorials and documentation available, which makes it an excellent choice for beginners navigating the world of NLP.
However, its unique feature—the rich set of algorithms—can be both a blessing and a curse. While it offers flexibility and a depth of functions, the trade-off can be complexity and slower performance compared to newer libraries. For a beginner, this complexity can lead to frustration if not handled with care.
spaCy
On the other hand, spaCy is lauded for its performance and efficiency in processing large amounts of text quickly. It focuses on practical functionality without the overhead of additional features that less advanced users may not need. One key characteristic of spaCy is its ability to provide state-of-the-art accuracy with its pre-trained models, making it a beneficial choice for students and developers looking to harness powerful machine learning capabilities.
The unique aspect of spaCy is its seamless integration with machine learning frameworks like TensorFlow and PyTorch. This can be quite useful for those who wish to implement NLP in more sophisticated ways. However, a downside is that spaCy may not be as user-friendly for total newcomers to the field of NLP, as the documentation might assume some prior understanding.
"Understanding and leveraging the right tools in NLP is essential for creating chatbots that can actually converse."
Designing the Chatbot
Designing a chatbot is akin to laying down the foundation of a house. Without a solid blueprint, the entire structure might come crumbling down. In the realm of chatbot development, the design phase plays a crucial role in shaping user experiences and determining the effectiveness of interactions. It’s not just about coding; it’s about understanding who your users are, what they need, and how best to deliver that information in a seamless manner.
When diving into design considerations, one can’t ignore the significance of user scenarios. These scenarios outline the different ways users might interact with the bot, providing context and clarity for developers. The more detailed your user scenarios are, the more intuitive your chatbot will be. Moreover, establishing a well-thought-out conversation flow ensures users have smooth exchanges without disorientation.
Defining User Scenarios
User scenarios serve as relatable stories that encapsulate potential interactions with your chatbot. Imagine a simple coffee shop chatbot. One scenario might depict a customer looking to place an order and another where they are inquiring about the shop's locality. These scenarios guide developers in understanding user intent and help map out functionalities tailored to specific needs.
When crafting these scenarios, it’s essential to include key elements such as:
- User profile: Who is the user? Are they regulars or first-timers?
- Goals and motivations: What does the user wish to achieve? Is it ordering coffee or finding store hours?
- Emotional tone: How might the user feel during the interaction? Frustrated, curious, or satisfied?
A well-defined user scenario fosters empathy and helps developers anticipate user questions. This proactive approach minimizes surprises and steadily steers the chatbot towards addressing real user pain points.
Creating a Conversation Flow
Once user scenarios are cast, the next step is mapping out the conversation flow. This represents the roadmap of interactions users will navigate. Think of it as choreographing a dance where each step leads into the next. A coherent and logical flow counters confusion, ensuring users find answers effortlessly.
This often involves:
- Branching paths: Establish diverse response routes based on user queries; for instance, if a user asks about the menu, they should see relevant dishes rather than random info.
- Fallback mechanisms: Develop strategies for handling unexpected questions, making sure users don’t feel ignored. For example, if the bot gets stumped, it might say, "I’m not sure about that, but I can help with our menu or store hours!"
- Visual aids: Sometimes, a well-placed graphic or button can lead the user to denote the journey ahead; consider integrating buttons for quick actions like "Order Now" or "Menu".
In a nutshell, designing a chatbot isn't a mere technical exercise but a deeply human understanding of interactions—capturing every nuance in user experiences.
"Design is not just what it looks like and feels like. Design is how it works." - Steve Jobs
By considering user scenarios alongside conversation flows, you can create chatbots that not only respond to inquiries but also engage and satisfy user needs. This balanced approach ensures the bot becomes a valuable asset rather than just a programmed machine.
Implementing the Chatbot
In this portion of the article, we delve into the crux of creating a functioning chatbot. The implementation phase is where the theoretical concepts you’ve learned are put to the test, and the rubber meets the road, so to speak. It’s essential because a well-crafted implementation serves as the backbone of your chatbot. A successful implementation not only enhances user satisfaction but also optimizes the bot's performance and utility.
To make this phase seamless, close attention must be paid to coding the chatbot logic and integrating natural language processing. These components play a pivotal role in ensuring that the chatbot operates effectively and meets user expectations. Let’s break down these two key aspects further.
Coding the Chatbot Logic
Coding the logic of the chatbot is akin to laying a solid foundation for a building. Without robust logic, you might find your chatbot as useful as a screen door on a submarine. It’s here where you define how the bot interacts with users, processes input, and produces responses.
- Understanding User Intent: The first step in coding the chatbot's logic involves grasping user intents. When users send messages, they convey specific meanings or inquiries. Recognizing these intents accurately ensures that your chatbot engages effectively. You might consider utilizing the popular library called ChatterBot to assist with intent recognition.
- Crafting Response Algorithms: Once user intents are deciphered, the next step involves creating algorithms for responses. It’s a bit like teaching a parrot to speak; only here, you're expecting more than repeated phrases. You must design responses that are contextually relevant and informative.
- Conditional Statements: Using conditional statements is a conventional way to guide the flow of conversation. They allow you to determine what message should follow based on the user's previous messages. A novice coder might find this challenging but, breaking down the logic into smaller steps can make it manageable.
- Testing Your Logic: Lastly, you must rigorously test your logic with various scenarios. Does the chatbot respond appropriately to different user inputs? This testing phase is crucial because you'll want to catch errors before users do. An amazing tool for testing is Rasa, which provides an environment to refine the chatbot's logic iteratively.
Integrating NLP Capabilities
Once the basic logic is established, the next step is integrating natural language processing capabilities. NLP breathes life into your chatbot, enabling it to understand and respond in a way that feels natural to users.
- Leveraging Libraries: Python boasts several libraries like spaCy and NLTK that facilitate NLP. These libraries offer tools for tasks such as tokenization, stemming, and lemmatization. Understanding how to use these within your chatbot’s code can significantly enhance its conversational ability.
- Handling Variability in Language: One challenge in chatbot development is dealing with the variability of human language. People express similar ideas in diverse ways. A good chatbot design should accommodate this variability by utilizing sophisticated NLP to comprehend user inputs accurately.
- Enhancing User Experience: With proper NLP integration, your chatbot can not only provide precise answers but also engage in small talk, follow up on user queries, and lead conversations in a more human-like manner. This creates a richer user experience.
- Continuous Learning: Finally, a chatbot with integrated NLP can learn over time. As more users interact, the bot gathers valuable data that can be analyzed to refine its responses and better understand user intents.
"NLP is like a bridge connecting human language with machine logic, enabling machines to converse with us in ways that feel almost intuitive."
In summary, the implementation stage is where you will see your chatbot concept come to life. Pay meticulous attention to the logic behind conversation flows and integrate NLP capabilities to create a more interactive and engaging experience for users. Together, these elements will ensure that your chatbot is not only functional but effective and user-friendly.
Testing and Debugging
When building a chatbot, testing and debugging is not just a formality; it's the backbone of delivering a reliable user experience. A bot operates in unpredictable environments, responding to various user inputs that can range from the simple to the profoundly complex. Not having a sound testing and debugging strategy could lead to a frustrating end-user experience, possibly harming a company's reputation and user trust. You wouldn't want your chatbot to throw a tantrum when asked a straightforward question, would you?
Incorporating a systematic approach to testing during development helps identify and remedy issues before deployment. It’s about rather than the product looking good on paper, making sure it actually performs well in practice. Here are some key benefits of embracing rigorous testing and debugging practices:
- User Satisfaction: A well-tested chatbot is more intuitive and meets user expectations, leading to greater satisfaction.
- Minimized Costs: Early detection of bugs saves time and money in the long run. Fixing issues after deployment is often far more challenging.
- Increased Confidence: Knowing your bot has undergone thorough testing instills confidence in both developers and users.
Considerations around testing methods should include functional tests, performance tests, and user acceptance tests. These fundamentally ensure that the chatbot responds accurately, handles expected loads, and meets real-world user needs respectively. And while we're at it, don't forget that good documentation of the testing process also contributes immensely to your chatbot’s lifecycle.
Importance of Testing
Testing is crucial in the chatbot development lifecycle, as it ensures the bot functions smoothly under various scenarios. Imagine preparing a dish for a group of friends—each friend has distinct taste preferences, and if you skip tasting your dish beforehand, the end result may not tickle anyone’s fancy. Similarly, testing allows you to evaluate different interactions and tweak your bot accordingly.
Testing helps to identify various performance metrics such as:
- Response Time: How quickly your chatbot replies after a user query can significantly affect user satisfaction.
- Accuracy: Ensuring that your bot understands user input correctly is paramount.
- Usability: This encompasses how easy it is for users to interact with the chatbot and whether it meets their expectations.
Regular testing creates a safety net, allowing developers to spot problems early and fix them before they become full-blown issues. Plus, continuity in testing after deployment, often termed as 'regression testing,' is equally vital to ascertain new updates don't introduce unexpected bugs.
Common Errors and Fixes
In the journey of chatbot development, errors are practically guaranteed, but understanding the most common ones can save you a great deal of time and frustration. Here are some frequent culprits:
- Misinterpretation of User Input: Bots often struggle with nuanced language or unexpected phrases. This can be fixed by regularly updating the training data and refining the NLP modules to adapt to varying linguistic patterns
- Missing Context: Users may expect the bot to understand the context of ongoing conversations. To alleviate this, designing your state management logic carefully is crucial.
- Slow Response Times: If your chatbot takes too long to respond, users may abandon the interaction. Optimizing backend processes or using more efficient data structures can often resolve this.
Here’s a compact list of strategies to address these common issues:
- Continuously feed your bot new data to improve its understanding.
- Test edge cases and outlier inputs.
- Monitor performance after deployment regularly with real user interactions to gather qualitative feedback.
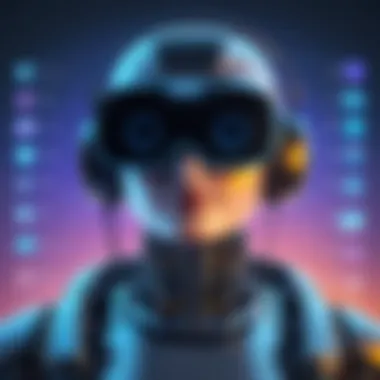
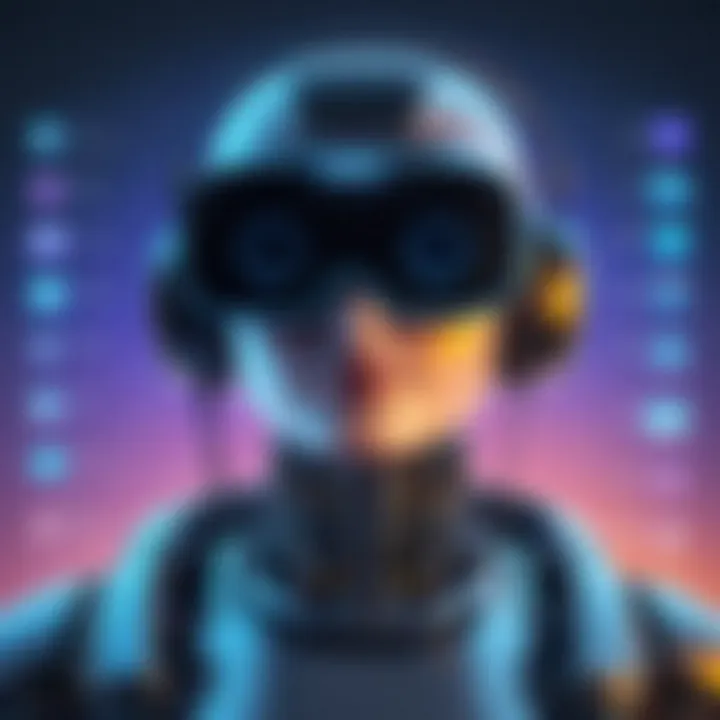
"Test early, test often, and plan for the unexpected."
Using these insights not only paves the way for smoother operations but also ensures your chatbot is a valuable tool rather than a source of frustration.
Deployment Options
When you've spent countless hours coding your chatbot, the next step is to figure out how to put it out there for users to interact with. Deployment options are key because they determine how accessible your chatbot will be. Choosing the correct deployment path can affect both your bot's performance and user experience. It's not just about making it available; it's about making sure it runs smoothly and efficiently. Let’s dive into two primary avenues: cloud platforms and messaging app integrations.
Deploying on Cloud Platforms
AWS
Amazon Web Services (AWS) is a heavyweight in the cloud arena, and for good reason. Its robust infrastructure supports a range of applications, making it a go-to choice for developers looking to host chatbots. One key characteristic of AWS is its scalability. If your bot suddenly gets a surge in users, AWS can seamlessly handle the increase without a hitch, allowing for smooth operation.
However, AWS does come with its own complexities; setting it up may require a deeper understanding of cloud services compared to other platforms. Its unique feature is the Elastic Compute Cloud (EC2), which allows you to launch instances of virtual servers tailored to your needs. This flexibility is beneficial for developers looking to customize their setup but can be overwhelming for beginners.
Some advantages of using AWS for your chatbot include:
- Scalability: Easily adjusts to user demand.
- Robustness: Reliable performance even during peak usage.
- Diverse Services: Offers tools for monitoring and analytics.
On the flip side, the learning curve can be steep, which might pose challenges for those who are new to cloud technology.
Heroku
Heroku presents itself as a more user-friendly alternative for deploying chatbots. One of its standout characteristics is simplicity; you can get your bot up and running with just a few commands. This is particularly appealing to those who might find complex cloud configurations daunting.
Heroku’s unique feature rests in its extensive buildpack system, which allows developers to easily deploy apps written in various languages, including Python. This is a big plus if your chatbot uses multiple libraries or dependencies, as it simplifies the deployment process considerably.
The advantages of using Heroku include:
- Ease of Use: Exceptional for beginners or those wanting quick deployment.
- Free Tier: Offers a free plan to get started without financial commitment.
- Community: Strong support and resources available for troubleshooting.
Yet, its free tier comes with limitations in terms of performance and uptime, which could be a concern for chatbots expecting regular traffic.
Integration with Messaging Apps
Integrating your chatbot with popular messaging applications can vastly improve its reach. By making your chatbot accessible on platforms where users already interact, you can increase engagement and usability without requiring users to download another application.
Slack
Slack is a collaboration tool widely utilized in workplaces. The specific aspect of Slack that draws developers is its API, which allows for smooth integration of bots. One of Slack's defining characteristics is its flexibility in facilitating communication across teams, making it ideal for chatbots focused on internal business functions.
A unique advantage of Slack is the ability to create interactive components such as buttons and menus within conversations. However, it may not be the best fit for consumer-facing chatbots due to its primarily professional user base.
The advantages include:
- Integration Capabilities: Great for adding functional bots to team communication.
- User Engagement: Directly interacts with users in familiar settings.
But keep in mind that building a user-friendly bot on Slack can be complicated owing to the platform's targeted audience.
Facebook Messenger
On the other hand, Facebook Messenger is a giant among messaging apps, boasting millions of potential users. A significant aspect is its tight integration with Facebook's ecosystem, which enables chatbots to easily tap into the social media landscape. Its key characteristic is widespread use; practically everyone has some exposure to it, making user acquisition easier.
A unique feature of Facebook Messenger is its rich media support, allowing bots to send images, videos, and even interactive elements like carousels. This can enhance user experience significantly.
Advantages include:
- Large Audience: Massive potential reach due to Facebook’s user base.
- Multimedia Engagement: Ability to deliver richer interactions compared to plain-text chats.
Conversely, the sheer scale can make it challenging to stand out, and privacy concerns have become increasingly prominent among users.
In summary, whether you choose AWS or Heroku for hosting, and Slack or Facebook Messenger for integration, considering your specific needs and audience will heavily influence your chatbot’s success.
Future of Chatbots
The future of chatbots is a pivotal area to consider in the field of technology. As chatbots continue to evolve, their potential applications seem almost limitless. They are becoming more integrated into daily life, providing customer support, personal assistance, and even engaging in casual conversations. Understanding where this technology is headed can inform development strategies and allow developers to create chatbots that not only meet current demands but also anticipate future needs. This section discusses key trends and potential advancements in chatbot technology that could reshape how they interact with users.
Trends in AI and Chatbots
Artificial intelligence (AI) plays a central role in the evolution of chatbots. These tools are no longer limited to simple keyword responses but are now capable of understanding context, sentiment, and even nuances of language. Some prominent trends contributing to the rapid development of chatbots include:
- Machine Learning Integration: Chatbots are increasingly using machine learning algorithms to improve their responses over time. By analyzing user interactions, they can learn from mistakes and adapt, providing better service with each conversation.
- Voice-Activated Chatbots: The popularity of voice assistants like Apple's Siri and Amazon's Alexa has prompted the rise of voice-activated chatbots. These tools allow users to interact without typing, creating a more natural and fluid communication experience.
- Multilingual Capabilities: As globalization increases, chatbots are being developed with capabilities to communicate in multiple languages. This trend significantly broadens the user base and enhances accessibility.
- Personalization: Modern chatbots are leveraging user data to offer personalized experiences. By understanding past interactions and preferences, they can suggest products, provide customized support, and create a more engaging user interaction.
"As the landscape of communication continues to shift, chatbots are set to keep pace by becoming more intuitive and adaptable to user needs."
Potential Advancements
Looking ahead, several advancements in chatbot technology are on the horizon. These developments promise to enhance functionality and user experience further:
- Enhanced Natural Language Processing (NLP): With ongoing research in NLP, future chatbots will likely become better at understanding and generating human language. This could lead to more meaningful interactions that feel less robotic.
- Integration with Augmented and Virtual Reality (AR/VR): The infusion of AR and VR technologies into chatbot applications could open new avenues for interaction. Imagine a scenario where a user could interact with a chatbot in a 3D environment, greatly enriching the experience.
- Behavioral Predictability: Future chatbots may utilize complex algorithms to predict user behavior more accurately. This could mean proactively providing assistance before a user even requests it, streamlining user experience significantly.
- Emotional Intelligence: The ability of chatbots to gauge and respond to user emotions could transform customer service. By detecting frustration or confusion, a chatbot could escalate the conversation to a human agent more effectively or adjust its responses accordingly.
Understanding these trends and potential advancements is crucial for developers. It shapes how they think about and design their chatbots today. Keeping an eye on the future enables the creation of more robust, functional, and user-centric chatbot systems.
The End
In wrapping up our exploration of building chatbots with Python, it's essential to underline how significant this subject is, especially in today's tech-driven environment. Chatbots are not just a passing trend; they are increasingly becoming an integral part of customer service, user interaction, and various other sectors. They offer businesses a streamlined way to communicate, ensuring responses are quick and efficient, thereby enhancing user experience.
Given the burgeoning role of chatbots, knowing how to create them using Python provides several advantages. This programming language presents a gentle learning curve, combined with a robust suite of libraries and frameworks. Developers can leverage these tools to create highly interactive and capable chatbots tailored to their needs.
Recap of Key Points
To sum up the pivotal elements discussed throughout this guide:
- Python's Popularity: The language's simplicity and extensive community support make it an ideal choice for chatbot development.
- Natural Language Processing Tools: Libraries like NLTK and spaCy allow for effective language understanding capabilities in chatbots.
- Framework Variety: Different frameworks such as Rasa, ChatterBot, and Flask offer flexibility, allowing developers to choose based on their project's requirements.
- Testing and Deployment: Robust testing ensures that the chatbot performs as expected, while various deployment platforms open up numerous possibilities for live interactions.
- Future Trends: Understanding current trends in AI can help developers stay ahead of the curve, making their chatbots more intuitive and user-friendly.
Encouragement for Further Exploration
Lastly, it's imperative to encourage you to delve deeper into chatbot development. The field is evolving rapidly, with new technologies and methods emerging continually. Engaging with online communities, such as those found on Reddit, may also prove invaluable. These platforms allow developers and enthusiasts to share insights, troubleshoot problems, and discover innovative approaches to improving chatbot capabilities.
Consider experimenting with different frameworks and libraries mentioned earlier. In addition, examining case studies of successful chatbots can provide practical examples of what works well in various contexts. As you advance your skills, remember that every exploration will enhance your insight and ability to create chatbots that not only meet user needs but also push the boundaries of what automation can accomplish.
"The journey of a thousand coding projects begins with a single line of code."
So grab your keyboard, venture into this exciting realm, and remember that the sky's the limit when it comes to possibilities in chatbot development with Python.